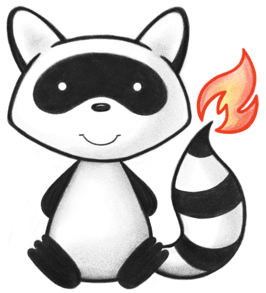
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * TriggerDefinition Type: A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element. 050 */ 051@DatatypeDef(name="TriggerDefinition") 052public class TriggerDefinition extends DataType implements ICompositeType { 053 054 public enum TriggerType { 055 /** 056 * The trigger occurs in response to a specific named event, and no other information about the trigger is specified. Named events are completely pre-coordinated, and the formal semantics of the trigger are not provided. 057 */ 058 NAMEDEVENT, 059 /** 060 * The trigger occurs at a specific time or periodically as described by a timing or schedule. A periodic event cannot have any data elements, but may have a name assigned as a shorthand for the event. 061 */ 062 PERIODIC, 063 /** 064 * The trigger occurs whenever data of a particular type is changed in any way, either added, modified, or removed. 065 */ 066 DATACHANGED, 067 /** 068 * The trigger occurs whenever data of a particular type is added. 069 */ 070 DATAADDED, 071 /** 072 * The trigger occurs whenever data of a particular type is modified. 073 */ 074 DATAMODIFIED, 075 /** 076 * The trigger occurs whenever data of a particular type is removed. 077 */ 078 DATAREMOVED, 079 /** 080 * The trigger occurs whenever data of a particular type is accessed. 081 */ 082 DATAACCESSED, 083 /** 084 * The trigger occurs whenever access to data of a particular type is completed. 085 */ 086 DATAACCESSENDED, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static TriggerType fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("named-event".equals(codeString)) 095 return NAMEDEVENT; 096 if ("periodic".equals(codeString)) 097 return PERIODIC; 098 if ("data-changed".equals(codeString)) 099 return DATACHANGED; 100 if ("data-added".equals(codeString)) 101 return DATAADDED; 102 if ("data-modified".equals(codeString)) 103 return DATAMODIFIED; 104 if ("data-removed".equals(codeString)) 105 return DATAREMOVED; 106 if ("data-accessed".equals(codeString)) 107 return DATAACCESSED; 108 if ("data-access-ended".equals(codeString)) 109 return DATAACCESSENDED; 110 if (Configuration.isAcceptInvalidEnums()) 111 return null; 112 else 113 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 114 } 115 public String toCode() { 116 switch (this) { 117 case NAMEDEVENT: return "named-event"; 118 case PERIODIC: return "periodic"; 119 case DATACHANGED: return "data-changed"; 120 case DATAADDED: return "data-added"; 121 case DATAMODIFIED: return "data-modified"; 122 case DATAREMOVED: return "data-removed"; 123 case DATAACCESSED: return "data-accessed"; 124 case DATAACCESSENDED: return "data-access-ended"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getSystem() { 130 switch (this) { 131 case NAMEDEVENT: return "http://hl7.org/fhir/trigger-type"; 132 case PERIODIC: return "http://hl7.org/fhir/trigger-type"; 133 case DATACHANGED: return "http://hl7.org/fhir/trigger-type"; 134 case DATAADDED: return "http://hl7.org/fhir/trigger-type"; 135 case DATAMODIFIED: return "http://hl7.org/fhir/trigger-type"; 136 case DATAREMOVED: return "http://hl7.org/fhir/trigger-type"; 137 case DATAACCESSED: return "http://hl7.org/fhir/trigger-type"; 138 case DATAACCESSENDED: return "http://hl7.org/fhir/trigger-type"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case NAMEDEVENT: return "The trigger occurs in response to a specific named event, and no other information about the trigger is specified. Named events are completely pre-coordinated, and the formal semantics of the trigger are not provided."; 146 case PERIODIC: return "The trigger occurs at a specific time or periodically as described by a timing or schedule. A periodic event cannot have any data elements, but may have a name assigned as a shorthand for the event."; 147 case DATACHANGED: return "The trigger occurs whenever data of a particular type is changed in any way, either added, modified, or removed."; 148 case DATAADDED: return "The trigger occurs whenever data of a particular type is added."; 149 case DATAMODIFIED: return "The trigger occurs whenever data of a particular type is modified."; 150 case DATAREMOVED: return "The trigger occurs whenever data of a particular type is removed."; 151 case DATAACCESSED: return "The trigger occurs whenever data of a particular type is accessed."; 152 case DATAACCESSENDED: return "The trigger occurs whenever access to data of a particular type is completed."; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getDisplay() { 158 switch (this) { 159 case NAMEDEVENT: return "Named Event"; 160 case PERIODIC: return "Periodic"; 161 case DATACHANGED: return "Data Changed"; 162 case DATAADDED: return "Data Added"; 163 case DATAMODIFIED: return "Data Updated"; 164 case DATAREMOVED: return "Data Removed"; 165 case DATAACCESSED: return "Data Accessed"; 166 case DATAACCESSENDED: return "Data Access Ended"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 } 172 173 public static class TriggerTypeEnumFactory implements EnumFactory<TriggerType> { 174 public TriggerType fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("named-event".equals(codeString)) 179 return TriggerType.NAMEDEVENT; 180 if ("periodic".equals(codeString)) 181 return TriggerType.PERIODIC; 182 if ("data-changed".equals(codeString)) 183 return TriggerType.DATACHANGED; 184 if ("data-added".equals(codeString)) 185 return TriggerType.DATAADDED; 186 if ("data-modified".equals(codeString)) 187 return TriggerType.DATAMODIFIED; 188 if ("data-removed".equals(codeString)) 189 return TriggerType.DATAREMOVED; 190 if ("data-accessed".equals(codeString)) 191 return TriggerType.DATAACCESSED; 192 if ("data-access-ended".equals(codeString)) 193 return TriggerType.DATAACCESSENDED; 194 throw new IllegalArgumentException("Unknown TriggerType code '"+codeString+"'"); 195 } 196 public Enumeration<TriggerType> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 201 String codeString = ((PrimitiveType) code).asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 204 if ("named-event".equals(codeString)) 205 return new Enumeration<TriggerType>(this, TriggerType.NAMEDEVENT, code); 206 if ("periodic".equals(codeString)) 207 return new Enumeration<TriggerType>(this, TriggerType.PERIODIC, code); 208 if ("data-changed".equals(codeString)) 209 return new Enumeration<TriggerType>(this, TriggerType.DATACHANGED, code); 210 if ("data-added".equals(codeString)) 211 return new Enumeration<TriggerType>(this, TriggerType.DATAADDED, code); 212 if ("data-modified".equals(codeString)) 213 return new Enumeration<TriggerType>(this, TriggerType.DATAMODIFIED, code); 214 if ("data-removed".equals(codeString)) 215 return new Enumeration<TriggerType>(this, TriggerType.DATAREMOVED, code); 216 if ("data-accessed".equals(codeString)) 217 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSED, code); 218 if ("data-access-ended".equals(codeString)) 219 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSENDED, code); 220 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 221 } 222 public String toCode(TriggerType code) { 223 if (code == TriggerType.NAMEDEVENT) 224 return "named-event"; 225 if (code == TriggerType.PERIODIC) 226 return "periodic"; 227 if (code == TriggerType.DATACHANGED) 228 return "data-changed"; 229 if (code == TriggerType.DATAADDED) 230 return "data-added"; 231 if (code == TriggerType.DATAMODIFIED) 232 return "data-modified"; 233 if (code == TriggerType.DATAREMOVED) 234 return "data-removed"; 235 if (code == TriggerType.DATAACCESSED) 236 return "data-accessed"; 237 if (code == TriggerType.DATAACCESSENDED) 238 return "data-access-ended"; 239 return "?"; 240 } 241 public String toSystem(TriggerType code) { 242 return code.getSystem(); 243 } 244 } 245 246 /** 247 * The type of triggering event. 248 */ 249 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 250 @Description(shortDefinition="named-event | periodic | data-changed | data-added | data-modified | data-removed | data-accessed | data-access-ended", formalDefinition="The type of triggering event." ) 251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/trigger-type") 252 protected Enumeration<TriggerType> type; 253 254 /** 255 * A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 256 */ 257 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 258 @Description(shortDefinition="Name or URI that identifies the event", formalDefinition="A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context." ) 259 protected StringType name; 260 261 /** 262 * A code that identifies the event. 263 */ 264 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 265 @Description(shortDefinition="Coded definition of the event", formalDefinition="A code that identifies the event." ) 266 protected CodeableConcept code; 267 268 /** 269 * A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 270 */ 271 @Child(name = "subscriptionTopic", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 272 @Description(shortDefinition="What event", formalDefinition="A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied." ) 273 protected CanonicalType subscriptionTopic; 274 275 /** 276 * The timing of the event (if this is a periodic trigger). 277 */ 278 @Child(name = "timing", type = {Timing.class, Schedule.class, DateType.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 279 @Description(shortDefinition="Timing of the event", formalDefinition="The timing of the event (if this is a periodic trigger)." ) 280 protected DataType timing; 281 282 /** 283 * The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true. 284 */ 285 @Child(name = "data", type = {DataRequirement.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 286 @Description(shortDefinition="Triggering data of the event (multiple = 'and')", formalDefinition="The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true." ) 287 protected List<DataRequirement> data; 288 289 /** 290 * A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires. 291 */ 292 @Child(name = "condition", type = {Expression.class}, order=6, min=0, max=1, modifier=false, summary=true) 293 @Description(shortDefinition="Whether the event triggers (boolean expression)", formalDefinition="A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires." ) 294 protected Expression condition; 295 296 private static final long serialVersionUID = -1823040479L; 297 298 /** 299 * Constructor 300 */ 301 public TriggerDefinition() { 302 super(); 303 } 304 305 /** 306 * Constructor 307 */ 308 public TriggerDefinition(TriggerType type) { 309 super(); 310 this.setType(type); 311 } 312 313 /** 314 * @return {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 315 */ 316 public Enumeration<TriggerType> getTypeElement() { 317 if (this.type == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create TriggerDefinition.type"); 320 else if (Configuration.doAutoCreate()) 321 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); // bb 322 return this.type; 323 } 324 325 public boolean hasTypeElement() { 326 return this.type != null && !this.type.isEmpty(); 327 } 328 329 public boolean hasType() { 330 return this.type != null && !this.type.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 335 */ 336 public TriggerDefinition setTypeElement(Enumeration<TriggerType> value) { 337 this.type = value; 338 return this; 339 } 340 341 /** 342 * @return The type of triggering event. 343 */ 344 public TriggerType getType() { 345 return this.type == null ? null : this.type.getValue(); 346 } 347 348 /** 349 * @param value The type of triggering event. 350 */ 351 public TriggerDefinition setType(TriggerType value) { 352 if (this.type == null) 353 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); 354 this.type.setValue(value); 355 return this; 356 } 357 358 /** 359 * @return {@link #name} (A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 360 */ 361 public StringType getNameElement() { 362 if (this.name == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create TriggerDefinition.name"); 365 else if (Configuration.doAutoCreate()) 366 this.name = new StringType(); // bb 367 return this.name; 368 } 369 370 public boolean hasNameElement() { 371 return this.name != null && !this.name.isEmpty(); 372 } 373 374 public boolean hasName() { 375 return this.name != null && !this.name.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #name} (A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 380 */ 381 public TriggerDefinition setNameElement(StringType value) { 382 this.name = value; 383 return this; 384 } 385 386 /** 387 * @return A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 388 */ 389 public String getName() { 390 return this.name == null ? null : this.name.getValue(); 391 } 392 393 /** 394 * @param value A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 395 */ 396 public TriggerDefinition setName(String value) { 397 if (Utilities.noString(value)) 398 this.name = null; 399 else { 400 if (this.name == null) 401 this.name = new StringType(); 402 this.name.setValue(value); 403 } 404 return this; 405 } 406 407 /** 408 * @return {@link #code} (A code that identifies the event.) 409 */ 410 public CodeableConcept getCode() { 411 if (this.code == null) 412 if (Configuration.errorOnAutoCreate()) 413 throw new Error("Attempt to auto-create TriggerDefinition.code"); 414 else if (Configuration.doAutoCreate()) 415 this.code = new CodeableConcept(); // cc 416 return this.code; 417 } 418 419 public boolean hasCode() { 420 return this.code != null && !this.code.isEmpty(); 421 } 422 423 /** 424 * @param value {@link #code} (A code that identifies the event.) 425 */ 426 public TriggerDefinition setCode(CodeableConcept value) { 427 this.code = value; 428 return this; 429 } 430 431 /** 432 * @return {@link #subscriptionTopic} (A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.). This is the underlying object with id, value and extensions. The accessor "getSubscriptionTopic" gives direct access to the value 433 */ 434 public CanonicalType getSubscriptionTopicElement() { 435 if (this.subscriptionTopic == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create TriggerDefinition.subscriptionTopic"); 438 else if (Configuration.doAutoCreate()) 439 this.subscriptionTopic = new CanonicalType(); // bb 440 return this.subscriptionTopic; 441 } 442 443 public boolean hasSubscriptionTopicElement() { 444 return this.subscriptionTopic != null && !this.subscriptionTopic.isEmpty(); 445 } 446 447 public boolean hasSubscriptionTopic() { 448 return this.subscriptionTopic != null && !this.subscriptionTopic.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #subscriptionTopic} (A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.). This is the underlying object with id, value and extensions. The accessor "getSubscriptionTopic" gives direct access to the value 453 */ 454 public TriggerDefinition setSubscriptionTopicElement(CanonicalType value) { 455 this.subscriptionTopic = value; 456 return this; 457 } 458 459 /** 460 * @return A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 461 */ 462 public String getSubscriptionTopic() { 463 return this.subscriptionTopic == null ? null : this.subscriptionTopic.getValue(); 464 } 465 466 /** 467 * @param value A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 468 */ 469 public TriggerDefinition setSubscriptionTopic(String value) { 470 if (Utilities.noString(value)) 471 this.subscriptionTopic = null; 472 else { 473 if (this.subscriptionTopic == null) 474 this.subscriptionTopic = new CanonicalType(); 475 this.subscriptionTopic.setValue(value); 476 } 477 return this; 478 } 479 480 /** 481 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 482 */ 483 public DataType getTiming() { 484 return this.timing; 485 } 486 487 /** 488 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 489 */ 490 public Timing getTimingTiming() throws FHIRException { 491 if (this.timing == null) 492 this.timing = new Timing(); 493 if (!(this.timing instanceof Timing)) 494 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 495 return (Timing) this.timing; 496 } 497 498 public boolean hasTimingTiming() { 499 return this != null && this.timing instanceof Timing; 500 } 501 502 /** 503 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 504 */ 505 public Reference getTimingReference() throws FHIRException { 506 if (this.timing == null) 507 this.timing = new Reference(); 508 if (!(this.timing instanceof Reference)) 509 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.timing.getClass().getName()+" was encountered"); 510 return (Reference) this.timing; 511 } 512 513 public boolean hasTimingReference() { 514 return this != null && this.timing instanceof Reference; 515 } 516 517 /** 518 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 519 */ 520 public DateType getTimingDateType() throws FHIRException { 521 if (this.timing == null) 522 this.timing = new DateType(); 523 if (!(this.timing instanceof DateType)) 524 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 525 return (DateType) this.timing; 526 } 527 528 public boolean hasTimingDateType() { 529 return this != null && this.timing instanceof DateType; 530 } 531 532 /** 533 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 534 */ 535 public DateTimeType getTimingDateTimeType() throws FHIRException { 536 if (this.timing == null) 537 this.timing = new DateTimeType(); 538 if (!(this.timing instanceof DateTimeType)) 539 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 540 return (DateTimeType) this.timing; 541 } 542 543 public boolean hasTimingDateTimeType() { 544 return this != null && this.timing instanceof DateTimeType; 545 } 546 547 public boolean hasTiming() { 548 return this.timing != null && !this.timing.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #timing} (The timing of the event (if this is a periodic trigger).) 553 */ 554 public TriggerDefinition setTiming(DataType value) { 555 if (value != null && !(value instanceof Timing || value instanceof Reference || value instanceof DateType || value instanceof DateTimeType)) 556 throw new FHIRException("Not the right type for TriggerDefinition.timing[x]: "+value.fhirType()); 557 this.timing = value; 558 return this; 559 } 560 561 /** 562 * @return {@link #data} (The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.) 563 */ 564 public List<DataRequirement> getData() { 565 if (this.data == null) 566 this.data = new ArrayList<DataRequirement>(); 567 return this.data; 568 } 569 570 /** 571 * @return Returns a reference to <code>this</code> for easy method chaining 572 */ 573 public TriggerDefinition setData(List<DataRequirement> theData) { 574 this.data = theData; 575 return this; 576 } 577 578 public boolean hasData() { 579 if (this.data == null) 580 return false; 581 for (DataRequirement item : this.data) 582 if (!item.isEmpty()) 583 return true; 584 return false; 585 } 586 587 public DataRequirement addData() { //3 588 DataRequirement t = new DataRequirement(); 589 if (this.data == null) 590 this.data = new ArrayList<DataRequirement>(); 591 this.data.add(t); 592 return t; 593 } 594 595 public TriggerDefinition addData(DataRequirement t) { //3 596 if (t == null) 597 return this; 598 if (this.data == null) 599 this.data = new ArrayList<DataRequirement>(); 600 this.data.add(t); 601 return this; 602 } 603 604 /** 605 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist {3} 606 */ 607 public DataRequirement getDataFirstRep() { 608 if (getData().isEmpty()) { 609 addData(); 610 } 611 return getData().get(0); 612 } 613 614 /** 615 * @return {@link #condition} (A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.) 616 */ 617 public Expression getCondition() { 618 if (this.condition == null) 619 if (Configuration.errorOnAutoCreate()) 620 throw new Error("Attempt to auto-create TriggerDefinition.condition"); 621 else if (Configuration.doAutoCreate()) 622 this.condition = new Expression(); // cc 623 return this.condition; 624 } 625 626 public boolean hasCondition() { 627 return this.condition != null && !this.condition.isEmpty(); 628 } 629 630 /** 631 * @param value {@link #condition} (A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.) 632 */ 633 public TriggerDefinition setCondition(Expression value) { 634 this.condition = value; 635 return this; 636 } 637 638 protected void listChildren(List<Property> children) { 639 super.listChildren(children); 640 children.add(new Property("type", "code", "The type of triggering event.", 0, 1, type)); 641 children.add(new Property("name", "string", "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 0, 1, name)); 642 children.add(new Property("code", "CodeableConcept", "A code that identifies the event.", 0, 1, code)); 643 children.add(new Property("subscriptionTopic", "canonical(SubscriptionTopic)", "A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.", 0, 1, subscriptionTopic)); 644 children.add(new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing)); 645 children.add(new Property("data", "DataRequirement", "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 0, java.lang.Integer.MAX_VALUE, data)); 646 children.add(new Property("condition", "Expression", "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 0, 1, condition)); 647 } 648 649 @Override 650 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 651 switch (_hash) { 652 case 3575610: /*type*/ return new Property("type", "code", "The type of triggering event.", 0, 1, type); 653 case 3373707: /*name*/ return new Property("name", "string", "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 0, 1, name); 654 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the event.", 0, 1, code); 655 case 1191816722: /*subscriptionTopic*/ return new Property("subscriptionTopic", "canonical(SubscriptionTopic)", "A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.", 0, 1, subscriptionTopic); 656 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 657 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 658 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 659 case -1792466399: /*timingReference*/ return new Property("timing[x]", "Reference(Schedule)", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 660 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 661 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 662 case 3076010: /*data*/ return new Property("data", "DataRequirement", "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 0, java.lang.Integer.MAX_VALUE, data); 663 case -861311717: /*condition*/ return new Property("condition", "Expression", "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 0, 1, condition); 664 default: return super.getNamedProperty(_hash, _name, _checkValid); 665 } 666 667 } 668 669 @Override 670 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 671 switch (hash) { 672 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TriggerType> 673 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 674 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 675 case 1191816722: /*subscriptionTopic*/ return this.subscriptionTopic == null ? new Base[0] : new Base[] {this.subscriptionTopic}; // CanonicalType 676 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 677 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // DataRequirement 678 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Expression 679 default: return super.getProperty(hash, name, checkValid); 680 } 681 682 } 683 684 @Override 685 public Base setProperty(int hash, String name, Base value) throws FHIRException { 686 switch (hash) { 687 case 3575610: // type 688 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 689 this.type = (Enumeration) value; // Enumeration<TriggerType> 690 return value; 691 case 3373707: // name 692 this.name = TypeConvertor.castToString(value); // StringType 693 return value; 694 case 3059181: // code 695 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 696 return value; 697 case 1191816722: // subscriptionTopic 698 this.subscriptionTopic = TypeConvertor.castToCanonical(value); // CanonicalType 699 return value; 700 case -873664438: // timing 701 this.timing = TypeConvertor.castToType(value); // DataType 702 return value; 703 case 3076010: // data 704 this.getData().add(TypeConvertor.castToDataRequirement(value)); // DataRequirement 705 return value; 706 case -861311717: // condition 707 this.condition = TypeConvertor.castToExpression(value); // Expression 708 return value; 709 default: return super.setProperty(hash, name, value); 710 } 711 712 } 713 714 @Override 715 public Base setProperty(String name, Base value) throws FHIRException { 716 if (name.equals("type")) { 717 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 718 this.type = (Enumeration) value; // Enumeration<TriggerType> 719 } else if (name.equals("name")) { 720 this.name = TypeConvertor.castToString(value); // StringType 721 } else if (name.equals("code")) { 722 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 723 } else if (name.equals("subscriptionTopic")) { 724 this.subscriptionTopic = TypeConvertor.castToCanonical(value); // CanonicalType 725 } else if (name.equals("timing[x]")) { 726 this.timing = TypeConvertor.castToType(value); // DataType 727 } else if (name.equals("data")) { 728 this.getData().add(TypeConvertor.castToDataRequirement(value)); 729 } else if (name.equals("condition")) { 730 this.condition = TypeConvertor.castToExpression(value); // Expression 731 } else 732 return super.setProperty(name, value); 733 return value; 734 } 735 736 @Override 737 public void removeChild(String name, Base value) throws FHIRException { 738 if (name.equals("type")) { 739 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 740 this.type = (Enumeration) value; // Enumeration<TriggerType> 741 } else if (name.equals("name")) { 742 this.name = null; 743 } else if (name.equals("code")) { 744 this.code = null; 745 } else if (name.equals("subscriptionTopic")) { 746 this.subscriptionTopic = null; 747 } else if (name.equals("timing[x]")) { 748 this.timing = null; 749 } else if (name.equals("data")) { 750 this.getData().remove(value); 751 } else if (name.equals("condition")) { 752 this.condition = null; 753 } else 754 super.removeChild(name, value); 755 756 } 757 758 @Override 759 public Base makeProperty(int hash, String name) throws FHIRException { 760 switch (hash) { 761 case 3575610: return getTypeElement(); 762 case 3373707: return getNameElement(); 763 case 3059181: return getCode(); 764 case 1191816722: return getSubscriptionTopicElement(); 765 case 164632566: return getTiming(); 766 case -873664438: return getTiming(); 767 case 3076010: return addData(); 768 case -861311717: return getCondition(); 769 default: return super.makeProperty(hash, name); 770 } 771 772 } 773 774 @Override 775 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 776 switch (hash) { 777 case 3575610: /*type*/ return new String[] {"code"}; 778 case 3373707: /*name*/ return new String[] {"string"}; 779 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 780 case 1191816722: /*subscriptionTopic*/ return new String[] {"canonical"}; 781 case -873664438: /*timing*/ return new String[] {"Timing", "Reference", "date", "dateTime"}; 782 case 3076010: /*data*/ return new String[] {"DataRequirement"}; 783 case -861311717: /*condition*/ return new String[] {"Expression"}; 784 default: return super.getTypesForProperty(hash, name); 785 } 786 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("type")) { 792 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.type"); 793 } 794 else if (name.equals("name")) { 795 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.name"); 796 } 797 else if (name.equals("code")) { 798 this.code = new CodeableConcept(); 799 return this.code; 800 } 801 else if (name.equals("subscriptionTopic")) { 802 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.subscriptionTopic"); 803 } 804 else if (name.equals("timingTiming")) { 805 this.timing = new Timing(); 806 return this.timing; 807 } 808 else if (name.equals("timingReference")) { 809 this.timing = new Reference(); 810 return this.timing; 811 } 812 else if (name.equals("timingDate")) { 813 this.timing = new DateType(); 814 return this.timing; 815 } 816 else if (name.equals("timingDateTime")) { 817 this.timing = new DateTimeType(); 818 return this.timing; 819 } 820 else if (name.equals("data")) { 821 return addData(); 822 } 823 else if (name.equals("condition")) { 824 this.condition = new Expression(); 825 return this.condition; 826 } 827 else 828 return super.addChild(name); 829 } 830 831 public String fhirType() { 832 return "TriggerDefinition"; 833 834 } 835 836 public TriggerDefinition copy() { 837 TriggerDefinition dst = new TriggerDefinition(); 838 copyValues(dst); 839 return dst; 840 } 841 842 public void copyValues(TriggerDefinition dst) { 843 super.copyValues(dst); 844 dst.type = type == null ? null : type.copy(); 845 dst.name = name == null ? null : name.copy(); 846 dst.code = code == null ? null : code.copy(); 847 dst.subscriptionTopic = subscriptionTopic == null ? null : subscriptionTopic.copy(); 848 dst.timing = timing == null ? null : timing.copy(); 849 if (data != null) { 850 dst.data = new ArrayList<DataRequirement>(); 851 for (DataRequirement i : data) 852 dst.data.add(i.copy()); 853 }; 854 dst.condition = condition == null ? null : condition.copy(); 855 } 856 857 protected TriggerDefinition typedCopy() { 858 return copy(); 859 } 860 861 @Override 862 public boolean equalsDeep(Base other_) { 863 if (!super.equalsDeep(other_)) 864 return false; 865 if (!(other_ instanceof TriggerDefinition)) 866 return false; 867 TriggerDefinition o = (TriggerDefinition) other_; 868 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(code, o.code, true) 869 && compareDeep(subscriptionTopic, o.subscriptionTopic, true) && compareDeep(timing, o.timing, true) 870 && compareDeep(data, o.data, true) && compareDeep(condition, o.condition, true); 871 } 872 873 @Override 874 public boolean equalsShallow(Base other_) { 875 if (!super.equalsShallow(other_)) 876 return false; 877 if (!(other_ instanceof TriggerDefinition)) 878 return false; 879 TriggerDefinition o = (TriggerDefinition) other_; 880 return compareValues(type, o.type, true) && compareValues(name, o.name, true) && compareValues(subscriptionTopic, o.subscriptionTopic, true) 881 ; 882 } 883 884 public boolean isEmpty() { 885 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, code, subscriptionTopic 886 , timing, data, condition); 887 } 888 889 890} 891