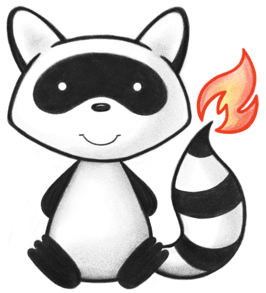
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Record of transport. 052 */ 053@ResourceDef(name="Transport", profile="http://hl7.org/fhir/StructureDefinition/Transport") 054public class Transport extends DomainResource { 055 056 public enum TransportIntent { 057 /** 058 * The intent is not known. When dealing with Transport, it's not always known (or relevant) how the transport was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously. 059 */ 060 UNKNOWN, 061 /** 062 * The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act. 063 */ 064 PROPOSAL, 065 /** 066 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 067 */ 068 PLAN, 069 /** 070 * The request represents a request/demand and authorization for action by the requestor. 071 */ 072 ORDER, 073 /** 074 * The request represents an original authorization for action. 075 */ 076 ORIGINALORDER, 077 /** 078 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization. 079 */ 080 REFLEXORDER, 081 /** 082 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 083 */ 084 FILLERORDER, 085 /** 086 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 087 */ 088 INSTANCEORDER, 089 /** 090 * The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used. 091 */ 092 OPTION, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 public static TransportIntent fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("unknown".equals(codeString)) 101 return UNKNOWN; 102 if ("proposal".equals(codeString)) 103 return PROPOSAL; 104 if ("plan".equals(codeString)) 105 return PLAN; 106 if ("order".equals(codeString)) 107 return ORDER; 108 if ("original-order".equals(codeString)) 109 return ORIGINALORDER; 110 if ("reflex-order".equals(codeString)) 111 return REFLEXORDER; 112 if ("filler-order".equals(codeString)) 113 return FILLERORDER; 114 if ("instance-order".equals(codeString)) 115 return INSTANCEORDER; 116 if ("option".equals(codeString)) 117 return OPTION; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown TransportIntent code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case UNKNOWN: return "unknown"; 126 case PROPOSAL: return "proposal"; 127 case PLAN: return "plan"; 128 case ORDER: return "order"; 129 case ORIGINALORDER: return "original-order"; 130 case REFLEXORDER: return "reflex-order"; 131 case FILLERORDER: return "filler-order"; 132 case INSTANCEORDER: return "instance-order"; 133 case OPTION: return "option"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getSystem() { 139 switch (this) { 140 case UNKNOWN: return "http://hl7.org/fhir/transport-intent"; 141 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 142 case PLAN: return "http://hl7.org/fhir/request-intent"; 143 case ORDER: return "http://hl7.org/fhir/request-intent"; 144 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 145 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 146 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 147 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 148 case OPTION: return "http://hl7.org/fhir/request-intent"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDefinition() { 154 switch (this) { 155 case UNKNOWN: return "The intent is not known. When dealing with Transport, it's not always known (or relevant) how the transport was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously."; 156 case PROPOSAL: return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 157 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 158 case ORDER: return "The request represents a request/demand and authorization for action by the requestor."; 159 case ORIGINALORDER: return "The request represents an original authorization for action."; 160 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 161 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 162 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 163 case OPTION: return "The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used."; 164 case NULL: return null; 165 default: return "?"; 166 } 167 } 168 public String getDisplay() { 169 switch (this) { 170 case UNKNOWN: return "Unknown"; 171 case PROPOSAL: return "Proposal"; 172 case PLAN: return "Plan"; 173 case ORDER: return "Order"; 174 case ORIGINALORDER: return "Original Order"; 175 case REFLEXORDER: return "Reflex Order"; 176 case FILLERORDER: return "Filler Order"; 177 case INSTANCEORDER: return "Instance Order"; 178 case OPTION: return "Option"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 } 184 185 public static class TransportIntentEnumFactory implements EnumFactory<TransportIntent> { 186 public TransportIntent fromCode(String codeString) throws IllegalArgumentException { 187 if (codeString == null || "".equals(codeString)) 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("unknown".equals(codeString)) 191 return TransportIntent.UNKNOWN; 192 if ("proposal".equals(codeString)) 193 return TransportIntent.PROPOSAL; 194 if ("plan".equals(codeString)) 195 return TransportIntent.PLAN; 196 if ("order".equals(codeString)) 197 return TransportIntent.ORDER; 198 if ("original-order".equals(codeString)) 199 return TransportIntent.ORIGINALORDER; 200 if ("reflex-order".equals(codeString)) 201 return TransportIntent.REFLEXORDER; 202 if ("filler-order".equals(codeString)) 203 return TransportIntent.FILLERORDER; 204 if ("instance-order".equals(codeString)) 205 return TransportIntent.INSTANCEORDER; 206 if ("option".equals(codeString)) 207 return TransportIntent.OPTION; 208 throw new IllegalArgumentException("Unknown TransportIntent code '"+codeString+"'"); 209 } 210 public Enumeration<TransportIntent> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<TransportIntent>(this, TransportIntent.NULL, code); 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<TransportIntent>(this, TransportIntent.NULL, code); 218 if ("unknown".equals(codeString)) 219 return new Enumeration<TransportIntent>(this, TransportIntent.UNKNOWN, code); 220 if ("proposal".equals(codeString)) 221 return new Enumeration<TransportIntent>(this, TransportIntent.PROPOSAL, code); 222 if ("plan".equals(codeString)) 223 return new Enumeration<TransportIntent>(this, TransportIntent.PLAN, code); 224 if ("order".equals(codeString)) 225 return new Enumeration<TransportIntent>(this, TransportIntent.ORDER, code); 226 if ("original-order".equals(codeString)) 227 return new Enumeration<TransportIntent>(this, TransportIntent.ORIGINALORDER, code); 228 if ("reflex-order".equals(codeString)) 229 return new Enumeration<TransportIntent>(this, TransportIntent.REFLEXORDER, code); 230 if ("filler-order".equals(codeString)) 231 return new Enumeration<TransportIntent>(this, TransportIntent.FILLERORDER, code); 232 if ("instance-order".equals(codeString)) 233 return new Enumeration<TransportIntent>(this, TransportIntent.INSTANCEORDER, code); 234 if ("option".equals(codeString)) 235 return new Enumeration<TransportIntent>(this, TransportIntent.OPTION, code); 236 throw new FHIRException("Unknown TransportIntent code '"+codeString+"'"); 237 } 238 public String toCode(TransportIntent code) { 239 if (code == TransportIntent.NULL) 240 return null; 241 if (code == TransportIntent.UNKNOWN) 242 return "unknown"; 243 if (code == TransportIntent.PROPOSAL) 244 return "proposal"; 245 if (code == TransportIntent.PLAN) 246 return "plan"; 247 if (code == TransportIntent.ORDER) 248 return "order"; 249 if (code == TransportIntent.ORIGINALORDER) 250 return "original-order"; 251 if (code == TransportIntent.REFLEXORDER) 252 return "reflex-order"; 253 if (code == TransportIntent.FILLERORDER) 254 return "filler-order"; 255 if (code == TransportIntent.INSTANCEORDER) 256 return "instance-order"; 257 if (code == TransportIntent.OPTION) 258 return "option"; 259 return "?"; 260 } 261 public String toSystem(TransportIntent code) { 262 return code.getSystem(); 263 } 264 } 265 266 public enum TransportStatus { 267 /** 268 * Transport has started but not completed. 269 */ 270 INPROGRESS, 271 /** 272 * Transport has been completed. 273 */ 274 COMPLETED, 275 /** 276 * Transport was started but not completed. 277 */ 278 ABANDONED, 279 /** 280 * Transport was cancelled before started. 281 */ 282 CANCELLED, 283 /** 284 * Planned transport that is not yet requested. 285 */ 286 PLANNED, 287 /** 288 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".). 289 */ 290 ENTEREDINERROR, 291 /** 292 * added to help the parsers with the generic types 293 */ 294 NULL; 295 public static TransportStatus fromCode(String codeString) throws FHIRException { 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("in-progress".equals(codeString)) 299 return INPROGRESS; 300 if ("completed".equals(codeString)) 301 return COMPLETED; 302 if ("abandoned".equals(codeString)) 303 return ABANDONED; 304 if ("cancelled".equals(codeString)) 305 return CANCELLED; 306 if ("planned".equals(codeString)) 307 return PLANNED; 308 if ("entered-in-error".equals(codeString)) 309 return ENTEREDINERROR; 310 if (Configuration.isAcceptInvalidEnums()) 311 return null; 312 else 313 throw new FHIRException("Unknown TransportStatus code '"+codeString+"'"); 314 } 315 public String toCode() { 316 switch (this) { 317 case INPROGRESS: return "in-progress"; 318 case COMPLETED: return "completed"; 319 case ABANDONED: return "abandoned"; 320 case CANCELLED: return "cancelled"; 321 case PLANNED: return "planned"; 322 case ENTEREDINERROR: return "entered-in-error"; 323 case NULL: return null; 324 default: return "?"; 325 } 326 } 327 public String getSystem() { 328 switch (this) { 329 case INPROGRESS: return "http://hl7.org/fhir/transport-status"; 330 case COMPLETED: return "http://hl7.org/fhir/transport-status"; 331 case ABANDONED: return "http://hl7.org/fhir/transport-status"; 332 case CANCELLED: return "http://hl7.org/fhir/transport-status"; 333 case PLANNED: return "http://hl7.org/fhir/transport-status"; 334 case ENTEREDINERROR: return "http://hl7.org/fhir/transport-status"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getDefinition() { 340 switch (this) { 341 case INPROGRESS: return "Transport has started but not completed."; 342 case COMPLETED: return "Transport has been completed."; 343 case ABANDONED: return "Transport was started but not completed."; 344 case CANCELLED: return "Transport was cancelled before started."; 345 case PLANNED: return "Planned transport that is not yet requested."; 346 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".)."; 347 case NULL: return null; 348 default: return "?"; 349 } 350 } 351 public String getDisplay() { 352 switch (this) { 353 case INPROGRESS: return "In Progress"; 354 case COMPLETED: return "Completed"; 355 case ABANDONED: return "Abandoned"; 356 case CANCELLED: return "Cancelled"; 357 case PLANNED: return "Planned"; 358 case ENTEREDINERROR: return "Entered In Error"; 359 case NULL: return null; 360 default: return "?"; 361 } 362 } 363 } 364 365 public static class TransportStatusEnumFactory implements EnumFactory<TransportStatus> { 366 public TransportStatus fromCode(String codeString) throws IllegalArgumentException { 367 if (codeString == null || "".equals(codeString)) 368 if (codeString == null || "".equals(codeString)) 369 return null; 370 if ("in-progress".equals(codeString)) 371 return TransportStatus.INPROGRESS; 372 if ("completed".equals(codeString)) 373 return TransportStatus.COMPLETED; 374 if ("abandoned".equals(codeString)) 375 return TransportStatus.ABANDONED; 376 if ("cancelled".equals(codeString)) 377 return TransportStatus.CANCELLED; 378 if ("planned".equals(codeString)) 379 return TransportStatus.PLANNED; 380 if ("entered-in-error".equals(codeString)) 381 return TransportStatus.ENTEREDINERROR; 382 throw new IllegalArgumentException("Unknown TransportStatus code '"+codeString+"'"); 383 } 384 public Enumeration<TransportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 385 if (code == null) 386 return null; 387 if (code.isEmpty()) 388 return new Enumeration<TransportStatus>(this, TransportStatus.NULL, code); 389 String codeString = ((PrimitiveType) code).asStringValue(); 390 if (codeString == null || "".equals(codeString)) 391 return new Enumeration<TransportStatus>(this, TransportStatus.NULL, code); 392 if ("in-progress".equals(codeString)) 393 return new Enumeration<TransportStatus>(this, TransportStatus.INPROGRESS, code); 394 if ("completed".equals(codeString)) 395 return new Enumeration<TransportStatus>(this, TransportStatus.COMPLETED, code); 396 if ("abandoned".equals(codeString)) 397 return new Enumeration<TransportStatus>(this, TransportStatus.ABANDONED, code); 398 if ("cancelled".equals(codeString)) 399 return new Enumeration<TransportStatus>(this, TransportStatus.CANCELLED, code); 400 if ("planned".equals(codeString)) 401 return new Enumeration<TransportStatus>(this, TransportStatus.PLANNED, code); 402 if ("entered-in-error".equals(codeString)) 403 return new Enumeration<TransportStatus>(this, TransportStatus.ENTEREDINERROR, code); 404 throw new FHIRException("Unknown TransportStatus code '"+codeString+"'"); 405 } 406 public String toCode(TransportStatus code) { 407 if (code == TransportStatus.NULL) 408 return null; 409 if (code == TransportStatus.INPROGRESS) 410 return "in-progress"; 411 if (code == TransportStatus.COMPLETED) 412 return "completed"; 413 if (code == TransportStatus.ABANDONED) 414 return "abandoned"; 415 if (code == TransportStatus.CANCELLED) 416 return "cancelled"; 417 if (code == TransportStatus.PLANNED) 418 return "planned"; 419 if (code == TransportStatus.ENTEREDINERROR) 420 return "entered-in-error"; 421 return "?"; 422 } 423 public String toSystem(TransportStatus code) { 424 return code.getSystem(); 425 } 426 } 427 428 @Block() 429 public static class TransportRestrictionComponent extends BackboneElement implements IBaseBackboneElement { 430 /** 431 * Indicates the number of times the requested action should occur. 432 */ 433 @Child(name = "repetitions", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 434 @Description(shortDefinition="How many times to repeat", formalDefinition="Indicates the number of times the requested action should occur." ) 435 protected PositiveIntType repetitions; 436 437 /** 438 * Over what time-period is fulfillment sought. 439 */ 440 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 441 @Description(shortDefinition="When fulfillment sought", formalDefinition="Over what time-period is fulfillment sought." ) 442 protected Period period; 443 444 /** 445 * For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for. 446 */ 447 @Child(name = "recipient", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Group.class, Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 448 @Description(shortDefinition="For whom is fulfillment sought?", formalDefinition="For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for." ) 449 protected List<Reference> recipient; 450 451 private static final long serialVersionUID = 1673996066L; 452 453 /** 454 * Constructor 455 */ 456 public TransportRestrictionComponent() { 457 super(); 458 } 459 460 /** 461 * @return {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 462 */ 463 public PositiveIntType getRepetitionsElement() { 464 if (this.repetitions == null) 465 if (Configuration.errorOnAutoCreate()) 466 throw new Error("Attempt to auto-create TransportRestrictionComponent.repetitions"); 467 else if (Configuration.doAutoCreate()) 468 this.repetitions = new PositiveIntType(); // bb 469 return this.repetitions; 470 } 471 472 public boolean hasRepetitionsElement() { 473 return this.repetitions != null && !this.repetitions.isEmpty(); 474 } 475 476 public boolean hasRepetitions() { 477 return this.repetitions != null && !this.repetitions.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 482 */ 483 public TransportRestrictionComponent setRepetitionsElement(PositiveIntType value) { 484 this.repetitions = value; 485 return this; 486 } 487 488 /** 489 * @return Indicates the number of times the requested action should occur. 490 */ 491 public int getRepetitions() { 492 return this.repetitions == null || this.repetitions.isEmpty() ? 0 : this.repetitions.getValue(); 493 } 494 495 /** 496 * @param value Indicates the number of times the requested action should occur. 497 */ 498 public TransportRestrictionComponent setRepetitions(int value) { 499 if (this.repetitions == null) 500 this.repetitions = new PositiveIntType(); 501 this.repetitions.setValue(value); 502 return this; 503 } 504 505 /** 506 * @return {@link #period} (Over what time-period is fulfillment sought.) 507 */ 508 public Period getPeriod() { 509 if (this.period == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create TransportRestrictionComponent.period"); 512 else if (Configuration.doAutoCreate()) 513 this.period = new Period(); // cc 514 return this.period; 515 } 516 517 public boolean hasPeriod() { 518 return this.period != null && !this.period.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #period} (Over what time-period is fulfillment sought.) 523 */ 524 public TransportRestrictionComponent setPeriod(Period value) { 525 this.period = value; 526 return this; 527 } 528 529 /** 530 * @return {@link #recipient} (For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.) 531 */ 532 public List<Reference> getRecipient() { 533 if (this.recipient == null) 534 this.recipient = new ArrayList<Reference>(); 535 return this.recipient; 536 } 537 538 /** 539 * @return Returns a reference to <code>this</code> for easy method chaining 540 */ 541 public TransportRestrictionComponent setRecipient(List<Reference> theRecipient) { 542 this.recipient = theRecipient; 543 return this; 544 } 545 546 public boolean hasRecipient() { 547 if (this.recipient == null) 548 return false; 549 for (Reference item : this.recipient) 550 if (!item.isEmpty()) 551 return true; 552 return false; 553 } 554 555 public Reference addRecipient() { //3 556 Reference t = new Reference(); 557 if (this.recipient == null) 558 this.recipient = new ArrayList<Reference>(); 559 this.recipient.add(t); 560 return t; 561 } 562 563 public TransportRestrictionComponent addRecipient(Reference t) { //3 564 if (t == null) 565 return this; 566 if (this.recipient == null) 567 this.recipient = new ArrayList<Reference>(); 568 this.recipient.add(t); 569 return this; 570 } 571 572 /** 573 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist {3} 574 */ 575 public Reference getRecipientFirstRep() { 576 if (getRecipient().isEmpty()) { 577 addRecipient(); 578 } 579 return getRecipient().get(0); 580 } 581 582 protected void listChildren(List<Property> children) { 583 super.listChildren(children); 584 children.add(new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions)); 585 children.add(new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period)); 586 children.add(new Property("recipient", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", "For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.", 0, java.lang.Integer.MAX_VALUE, recipient)); 587 } 588 589 @Override 590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 591 switch (_hash) { 592 case 984367650: /*repetitions*/ return new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions); 593 case -991726143: /*period*/ return new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period); 594 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", "For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.", 0, java.lang.Integer.MAX_VALUE, recipient); 595 default: return super.getNamedProperty(_hash, _name, _checkValid); 596 } 597 598 } 599 600 @Override 601 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 602 switch (hash) { 603 case 984367650: /*repetitions*/ return this.repetitions == null ? new Base[0] : new Base[] {this.repetitions}; // PositiveIntType 604 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 605 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 606 default: return super.getProperty(hash, name, checkValid); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 613 switch (hash) { 614 case 984367650: // repetitions 615 this.repetitions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 616 return value; 617 case -991726143: // period 618 this.period = TypeConvertor.castToPeriod(value); // Period 619 return value; 620 case 820081177: // recipient 621 this.getRecipient().add(TypeConvertor.castToReference(value)); // Reference 622 return value; 623 default: return super.setProperty(hash, name, value); 624 } 625 626 } 627 628 @Override 629 public Base setProperty(String name, Base value) throws FHIRException { 630 if (name.equals("repetitions")) { 631 this.repetitions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 632 } else if (name.equals("period")) { 633 this.period = TypeConvertor.castToPeriod(value); // Period 634 } else if (name.equals("recipient")) { 635 this.getRecipient().add(TypeConvertor.castToReference(value)); 636 } else 637 return super.setProperty(name, value); 638 return value; 639 } 640 641 @Override 642 public void removeChild(String name, Base value) throws FHIRException { 643 if (name.equals("repetitions")) { 644 this.repetitions = null; 645 } else if (name.equals("period")) { 646 this.period = null; 647 } else if (name.equals("recipient")) { 648 this.getRecipient().remove(value); 649 } else 650 super.removeChild(name, value); 651 652 } 653 654 @Override 655 public Base makeProperty(int hash, String name) throws FHIRException { 656 switch (hash) { 657 case 984367650: return getRepetitionsElement(); 658 case -991726143: return getPeriod(); 659 case 820081177: return addRecipient(); 660 default: return super.makeProperty(hash, name); 661 } 662 663 } 664 665 @Override 666 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 667 switch (hash) { 668 case 984367650: /*repetitions*/ return new String[] {"positiveInt"}; 669 case -991726143: /*period*/ return new String[] {"Period"}; 670 case 820081177: /*recipient*/ return new String[] {"Reference"}; 671 default: return super.getTypesForProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public Base addChild(String name) throws FHIRException { 678 if (name.equals("repetitions")) { 679 throw new FHIRException("Cannot call addChild on a singleton property Transport.restriction.repetitions"); 680 } 681 else if (name.equals("period")) { 682 this.period = new Period(); 683 return this.period; 684 } 685 else if (name.equals("recipient")) { 686 return addRecipient(); 687 } 688 else 689 return super.addChild(name); 690 } 691 692 public TransportRestrictionComponent copy() { 693 TransportRestrictionComponent dst = new TransportRestrictionComponent(); 694 copyValues(dst); 695 return dst; 696 } 697 698 public void copyValues(TransportRestrictionComponent dst) { 699 super.copyValues(dst); 700 dst.repetitions = repetitions == null ? null : repetitions.copy(); 701 dst.period = period == null ? null : period.copy(); 702 if (recipient != null) { 703 dst.recipient = new ArrayList<Reference>(); 704 for (Reference i : recipient) 705 dst.recipient.add(i.copy()); 706 }; 707 } 708 709 @Override 710 public boolean equalsDeep(Base other_) { 711 if (!super.equalsDeep(other_)) 712 return false; 713 if (!(other_ instanceof TransportRestrictionComponent)) 714 return false; 715 TransportRestrictionComponent o = (TransportRestrictionComponent) other_; 716 return compareDeep(repetitions, o.repetitions, true) && compareDeep(period, o.period, true) && compareDeep(recipient, o.recipient, true) 717 ; 718 } 719 720 @Override 721 public boolean equalsShallow(Base other_) { 722 if (!super.equalsShallow(other_)) 723 return false; 724 if (!(other_ instanceof TransportRestrictionComponent)) 725 return false; 726 TransportRestrictionComponent o = (TransportRestrictionComponent) other_; 727 return compareValues(repetitions, o.repetitions, true); 728 } 729 730 public boolean isEmpty() { 731 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(repetitions, period, recipient 732 ); 733 } 734 735 public String fhirType() { 736 return "Transport.restriction"; 737 738 } 739 740 } 741 742 @Block() 743 public static class ParameterComponent extends BackboneElement implements IBaseBackboneElement { 744 /** 745 * A code or description indicating how the input is intended to be used as part of the transport execution. 746 */ 747 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 748 @Description(shortDefinition="Label for the input", formalDefinition="A code or description indicating how the input is intended to be used as part of the transport execution." ) 749 protected CodeableConcept type; 750 751 /** 752 * The value of the input parameter as a basic type. 753 */ 754 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=false) 755 @Description(shortDefinition="Content to use in performing the transport", formalDefinition="The value of the input parameter as a basic type." ) 756 protected DataType value; 757 758 private static final long serialVersionUID = -1659186716L; 759 760 /** 761 * Constructor 762 */ 763 public ParameterComponent() { 764 super(); 765 } 766 767 /** 768 * Constructor 769 */ 770 public ParameterComponent(CodeableConcept type, DataType value) { 771 super(); 772 this.setType(type); 773 this.setValue(value); 774 } 775 776 /** 777 * @return {@link #type} (A code or description indicating how the input is intended to be used as part of the transport execution.) 778 */ 779 public CodeableConcept getType() { 780 if (this.type == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create ParameterComponent.type"); 783 else if (Configuration.doAutoCreate()) 784 this.type = new CodeableConcept(); // cc 785 return this.type; 786 } 787 788 public boolean hasType() { 789 return this.type != null && !this.type.isEmpty(); 790 } 791 792 /** 793 * @param value {@link #type} (A code or description indicating how the input is intended to be used as part of the transport execution.) 794 */ 795 public ParameterComponent setType(CodeableConcept value) { 796 this.type = value; 797 return this; 798 } 799 800 /** 801 * @return {@link #value} (The value of the input parameter as a basic type.) 802 */ 803 public DataType getValue() { 804 return this.value; 805 } 806 807 /** 808 * @return {@link #value} (The value of the input parameter as a basic type.) 809 */ 810 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 811 if (this.value == null) 812 this.value = new Base64BinaryType(); 813 if (!(this.value instanceof Base64BinaryType)) 814 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 815 return (Base64BinaryType) this.value; 816 } 817 818 public boolean hasValueBase64BinaryType() { 819 return this != null && this.value instanceof Base64BinaryType; 820 } 821 822 /** 823 * @return {@link #value} (The value of the input parameter as a basic type.) 824 */ 825 public BooleanType getValueBooleanType() throws FHIRException { 826 if (this.value == null) 827 this.value = new BooleanType(); 828 if (!(this.value instanceof BooleanType)) 829 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 830 return (BooleanType) this.value; 831 } 832 833 public boolean hasValueBooleanType() { 834 return this != null && this.value instanceof BooleanType; 835 } 836 837 /** 838 * @return {@link #value} (The value of the input parameter as a basic type.) 839 */ 840 public CanonicalType getValueCanonicalType() throws FHIRException { 841 if (this.value == null) 842 this.value = new CanonicalType(); 843 if (!(this.value instanceof CanonicalType)) 844 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 845 return (CanonicalType) this.value; 846 } 847 848 public boolean hasValueCanonicalType() { 849 return this != null && this.value instanceof CanonicalType; 850 } 851 852 /** 853 * @return {@link #value} (The value of the input parameter as a basic type.) 854 */ 855 public CodeType getValueCodeType() throws FHIRException { 856 if (this.value == null) 857 this.value = new CodeType(); 858 if (!(this.value instanceof CodeType)) 859 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 860 return (CodeType) this.value; 861 } 862 863 public boolean hasValueCodeType() { 864 return this != null && this.value instanceof CodeType; 865 } 866 867 /** 868 * @return {@link #value} (The value of the input parameter as a basic type.) 869 */ 870 public DateType getValueDateType() throws FHIRException { 871 if (this.value == null) 872 this.value = new DateType(); 873 if (!(this.value instanceof DateType)) 874 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 875 return (DateType) this.value; 876 } 877 878 public boolean hasValueDateType() { 879 return this != null && this.value instanceof DateType; 880 } 881 882 /** 883 * @return {@link #value} (The value of the input parameter as a basic type.) 884 */ 885 public DateTimeType getValueDateTimeType() throws FHIRException { 886 if (this.value == null) 887 this.value = new DateTimeType(); 888 if (!(this.value instanceof DateTimeType)) 889 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 890 return (DateTimeType) this.value; 891 } 892 893 public boolean hasValueDateTimeType() { 894 return this != null && this.value instanceof DateTimeType; 895 } 896 897 /** 898 * @return {@link #value} (The value of the input parameter as a basic type.) 899 */ 900 public DecimalType getValueDecimalType() throws FHIRException { 901 if (this.value == null) 902 this.value = new DecimalType(); 903 if (!(this.value instanceof DecimalType)) 904 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 905 return (DecimalType) this.value; 906 } 907 908 public boolean hasValueDecimalType() { 909 return this != null && this.value instanceof DecimalType; 910 } 911 912 /** 913 * @return {@link #value} (The value of the input parameter as a basic type.) 914 */ 915 public IdType getValueIdType() throws FHIRException { 916 if (this.value == null) 917 this.value = new IdType(); 918 if (!(this.value instanceof IdType)) 919 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 920 return (IdType) this.value; 921 } 922 923 public boolean hasValueIdType() { 924 return this != null && this.value instanceof IdType; 925 } 926 927 /** 928 * @return {@link #value} (The value of the input parameter as a basic type.) 929 */ 930 public InstantType getValueInstantType() throws FHIRException { 931 if (this.value == null) 932 this.value = new InstantType(); 933 if (!(this.value instanceof InstantType)) 934 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 935 return (InstantType) this.value; 936 } 937 938 public boolean hasValueInstantType() { 939 return this != null && this.value instanceof InstantType; 940 } 941 942 /** 943 * @return {@link #value} (The value of the input parameter as a basic type.) 944 */ 945 public IntegerType getValueIntegerType() throws FHIRException { 946 if (this.value == null) 947 this.value = new IntegerType(); 948 if (!(this.value instanceof IntegerType)) 949 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 950 return (IntegerType) this.value; 951 } 952 953 public boolean hasValueIntegerType() { 954 return this != null && this.value instanceof IntegerType; 955 } 956 957 /** 958 * @return {@link #value} (The value of the input parameter as a basic type.) 959 */ 960 public Integer64Type getValueInteger64Type() throws FHIRException { 961 if (this.value == null) 962 this.value = new Integer64Type(); 963 if (!(this.value instanceof Integer64Type)) 964 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 965 return (Integer64Type) this.value; 966 } 967 968 public boolean hasValueInteger64Type() { 969 return this != null && this.value instanceof Integer64Type; 970 } 971 972 /** 973 * @return {@link #value} (The value of the input parameter as a basic type.) 974 */ 975 public MarkdownType getValueMarkdownType() throws FHIRException { 976 if (this.value == null) 977 this.value = new MarkdownType(); 978 if (!(this.value instanceof MarkdownType)) 979 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 980 return (MarkdownType) this.value; 981 } 982 983 public boolean hasValueMarkdownType() { 984 return this != null && this.value instanceof MarkdownType; 985 } 986 987 /** 988 * @return {@link #value} (The value of the input parameter as a basic type.) 989 */ 990 public OidType getValueOidType() throws FHIRException { 991 if (this.value == null) 992 this.value = new OidType(); 993 if (!(this.value instanceof OidType)) 994 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 995 return (OidType) this.value; 996 } 997 998 public boolean hasValueOidType() { 999 return this != null && this.value instanceof OidType; 1000 } 1001 1002 /** 1003 * @return {@link #value} (The value of the input parameter as a basic type.) 1004 */ 1005 public PositiveIntType getValuePositiveIntType() throws FHIRException { 1006 if (this.value == null) 1007 this.value = new PositiveIntType(); 1008 if (!(this.value instanceof PositiveIntType)) 1009 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 1010 return (PositiveIntType) this.value; 1011 } 1012 1013 public boolean hasValuePositiveIntType() { 1014 return this != null && this.value instanceof PositiveIntType; 1015 } 1016 1017 /** 1018 * @return {@link #value} (The value of the input parameter as a basic type.) 1019 */ 1020 public StringType getValueStringType() throws FHIRException { 1021 if (this.value == null) 1022 this.value = new StringType(); 1023 if (!(this.value instanceof StringType)) 1024 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1025 return (StringType) this.value; 1026 } 1027 1028 public boolean hasValueStringType() { 1029 return this != null && this.value instanceof StringType; 1030 } 1031 1032 /** 1033 * @return {@link #value} (The value of the input parameter as a basic type.) 1034 */ 1035 public TimeType getValueTimeType() throws FHIRException { 1036 if (this.value == null) 1037 this.value = new TimeType(); 1038 if (!(this.value instanceof TimeType)) 1039 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1040 return (TimeType) this.value; 1041 } 1042 1043 public boolean hasValueTimeType() { 1044 return this != null && this.value instanceof TimeType; 1045 } 1046 1047 /** 1048 * @return {@link #value} (The value of the input parameter as a basic type.) 1049 */ 1050 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 1051 if (this.value == null) 1052 this.value = new UnsignedIntType(); 1053 if (!(this.value instanceof UnsignedIntType)) 1054 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 1055 return (UnsignedIntType) this.value; 1056 } 1057 1058 public boolean hasValueUnsignedIntType() { 1059 return this != null && this.value instanceof UnsignedIntType; 1060 } 1061 1062 /** 1063 * @return {@link #value} (The value of the input parameter as a basic type.) 1064 */ 1065 public UriType getValueUriType() throws FHIRException { 1066 if (this.value == null) 1067 this.value = new UriType(); 1068 if (!(this.value instanceof UriType)) 1069 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 1070 return (UriType) this.value; 1071 } 1072 1073 public boolean hasValueUriType() { 1074 return this != null && this.value instanceof UriType; 1075 } 1076 1077 /** 1078 * @return {@link #value} (The value of the input parameter as a basic type.) 1079 */ 1080 public UrlType getValueUrlType() throws FHIRException { 1081 if (this.value == null) 1082 this.value = new UrlType(); 1083 if (!(this.value instanceof UrlType)) 1084 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 1085 return (UrlType) this.value; 1086 } 1087 1088 public boolean hasValueUrlType() { 1089 return this != null && this.value instanceof UrlType; 1090 } 1091 1092 /** 1093 * @return {@link #value} (The value of the input parameter as a basic type.) 1094 */ 1095 public UuidType getValueUuidType() throws FHIRException { 1096 if (this.value == null) 1097 this.value = new UuidType(); 1098 if (!(this.value instanceof UuidType)) 1099 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 1100 return (UuidType) this.value; 1101 } 1102 1103 public boolean hasValueUuidType() { 1104 return this != null && this.value instanceof UuidType; 1105 } 1106 1107 /** 1108 * @return {@link #value} (The value of the input parameter as a basic type.) 1109 */ 1110 public Address getValueAddress() throws FHIRException { 1111 if (this.value == null) 1112 this.value = new Address(); 1113 if (!(this.value instanceof Address)) 1114 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 1115 return (Address) this.value; 1116 } 1117 1118 public boolean hasValueAddress() { 1119 return this != null && this.value instanceof Address; 1120 } 1121 1122 /** 1123 * @return {@link #value} (The value of the input parameter as a basic type.) 1124 */ 1125 public Age getValueAge() throws FHIRException { 1126 if (this.value == null) 1127 this.value = new Age(); 1128 if (!(this.value instanceof Age)) 1129 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 1130 return (Age) this.value; 1131 } 1132 1133 public boolean hasValueAge() { 1134 return this != null && this.value instanceof Age; 1135 } 1136 1137 /** 1138 * @return {@link #value} (The value of the input parameter as a basic type.) 1139 */ 1140 public Annotation getValueAnnotation() throws FHIRException { 1141 if (this.value == null) 1142 this.value = new Annotation(); 1143 if (!(this.value instanceof Annotation)) 1144 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 1145 return (Annotation) this.value; 1146 } 1147 1148 public boolean hasValueAnnotation() { 1149 return this != null && this.value instanceof Annotation; 1150 } 1151 1152 /** 1153 * @return {@link #value} (The value of the input parameter as a basic type.) 1154 */ 1155 public Attachment getValueAttachment() throws FHIRException { 1156 if (this.value == null) 1157 this.value = new Attachment(); 1158 if (!(this.value instanceof Attachment)) 1159 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1160 return (Attachment) this.value; 1161 } 1162 1163 public boolean hasValueAttachment() { 1164 return this != null && this.value instanceof Attachment; 1165 } 1166 1167 /** 1168 * @return {@link #value} (The value of the input parameter as a basic type.) 1169 */ 1170 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1171 if (this.value == null) 1172 this.value = new CodeableConcept(); 1173 if (!(this.value instanceof CodeableConcept)) 1174 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1175 return (CodeableConcept) this.value; 1176 } 1177 1178 public boolean hasValueCodeableConcept() { 1179 return this != null && this.value instanceof CodeableConcept; 1180 } 1181 1182 /** 1183 * @return {@link #value} (The value of the input parameter as a basic type.) 1184 */ 1185 public CodeableReference getValueCodeableReference() throws FHIRException { 1186 if (this.value == null) 1187 this.value = new CodeableReference(); 1188 if (!(this.value instanceof CodeableReference)) 1189 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 1190 return (CodeableReference) this.value; 1191 } 1192 1193 public boolean hasValueCodeableReference() { 1194 return this != null && this.value instanceof CodeableReference; 1195 } 1196 1197 /** 1198 * @return {@link #value} (The value of the input parameter as a basic type.) 1199 */ 1200 public Coding getValueCoding() throws FHIRException { 1201 if (this.value == null) 1202 this.value = new Coding(); 1203 if (!(this.value instanceof Coding)) 1204 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 1205 return (Coding) this.value; 1206 } 1207 1208 public boolean hasValueCoding() { 1209 return this != null && this.value instanceof Coding; 1210 } 1211 1212 /** 1213 * @return {@link #value} (The value of the input parameter as a basic type.) 1214 */ 1215 public ContactPoint getValueContactPoint() throws FHIRException { 1216 if (this.value == null) 1217 this.value = new ContactPoint(); 1218 if (!(this.value instanceof ContactPoint)) 1219 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 1220 return (ContactPoint) this.value; 1221 } 1222 1223 public boolean hasValueContactPoint() { 1224 return this != null && this.value instanceof ContactPoint; 1225 } 1226 1227 /** 1228 * @return {@link #value} (The value of the input parameter as a basic type.) 1229 */ 1230 public Count getValueCount() throws FHIRException { 1231 if (this.value == null) 1232 this.value = new Count(); 1233 if (!(this.value instanceof Count)) 1234 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 1235 return (Count) this.value; 1236 } 1237 1238 public boolean hasValueCount() { 1239 return this != null && this.value instanceof Count; 1240 } 1241 1242 /** 1243 * @return {@link #value} (The value of the input parameter as a basic type.) 1244 */ 1245 public Distance getValueDistance() throws FHIRException { 1246 if (this.value == null) 1247 this.value = new Distance(); 1248 if (!(this.value instanceof Distance)) 1249 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 1250 return (Distance) this.value; 1251 } 1252 1253 public boolean hasValueDistance() { 1254 return this != null && this.value instanceof Distance; 1255 } 1256 1257 /** 1258 * @return {@link #value} (The value of the input parameter as a basic type.) 1259 */ 1260 public Duration getValueDuration() throws FHIRException { 1261 if (this.value == null) 1262 this.value = new Duration(); 1263 if (!(this.value instanceof Duration)) 1264 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 1265 return (Duration) this.value; 1266 } 1267 1268 public boolean hasValueDuration() { 1269 return this != null && this.value instanceof Duration; 1270 } 1271 1272 /** 1273 * @return {@link #value} (The value of the input parameter as a basic type.) 1274 */ 1275 public HumanName getValueHumanName() throws FHIRException { 1276 if (this.value == null) 1277 this.value = new HumanName(); 1278 if (!(this.value instanceof HumanName)) 1279 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 1280 return (HumanName) this.value; 1281 } 1282 1283 public boolean hasValueHumanName() { 1284 return this != null && this.value instanceof HumanName; 1285 } 1286 1287 /** 1288 * @return {@link #value} (The value of the input parameter as a basic type.) 1289 */ 1290 public Identifier getValueIdentifier() throws FHIRException { 1291 if (this.value == null) 1292 this.value = new Identifier(); 1293 if (!(this.value instanceof Identifier)) 1294 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1295 return (Identifier) this.value; 1296 } 1297 1298 public boolean hasValueIdentifier() { 1299 return this != null && this.value instanceof Identifier; 1300 } 1301 1302 /** 1303 * @return {@link #value} (The value of the input parameter as a basic type.) 1304 */ 1305 public Money getValueMoney() throws FHIRException { 1306 if (this.value == null) 1307 this.value = new Money(); 1308 if (!(this.value instanceof Money)) 1309 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 1310 return (Money) this.value; 1311 } 1312 1313 public boolean hasValueMoney() { 1314 return this != null && this.value instanceof Money; 1315 } 1316 1317 /** 1318 * @return {@link #value} (The value of the input parameter as a basic type.) 1319 */ 1320 public Period getValuePeriod() throws FHIRException { 1321 if (this.value == null) 1322 this.value = new Period(); 1323 if (!(this.value instanceof Period)) 1324 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1325 return (Period) this.value; 1326 } 1327 1328 public boolean hasValuePeriod() { 1329 return this != null && this.value instanceof Period; 1330 } 1331 1332 /** 1333 * @return {@link #value} (The value of the input parameter as a basic type.) 1334 */ 1335 public Quantity getValueQuantity() throws FHIRException { 1336 if (this.value == null) 1337 this.value = new Quantity(); 1338 if (!(this.value instanceof Quantity)) 1339 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1340 return (Quantity) this.value; 1341 } 1342 1343 public boolean hasValueQuantity() { 1344 return this != null && this.value instanceof Quantity; 1345 } 1346 1347 /** 1348 * @return {@link #value} (The value of the input parameter as a basic type.) 1349 */ 1350 public Range getValueRange() throws FHIRException { 1351 if (this.value == null) 1352 this.value = new Range(); 1353 if (!(this.value instanceof Range)) 1354 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1355 return (Range) this.value; 1356 } 1357 1358 public boolean hasValueRange() { 1359 return this != null && this.value instanceof Range; 1360 } 1361 1362 /** 1363 * @return {@link #value} (The value of the input parameter as a basic type.) 1364 */ 1365 public Ratio getValueRatio() throws FHIRException { 1366 if (this.value == null) 1367 this.value = new Ratio(); 1368 if (!(this.value instanceof Ratio)) 1369 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1370 return (Ratio) this.value; 1371 } 1372 1373 public boolean hasValueRatio() { 1374 return this != null && this.value instanceof Ratio; 1375 } 1376 1377 /** 1378 * @return {@link #value} (The value of the input parameter as a basic type.) 1379 */ 1380 public RatioRange getValueRatioRange() throws FHIRException { 1381 if (this.value == null) 1382 this.value = new RatioRange(); 1383 if (!(this.value instanceof RatioRange)) 1384 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 1385 return (RatioRange) this.value; 1386 } 1387 1388 public boolean hasValueRatioRange() { 1389 return this != null && this.value instanceof RatioRange; 1390 } 1391 1392 /** 1393 * @return {@link #value} (The value of the input parameter as a basic type.) 1394 */ 1395 public Reference getValueReference() throws FHIRException { 1396 if (this.value == null) 1397 this.value = new Reference(); 1398 if (!(this.value instanceof Reference)) 1399 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1400 return (Reference) this.value; 1401 } 1402 1403 public boolean hasValueReference() { 1404 return this != null && this.value instanceof Reference; 1405 } 1406 1407 /** 1408 * @return {@link #value} (The value of the input parameter as a basic type.) 1409 */ 1410 public SampledData getValueSampledData() throws FHIRException { 1411 if (this.value == null) 1412 this.value = new SampledData(); 1413 if (!(this.value instanceof SampledData)) 1414 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 1415 return (SampledData) this.value; 1416 } 1417 1418 public boolean hasValueSampledData() { 1419 return this != null && this.value instanceof SampledData; 1420 } 1421 1422 /** 1423 * @return {@link #value} (The value of the input parameter as a basic type.) 1424 */ 1425 public Signature getValueSignature() throws FHIRException { 1426 if (this.value == null) 1427 this.value = new Signature(); 1428 if (!(this.value instanceof Signature)) 1429 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 1430 return (Signature) this.value; 1431 } 1432 1433 public boolean hasValueSignature() { 1434 return this != null && this.value instanceof Signature; 1435 } 1436 1437 /** 1438 * @return {@link #value} (The value of the input parameter as a basic type.) 1439 */ 1440 public Timing getValueTiming() throws FHIRException { 1441 if (this.value == null) 1442 this.value = new Timing(); 1443 if (!(this.value instanceof Timing)) 1444 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 1445 return (Timing) this.value; 1446 } 1447 1448 public boolean hasValueTiming() { 1449 return this != null && this.value instanceof Timing; 1450 } 1451 1452 /** 1453 * @return {@link #value} (The value of the input parameter as a basic type.) 1454 */ 1455 public ContactDetail getValueContactDetail() throws FHIRException { 1456 if (this.value == null) 1457 this.value = new ContactDetail(); 1458 if (!(this.value instanceof ContactDetail)) 1459 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 1460 return (ContactDetail) this.value; 1461 } 1462 1463 public boolean hasValueContactDetail() { 1464 return this != null && this.value instanceof ContactDetail; 1465 } 1466 1467 /** 1468 * @return {@link #value} (The value of the input parameter as a basic type.) 1469 */ 1470 public DataRequirement getValueDataRequirement() throws FHIRException { 1471 if (this.value == null) 1472 this.value = new DataRequirement(); 1473 if (!(this.value instanceof DataRequirement)) 1474 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 1475 return (DataRequirement) this.value; 1476 } 1477 1478 public boolean hasValueDataRequirement() { 1479 return this != null && this.value instanceof DataRequirement; 1480 } 1481 1482 /** 1483 * @return {@link #value} (The value of the input parameter as a basic type.) 1484 */ 1485 public Expression getValueExpression() throws FHIRException { 1486 if (this.value == null) 1487 this.value = new Expression(); 1488 if (!(this.value instanceof Expression)) 1489 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 1490 return (Expression) this.value; 1491 } 1492 1493 public boolean hasValueExpression() { 1494 return this != null && this.value instanceof Expression; 1495 } 1496 1497 /** 1498 * @return {@link #value} (The value of the input parameter as a basic type.) 1499 */ 1500 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 1501 if (this.value == null) 1502 this.value = new ParameterDefinition(); 1503 if (!(this.value instanceof ParameterDefinition)) 1504 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 1505 return (ParameterDefinition) this.value; 1506 } 1507 1508 public boolean hasValueParameterDefinition() { 1509 return this != null && this.value instanceof ParameterDefinition; 1510 } 1511 1512 /** 1513 * @return {@link #value} (The value of the input parameter as a basic type.) 1514 */ 1515 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 1516 if (this.value == null) 1517 this.value = new RelatedArtifact(); 1518 if (!(this.value instanceof RelatedArtifact)) 1519 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 1520 return (RelatedArtifact) this.value; 1521 } 1522 1523 public boolean hasValueRelatedArtifact() { 1524 return this != null && this.value instanceof RelatedArtifact; 1525 } 1526 1527 /** 1528 * @return {@link #value} (The value of the input parameter as a basic type.) 1529 */ 1530 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 1531 if (this.value == null) 1532 this.value = new TriggerDefinition(); 1533 if (!(this.value instanceof TriggerDefinition)) 1534 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 1535 return (TriggerDefinition) this.value; 1536 } 1537 1538 public boolean hasValueTriggerDefinition() { 1539 return this != null && this.value instanceof TriggerDefinition; 1540 } 1541 1542 /** 1543 * @return {@link #value} (The value of the input parameter as a basic type.) 1544 */ 1545 public UsageContext getValueUsageContext() throws FHIRException { 1546 if (this.value == null) 1547 this.value = new UsageContext(); 1548 if (!(this.value instanceof UsageContext)) 1549 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 1550 return (UsageContext) this.value; 1551 } 1552 1553 public boolean hasValueUsageContext() { 1554 return this != null && this.value instanceof UsageContext; 1555 } 1556 1557 /** 1558 * @return {@link #value} (The value of the input parameter as a basic type.) 1559 */ 1560 public Availability getValueAvailability() throws FHIRException { 1561 if (this.value == null) 1562 this.value = new Availability(); 1563 if (!(this.value instanceof Availability)) 1564 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 1565 return (Availability) this.value; 1566 } 1567 1568 public boolean hasValueAvailability() { 1569 return this != null && this.value instanceof Availability; 1570 } 1571 1572 /** 1573 * @return {@link #value} (The value of the input parameter as a basic type.) 1574 */ 1575 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 1576 if (this.value == null) 1577 this.value = new ExtendedContactDetail(); 1578 if (!(this.value instanceof ExtendedContactDetail)) 1579 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 1580 return (ExtendedContactDetail) this.value; 1581 } 1582 1583 public boolean hasValueExtendedContactDetail() { 1584 return this != null && this.value instanceof ExtendedContactDetail; 1585 } 1586 1587 /** 1588 * @return {@link #value} (The value of the input parameter as a basic type.) 1589 */ 1590 public Dosage getValueDosage() throws FHIRException { 1591 if (this.value == null) 1592 this.value = new Dosage(); 1593 if (!(this.value instanceof Dosage)) 1594 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 1595 return (Dosage) this.value; 1596 } 1597 1598 public boolean hasValueDosage() { 1599 return this != null && this.value instanceof Dosage; 1600 } 1601 1602 /** 1603 * @return {@link #value} (The value of the input parameter as a basic type.) 1604 */ 1605 public Meta getValueMeta() throws FHIRException { 1606 if (this.value == null) 1607 this.value = new Meta(); 1608 if (!(this.value instanceof Meta)) 1609 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 1610 return (Meta) this.value; 1611 } 1612 1613 public boolean hasValueMeta() { 1614 return this != null && this.value instanceof Meta; 1615 } 1616 1617 public boolean hasValue() { 1618 return this.value != null && !this.value.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #value} (The value of the input parameter as a basic type.) 1623 */ 1624 public ParameterComponent setValue(DataType value) { 1625 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 1626 throw new FHIRException("Not the right type for Transport.input.value[x]: "+value.fhirType()); 1627 this.value = value; 1628 return this; 1629 } 1630 1631 protected void listChildren(List<Property> children) { 1632 super.listChildren(children); 1633 children.add(new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the transport execution.", 0, 1, type)); 1634 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value)); 1635 } 1636 1637 @Override 1638 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1639 switch (_hash) { 1640 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the transport execution.", 0, 1, type); 1641 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value); 1642 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value); 1643 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The value of the input parameter as a basic type.", 0, 1, value); 1644 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the input parameter as a basic type.", 0, 1, value); 1645 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The value of the input parameter as a basic type.", 0, 1, value); 1646 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of the input parameter as a basic type.", 0, 1, value); 1647 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The value of the input parameter as a basic type.", 0, 1, value); 1648 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the input parameter as a basic type.", 0, 1, value); 1649 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the input parameter as a basic type.", 0, 1, value); 1650 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The value of the input parameter as a basic type.", 0, 1, value); 1651 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The value of the input parameter as a basic type.", 0, 1, value); 1652 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the input parameter as a basic type.", 0, 1, value); 1653 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The value of the input parameter as a basic type.", 0, 1, value); 1654 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The value of the input parameter as a basic type.", 0, 1, value); 1655 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The value of the input parameter as a basic type.", 0, 1, value); 1656 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The value of the input parameter as a basic type.", 0, 1, value); 1657 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the input parameter as a basic type.", 0, 1, value); 1658 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The value of the input parameter as a basic type.", 0, 1, value); 1659 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The value of the input parameter as a basic type.", 0, 1, value); 1660 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the input parameter as a basic type.", 0, 1, value); 1661 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the input parameter as a basic type.", 0, 1, value); 1662 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The value of the input parameter as a basic type.", 0, 1, value); 1663 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the input parameter as a basic type.", 0, 1, value); 1664 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The value of the input parameter as a basic type.", 0, 1, value); 1665 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the input parameter as a basic type.", 0, 1, value); 1666 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the input parameter as a basic type.", 0, 1, value); 1667 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the input parameter as a basic type.", 0, 1, value); 1668 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The value of the input parameter as a basic type.", 0, 1, value); 1669 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of the input parameter as a basic type.", 0, 1, value); 1670 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The value of the input parameter as a basic type.", 0, 1, value); 1671 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The value of the input parameter as a basic type.", 0, 1, value); 1672 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The value of the input parameter as a basic type.", 0, 1, value); 1673 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the input parameter as a basic type.", 0, 1, value); 1674 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The value of the input parameter as a basic type.", 0, 1, value); 1675 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The value of the input parameter as a basic type.", 0, 1, value); 1676 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The value of the input parameter as a basic type.", 0, 1, value); 1677 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the input parameter as a basic type.", 0, 1, value); 1678 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the input parameter as a basic type.", 0, 1, value); 1679 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the input parameter as a basic type.", 0, 1, value); 1680 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the input parameter as a basic type.", 0, 1, value); 1681 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The value of the input parameter as a basic type.", 0, 1, value); 1682 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value of the input parameter as a basic type.", 0, 1, value); 1683 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The value of the input parameter as a basic type.", 0, 1, value); 1684 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The value of the input parameter as a basic type.", 0, 1, value); 1685 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The value of the input parameter as a basic type.", 0, 1, value); 1686 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The value of the input parameter as a basic type.", 0, 1, value); 1687 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The value of the input parameter as a basic type.", 0, 1, value); 1688 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The value of the input parameter as a basic type.", 0, 1, value); 1689 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The value of the input parameter as a basic type.", 0, 1, value); 1690 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The value of the input parameter as a basic type.", 0, 1, value); 1691 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The value of the input parameter as a basic type.", 0, 1, value); 1692 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The value of the input parameter as a basic type.", 0, 1, value); 1693 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The value of the input parameter as a basic type.", 0, 1, value); 1694 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The value of the input parameter as a basic type.", 0, 1, value); 1695 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The value of the input parameter as a basic type.", 0, 1, value); 1696 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The value of the input parameter as a basic type.", 0, 1, value); 1697 default: return super.getNamedProperty(_hash, _name, _checkValid); 1698 } 1699 1700 } 1701 1702 @Override 1703 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1704 switch (hash) { 1705 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1706 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1707 default: return super.getProperty(hash, name, checkValid); 1708 } 1709 1710 } 1711 1712 @Override 1713 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1714 switch (hash) { 1715 case 3575610: // type 1716 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1717 return value; 1718 case 111972721: // value 1719 this.value = TypeConvertor.castToType(value); // DataType 1720 return value; 1721 default: return super.setProperty(hash, name, value); 1722 } 1723 1724 } 1725 1726 @Override 1727 public Base setProperty(String name, Base value) throws FHIRException { 1728 if (name.equals("type")) { 1729 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1730 } else if (name.equals("value[x]")) { 1731 this.value = TypeConvertor.castToType(value); // DataType 1732 } else 1733 return super.setProperty(name, value); 1734 return value; 1735 } 1736 1737 @Override 1738 public void removeChild(String name, Base value) throws FHIRException { 1739 if (name.equals("type")) { 1740 this.type = null; 1741 } else if (name.equals("value[x]")) { 1742 this.value = null; 1743 } else 1744 super.removeChild(name, value); 1745 1746 } 1747 1748 @Override 1749 public Base makeProperty(int hash, String name) throws FHIRException { 1750 switch (hash) { 1751 case 3575610: return getType(); 1752 case -1410166417: return getValue(); 1753 case 111972721: return getValue(); 1754 default: return super.makeProperty(hash, name); 1755 } 1756 1757 } 1758 1759 @Override 1760 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1761 switch (hash) { 1762 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1763 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 1764 default: return super.getTypesForProperty(hash, name); 1765 } 1766 1767 } 1768 1769 @Override 1770 public Base addChild(String name) throws FHIRException { 1771 if (name.equals("type")) { 1772 this.type = new CodeableConcept(); 1773 return this.type; 1774 } 1775 else if (name.equals("valueBase64Binary")) { 1776 this.value = new Base64BinaryType(); 1777 return this.value; 1778 } 1779 else if (name.equals("valueBoolean")) { 1780 this.value = new BooleanType(); 1781 return this.value; 1782 } 1783 else if (name.equals("valueCanonical")) { 1784 this.value = new CanonicalType(); 1785 return this.value; 1786 } 1787 else if (name.equals("valueCode")) { 1788 this.value = new CodeType(); 1789 return this.value; 1790 } 1791 else if (name.equals("valueDate")) { 1792 this.value = new DateType(); 1793 return this.value; 1794 } 1795 else if (name.equals("valueDateTime")) { 1796 this.value = new DateTimeType(); 1797 return this.value; 1798 } 1799 else if (name.equals("valueDecimal")) { 1800 this.value = new DecimalType(); 1801 return this.value; 1802 } 1803 else if (name.equals("valueId")) { 1804 this.value = new IdType(); 1805 return this.value; 1806 } 1807 else if (name.equals("valueInstant")) { 1808 this.value = new InstantType(); 1809 return this.value; 1810 } 1811 else if (name.equals("valueInteger")) { 1812 this.value = new IntegerType(); 1813 return this.value; 1814 } 1815 else if (name.equals("valueInteger64")) { 1816 this.value = new Integer64Type(); 1817 return this.value; 1818 } 1819 else if (name.equals("valueMarkdown")) { 1820 this.value = new MarkdownType(); 1821 return this.value; 1822 } 1823 else if (name.equals("valueOid")) { 1824 this.value = new OidType(); 1825 return this.value; 1826 } 1827 else if (name.equals("valuePositiveInt")) { 1828 this.value = new PositiveIntType(); 1829 return this.value; 1830 } 1831 else if (name.equals("valueString")) { 1832 this.value = new StringType(); 1833 return this.value; 1834 } 1835 else if (name.equals("valueTime")) { 1836 this.value = new TimeType(); 1837 return this.value; 1838 } 1839 else if (name.equals("valueUnsignedInt")) { 1840 this.value = new UnsignedIntType(); 1841 return this.value; 1842 } 1843 else if (name.equals("valueUri")) { 1844 this.value = new UriType(); 1845 return this.value; 1846 } 1847 else if (name.equals("valueUrl")) { 1848 this.value = new UrlType(); 1849 return this.value; 1850 } 1851 else if (name.equals("valueUuid")) { 1852 this.value = new UuidType(); 1853 return this.value; 1854 } 1855 else if (name.equals("valueAddress")) { 1856 this.value = new Address(); 1857 return this.value; 1858 } 1859 else if (name.equals("valueAge")) { 1860 this.value = new Age(); 1861 return this.value; 1862 } 1863 else if (name.equals("valueAnnotation")) { 1864 this.value = new Annotation(); 1865 return this.value; 1866 } 1867 else if (name.equals("valueAttachment")) { 1868 this.value = new Attachment(); 1869 return this.value; 1870 } 1871 else if (name.equals("valueCodeableConcept")) { 1872 this.value = new CodeableConcept(); 1873 return this.value; 1874 } 1875 else if (name.equals("valueCodeableReference")) { 1876 this.value = new CodeableReference(); 1877 return this.value; 1878 } 1879 else if (name.equals("valueCoding")) { 1880 this.value = new Coding(); 1881 return this.value; 1882 } 1883 else if (name.equals("valueContactPoint")) { 1884 this.value = new ContactPoint(); 1885 return this.value; 1886 } 1887 else if (name.equals("valueCount")) { 1888 this.value = new Count(); 1889 return this.value; 1890 } 1891 else if (name.equals("valueDistance")) { 1892 this.value = new Distance(); 1893 return this.value; 1894 } 1895 else if (name.equals("valueDuration")) { 1896 this.value = new Duration(); 1897 return this.value; 1898 } 1899 else if (name.equals("valueHumanName")) { 1900 this.value = new HumanName(); 1901 return this.value; 1902 } 1903 else if (name.equals("valueIdentifier")) { 1904 this.value = new Identifier(); 1905 return this.value; 1906 } 1907 else if (name.equals("valueMoney")) { 1908 this.value = new Money(); 1909 return this.value; 1910 } 1911 else if (name.equals("valuePeriod")) { 1912 this.value = new Period(); 1913 return this.value; 1914 } 1915 else if (name.equals("valueQuantity")) { 1916 this.value = new Quantity(); 1917 return this.value; 1918 } 1919 else if (name.equals("valueRange")) { 1920 this.value = new Range(); 1921 return this.value; 1922 } 1923 else if (name.equals("valueRatio")) { 1924 this.value = new Ratio(); 1925 return this.value; 1926 } 1927 else if (name.equals("valueRatioRange")) { 1928 this.value = new RatioRange(); 1929 return this.value; 1930 } 1931 else if (name.equals("valueReference")) { 1932 this.value = new Reference(); 1933 return this.value; 1934 } 1935 else if (name.equals("valueSampledData")) { 1936 this.value = new SampledData(); 1937 return this.value; 1938 } 1939 else if (name.equals("valueSignature")) { 1940 this.value = new Signature(); 1941 return this.value; 1942 } 1943 else if (name.equals("valueTiming")) { 1944 this.value = new Timing(); 1945 return this.value; 1946 } 1947 else if (name.equals("valueContactDetail")) { 1948 this.value = new ContactDetail(); 1949 return this.value; 1950 } 1951 else if (name.equals("valueDataRequirement")) { 1952 this.value = new DataRequirement(); 1953 return this.value; 1954 } 1955 else if (name.equals("valueExpression")) { 1956 this.value = new Expression(); 1957 return this.value; 1958 } 1959 else if (name.equals("valueParameterDefinition")) { 1960 this.value = new ParameterDefinition(); 1961 return this.value; 1962 } 1963 else if (name.equals("valueRelatedArtifact")) { 1964 this.value = new RelatedArtifact(); 1965 return this.value; 1966 } 1967 else if (name.equals("valueTriggerDefinition")) { 1968 this.value = new TriggerDefinition(); 1969 return this.value; 1970 } 1971 else if (name.equals("valueUsageContext")) { 1972 this.value = new UsageContext(); 1973 return this.value; 1974 } 1975 else if (name.equals("valueAvailability")) { 1976 this.value = new Availability(); 1977 return this.value; 1978 } 1979 else if (name.equals("valueExtendedContactDetail")) { 1980 this.value = new ExtendedContactDetail(); 1981 return this.value; 1982 } 1983 else if (name.equals("valueDosage")) { 1984 this.value = new Dosage(); 1985 return this.value; 1986 } 1987 else if (name.equals("valueMeta")) { 1988 this.value = new Meta(); 1989 return this.value; 1990 } 1991 else 1992 return super.addChild(name); 1993 } 1994 1995 public ParameterComponent copy() { 1996 ParameterComponent dst = new ParameterComponent(); 1997 copyValues(dst); 1998 return dst; 1999 } 2000 2001 public void copyValues(ParameterComponent dst) { 2002 super.copyValues(dst); 2003 dst.type = type == null ? null : type.copy(); 2004 dst.value = value == null ? null : value.copy(); 2005 } 2006 2007 @Override 2008 public boolean equalsDeep(Base other_) { 2009 if (!super.equalsDeep(other_)) 2010 return false; 2011 if (!(other_ instanceof ParameterComponent)) 2012 return false; 2013 ParameterComponent o = (ParameterComponent) other_; 2014 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2015 } 2016 2017 @Override 2018 public boolean equalsShallow(Base other_) { 2019 if (!super.equalsShallow(other_)) 2020 return false; 2021 if (!(other_ instanceof ParameterComponent)) 2022 return false; 2023 ParameterComponent o = (ParameterComponent) other_; 2024 return true; 2025 } 2026 2027 public boolean isEmpty() { 2028 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2029 } 2030 2031 public String fhirType() { 2032 return "Transport.input"; 2033 2034 } 2035 2036 } 2037 2038 @Block() 2039 public static class TransportOutputComponent extends BackboneElement implements IBaseBackboneElement { 2040 /** 2041 * The name of the Output parameter. 2042 */ 2043 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2044 @Description(shortDefinition="Label for output", formalDefinition="The name of the Output parameter." ) 2045 protected CodeableConcept type; 2046 2047 /** 2048 * The value of the Output parameter as a basic type. 2049 */ 2050 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=false) 2051 @Description(shortDefinition="Result of output", formalDefinition="The value of the Output parameter as a basic type." ) 2052 protected DataType value; 2053 2054 private static final long serialVersionUID = -1659186716L; 2055 2056 /** 2057 * Constructor 2058 */ 2059 public TransportOutputComponent() { 2060 super(); 2061 } 2062 2063 /** 2064 * Constructor 2065 */ 2066 public TransportOutputComponent(CodeableConcept type, DataType value) { 2067 super(); 2068 this.setType(type); 2069 this.setValue(value); 2070 } 2071 2072 /** 2073 * @return {@link #type} (The name of the Output parameter.) 2074 */ 2075 public CodeableConcept getType() { 2076 if (this.type == null) 2077 if (Configuration.errorOnAutoCreate()) 2078 throw new Error("Attempt to auto-create TransportOutputComponent.type"); 2079 else if (Configuration.doAutoCreate()) 2080 this.type = new CodeableConcept(); // cc 2081 return this.type; 2082 } 2083 2084 public boolean hasType() { 2085 return this.type != null && !this.type.isEmpty(); 2086 } 2087 2088 /** 2089 * @param value {@link #type} (The name of the Output parameter.) 2090 */ 2091 public TransportOutputComponent setType(CodeableConcept value) { 2092 this.type = value; 2093 return this; 2094 } 2095 2096 /** 2097 * @return {@link #value} (The value of the Output parameter as a basic type.) 2098 */ 2099 public DataType getValue() { 2100 return this.value; 2101 } 2102 2103 /** 2104 * @return {@link #value} (The value of the Output parameter as a basic type.) 2105 */ 2106 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 2107 if (this.value == null) 2108 this.value = new Base64BinaryType(); 2109 if (!(this.value instanceof Base64BinaryType)) 2110 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 2111 return (Base64BinaryType) this.value; 2112 } 2113 2114 public boolean hasValueBase64BinaryType() { 2115 return this != null && this.value instanceof Base64BinaryType; 2116 } 2117 2118 /** 2119 * @return {@link #value} (The value of the Output parameter as a basic type.) 2120 */ 2121 public BooleanType getValueBooleanType() throws FHIRException { 2122 if (this.value == null) 2123 this.value = new BooleanType(); 2124 if (!(this.value instanceof BooleanType)) 2125 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2126 return (BooleanType) this.value; 2127 } 2128 2129 public boolean hasValueBooleanType() { 2130 return this != null && this.value instanceof BooleanType; 2131 } 2132 2133 /** 2134 * @return {@link #value} (The value of the Output parameter as a basic type.) 2135 */ 2136 public CanonicalType getValueCanonicalType() throws FHIRException { 2137 if (this.value == null) 2138 this.value = new CanonicalType(); 2139 if (!(this.value instanceof CanonicalType)) 2140 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2141 return (CanonicalType) this.value; 2142 } 2143 2144 public boolean hasValueCanonicalType() { 2145 return this != null && this.value instanceof CanonicalType; 2146 } 2147 2148 /** 2149 * @return {@link #value} (The value of the Output parameter as a basic type.) 2150 */ 2151 public CodeType getValueCodeType() throws FHIRException { 2152 if (this.value == null) 2153 this.value = new CodeType(); 2154 if (!(this.value instanceof CodeType)) 2155 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2156 return (CodeType) this.value; 2157 } 2158 2159 public boolean hasValueCodeType() { 2160 return this != null && this.value instanceof CodeType; 2161 } 2162 2163 /** 2164 * @return {@link #value} (The value of the Output parameter as a basic type.) 2165 */ 2166 public DateType getValueDateType() throws FHIRException { 2167 if (this.value == null) 2168 this.value = new DateType(); 2169 if (!(this.value instanceof DateType)) 2170 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2171 return (DateType) this.value; 2172 } 2173 2174 public boolean hasValueDateType() { 2175 return this != null && this.value instanceof DateType; 2176 } 2177 2178 /** 2179 * @return {@link #value} (The value of the Output parameter as a basic type.) 2180 */ 2181 public DateTimeType getValueDateTimeType() throws FHIRException { 2182 if (this.value == null) 2183 this.value = new DateTimeType(); 2184 if (!(this.value instanceof DateTimeType)) 2185 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2186 return (DateTimeType) this.value; 2187 } 2188 2189 public boolean hasValueDateTimeType() { 2190 return this != null && this.value instanceof DateTimeType; 2191 } 2192 2193 /** 2194 * @return {@link #value} (The value of the Output parameter as a basic type.) 2195 */ 2196 public DecimalType getValueDecimalType() throws FHIRException { 2197 if (this.value == null) 2198 this.value = new DecimalType(); 2199 if (!(this.value instanceof DecimalType)) 2200 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2201 return (DecimalType) this.value; 2202 } 2203 2204 public boolean hasValueDecimalType() { 2205 return this != null && this.value instanceof DecimalType; 2206 } 2207 2208 /** 2209 * @return {@link #value} (The value of the Output parameter as a basic type.) 2210 */ 2211 public IdType getValueIdType() throws FHIRException { 2212 if (this.value == null) 2213 this.value = new IdType(); 2214 if (!(this.value instanceof IdType)) 2215 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 2216 return (IdType) this.value; 2217 } 2218 2219 public boolean hasValueIdType() { 2220 return this != null && this.value instanceof IdType; 2221 } 2222 2223 /** 2224 * @return {@link #value} (The value of the Output parameter as a basic type.) 2225 */ 2226 public InstantType getValueInstantType() throws FHIRException { 2227 if (this.value == null) 2228 this.value = new InstantType(); 2229 if (!(this.value instanceof InstantType)) 2230 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 2231 return (InstantType) this.value; 2232 } 2233 2234 public boolean hasValueInstantType() { 2235 return this != null && this.value instanceof InstantType; 2236 } 2237 2238 /** 2239 * @return {@link #value} (The value of the Output parameter as a basic type.) 2240 */ 2241 public IntegerType getValueIntegerType() throws FHIRException { 2242 if (this.value == null) 2243 this.value = new IntegerType(); 2244 if (!(this.value instanceof IntegerType)) 2245 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2246 return (IntegerType) this.value; 2247 } 2248 2249 public boolean hasValueIntegerType() { 2250 return this != null && this.value instanceof IntegerType; 2251 } 2252 2253 /** 2254 * @return {@link #value} (The value of the Output parameter as a basic type.) 2255 */ 2256 public Integer64Type getValueInteger64Type() throws FHIRException { 2257 if (this.value == null) 2258 this.value = new Integer64Type(); 2259 if (!(this.value instanceof Integer64Type)) 2260 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 2261 return (Integer64Type) this.value; 2262 } 2263 2264 public boolean hasValueInteger64Type() { 2265 return this != null && this.value instanceof Integer64Type; 2266 } 2267 2268 /** 2269 * @return {@link #value} (The value of the Output parameter as a basic type.) 2270 */ 2271 public MarkdownType getValueMarkdownType() throws FHIRException { 2272 if (this.value == null) 2273 this.value = new MarkdownType(); 2274 if (!(this.value instanceof MarkdownType)) 2275 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 2276 return (MarkdownType) this.value; 2277 } 2278 2279 public boolean hasValueMarkdownType() { 2280 return this != null && this.value instanceof MarkdownType; 2281 } 2282 2283 /** 2284 * @return {@link #value} (The value of the Output parameter as a basic type.) 2285 */ 2286 public OidType getValueOidType() throws FHIRException { 2287 if (this.value == null) 2288 this.value = new OidType(); 2289 if (!(this.value instanceof OidType)) 2290 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2291 return (OidType) this.value; 2292 } 2293 2294 public boolean hasValueOidType() { 2295 return this != null && this.value instanceof OidType; 2296 } 2297 2298 /** 2299 * @return {@link #value} (The value of the Output parameter as a basic type.) 2300 */ 2301 public PositiveIntType getValuePositiveIntType() throws FHIRException { 2302 if (this.value == null) 2303 this.value = new PositiveIntType(); 2304 if (!(this.value instanceof PositiveIntType)) 2305 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 2306 return (PositiveIntType) this.value; 2307 } 2308 2309 public boolean hasValuePositiveIntType() { 2310 return this != null && this.value instanceof PositiveIntType; 2311 } 2312 2313 /** 2314 * @return {@link #value} (The value of the Output parameter as a basic type.) 2315 */ 2316 public StringType getValueStringType() throws FHIRException { 2317 if (this.value == null) 2318 this.value = new StringType(); 2319 if (!(this.value instanceof StringType)) 2320 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2321 return (StringType) this.value; 2322 } 2323 2324 public boolean hasValueStringType() { 2325 return this != null && this.value instanceof StringType; 2326 } 2327 2328 /** 2329 * @return {@link #value} (The value of the Output parameter as a basic type.) 2330 */ 2331 public TimeType getValueTimeType() throws FHIRException { 2332 if (this.value == null) 2333 this.value = new TimeType(); 2334 if (!(this.value instanceof TimeType)) 2335 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2336 return (TimeType) this.value; 2337 } 2338 2339 public boolean hasValueTimeType() { 2340 return this != null && this.value instanceof TimeType; 2341 } 2342 2343 /** 2344 * @return {@link #value} (The value of the Output parameter as a basic type.) 2345 */ 2346 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 2347 if (this.value == null) 2348 this.value = new UnsignedIntType(); 2349 if (!(this.value instanceof UnsignedIntType)) 2350 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 2351 return (UnsignedIntType) this.value; 2352 } 2353 2354 public boolean hasValueUnsignedIntType() { 2355 return this != null && this.value instanceof UnsignedIntType; 2356 } 2357 2358 /** 2359 * @return {@link #value} (The value of the Output parameter as a basic type.) 2360 */ 2361 public UriType getValueUriType() throws FHIRException { 2362 if (this.value == null) 2363 this.value = new UriType(); 2364 if (!(this.value instanceof UriType)) 2365 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 2366 return (UriType) this.value; 2367 } 2368 2369 public boolean hasValueUriType() { 2370 return this != null && this.value instanceof UriType; 2371 } 2372 2373 /** 2374 * @return {@link #value} (The value of the Output parameter as a basic type.) 2375 */ 2376 public UrlType getValueUrlType() throws FHIRException { 2377 if (this.value == null) 2378 this.value = new UrlType(); 2379 if (!(this.value instanceof UrlType)) 2380 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 2381 return (UrlType) this.value; 2382 } 2383 2384 public boolean hasValueUrlType() { 2385 return this != null && this.value instanceof UrlType; 2386 } 2387 2388 /** 2389 * @return {@link #value} (The value of the Output parameter as a basic type.) 2390 */ 2391 public UuidType getValueUuidType() throws FHIRException { 2392 if (this.value == null) 2393 this.value = new UuidType(); 2394 if (!(this.value instanceof UuidType)) 2395 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2396 return (UuidType) this.value; 2397 } 2398 2399 public boolean hasValueUuidType() { 2400 return this != null && this.value instanceof UuidType; 2401 } 2402 2403 /** 2404 * @return {@link #value} (The value of the Output parameter as a basic type.) 2405 */ 2406 public Address getValueAddress() throws FHIRException { 2407 if (this.value == null) 2408 this.value = new Address(); 2409 if (!(this.value instanceof Address)) 2410 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 2411 return (Address) this.value; 2412 } 2413 2414 public boolean hasValueAddress() { 2415 return this != null && this.value instanceof Address; 2416 } 2417 2418 /** 2419 * @return {@link #value} (The value of the Output parameter as a basic type.) 2420 */ 2421 public Age getValueAge() throws FHIRException { 2422 if (this.value == null) 2423 this.value = new Age(); 2424 if (!(this.value instanceof Age)) 2425 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 2426 return (Age) this.value; 2427 } 2428 2429 public boolean hasValueAge() { 2430 return this != null && this.value instanceof Age; 2431 } 2432 2433 /** 2434 * @return {@link #value} (The value of the Output parameter as a basic type.) 2435 */ 2436 public Annotation getValueAnnotation() throws FHIRException { 2437 if (this.value == null) 2438 this.value = new Annotation(); 2439 if (!(this.value instanceof Annotation)) 2440 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 2441 return (Annotation) this.value; 2442 } 2443 2444 public boolean hasValueAnnotation() { 2445 return this != null && this.value instanceof Annotation; 2446 } 2447 2448 /** 2449 * @return {@link #value} (The value of the Output parameter as a basic type.) 2450 */ 2451 public Attachment getValueAttachment() throws FHIRException { 2452 if (this.value == null) 2453 this.value = new Attachment(); 2454 if (!(this.value instanceof Attachment)) 2455 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2456 return (Attachment) this.value; 2457 } 2458 2459 public boolean hasValueAttachment() { 2460 return this != null && this.value instanceof Attachment; 2461 } 2462 2463 /** 2464 * @return {@link #value} (The value of the Output parameter as a basic type.) 2465 */ 2466 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2467 if (this.value == null) 2468 this.value = new CodeableConcept(); 2469 if (!(this.value instanceof CodeableConcept)) 2470 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2471 return (CodeableConcept) this.value; 2472 } 2473 2474 public boolean hasValueCodeableConcept() { 2475 return this != null && this.value instanceof CodeableConcept; 2476 } 2477 2478 /** 2479 * @return {@link #value} (The value of the Output parameter as a basic type.) 2480 */ 2481 public CodeableReference getValueCodeableReference() throws FHIRException { 2482 if (this.value == null) 2483 this.value = new CodeableReference(); 2484 if (!(this.value instanceof CodeableReference)) 2485 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 2486 return (CodeableReference) this.value; 2487 } 2488 2489 public boolean hasValueCodeableReference() { 2490 return this != null && this.value instanceof CodeableReference; 2491 } 2492 2493 /** 2494 * @return {@link #value} (The value of the Output parameter as a basic type.) 2495 */ 2496 public Coding getValueCoding() throws FHIRException { 2497 if (this.value == null) 2498 this.value = new Coding(); 2499 if (!(this.value instanceof Coding)) 2500 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2501 return (Coding) this.value; 2502 } 2503 2504 public boolean hasValueCoding() { 2505 return this != null && this.value instanceof Coding; 2506 } 2507 2508 /** 2509 * @return {@link #value} (The value of the Output parameter as a basic type.) 2510 */ 2511 public ContactPoint getValueContactPoint() throws FHIRException { 2512 if (this.value == null) 2513 this.value = new ContactPoint(); 2514 if (!(this.value instanceof ContactPoint)) 2515 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 2516 return (ContactPoint) this.value; 2517 } 2518 2519 public boolean hasValueContactPoint() { 2520 return this != null && this.value instanceof ContactPoint; 2521 } 2522 2523 /** 2524 * @return {@link #value} (The value of the Output parameter as a basic type.) 2525 */ 2526 public Count getValueCount() throws FHIRException { 2527 if (this.value == null) 2528 this.value = new Count(); 2529 if (!(this.value instanceof Count)) 2530 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 2531 return (Count) this.value; 2532 } 2533 2534 public boolean hasValueCount() { 2535 return this != null && this.value instanceof Count; 2536 } 2537 2538 /** 2539 * @return {@link #value} (The value of the Output parameter as a basic type.) 2540 */ 2541 public Distance getValueDistance() throws FHIRException { 2542 if (this.value == null) 2543 this.value = new Distance(); 2544 if (!(this.value instanceof Distance)) 2545 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 2546 return (Distance) this.value; 2547 } 2548 2549 public boolean hasValueDistance() { 2550 return this != null && this.value instanceof Distance; 2551 } 2552 2553 /** 2554 * @return {@link #value} (The value of the Output parameter as a basic type.) 2555 */ 2556 public Duration getValueDuration() throws FHIRException { 2557 if (this.value == null) 2558 this.value = new Duration(); 2559 if (!(this.value instanceof Duration)) 2560 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 2561 return (Duration) this.value; 2562 } 2563 2564 public boolean hasValueDuration() { 2565 return this != null && this.value instanceof Duration; 2566 } 2567 2568 /** 2569 * @return {@link #value} (The value of the Output parameter as a basic type.) 2570 */ 2571 public HumanName getValueHumanName() throws FHIRException { 2572 if (this.value == null) 2573 this.value = new HumanName(); 2574 if (!(this.value instanceof HumanName)) 2575 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 2576 return (HumanName) this.value; 2577 } 2578 2579 public boolean hasValueHumanName() { 2580 return this != null && this.value instanceof HumanName; 2581 } 2582 2583 /** 2584 * @return {@link #value} (The value of the Output parameter as a basic type.) 2585 */ 2586 public Identifier getValueIdentifier() throws FHIRException { 2587 if (this.value == null) 2588 this.value = new Identifier(); 2589 if (!(this.value instanceof Identifier)) 2590 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 2591 return (Identifier) this.value; 2592 } 2593 2594 public boolean hasValueIdentifier() { 2595 return this != null && this.value instanceof Identifier; 2596 } 2597 2598 /** 2599 * @return {@link #value} (The value of the Output parameter as a basic type.) 2600 */ 2601 public Money getValueMoney() throws FHIRException { 2602 if (this.value == null) 2603 this.value = new Money(); 2604 if (!(this.value instanceof Money)) 2605 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 2606 return (Money) this.value; 2607 } 2608 2609 public boolean hasValueMoney() { 2610 return this != null && this.value instanceof Money; 2611 } 2612 2613 /** 2614 * @return {@link #value} (The value of the Output parameter as a basic type.) 2615 */ 2616 public Period getValuePeriod() throws FHIRException { 2617 if (this.value == null) 2618 this.value = new Period(); 2619 if (!(this.value instanceof Period)) 2620 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2621 return (Period) this.value; 2622 } 2623 2624 public boolean hasValuePeriod() { 2625 return this != null && this.value instanceof Period; 2626 } 2627 2628 /** 2629 * @return {@link #value} (The value of the Output parameter as a basic type.) 2630 */ 2631 public Quantity getValueQuantity() throws FHIRException { 2632 if (this.value == null) 2633 this.value = new Quantity(); 2634 if (!(this.value instanceof Quantity)) 2635 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2636 return (Quantity) this.value; 2637 } 2638 2639 public boolean hasValueQuantity() { 2640 return this != null && this.value instanceof Quantity; 2641 } 2642 2643 /** 2644 * @return {@link #value} (The value of the Output parameter as a basic type.) 2645 */ 2646 public Range getValueRange() throws FHIRException { 2647 if (this.value == null) 2648 this.value = new Range(); 2649 if (!(this.value instanceof Range)) 2650 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2651 return (Range) this.value; 2652 } 2653 2654 public boolean hasValueRange() { 2655 return this != null && this.value instanceof Range; 2656 } 2657 2658 /** 2659 * @return {@link #value} (The value of the Output parameter as a basic type.) 2660 */ 2661 public Ratio getValueRatio() throws FHIRException { 2662 if (this.value == null) 2663 this.value = new Ratio(); 2664 if (!(this.value instanceof Ratio)) 2665 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2666 return (Ratio) this.value; 2667 } 2668 2669 public boolean hasValueRatio() { 2670 return this != null && this.value instanceof Ratio; 2671 } 2672 2673 /** 2674 * @return {@link #value} (The value of the Output parameter as a basic type.) 2675 */ 2676 public RatioRange getValueRatioRange() throws FHIRException { 2677 if (this.value == null) 2678 this.value = new RatioRange(); 2679 if (!(this.value instanceof RatioRange)) 2680 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 2681 return (RatioRange) this.value; 2682 } 2683 2684 public boolean hasValueRatioRange() { 2685 return this != null && this.value instanceof RatioRange; 2686 } 2687 2688 /** 2689 * @return {@link #value} (The value of the Output parameter as a basic type.) 2690 */ 2691 public Reference getValueReference() throws FHIRException { 2692 if (this.value == null) 2693 this.value = new Reference(); 2694 if (!(this.value instanceof Reference)) 2695 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 2696 return (Reference) this.value; 2697 } 2698 2699 public boolean hasValueReference() { 2700 return this != null && this.value instanceof Reference; 2701 } 2702 2703 /** 2704 * @return {@link #value} (The value of the Output parameter as a basic type.) 2705 */ 2706 public SampledData getValueSampledData() throws FHIRException { 2707 if (this.value == null) 2708 this.value = new SampledData(); 2709 if (!(this.value instanceof SampledData)) 2710 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 2711 return (SampledData) this.value; 2712 } 2713 2714 public boolean hasValueSampledData() { 2715 return this != null && this.value instanceof SampledData; 2716 } 2717 2718 /** 2719 * @return {@link #value} (The value of the Output parameter as a basic type.) 2720 */ 2721 public Signature getValueSignature() throws FHIRException { 2722 if (this.value == null) 2723 this.value = new Signature(); 2724 if (!(this.value instanceof Signature)) 2725 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 2726 return (Signature) this.value; 2727 } 2728 2729 public boolean hasValueSignature() { 2730 return this != null && this.value instanceof Signature; 2731 } 2732 2733 /** 2734 * @return {@link #value} (The value of the Output parameter as a basic type.) 2735 */ 2736 public Timing getValueTiming() throws FHIRException { 2737 if (this.value == null) 2738 this.value = new Timing(); 2739 if (!(this.value instanceof Timing)) 2740 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 2741 return (Timing) this.value; 2742 } 2743 2744 public boolean hasValueTiming() { 2745 return this != null && this.value instanceof Timing; 2746 } 2747 2748 /** 2749 * @return {@link #value} (The value of the Output parameter as a basic type.) 2750 */ 2751 public ContactDetail getValueContactDetail() throws FHIRException { 2752 if (this.value == null) 2753 this.value = new ContactDetail(); 2754 if (!(this.value instanceof ContactDetail)) 2755 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 2756 return (ContactDetail) this.value; 2757 } 2758 2759 public boolean hasValueContactDetail() { 2760 return this != null && this.value instanceof ContactDetail; 2761 } 2762 2763 /** 2764 * @return {@link #value} (The value of the Output parameter as a basic type.) 2765 */ 2766 public DataRequirement getValueDataRequirement() throws FHIRException { 2767 if (this.value == null) 2768 this.value = new DataRequirement(); 2769 if (!(this.value instanceof DataRequirement)) 2770 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 2771 return (DataRequirement) this.value; 2772 } 2773 2774 public boolean hasValueDataRequirement() { 2775 return this != null && this.value instanceof DataRequirement; 2776 } 2777 2778 /** 2779 * @return {@link #value} (The value of the Output parameter as a basic type.) 2780 */ 2781 public Expression getValueExpression() throws FHIRException { 2782 if (this.value == null) 2783 this.value = new Expression(); 2784 if (!(this.value instanceof Expression)) 2785 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 2786 return (Expression) this.value; 2787 } 2788 2789 public boolean hasValueExpression() { 2790 return this != null && this.value instanceof Expression; 2791 } 2792 2793 /** 2794 * @return {@link #value} (The value of the Output parameter as a basic type.) 2795 */ 2796 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 2797 if (this.value == null) 2798 this.value = new ParameterDefinition(); 2799 if (!(this.value instanceof ParameterDefinition)) 2800 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 2801 return (ParameterDefinition) this.value; 2802 } 2803 2804 public boolean hasValueParameterDefinition() { 2805 return this != null && this.value instanceof ParameterDefinition; 2806 } 2807 2808 /** 2809 * @return {@link #value} (The value of the Output parameter as a basic type.) 2810 */ 2811 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 2812 if (this.value == null) 2813 this.value = new RelatedArtifact(); 2814 if (!(this.value instanceof RelatedArtifact)) 2815 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 2816 return (RelatedArtifact) this.value; 2817 } 2818 2819 public boolean hasValueRelatedArtifact() { 2820 return this != null && this.value instanceof RelatedArtifact; 2821 } 2822 2823 /** 2824 * @return {@link #value} (The value of the Output parameter as a basic type.) 2825 */ 2826 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 2827 if (this.value == null) 2828 this.value = new TriggerDefinition(); 2829 if (!(this.value instanceof TriggerDefinition)) 2830 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 2831 return (TriggerDefinition) this.value; 2832 } 2833 2834 public boolean hasValueTriggerDefinition() { 2835 return this != null && this.value instanceof TriggerDefinition; 2836 } 2837 2838 /** 2839 * @return {@link #value} (The value of the Output parameter as a basic type.) 2840 */ 2841 public UsageContext getValueUsageContext() throws FHIRException { 2842 if (this.value == null) 2843 this.value = new UsageContext(); 2844 if (!(this.value instanceof UsageContext)) 2845 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 2846 return (UsageContext) this.value; 2847 } 2848 2849 public boolean hasValueUsageContext() { 2850 return this != null && this.value instanceof UsageContext; 2851 } 2852 2853 /** 2854 * @return {@link #value} (The value of the Output parameter as a basic type.) 2855 */ 2856 public Availability getValueAvailability() throws FHIRException { 2857 if (this.value == null) 2858 this.value = new Availability(); 2859 if (!(this.value instanceof Availability)) 2860 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 2861 return (Availability) this.value; 2862 } 2863 2864 public boolean hasValueAvailability() { 2865 return this != null && this.value instanceof Availability; 2866 } 2867 2868 /** 2869 * @return {@link #value} (The value of the Output parameter as a basic type.) 2870 */ 2871 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 2872 if (this.value == null) 2873 this.value = new ExtendedContactDetail(); 2874 if (!(this.value instanceof ExtendedContactDetail)) 2875 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 2876 return (ExtendedContactDetail) this.value; 2877 } 2878 2879 public boolean hasValueExtendedContactDetail() { 2880 return this != null && this.value instanceof ExtendedContactDetail; 2881 } 2882 2883 /** 2884 * @return {@link #value} (The value of the Output parameter as a basic type.) 2885 */ 2886 public Dosage getValueDosage() throws FHIRException { 2887 if (this.value == null) 2888 this.value = new Dosage(); 2889 if (!(this.value instanceof Dosage)) 2890 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 2891 return (Dosage) this.value; 2892 } 2893 2894 public boolean hasValueDosage() { 2895 return this != null && this.value instanceof Dosage; 2896 } 2897 2898 /** 2899 * @return {@link #value} (The value of the Output parameter as a basic type.) 2900 */ 2901 public Meta getValueMeta() throws FHIRException { 2902 if (this.value == null) 2903 this.value = new Meta(); 2904 if (!(this.value instanceof Meta)) 2905 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 2906 return (Meta) this.value; 2907 } 2908 2909 public boolean hasValueMeta() { 2910 return this != null && this.value instanceof Meta; 2911 } 2912 2913 public boolean hasValue() { 2914 return this.value != null && !this.value.isEmpty(); 2915 } 2916 2917 /** 2918 * @param value {@link #value} (The value of the Output parameter as a basic type.) 2919 */ 2920 public TransportOutputComponent setValue(DataType value) { 2921 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 2922 throw new FHIRException("Not the right type for Transport.output.value[x]: "+value.fhirType()); 2923 this.value = value; 2924 return this; 2925 } 2926 2927 protected void listChildren(List<Property> children) { 2928 super.listChildren(children); 2929 children.add(new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type)); 2930 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value)); 2931 } 2932 2933 @Override 2934 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2935 switch (_hash) { 2936 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type); 2937 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 2938 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 2939 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The value of the Output parameter as a basic type.", 0, 1, value); 2940 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the Output parameter as a basic type.", 0, 1, value); 2941 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The value of the Output parameter as a basic type.", 0, 1, value); 2942 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of the Output parameter as a basic type.", 0, 1, value); 2943 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The value of the Output parameter as a basic type.", 0, 1, value); 2944 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the Output parameter as a basic type.", 0, 1, value); 2945 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the Output parameter as a basic type.", 0, 1, value); 2946 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The value of the Output parameter as a basic type.", 0, 1, value); 2947 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The value of the Output parameter as a basic type.", 0, 1, value); 2948 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the Output parameter as a basic type.", 0, 1, value); 2949 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The value of the Output parameter as a basic type.", 0, 1, value); 2950 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The value of the Output parameter as a basic type.", 0, 1, value); 2951 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The value of the Output parameter as a basic type.", 0, 1, value); 2952 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The value of the Output parameter as a basic type.", 0, 1, value); 2953 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the Output parameter as a basic type.", 0, 1, value); 2954 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The value of the Output parameter as a basic type.", 0, 1, value); 2955 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The value of the Output parameter as a basic type.", 0, 1, value); 2956 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the Output parameter as a basic type.", 0, 1, value); 2957 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the Output parameter as a basic type.", 0, 1, value); 2958 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The value of the Output parameter as a basic type.", 0, 1, value); 2959 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the Output parameter as a basic type.", 0, 1, value); 2960 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The value of the Output parameter as a basic type.", 0, 1, value); 2961 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the Output parameter as a basic type.", 0, 1, value); 2962 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the Output parameter as a basic type.", 0, 1, value); 2963 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the Output parameter as a basic type.", 0, 1, value); 2964 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The value of the Output parameter as a basic type.", 0, 1, value); 2965 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of the Output parameter as a basic type.", 0, 1, value); 2966 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The value of the Output parameter as a basic type.", 0, 1, value); 2967 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The value of the Output parameter as a basic type.", 0, 1, value); 2968 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The value of the Output parameter as a basic type.", 0, 1, value); 2969 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the Output parameter as a basic type.", 0, 1, value); 2970 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The value of the Output parameter as a basic type.", 0, 1, value); 2971 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The value of the Output parameter as a basic type.", 0, 1, value); 2972 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The value of the Output parameter as a basic type.", 0, 1, value); 2973 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the Output parameter as a basic type.", 0, 1, value); 2974 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the Output parameter as a basic type.", 0, 1, value); 2975 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the Output parameter as a basic type.", 0, 1, value); 2976 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the Output parameter as a basic type.", 0, 1, value); 2977 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The value of the Output parameter as a basic type.", 0, 1, value); 2978 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value of the Output parameter as a basic type.", 0, 1, value); 2979 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The value of the Output parameter as a basic type.", 0, 1, value); 2980 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The value of the Output parameter as a basic type.", 0, 1, value); 2981 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The value of the Output parameter as a basic type.", 0, 1, value); 2982 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The value of the Output parameter as a basic type.", 0, 1, value); 2983 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The value of the Output parameter as a basic type.", 0, 1, value); 2984 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The value of the Output parameter as a basic type.", 0, 1, value); 2985 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The value of the Output parameter as a basic type.", 0, 1, value); 2986 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The value of the Output parameter as a basic type.", 0, 1, value); 2987 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The value of the Output parameter as a basic type.", 0, 1, value); 2988 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The value of the Output parameter as a basic type.", 0, 1, value); 2989 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The value of the Output parameter as a basic type.", 0, 1, value); 2990 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The value of the Output parameter as a basic type.", 0, 1, value); 2991 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The value of the Output parameter as a basic type.", 0, 1, value); 2992 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 2993 default: return super.getNamedProperty(_hash, _name, _checkValid); 2994 } 2995 2996 } 2997 2998 @Override 2999 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3000 switch (hash) { 3001 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3002 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3003 default: return super.getProperty(hash, name, checkValid); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3010 switch (hash) { 3011 case 3575610: // type 3012 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3013 return value; 3014 case 111972721: // value 3015 this.value = TypeConvertor.castToType(value); // DataType 3016 return value; 3017 default: return super.setProperty(hash, name, value); 3018 } 3019 3020 } 3021 3022 @Override 3023 public Base setProperty(String name, Base value) throws FHIRException { 3024 if (name.equals("type")) { 3025 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3026 } else if (name.equals("value[x]")) { 3027 this.value = TypeConvertor.castToType(value); // DataType 3028 } else 3029 return super.setProperty(name, value); 3030 return value; 3031 } 3032 3033 @Override 3034 public void removeChild(String name, Base value) throws FHIRException { 3035 if (name.equals("type")) { 3036 this.type = null; 3037 } else if (name.equals("value[x]")) { 3038 this.value = null; 3039 } else 3040 super.removeChild(name, value); 3041 3042 } 3043 3044 @Override 3045 public Base makeProperty(int hash, String name) throws FHIRException { 3046 switch (hash) { 3047 case 3575610: return getType(); 3048 case -1410166417: return getValue(); 3049 case 111972721: return getValue(); 3050 default: return super.makeProperty(hash, name); 3051 } 3052 3053 } 3054 3055 @Override 3056 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3057 switch (hash) { 3058 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3059 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 3060 default: return super.getTypesForProperty(hash, name); 3061 } 3062 3063 } 3064 3065 @Override 3066 public Base addChild(String name) throws FHIRException { 3067 if (name.equals("type")) { 3068 this.type = new CodeableConcept(); 3069 return this.type; 3070 } 3071 else if (name.equals("valueBase64Binary")) { 3072 this.value = new Base64BinaryType(); 3073 return this.value; 3074 } 3075 else if (name.equals("valueBoolean")) { 3076 this.value = new BooleanType(); 3077 return this.value; 3078 } 3079 else if (name.equals("valueCanonical")) { 3080 this.value = new CanonicalType(); 3081 return this.value; 3082 } 3083 else if (name.equals("valueCode")) { 3084 this.value = new CodeType(); 3085 return this.value; 3086 } 3087 else if (name.equals("valueDate")) { 3088 this.value = new DateType(); 3089 return this.value; 3090 } 3091 else if (name.equals("valueDateTime")) { 3092 this.value = new DateTimeType(); 3093 return this.value; 3094 } 3095 else if (name.equals("valueDecimal")) { 3096 this.value = new DecimalType(); 3097 return this.value; 3098 } 3099 else if (name.equals("valueId")) { 3100 this.value = new IdType(); 3101 return this.value; 3102 } 3103 else if (name.equals("valueInstant")) { 3104 this.value = new InstantType(); 3105 return this.value; 3106 } 3107 else if (name.equals("valueInteger")) { 3108 this.value = new IntegerType(); 3109 return this.value; 3110 } 3111 else if (name.equals("valueInteger64")) { 3112 this.value = new Integer64Type(); 3113 return this.value; 3114 } 3115 else if (name.equals("valueMarkdown")) { 3116 this.value = new MarkdownType(); 3117 return this.value; 3118 } 3119 else if (name.equals("valueOid")) { 3120 this.value = new OidType(); 3121 return this.value; 3122 } 3123 else if (name.equals("valuePositiveInt")) { 3124 this.value = new PositiveIntType(); 3125 return this.value; 3126 } 3127 else if (name.equals("valueString")) { 3128 this.value = new StringType(); 3129 return this.value; 3130 } 3131 else if (name.equals("valueTime")) { 3132 this.value = new TimeType(); 3133 return this.value; 3134 } 3135 else if (name.equals("valueUnsignedInt")) { 3136 this.value = new UnsignedIntType(); 3137 return this.value; 3138 } 3139 else if (name.equals("valueUri")) { 3140 this.value = new UriType(); 3141 return this.value; 3142 } 3143 else if (name.equals("valueUrl")) { 3144 this.value = new UrlType(); 3145 return this.value; 3146 } 3147 else if (name.equals("valueUuid")) { 3148 this.value = new UuidType(); 3149 return this.value; 3150 } 3151 else if (name.equals("valueAddress")) { 3152 this.value = new Address(); 3153 return this.value; 3154 } 3155 else if (name.equals("valueAge")) { 3156 this.value = new Age(); 3157 return this.value; 3158 } 3159 else if (name.equals("valueAnnotation")) { 3160 this.value = new Annotation(); 3161 return this.value; 3162 } 3163 else if (name.equals("valueAttachment")) { 3164 this.value = new Attachment(); 3165 return this.value; 3166 } 3167 else if (name.equals("valueCodeableConcept")) { 3168 this.value = new CodeableConcept(); 3169 return this.value; 3170 } 3171 else if (name.equals("valueCodeableReference")) { 3172 this.value = new CodeableReference(); 3173 return this.value; 3174 } 3175 else if (name.equals("valueCoding")) { 3176 this.value = new Coding(); 3177 return this.value; 3178 } 3179 else if (name.equals("valueContactPoint")) { 3180 this.value = new ContactPoint(); 3181 return this.value; 3182 } 3183 else if (name.equals("valueCount")) { 3184 this.value = new Count(); 3185 return this.value; 3186 } 3187 else if (name.equals("valueDistance")) { 3188 this.value = new Distance(); 3189 return this.value; 3190 } 3191 else if (name.equals("valueDuration")) { 3192 this.value = new Duration(); 3193 return this.value; 3194 } 3195 else if (name.equals("valueHumanName")) { 3196 this.value = new HumanName(); 3197 return this.value; 3198 } 3199 else if (name.equals("valueIdentifier")) { 3200 this.value = new Identifier(); 3201 return this.value; 3202 } 3203 else if (name.equals("valueMoney")) { 3204 this.value = new Money(); 3205 return this.value; 3206 } 3207 else if (name.equals("valuePeriod")) { 3208 this.value = new Period(); 3209 return this.value; 3210 } 3211 else if (name.equals("valueQuantity")) { 3212 this.value = new Quantity(); 3213 return this.value; 3214 } 3215 else if (name.equals("valueRange")) { 3216 this.value = new Range(); 3217 return this.value; 3218 } 3219 else if (name.equals("valueRatio")) { 3220 this.value = new Ratio(); 3221 return this.value; 3222 } 3223 else if (name.equals("valueRatioRange")) { 3224 this.value = new RatioRange(); 3225 return this.value; 3226 } 3227 else if (name.equals("valueReference")) { 3228 this.value = new Reference(); 3229 return this.value; 3230 } 3231 else if (name.equals("valueSampledData")) { 3232 this.value = new SampledData(); 3233 return this.value; 3234 } 3235 else if (name.equals("valueSignature")) { 3236 this.value = new Signature(); 3237 return this.value; 3238 } 3239 else if (name.equals("valueTiming")) { 3240 this.value = new Timing(); 3241 return this.value; 3242 } 3243 else if (name.equals("valueContactDetail")) { 3244 this.value = new ContactDetail(); 3245 return this.value; 3246 } 3247 else if (name.equals("valueDataRequirement")) { 3248 this.value = new DataRequirement(); 3249 return this.value; 3250 } 3251 else if (name.equals("valueExpression")) { 3252 this.value = new Expression(); 3253 return this.value; 3254 } 3255 else if (name.equals("valueParameterDefinition")) { 3256 this.value = new ParameterDefinition(); 3257 return this.value; 3258 } 3259 else if (name.equals("valueRelatedArtifact")) { 3260 this.value = new RelatedArtifact(); 3261 return this.value; 3262 } 3263 else if (name.equals("valueTriggerDefinition")) { 3264 this.value = new TriggerDefinition(); 3265 return this.value; 3266 } 3267 else if (name.equals("valueUsageContext")) { 3268 this.value = new UsageContext(); 3269 return this.value; 3270 } 3271 else if (name.equals("valueAvailability")) { 3272 this.value = new Availability(); 3273 return this.value; 3274 } 3275 else if (name.equals("valueExtendedContactDetail")) { 3276 this.value = new ExtendedContactDetail(); 3277 return this.value; 3278 } 3279 else if (name.equals("valueDosage")) { 3280 this.value = new Dosage(); 3281 return this.value; 3282 } 3283 else if (name.equals("valueMeta")) { 3284 this.value = new Meta(); 3285 return this.value; 3286 } 3287 else 3288 return super.addChild(name); 3289 } 3290 3291 public TransportOutputComponent copy() { 3292 TransportOutputComponent dst = new TransportOutputComponent(); 3293 copyValues(dst); 3294 return dst; 3295 } 3296 3297 public void copyValues(TransportOutputComponent dst) { 3298 super.copyValues(dst); 3299 dst.type = type == null ? null : type.copy(); 3300 dst.value = value == null ? null : value.copy(); 3301 } 3302 3303 @Override 3304 public boolean equalsDeep(Base other_) { 3305 if (!super.equalsDeep(other_)) 3306 return false; 3307 if (!(other_ instanceof TransportOutputComponent)) 3308 return false; 3309 TransportOutputComponent o = (TransportOutputComponent) other_; 3310 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3311 } 3312 3313 @Override 3314 public boolean equalsShallow(Base other_) { 3315 if (!super.equalsShallow(other_)) 3316 return false; 3317 if (!(other_ instanceof TransportOutputComponent)) 3318 return false; 3319 TransportOutputComponent o = (TransportOutputComponent) other_; 3320 return true; 3321 } 3322 3323 public boolean isEmpty() { 3324 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3325 } 3326 3327 public String fhirType() { 3328 return "Transport.output"; 3329 3330 } 3331 3332 } 3333 3334 /** 3335 * Identifier for the transport event that is used to identify it across multiple disparate systems. 3336 */ 3337 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3338 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the transport event that is used to identify it across multiple disparate systems." ) 3339 protected List<Identifier> identifier; 3340 3341 /** 3342 * The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3343 */ 3344 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3345 @Description(shortDefinition="Formal definition of transport", formalDefinition="The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport." ) 3346 protected CanonicalType instantiatesCanonical; 3347 3348 /** 3349 * The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3350 */ 3351 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3352 @Description(shortDefinition="Formal definition of transport", formalDefinition="The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport." ) 3353 protected UriType instantiatesUri; 3354 3355 /** 3356 * BasedOn refers to a higher-level authorization that triggered the creation of the transport. It references a "request" resource such as a ServiceRequest or Transport, which is distinct from the "request" resource the Transport is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a transport is created to fulfill a procedureRequest ( = FocusOn ) to transport a specimen to the lab. 3357 */ 3358 @Child(name = "basedOn", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3359 @Description(shortDefinition="Request fulfilled by this transport", formalDefinition="BasedOn refers to a higher-level authorization that triggered the creation of the transport. It references a \"request\" resource such as a ServiceRequest or Transport, which is distinct from the \"request\" resource the Transport is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a transport is created to fulfill a procedureRequest ( = FocusOn ) to transport a specimen to the lab." ) 3360 protected List<Reference> basedOn; 3361 3362 /** 3363 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 3364 */ 3365 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 3366 @Description(shortDefinition="Requisition or grouper id", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 3367 protected Identifier groupIdentifier; 3368 3369 /** 3370 * A larger event of which this particular event is a component or step. 3371 */ 3372 @Child(name = "partOf", type = {Transport.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3373 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 3374 protected List<Reference> partOf; 3375 3376 /** 3377 * A code specifying the state of the transport event. 3378 */ 3379 @Child(name = "status", type = {CodeType.class}, order=6, min=0, max=1, modifier=true, summary=true) 3380 @Description(shortDefinition="in-progress | completed | abandoned | cancelled | planned | entered-in-error", formalDefinition="A code specifying the state of the transport event." ) 3381 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/transport-status") 3382 protected Enumeration<TransportStatus> status; 3383 3384 /** 3385 * An explanation as to why this transport is held, failed, was refused, etc. 3386 */ 3387 @Child(name = "statusReason", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 3388 @Description(shortDefinition="Reason for current status", formalDefinition="An explanation as to why this transport is held, failed, was refused, etc." ) 3389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/transport-status-reason") 3390 protected CodeableConcept statusReason; 3391 3392 /** 3393 * Indicates the "level" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc. 3394 */ 3395 @Child(name = "intent", type = {CodeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 3396 @Description(shortDefinition="unknown | proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the \"level\" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc." ) 3397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/transport-intent") 3398 protected Enumeration<TransportIntent> intent; 3399 3400 /** 3401 * Indicates how quickly the Transport should be addressed with respect to other requests. 3402 */ 3403 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 3404 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the Transport should be addressed with respect to other requests." ) 3405 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 3406 protected Enumeration<RequestPriority> priority; 3407 3408 /** 3409 * A name or code (or both) briefly describing what the transport involves. 3410 */ 3411 @Child(name = "code", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 3412 @Description(shortDefinition="Transport Type", formalDefinition="A name or code (or both) briefly describing what the transport involves." ) 3413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/transport-code") 3414 protected CodeableConcept code; 3415 3416 /** 3417 * A free-text description of what is to be performed. 3418 */ 3419 @Child(name = "description", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3420 @Description(shortDefinition="Human-readable explanation of transport", formalDefinition="A free-text description of what is to be performed." ) 3421 protected StringType description; 3422 3423 /** 3424 * The request being actioned or the resource being manipulated by this transport. 3425 */ 3426 @Child(name = "focus", type = {Reference.class}, order=12, min=0, max=1, modifier=false, summary=true) 3427 @Description(shortDefinition="What transport is acting on", formalDefinition="The request being actioned or the resource being manipulated by this transport." ) 3428 protected Reference focus; 3429 3430 /** 3431 * The entity who benefits from the performance of the service specified in the transport (e.g., the patient). 3432 */ 3433 @Child(name = "for", type = {Reference.class}, order=13, min=0, max=1, modifier=false, summary=true) 3434 @Description(shortDefinition="Beneficiary of the Transport", formalDefinition="The entity who benefits from the performance of the service specified in the transport (e.g., the patient)." ) 3435 protected Reference for_; 3436 3437 /** 3438 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created. 3439 */ 3440 @Child(name = "encounter", type = {Encounter.class}, order=14, min=0, max=1, modifier=false, summary=true) 3441 @Description(shortDefinition="Healthcare event during which this transport originated", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created." ) 3442 protected Reference encounter; 3443 3444 /** 3445 * Identifies the completion time of the event (the occurrence). 3446 */ 3447 @Child(name = "completionTime", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=true) 3448 @Description(shortDefinition="Completion time of the event (the occurrence)", formalDefinition="Identifies the completion time of the event (the occurrence)." ) 3449 protected DateTimeType completionTime; 3450 3451 /** 3452 * The date and time this transport was created. 3453 */ 3454 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 3455 @Description(shortDefinition="Transport Creation Date", formalDefinition="The date and time this transport was created." ) 3456 protected DateTimeType authoredOn; 3457 3458 /** 3459 * The date and time of last modification to this transport. 3460 */ 3461 @Child(name = "lastModified", type = {DateTimeType.class}, order=17, min=0, max=1, modifier=false, summary=true) 3462 @Description(shortDefinition="Transport Last Modified Date", formalDefinition="The date and time of last modification to this transport." ) 3463 protected DateTimeType lastModified; 3464 3465 /** 3466 * The creator of the transport. 3467 */ 3468 @Child(name = "requester", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=18, min=0, max=1, modifier=false, summary=true) 3469 @Description(shortDefinition="Who is asking for transport to be done", formalDefinition="The creator of the transport." ) 3470 protected Reference requester; 3471 3472 /** 3473 * The kind of participant that should perform the transport. 3474 */ 3475 @Child(name = "performerType", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3476 @Description(shortDefinition="Requested performer", formalDefinition="The kind of participant that should perform the transport." ) 3477 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 3478 protected List<CodeableConcept> performerType; 3479 3480 /** 3481 * Individual organization or Device currently responsible for transport execution. 3482 */ 3483 @Child(name = "owner", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, HealthcareService.class, Patient.class, Device.class, RelatedPerson.class}, order=20, min=0, max=1, modifier=false, summary=true) 3484 @Description(shortDefinition="Responsible individual", formalDefinition="Individual organization or Device currently responsible for transport execution." ) 3485 protected Reference owner; 3486 3487 /** 3488 * Principal physical location where this transport is performed. 3489 */ 3490 @Child(name = "location", type = {Location.class}, order=21, min=0, max=1, modifier=false, summary=true) 3491 @Description(shortDefinition="Where transport occurs", formalDefinition="Principal physical location where this transport is performed." ) 3492 protected Reference location; 3493 3494 /** 3495 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Transport. 3496 */ 3497 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3498 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Transport." ) 3499 protected List<Reference> insurance; 3500 3501 /** 3502 * Free-text information captured about the transport as it progresses. 3503 */ 3504 @Child(name = "note", type = {Annotation.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3505 @Description(shortDefinition="Comments made about the transport", formalDefinition="Free-text information captured about the transport as it progresses." ) 3506 protected List<Annotation> note; 3507 3508 /** 3509 * Links to Provenance records for past versions of this Transport that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the transport. 3510 */ 3511 @Child(name = "relevantHistory", type = {Provenance.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3512 @Description(shortDefinition="Key events in history of the Transport", formalDefinition="Links to Provenance records for past versions of this Transport that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the transport." ) 3513 protected List<Reference> relevantHistory; 3514 3515 /** 3516 * If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned. 3517 */ 3518 @Child(name = "restriction", type = {}, order=25, min=0, max=1, modifier=false, summary=false) 3519 @Description(shortDefinition="Constraints on fulfillment transports", formalDefinition="If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned." ) 3520 protected TransportRestrictionComponent restriction; 3521 3522 /** 3523 * Additional information that may be needed in the execution of the transport. 3524 */ 3525 @Child(name = "input", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3526 @Description(shortDefinition="Information used to perform transport", formalDefinition="Additional information that may be needed in the execution of the transport." ) 3527 protected List<ParameterComponent> input; 3528 3529 /** 3530 * Outputs produced by the Transport. 3531 */ 3532 @Child(name = "output", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3533 @Description(shortDefinition="Information produced as part of transport", formalDefinition="Outputs produced by the Transport." ) 3534 protected List<TransportOutputComponent> output; 3535 3536 /** 3537 * The desired or final location for the transport. 3538 */ 3539 @Child(name = "requestedLocation", type = {Location.class}, order=28, min=1, max=1, modifier=false, summary=true) 3540 @Description(shortDefinition="The desired location", formalDefinition="The desired or final location for the transport." ) 3541 protected Reference requestedLocation; 3542 3543 /** 3544 * The current location for the entity to be transported. 3545 */ 3546 @Child(name = "currentLocation", type = {Location.class}, order=29, min=1, max=1, modifier=false, summary=true) 3547 @Description(shortDefinition="The entity current location", formalDefinition="The current location for the entity to be transported." ) 3548 protected Reference currentLocation; 3549 3550 /** 3551 * A resource reference indicating why this transport needs to be performed. 3552 */ 3553 @Child(name = "reason", type = {CodeableReference.class}, order=30, min=0, max=1, modifier=false, summary=false) 3554 @Description(shortDefinition="Why transport is needed", formalDefinition="A resource reference indicating why this transport needs to be performed." ) 3555 protected CodeableReference reason; 3556 3557 /** 3558 * The transport event prior to this one. 3559 */ 3560 @Child(name = "history", type = {Transport.class}, order=31, min=0, max=1, modifier=false, summary=false) 3561 @Description(shortDefinition="Parent (or preceding) transport", formalDefinition="The transport event prior to this one." ) 3562 protected Reference history; 3563 3564 private static final long serialVersionUID = -2095581755L; 3565 3566 /** 3567 * Constructor 3568 */ 3569 public Transport() { 3570 super(); 3571 } 3572 3573 /** 3574 * Constructor 3575 */ 3576 public Transport(TransportIntent intent, Reference requestedLocation, Reference currentLocation) { 3577 super(); 3578 this.setIntent(intent); 3579 this.setRequestedLocation(requestedLocation); 3580 this.setCurrentLocation(currentLocation); 3581 } 3582 3583 /** 3584 * @return {@link #identifier} (Identifier for the transport event that is used to identify it across multiple disparate systems.) 3585 */ 3586 public List<Identifier> getIdentifier() { 3587 if (this.identifier == null) 3588 this.identifier = new ArrayList<Identifier>(); 3589 return this.identifier; 3590 } 3591 3592 /** 3593 * @return Returns a reference to <code>this</code> for easy method chaining 3594 */ 3595 public Transport setIdentifier(List<Identifier> theIdentifier) { 3596 this.identifier = theIdentifier; 3597 return this; 3598 } 3599 3600 public boolean hasIdentifier() { 3601 if (this.identifier == null) 3602 return false; 3603 for (Identifier item : this.identifier) 3604 if (!item.isEmpty()) 3605 return true; 3606 return false; 3607 } 3608 3609 public Identifier addIdentifier() { //3 3610 Identifier t = new Identifier(); 3611 if (this.identifier == null) 3612 this.identifier = new ArrayList<Identifier>(); 3613 this.identifier.add(t); 3614 return t; 3615 } 3616 3617 public Transport addIdentifier(Identifier t) { //3 3618 if (t == null) 3619 return this; 3620 if (this.identifier == null) 3621 this.identifier = new ArrayList<Identifier>(); 3622 this.identifier.add(t); 3623 return this; 3624 } 3625 3626 /** 3627 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3628 */ 3629 public Identifier getIdentifierFirstRep() { 3630 if (getIdentifier().isEmpty()) { 3631 addIdentifier(); 3632 } 3633 return getIdentifier().get(0); 3634 } 3635 3636 /** 3637 * @return {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3638 */ 3639 public CanonicalType getInstantiatesCanonicalElement() { 3640 if (this.instantiatesCanonical == null) 3641 if (Configuration.errorOnAutoCreate()) 3642 throw new Error("Attempt to auto-create Transport.instantiatesCanonical"); 3643 else if (Configuration.doAutoCreate()) 3644 this.instantiatesCanonical = new CanonicalType(); // bb 3645 return this.instantiatesCanonical; 3646 } 3647 3648 public boolean hasInstantiatesCanonicalElement() { 3649 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3650 } 3651 3652 public boolean hasInstantiatesCanonical() { 3653 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3654 } 3655 3656 /** 3657 * @param value {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3658 */ 3659 public Transport setInstantiatesCanonicalElement(CanonicalType value) { 3660 this.instantiatesCanonical = value; 3661 return this; 3662 } 3663 3664 /** 3665 * @return The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3666 */ 3667 public String getInstantiatesCanonical() { 3668 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 3669 } 3670 3671 /** 3672 * @param value The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3673 */ 3674 public Transport setInstantiatesCanonical(String value) { 3675 if (Utilities.noString(value)) 3676 this.instantiatesCanonical = null; 3677 else { 3678 if (this.instantiatesCanonical == null) 3679 this.instantiatesCanonical = new CanonicalType(); 3680 this.instantiatesCanonical.setValue(value); 3681 } 3682 return this; 3683 } 3684 3685 /** 3686 * @return {@link #instantiatesUri} (The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 3687 */ 3688 public UriType getInstantiatesUriElement() { 3689 if (this.instantiatesUri == null) 3690 if (Configuration.errorOnAutoCreate()) 3691 throw new Error("Attempt to auto-create Transport.instantiatesUri"); 3692 else if (Configuration.doAutoCreate()) 3693 this.instantiatesUri = new UriType(); // bb 3694 return this.instantiatesUri; 3695 } 3696 3697 public boolean hasInstantiatesUriElement() { 3698 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 3699 } 3700 3701 public boolean hasInstantiatesUri() { 3702 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 3703 } 3704 3705 /** 3706 * @param value {@link #instantiatesUri} (The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 3707 */ 3708 public Transport setInstantiatesUriElement(UriType value) { 3709 this.instantiatesUri = value; 3710 return this; 3711 } 3712 3713 /** 3714 * @return The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3715 */ 3716 public String getInstantiatesUri() { 3717 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 3718 } 3719 3720 /** 3721 * @param value The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport. 3722 */ 3723 public Transport setInstantiatesUri(String value) { 3724 if (Utilities.noString(value)) 3725 this.instantiatesUri = null; 3726 else { 3727 if (this.instantiatesUri == null) 3728 this.instantiatesUri = new UriType(); 3729 this.instantiatesUri.setValue(value); 3730 } 3731 return this; 3732 } 3733 3734 /** 3735 * @return {@link #basedOn} (BasedOn refers to a higher-level authorization that triggered the creation of the transport. It references a "request" resource such as a ServiceRequest or Transport, which is distinct from the "request" resource the Transport is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a transport is created to fulfill a procedureRequest ( = FocusOn ) to transport a specimen to the lab.) 3736 */ 3737 public List<Reference> getBasedOn() { 3738 if (this.basedOn == null) 3739 this.basedOn = new ArrayList<Reference>(); 3740 return this.basedOn; 3741 } 3742 3743 /** 3744 * @return Returns a reference to <code>this</code> for easy method chaining 3745 */ 3746 public Transport setBasedOn(List<Reference> theBasedOn) { 3747 this.basedOn = theBasedOn; 3748 return this; 3749 } 3750 3751 public boolean hasBasedOn() { 3752 if (this.basedOn == null) 3753 return false; 3754 for (Reference item : this.basedOn) 3755 if (!item.isEmpty()) 3756 return true; 3757 return false; 3758 } 3759 3760 public Reference addBasedOn() { //3 3761 Reference t = new Reference(); 3762 if (this.basedOn == null) 3763 this.basedOn = new ArrayList<Reference>(); 3764 this.basedOn.add(t); 3765 return t; 3766 } 3767 3768 public Transport addBasedOn(Reference t) { //3 3769 if (t == null) 3770 return this; 3771 if (this.basedOn == null) 3772 this.basedOn = new ArrayList<Reference>(); 3773 this.basedOn.add(t); 3774 return this; 3775 } 3776 3777 /** 3778 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 3779 */ 3780 public Reference getBasedOnFirstRep() { 3781 if (getBasedOn().isEmpty()) { 3782 addBasedOn(); 3783 } 3784 return getBasedOn().get(0); 3785 } 3786 3787 /** 3788 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 3789 */ 3790 public Identifier getGroupIdentifier() { 3791 if (this.groupIdentifier == null) 3792 if (Configuration.errorOnAutoCreate()) 3793 throw new Error("Attempt to auto-create Transport.groupIdentifier"); 3794 else if (Configuration.doAutoCreate()) 3795 this.groupIdentifier = new Identifier(); // cc 3796 return this.groupIdentifier; 3797 } 3798 3799 public boolean hasGroupIdentifier() { 3800 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 3801 } 3802 3803 /** 3804 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 3805 */ 3806 public Transport setGroupIdentifier(Identifier value) { 3807 this.groupIdentifier = value; 3808 return this; 3809 } 3810 3811 /** 3812 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 3813 */ 3814 public List<Reference> getPartOf() { 3815 if (this.partOf == null) 3816 this.partOf = new ArrayList<Reference>(); 3817 return this.partOf; 3818 } 3819 3820 /** 3821 * @return Returns a reference to <code>this</code> for easy method chaining 3822 */ 3823 public Transport setPartOf(List<Reference> thePartOf) { 3824 this.partOf = thePartOf; 3825 return this; 3826 } 3827 3828 public boolean hasPartOf() { 3829 if (this.partOf == null) 3830 return false; 3831 for (Reference item : this.partOf) 3832 if (!item.isEmpty()) 3833 return true; 3834 return false; 3835 } 3836 3837 public Reference addPartOf() { //3 3838 Reference t = new Reference(); 3839 if (this.partOf == null) 3840 this.partOf = new ArrayList<Reference>(); 3841 this.partOf.add(t); 3842 return t; 3843 } 3844 3845 public Transport addPartOf(Reference t) { //3 3846 if (t == null) 3847 return this; 3848 if (this.partOf == null) 3849 this.partOf = new ArrayList<Reference>(); 3850 this.partOf.add(t); 3851 return this; 3852 } 3853 3854 /** 3855 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 3856 */ 3857 public Reference getPartOfFirstRep() { 3858 if (getPartOf().isEmpty()) { 3859 addPartOf(); 3860 } 3861 return getPartOf().get(0); 3862 } 3863 3864 /** 3865 * @return {@link #status} (A code specifying the state of the transport event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3866 */ 3867 public Enumeration<TransportStatus> getStatusElement() { 3868 if (this.status == null) 3869 if (Configuration.errorOnAutoCreate()) 3870 throw new Error("Attempt to auto-create Transport.status"); 3871 else if (Configuration.doAutoCreate()) 3872 this.status = new Enumeration<TransportStatus>(new TransportStatusEnumFactory()); // bb 3873 return this.status; 3874 } 3875 3876 public boolean hasStatusElement() { 3877 return this.status != null && !this.status.isEmpty(); 3878 } 3879 3880 public boolean hasStatus() { 3881 return this.status != null && !this.status.isEmpty(); 3882 } 3883 3884 /** 3885 * @param value {@link #status} (A code specifying the state of the transport event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3886 */ 3887 public Transport setStatusElement(Enumeration<TransportStatus> value) { 3888 this.status = value; 3889 return this; 3890 } 3891 3892 /** 3893 * @return A code specifying the state of the transport event. 3894 */ 3895 public TransportStatus getStatus() { 3896 return this.status == null ? null : this.status.getValue(); 3897 } 3898 3899 /** 3900 * @param value A code specifying the state of the transport event. 3901 */ 3902 public Transport setStatus(TransportStatus value) { 3903 if (value == null) 3904 this.status = null; 3905 else { 3906 if (this.status == null) 3907 this.status = new Enumeration<TransportStatus>(new TransportStatusEnumFactory()); 3908 this.status.setValue(value); 3909 } 3910 return this; 3911 } 3912 3913 /** 3914 * @return {@link #statusReason} (An explanation as to why this transport is held, failed, was refused, etc.) 3915 */ 3916 public CodeableConcept getStatusReason() { 3917 if (this.statusReason == null) 3918 if (Configuration.errorOnAutoCreate()) 3919 throw new Error("Attempt to auto-create Transport.statusReason"); 3920 else if (Configuration.doAutoCreate()) 3921 this.statusReason = new CodeableConcept(); // cc 3922 return this.statusReason; 3923 } 3924 3925 public boolean hasStatusReason() { 3926 return this.statusReason != null && !this.statusReason.isEmpty(); 3927 } 3928 3929 /** 3930 * @param value {@link #statusReason} (An explanation as to why this transport is held, failed, was refused, etc.) 3931 */ 3932 public Transport setStatusReason(CodeableConcept value) { 3933 this.statusReason = value; 3934 return this; 3935 } 3936 3937 /** 3938 * @return {@link #intent} (Indicates the "level" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 3939 */ 3940 public Enumeration<TransportIntent> getIntentElement() { 3941 if (this.intent == null) 3942 if (Configuration.errorOnAutoCreate()) 3943 throw new Error("Attempt to auto-create Transport.intent"); 3944 else if (Configuration.doAutoCreate()) 3945 this.intent = new Enumeration<TransportIntent>(new TransportIntentEnumFactory()); // bb 3946 return this.intent; 3947 } 3948 3949 public boolean hasIntentElement() { 3950 return this.intent != null && !this.intent.isEmpty(); 3951 } 3952 3953 public boolean hasIntent() { 3954 return this.intent != null && !this.intent.isEmpty(); 3955 } 3956 3957 /** 3958 * @param value {@link #intent} (Indicates the "level" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 3959 */ 3960 public Transport setIntentElement(Enumeration<TransportIntent> value) { 3961 this.intent = value; 3962 return this; 3963 } 3964 3965 /** 3966 * @return Indicates the "level" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc. 3967 */ 3968 public TransportIntent getIntent() { 3969 return this.intent == null ? null : this.intent.getValue(); 3970 } 3971 3972 /** 3973 * @param value Indicates the "level" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc. 3974 */ 3975 public Transport setIntent(TransportIntent value) { 3976 if (this.intent == null) 3977 this.intent = new Enumeration<TransportIntent>(new TransportIntentEnumFactory()); 3978 this.intent.setValue(value); 3979 return this; 3980 } 3981 3982 /** 3983 * @return {@link #priority} (Indicates how quickly the Transport should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 3984 */ 3985 public Enumeration<RequestPriority> getPriorityElement() { 3986 if (this.priority == null) 3987 if (Configuration.errorOnAutoCreate()) 3988 throw new Error("Attempt to auto-create Transport.priority"); 3989 else if (Configuration.doAutoCreate()) 3990 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3991 return this.priority; 3992 } 3993 3994 public boolean hasPriorityElement() { 3995 return this.priority != null && !this.priority.isEmpty(); 3996 } 3997 3998 public boolean hasPriority() { 3999 return this.priority != null && !this.priority.isEmpty(); 4000 } 4001 4002 /** 4003 * @param value {@link #priority} (Indicates how quickly the Transport should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4004 */ 4005 public Transport setPriorityElement(Enumeration<RequestPriority> value) { 4006 this.priority = value; 4007 return this; 4008 } 4009 4010 /** 4011 * @return Indicates how quickly the Transport should be addressed with respect to other requests. 4012 */ 4013 public RequestPriority getPriority() { 4014 return this.priority == null ? null : this.priority.getValue(); 4015 } 4016 4017 /** 4018 * @param value Indicates how quickly the Transport should be addressed with respect to other requests. 4019 */ 4020 public Transport setPriority(RequestPriority value) { 4021 if (value == null) 4022 this.priority = null; 4023 else { 4024 if (this.priority == null) 4025 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4026 this.priority.setValue(value); 4027 } 4028 return this; 4029 } 4030 4031 /** 4032 * @return {@link #code} (A name or code (or both) briefly describing what the transport involves.) 4033 */ 4034 public CodeableConcept getCode() { 4035 if (this.code == null) 4036 if (Configuration.errorOnAutoCreate()) 4037 throw new Error("Attempt to auto-create Transport.code"); 4038 else if (Configuration.doAutoCreate()) 4039 this.code = new CodeableConcept(); // cc 4040 return this.code; 4041 } 4042 4043 public boolean hasCode() { 4044 return this.code != null && !this.code.isEmpty(); 4045 } 4046 4047 /** 4048 * @param value {@link #code} (A name or code (or both) briefly describing what the transport involves.) 4049 */ 4050 public Transport setCode(CodeableConcept value) { 4051 this.code = value; 4052 return this; 4053 } 4054 4055 /** 4056 * @return {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4057 */ 4058 public StringType getDescriptionElement() { 4059 if (this.description == null) 4060 if (Configuration.errorOnAutoCreate()) 4061 throw new Error("Attempt to auto-create Transport.description"); 4062 else if (Configuration.doAutoCreate()) 4063 this.description = new StringType(); // bb 4064 return this.description; 4065 } 4066 4067 public boolean hasDescriptionElement() { 4068 return this.description != null && !this.description.isEmpty(); 4069 } 4070 4071 public boolean hasDescription() { 4072 return this.description != null && !this.description.isEmpty(); 4073 } 4074 4075 /** 4076 * @param value {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4077 */ 4078 public Transport setDescriptionElement(StringType value) { 4079 this.description = value; 4080 return this; 4081 } 4082 4083 /** 4084 * @return A free-text description of what is to be performed. 4085 */ 4086 public String getDescription() { 4087 return this.description == null ? null : this.description.getValue(); 4088 } 4089 4090 /** 4091 * @param value A free-text description of what is to be performed. 4092 */ 4093 public Transport setDescription(String value) { 4094 if (Utilities.noString(value)) 4095 this.description = null; 4096 else { 4097 if (this.description == null) 4098 this.description = new StringType(); 4099 this.description.setValue(value); 4100 } 4101 return this; 4102 } 4103 4104 /** 4105 * @return {@link #focus} (The request being actioned or the resource being manipulated by this transport.) 4106 */ 4107 public Reference getFocus() { 4108 if (this.focus == null) 4109 if (Configuration.errorOnAutoCreate()) 4110 throw new Error("Attempt to auto-create Transport.focus"); 4111 else if (Configuration.doAutoCreate()) 4112 this.focus = new Reference(); // cc 4113 return this.focus; 4114 } 4115 4116 public boolean hasFocus() { 4117 return this.focus != null && !this.focus.isEmpty(); 4118 } 4119 4120 /** 4121 * @param value {@link #focus} (The request being actioned or the resource being manipulated by this transport.) 4122 */ 4123 public Transport setFocus(Reference value) { 4124 this.focus = value; 4125 return this; 4126 } 4127 4128 /** 4129 * @return {@link #for_} (The entity who benefits from the performance of the service specified in the transport (e.g., the patient).) 4130 */ 4131 public Reference getFor() { 4132 if (this.for_ == null) 4133 if (Configuration.errorOnAutoCreate()) 4134 throw new Error("Attempt to auto-create Transport.for_"); 4135 else if (Configuration.doAutoCreate()) 4136 this.for_ = new Reference(); // cc 4137 return this.for_; 4138 } 4139 4140 public boolean hasFor() { 4141 return this.for_ != null && !this.for_.isEmpty(); 4142 } 4143 4144 /** 4145 * @param value {@link #for_} (The entity who benefits from the performance of the service specified in the transport (e.g., the patient).) 4146 */ 4147 public Transport setFor(Reference value) { 4148 this.for_ = value; 4149 return this; 4150 } 4151 4152 /** 4153 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created.) 4154 */ 4155 public Reference getEncounter() { 4156 if (this.encounter == null) 4157 if (Configuration.errorOnAutoCreate()) 4158 throw new Error("Attempt to auto-create Transport.encounter"); 4159 else if (Configuration.doAutoCreate()) 4160 this.encounter = new Reference(); // cc 4161 return this.encounter; 4162 } 4163 4164 public boolean hasEncounter() { 4165 return this.encounter != null && !this.encounter.isEmpty(); 4166 } 4167 4168 /** 4169 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created.) 4170 */ 4171 public Transport setEncounter(Reference value) { 4172 this.encounter = value; 4173 return this; 4174 } 4175 4176 /** 4177 * @return {@link #completionTime} (Identifies the completion time of the event (the occurrence).). This is the underlying object with id, value and extensions. The accessor "getCompletionTime" gives direct access to the value 4178 */ 4179 public DateTimeType getCompletionTimeElement() { 4180 if (this.completionTime == null) 4181 if (Configuration.errorOnAutoCreate()) 4182 throw new Error("Attempt to auto-create Transport.completionTime"); 4183 else if (Configuration.doAutoCreate()) 4184 this.completionTime = new DateTimeType(); // bb 4185 return this.completionTime; 4186 } 4187 4188 public boolean hasCompletionTimeElement() { 4189 return this.completionTime != null && !this.completionTime.isEmpty(); 4190 } 4191 4192 public boolean hasCompletionTime() { 4193 return this.completionTime != null && !this.completionTime.isEmpty(); 4194 } 4195 4196 /** 4197 * @param value {@link #completionTime} (Identifies the completion time of the event (the occurrence).). This is the underlying object with id, value and extensions. The accessor "getCompletionTime" gives direct access to the value 4198 */ 4199 public Transport setCompletionTimeElement(DateTimeType value) { 4200 this.completionTime = value; 4201 return this; 4202 } 4203 4204 /** 4205 * @return Identifies the completion time of the event (the occurrence). 4206 */ 4207 public Date getCompletionTime() { 4208 return this.completionTime == null ? null : this.completionTime.getValue(); 4209 } 4210 4211 /** 4212 * @param value Identifies the completion time of the event (the occurrence). 4213 */ 4214 public Transport setCompletionTime(Date value) { 4215 if (value == null) 4216 this.completionTime = null; 4217 else { 4218 if (this.completionTime == null) 4219 this.completionTime = new DateTimeType(); 4220 this.completionTime.setValue(value); 4221 } 4222 return this; 4223 } 4224 4225 /** 4226 * @return {@link #authoredOn} (The date and time this transport was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4227 */ 4228 public DateTimeType getAuthoredOnElement() { 4229 if (this.authoredOn == null) 4230 if (Configuration.errorOnAutoCreate()) 4231 throw new Error("Attempt to auto-create Transport.authoredOn"); 4232 else if (Configuration.doAutoCreate()) 4233 this.authoredOn = new DateTimeType(); // bb 4234 return this.authoredOn; 4235 } 4236 4237 public boolean hasAuthoredOnElement() { 4238 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4239 } 4240 4241 public boolean hasAuthoredOn() { 4242 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4243 } 4244 4245 /** 4246 * @param value {@link #authoredOn} (The date and time this transport was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4247 */ 4248 public Transport setAuthoredOnElement(DateTimeType value) { 4249 this.authoredOn = value; 4250 return this; 4251 } 4252 4253 /** 4254 * @return The date and time this transport was created. 4255 */ 4256 public Date getAuthoredOn() { 4257 return this.authoredOn == null ? null : this.authoredOn.getValue(); 4258 } 4259 4260 /** 4261 * @param value The date and time this transport was created. 4262 */ 4263 public Transport setAuthoredOn(Date value) { 4264 if (value == null) 4265 this.authoredOn = null; 4266 else { 4267 if (this.authoredOn == null) 4268 this.authoredOn = new DateTimeType(); 4269 this.authoredOn.setValue(value); 4270 } 4271 return this; 4272 } 4273 4274 /** 4275 * @return {@link #lastModified} (The date and time of last modification to this transport.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4276 */ 4277 public DateTimeType getLastModifiedElement() { 4278 if (this.lastModified == null) 4279 if (Configuration.errorOnAutoCreate()) 4280 throw new Error("Attempt to auto-create Transport.lastModified"); 4281 else if (Configuration.doAutoCreate()) 4282 this.lastModified = new DateTimeType(); // bb 4283 return this.lastModified; 4284 } 4285 4286 public boolean hasLastModifiedElement() { 4287 return this.lastModified != null && !this.lastModified.isEmpty(); 4288 } 4289 4290 public boolean hasLastModified() { 4291 return this.lastModified != null && !this.lastModified.isEmpty(); 4292 } 4293 4294 /** 4295 * @param value {@link #lastModified} (The date and time of last modification to this transport.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4296 */ 4297 public Transport setLastModifiedElement(DateTimeType value) { 4298 this.lastModified = value; 4299 return this; 4300 } 4301 4302 /** 4303 * @return The date and time of last modification to this transport. 4304 */ 4305 public Date getLastModified() { 4306 return this.lastModified == null ? null : this.lastModified.getValue(); 4307 } 4308 4309 /** 4310 * @param value The date and time of last modification to this transport. 4311 */ 4312 public Transport setLastModified(Date value) { 4313 if (value == null) 4314 this.lastModified = null; 4315 else { 4316 if (this.lastModified == null) 4317 this.lastModified = new DateTimeType(); 4318 this.lastModified.setValue(value); 4319 } 4320 return this; 4321 } 4322 4323 /** 4324 * @return {@link #requester} (The creator of the transport.) 4325 */ 4326 public Reference getRequester() { 4327 if (this.requester == null) 4328 if (Configuration.errorOnAutoCreate()) 4329 throw new Error("Attempt to auto-create Transport.requester"); 4330 else if (Configuration.doAutoCreate()) 4331 this.requester = new Reference(); // cc 4332 return this.requester; 4333 } 4334 4335 public boolean hasRequester() { 4336 return this.requester != null && !this.requester.isEmpty(); 4337 } 4338 4339 /** 4340 * @param value {@link #requester} (The creator of the transport.) 4341 */ 4342 public Transport setRequester(Reference value) { 4343 this.requester = value; 4344 return this; 4345 } 4346 4347 /** 4348 * @return {@link #performerType} (The kind of participant that should perform the transport.) 4349 */ 4350 public List<CodeableConcept> getPerformerType() { 4351 if (this.performerType == null) 4352 this.performerType = new ArrayList<CodeableConcept>(); 4353 return this.performerType; 4354 } 4355 4356 /** 4357 * @return Returns a reference to <code>this</code> for easy method chaining 4358 */ 4359 public Transport setPerformerType(List<CodeableConcept> thePerformerType) { 4360 this.performerType = thePerformerType; 4361 return this; 4362 } 4363 4364 public boolean hasPerformerType() { 4365 if (this.performerType == null) 4366 return false; 4367 for (CodeableConcept item : this.performerType) 4368 if (!item.isEmpty()) 4369 return true; 4370 return false; 4371 } 4372 4373 public CodeableConcept addPerformerType() { //3 4374 CodeableConcept t = new CodeableConcept(); 4375 if (this.performerType == null) 4376 this.performerType = new ArrayList<CodeableConcept>(); 4377 this.performerType.add(t); 4378 return t; 4379 } 4380 4381 public Transport addPerformerType(CodeableConcept t) { //3 4382 if (t == null) 4383 return this; 4384 if (this.performerType == null) 4385 this.performerType = new ArrayList<CodeableConcept>(); 4386 this.performerType.add(t); 4387 return this; 4388 } 4389 4390 /** 4391 * @return The first repetition of repeating field {@link #performerType}, creating it if it does not already exist {3} 4392 */ 4393 public CodeableConcept getPerformerTypeFirstRep() { 4394 if (getPerformerType().isEmpty()) { 4395 addPerformerType(); 4396 } 4397 return getPerformerType().get(0); 4398 } 4399 4400 /** 4401 * @return {@link #owner} (Individual organization or Device currently responsible for transport execution.) 4402 */ 4403 public Reference getOwner() { 4404 if (this.owner == null) 4405 if (Configuration.errorOnAutoCreate()) 4406 throw new Error("Attempt to auto-create Transport.owner"); 4407 else if (Configuration.doAutoCreate()) 4408 this.owner = new Reference(); // cc 4409 return this.owner; 4410 } 4411 4412 public boolean hasOwner() { 4413 return this.owner != null && !this.owner.isEmpty(); 4414 } 4415 4416 /** 4417 * @param value {@link #owner} (Individual organization or Device currently responsible for transport execution.) 4418 */ 4419 public Transport setOwner(Reference value) { 4420 this.owner = value; 4421 return this; 4422 } 4423 4424 /** 4425 * @return {@link #location} (Principal physical location where this transport is performed.) 4426 */ 4427 public Reference getLocation() { 4428 if (this.location == null) 4429 if (Configuration.errorOnAutoCreate()) 4430 throw new Error("Attempt to auto-create Transport.location"); 4431 else if (Configuration.doAutoCreate()) 4432 this.location = new Reference(); // cc 4433 return this.location; 4434 } 4435 4436 public boolean hasLocation() { 4437 return this.location != null && !this.location.isEmpty(); 4438 } 4439 4440 /** 4441 * @param value {@link #location} (Principal physical location where this transport is performed.) 4442 */ 4443 public Transport setLocation(Reference value) { 4444 this.location = value; 4445 return this; 4446 } 4447 4448 /** 4449 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Transport.) 4450 */ 4451 public List<Reference> getInsurance() { 4452 if (this.insurance == null) 4453 this.insurance = new ArrayList<Reference>(); 4454 return this.insurance; 4455 } 4456 4457 /** 4458 * @return Returns a reference to <code>this</code> for easy method chaining 4459 */ 4460 public Transport setInsurance(List<Reference> theInsurance) { 4461 this.insurance = theInsurance; 4462 return this; 4463 } 4464 4465 public boolean hasInsurance() { 4466 if (this.insurance == null) 4467 return false; 4468 for (Reference item : this.insurance) 4469 if (!item.isEmpty()) 4470 return true; 4471 return false; 4472 } 4473 4474 public Reference addInsurance() { //3 4475 Reference t = new Reference(); 4476 if (this.insurance == null) 4477 this.insurance = new ArrayList<Reference>(); 4478 this.insurance.add(t); 4479 return t; 4480 } 4481 4482 public Transport addInsurance(Reference t) { //3 4483 if (t == null) 4484 return this; 4485 if (this.insurance == null) 4486 this.insurance = new ArrayList<Reference>(); 4487 this.insurance.add(t); 4488 return this; 4489 } 4490 4491 /** 4492 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 4493 */ 4494 public Reference getInsuranceFirstRep() { 4495 if (getInsurance().isEmpty()) { 4496 addInsurance(); 4497 } 4498 return getInsurance().get(0); 4499 } 4500 4501 /** 4502 * @return {@link #note} (Free-text information captured about the transport as it progresses.) 4503 */ 4504 public List<Annotation> getNote() { 4505 if (this.note == null) 4506 this.note = new ArrayList<Annotation>(); 4507 return this.note; 4508 } 4509 4510 /** 4511 * @return Returns a reference to <code>this</code> for easy method chaining 4512 */ 4513 public Transport setNote(List<Annotation> theNote) { 4514 this.note = theNote; 4515 return this; 4516 } 4517 4518 public boolean hasNote() { 4519 if (this.note == null) 4520 return false; 4521 for (Annotation item : this.note) 4522 if (!item.isEmpty()) 4523 return true; 4524 return false; 4525 } 4526 4527 public Annotation addNote() { //3 4528 Annotation t = new Annotation(); 4529 if (this.note == null) 4530 this.note = new ArrayList<Annotation>(); 4531 this.note.add(t); 4532 return t; 4533 } 4534 4535 public Transport addNote(Annotation t) { //3 4536 if (t == null) 4537 return this; 4538 if (this.note == null) 4539 this.note = new ArrayList<Annotation>(); 4540 this.note.add(t); 4541 return this; 4542 } 4543 4544 /** 4545 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 4546 */ 4547 public Annotation getNoteFirstRep() { 4548 if (getNote().isEmpty()) { 4549 addNote(); 4550 } 4551 return getNote().get(0); 4552 } 4553 4554 /** 4555 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this Transport that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the transport.) 4556 */ 4557 public List<Reference> getRelevantHistory() { 4558 if (this.relevantHistory == null) 4559 this.relevantHistory = new ArrayList<Reference>(); 4560 return this.relevantHistory; 4561 } 4562 4563 /** 4564 * @return Returns a reference to <code>this</code> for easy method chaining 4565 */ 4566 public Transport setRelevantHistory(List<Reference> theRelevantHistory) { 4567 this.relevantHistory = theRelevantHistory; 4568 return this; 4569 } 4570 4571 public boolean hasRelevantHistory() { 4572 if (this.relevantHistory == null) 4573 return false; 4574 for (Reference item : this.relevantHistory) 4575 if (!item.isEmpty()) 4576 return true; 4577 return false; 4578 } 4579 4580 public Reference addRelevantHistory() { //3 4581 Reference t = new Reference(); 4582 if (this.relevantHistory == null) 4583 this.relevantHistory = new ArrayList<Reference>(); 4584 this.relevantHistory.add(t); 4585 return t; 4586 } 4587 4588 public Transport addRelevantHistory(Reference t) { //3 4589 if (t == null) 4590 return this; 4591 if (this.relevantHistory == null) 4592 this.relevantHistory = new ArrayList<Reference>(); 4593 this.relevantHistory.add(t); 4594 return this; 4595 } 4596 4597 /** 4598 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist {3} 4599 */ 4600 public Reference getRelevantHistoryFirstRep() { 4601 if (getRelevantHistory().isEmpty()) { 4602 addRelevantHistory(); 4603 } 4604 return getRelevantHistory().get(0); 4605 } 4606 4607 /** 4608 * @return {@link #restriction} (If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 4609 */ 4610 public TransportRestrictionComponent getRestriction() { 4611 if (this.restriction == null) 4612 if (Configuration.errorOnAutoCreate()) 4613 throw new Error("Attempt to auto-create Transport.restriction"); 4614 else if (Configuration.doAutoCreate()) 4615 this.restriction = new TransportRestrictionComponent(); // cc 4616 return this.restriction; 4617 } 4618 4619 public boolean hasRestriction() { 4620 return this.restriction != null && !this.restriction.isEmpty(); 4621 } 4622 4623 /** 4624 * @param value {@link #restriction} (If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 4625 */ 4626 public Transport setRestriction(TransportRestrictionComponent value) { 4627 this.restriction = value; 4628 return this; 4629 } 4630 4631 /** 4632 * @return {@link #input} (Additional information that may be needed in the execution of the transport.) 4633 */ 4634 public List<ParameterComponent> getInput() { 4635 if (this.input == null) 4636 this.input = new ArrayList<ParameterComponent>(); 4637 return this.input; 4638 } 4639 4640 /** 4641 * @return Returns a reference to <code>this</code> for easy method chaining 4642 */ 4643 public Transport setInput(List<ParameterComponent> theInput) { 4644 this.input = theInput; 4645 return this; 4646 } 4647 4648 public boolean hasInput() { 4649 if (this.input == null) 4650 return false; 4651 for (ParameterComponent item : this.input) 4652 if (!item.isEmpty()) 4653 return true; 4654 return false; 4655 } 4656 4657 public ParameterComponent addInput() { //3 4658 ParameterComponent t = new ParameterComponent(); 4659 if (this.input == null) 4660 this.input = new ArrayList<ParameterComponent>(); 4661 this.input.add(t); 4662 return t; 4663 } 4664 4665 public Transport addInput(ParameterComponent t) { //3 4666 if (t == null) 4667 return this; 4668 if (this.input == null) 4669 this.input = new ArrayList<ParameterComponent>(); 4670 this.input.add(t); 4671 return this; 4672 } 4673 4674 /** 4675 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 4676 */ 4677 public ParameterComponent getInputFirstRep() { 4678 if (getInput().isEmpty()) { 4679 addInput(); 4680 } 4681 return getInput().get(0); 4682 } 4683 4684 /** 4685 * @return {@link #output} (Outputs produced by the Transport.) 4686 */ 4687 public List<TransportOutputComponent> getOutput() { 4688 if (this.output == null) 4689 this.output = new ArrayList<TransportOutputComponent>(); 4690 return this.output; 4691 } 4692 4693 /** 4694 * @return Returns a reference to <code>this</code> for easy method chaining 4695 */ 4696 public Transport setOutput(List<TransportOutputComponent> theOutput) { 4697 this.output = theOutput; 4698 return this; 4699 } 4700 4701 public boolean hasOutput() { 4702 if (this.output == null) 4703 return false; 4704 for (TransportOutputComponent item : this.output) 4705 if (!item.isEmpty()) 4706 return true; 4707 return false; 4708 } 4709 4710 public TransportOutputComponent addOutput() { //3 4711 TransportOutputComponent t = new TransportOutputComponent(); 4712 if (this.output == null) 4713 this.output = new ArrayList<TransportOutputComponent>(); 4714 this.output.add(t); 4715 return t; 4716 } 4717 4718 public Transport addOutput(TransportOutputComponent t) { //3 4719 if (t == null) 4720 return this; 4721 if (this.output == null) 4722 this.output = new ArrayList<TransportOutputComponent>(); 4723 this.output.add(t); 4724 return this; 4725 } 4726 4727 /** 4728 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 4729 */ 4730 public TransportOutputComponent getOutputFirstRep() { 4731 if (getOutput().isEmpty()) { 4732 addOutput(); 4733 } 4734 return getOutput().get(0); 4735 } 4736 4737 /** 4738 * @return {@link #requestedLocation} (The desired or final location for the transport.) 4739 */ 4740 public Reference getRequestedLocation() { 4741 if (this.requestedLocation == null) 4742 if (Configuration.errorOnAutoCreate()) 4743 throw new Error("Attempt to auto-create Transport.requestedLocation"); 4744 else if (Configuration.doAutoCreate()) 4745 this.requestedLocation = new Reference(); // cc 4746 return this.requestedLocation; 4747 } 4748 4749 public boolean hasRequestedLocation() { 4750 return this.requestedLocation != null && !this.requestedLocation.isEmpty(); 4751 } 4752 4753 /** 4754 * @param value {@link #requestedLocation} (The desired or final location for the transport.) 4755 */ 4756 public Transport setRequestedLocation(Reference value) { 4757 this.requestedLocation = value; 4758 return this; 4759 } 4760 4761 /** 4762 * @return {@link #currentLocation} (The current location for the entity to be transported.) 4763 */ 4764 public Reference getCurrentLocation() { 4765 if (this.currentLocation == null) 4766 if (Configuration.errorOnAutoCreate()) 4767 throw new Error("Attempt to auto-create Transport.currentLocation"); 4768 else if (Configuration.doAutoCreate()) 4769 this.currentLocation = new Reference(); // cc 4770 return this.currentLocation; 4771 } 4772 4773 public boolean hasCurrentLocation() { 4774 return this.currentLocation != null && !this.currentLocation.isEmpty(); 4775 } 4776 4777 /** 4778 * @param value {@link #currentLocation} (The current location for the entity to be transported.) 4779 */ 4780 public Transport setCurrentLocation(Reference value) { 4781 this.currentLocation = value; 4782 return this; 4783 } 4784 4785 /** 4786 * @return {@link #reason} (A resource reference indicating why this transport needs to be performed.) 4787 */ 4788 public CodeableReference getReason() { 4789 if (this.reason == null) 4790 if (Configuration.errorOnAutoCreate()) 4791 throw new Error("Attempt to auto-create Transport.reason"); 4792 else if (Configuration.doAutoCreate()) 4793 this.reason = new CodeableReference(); // cc 4794 return this.reason; 4795 } 4796 4797 public boolean hasReason() { 4798 return this.reason != null && !this.reason.isEmpty(); 4799 } 4800 4801 /** 4802 * @param value {@link #reason} (A resource reference indicating why this transport needs to be performed.) 4803 */ 4804 public Transport setReason(CodeableReference value) { 4805 this.reason = value; 4806 return this; 4807 } 4808 4809 /** 4810 * @return {@link #history} (The transport event prior to this one.) 4811 */ 4812 public Reference getHistory() { 4813 if (this.history == null) 4814 if (Configuration.errorOnAutoCreate()) 4815 throw new Error("Attempt to auto-create Transport.history"); 4816 else if (Configuration.doAutoCreate()) 4817 this.history = new Reference(); // cc 4818 return this.history; 4819 } 4820 4821 public boolean hasHistory() { 4822 return this.history != null && !this.history.isEmpty(); 4823 } 4824 4825 /** 4826 * @param value {@link #history} (The transport event prior to this one.) 4827 */ 4828 public Transport setHistory(Reference value) { 4829 this.history = value; 4830 return this; 4831 } 4832 4833 protected void listChildren(List<Property> children) { 4834 super.listChildren(children); 4835 children.add(new Property("identifier", "Identifier", "Identifier for the transport event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4836 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition)", "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.", 0, 1, instantiatesCanonical)); 4837 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.", 0, 1, instantiatesUri)); 4838 children.add(new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the transport. It references a \"request\" resource such as a ServiceRequest or Transport, which is distinct from the \"request\" resource the Transport is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a transport is created to fulfill a procedureRequest ( = FocusOn ) to transport a specimen to the lab.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 4839 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 4840 children.add(new Property("partOf", "Reference(Transport)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 4841 children.add(new Property("status", "code", "A code specifying the state of the transport event.", 0, 1, status)); 4842 children.add(new Property("statusReason", "CodeableConcept", "An explanation as to why this transport is held, failed, was refused, etc.", 0, 1, statusReason)); 4843 children.add(new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc.", 0, 1, intent)); 4844 children.add(new Property("priority", "code", "Indicates how quickly the Transport should be addressed with respect to other requests.", 0, 1, priority)); 4845 children.add(new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the transport involves.", 0, 1, code)); 4846 children.add(new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description)); 4847 children.add(new Property("focus", "Reference(Any)", "The request being actioned or the resource being manipulated by this transport.", 0, 1, focus)); 4848 children.add(new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the transport (e.g., the patient).", 0, 1, for_)); 4849 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created.", 0, 1, encounter)); 4850 children.add(new Property("completionTime", "dateTime", "Identifies the completion time of the event (the occurrence).", 0, 1, completionTime)); 4851 children.add(new Property("authoredOn", "dateTime", "The date and time this transport was created.", 0, 1, authoredOn)); 4852 children.add(new Property("lastModified", "dateTime", "The date and time of last modification to this transport.", 0, 1, lastModified)); 4853 children.add(new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The creator of the transport.", 0, 1, requester)); 4854 children.add(new Property("performerType", "CodeableConcept", "The kind of participant that should perform the transport.", 0, java.lang.Integer.MAX_VALUE, performerType)); 4855 children.add(new Property("owner", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "Individual organization or Device currently responsible for transport execution.", 0, 1, owner)); 4856 children.add(new Property("location", "Reference(Location)", "Principal physical location where this transport is performed.", 0, 1, location)); 4857 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Transport.", 0, java.lang.Integer.MAX_VALUE, insurance)); 4858 children.add(new Property("note", "Annotation", "Free-text information captured about the transport as it progresses.", 0, java.lang.Integer.MAX_VALUE, note)); 4859 children.add(new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Transport that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the transport.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 4860 children.add(new Property("restriction", "", "If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction)); 4861 children.add(new Property("input", "", "Additional information that may be needed in the execution of the transport.", 0, java.lang.Integer.MAX_VALUE, input)); 4862 children.add(new Property("output", "", "Outputs produced by the Transport.", 0, java.lang.Integer.MAX_VALUE, output)); 4863 children.add(new Property("requestedLocation", "Reference(Location)", "The desired or final location for the transport.", 0, 1, requestedLocation)); 4864 children.add(new Property("currentLocation", "Reference(Location)", "The current location for the entity to be transported.", 0, 1, currentLocation)); 4865 children.add(new Property("reason", "CodeableReference(Any)", "A resource reference indicating why this transport needs to be performed.", 0, 1, reason)); 4866 children.add(new Property("history", "Reference(Transport)", "The transport event prior to this one.", 0, 1, history)); 4867 } 4868 4869 @Override 4870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4871 switch (_hash) { 4872 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the transport event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 4873 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition)", "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.", 0, 1, instantiatesCanonical); 4874 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Transport.", 0, 1, instantiatesUri); 4875 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the transport. It references a \"request\" resource such as a ServiceRequest or Transport, which is distinct from the \"request\" resource the Transport is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a transport is created to fulfill a procedureRequest ( = FocusOn ) to transport a specimen to the lab.", 0, java.lang.Integer.MAX_VALUE, basedOn); 4876 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 4877 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Transport)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 4878 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the transport event.", 0, 1, status); 4879 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "An explanation as to why this transport is held, failed, was refused, etc.", 0, 1, statusReason); 4880 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Transport, i.e. i+R[9]Cs this a proposed transport, a planned transport, an actionable transport, etc.", 0, 1, intent); 4881 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Transport should be addressed with respect to other requests.", 0, 1, priority); 4882 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the transport involves.", 0, 1, code); 4883 case -1724546052: /*description*/ return new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description); 4884 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The request being actioned or the resource being manipulated by this transport.", 0, 1, focus); 4885 case 101577: /*for*/ return new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the transport (e.g., the patient).", 0, 1, for_); 4886 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this transport was created.", 0, 1, encounter); 4887 case 1146641609: /*completionTime*/ return new Property("completionTime", "dateTime", "Identifies the completion time of the event (the occurrence).", 0, 1, completionTime); 4888 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date and time this transport was created.", 0, 1, authoredOn); 4889 case 1959003007: /*lastModified*/ return new Property("lastModified", "dateTime", "The date and time of last modification to this transport.", 0, 1, lastModified); 4890 case 693933948: /*requester*/ return new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The creator of the transport.", 0, 1, requester); 4891 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "The kind of participant that should perform the transport.", 0, java.lang.Integer.MAX_VALUE, performerType); 4892 case 106164915: /*owner*/ return new Property("owner", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "Individual organization or Device currently responsible for transport execution.", 0, 1, owner); 4893 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Principal physical location where this transport is performed.", 0, 1, location); 4894 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Transport.", 0, java.lang.Integer.MAX_VALUE, insurance); 4895 case 3387378: /*note*/ return new Property("note", "Annotation", "Free-text information captured about the transport as it progresses.", 0, java.lang.Integer.MAX_VALUE, note); 4896 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Transport that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the transport.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 4897 case -1561062452: /*restriction*/ return new Property("restriction", "", "If the Transport.focus is a request resource and the transport is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction); 4898 case 100358090: /*input*/ return new Property("input", "", "Additional information that may be needed in the execution of the transport.", 0, java.lang.Integer.MAX_VALUE, input); 4899 case -1005512447: /*output*/ return new Property("output", "", "Outputs produced by the Transport.", 0, java.lang.Integer.MAX_VALUE, output); 4900 case -1788392125: /*requestedLocation*/ return new Property("requestedLocation", "Reference(Location)", "The desired or final location for the transport.", 0, 1, requestedLocation); 4901 case -140429234: /*currentLocation*/ return new Property("currentLocation", "Reference(Location)", "The current location for the entity to be transported.", 0, 1, currentLocation); 4902 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Any)", "A resource reference indicating why this transport needs to be performed.", 0, 1, reason); 4903 case 926934164: /*history*/ return new Property("history", "Reference(Transport)", "The transport event prior to this one.", 0, 1, history); 4904 default: return super.getNamedProperty(_hash, _name, _checkValid); 4905 } 4906 4907 } 4908 4909 @Override 4910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4911 switch (hash) { 4912 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4913 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : new Base[] {this.instantiatesCanonical}; // CanonicalType 4914 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : new Base[] {this.instantiatesUri}; // UriType 4915 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4916 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 4917 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 4918 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TransportStatus> 4919 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 4920 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<TransportIntent> 4921 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 4922 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 4923 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 4924 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 4925 case 101577: /*for*/ return this.for_ == null ? new Base[0] : new Base[] {this.for_}; // Reference 4926 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 4927 case 1146641609: /*completionTime*/ return this.completionTime == null ? new Base[0] : new Base[] {this.completionTime}; // DateTimeType 4928 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 4929 case 1959003007: /*lastModified*/ return this.lastModified == null ? new Base[0] : new Base[] {this.lastModified}; // DateTimeType 4930 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 4931 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 4932 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 4933 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 4934 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 4935 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4936 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 4937 case -1561062452: /*restriction*/ return this.restriction == null ? new Base[0] : new Base[] {this.restriction}; // TransportRestrictionComponent 4938 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // ParameterComponent 4939 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // TransportOutputComponent 4940 case -1788392125: /*requestedLocation*/ return this.requestedLocation == null ? new Base[0] : new Base[] {this.requestedLocation}; // Reference 4941 case -140429234: /*currentLocation*/ return this.currentLocation == null ? new Base[0] : new Base[] {this.currentLocation}; // Reference 4942 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableReference 4943 case 926934164: /*history*/ return this.history == null ? new Base[0] : new Base[] {this.history}; // Reference 4944 default: return super.getProperty(hash, name, checkValid); 4945 } 4946 4947 } 4948 4949 @Override 4950 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4951 switch (hash) { 4952 case -1618432855: // identifier 4953 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4954 return value; 4955 case 8911915: // instantiatesCanonical 4956 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 4957 return value; 4958 case -1926393373: // instantiatesUri 4959 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 4960 return value; 4961 case -332612366: // basedOn 4962 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 4963 return value; 4964 case -445338488: // groupIdentifier 4965 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 4966 return value; 4967 case -995410646: // partOf 4968 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 4969 return value; 4970 case -892481550: // status 4971 value = new TransportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4972 this.status = (Enumeration) value; // Enumeration<TransportStatus> 4973 return value; 4974 case 2051346646: // statusReason 4975 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4976 return value; 4977 case -1183762788: // intent 4978 value = new TransportIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 4979 this.intent = (Enumeration) value; // Enumeration<TransportIntent> 4980 return value; 4981 case -1165461084: // priority 4982 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4983 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4984 return value; 4985 case 3059181: // code 4986 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4987 return value; 4988 case -1724546052: // description 4989 this.description = TypeConvertor.castToString(value); // StringType 4990 return value; 4991 case 97604824: // focus 4992 this.focus = TypeConvertor.castToReference(value); // Reference 4993 return value; 4994 case 101577: // for 4995 this.for_ = TypeConvertor.castToReference(value); // Reference 4996 return value; 4997 case 1524132147: // encounter 4998 this.encounter = TypeConvertor.castToReference(value); // Reference 4999 return value; 5000 case 1146641609: // completionTime 5001 this.completionTime = TypeConvertor.castToDateTime(value); // DateTimeType 5002 return value; 5003 case -1500852503: // authoredOn 5004 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5005 return value; 5006 case 1959003007: // lastModified 5007 this.lastModified = TypeConvertor.castToDateTime(value); // DateTimeType 5008 return value; 5009 case 693933948: // requester 5010 this.requester = TypeConvertor.castToReference(value); // Reference 5011 return value; 5012 case -901444568: // performerType 5013 this.getPerformerType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5014 return value; 5015 case 106164915: // owner 5016 this.owner = TypeConvertor.castToReference(value); // Reference 5017 return value; 5018 case 1901043637: // location 5019 this.location = TypeConvertor.castToReference(value); // Reference 5020 return value; 5021 case 73049818: // insurance 5022 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 5023 return value; 5024 case 3387378: // note 5025 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 5026 return value; 5027 case 1538891575: // relevantHistory 5028 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); // Reference 5029 return value; 5030 case -1561062452: // restriction 5031 this.restriction = (TransportRestrictionComponent) value; // TransportRestrictionComponent 5032 return value; 5033 case 100358090: // input 5034 this.getInput().add((ParameterComponent) value); // ParameterComponent 5035 return value; 5036 case -1005512447: // output 5037 this.getOutput().add((TransportOutputComponent) value); // TransportOutputComponent 5038 return value; 5039 case -1788392125: // requestedLocation 5040 this.requestedLocation = TypeConvertor.castToReference(value); // Reference 5041 return value; 5042 case -140429234: // currentLocation 5043 this.currentLocation = TypeConvertor.castToReference(value); // Reference 5044 return value; 5045 case -934964668: // reason 5046 this.reason = TypeConvertor.castToCodeableReference(value); // CodeableReference 5047 return value; 5048 case 926934164: // history 5049 this.history = TypeConvertor.castToReference(value); // Reference 5050 return value; 5051 default: return super.setProperty(hash, name, value); 5052 } 5053 5054 } 5055 5056 @Override 5057 public Base setProperty(String name, Base value) throws FHIRException { 5058 if (name.equals("identifier")) { 5059 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5060 } else if (name.equals("instantiatesCanonical")) { 5061 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 5062 } else if (name.equals("instantiatesUri")) { 5063 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 5064 } else if (name.equals("basedOn")) { 5065 this.getBasedOn().add(TypeConvertor.castToReference(value)); 5066 } else if (name.equals("groupIdentifier")) { 5067 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5068 } else if (name.equals("partOf")) { 5069 this.getPartOf().add(TypeConvertor.castToReference(value)); 5070 } else if (name.equals("status")) { 5071 value = new TransportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5072 this.status = (Enumeration) value; // Enumeration<TransportStatus> 5073 } else if (name.equals("statusReason")) { 5074 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5075 } else if (name.equals("intent")) { 5076 value = new TransportIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5077 this.intent = (Enumeration) value; // Enumeration<TransportIntent> 5078 } else if (name.equals("priority")) { 5079 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5080 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5081 } else if (name.equals("code")) { 5082 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5083 } else if (name.equals("description")) { 5084 this.description = TypeConvertor.castToString(value); // StringType 5085 } else if (name.equals("focus")) { 5086 this.focus = TypeConvertor.castToReference(value); // Reference 5087 } else if (name.equals("for")) { 5088 this.for_ = TypeConvertor.castToReference(value); // Reference 5089 } else if (name.equals("encounter")) { 5090 this.encounter = TypeConvertor.castToReference(value); // Reference 5091 } else if (name.equals("completionTime")) { 5092 this.completionTime = TypeConvertor.castToDateTime(value); // DateTimeType 5093 } else if (name.equals("authoredOn")) { 5094 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5095 } else if (name.equals("lastModified")) { 5096 this.lastModified = TypeConvertor.castToDateTime(value); // DateTimeType 5097 } else if (name.equals("requester")) { 5098 this.requester = TypeConvertor.castToReference(value); // Reference 5099 } else if (name.equals("performerType")) { 5100 this.getPerformerType().add(TypeConvertor.castToCodeableConcept(value)); 5101 } else if (name.equals("owner")) { 5102 this.owner = TypeConvertor.castToReference(value); // Reference 5103 } else if (name.equals("location")) { 5104 this.location = TypeConvertor.castToReference(value); // Reference 5105 } else if (name.equals("insurance")) { 5106 this.getInsurance().add(TypeConvertor.castToReference(value)); 5107 } else if (name.equals("note")) { 5108 this.getNote().add(TypeConvertor.castToAnnotation(value)); 5109 } else if (name.equals("relevantHistory")) { 5110 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); 5111 } else if (name.equals("restriction")) { 5112 this.restriction = (TransportRestrictionComponent) value; // TransportRestrictionComponent 5113 } else if (name.equals("input")) { 5114 this.getInput().add((ParameterComponent) value); 5115 } else if (name.equals("output")) { 5116 this.getOutput().add((TransportOutputComponent) value); 5117 } else if (name.equals("requestedLocation")) { 5118 this.requestedLocation = TypeConvertor.castToReference(value); // Reference 5119 } else if (name.equals("currentLocation")) { 5120 this.currentLocation = TypeConvertor.castToReference(value); // Reference 5121 } else if (name.equals("reason")) { 5122 this.reason = TypeConvertor.castToCodeableReference(value); // CodeableReference 5123 } else if (name.equals("history")) { 5124 this.history = TypeConvertor.castToReference(value); // Reference 5125 } else 5126 return super.setProperty(name, value); 5127 return value; 5128 } 5129 5130 @Override 5131 public void removeChild(String name, Base value) throws FHIRException { 5132 if (name.equals("identifier")) { 5133 this.getIdentifier().remove(value); 5134 } else if (name.equals("instantiatesCanonical")) { 5135 this.instantiatesCanonical = null; 5136 } else if (name.equals("instantiatesUri")) { 5137 this.instantiatesUri = null; 5138 } else if (name.equals("basedOn")) { 5139 this.getBasedOn().remove(value); 5140 } else if (name.equals("groupIdentifier")) { 5141 this.groupIdentifier = null; 5142 } else if (name.equals("partOf")) { 5143 this.getPartOf().remove(value); 5144 } else if (name.equals("status")) { 5145 value = new TransportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5146 this.status = (Enumeration) value; // Enumeration<TransportStatus> 5147 } else if (name.equals("statusReason")) { 5148 this.statusReason = null; 5149 } else if (name.equals("intent")) { 5150 value = new TransportIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5151 this.intent = (Enumeration) value; // Enumeration<TransportIntent> 5152 } else if (name.equals("priority")) { 5153 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5154 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5155 } else if (name.equals("code")) { 5156 this.code = null; 5157 } else if (name.equals("description")) { 5158 this.description = null; 5159 } else if (name.equals("focus")) { 5160 this.focus = null; 5161 } else if (name.equals("for")) { 5162 this.for_ = null; 5163 } else if (name.equals("encounter")) { 5164 this.encounter = null; 5165 } else if (name.equals("completionTime")) { 5166 this.completionTime = null; 5167 } else if (name.equals("authoredOn")) { 5168 this.authoredOn = null; 5169 } else if (name.equals("lastModified")) { 5170 this.lastModified = null; 5171 } else if (name.equals("requester")) { 5172 this.requester = null; 5173 } else if (name.equals("performerType")) { 5174 this.getPerformerType().remove(value); 5175 } else if (name.equals("owner")) { 5176 this.owner = null; 5177 } else if (name.equals("location")) { 5178 this.location = null; 5179 } else if (name.equals("insurance")) { 5180 this.getInsurance().remove(value); 5181 } else if (name.equals("note")) { 5182 this.getNote().remove(value); 5183 } else if (name.equals("relevantHistory")) { 5184 this.getRelevantHistory().remove(value); 5185 } else if (name.equals("restriction")) { 5186 this.restriction = (TransportRestrictionComponent) value; // TransportRestrictionComponent 5187 } else if (name.equals("input")) { 5188 this.getInput().remove((ParameterComponent) value); 5189 } else if (name.equals("output")) { 5190 this.getOutput().remove((TransportOutputComponent) value); 5191 } else if (name.equals("requestedLocation")) { 5192 this.requestedLocation = null; 5193 } else if (name.equals("currentLocation")) { 5194 this.currentLocation = null; 5195 } else if (name.equals("reason")) { 5196 this.reason = null; 5197 } else if (name.equals("history")) { 5198 this.history = null; 5199 } else 5200 super.removeChild(name, value); 5201 5202 } 5203 5204 @Override 5205 public Base makeProperty(int hash, String name) throws FHIRException { 5206 switch (hash) { 5207 case -1618432855: return addIdentifier(); 5208 case 8911915: return getInstantiatesCanonicalElement(); 5209 case -1926393373: return getInstantiatesUriElement(); 5210 case -332612366: return addBasedOn(); 5211 case -445338488: return getGroupIdentifier(); 5212 case -995410646: return addPartOf(); 5213 case -892481550: return getStatusElement(); 5214 case 2051346646: return getStatusReason(); 5215 case -1183762788: return getIntentElement(); 5216 case -1165461084: return getPriorityElement(); 5217 case 3059181: return getCode(); 5218 case -1724546052: return getDescriptionElement(); 5219 case 97604824: return getFocus(); 5220 case 101577: return getFor(); 5221 case 1524132147: return getEncounter(); 5222 case 1146641609: return getCompletionTimeElement(); 5223 case -1500852503: return getAuthoredOnElement(); 5224 case 1959003007: return getLastModifiedElement(); 5225 case 693933948: return getRequester(); 5226 case -901444568: return addPerformerType(); 5227 case 106164915: return getOwner(); 5228 case 1901043637: return getLocation(); 5229 case 73049818: return addInsurance(); 5230 case 3387378: return addNote(); 5231 case 1538891575: return addRelevantHistory(); 5232 case -1561062452: return getRestriction(); 5233 case 100358090: return addInput(); 5234 case -1005512447: return addOutput(); 5235 case -1788392125: return getRequestedLocation(); 5236 case -140429234: return getCurrentLocation(); 5237 case -934964668: return getReason(); 5238 case 926934164: return getHistory(); 5239 default: return super.makeProperty(hash, name); 5240 } 5241 5242 } 5243 5244 @Override 5245 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5246 switch (hash) { 5247 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5248 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 5249 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 5250 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 5251 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 5252 case -995410646: /*partOf*/ return new String[] {"Reference"}; 5253 case -892481550: /*status*/ return new String[] {"code"}; 5254 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 5255 case -1183762788: /*intent*/ return new String[] {"code"}; 5256 case -1165461084: /*priority*/ return new String[] {"code"}; 5257 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 5258 case -1724546052: /*description*/ return new String[] {"string"}; 5259 case 97604824: /*focus*/ return new String[] {"Reference"}; 5260 case 101577: /*for*/ return new String[] {"Reference"}; 5261 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5262 case 1146641609: /*completionTime*/ return new String[] {"dateTime"}; 5263 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 5264 case 1959003007: /*lastModified*/ return new String[] {"dateTime"}; 5265 case 693933948: /*requester*/ return new String[] {"Reference"}; 5266 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 5267 case 106164915: /*owner*/ return new String[] {"Reference"}; 5268 case 1901043637: /*location*/ return new String[] {"Reference"}; 5269 case 73049818: /*insurance*/ return new String[] {"Reference"}; 5270 case 3387378: /*note*/ return new String[] {"Annotation"}; 5271 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 5272 case -1561062452: /*restriction*/ return new String[] {}; 5273 case 100358090: /*input*/ return new String[] {}; 5274 case -1005512447: /*output*/ return new String[] {}; 5275 case -1788392125: /*requestedLocation*/ return new String[] {"Reference"}; 5276 case -140429234: /*currentLocation*/ return new String[] {"Reference"}; 5277 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 5278 case 926934164: /*history*/ return new String[] {"Reference"}; 5279 default: return super.getTypesForProperty(hash, name); 5280 } 5281 5282 } 5283 5284 @Override 5285 public Base addChild(String name) throws FHIRException { 5286 if (name.equals("identifier")) { 5287 return addIdentifier(); 5288 } 5289 else if (name.equals("instantiatesCanonical")) { 5290 throw new FHIRException("Cannot call addChild on a singleton property Transport.instantiatesCanonical"); 5291 } 5292 else if (name.equals("instantiatesUri")) { 5293 throw new FHIRException("Cannot call addChild on a singleton property Transport.instantiatesUri"); 5294 } 5295 else if (name.equals("basedOn")) { 5296 return addBasedOn(); 5297 } 5298 else if (name.equals("groupIdentifier")) { 5299 this.groupIdentifier = new Identifier(); 5300 return this.groupIdentifier; 5301 } 5302 else if (name.equals("partOf")) { 5303 return addPartOf(); 5304 } 5305 else if (name.equals("status")) { 5306 throw new FHIRException("Cannot call addChild on a singleton property Transport.status"); 5307 } 5308 else if (name.equals("statusReason")) { 5309 this.statusReason = new CodeableConcept(); 5310 return this.statusReason; 5311 } 5312 else if (name.equals("intent")) { 5313 throw new FHIRException("Cannot call addChild on a singleton property Transport.intent"); 5314 } 5315 else if (name.equals("priority")) { 5316 throw new FHIRException("Cannot call addChild on a singleton property Transport.priority"); 5317 } 5318 else if (name.equals("code")) { 5319 this.code = new CodeableConcept(); 5320 return this.code; 5321 } 5322 else if (name.equals("description")) { 5323 throw new FHIRException("Cannot call addChild on a singleton property Transport.description"); 5324 } 5325 else if (name.equals("focus")) { 5326 this.focus = new Reference(); 5327 return this.focus; 5328 } 5329 else if (name.equals("for")) { 5330 this.for_ = new Reference(); 5331 return this.for_; 5332 } 5333 else if (name.equals("encounter")) { 5334 this.encounter = new Reference(); 5335 return this.encounter; 5336 } 5337 else if (name.equals("completionTime")) { 5338 throw new FHIRException("Cannot call addChild on a singleton property Transport.completionTime"); 5339 } 5340 else if (name.equals("authoredOn")) { 5341 throw new FHIRException("Cannot call addChild on a singleton property Transport.authoredOn"); 5342 } 5343 else if (name.equals("lastModified")) { 5344 throw new FHIRException("Cannot call addChild on a singleton property Transport.lastModified"); 5345 } 5346 else if (name.equals("requester")) { 5347 this.requester = new Reference(); 5348 return this.requester; 5349 } 5350 else if (name.equals("performerType")) { 5351 return addPerformerType(); 5352 } 5353 else if (name.equals("owner")) { 5354 this.owner = new Reference(); 5355 return this.owner; 5356 } 5357 else if (name.equals("location")) { 5358 this.location = new Reference(); 5359 return this.location; 5360 } 5361 else if (name.equals("insurance")) { 5362 return addInsurance(); 5363 } 5364 else if (name.equals("note")) { 5365 return addNote(); 5366 } 5367 else if (name.equals("relevantHistory")) { 5368 return addRelevantHistory(); 5369 } 5370 else if (name.equals("restriction")) { 5371 this.restriction = new TransportRestrictionComponent(); 5372 return this.restriction; 5373 } 5374 else if (name.equals("input")) { 5375 return addInput(); 5376 } 5377 else if (name.equals("output")) { 5378 return addOutput(); 5379 } 5380 else if (name.equals("requestedLocation")) { 5381 this.requestedLocation = new Reference(); 5382 return this.requestedLocation; 5383 } 5384 else if (name.equals("currentLocation")) { 5385 this.currentLocation = new Reference(); 5386 return this.currentLocation; 5387 } 5388 else if (name.equals("reason")) { 5389 this.reason = new CodeableReference(); 5390 return this.reason; 5391 } 5392 else if (name.equals("history")) { 5393 this.history = new Reference(); 5394 return this.history; 5395 } 5396 else 5397 return super.addChild(name); 5398 } 5399 5400 public String fhirType() { 5401 return "Transport"; 5402 5403 } 5404 5405 public Transport copy() { 5406 Transport dst = new Transport(); 5407 copyValues(dst); 5408 return dst; 5409 } 5410 5411 public void copyValues(Transport dst) { 5412 super.copyValues(dst); 5413 if (identifier != null) { 5414 dst.identifier = new ArrayList<Identifier>(); 5415 for (Identifier i : identifier) 5416 dst.identifier.add(i.copy()); 5417 }; 5418 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 5419 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 5420 if (basedOn != null) { 5421 dst.basedOn = new ArrayList<Reference>(); 5422 for (Reference i : basedOn) 5423 dst.basedOn.add(i.copy()); 5424 }; 5425 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 5426 if (partOf != null) { 5427 dst.partOf = new ArrayList<Reference>(); 5428 for (Reference i : partOf) 5429 dst.partOf.add(i.copy()); 5430 }; 5431 dst.status = status == null ? null : status.copy(); 5432 dst.statusReason = statusReason == null ? null : statusReason.copy(); 5433 dst.intent = intent == null ? null : intent.copy(); 5434 dst.priority = priority == null ? null : priority.copy(); 5435 dst.code = code == null ? null : code.copy(); 5436 dst.description = description == null ? null : description.copy(); 5437 dst.focus = focus == null ? null : focus.copy(); 5438 dst.for_ = for_ == null ? null : for_.copy(); 5439 dst.encounter = encounter == null ? null : encounter.copy(); 5440 dst.completionTime = completionTime == null ? null : completionTime.copy(); 5441 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 5442 dst.lastModified = lastModified == null ? null : lastModified.copy(); 5443 dst.requester = requester == null ? null : requester.copy(); 5444 if (performerType != null) { 5445 dst.performerType = new ArrayList<CodeableConcept>(); 5446 for (CodeableConcept i : performerType) 5447 dst.performerType.add(i.copy()); 5448 }; 5449 dst.owner = owner == null ? null : owner.copy(); 5450 dst.location = location == null ? null : location.copy(); 5451 if (insurance != null) { 5452 dst.insurance = new ArrayList<Reference>(); 5453 for (Reference i : insurance) 5454 dst.insurance.add(i.copy()); 5455 }; 5456 if (note != null) { 5457 dst.note = new ArrayList<Annotation>(); 5458 for (Annotation i : note) 5459 dst.note.add(i.copy()); 5460 }; 5461 if (relevantHistory != null) { 5462 dst.relevantHistory = new ArrayList<Reference>(); 5463 for (Reference i : relevantHistory) 5464 dst.relevantHistory.add(i.copy()); 5465 }; 5466 dst.restriction = restriction == null ? null : restriction.copy(); 5467 if (input != null) { 5468 dst.input = new ArrayList<ParameterComponent>(); 5469 for (ParameterComponent i : input) 5470 dst.input.add(i.copy()); 5471 }; 5472 if (output != null) { 5473 dst.output = new ArrayList<TransportOutputComponent>(); 5474 for (TransportOutputComponent i : output) 5475 dst.output.add(i.copy()); 5476 }; 5477 dst.requestedLocation = requestedLocation == null ? null : requestedLocation.copy(); 5478 dst.currentLocation = currentLocation == null ? null : currentLocation.copy(); 5479 dst.reason = reason == null ? null : reason.copy(); 5480 dst.history = history == null ? null : history.copy(); 5481 } 5482 5483 protected Transport typedCopy() { 5484 return copy(); 5485 } 5486 5487 @Override 5488 public boolean equalsDeep(Base other_) { 5489 if (!super.equalsDeep(other_)) 5490 return false; 5491 if (!(other_ instanceof Transport)) 5492 return false; 5493 Transport o = (Transport) other_; 5494 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5495 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 5496 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(partOf, o.partOf, true) 5497 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(intent, o.intent, true) 5498 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 5499 && compareDeep(focus, o.focus, true) && compareDeep(for_, o.for_, true) && compareDeep(encounter, o.encounter, true) 5500 && compareDeep(completionTime, o.completionTime, true) && compareDeep(authoredOn, o.authoredOn, true) 5501 && compareDeep(lastModified, o.lastModified, true) && compareDeep(requester, o.requester, true) 5502 && compareDeep(performerType, o.performerType, true) && compareDeep(owner, o.owner, true) && compareDeep(location, o.location, true) 5503 && compareDeep(insurance, o.insurance, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 5504 && compareDeep(restriction, o.restriction, true) && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) 5505 && compareDeep(requestedLocation, o.requestedLocation, true) && compareDeep(currentLocation, o.currentLocation, true) 5506 && compareDeep(reason, o.reason, true) && compareDeep(history, o.history, true); 5507 } 5508 5509 @Override 5510 public boolean equalsShallow(Base other_) { 5511 if (!super.equalsShallow(other_)) 5512 return false; 5513 if (!(other_ instanceof Transport)) 5514 return false; 5515 Transport o = (Transport) other_; 5516 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 5517 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 5518 && compareValues(description, o.description, true) && compareValues(completionTime, o.completionTime, true) 5519 && compareValues(authoredOn, o.authoredOn, true) && compareValues(lastModified, o.lastModified, true) 5520 ; 5521 } 5522 5523 public boolean isEmpty() { 5524 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 5525 , instantiatesUri, basedOn, groupIdentifier, partOf, status, statusReason, intent 5526 , priority, code, description, focus, for_, encounter, completionTime, authoredOn 5527 , lastModified, requester, performerType, owner, location, insurance, note, relevantHistory 5528 , restriction, input, output, requestedLocation, currentLocation, reason, history 5529 ); 5530 } 5531 5532 @Override 5533 public ResourceType getResourceType() { 5534 return ResourceType.Transport; 5535 } 5536 5537 /** 5538 * Search parameter: <b>identifier</b> 5539 * <p> 5540 * Description: <b>External identifier</b><br> 5541 * Type: <b>token</b><br> 5542 * Path: <b>Transport.identifier</b><br> 5543 * </p> 5544 */ 5545 @SearchParamDefinition(name="identifier", path="Transport.identifier", description="External identifier", type="token" ) 5546 public static final String SP_IDENTIFIER = "identifier"; 5547 /** 5548 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5549 * <p> 5550 * Description: <b>External identifier</b><br> 5551 * Type: <b>token</b><br> 5552 * Path: <b>Transport.identifier</b><br> 5553 * </p> 5554 */ 5555 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5556 5557 /** 5558 * Search parameter: <b>status</b> 5559 * <p> 5560 * Description: <b>in-progress | completed | entered-in-error</b><br> 5561 * Type: <b>token</b><br> 5562 * Path: <b>Transport.status</b><br> 5563 * </p> 5564 */ 5565 @SearchParamDefinition(name="status", path="Transport.status", description="in-progress | completed | entered-in-error", type="token" ) 5566 public static final String SP_STATUS = "status"; 5567 /** 5568 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5569 * <p> 5570 * Description: <b>in-progress | completed | entered-in-error</b><br> 5571 * Type: <b>token</b><br> 5572 * Path: <b>Transport.status</b><br> 5573 * </p> 5574 */ 5575 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5576 5577 5578} 5579