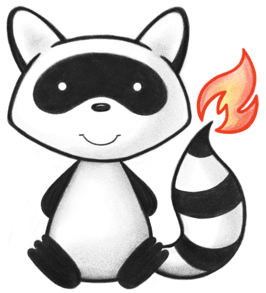
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * TriggerDefinition Type: A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element. 050 */ 051@DatatypeDef(name="TriggerDefinition") 052public class TriggerDefinition extends DataType implements ICompositeType { 053 054 public enum TriggerType { 055 /** 056 * The trigger occurs in response to a specific named event, and no other information about the trigger is specified. Named events are completely pre-coordinated, and the formal semantics of the trigger are not provided. 057 */ 058 NAMEDEVENT, 059 /** 060 * The trigger occurs at a specific time or periodically as described by a timing or schedule. A periodic event cannot have any data elements, but may have a name assigned as a shorthand for the event. 061 */ 062 PERIODIC, 063 /** 064 * The trigger occurs whenever data of a particular type is changed in any way, either added, modified, or removed. 065 */ 066 DATACHANGED, 067 /** 068 * The trigger occurs whenever data of a particular type is added. 069 */ 070 DATAADDED, 071 /** 072 * The trigger occurs whenever data of a particular type is modified. 073 */ 074 DATAMODIFIED, 075 /** 076 * The trigger occurs whenever data of a particular type is removed. 077 */ 078 DATAREMOVED, 079 /** 080 * The trigger occurs whenever data of a particular type is accessed. 081 */ 082 DATAACCESSED, 083 /** 084 * The trigger occurs whenever access to data of a particular type is completed. 085 */ 086 DATAACCESSENDED, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static TriggerType fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("named-event".equals(codeString)) 095 return NAMEDEVENT; 096 if ("periodic".equals(codeString)) 097 return PERIODIC; 098 if ("data-changed".equals(codeString)) 099 return DATACHANGED; 100 if ("data-added".equals(codeString)) 101 return DATAADDED; 102 if ("data-modified".equals(codeString)) 103 return DATAMODIFIED; 104 if ("data-removed".equals(codeString)) 105 return DATAREMOVED; 106 if ("data-accessed".equals(codeString)) 107 return DATAACCESSED; 108 if ("data-access-ended".equals(codeString)) 109 return DATAACCESSENDED; 110 if (Configuration.isAcceptInvalidEnums()) 111 return null; 112 else 113 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 114 } 115 public String toCode() { 116 switch (this) { 117 case NAMEDEVENT: return "named-event"; 118 case PERIODIC: return "periodic"; 119 case DATACHANGED: return "data-changed"; 120 case DATAADDED: return "data-added"; 121 case DATAMODIFIED: return "data-modified"; 122 case DATAREMOVED: return "data-removed"; 123 case DATAACCESSED: return "data-accessed"; 124 case DATAACCESSENDED: return "data-access-ended"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getSystem() { 130 switch (this) { 131 case NAMEDEVENT: return "http://hl7.org/fhir/trigger-type"; 132 case PERIODIC: return "http://hl7.org/fhir/trigger-type"; 133 case DATACHANGED: return "http://hl7.org/fhir/trigger-type"; 134 case DATAADDED: return "http://hl7.org/fhir/trigger-type"; 135 case DATAMODIFIED: return "http://hl7.org/fhir/trigger-type"; 136 case DATAREMOVED: return "http://hl7.org/fhir/trigger-type"; 137 case DATAACCESSED: return "http://hl7.org/fhir/trigger-type"; 138 case DATAACCESSENDED: return "http://hl7.org/fhir/trigger-type"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case NAMEDEVENT: return "The trigger occurs in response to a specific named event, and no other information about the trigger is specified. Named events are completely pre-coordinated, and the formal semantics of the trigger are not provided."; 146 case PERIODIC: return "The trigger occurs at a specific time or periodically as described by a timing or schedule. A periodic event cannot have any data elements, but may have a name assigned as a shorthand for the event."; 147 case DATACHANGED: return "The trigger occurs whenever data of a particular type is changed in any way, either added, modified, or removed."; 148 case DATAADDED: return "The trigger occurs whenever data of a particular type is added."; 149 case DATAMODIFIED: return "The trigger occurs whenever data of a particular type is modified."; 150 case DATAREMOVED: return "The trigger occurs whenever data of a particular type is removed."; 151 case DATAACCESSED: return "The trigger occurs whenever data of a particular type is accessed."; 152 case DATAACCESSENDED: return "The trigger occurs whenever access to data of a particular type is completed."; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getDisplay() { 158 switch (this) { 159 case NAMEDEVENT: return "Named Event"; 160 case PERIODIC: return "Periodic"; 161 case DATACHANGED: return "Data Changed"; 162 case DATAADDED: return "Data Added"; 163 case DATAMODIFIED: return "Data Updated"; 164 case DATAREMOVED: return "Data Removed"; 165 case DATAACCESSED: return "Data Accessed"; 166 case DATAACCESSENDED: return "Data Access Ended"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 } 172 173 public static class TriggerTypeEnumFactory implements EnumFactory<TriggerType> { 174 public TriggerType fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("named-event".equals(codeString)) 179 return TriggerType.NAMEDEVENT; 180 if ("periodic".equals(codeString)) 181 return TriggerType.PERIODIC; 182 if ("data-changed".equals(codeString)) 183 return TriggerType.DATACHANGED; 184 if ("data-added".equals(codeString)) 185 return TriggerType.DATAADDED; 186 if ("data-modified".equals(codeString)) 187 return TriggerType.DATAMODIFIED; 188 if ("data-removed".equals(codeString)) 189 return TriggerType.DATAREMOVED; 190 if ("data-accessed".equals(codeString)) 191 return TriggerType.DATAACCESSED; 192 if ("data-access-ended".equals(codeString)) 193 return TriggerType.DATAACCESSENDED; 194 throw new IllegalArgumentException("Unknown TriggerType code '"+codeString+"'"); 195 } 196 public Enumeration<TriggerType> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 201 String codeString = ((PrimitiveType) code).asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 204 if ("named-event".equals(codeString)) 205 return new Enumeration<TriggerType>(this, TriggerType.NAMEDEVENT, code); 206 if ("periodic".equals(codeString)) 207 return new Enumeration<TriggerType>(this, TriggerType.PERIODIC, code); 208 if ("data-changed".equals(codeString)) 209 return new Enumeration<TriggerType>(this, TriggerType.DATACHANGED, code); 210 if ("data-added".equals(codeString)) 211 return new Enumeration<TriggerType>(this, TriggerType.DATAADDED, code); 212 if ("data-modified".equals(codeString)) 213 return new Enumeration<TriggerType>(this, TriggerType.DATAMODIFIED, code); 214 if ("data-removed".equals(codeString)) 215 return new Enumeration<TriggerType>(this, TriggerType.DATAREMOVED, code); 216 if ("data-accessed".equals(codeString)) 217 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSED, code); 218 if ("data-access-ended".equals(codeString)) 219 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSENDED, code); 220 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 221 } 222 public String toCode(TriggerType code) { 223 if (code == TriggerType.NULL) 224 return null; 225 if (code == TriggerType.NAMEDEVENT) 226 return "named-event"; 227 if (code == TriggerType.PERIODIC) 228 return "periodic"; 229 if (code == TriggerType.DATACHANGED) 230 return "data-changed"; 231 if (code == TriggerType.DATAADDED) 232 return "data-added"; 233 if (code == TriggerType.DATAMODIFIED) 234 return "data-modified"; 235 if (code == TriggerType.DATAREMOVED) 236 return "data-removed"; 237 if (code == TriggerType.DATAACCESSED) 238 return "data-accessed"; 239 if (code == TriggerType.DATAACCESSENDED) 240 return "data-access-ended"; 241 return "?"; 242 } 243 public String toSystem(TriggerType code) { 244 return code.getSystem(); 245 } 246 } 247 248 /** 249 * The type of triggering event. 250 */ 251 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 252 @Description(shortDefinition="named-event | periodic | data-changed | data-added | data-modified | data-removed | data-accessed | data-access-ended", formalDefinition="The type of triggering event." ) 253 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/trigger-type") 254 protected Enumeration<TriggerType> type; 255 256 /** 257 * A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 258 */ 259 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 260 @Description(shortDefinition="Name or URI that identifies the event", formalDefinition="A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context." ) 261 protected StringType name; 262 263 /** 264 * A code that identifies the event. 265 */ 266 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 267 @Description(shortDefinition="Coded definition of the event", formalDefinition="A code that identifies the event." ) 268 protected CodeableConcept code; 269 270 /** 271 * A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 272 */ 273 @Child(name = "subscriptionTopic", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 274 @Description(shortDefinition="What event", formalDefinition="A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied." ) 275 protected CanonicalType subscriptionTopic; 276 277 /** 278 * The timing of the event (if this is a periodic trigger). 279 */ 280 @Child(name = "timing", type = {Timing.class, Schedule.class, DateType.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 281 @Description(shortDefinition="Timing of the event", formalDefinition="The timing of the event (if this is a periodic trigger)." ) 282 protected DataType timing; 283 284 /** 285 * The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true. 286 */ 287 @Child(name = "data", type = {DataRequirement.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 288 @Description(shortDefinition="Triggering data of the event (multiple = 'and')", formalDefinition="The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true." ) 289 protected List<DataRequirement> data; 290 291 /** 292 * A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires. 293 */ 294 @Child(name = "condition", type = {Expression.class}, order=6, min=0, max=1, modifier=false, summary=true) 295 @Description(shortDefinition="Whether the event triggers (boolean expression)", formalDefinition="A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires." ) 296 protected Expression condition; 297 298 private static final long serialVersionUID = -1823040479L; 299 300 /** 301 * Constructor 302 */ 303 public TriggerDefinition() { 304 super(); 305 } 306 307 /** 308 * Constructor 309 */ 310 public TriggerDefinition(TriggerType type) { 311 super(); 312 this.setType(type); 313 } 314 315 /** 316 * @return {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 317 */ 318 public Enumeration<TriggerType> getTypeElement() { 319 if (this.type == null) 320 if (Configuration.errorOnAutoCreate()) 321 throw new Error("Attempt to auto-create TriggerDefinition.type"); 322 else if (Configuration.doAutoCreate()) 323 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); // bb 324 return this.type; 325 } 326 327 public boolean hasTypeElement() { 328 return this.type != null && !this.type.isEmpty(); 329 } 330 331 public boolean hasType() { 332 return this.type != null && !this.type.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 337 */ 338 public TriggerDefinition setTypeElement(Enumeration<TriggerType> value) { 339 this.type = value; 340 return this; 341 } 342 343 /** 344 * @return The type of triggering event. 345 */ 346 public TriggerType getType() { 347 return this.type == null ? null : this.type.getValue(); 348 } 349 350 /** 351 * @param value The type of triggering event. 352 */ 353 public TriggerDefinition setType(TriggerType value) { 354 if (this.type == null) 355 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); 356 this.type.setValue(value); 357 return this; 358 } 359 360 /** 361 * @return {@link #name} (A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 362 */ 363 public StringType getNameElement() { 364 if (this.name == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create TriggerDefinition.name"); 367 else if (Configuration.doAutoCreate()) 368 this.name = new StringType(); // bb 369 return this.name; 370 } 371 372 public boolean hasNameElement() { 373 return this.name != null && !this.name.isEmpty(); 374 } 375 376 public boolean hasName() { 377 return this.name != null && !this.name.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #name} (A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 382 */ 383 public TriggerDefinition setNameElement(StringType value) { 384 this.name = value; 385 return this; 386 } 387 388 /** 389 * @return A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 390 */ 391 public String getName() { 392 return this.name == null ? null : this.name.getValue(); 393 } 394 395 /** 396 * @param value A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context. 397 */ 398 public TriggerDefinition setName(String value) { 399 if (Utilities.noString(value)) 400 this.name = null; 401 else { 402 if (this.name == null) 403 this.name = new StringType(); 404 this.name.setValue(value); 405 } 406 return this; 407 } 408 409 /** 410 * @return {@link #code} (A code that identifies the event.) 411 */ 412 public CodeableConcept getCode() { 413 if (this.code == null) 414 if (Configuration.errorOnAutoCreate()) 415 throw new Error("Attempt to auto-create TriggerDefinition.code"); 416 else if (Configuration.doAutoCreate()) 417 this.code = new CodeableConcept(); // cc 418 return this.code; 419 } 420 421 public boolean hasCode() { 422 return this.code != null && !this.code.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #code} (A code that identifies the event.) 427 */ 428 public TriggerDefinition setCode(CodeableConcept value) { 429 this.code = value; 430 return this; 431 } 432 433 /** 434 * @return {@link #subscriptionTopic} (A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.). This is the underlying object with id, value and extensions. The accessor "getSubscriptionTopic" gives direct access to the value 435 */ 436 public CanonicalType getSubscriptionTopicElement() { 437 if (this.subscriptionTopic == null) 438 if (Configuration.errorOnAutoCreate()) 439 throw new Error("Attempt to auto-create TriggerDefinition.subscriptionTopic"); 440 else if (Configuration.doAutoCreate()) 441 this.subscriptionTopic = new CanonicalType(); // bb 442 return this.subscriptionTopic; 443 } 444 445 public boolean hasSubscriptionTopicElement() { 446 return this.subscriptionTopic != null && !this.subscriptionTopic.isEmpty(); 447 } 448 449 public boolean hasSubscriptionTopic() { 450 return this.subscriptionTopic != null && !this.subscriptionTopic.isEmpty(); 451 } 452 453 /** 454 * @param value {@link #subscriptionTopic} (A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.). This is the underlying object with id, value and extensions. The accessor "getSubscriptionTopic" gives direct access to the value 455 */ 456 public TriggerDefinition setSubscriptionTopicElement(CanonicalType value) { 457 this.subscriptionTopic = value; 458 return this; 459 } 460 461 /** 462 * @return A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 463 */ 464 public String getSubscriptionTopic() { 465 return this.subscriptionTopic == null ? null : this.subscriptionTopic.getValue(); 466 } 467 468 /** 469 * @param value A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied. 470 */ 471 public TriggerDefinition setSubscriptionTopic(String value) { 472 if (Utilities.noString(value)) 473 this.subscriptionTopic = null; 474 else { 475 if (this.subscriptionTopic == null) 476 this.subscriptionTopic = new CanonicalType(); 477 this.subscriptionTopic.setValue(value); 478 } 479 return this; 480 } 481 482 /** 483 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 484 */ 485 public DataType getTiming() { 486 return this.timing; 487 } 488 489 /** 490 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 491 */ 492 public Timing getTimingTiming() throws FHIRException { 493 if (this.timing == null) 494 this.timing = new Timing(); 495 if (!(this.timing instanceof Timing)) 496 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 497 return (Timing) this.timing; 498 } 499 500 public boolean hasTimingTiming() { 501 return this != null && this.timing instanceof Timing; 502 } 503 504 /** 505 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 506 */ 507 public Reference getTimingReference() throws FHIRException { 508 if (this.timing == null) 509 this.timing = new Reference(); 510 if (!(this.timing instanceof Reference)) 511 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.timing.getClass().getName()+" was encountered"); 512 return (Reference) this.timing; 513 } 514 515 public boolean hasTimingReference() { 516 return this != null && this.timing instanceof Reference; 517 } 518 519 /** 520 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 521 */ 522 public DateType getTimingDateType() throws FHIRException { 523 if (this.timing == null) 524 this.timing = new DateType(); 525 if (!(this.timing instanceof DateType)) 526 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 527 return (DateType) this.timing; 528 } 529 530 public boolean hasTimingDateType() { 531 return this != null && this.timing instanceof DateType; 532 } 533 534 /** 535 * @return {@link #timing} (The timing of the event (if this is a periodic trigger).) 536 */ 537 public DateTimeType getTimingDateTimeType() throws FHIRException { 538 if (this.timing == null) 539 this.timing = new DateTimeType(); 540 if (!(this.timing instanceof DateTimeType)) 541 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 542 return (DateTimeType) this.timing; 543 } 544 545 public boolean hasTimingDateTimeType() { 546 return this != null && this.timing instanceof DateTimeType; 547 } 548 549 public boolean hasTiming() { 550 return this.timing != null && !this.timing.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #timing} (The timing of the event (if this is a periodic trigger).) 555 */ 556 public TriggerDefinition setTiming(DataType value) { 557 if (value != null && !(value instanceof Timing || value instanceof Reference || value instanceof DateType || value instanceof DateTimeType)) 558 throw new FHIRException("Not the right type for TriggerDefinition.timing[x]: "+value.fhirType()); 559 this.timing = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #data} (The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.) 565 */ 566 public List<DataRequirement> getData() { 567 if (this.data == null) 568 this.data = new ArrayList<DataRequirement>(); 569 return this.data; 570 } 571 572 /** 573 * @return Returns a reference to <code>this</code> for easy method chaining 574 */ 575 public TriggerDefinition setData(List<DataRequirement> theData) { 576 this.data = theData; 577 return this; 578 } 579 580 public boolean hasData() { 581 if (this.data == null) 582 return false; 583 for (DataRequirement item : this.data) 584 if (!item.isEmpty()) 585 return true; 586 return false; 587 } 588 589 public DataRequirement addData() { //3 590 DataRequirement t = new DataRequirement(); 591 if (this.data == null) 592 this.data = new ArrayList<DataRequirement>(); 593 this.data.add(t); 594 return t; 595 } 596 597 public TriggerDefinition addData(DataRequirement t) { //3 598 if (t == null) 599 return this; 600 if (this.data == null) 601 this.data = new ArrayList<DataRequirement>(); 602 this.data.add(t); 603 return this; 604 } 605 606 /** 607 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist {3} 608 */ 609 public DataRequirement getDataFirstRep() { 610 if (getData().isEmpty()) { 611 addData(); 612 } 613 return getData().get(0); 614 } 615 616 /** 617 * @return {@link #condition} (A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.) 618 */ 619 public Expression getCondition() { 620 if (this.condition == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create TriggerDefinition.condition"); 623 else if (Configuration.doAutoCreate()) 624 this.condition = new Expression(); // cc 625 return this.condition; 626 } 627 628 public boolean hasCondition() { 629 return this.condition != null && !this.condition.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #condition} (A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.) 634 */ 635 public TriggerDefinition setCondition(Expression value) { 636 this.condition = value; 637 return this; 638 } 639 640 protected void listChildren(List<Property> children) { 641 super.listChildren(children); 642 children.add(new Property("type", "code", "The type of triggering event.", 0, 1, type)); 643 children.add(new Property("name", "string", "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 0, 1, name)); 644 children.add(new Property("code", "CodeableConcept", "A code that identifies the event.", 0, 1, code)); 645 children.add(new Property("subscriptionTopic", "canonical(SubscriptionTopic)", "A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.", 0, 1, subscriptionTopic)); 646 children.add(new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing)); 647 children.add(new Property("data", "DataRequirement", "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 0, java.lang.Integer.MAX_VALUE, data)); 648 children.add(new Property("condition", "Expression", "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 0, 1, condition)); 649 } 650 651 @Override 652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 653 switch (_hash) { 654 case 3575610: /*type*/ return new Property("type", "code", "The type of triggering event.", 0, 1, type); 655 case 3373707: /*name*/ return new Property("name", "string", "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 0, 1, name); 656 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the event.", 0, 1, code); 657 case 1191816722: /*subscriptionTopic*/ return new Property("subscriptionTopic", "canonical(SubscriptionTopic)", "A reference to a SubscriptionTopic resource that defines the event. If this element is provided, no other information about the trigger definition may be supplied.", 0, 1, subscriptionTopic); 658 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 659 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 660 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 661 case -1792466399: /*timingReference*/ return new Property("timing[x]", "Reference(Schedule)", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 662 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 663 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime", "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 664 case 3076010: /*data*/ return new Property("data", "DataRequirement", "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 0, java.lang.Integer.MAX_VALUE, data); 665 case -861311717: /*condition*/ return new Property("condition", "Expression", "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 0, 1, condition); 666 default: return super.getNamedProperty(_hash, _name, _checkValid); 667 } 668 669 } 670 671 @Override 672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 673 switch (hash) { 674 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TriggerType> 675 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 676 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 677 case 1191816722: /*subscriptionTopic*/ return this.subscriptionTopic == null ? new Base[0] : new Base[] {this.subscriptionTopic}; // CanonicalType 678 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 679 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // DataRequirement 680 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Expression 681 default: return super.getProperty(hash, name, checkValid); 682 } 683 684 } 685 686 @Override 687 public Base setProperty(int hash, String name, Base value) throws FHIRException { 688 switch (hash) { 689 case 3575610: // type 690 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 691 this.type = (Enumeration) value; // Enumeration<TriggerType> 692 return value; 693 case 3373707: // name 694 this.name = TypeConvertor.castToString(value); // StringType 695 return value; 696 case 3059181: // code 697 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 698 return value; 699 case 1191816722: // subscriptionTopic 700 this.subscriptionTopic = TypeConvertor.castToCanonical(value); // CanonicalType 701 return value; 702 case -873664438: // timing 703 this.timing = TypeConvertor.castToType(value); // DataType 704 return value; 705 case 3076010: // data 706 this.getData().add(TypeConvertor.castToDataRequirement(value)); // DataRequirement 707 return value; 708 case -861311717: // condition 709 this.condition = TypeConvertor.castToExpression(value); // Expression 710 return value; 711 default: return super.setProperty(hash, name, value); 712 } 713 714 } 715 716 @Override 717 public Base setProperty(String name, Base value) throws FHIRException { 718 if (name.equals("type")) { 719 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 720 this.type = (Enumeration) value; // Enumeration<TriggerType> 721 } else if (name.equals("name")) { 722 this.name = TypeConvertor.castToString(value); // StringType 723 } else if (name.equals("code")) { 724 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 725 } else if (name.equals("subscriptionTopic")) { 726 this.subscriptionTopic = TypeConvertor.castToCanonical(value); // CanonicalType 727 } else if (name.equals("timing[x]")) { 728 this.timing = TypeConvertor.castToType(value); // DataType 729 } else if (name.equals("data")) { 730 this.getData().add(TypeConvertor.castToDataRequirement(value)); 731 } else if (name.equals("condition")) { 732 this.condition = TypeConvertor.castToExpression(value); // Expression 733 } else 734 return super.setProperty(name, value); 735 return value; 736 } 737 738 @Override 739 public void removeChild(String name, Base value) throws FHIRException { 740 if (name.equals("type")) { 741 value = new TriggerTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 742 this.type = (Enumeration) value; // Enumeration<TriggerType> 743 } else if (name.equals("name")) { 744 this.name = null; 745 } else if (name.equals("code")) { 746 this.code = null; 747 } else if (name.equals("subscriptionTopic")) { 748 this.subscriptionTopic = null; 749 } else if (name.equals("timing[x]")) { 750 this.timing = null; 751 } else if (name.equals("data")) { 752 this.getData().remove(value); 753 } else if (name.equals("condition")) { 754 this.condition = null; 755 } else 756 super.removeChild(name, value); 757 758 } 759 760 @Override 761 public Base makeProperty(int hash, String name) throws FHIRException { 762 switch (hash) { 763 case 3575610: return getTypeElement(); 764 case 3373707: return getNameElement(); 765 case 3059181: return getCode(); 766 case 1191816722: return getSubscriptionTopicElement(); 767 case 164632566: return getTiming(); 768 case -873664438: return getTiming(); 769 case 3076010: return addData(); 770 case -861311717: return getCondition(); 771 default: return super.makeProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 778 switch (hash) { 779 case 3575610: /*type*/ return new String[] {"code"}; 780 case 3373707: /*name*/ return new String[] {"string"}; 781 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 782 case 1191816722: /*subscriptionTopic*/ return new String[] {"canonical"}; 783 case -873664438: /*timing*/ return new String[] {"Timing", "Reference", "date", "dateTime"}; 784 case 3076010: /*data*/ return new String[] {"DataRequirement"}; 785 case -861311717: /*condition*/ return new String[] {"Expression"}; 786 default: return super.getTypesForProperty(hash, name); 787 } 788 789 } 790 791 @Override 792 public Base addChild(String name) throws FHIRException { 793 if (name.equals("type")) { 794 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.type"); 795 } 796 else if (name.equals("name")) { 797 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.name"); 798 } 799 else if (name.equals("code")) { 800 this.code = new CodeableConcept(); 801 return this.code; 802 } 803 else if (name.equals("subscriptionTopic")) { 804 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.subscriptionTopic"); 805 } 806 else if (name.equals("timingTiming")) { 807 this.timing = new Timing(); 808 return this.timing; 809 } 810 else if (name.equals("timingReference")) { 811 this.timing = new Reference(); 812 return this.timing; 813 } 814 else if (name.equals("timingDate")) { 815 this.timing = new DateType(); 816 return this.timing; 817 } 818 else if (name.equals("timingDateTime")) { 819 this.timing = new DateTimeType(); 820 return this.timing; 821 } 822 else if (name.equals("data")) { 823 return addData(); 824 } 825 else if (name.equals("condition")) { 826 this.condition = new Expression(); 827 return this.condition; 828 } 829 else 830 return super.addChild(name); 831 } 832 833 public String fhirType() { 834 return "TriggerDefinition"; 835 836 } 837 838 public TriggerDefinition copy() { 839 TriggerDefinition dst = new TriggerDefinition(); 840 copyValues(dst); 841 return dst; 842 } 843 844 public void copyValues(TriggerDefinition dst) { 845 super.copyValues(dst); 846 dst.type = type == null ? null : type.copy(); 847 dst.name = name == null ? null : name.copy(); 848 dst.code = code == null ? null : code.copy(); 849 dst.subscriptionTopic = subscriptionTopic == null ? null : subscriptionTopic.copy(); 850 dst.timing = timing == null ? null : timing.copy(); 851 if (data != null) { 852 dst.data = new ArrayList<DataRequirement>(); 853 for (DataRequirement i : data) 854 dst.data.add(i.copy()); 855 }; 856 dst.condition = condition == null ? null : condition.copy(); 857 } 858 859 protected TriggerDefinition typedCopy() { 860 return copy(); 861 } 862 863 @Override 864 public boolean equalsDeep(Base other_) { 865 if (!super.equalsDeep(other_)) 866 return false; 867 if (!(other_ instanceof TriggerDefinition)) 868 return false; 869 TriggerDefinition o = (TriggerDefinition) other_; 870 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(code, o.code, true) 871 && compareDeep(subscriptionTopic, o.subscriptionTopic, true) && compareDeep(timing, o.timing, true) 872 && compareDeep(data, o.data, true) && compareDeep(condition, o.condition, true); 873 } 874 875 @Override 876 public boolean equalsShallow(Base other_) { 877 if (!super.equalsShallow(other_)) 878 return false; 879 if (!(other_ instanceof TriggerDefinition)) 880 return false; 881 TriggerDefinition o = (TriggerDefinition) other_; 882 return compareValues(type, o.type, true) && compareValues(name, o.name, true) && compareValues(subscriptionTopic, o.subscriptionTopic, true) 883 ; 884 } 885 886 public boolean isEmpty() { 887 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, code, subscriptionTopic 888 , timing, data, condition); 889 } 890 891 892} 893