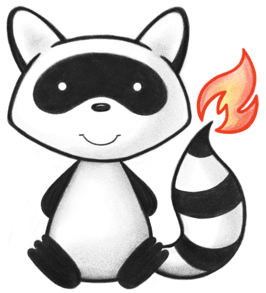
001package org.hl7.fhir.r5.model; 002 003import java.io.IOException; 004 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.elementmodel.Element; 007import org.hl7.fhir.r5.elementmodel.ObjectConverter; 008import org.hl7.fhir.utilities.Utilities; 009import org.hl7.fhir.utilities.xhtml.XhtmlComposer; 010import org.hl7.fhir.utilities.xhtml.XhtmlNode; 011import org.hl7.fhir.utilities.xhtml.XhtmlParser; 012 013public class TypeConvertor { 014 015 // -- converters for property setters 016 017 public static DataType castToType(Base b) throws FHIRException { 018 if (b == null) { 019 return null; 020 } 021 if (b instanceof DataType) 022 return (DataType) b; 023 else if (b.isMetadataBased()) 024 return ((org.hl7.fhir.r5.elementmodel.Element) b).asType(); 025 else 026 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Reference"); 027 } 028 029 030 public static BooleanType castToBoolean(Base b) throws FHIRException { 031 if (b == null) { 032 return null; 033 } 034 if (b instanceof BooleanType) 035 return (BooleanType) b; 036 else 037 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Boolean"); 038 } 039 040 public static IntegerType castToInteger(Base b) throws FHIRException { 041 if (b == null) { 042 return null; 043 } 044 if (b instanceof IntegerType) 045 return (IntegerType) b; 046 else 047 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Integer"); 048 } 049 050 public static Integer64Type castToInteger64(Base b) throws FHIRException { 051 if (b == null) { 052 return null; 053 } 054 if (b instanceof Integer64Type) 055 return (Integer64Type) b; 056 else 057 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Integer"); 058 } 059 060 public static DecimalType castToDecimal(Base b) throws FHIRException { 061 if (b == null) { 062 return null; 063 } 064 if (b instanceof DecimalType) 065 return (DecimalType) b; 066 else if (b.hasPrimitiveValue()) 067 return new DecimalType(b.primitiveValue()); 068 else 069 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Decimal"); 070 } 071 072 public static Base64BinaryType castToBase64Binary(Base b) throws FHIRException { 073 if (b == null) { 074 return null; 075 } 076 if (b instanceof Base64BinaryType) 077 return (Base64BinaryType) b; 078 else 079 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Base64Binary"); 080 } 081 082 public static InstantType castToInstant(Base b) throws FHIRException { 083 if (b == null) { 084 return null; 085 } 086 if (b instanceof InstantType) 087 return (InstantType) b; 088 else 089 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Instant"); 090 } 091 092 public static StringType castToString(Base b) throws FHIRException { 093 if (b == null) { 094 return null; 095 } 096 097 if (b instanceof StringType) 098 return (StringType) b; 099 else if (b.hasPrimitiveValue()) 100 return new StringType(b.primitiveValue()); 101 else 102 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a String"); 103 } 104 105 public static UriType castToUri(Base b) throws FHIRException { 106 if (b == null) { 107 return null; 108 } 109 110 if (b instanceof UriType) 111 return (UriType) b; 112 else if (b.hasPrimitiveValue()) 113 return new UriType(b.primitiveValue()); 114 else 115 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Uri"); 116 } 117 118 public static UrlType castToUrl(Base b) throws FHIRException { 119 if (b == null) { 120 return null; 121 } 122 123 if (b instanceof UrlType) 124 return (UrlType) b; 125 else if (b.hasPrimitiveValue()) 126 return new UrlType(b.primitiveValue()); 127 else 128 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Uri"); 129 } 130 131 public static CanonicalType castToCanonical(Base b) throws FHIRException { 132 if (b == null) { 133 return null; 134 } 135 136 if (b instanceof CanonicalType) 137 return (CanonicalType) b; 138 else if (b.hasPrimitiveValue()) 139 return new CanonicalType(b.primitiveValue()); 140 else 141 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Uri"); 142 } 143 144 public static DateType castToDate(Base b) throws FHIRException { 145 if (b == null) { 146 return null; 147 } 148 149 if (b instanceof DateType) 150 return (DateType) b; 151 else if (b.hasPrimitiveValue()) 152 return new DateType(b.primitiveValue()); 153 else 154 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Date"); 155 } 156 157 public static DateTimeType castToDateTime(Base b) throws FHIRException { 158 if (b == null) { 159 return null; 160 } 161 162 if (b instanceof DateTimeType) 163 return (DateTimeType) b; 164 else if (Utilities.existsInList(b.fhirType(), "dateTime", "date", "instant")) 165 return new DateTimeType(b.primitiveValue()); 166 else 167 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a DateTime"); 168 } 169 170 public static TimeType castToTime(Base b) throws FHIRException { 171 if (b == null) { 172 return null; 173 } 174 175 if (b instanceof TimeType) 176 return (TimeType) b; 177 else 178 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Time"); 179 } 180 181 public static CodeType castToCode(Base b) throws FHIRException { 182 if (b == null) { 183 return null; 184 } 185 186 if (b instanceof CodeType) 187 return (CodeType) b; 188 else if (b instanceof PrimitiveType<?>) { 189 return new CodeType(b.primitiveValue(), (PrimitiveType<?>) b); 190 } else if (b.isPrimitive()) 191 return new CodeType(b.primitiveValue()); 192 else 193 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Code"); 194 } 195 196 public static OidType castToOid(Base b) throws FHIRException { 197 if (b == null) { 198 return null; 199 } 200 201 if (b instanceof OidType) 202 return (OidType) b; 203 else 204 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Oid"); 205 } 206 207 public static IdType castToId(Base b) throws FHIRException { 208 if (b == null) { 209 return null; 210 } 211 212 if (b instanceof IdType) 213 return (IdType) b; 214 else 215 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Id"); 216 } 217 218 public static UnsignedIntType castToUnsignedInt(Base b) throws FHIRException { 219 if (b == null) { 220 return null; 221 } 222 223 if (b instanceof UnsignedIntType) 224 return (UnsignedIntType) b; 225 else 226 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a UnsignedInt"); 227 } 228 229 public static PositiveIntType castToPositiveInt(Base b) throws FHIRException { 230 if (b == null) { 231 return null; 232 } 233 234 if (b instanceof PositiveIntType) 235 return (PositiveIntType) b; 236 else 237 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a PositiveInt"); 238 } 239 240 public static MarkdownType castToMarkdown(Base b) throws FHIRException { 241 if (b == null) { 242 return null; 243 } 244 245 if (b instanceof MarkdownType) 246 return (MarkdownType) b; 247 else if (b.hasPrimitiveValue()) 248 return new MarkdownType(b.primitiveValue()); 249 else 250 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Markdown"); 251 } 252 253 public static Annotation castToAnnotation(Base b) throws FHIRException { 254 if (b == null) { 255 return null; 256 } 257 258 if (b instanceof Annotation) 259 return (Annotation) b; 260 else 261 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Annotation"); 262 } 263 264 public static Dosage castToDosage(Base b) throws FHIRException { 265 if (b == null) { 266 return null; 267 } 268 269 if (b instanceof Dosage) 270 return (Dosage) b; 271 else 272 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an DosageInstruction"); 273 } 274 275 276 public static Attachment castToAttachment(Base b) throws FHIRException { 277 if (b == null) { 278 return null; 279 } 280 281 if (b instanceof Attachment) 282 return (Attachment) b; 283 else 284 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Attachment"); 285 } 286 287 public static Identifier castToIdentifier(Base b) throws FHIRException { 288 if (b == null) { 289 return null; 290 } 291 292 if (b instanceof Identifier) 293 return (Identifier) b; 294 else 295 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Identifier"); 296 } 297 298 public static CodeableConcept castToCodeableConcept(Base b) throws FHIRException { 299 if (b == null) { 300 return null; 301 } 302 303 if (b instanceof CodeableConcept) 304 return (CodeableConcept) b; 305 else if (b instanceof Element) { 306 return ObjectConverter.readAsCodeableConcept((Element) b); 307 } else if (b instanceof CodeType) { 308 CodeableConcept cc = new CodeableConcept(); 309 cc.addCoding().setCode(((CodeType) b).asStringValue()); 310 return cc; 311 } else if(b instanceof StringType) { 312 CodeableConcept cc = new CodeableConcept(); 313 cc.addCoding().setCode(((StringType) b).asStringValue()); 314 return cc; 315 } else 316 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a CodeableConcept"); 317 } 318 319 public static CodeableReference castToCodeableReference(Base b) throws FHIRException { 320 if (b == null) { 321 return null; 322 } 323 324 if (b instanceof CodeableReference) { 325 return (CodeableReference) b; 326 } else if (b instanceof CodeType) { 327 CodeableReference cc = new CodeableReference(); 328 cc.getConcept().addCoding().setCode(((CodeType) b).asStringValue()); 329 return cc; 330 } else if (b instanceof Reference) { 331 CodeableReference cc = new CodeableReference(); 332 cc.setReference((Reference) b); 333 return cc; 334 } else if(b instanceof StringType) { 335 CodeableReference cc = new CodeableReference(); 336 cc.getConcept().addCoding().setCode(((StringType) b).asStringValue()); 337 return cc; 338 } else 339 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a CodeableConcept"); 340 } 341 342 public static Coding castToCoding(Base b) throws FHIRException { 343 if (b == null) { 344 return null; 345 } 346 347 if (b instanceof Coding) 348 return (Coding) b; 349 else if (b instanceof Element) { 350 ICoding c = ((Element) b).getAsICoding(); 351 if (c != null) { 352 return new Coding().setCode(c.getCode()).setSystem(c.getSystem()).setVersion(c.getVersion()).setDisplay(c.getDisplay()); 353 } else if (b instanceof PrimitiveType<?>) { 354 PrimitiveType<?> p = (PrimitiveType<?>) b; 355 Coding cc = new Coding(); 356 cc.setCode(b.primitiveValue()).setId(p.getId()).getExtension().addAll(p.getExtension()); 357 return cc; 358 } else if (b.isPrimitive()) { 359 return new Coding().setCode(b.primitiveValue()); 360 } else { 361 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Coding"); 362 } 363 } else if (b instanceof ICoding) { 364 ICoding c = (ICoding) b; 365 return new Coding().setCode(c.getCode()).setSystem(c.getSystem()).setVersion(c.getVersion()).setDisplay(c.getDisplay()); 366 } else if (b instanceof PrimitiveType<?>) { 367 PrimitiveType<?> p = (PrimitiveType<?>) b; 368 Coding cc = new Coding(); 369 cc.setCode(b.primitiveValue()).setId(p.getId()).getExtension().addAll(p.getExtension()); 370 return cc; 371 } else if (b.isPrimitive()) { 372 return new Coding().setCode(b.primitiveValue()); 373 } else { 374 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Coding"); 375 } 376 } 377 378 public static Quantity castToQuantity(Base b) throws FHIRException { 379 if (b == null) { 380 return null; 381 } 382 383 if (b instanceof Quantity) 384 return (Quantity) b; 385 else 386 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Quantity"); 387 } 388 389 public static Count castToCount(Base b) throws FHIRException { 390 if (b == null) { 391 return null; 392 } 393 394 if (b instanceof Count) 395 return (Count) b; 396 else 397 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Count"); 398 } 399 400 public static Money castToMoney(Base b) throws FHIRException { 401 if (b == null) { 402 return null; 403 } 404 405 if (b instanceof Money) 406 return (Money) b; 407 else if (b instanceof org.hl7.fhir.r5.elementmodel.Element && Utilities.tail(b.fhirType()).equals("Money")) { 408 org.hl7.fhir.r5.elementmodel.Element e = (org.hl7.fhir.r5.elementmodel.Element) b; 409 return new Money().setCurrency(e.getChildValue("currency")).setValue(Long.parseLong(e.getChildValue("value"))); 410 } else 411 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Money"); 412 } 413 414 public static Duration castToDuration(Base b) throws FHIRException { 415 if (b == null) { 416 return null; 417 } 418 419 if (b instanceof Duration) 420 return (Duration) b; 421 else 422 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an Duration"); 423 } 424 425 public static SimpleQuantity castToSimpleQuantity(Base b) throws FHIRException { 426 if (b == null) { 427 return null; 428 } 429 430 if (b instanceof SimpleQuantity) 431 return (SimpleQuantity) b; 432 else if (b instanceof Quantity) { 433 Quantity q = (Quantity) b; 434 SimpleQuantity sq = new SimpleQuantity(); 435 sq.setValueElement(q.getValueElement()); 436 sq.setComparatorElement(q.getComparatorElement()); 437 sq.setUnitElement(q.getUnitElement()); 438 sq.setSystemElement(q.getSystemElement()); 439 sq.setCodeElement(q.getCodeElement()); 440 return sq; 441 } else 442 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to an SimpleQuantity"); 443 } 444 445 public static Range castToRange(Base b) throws FHIRException { 446 if (b == null) { 447 return null; 448 } 449 450 if (b instanceof Range) 451 return (Range) b; 452 else 453 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Range"); 454 } 455 456 public static Period castToPeriod(Base b) throws FHIRException { 457 if (b == null) { 458 return null; 459 } 460 461 if (b instanceof Period) 462 return (Period) b; 463 else 464 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Period"); 465 } 466 467 public static Ratio castToRatio(Base b) throws FHIRException { 468 if (b == null) { 469 return null; 470 } 471 472 if (b instanceof Ratio) 473 return (Ratio) b; 474 else 475 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Ratio"); 476 } 477 478 public static SampledData castToSampledData(Base b) throws FHIRException { 479 if (b == null) { 480 return null; 481 } 482 483 if (b instanceof SampledData) 484 return (SampledData) b; 485 else 486 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a SampledData"); 487 } 488 489 public static Signature castToSignature(Base b) throws FHIRException { 490 if (b == null) { 491 return null; 492 } 493 494 if (b instanceof Signature) 495 return (Signature) b; 496 else 497 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Signature"); 498 } 499 500 public static HumanName castToHumanName(Base b) throws FHIRException { 501 if (b == null) { 502 return null; 503 } 504 505 if (b instanceof HumanName) 506 return (HumanName) b; 507 else 508 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a HumanName"); 509 } 510 511 public static Address castToAddress(Base b) throws FHIRException { 512 if (b == null) { 513 return null; 514 } 515 516 if (b instanceof Address) 517 return (Address) b; 518 else 519 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Address"); 520 } 521 522 public static ContactDetail castToContactDetail(Base b) throws FHIRException { 523 if (b == null) { 524 return null; 525 } 526 527 if (b instanceof ContactDetail) 528 return (ContactDetail) b; 529 else 530 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ContactDetail"); 531 } 532 533 public static Contributor castToContributor(Base b) throws FHIRException { 534 if (b == null) { 535 return null; 536 } 537 538 if (b instanceof Contributor) 539 return (Contributor) b; 540 else 541 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Contributor"); 542 } 543 544 public static UsageContext castToUsageContext(Base b) throws FHIRException { 545 if (b == null) { 546 return null; 547 } 548 549 if (b instanceof UsageContext) 550 return (UsageContext) b; 551 else 552 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a UsageContext"); 553 } 554 555 public static RelatedArtifact castToRelatedArtifact(Base b) throws FHIRException { 556 if (b == null) { 557 return null; 558 } 559 560 if (b instanceof RelatedArtifact) 561 return (RelatedArtifact) b; 562 else 563 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a RelatedArtifact"); 564 } 565 566 public static ContactPoint castToContactPoint(Base b) throws FHIRException { 567 if (b == null) { 568 return null; 569 } 570 571 if (b instanceof ContactPoint) 572 return (ContactPoint) b; 573 else 574 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ContactPoint"); 575 } 576 577 public static Timing castToTiming(Base b) throws FHIRException { 578 if (b == null) { 579 return null; 580 } 581 582 if (b instanceof Timing) 583 return (Timing) b; 584 else 585 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Timing"); 586 } 587 588 public static Reference castToReference(Base b) throws FHIRException { 589 if (b == null) { 590 return null; 591 } 592 593 if (b instanceof Reference) 594 return (Reference) b; 595 else if (b.isPrimitive() && Utilities.isURL(b.primitiveValue())) 596 return new Reference().setReference(b.primitiveValue()); 597 else if (b instanceof org.hl7.fhir.r5.elementmodel.Element && Utilities.tail(b.fhirType()).equals("Reference")) { 598 org.hl7.fhir.r5.elementmodel.Element e = (org.hl7.fhir.r5.elementmodel.Element) b; 599 return new Reference().setReference(e.getChildValue("reference")).setDisplay(e.getChildValue("display")); 600 } else 601 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Reference"); 602 } 603 604 public static Meta castToMeta(Base b) throws FHIRException { 605 if (b == null) { 606 return null; 607 } 608 609 if (b instanceof Meta) 610 return (Meta) b; 611 else 612 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Meta"); 613 } 614 615 616 public static MarketingStatus castToMarketingStatus(Base b) throws FHIRException { 617 if (b == null) { 618 return null; 619 } 620 621 if (b instanceof MarketingStatus) 622 return (MarketingStatus) b; 623 else 624 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a MarketingStatus"); 625 } 626 627 public static Statistic castToStatistic(Base b) throws FHIRException { 628 if (b == null) { 629 return null; 630 } 631 632 if (b instanceof Statistic) 633 return (Statistic) b; 634 else 635 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Statistic"); 636 } 637 638 639 public static OrderedDistribution castToOrderedDistribution(Base b) throws FHIRException { 640 if (b == null) { 641 return null; 642 } 643 644 if (b instanceof OrderedDistribution) 645 return (OrderedDistribution) b; 646 else 647 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a OrderedDistribution"); 648 } 649 650 public static ProductShelfLife castToProductShelfLife(Base b) throws FHIRException { 651 if (b == null) { 652 return null; 653 } 654 655 if (b instanceof ProductShelfLife) 656 return (ProductShelfLife) b; 657 else 658 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ProductShelfLife"); 659 } 660 661 public static ProdCharacteristic castToProdCharacteristic(Base b) throws FHIRException { 662 if (b == null) { 663 return null; 664 } 665 666 if (b instanceof ProdCharacteristic) 667 return (ProdCharacteristic) b; 668 else 669 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ProdCharacteristic"); 670 } 671 672 673 public static SubstanceAmount castToSubstanceAmount(Base b) throws FHIRException { 674 if (b == null) { 675 return null; 676 } 677 678 if (b instanceof SubstanceAmount) 679 return (SubstanceAmount) b; 680 else 681 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a SubstanceAmount"); 682 } 683 684 public static Extension castToExtension(Base b) throws FHIRException { 685 if (b == null) { 686 return null; 687 } 688 689 if (b instanceof Extension) 690 return (Extension) b; 691 else 692 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Extension"); 693 } 694 695 public static Resource castToResource(Base b) throws FHIRException { 696 if (b == null) { 697 return null; 698 } 699 700 if (b instanceof Resource) 701 return (Resource) b; 702 else 703 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Resource"); 704 } 705 706 public static Narrative castToNarrative(Base b) throws FHIRException { 707 if (b == null) { 708 return null; 709 } 710 711 if (b instanceof Narrative) 712 return (Narrative) b; 713 else 714 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Narrative"); 715 } 716 717 718 public static ElementDefinition castToElementDefinition(Base b) throws FHIRException { 719 if (b == null) { 720 return null; 721 } 722 723 if (b instanceof ElementDefinition) 724 return (ElementDefinition) b; 725 else 726 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ElementDefinition"); 727 } 728 729 public static DataRequirement castToDataRequirement(Base b) throws FHIRException { 730 if (b == null) { 731 return null; 732 } 733 734 if (b instanceof DataRequirement) 735 return (DataRequirement) b; 736 else 737 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a DataRequirement"); 738 } 739 740 public static Expression castToExpression(Base b) throws FHIRException { 741 if (b == null) { 742 return null; 743 } 744 745 if (b instanceof Expression) 746 return (Expression) b; 747 else 748 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Expression"); 749 } 750 751 752 public static ParameterDefinition castToParameterDefinition(Base b) throws FHIRException { 753 if (b == null) { 754 return null; 755 } 756 757 if (b instanceof ParameterDefinition) 758 return (ParameterDefinition) b; 759 else 760 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ParameterDefinition"); 761 } 762 763 public static TriggerDefinition castToTriggerDefinition(Base b) throws FHIRException { 764 if (b == null) { 765 return null; 766 } 767 768 if (b instanceof TriggerDefinition) 769 return (TriggerDefinition) b; 770 else 771 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a TriggerDefinition"); 772 } 773 774 public static ExtendedContactDetail castToExtendedContactDetail(Base b) throws FHIRException { 775 if (b == null) { 776 return null; 777 } 778 779 if (b instanceof ExtendedContactDetail) 780 return (ExtendedContactDetail) b; 781 else 782 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a ExtendedContactDetail"); 783 } 784 785 786 787 788 public static XhtmlNode castToXhtml(Base b) throws FHIRException { 789 if (b == null) { 790 return null; 791 } 792 793 if (b instanceof Element) { 794 return ((Element) b).getXhtml(); 795 } else if (b instanceof XhtmlType) { 796 return ((XhtmlType) b).getXhtml(); 797 } else if (b instanceof StringType) { 798 try { 799 return new XhtmlParser().parseFragment(((StringType) b).asStringValue()); 800 } catch (IOException e) { 801 throw new FHIRException(e); 802 } 803 } else 804 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to XHtml"); 805 } 806 807 public static String castToXhtmlString(Base b) throws FHIRException { 808 if (b == null) { 809 return null; 810 } 811 812 if (b instanceof Element) { 813 return ((Element) b).getValue(); 814 } else if (b instanceof XhtmlType) { 815 try { 816 return new XhtmlComposer(true).compose(((XhtmlType) b).getXhtml()); 817 } catch (IOException e) { 818 return null; 819 } 820 } else if (b instanceof StringType) { 821 return ((StringType) b).asStringValue(); 822 } else 823 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to XHtml string"); 824 } 825 826 827 public static VirtualServiceDetail castToVirtualServiceDetail(Base b) throws FHIRException { 828 if (b == null) { 829 return null; 830 } 831 832 if (b instanceof VirtualServiceDetail) 833 return (VirtualServiceDetail) b; 834 else 835 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a VirtualServiceDetail"); 836 } 837 838 839 public static Availability castToAvailability(Base b) throws FHIRException { 840 if (b == null) { 841 return null; 842 } 843 844 if (b instanceof Availability) 845 return (Availability) b; 846 else 847 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a Availability"); 848 } 849 850 851 public static MonetaryComponent castToMonetaryComponent(Base b) throws FHIRException { 852 if (b == null) { 853 return null; 854 } 855 856 if (b instanceof MonetaryComponent) 857 return (MonetaryComponent) b; 858 else 859 throw new FHIRException("Unable to convert a "+b.fhirType()+"("+b.getClass().getName()+") to a MonetaryComponent"); 860 } 861}