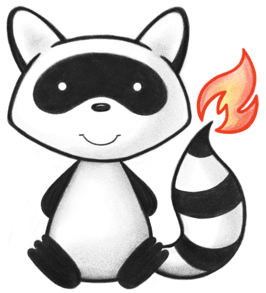
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.net.URI; 035import java.net.URISyntaxException; 036 037import org.apache.commons.lang3.StringUtils; 038 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040 041/** 042 * Primitive type "uri" in FHIR: any valid URI. Sometimes constrained to be only an absolute URI, and sometimes constrained to be a literal reference 043 */ 044@DatatypeDef(name = "uri") 045public class UriType extends PrimitiveType<String> { 046 047 private static final long serialVersionUID = 3L; 048 049 /** 050 * Constructor 051 */ 052 public UriType() { 053 // nothing 054 } 055 056 /** 057 * Constructor 058 */ 059 public UriType(String theValue) { 060 setValueAsString(theValue); 061 } 062 063 /** 064 * Constructor 065 */ 066 public UriType(URI theValue) { 067 setValue(theValue.toString()); 068 } 069 070 @Override 071 public UriType copy() { 072 UriType ret = new UriType(getValue()); 073 copyValues(ret); 074 return ret; 075 } 076 077 @Override 078 protected String encode(String theValue) { 079 return theValue; 080 } 081 082 /** 083 * Compares the given string to the string representation of this URI. In many cases it is preferable to use this 084 * instead of the standard {@link #equals(Object)} method, since that method returns <code>false</code> unless it is 085 * passed an instance of {@link UriType} 086 */ 087 public boolean equals(String theString) { 088 return StringUtils.equals(getValueAsString(), theString); 089 } 090 091 @Override 092 public int hashCode() { 093 final int prime = 31; 094 int result = 1; 095 096 String normalize = normalize(getValue()); 097 result = prime * result + ((normalize == null) ? 0 : normalize.hashCode()); 098 099 return result; 100 } 101 102 private String normalize(String theValue) { 103 if (theValue == null) { 104 return null; 105 } 106 try { 107 URI retVal = new URI(getValue()).normalize(); 108 String urlString = retVal.toString(); 109 if (urlString.endsWith("/") && urlString.length() > 1) { 110 retVal = new URI(urlString.substring(0, urlString.length() - 1)); 111 } 112 return retVal.toASCIIString(); 113 } catch (URISyntaxException e) { 114 // ourLog.debug("Failed to normalize URL '{}', message was: {}", urlString, e.toString()); 115 return theValue; 116 } 117 } 118 119 @Override 120 protected String parse(String theValue) { 121 return theValue; 122 } 123 124 /** 125 * Creates a new OidType instance which uses the given OID as the content (and prepends "urn:oid:" to the OID string 126 * in the value of the newly created OidType, per the FHIR specification). 127 * 128 * @param theOid 129 * The OID to use (<code>null</code> is acceptable and will result in a UriDt instance with a 130 * <code>null</code> value) 131 * @return A new UriDt instance 132 */ 133 public static OidType fromOid(String theOid) { 134 if (theOid == null) { 135 return new OidType(); 136 } 137 return new OidType("urn:oid:" + theOid); 138 } 139 140 @Override 141 public boolean equalsDeep(Base obj) { 142 if (!super.equalsDeep(obj)) 143 return false; 144 if (this == obj) 145 return true; 146 if (obj == null) 147 return false; 148 if (getClass() != obj.getClass()) 149 return false; 150 151 UriType other = (UriType) obj; 152 if (getValue() == null && other.getValue() == null) { 153 return true; 154 } 155 if (getValue() == null || other.getValue() == null) { 156 return false; 157 } 158 if (getValue().equals(other.getValue())) { 159 return true; 160 } 161 162 String normalize = normalize(getValue()); 163 String normalize2 = normalize(other.getValue()); 164 return normalize.equals(normalize2); 165 } 166 167 public String fhirType() { 168 return "uri"; 169 } 170 171}