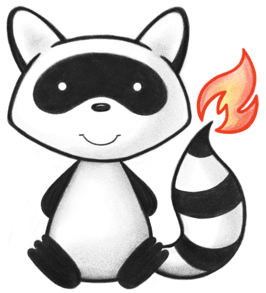
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html). 052 */ 053@ResourceDef(name="ValueSet", profile="http://hl7.org/fhir/StructureDefinition/ValueSet") 054public class ValueSet extends MetadataResource { 055 056 @Block() 057 public static class ValueSetComposeComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version. 060 */ 061 @Child(name = "lockedDate", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Fixed date for references with no specified version (transitive)", formalDefinition="The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version." ) 063 protected DateType lockedDate; 064 065 /** 066 * Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included). 067 */ 068 @Child(name = "inactive", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Whether inactive codes are in the value set", formalDefinition="Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included)." ) 070 protected BooleanType inactive; 071 072 /** 073 * Include one or more codes from a code system or other value set(s). 074 */ 075 @Child(name = "include", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 076 @Description(shortDefinition="Include one or more codes from a code system or other value set(s)", formalDefinition="Include one or more codes from a code system or other value set(s)." ) 077 protected List<ConceptSetComponent> include; 078 079 /** 080 * Exclude one or more codes from the value set based on code system filters and/or other value sets. 081 */ 082 @Child(name = "exclude", type = {ConceptSetComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 083 @Description(shortDefinition="Explicitly exclude codes from a code system or other value sets", formalDefinition="Exclude one or more codes from the value set based on code system filters and/or other value sets." ) 084 protected List<ConceptSetComponent> exclude; 085 086 /** 087 * A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server. 088 */ 089 @Child(name = "property", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 090 @Description(shortDefinition="Property to return if client doesn't override", formalDefinition="A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server." ) 091 protected List<StringType> property; 092 093 private static final long serialVersionUID = -15414259L; 094 095 /** 096 * Constructor 097 */ 098 public ValueSetComposeComponent() { 099 super(); 100 } 101 102 /** 103 * Constructor 104 */ 105 public ValueSetComposeComponent(ConceptSetComponent include) { 106 super(); 107 this.addInclude(include); 108 } 109 110 /** 111 * @return {@link #lockedDate} (The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 112 */ 113 public DateType getLockedDateElement() { 114 if (this.lockedDate == null) 115 if (Configuration.errorOnAutoCreate()) 116 throw new Error("Attempt to auto-create ValueSetComposeComponent.lockedDate"); 117 else if (Configuration.doAutoCreate()) 118 this.lockedDate = new DateType(); // bb 119 return this.lockedDate; 120 } 121 122 public boolean hasLockedDateElement() { 123 return this.lockedDate != null && !this.lockedDate.isEmpty(); 124 } 125 126 public boolean hasLockedDate() { 127 return this.lockedDate != null && !this.lockedDate.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #lockedDate} (The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 132 */ 133 public ValueSetComposeComponent setLockedDateElement(DateType value) { 134 this.lockedDate = value; 135 return this; 136 } 137 138 /** 139 * @return The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version. 140 */ 141 public Date getLockedDate() { 142 return this.lockedDate == null ? null : this.lockedDate.getValue(); 143 } 144 145 /** 146 * @param value The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version. 147 */ 148 public ValueSetComposeComponent setLockedDate(Date value) { 149 if (value == null) 150 this.lockedDate = null; 151 else { 152 if (this.lockedDate == null) 153 this.lockedDate = new DateType(); 154 this.lockedDate.setValue(value); 155 } 156 return this; 157 } 158 159 /** 160 * @return {@link #inactive} (Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 161 */ 162 public BooleanType getInactiveElement() { 163 if (this.inactive == null) 164 if (Configuration.errorOnAutoCreate()) 165 throw new Error("Attempt to auto-create ValueSetComposeComponent.inactive"); 166 else if (Configuration.doAutoCreate()) 167 this.inactive = new BooleanType(); // bb 168 return this.inactive; 169 } 170 171 public boolean hasInactiveElement() { 172 return this.inactive != null && !this.inactive.isEmpty(); 173 } 174 175 public boolean hasInactive() { 176 return this.inactive != null && !this.inactive.isEmpty(); 177 } 178 179 /** 180 * @param value {@link #inactive} (Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 181 */ 182 public ValueSetComposeComponent setInactiveElement(BooleanType value) { 183 this.inactive = value; 184 return this; 185 } 186 187 /** 188 * @return Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included). 189 */ 190 public boolean getInactive() { 191 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 192 } 193 194 /** 195 * @param value Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included). 196 */ 197 public ValueSetComposeComponent setInactive(boolean value) { 198 if (this.inactive == null) 199 this.inactive = new BooleanType(); 200 this.inactive.setValue(value); 201 return this; 202 } 203 204 /** 205 * @return {@link #include} (Include one or more codes from a code system or other value set(s).) 206 */ 207 public List<ConceptSetComponent> getInclude() { 208 if (this.include == null) 209 this.include = new ArrayList<ConceptSetComponent>(); 210 return this.include; 211 } 212 213 /** 214 * @return Returns a reference to <code>this</code> for easy method chaining 215 */ 216 public ValueSetComposeComponent setInclude(List<ConceptSetComponent> theInclude) { 217 this.include = theInclude; 218 return this; 219 } 220 221 public boolean hasInclude() { 222 if (this.include == null) 223 return false; 224 for (ConceptSetComponent item : this.include) 225 if (!item.isEmpty()) 226 return true; 227 return false; 228 } 229 230 public ConceptSetComponent addInclude() { //3 231 ConceptSetComponent t = new ConceptSetComponent(); 232 if (this.include == null) 233 this.include = new ArrayList<ConceptSetComponent>(); 234 this.include.add(t); 235 return t; 236 } 237 238 public ValueSetComposeComponent addInclude(ConceptSetComponent t) { //3 239 if (t == null) 240 return this; 241 if (this.include == null) 242 this.include = new ArrayList<ConceptSetComponent>(); 243 this.include.add(t); 244 return this; 245 } 246 247 /** 248 * @return The first repetition of repeating field {@link #include}, creating it if it does not already exist {3} 249 */ 250 public ConceptSetComponent getIncludeFirstRep() { 251 if (getInclude().isEmpty()) { 252 addInclude(); 253 } 254 return getInclude().get(0); 255 } 256 257 /** 258 * @return {@link #exclude} (Exclude one or more codes from the value set based on code system filters and/or other value sets.) 259 */ 260 public List<ConceptSetComponent> getExclude() { 261 if (this.exclude == null) 262 this.exclude = new ArrayList<ConceptSetComponent>(); 263 return this.exclude; 264 } 265 266 /** 267 * @return Returns a reference to <code>this</code> for easy method chaining 268 */ 269 public ValueSetComposeComponent setExclude(List<ConceptSetComponent> theExclude) { 270 this.exclude = theExclude; 271 return this; 272 } 273 274 public boolean hasExclude() { 275 if (this.exclude == null) 276 return false; 277 for (ConceptSetComponent item : this.exclude) 278 if (!item.isEmpty()) 279 return true; 280 return false; 281 } 282 283 public ConceptSetComponent addExclude() { //3 284 ConceptSetComponent t = new ConceptSetComponent(); 285 if (this.exclude == null) 286 this.exclude = new ArrayList<ConceptSetComponent>(); 287 this.exclude.add(t); 288 return t; 289 } 290 291 public ValueSetComposeComponent addExclude(ConceptSetComponent t) { //3 292 if (t == null) 293 return this; 294 if (this.exclude == null) 295 this.exclude = new ArrayList<ConceptSetComponent>(); 296 this.exclude.add(t); 297 return this; 298 } 299 300 /** 301 * @return The first repetition of repeating field {@link #exclude}, creating it if it does not already exist {3} 302 */ 303 public ConceptSetComponent getExcludeFirstRep() { 304 if (getExclude().isEmpty()) { 305 addExclude(); 306 } 307 return getExclude().get(0); 308 } 309 310 /** 311 * @return {@link #property} (A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.) 312 */ 313 public List<StringType> getProperty() { 314 if (this.property == null) 315 this.property = new ArrayList<StringType>(); 316 return this.property; 317 } 318 319 /** 320 * @return Returns a reference to <code>this</code> for easy method chaining 321 */ 322 public ValueSetComposeComponent setProperty(List<StringType> theProperty) { 323 this.property = theProperty; 324 return this; 325 } 326 327 public boolean hasProperty() { 328 if (this.property == null) 329 return false; 330 for (StringType item : this.property) 331 if (!item.isEmpty()) 332 return true; 333 return false; 334 } 335 336 /** 337 * @return {@link #property} (A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.) 338 */ 339 public StringType addPropertyElement() {//2 340 StringType t = new StringType(); 341 if (this.property == null) 342 this.property = new ArrayList<StringType>(); 343 this.property.add(t); 344 return t; 345 } 346 347 /** 348 * @param value {@link #property} (A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.) 349 */ 350 public ValueSetComposeComponent addProperty(String value) { //1 351 StringType t = new StringType(); 352 t.setValue(value); 353 if (this.property == null) 354 this.property = new ArrayList<StringType>(); 355 this.property.add(t); 356 return this; 357 } 358 359 /** 360 * @param value {@link #property} (A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.) 361 */ 362 public boolean hasProperty(String value) { 363 if (this.property == null) 364 return false; 365 for (StringType v : this.property) 366 if (v.getValue().equals(value)) // string 367 return true; 368 return false; 369 } 370 371 protected void listChildren(List<Property> children) { 372 super.listChildren(children); 373 children.add(new Property("lockedDate", "date", "The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.", 0, 1, lockedDate)); 374 children.add(new Property("inactive", "boolean", "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).", 0, 1, inactive)); 375 children.add(new Property("include", "", "Include one or more codes from a code system or other value set(s).", 0, java.lang.Integer.MAX_VALUE, include)); 376 children.add(new Property("exclude", "@ValueSet.compose.include", "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, java.lang.Integer.MAX_VALUE, exclude)); 377 children.add(new Property("property", "string", "A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.", 0, java.lang.Integer.MAX_VALUE, property)); 378 } 379 380 @Override 381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 382 switch (_hash) { 383 case 1391591896: /*lockedDate*/ return new Property("lockedDate", "date", "The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.", 0, 1, lockedDate); 384 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).", 0, 1, inactive); 385 case 1942574248: /*include*/ return new Property("include", "", "Include one or more codes from a code system or other value set(s).", 0, java.lang.Integer.MAX_VALUE, include); 386 case -1321148966: /*exclude*/ return new Property("exclude", "@ValueSet.compose.include", "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, java.lang.Integer.MAX_VALUE, exclude); 387 case -993141291: /*property*/ return new Property("property", "string", "A property to return in the expansion, if the client doesn't ask for any particular properties. May be either a code from the code system definition (convenient) or a the formal URI that refers to the property. The special value '*' means all properties known to the server.", 0, java.lang.Integer.MAX_VALUE, property); 388 default: return super.getNamedProperty(_hash, _name, _checkValid); 389 } 390 391 } 392 393 @Override 394 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 395 switch (hash) { 396 case 1391591896: /*lockedDate*/ return this.lockedDate == null ? new Base[0] : new Base[] {this.lockedDate}; // DateType 397 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 398 case 1942574248: /*include*/ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // ConceptSetComponent 399 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : this.exclude.toArray(new Base[this.exclude.size()]); // ConceptSetComponent 400 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // StringType 401 default: return super.getProperty(hash, name, checkValid); 402 } 403 404 } 405 406 @Override 407 public Base setProperty(int hash, String name, Base value) throws FHIRException { 408 switch (hash) { 409 case 1391591896: // lockedDate 410 this.lockedDate = TypeConvertor.castToDate(value); // DateType 411 return value; 412 case 24665195: // inactive 413 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 414 return value; 415 case 1942574248: // include 416 this.getInclude().add((ConceptSetComponent) value); // ConceptSetComponent 417 return value; 418 case -1321148966: // exclude 419 this.getExclude().add((ConceptSetComponent) value); // ConceptSetComponent 420 return value; 421 case -993141291: // property 422 this.getProperty().add(TypeConvertor.castToString(value)); // StringType 423 return value; 424 default: return super.setProperty(hash, name, value); 425 } 426 427 } 428 429 @Override 430 public Base setProperty(String name, Base value) throws FHIRException { 431 if (name.equals("lockedDate")) { 432 this.lockedDate = TypeConvertor.castToDate(value); // DateType 433 } else if (name.equals("inactive")) { 434 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 435 } else if (name.equals("include")) { 436 this.getInclude().add((ConceptSetComponent) value); 437 } else if (name.equals("exclude")) { 438 this.getExclude().add((ConceptSetComponent) value); 439 } else if (name.equals("property")) { 440 this.getProperty().add(TypeConvertor.castToString(value)); 441 } else 442 return super.setProperty(name, value); 443 return value; 444 } 445 446 @Override 447 public void removeChild(String name, Base value) throws FHIRException { 448 if (name.equals("lockedDate")) { 449 this.lockedDate = null; 450 } else if (name.equals("inactive")) { 451 this.inactive = null; 452 } else if (name.equals("include")) { 453 this.getInclude().remove((ConceptSetComponent) value); 454 } else if (name.equals("exclude")) { 455 this.getExclude().remove((ConceptSetComponent) value); 456 } else if (name.equals("property")) { 457 this.getProperty().remove(value); 458 } else 459 super.removeChild(name, value); 460 461 } 462 463 @Override 464 public Base makeProperty(int hash, String name) throws FHIRException { 465 switch (hash) { 466 case 1391591896: return getLockedDateElement(); 467 case 24665195: return getInactiveElement(); 468 case 1942574248: return addInclude(); 469 case -1321148966: return addExclude(); 470 case -993141291: return addPropertyElement(); 471 default: return super.makeProperty(hash, name); 472 } 473 474 } 475 476 @Override 477 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 478 switch (hash) { 479 case 1391591896: /*lockedDate*/ return new String[] {"date"}; 480 case 24665195: /*inactive*/ return new String[] {"boolean"}; 481 case 1942574248: /*include*/ return new String[] {}; 482 case -1321148966: /*exclude*/ return new String[] {"@ValueSet.compose.include"}; 483 case -993141291: /*property*/ return new String[] {"string"}; 484 default: return super.getTypesForProperty(hash, name); 485 } 486 487 } 488 489 @Override 490 public Base addChild(String name) throws FHIRException { 491 if (name.equals("lockedDate")) { 492 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.lockedDate"); 493 } 494 else if (name.equals("inactive")) { 495 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.inactive"); 496 } 497 else if (name.equals("include")) { 498 return addInclude(); 499 } 500 else if (name.equals("exclude")) { 501 return addExclude(); 502 } 503 else if (name.equals("property")) { 504 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.property"); 505 } 506 else 507 return super.addChild(name); 508 } 509 510 public ValueSetComposeComponent copy() { 511 ValueSetComposeComponent dst = new ValueSetComposeComponent(); 512 copyValues(dst); 513 return dst; 514 } 515 516 public void copyValues(ValueSetComposeComponent dst) { 517 super.copyValues(dst); 518 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 519 dst.inactive = inactive == null ? null : inactive.copy(); 520 if (include != null) { 521 dst.include = new ArrayList<ConceptSetComponent>(); 522 for (ConceptSetComponent i : include) 523 dst.include.add(i.copy()); 524 }; 525 if (exclude != null) { 526 dst.exclude = new ArrayList<ConceptSetComponent>(); 527 for (ConceptSetComponent i : exclude) 528 dst.exclude.add(i.copy()); 529 }; 530 if (property != null) { 531 dst.property = new ArrayList<StringType>(); 532 for (StringType i : property) 533 dst.property.add(i.copy()); 534 }; 535 } 536 537 @Override 538 public boolean equalsDeep(Base other_) { 539 if (!super.equalsDeep(other_)) 540 return false; 541 if (!(other_ instanceof ValueSetComposeComponent)) 542 return false; 543 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 544 return compareDeep(lockedDate, o.lockedDate, true) && compareDeep(inactive, o.inactive, true) && compareDeep(include, o.include, true) 545 && compareDeep(exclude, o.exclude, true) && compareDeep(property, o.property, true); 546 } 547 548 @Override 549 public boolean equalsShallow(Base other_) { 550 if (!super.equalsShallow(other_)) 551 return false; 552 if (!(other_ instanceof ValueSetComposeComponent)) 553 return false; 554 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 555 return compareValues(lockedDate, o.lockedDate, true) && compareValues(inactive, o.inactive, true) && compareValues(property, o.property, true) 556 ; 557 } 558 559 public boolean isEmpty() { 560 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lockedDate, inactive, include 561 , exclude, property); 562 } 563 564 public String fhirType() { 565 return "ValueSet.compose"; 566 567 } 568 569 } 570 571 @Block() 572 public static class ConceptSetComponent extends BackboneElement implements IBaseBackboneElement { 573 /** 574 * An absolute URI which is the code system from which the selected codes come from. 575 */ 576 @Child(name = "system", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=true) 577 @Description(shortDefinition="The system the codes come from", formalDefinition="An absolute URI which is the code system from which the selected codes come from." ) 578 protected UriType system; 579 580 /** 581 * The version of the code system that the codes are selected from, or the special version '*' for all versions. 582 */ 583 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 584 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system that the codes are selected from, or the special version '*' for all versions." ) 585 protected StringType version; 586 587 /** 588 * Specifies a concept to be included or excluded. 589 */ 590 @Child(name = "concept", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 591 @Description(shortDefinition="A concept defined in the system", formalDefinition="Specifies a concept to be included or excluded." ) 592 protected List<ConceptReferenceComponent> concept; 593 594 /** 595 * Select concepts by specifying a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified within the include, they SHALL all be true. 596 */ 597 @Child(name = "filter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 598 @Description(shortDefinition="Select codes/concepts by their properties (including relationships)", formalDefinition="Select concepts by specifying a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified within the include, they SHALL all be true." ) 599 protected List<ConceptSetFilterComponent> filter; 600 601 /** 602 * Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets. 603 */ 604 @Child(name = "valueSet", type = {CanonicalType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 605 @Description(shortDefinition="Select the contents included in this value set", formalDefinition="Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets." ) 606 protected List<CanonicalType> valueSet; 607 608 /** 609 * A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present). 610 */ 611 @Child(name = "copyright", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 612 @Description(shortDefinition="A copyright statement for the specific code system included in the value set", formalDefinition="A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present)." ) 613 protected StringType copyright; 614 615 private static final long serialVersionUID = -517139062L; 616 617 /** 618 * Constructor 619 */ 620 public ConceptSetComponent() { 621 super(); 622 } 623 624 /** 625 * @return {@link #system} (An absolute URI which is the code system from which the selected codes come from.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 626 */ 627 public UriType getSystemElement() { 628 if (this.system == null) 629 if (Configuration.errorOnAutoCreate()) 630 throw new Error("Attempt to auto-create ConceptSetComponent.system"); 631 else if (Configuration.doAutoCreate()) 632 this.system = new UriType(); // bb 633 return this.system; 634 } 635 636 public boolean hasSystemElement() { 637 return this.system != null && !this.system.isEmpty(); 638 } 639 640 public boolean hasSystem() { 641 return this.system != null && !this.system.isEmpty(); 642 } 643 644 /** 645 * @param value {@link #system} (An absolute URI which is the code system from which the selected codes come from.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 646 */ 647 public ConceptSetComponent setSystemElement(UriType value) { 648 this.system = value; 649 return this; 650 } 651 652 /** 653 * @return An absolute URI which is the code system from which the selected codes come from. 654 */ 655 public String getSystem() { 656 return this.system == null ? null : this.system.getValue(); 657 } 658 659 /** 660 * @param value An absolute URI which is the code system from which the selected codes come from. 661 */ 662 public ConceptSetComponent setSystem(String value) { 663 if (Utilities.noString(value)) 664 this.system = null; 665 else { 666 if (this.system == null) 667 this.system = new UriType(); 668 this.system.setValue(value); 669 } 670 return this; 671 } 672 673 /** 674 * @return {@link #version} (The version of the code system that the codes are selected from, or the special version '*' for all versions.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 675 */ 676 public StringType getVersionElement() { 677 if (this.version == null) 678 if (Configuration.errorOnAutoCreate()) 679 throw new Error("Attempt to auto-create ConceptSetComponent.version"); 680 else if (Configuration.doAutoCreate()) 681 this.version = new StringType(); // bb 682 return this.version; 683 } 684 685 public boolean hasVersionElement() { 686 return this.version != null && !this.version.isEmpty(); 687 } 688 689 public boolean hasVersion() { 690 return this.version != null && !this.version.isEmpty(); 691 } 692 693 /** 694 * @param value {@link #version} (The version of the code system that the codes are selected from, or the special version '*' for all versions.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 695 */ 696 public ConceptSetComponent setVersionElement(StringType value) { 697 this.version = value; 698 return this; 699 } 700 701 /** 702 * @return The version of the code system that the codes are selected from, or the special version '*' for all versions. 703 */ 704 public String getVersion() { 705 return this.version == null ? null : this.version.getValue(); 706 } 707 708 /** 709 * @param value The version of the code system that the codes are selected from, or the special version '*' for all versions. 710 */ 711 public ConceptSetComponent setVersion(String value) { 712 if (Utilities.noString(value)) 713 this.version = null; 714 else { 715 if (this.version == null) 716 this.version = new StringType(); 717 this.version.setValue(value); 718 } 719 return this; 720 } 721 722 /** 723 * @return {@link #concept} (Specifies a concept to be included or excluded.) 724 */ 725 public List<ConceptReferenceComponent> getConcept() { 726 if (this.concept == null) 727 this.concept = new ArrayList<ConceptReferenceComponent>(); 728 return this.concept; 729 } 730 731 /** 732 * @return Returns a reference to <code>this</code> for easy method chaining 733 */ 734 public ConceptSetComponent setConcept(List<ConceptReferenceComponent> theConcept) { 735 this.concept = theConcept; 736 return this; 737 } 738 739 public boolean hasConcept() { 740 if (this.concept == null) 741 return false; 742 for (ConceptReferenceComponent item : this.concept) 743 if (!item.isEmpty()) 744 return true; 745 return false; 746 } 747 748 public ConceptReferenceComponent addConcept() { //3 749 ConceptReferenceComponent t = new ConceptReferenceComponent(); 750 if (this.concept == null) 751 this.concept = new ArrayList<ConceptReferenceComponent>(); 752 this.concept.add(t); 753 return t; 754 } 755 756 public ConceptSetComponent addConcept(ConceptReferenceComponent t) { //3 757 if (t == null) 758 return this; 759 if (this.concept == null) 760 this.concept = new ArrayList<ConceptReferenceComponent>(); 761 this.concept.add(t); 762 return this; 763 } 764 765 /** 766 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist {3} 767 */ 768 public ConceptReferenceComponent getConceptFirstRep() { 769 if (getConcept().isEmpty()) { 770 addConcept(); 771 } 772 return getConcept().get(0); 773 } 774 775 /** 776 * @return {@link #filter} (Select concepts by specifying a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified within the include, they SHALL all be true.) 777 */ 778 public List<ConceptSetFilterComponent> getFilter() { 779 if (this.filter == null) 780 this.filter = new ArrayList<ConceptSetFilterComponent>(); 781 return this.filter; 782 } 783 784 /** 785 * @return Returns a reference to <code>this</code> for easy method chaining 786 */ 787 public ConceptSetComponent setFilter(List<ConceptSetFilterComponent> theFilter) { 788 this.filter = theFilter; 789 return this; 790 } 791 792 public boolean hasFilter() { 793 if (this.filter == null) 794 return false; 795 for (ConceptSetFilterComponent item : this.filter) 796 if (!item.isEmpty()) 797 return true; 798 return false; 799 } 800 801 public ConceptSetFilterComponent addFilter() { //3 802 ConceptSetFilterComponent t = new ConceptSetFilterComponent(); 803 if (this.filter == null) 804 this.filter = new ArrayList<ConceptSetFilterComponent>(); 805 this.filter.add(t); 806 return t; 807 } 808 809 public ConceptSetComponent addFilter(ConceptSetFilterComponent t) { //3 810 if (t == null) 811 return this; 812 if (this.filter == null) 813 this.filter = new ArrayList<ConceptSetFilterComponent>(); 814 this.filter.add(t); 815 return this; 816 } 817 818 /** 819 * @return The first repetition of repeating field {@link #filter}, creating it if it does not already exist {3} 820 */ 821 public ConceptSetFilterComponent getFilterFirstRep() { 822 if (getFilter().isEmpty()) { 823 addFilter(); 824 } 825 return getFilter().get(0); 826 } 827 828 /** 829 * @return {@link #valueSet} (Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.) 830 */ 831 public List<CanonicalType> getValueSet() { 832 if (this.valueSet == null) 833 this.valueSet = new ArrayList<CanonicalType>(); 834 return this.valueSet; 835 } 836 837 /** 838 * @return Returns a reference to <code>this</code> for easy method chaining 839 */ 840 public ConceptSetComponent setValueSet(List<CanonicalType> theValueSet) { 841 this.valueSet = theValueSet; 842 return this; 843 } 844 845 public boolean hasValueSet() { 846 if (this.valueSet == null) 847 return false; 848 for (CanonicalType item : this.valueSet) 849 if (!item.isEmpty()) 850 return true; 851 return false; 852 } 853 854 /** 855 * @return {@link #valueSet} (Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.) 856 */ 857 public CanonicalType addValueSetElement() {//2 858 CanonicalType t = new CanonicalType(); 859 if (this.valueSet == null) 860 this.valueSet = new ArrayList<CanonicalType>(); 861 this.valueSet.add(t); 862 return t; 863 } 864 865 /** 866 * @param value {@link #valueSet} (Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.) 867 */ 868 public ConceptSetComponent addValueSet(String value) { //1 869 CanonicalType t = new CanonicalType(); 870 t.setValue(value); 871 if (this.valueSet == null) 872 this.valueSet = new ArrayList<CanonicalType>(); 873 this.valueSet.add(t); 874 return this; 875 } 876 877 /** 878 * @param value {@link #valueSet} (Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.) 879 */ 880 public boolean hasValueSet(String value) { 881 if (this.valueSet == null) 882 return false; 883 for (CanonicalType v : this.valueSet) 884 if (v.getValue().equals(value)) // canonical 885 return true; 886 return false; 887 } 888 889 /** 890 * @return {@link #copyright} (A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present).). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 891 */ 892 public StringType getCopyrightElement() { 893 if (this.copyright == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create ConceptSetComponent.copyright"); 896 else if (Configuration.doAutoCreate()) 897 this.copyright = new StringType(); // bb 898 return this.copyright; 899 } 900 901 public boolean hasCopyrightElement() { 902 return this.copyright != null && !this.copyright.isEmpty(); 903 } 904 905 public boolean hasCopyright() { 906 return this.copyright != null && !this.copyright.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #copyright} (A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present).). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 911 */ 912 public ConceptSetComponent setCopyrightElement(StringType value) { 913 this.copyright = value; 914 return this; 915 } 916 917 /** 918 * @return A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present). 919 */ 920 public String getCopyright() { 921 return this.copyright == null ? null : this.copyright.getValue(); 922 } 923 924 /** 925 * @param value A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present). 926 */ 927 public ConceptSetComponent setCopyright(String value) { 928 if (Utilities.noString(value)) 929 this.copyright = null; 930 else { 931 if (this.copyright == null) 932 this.copyright = new StringType(); 933 this.copyright.setValue(value); 934 } 935 return this; 936 } 937 938 protected void listChildren(List<Property> children) { 939 super.listChildren(children); 940 children.add(new Property("system", "uri", "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system)); 941 children.add(new Property("version", "string", "The version of the code system that the codes are selected from, or the special version '*' for all versions.", 0, 1, version)); 942 children.add(new Property("concept", "", "Specifies a concept to be included or excluded.", 0, java.lang.Integer.MAX_VALUE, concept)); 943 children.add(new Property("filter", "", "Select concepts by specifying a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified within the include, they SHALL all be true.", 0, java.lang.Integer.MAX_VALUE, filter)); 944 children.add(new Property("valueSet", "canonical(ValueSet)", "Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.", 0, java.lang.Integer.MAX_VALUE, valueSet)); 945 children.add(new Property("copyright", "string", "A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present).", 0, 1, copyright)); 946 } 947 948 @Override 949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 950 switch (_hash) { 951 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system); 952 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system that the codes are selected from, or the special version '*' for all versions.", 0, 1, version); 953 case 951024232: /*concept*/ return new Property("concept", "", "Specifies a concept to be included or excluded.", 0, java.lang.Integer.MAX_VALUE, concept); 954 case -1274492040: /*filter*/ return new Property("filter", "", "Select concepts by specifying a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified within the include, they SHALL all be true.", 0, java.lang.Integer.MAX_VALUE, filter); 955 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the intersection of the contents of all of the referenced value sets.", 0, java.lang.Integer.MAX_VALUE, valueSet); 956 case 1522889671: /*copyright*/ return new Property("copyright", "string", "A copyright statement for the specific code system asserted by the containing ValueSet.compose.include element's system value (if the associated ValueSet.compose.include.version element is not present); or the code system and version combination (if the associated ValueSet.compose.include.version element is present).", 0, 1, copyright); 957 default: return super.getNamedProperty(_hash, _name, _checkValid); 958 } 959 960 } 961 962 @Override 963 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 964 switch (hash) { 965 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 966 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 967 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptReferenceComponent 968 case -1274492040: /*filter*/ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // ConceptSetFilterComponent 969 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : this.valueSet.toArray(new Base[this.valueSet.size()]); // CanonicalType 970 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // StringType 971 default: return super.getProperty(hash, name, checkValid); 972 } 973 974 } 975 976 @Override 977 public Base setProperty(int hash, String name, Base value) throws FHIRException { 978 switch (hash) { 979 case -887328209: // system 980 this.system = TypeConvertor.castToUri(value); // UriType 981 return value; 982 case 351608024: // version 983 this.version = TypeConvertor.castToString(value); // StringType 984 return value; 985 case 951024232: // concept 986 this.getConcept().add((ConceptReferenceComponent) value); // ConceptReferenceComponent 987 return value; 988 case -1274492040: // filter 989 this.getFilter().add((ConceptSetFilterComponent) value); // ConceptSetFilterComponent 990 return value; 991 case -1410174671: // valueSet 992 this.getValueSet().add(TypeConvertor.castToCanonical(value)); // CanonicalType 993 return value; 994 case 1522889671: // copyright 995 this.copyright = TypeConvertor.castToString(value); // StringType 996 return value; 997 default: return super.setProperty(hash, name, value); 998 } 999 1000 } 1001 1002 @Override 1003 public Base setProperty(String name, Base value) throws FHIRException { 1004 if (name.equals("system")) { 1005 this.system = TypeConvertor.castToUri(value); // UriType 1006 } else if (name.equals("version")) { 1007 this.version = TypeConvertor.castToString(value); // StringType 1008 } else if (name.equals("concept")) { 1009 this.getConcept().add((ConceptReferenceComponent) value); 1010 } else if (name.equals("filter")) { 1011 this.getFilter().add((ConceptSetFilterComponent) value); 1012 } else if (name.equals("valueSet")) { 1013 this.getValueSet().add(TypeConvertor.castToCanonical(value)); 1014 } else if (name.equals("copyright")) { 1015 this.copyright = TypeConvertor.castToString(value); // StringType 1016 } else 1017 return super.setProperty(name, value); 1018 return value; 1019 } 1020 1021 @Override 1022 public void removeChild(String name, Base value) throws FHIRException { 1023 if (name.equals("system")) { 1024 this.system = null; 1025 } else if (name.equals("version")) { 1026 this.version = null; 1027 } else if (name.equals("concept")) { 1028 this.getConcept().remove((ConceptReferenceComponent) value); 1029 } else if (name.equals("filter")) { 1030 this.getFilter().remove((ConceptSetFilterComponent) value); 1031 } else if (name.equals("valueSet")) { 1032 this.getValueSet().remove(value); 1033 } else if (name.equals("copyright")) { 1034 this.copyright = null; 1035 } else 1036 super.removeChild(name, value); 1037 1038 } 1039 1040 @Override 1041 public Base makeProperty(int hash, String name) throws FHIRException { 1042 switch (hash) { 1043 case -887328209: return getSystemElement(); 1044 case 351608024: return getVersionElement(); 1045 case 951024232: return addConcept(); 1046 case -1274492040: return addFilter(); 1047 case -1410174671: return addValueSetElement(); 1048 case 1522889671: return getCopyrightElement(); 1049 default: return super.makeProperty(hash, name); 1050 } 1051 1052 } 1053 1054 @Override 1055 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1056 switch (hash) { 1057 case -887328209: /*system*/ return new String[] {"uri"}; 1058 case 351608024: /*version*/ return new String[] {"string"}; 1059 case 951024232: /*concept*/ return new String[] {}; 1060 case -1274492040: /*filter*/ return new String[] {}; 1061 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 1062 case 1522889671: /*copyright*/ return new String[] {"string"}; 1063 default: return super.getTypesForProperty(hash, name); 1064 } 1065 1066 } 1067 1068 @Override 1069 public Base addChild(String name) throws FHIRException { 1070 if (name.equals("system")) { 1071 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.system"); 1072 } 1073 else if (name.equals("version")) { 1074 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.version"); 1075 } 1076 else if (name.equals("concept")) { 1077 return addConcept(); 1078 } 1079 else if (name.equals("filter")) { 1080 return addFilter(); 1081 } 1082 else if (name.equals("valueSet")) { 1083 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.valueSet"); 1084 } 1085 else if (name.equals("copyright")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.copyright"); 1087 } 1088 else 1089 return super.addChild(name); 1090 } 1091 1092 public ConceptSetComponent copy() { 1093 ConceptSetComponent dst = new ConceptSetComponent(); 1094 copyValues(dst); 1095 return dst; 1096 } 1097 1098 public void copyValues(ConceptSetComponent dst) { 1099 super.copyValues(dst); 1100 dst.system = system == null ? null : system.copy(); 1101 dst.version = version == null ? null : version.copy(); 1102 if (concept != null) { 1103 dst.concept = new ArrayList<ConceptReferenceComponent>(); 1104 for (ConceptReferenceComponent i : concept) 1105 dst.concept.add(i.copy()); 1106 }; 1107 if (filter != null) { 1108 dst.filter = new ArrayList<ConceptSetFilterComponent>(); 1109 for (ConceptSetFilterComponent i : filter) 1110 dst.filter.add(i.copy()); 1111 }; 1112 if (valueSet != null) { 1113 dst.valueSet = new ArrayList<CanonicalType>(); 1114 for (CanonicalType i : valueSet) 1115 dst.valueSet.add(i.copy()); 1116 }; 1117 dst.copyright = copyright == null ? null : copyright.copy(); 1118 } 1119 1120 @Override 1121 public boolean equalsDeep(Base other_) { 1122 if (!super.equalsDeep(other_)) 1123 return false; 1124 if (!(other_ instanceof ConceptSetComponent)) 1125 return false; 1126 ConceptSetComponent o = (ConceptSetComponent) other_; 1127 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(concept, o.concept, true) 1128 && compareDeep(filter, o.filter, true) && compareDeep(valueSet, o.valueSet, true) && compareDeep(copyright, o.copyright, true) 1129 ; 1130 } 1131 1132 @Override 1133 public boolean equalsShallow(Base other_) { 1134 if (!super.equalsShallow(other_)) 1135 return false; 1136 if (!(other_ instanceof ConceptSetComponent)) 1137 return false; 1138 ConceptSetComponent o = (ConceptSetComponent) other_; 1139 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(valueSet, o.valueSet, true) 1140 && compareValues(copyright, o.copyright, true); 1141 } 1142 1143 public boolean isEmpty() { 1144 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, concept 1145 , filter, valueSet, copyright); 1146 } 1147 1148 public String fhirType() { 1149 return "ValueSet.compose.include"; 1150 1151 } 1152 1153 public boolean hasConcept(String code) { 1154 for (ConceptReferenceComponent c : getConcept()) { 1155 if (code.equals(c.getCode())) { 1156 return true; 1157 } 1158 } 1159 return false; 1160 } 1161 1162 } 1163 1164 @Block() 1165 public static class ConceptReferenceComponent extends BackboneElement implements IBaseBackboneElement { 1166 /** 1167 * Specifies a code for the concept to be included or excluded. 1168 */ 1169 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1170 @Description(shortDefinition="Code or expression from system", formalDefinition="Specifies a code for the concept to be included or excluded." ) 1171 protected CodeType code; 1172 1173 /** 1174 * The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1175 */ 1176 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1177 @Description(shortDefinition="Text to display for this code for this value set in this valueset", formalDefinition="The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system." ) 1178 protected StringType display; 1179 1180 /** 1181 * Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc. 1182 */ 1183 @Child(name = "designation", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1184 @Description(shortDefinition="Additional representations for this concept", formalDefinition="Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc." ) 1185 protected List<ConceptReferenceDesignationComponent> designation; 1186 1187 private static final long serialVersionUID = 260579971L; 1188 1189 /** 1190 * Constructor 1191 */ 1192 public ConceptReferenceComponent() { 1193 super(); 1194 } 1195 1196 /** 1197 * Constructor 1198 */ 1199 public ConceptReferenceComponent(String code) { 1200 super(); 1201 this.setCode(code); 1202 } 1203 1204 /** 1205 * @return {@link #code} (Specifies a code for the concept to be included or excluded.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1206 */ 1207 public CodeType getCodeElement() { 1208 if (this.code == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create ConceptReferenceComponent.code"); 1211 else if (Configuration.doAutoCreate()) 1212 this.code = new CodeType(); // bb 1213 return this.code; 1214 } 1215 1216 public boolean hasCodeElement() { 1217 return this.code != null && !this.code.isEmpty(); 1218 } 1219 1220 public boolean hasCode() { 1221 return this.code != null && !this.code.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #code} (Specifies a code for the concept to be included or excluded.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1226 */ 1227 public ConceptReferenceComponent setCodeElement(CodeType value) { 1228 this.code = value; 1229 return this; 1230 } 1231 1232 /** 1233 * @return Specifies a code for the concept to be included or excluded. 1234 */ 1235 public String getCode() { 1236 return this.code == null ? null : this.code.getValue(); 1237 } 1238 1239 /** 1240 * @param value Specifies a code for the concept to be included or excluded. 1241 */ 1242 public ConceptReferenceComponent setCode(String value) { 1243 if (this.code == null) 1244 this.code = new CodeType(); 1245 this.code.setValue(value); 1246 return this; 1247 } 1248 1249 /** 1250 * @return {@link #display} (The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1251 */ 1252 public StringType getDisplayElement() { 1253 if (this.display == null) 1254 if (Configuration.errorOnAutoCreate()) 1255 throw new Error("Attempt to auto-create ConceptReferenceComponent.display"); 1256 else if (Configuration.doAutoCreate()) 1257 this.display = new StringType(); // bb 1258 return this.display; 1259 } 1260 1261 public boolean hasDisplayElement() { 1262 return this.display != null && !this.display.isEmpty(); 1263 } 1264 1265 public boolean hasDisplay() { 1266 return this.display != null && !this.display.isEmpty(); 1267 } 1268 1269 /** 1270 * @param value {@link #display} (The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1271 */ 1272 public ConceptReferenceComponent setDisplayElement(StringType value) { 1273 this.display = value; 1274 return this; 1275 } 1276 1277 /** 1278 * @return The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1279 */ 1280 public String getDisplay() { 1281 return this.display == null ? null : this.display.getValue(); 1282 } 1283 1284 /** 1285 * @param value The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1286 */ 1287 public ConceptReferenceComponent setDisplay(String value) { 1288 if (Utilities.noString(value)) 1289 this.display = null; 1290 else { 1291 if (this.display == null) 1292 this.display = new StringType(); 1293 this.display.setValue(value); 1294 } 1295 return this; 1296 } 1297 1298 /** 1299 * @return {@link #designation} (Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.) 1300 */ 1301 public List<ConceptReferenceDesignationComponent> getDesignation() { 1302 if (this.designation == null) 1303 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1304 return this.designation; 1305 } 1306 1307 /** 1308 * @return Returns a reference to <code>this</code> for easy method chaining 1309 */ 1310 public ConceptReferenceComponent setDesignation(List<ConceptReferenceDesignationComponent> theDesignation) { 1311 this.designation = theDesignation; 1312 return this; 1313 } 1314 1315 public boolean hasDesignation() { 1316 if (this.designation == null) 1317 return false; 1318 for (ConceptReferenceDesignationComponent item : this.designation) 1319 if (!item.isEmpty()) 1320 return true; 1321 return false; 1322 } 1323 1324 public ConceptReferenceDesignationComponent addDesignation() { //3 1325 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 1326 if (this.designation == null) 1327 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1328 this.designation.add(t); 1329 return t; 1330 } 1331 1332 public ConceptReferenceComponent addDesignation(ConceptReferenceDesignationComponent t) { //3 1333 if (t == null) 1334 return this; 1335 if (this.designation == null) 1336 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1337 this.designation.add(t); 1338 return this; 1339 } 1340 1341 /** 1342 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist {3} 1343 */ 1344 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 1345 if (getDesignation().isEmpty()) { 1346 addDesignation(); 1347 } 1348 return getDesignation().get(0); 1349 } 1350 1351 protected void listChildren(List<Property> children) { 1352 super.listChildren(children); 1353 children.add(new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 1, code)); 1354 children.add(new Property("display", "string", "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 0, 1, display)); 1355 children.add(new Property("designation", "", "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation)); 1356 } 1357 1358 @Override 1359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1360 switch (_hash) { 1361 case 3059181: /*code*/ return new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 1, code); 1362 case 1671764162: /*display*/ return new Property("display", "string", "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 0, 1, display); 1363 case -900931593: /*designation*/ return new Property("designation", "", "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation); 1364 default: return super.getNamedProperty(_hash, _name, _checkValid); 1365 } 1366 1367 } 1368 1369 @Override 1370 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1371 switch (hash) { 1372 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1373 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1374 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 1375 default: return super.getProperty(hash, name, checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1382 switch (hash) { 1383 case 3059181: // code 1384 this.code = TypeConvertor.castToCode(value); // CodeType 1385 return value; 1386 case 1671764162: // display 1387 this.display = TypeConvertor.castToString(value); // StringType 1388 return value; 1389 case -900931593: // designation 1390 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 1391 return value; 1392 default: return super.setProperty(hash, name, value); 1393 } 1394 1395 } 1396 1397 @Override 1398 public Base setProperty(String name, Base value) throws FHIRException { 1399 if (name.equals("code")) { 1400 this.code = TypeConvertor.castToCode(value); // CodeType 1401 } else if (name.equals("display")) { 1402 this.display = TypeConvertor.castToString(value); // StringType 1403 } else if (name.equals("designation")) { 1404 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 1405 } else 1406 return super.setProperty(name, value); 1407 return value; 1408 } 1409 1410 @Override 1411 public void removeChild(String name, Base value) throws FHIRException { 1412 if (name.equals("code")) { 1413 this.code = null; 1414 } else if (name.equals("display")) { 1415 this.display = null; 1416 } else if (name.equals("designation")) { 1417 this.getDesignation().remove((ConceptReferenceDesignationComponent) value); 1418 } else 1419 super.removeChild(name, value); 1420 1421 } 1422 1423 @Override 1424 public Base makeProperty(int hash, String name) throws FHIRException { 1425 switch (hash) { 1426 case 3059181: return getCodeElement(); 1427 case 1671764162: return getDisplayElement(); 1428 case -900931593: return addDesignation(); 1429 default: return super.makeProperty(hash, name); 1430 } 1431 1432 } 1433 1434 @Override 1435 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1436 switch (hash) { 1437 case 3059181: /*code*/ return new String[] {"code"}; 1438 case 1671764162: /*display*/ return new String[] {"string"}; 1439 case -900931593: /*designation*/ return new String[] {}; 1440 default: return super.getTypesForProperty(hash, name); 1441 } 1442 1443 } 1444 1445 @Override 1446 public Base addChild(String name) throws FHIRException { 1447 if (name.equals("code")) { 1448 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.concept.code"); 1449 } 1450 else if (name.equals("display")) { 1451 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.concept.display"); 1452 } 1453 else if (name.equals("designation")) { 1454 return addDesignation(); 1455 } 1456 else 1457 return super.addChild(name); 1458 } 1459 1460 public ConceptReferenceComponent copy() { 1461 ConceptReferenceComponent dst = new ConceptReferenceComponent(); 1462 copyValues(dst); 1463 return dst; 1464 } 1465 1466 public void copyValues(ConceptReferenceComponent dst) { 1467 super.copyValues(dst); 1468 dst.code = code == null ? null : code.copy(); 1469 dst.display = display == null ? null : display.copy(); 1470 if (designation != null) { 1471 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1472 for (ConceptReferenceDesignationComponent i : designation) 1473 dst.designation.add(i.copy()); 1474 }; 1475 } 1476 1477 @Override 1478 public boolean equalsDeep(Base other_) { 1479 if (!super.equalsDeep(other_)) 1480 return false; 1481 if (!(other_ instanceof ConceptReferenceComponent)) 1482 return false; 1483 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1484 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(designation, o.designation, true) 1485 ; 1486 } 1487 1488 @Override 1489 public boolean equalsShallow(Base other_) { 1490 if (!super.equalsShallow(other_)) 1491 return false; 1492 if (!(other_ instanceof ConceptReferenceComponent)) 1493 return false; 1494 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1495 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1496 } 1497 1498 public boolean isEmpty() { 1499 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, designation 1500 ); 1501 } 1502 1503 public String fhirType() { 1504 return "ValueSet.compose.include.concept"; 1505 1506 } 1507 1508 } 1509 1510 @Block() 1511 public static class ConceptReferenceDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1512 /** 1513 * The language this designation is defined for. 1514 */ 1515 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1516 @Description(shortDefinition="Human language of the designation", formalDefinition="The language this designation is defined for." ) 1517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 1518 protected CodeType language; 1519 1520 /** 1521 * A code that represents types of uses of designations. 1522 */ 1523 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 1524 @Description(shortDefinition="Types of uses of designations", formalDefinition="A code that represents types of uses of designations." ) 1525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1526 protected Coding use; 1527 1528 /** 1529 * Additional codes that detail how this designation would be used, if there is more than one use. 1530 */ 1531 @Child(name = "additionalUse", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1532 @Description(shortDefinition="Additional ways how this designation would be used", formalDefinition="Additional codes that detail how this designation would be used, if there is more than one use." ) 1533 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1534 protected List<Coding> additionalUse; 1535 1536 /** 1537 * The text value for this designation. 1538 */ 1539 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 1540 @Description(shortDefinition="The text value for this designation", formalDefinition="The text value for this designation." ) 1541 protected StringType value; 1542 1543 private static final long serialVersionUID = -141147882L; 1544 1545 /** 1546 * Constructor 1547 */ 1548 public ConceptReferenceDesignationComponent() { 1549 super(); 1550 } 1551 1552 /** 1553 * Constructor 1554 */ 1555 public ConceptReferenceDesignationComponent(String value) { 1556 super(); 1557 this.setValue(value); 1558 } 1559 1560 /** 1561 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1562 */ 1563 public CodeType getLanguageElement() { 1564 if (this.language == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.language"); 1567 else if (Configuration.doAutoCreate()) 1568 this.language = new CodeType(); // bb 1569 return this.language; 1570 } 1571 1572 public boolean hasLanguageElement() { 1573 return this.language != null && !this.language.isEmpty(); 1574 } 1575 1576 public boolean hasLanguage() { 1577 return this.language != null && !this.language.isEmpty(); 1578 } 1579 1580 /** 1581 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1582 */ 1583 public ConceptReferenceDesignationComponent setLanguageElement(CodeType value) { 1584 this.language = value; 1585 return this; 1586 } 1587 1588 /** 1589 * @return The language this designation is defined for. 1590 */ 1591 public String getLanguage() { 1592 return this.language == null ? null : this.language.getValue(); 1593 } 1594 1595 /** 1596 * @param value The language this designation is defined for. 1597 */ 1598 public ConceptReferenceDesignationComponent setLanguage(String value) { 1599 if (Utilities.noString(value)) 1600 this.language = null; 1601 else { 1602 if (this.language == null) 1603 this.language = new CodeType(); 1604 this.language.setValue(value); 1605 } 1606 return this; 1607 } 1608 1609 /** 1610 * @return {@link #use} (A code that represents types of uses of designations.) 1611 */ 1612 public Coding getUse() { 1613 if (this.use == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.use"); 1616 else if (Configuration.doAutoCreate()) 1617 this.use = new Coding(); // cc 1618 return this.use; 1619 } 1620 1621 public boolean hasUse() { 1622 return this.use != null && !this.use.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #use} (A code that represents types of uses of designations.) 1627 */ 1628 public ConceptReferenceDesignationComponent setUse(Coding value) { 1629 this.use = value; 1630 return this; 1631 } 1632 1633 /** 1634 * @return {@link #additionalUse} (Additional codes that detail how this designation would be used, if there is more than one use.) 1635 */ 1636 public List<Coding> getAdditionalUse() { 1637 if (this.additionalUse == null) 1638 this.additionalUse = new ArrayList<Coding>(); 1639 return this.additionalUse; 1640 } 1641 1642 /** 1643 * @return Returns a reference to <code>this</code> for easy method chaining 1644 */ 1645 public ConceptReferenceDesignationComponent setAdditionalUse(List<Coding> theAdditionalUse) { 1646 this.additionalUse = theAdditionalUse; 1647 return this; 1648 } 1649 1650 public boolean hasAdditionalUse() { 1651 if (this.additionalUse == null) 1652 return false; 1653 for (Coding item : this.additionalUse) 1654 if (!item.isEmpty()) 1655 return true; 1656 return false; 1657 } 1658 1659 public Coding addAdditionalUse() { //3 1660 Coding t = new Coding(); 1661 if (this.additionalUse == null) 1662 this.additionalUse = new ArrayList<Coding>(); 1663 this.additionalUse.add(t); 1664 return t; 1665 } 1666 1667 public ConceptReferenceDesignationComponent addAdditionalUse(Coding t) { //3 1668 if (t == null) 1669 return this; 1670 if (this.additionalUse == null) 1671 this.additionalUse = new ArrayList<Coding>(); 1672 this.additionalUse.add(t); 1673 return this; 1674 } 1675 1676 /** 1677 * @return The first repetition of repeating field {@link #additionalUse}, creating it if it does not already exist {3} 1678 */ 1679 public Coding getAdditionalUseFirstRep() { 1680 if (getAdditionalUse().isEmpty()) { 1681 addAdditionalUse(); 1682 } 1683 return getAdditionalUse().get(0); 1684 } 1685 1686 /** 1687 * @return {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1688 */ 1689 public StringType getValueElement() { 1690 if (this.value == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.value"); 1693 else if (Configuration.doAutoCreate()) 1694 this.value = new StringType(); // bb 1695 return this.value; 1696 } 1697 1698 public boolean hasValueElement() { 1699 return this.value != null && !this.value.isEmpty(); 1700 } 1701 1702 public boolean hasValue() { 1703 return this.value != null && !this.value.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1708 */ 1709 public ConceptReferenceDesignationComponent setValueElement(StringType value) { 1710 this.value = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return The text value for this designation. 1716 */ 1717 public String getValue() { 1718 return this.value == null ? null : this.value.getValue(); 1719 } 1720 1721 /** 1722 * @param value The text value for this designation. 1723 */ 1724 public ConceptReferenceDesignationComponent setValue(String value) { 1725 if (this.value == null) 1726 this.value = new StringType(); 1727 this.value.setValue(value); 1728 return this; 1729 } 1730 1731 protected void listChildren(List<Property> children) { 1732 super.listChildren(children); 1733 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1734 children.add(new Property("use", "Coding", "A code that represents types of uses of designations.", 0, 1, use)); 1735 children.add(new Property("additionalUse", "Coding", "Additional codes that detail how this designation would be used, if there is more than one use.", 0, java.lang.Integer.MAX_VALUE, additionalUse)); 1736 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 1737 } 1738 1739 @Override 1740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1741 switch (_hash) { 1742 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1743 case 116103: /*use*/ return new Property("use", "Coding", "A code that represents types of uses of designations.", 0, 1, use); 1744 case 938414048: /*additionalUse*/ return new Property("additionalUse", "Coding", "Additional codes that detail how this designation would be used, if there is more than one use.", 0, java.lang.Integer.MAX_VALUE, additionalUse); 1745 case 111972721: /*value*/ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 1746 default: return super.getNamedProperty(_hash, _name, _checkValid); 1747 } 1748 1749 } 1750 1751 @Override 1752 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1753 switch (hash) { 1754 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1755 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1756 case 938414048: /*additionalUse*/ return this.additionalUse == null ? new Base[0] : this.additionalUse.toArray(new Base[this.additionalUse.size()]); // Coding 1757 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1758 default: return super.getProperty(hash, name, checkValid); 1759 } 1760 1761 } 1762 1763 @Override 1764 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1765 switch (hash) { 1766 case -1613589672: // language 1767 this.language = TypeConvertor.castToCode(value); // CodeType 1768 return value; 1769 case 116103: // use 1770 this.use = TypeConvertor.castToCoding(value); // Coding 1771 return value; 1772 case 938414048: // additionalUse 1773 this.getAdditionalUse().add(TypeConvertor.castToCoding(value)); // Coding 1774 return value; 1775 case 111972721: // value 1776 this.value = TypeConvertor.castToString(value); // StringType 1777 return value; 1778 default: return super.setProperty(hash, name, value); 1779 } 1780 1781 } 1782 1783 @Override 1784 public Base setProperty(String name, Base value) throws FHIRException { 1785 if (name.equals("language")) { 1786 this.language = TypeConvertor.castToCode(value); // CodeType 1787 } else if (name.equals("use")) { 1788 this.use = TypeConvertor.castToCoding(value); // Coding 1789 } else if (name.equals("additionalUse")) { 1790 this.getAdditionalUse().add(TypeConvertor.castToCoding(value)); 1791 } else if (name.equals("value")) { 1792 this.value = TypeConvertor.castToString(value); // StringType 1793 } else 1794 return super.setProperty(name, value); 1795 return value; 1796 } 1797 1798 @Override 1799 public void removeChild(String name, Base value) throws FHIRException { 1800 if (name.equals("language")) { 1801 this.language = null; 1802 } else if (name.equals("use")) { 1803 this.use = null; 1804 } else if (name.equals("additionalUse")) { 1805 this.getAdditionalUse().remove(value); 1806 } else if (name.equals("value")) { 1807 this.value = null; 1808 } else 1809 super.removeChild(name, value); 1810 1811 } 1812 1813 @Override 1814 public Base makeProperty(int hash, String name) throws FHIRException { 1815 switch (hash) { 1816 case -1613589672: return getLanguageElement(); 1817 case 116103: return getUse(); 1818 case 938414048: return addAdditionalUse(); 1819 case 111972721: return getValueElement(); 1820 default: return super.makeProperty(hash, name); 1821 } 1822 1823 } 1824 1825 @Override 1826 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1827 switch (hash) { 1828 case -1613589672: /*language*/ return new String[] {"code"}; 1829 case 116103: /*use*/ return new String[] {"Coding"}; 1830 case 938414048: /*additionalUse*/ return new String[] {"Coding"}; 1831 case 111972721: /*value*/ return new String[] {"string"}; 1832 default: return super.getTypesForProperty(hash, name); 1833 } 1834 1835 } 1836 1837 @Override 1838 public Base addChild(String name) throws FHIRException { 1839 if (name.equals("language")) { 1840 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.concept.designation.language"); 1841 } 1842 else if (name.equals("use")) { 1843 this.use = new Coding(); 1844 return this.use; 1845 } 1846 else if (name.equals("additionalUse")) { 1847 return addAdditionalUse(); 1848 } 1849 else if (name.equals("value")) { 1850 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.concept.designation.value"); 1851 } 1852 else 1853 return super.addChild(name); 1854 } 1855 1856 public ConceptReferenceDesignationComponent copy() { 1857 ConceptReferenceDesignationComponent dst = new ConceptReferenceDesignationComponent(); 1858 copyValues(dst); 1859 return dst; 1860 } 1861 1862 public void copyValues(ConceptReferenceDesignationComponent dst) { 1863 super.copyValues(dst); 1864 dst.language = language == null ? null : language.copy(); 1865 dst.use = use == null ? null : use.copy(); 1866 if (additionalUse != null) { 1867 dst.additionalUse = new ArrayList<Coding>(); 1868 for (Coding i : additionalUse) 1869 dst.additionalUse.add(i.copy()); 1870 }; 1871 dst.value = value == null ? null : value.copy(); 1872 } 1873 1874 @Override 1875 public boolean equalsDeep(Base other_) { 1876 if (!super.equalsDeep(other_)) 1877 return false; 1878 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 1879 return false; 1880 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 1881 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) && compareDeep(additionalUse, o.additionalUse, true) 1882 && compareDeep(value, o.value, true); 1883 } 1884 1885 @Override 1886 public boolean equalsShallow(Base other_) { 1887 if (!super.equalsShallow(other_)) 1888 return false; 1889 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 1890 return false; 1891 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 1892 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 1893 } 1894 1895 public boolean isEmpty() { 1896 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, additionalUse 1897 , value); 1898 } 1899 1900 public String fhirType() { 1901 return "ValueSet.compose.include.concept.designation"; 1902 1903 } 1904 1905 } 1906 1907 @Block() 1908 public static class ConceptSetFilterComponent extends BackboneElement implements IBaseBackboneElement { 1909 /** 1910 * A code that identifies a property or a filter defined in the code system. 1911 */ 1912 @Child(name = "property", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1913 @Description(shortDefinition="A property/filter defined by the code system", formalDefinition="A code that identifies a property or a filter defined in the code system." ) 1914 protected CodeType property; 1915 1916 /** 1917 * The kind of operation to perform as a part of the filter criteria. 1918 */ 1919 @Child(name = "op", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1920 @Description(shortDefinition="= | is-a | descendent-of | is-not-a | regex | in | not-in | generalizes | child-of | descendent-leaf | exists", formalDefinition="The kind of operation to perform as a part of the filter criteria." ) 1921 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/filter-operator") 1922 protected Enumeration<FilterOperator> op; 1923 1924 /** 1925 * The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 1926 */ 1927 @Child(name = "value", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1928 @Description(shortDefinition="Code from the system, or regex criteria, or boolean value for exists", formalDefinition="The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'." ) 1929 protected StringType value; 1930 1931 private static final long serialVersionUID = 1259153492L; 1932 1933 /** 1934 * Constructor 1935 */ 1936 public ConceptSetFilterComponent() { 1937 super(); 1938 } 1939 1940 /** 1941 * Constructor 1942 */ 1943 public ConceptSetFilterComponent(String property, FilterOperator op, String value) { 1944 super(); 1945 this.setProperty(property); 1946 this.setOp(op); 1947 this.setValue(value); 1948 } 1949 1950 /** 1951 * @return {@link #property} (A code that identifies a property or a filter defined in the code system.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1952 */ 1953 public CodeType getPropertyElement() { 1954 if (this.property == null) 1955 if (Configuration.errorOnAutoCreate()) 1956 throw new Error("Attempt to auto-create ConceptSetFilterComponent.property"); 1957 else if (Configuration.doAutoCreate()) 1958 this.property = new CodeType(); // bb 1959 return this.property; 1960 } 1961 1962 public boolean hasPropertyElement() { 1963 return this.property != null && !this.property.isEmpty(); 1964 } 1965 1966 public boolean hasProperty() { 1967 return this.property != null && !this.property.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #property} (A code that identifies a property or a filter defined in the code system.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1972 */ 1973 public ConceptSetFilterComponent setPropertyElement(CodeType value) { 1974 this.property = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return A code that identifies a property or a filter defined in the code system. 1980 */ 1981 public String getProperty() { 1982 return this.property == null ? null : this.property.getValue(); 1983 } 1984 1985 /** 1986 * @param value A code that identifies a property or a filter defined in the code system. 1987 */ 1988 public ConceptSetFilterComponent setProperty(String value) { 1989 if (this.property == null) 1990 this.property = new CodeType(); 1991 this.property.setValue(value); 1992 return this; 1993 } 1994 1995 /** 1996 * @return {@link #op} (The kind of operation to perform as a part of the filter criteria.). This is the underlying object with id, value and extensions. The accessor "getOp" gives direct access to the value 1997 */ 1998 public Enumeration<FilterOperator> getOpElement() { 1999 if (this.op == null) 2000 if (Configuration.errorOnAutoCreate()) 2001 throw new Error("Attempt to auto-create ConceptSetFilterComponent.op"); 2002 else if (Configuration.doAutoCreate()) 2003 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); // bb 2004 return this.op; 2005 } 2006 2007 public boolean hasOpElement() { 2008 return this.op != null && !this.op.isEmpty(); 2009 } 2010 2011 public boolean hasOp() { 2012 return this.op != null && !this.op.isEmpty(); 2013 } 2014 2015 /** 2016 * @param value {@link #op} (The kind of operation to perform as a part of the filter criteria.). This is the underlying object with id, value and extensions. The accessor "getOp" gives direct access to the value 2017 */ 2018 public ConceptSetFilterComponent setOpElement(Enumeration<FilterOperator> value) { 2019 this.op = value; 2020 return this; 2021 } 2022 2023 /** 2024 * @return The kind of operation to perform as a part of the filter criteria. 2025 */ 2026 public FilterOperator getOp() { 2027 return this.op == null ? null : this.op.getValue(); 2028 } 2029 2030 /** 2031 * @param value The kind of operation to perform as a part of the filter criteria. 2032 */ 2033 public ConceptSetFilterComponent setOp(FilterOperator value) { 2034 if (this.op == null) 2035 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 2036 this.op.setValue(value); 2037 return this; 2038 } 2039 2040 /** 2041 * @return {@link #value} (The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2042 */ 2043 public StringType getValueElement() { 2044 if (this.value == null) 2045 if (Configuration.errorOnAutoCreate()) 2046 throw new Error("Attempt to auto-create ConceptSetFilterComponent.value"); 2047 else if (Configuration.doAutoCreate()) 2048 this.value = new StringType(); // bb 2049 return this.value; 2050 } 2051 2052 public boolean hasValueElement() { 2053 return this.value != null && !this.value.isEmpty(); 2054 } 2055 2056 public boolean hasValue() { 2057 return this.value != null && !this.value.isEmpty(); 2058 } 2059 2060 /** 2061 * @param value {@link #value} (The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2062 */ 2063 public ConceptSetFilterComponent setValueElement(StringType value) { 2064 this.value = value; 2065 return this; 2066 } 2067 2068 /** 2069 * @return The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 2070 */ 2071 public String getValue() { 2072 return this.value == null ? null : this.value.getValue(); 2073 } 2074 2075 /** 2076 * @param value The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 2077 */ 2078 public ConceptSetFilterComponent setValue(String value) { 2079 if (this.value == null) 2080 this.value = new StringType(); 2081 this.value.setValue(value); 2082 return this; 2083 } 2084 2085 protected void listChildren(List<Property> children) { 2086 super.listChildren(children); 2087 children.add(new Property("property", "code", "A code that identifies a property or a filter defined in the code system.", 0, 1, property)); 2088 children.add(new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 0, 1, op)); 2089 children.add(new Property("value", "string", "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 0, 1, value)); 2090 } 2091 2092 @Override 2093 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2094 switch (_hash) { 2095 case -993141291: /*property*/ return new Property("property", "code", "A code that identifies a property or a filter defined in the code system.", 0, 1, property); 2096 case 3553: /*op*/ return new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 0, 1, op); 2097 case 111972721: /*value*/ return new Property("value", "string", "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 0, 1, value); 2098 default: return super.getNamedProperty(_hash, _name, _checkValid); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2105 switch (hash) { 2106 case -993141291: /*property*/ return this.property == null ? new Base[0] : new Base[] {this.property}; // CodeType 2107 case 3553: /*op*/ return this.op == null ? new Base[0] : new Base[] {this.op}; // Enumeration<FilterOperator> 2108 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 2109 default: return super.getProperty(hash, name, checkValid); 2110 } 2111 2112 } 2113 2114 @Override 2115 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2116 switch (hash) { 2117 case -993141291: // property 2118 this.property = TypeConvertor.castToCode(value); // CodeType 2119 return value; 2120 case 3553: // op 2121 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2122 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2123 return value; 2124 case 111972721: // value 2125 this.value = TypeConvertor.castToString(value); // StringType 2126 return value; 2127 default: return super.setProperty(hash, name, value); 2128 } 2129 2130 } 2131 2132 @Override 2133 public Base setProperty(String name, Base value) throws FHIRException { 2134 if (name.equals("property")) { 2135 this.property = TypeConvertor.castToCode(value); // CodeType 2136 } else if (name.equals("op")) { 2137 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2138 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2139 } else if (name.equals("value")) { 2140 this.value = TypeConvertor.castToString(value); // StringType 2141 } else 2142 return super.setProperty(name, value); 2143 return value; 2144 } 2145 2146 @Override 2147 public void removeChild(String name, Base value) throws FHIRException { 2148 if (name.equals("property")) { 2149 this.property = null; 2150 } else if (name.equals("op")) { 2151 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2152 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2153 } else if (name.equals("value")) { 2154 this.value = null; 2155 } else 2156 super.removeChild(name, value); 2157 2158 } 2159 2160 @Override 2161 public Base makeProperty(int hash, String name) throws FHIRException { 2162 switch (hash) { 2163 case -993141291: return getPropertyElement(); 2164 case 3553: return getOpElement(); 2165 case 111972721: return getValueElement(); 2166 default: return super.makeProperty(hash, name); 2167 } 2168 2169 } 2170 2171 @Override 2172 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2173 switch (hash) { 2174 case -993141291: /*property*/ return new String[] {"code"}; 2175 case 3553: /*op*/ return new String[] {"code"}; 2176 case 111972721: /*value*/ return new String[] {"string"}; 2177 default: return super.getTypesForProperty(hash, name); 2178 } 2179 2180 } 2181 2182 @Override 2183 public Base addChild(String name) throws FHIRException { 2184 if (name.equals("property")) { 2185 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.filter.property"); 2186 } 2187 else if (name.equals("op")) { 2188 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.filter.op"); 2189 } 2190 else if (name.equals("value")) { 2191 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.compose.include.filter.value"); 2192 } 2193 else 2194 return super.addChild(name); 2195 } 2196 2197 public ConceptSetFilterComponent copy() { 2198 ConceptSetFilterComponent dst = new ConceptSetFilterComponent(); 2199 copyValues(dst); 2200 return dst; 2201 } 2202 2203 public void copyValues(ConceptSetFilterComponent dst) { 2204 super.copyValues(dst); 2205 dst.property = property == null ? null : property.copy(); 2206 dst.op = op == null ? null : op.copy(); 2207 dst.value = value == null ? null : value.copy(); 2208 } 2209 2210 @Override 2211 public boolean equalsDeep(Base other_) { 2212 if (!super.equalsDeep(other_)) 2213 return false; 2214 if (!(other_ instanceof ConceptSetFilterComponent)) 2215 return false; 2216 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2217 return compareDeep(property, o.property, true) && compareDeep(op, o.op, true) && compareDeep(value, o.value, true) 2218 ; 2219 } 2220 2221 @Override 2222 public boolean equalsShallow(Base other_) { 2223 if (!super.equalsShallow(other_)) 2224 return false; 2225 if (!(other_ instanceof ConceptSetFilterComponent)) 2226 return false; 2227 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2228 return compareValues(property, o.property, true) && compareValues(op, o.op, true) && compareValues(value, o.value, true) 2229 ; 2230 } 2231 2232 public boolean isEmpty() { 2233 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, op, value); 2234 } 2235 2236 public String fhirType() { 2237 return "ValueSet.compose.include.filter"; 2238 2239 } 2240 2241 } 2242 2243 @Block() 2244 public static class ValueSetExpansionComponent extends BackboneElement implements IBaseBackboneElement { 2245 /** 2246 * An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier. 2247 */ 2248 @Child(name = "identifier", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2249 @Description(shortDefinition="Identifies the value set expansion (business identifier)", formalDefinition="An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier." ) 2250 protected UriType identifier; 2251 2252 /** 2253 * As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them. 2254 */ 2255 @Child(name = "next", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2256 @Description(shortDefinition="Opaque urls for paging through expansion results", formalDefinition="As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them." ) 2257 protected UriType next; 2258 2259 /** 2260 * The time at which the expansion was produced by the expanding system. 2261 */ 2262 @Child(name = "timestamp", type = {DateTimeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 2263 @Description(shortDefinition="Time ValueSet expansion happened", formalDefinition="The time at which the expansion was produced by the expanding system." ) 2264 protected DateTimeType timestamp; 2265 2266 /** 2267 * The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2268 */ 2269 @Child(name = "total", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2270 @Description(shortDefinition="Total number of codes in the expansion", formalDefinition="The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter." ) 2271 protected IntegerType total; 2272 2273 /** 2274 * If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present. 2275 */ 2276 @Child(name = "offset", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2277 @Description(shortDefinition="Offset at which this resource starts", formalDefinition="If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present." ) 2278 protected IntegerType offset; 2279 2280 /** 2281 * A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion. 2282 */ 2283 @Child(name = "parameter", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2284 @Description(shortDefinition="Parameter that controlled the expansion process", formalDefinition="A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion." ) 2285 protected List<ValueSetExpansionParameterComponent> parameter; 2286 2287 /** 2288 * A property defines an additional slot through which additional information can be provided about a concept. 2289 */ 2290 @Child(name = "property", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2291 @Description(shortDefinition="Additional information supplied about each concept", formalDefinition="A property defines an additional slot through which additional information can be provided about a concept." ) 2292 protected List<ValueSetExpansionPropertyComponent> property; 2293 2294 /** 2295 * The codes that are contained in the value set expansion. 2296 */ 2297 @Child(name = "contains", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2298 @Description(shortDefinition="Codes in the value set", formalDefinition="The codes that are contained in the value set expansion." ) 2299 protected List<ValueSetExpansionContainsComponent> contains; 2300 2301 private static final long serialVersionUID = 1141573269L; 2302 2303 /** 2304 * Constructor 2305 */ 2306 public ValueSetExpansionComponent() { 2307 super(); 2308 } 2309 2310 /** 2311 * Constructor 2312 */ 2313 public ValueSetExpansionComponent(Date timestamp) { 2314 super(); 2315 this.setTimestamp(timestamp); 2316 } 2317 2318 /** 2319 * @return {@link #identifier} (An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 2320 */ 2321 public UriType getIdentifierElement() { 2322 if (this.identifier == null) 2323 if (Configuration.errorOnAutoCreate()) 2324 throw new Error("Attempt to auto-create ValueSetExpansionComponent.identifier"); 2325 else if (Configuration.doAutoCreate()) 2326 this.identifier = new UriType(); // bb 2327 return this.identifier; 2328 } 2329 2330 public boolean hasIdentifierElement() { 2331 return this.identifier != null && !this.identifier.isEmpty(); 2332 } 2333 2334 public boolean hasIdentifier() { 2335 return this.identifier != null && !this.identifier.isEmpty(); 2336 } 2337 2338 /** 2339 * @param value {@link #identifier} (An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 2340 */ 2341 public ValueSetExpansionComponent setIdentifierElement(UriType value) { 2342 this.identifier = value; 2343 return this; 2344 } 2345 2346 /** 2347 * @return An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier. 2348 */ 2349 public String getIdentifier() { 2350 return this.identifier == null ? null : this.identifier.getValue(); 2351 } 2352 2353 /** 2354 * @param value An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier. 2355 */ 2356 public ValueSetExpansionComponent setIdentifier(String value) { 2357 if (Utilities.noString(value)) 2358 this.identifier = null; 2359 else { 2360 if (this.identifier == null) 2361 this.identifier = new UriType(); 2362 this.identifier.setValue(value); 2363 } 2364 return this; 2365 } 2366 2367 /** 2368 * @return {@link #next} (As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them.). This is the underlying object with id, value and extensions. The accessor "getNext" gives direct access to the value 2369 */ 2370 public UriType getNextElement() { 2371 if (this.next == null) 2372 if (Configuration.errorOnAutoCreate()) 2373 throw new Error("Attempt to auto-create ValueSetExpansionComponent.next"); 2374 else if (Configuration.doAutoCreate()) 2375 this.next = new UriType(); // bb 2376 return this.next; 2377 } 2378 2379 public boolean hasNextElement() { 2380 return this.next != null && !this.next.isEmpty(); 2381 } 2382 2383 public boolean hasNext() { 2384 return this.next != null && !this.next.isEmpty(); 2385 } 2386 2387 /** 2388 * @param value {@link #next} (As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them.). This is the underlying object with id, value and extensions. The accessor "getNext" gives direct access to the value 2389 */ 2390 public ValueSetExpansionComponent setNextElement(UriType value) { 2391 this.next = value; 2392 return this; 2393 } 2394 2395 /** 2396 * @return As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them. 2397 */ 2398 public String getNext() { 2399 return this.next == null ? null : this.next.getValue(); 2400 } 2401 2402 /** 2403 * @param value As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them. 2404 */ 2405 public ValueSetExpansionComponent setNext(String value) { 2406 if (Utilities.noString(value)) 2407 this.next = null; 2408 else { 2409 if (this.next == null) 2410 this.next = new UriType(); 2411 this.next.setValue(value); 2412 } 2413 return this; 2414 } 2415 2416 /** 2417 * @return {@link #timestamp} (The time at which the expansion was produced by the expanding system.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 2418 */ 2419 public DateTimeType getTimestampElement() { 2420 if (this.timestamp == null) 2421 if (Configuration.errorOnAutoCreate()) 2422 throw new Error("Attempt to auto-create ValueSetExpansionComponent.timestamp"); 2423 else if (Configuration.doAutoCreate()) 2424 this.timestamp = new DateTimeType(); // bb 2425 return this.timestamp; 2426 } 2427 2428 public boolean hasTimestampElement() { 2429 return this.timestamp != null && !this.timestamp.isEmpty(); 2430 } 2431 2432 public boolean hasTimestamp() { 2433 return this.timestamp != null && !this.timestamp.isEmpty(); 2434 } 2435 2436 /** 2437 * @param value {@link #timestamp} (The time at which the expansion was produced by the expanding system.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 2438 */ 2439 public ValueSetExpansionComponent setTimestampElement(DateTimeType value) { 2440 this.timestamp = value; 2441 return this; 2442 } 2443 2444 /** 2445 * @return The time at which the expansion was produced by the expanding system. 2446 */ 2447 public Date getTimestamp() { 2448 return this.timestamp == null ? null : this.timestamp.getValue(); 2449 } 2450 2451 /** 2452 * @param value The time at which the expansion was produced by the expanding system. 2453 */ 2454 public ValueSetExpansionComponent setTimestamp(Date value) { 2455 if (this.timestamp == null) 2456 this.timestamp = new DateTimeType(); 2457 this.timestamp.setValue(value); 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #total} (The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2463 */ 2464 public IntegerType getTotalElement() { 2465 if (this.total == null) 2466 if (Configuration.errorOnAutoCreate()) 2467 throw new Error("Attempt to auto-create ValueSetExpansionComponent.total"); 2468 else if (Configuration.doAutoCreate()) 2469 this.total = new IntegerType(); // bb 2470 return this.total; 2471 } 2472 2473 public boolean hasTotalElement() { 2474 return this.total != null && !this.total.isEmpty(); 2475 } 2476 2477 public boolean hasTotal() { 2478 return this.total != null && !this.total.isEmpty(); 2479 } 2480 2481 /** 2482 * @param value {@link #total} (The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2483 */ 2484 public ValueSetExpansionComponent setTotalElement(IntegerType value) { 2485 this.total = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2491 */ 2492 public int getTotal() { 2493 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2494 } 2495 2496 /** 2497 * @param value The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2498 */ 2499 public ValueSetExpansionComponent setTotal(int value) { 2500 if (this.total == null) 2501 this.total = new IntegerType(); 2502 this.total.setValue(value); 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #offset} (If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 2508 */ 2509 public IntegerType getOffsetElement() { 2510 if (this.offset == null) 2511 if (Configuration.errorOnAutoCreate()) 2512 throw new Error("Attempt to auto-create ValueSetExpansionComponent.offset"); 2513 else if (Configuration.doAutoCreate()) 2514 this.offset = new IntegerType(); // bb 2515 return this.offset; 2516 } 2517 2518 public boolean hasOffsetElement() { 2519 return this.offset != null && !this.offset.isEmpty(); 2520 } 2521 2522 public boolean hasOffset() { 2523 return this.offset != null && !this.offset.isEmpty(); 2524 } 2525 2526 /** 2527 * @param value {@link #offset} (If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 2528 */ 2529 public ValueSetExpansionComponent setOffsetElement(IntegerType value) { 2530 this.offset = value; 2531 return this; 2532 } 2533 2534 /** 2535 * @return If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present. 2536 */ 2537 public int getOffset() { 2538 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 2539 } 2540 2541 /** 2542 * @param value If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present. 2543 */ 2544 public ValueSetExpansionComponent setOffset(int value) { 2545 if (this.offset == null) 2546 this.offset = new IntegerType(); 2547 this.offset.setValue(value); 2548 return this; 2549 } 2550 2551 /** 2552 * @return {@link #parameter} (A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.) 2553 */ 2554 public List<ValueSetExpansionParameterComponent> getParameter() { 2555 if (this.parameter == null) 2556 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2557 return this.parameter; 2558 } 2559 2560 /** 2561 * @return Returns a reference to <code>this</code> for easy method chaining 2562 */ 2563 public ValueSetExpansionComponent setParameter(List<ValueSetExpansionParameterComponent> theParameter) { 2564 this.parameter = theParameter; 2565 return this; 2566 } 2567 2568 public boolean hasParameter() { 2569 if (this.parameter == null) 2570 return false; 2571 for (ValueSetExpansionParameterComponent item : this.parameter) 2572 if (!item.isEmpty()) 2573 return true; 2574 return false; 2575 } 2576 2577 public ValueSetExpansionParameterComponent addParameter() { //3 2578 ValueSetExpansionParameterComponent t = new ValueSetExpansionParameterComponent(); 2579 if (this.parameter == null) 2580 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2581 this.parameter.add(t); 2582 return t; 2583 } 2584 2585 public ValueSetExpansionComponent addParameter(ValueSetExpansionParameterComponent t) { //3 2586 if (t == null) 2587 return this; 2588 if (this.parameter == null) 2589 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2590 this.parameter.add(t); 2591 return this; 2592 } 2593 2594 /** 2595 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 2596 */ 2597 public ValueSetExpansionParameterComponent getParameterFirstRep() { 2598 if (getParameter().isEmpty()) { 2599 addParameter(); 2600 } 2601 return getParameter().get(0); 2602 } 2603 2604 /** 2605 * @return {@link #property} (A property defines an additional slot through which additional information can be provided about a concept.) 2606 */ 2607 public List<ValueSetExpansionPropertyComponent> getProperty() { 2608 if (this.property == null) 2609 this.property = new ArrayList<ValueSetExpansionPropertyComponent>(); 2610 return this.property; 2611 } 2612 2613 /** 2614 * @return Returns a reference to <code>this</code> for easy method chaining 2615 */ 2616 public ValueSetExpansionComponent setProperty(List<ValueSetExpansionPropertyComponent> theProperty) { 2617 this.property = theProperty; 2618 return this; 2619 } 2620 2621 public boolean hasProperty() { 2622 if (this.property == null) 2623 return false; 2624 for (ValueSetExpansionPropertyComponent item : this.property) 2625 if (!item.isEmpty()) 2626 return true; 2627 return false; 2628 } 2629 2630 public ValueSetExpansionPropertyComponent addProperty() { //3 2631 ValueSetExpansionPropertyComponent t = new ValueSetExpansionPropertyComponent(); 2632 if (this.property == null) 2633 this.property = new ArrayList<ValueSetExpansionPropertyComponent>(); 2634 this.property.add(t); 2635 return t; 2636 } 2637 2638 public ValueSetExpansionComponent addProperty(ValueSetExpansionPropertyComponent t) { //3 2639 if (t == null) 2640 return this; 2641 if (this.property == null) 2642 this.property = new ArrayList<ValueSetExpansionPropertyComponent>(); 2643 this.property.add(t); 2644 return this; 2645 } 2646 2647 /** 2648 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 2649 */ 2650 public ValueSetExpansionPropertyComponent getPropertyFirstRep() { 2651 if (getProperty().isEmpty()) { 2652 addProperty(); 2653 } 2654 return getProperty().get(0); 2655 } 2656 2657 /** 2658 * @return {@link #contains} (The codes that are contained in the value set expansion.) 2659 */ 2660 public List<ValueSetExpansionContainsComponent> getContains() { 2661 if (this.contains == null) 2662 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2663 return this.contains; 2664 } 2665 2666 /** 2667 * @return Returns a reference to <code>this</code> for easy method chaining 2668 */ 2669 public ValueSetExpansionComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 2670 this.contains = theContains; 2671 return this; 2672 } 2673 2674 public boolean hasContains() { 2675 if (this.contains == null) 2676 return false; 2677 for (ValueSetExpansionContainsComponent item : this.contains) 2678 if (!item.isEmpty()) 2679 return true; 2680 return false; 2681 } 2682 2683 public ValueSetExpansionContainsComponent addContains() { //3 2684 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 2685 if (this.contains == null) 2686 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2687 this.contains.add(t); 2688 return t; 2689 } 2690 2691 public ValueSetExpansionComponent addContains(ValueSetExpansionContainsComponent t) { //3 2692 if (t == null) 2693 return this; 2694 if (this.contains == null) 2695 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2696 this.contains.add(t); 2697 return this; 2698 } 2699 2700 /** 2701 * @return The first repetition of repeating field {@link #contains}, creating it if it does not already exist {3} 2702 */ 2703 public ValueSetExpansionContainsComponent getContainsFirstRep() { 2704 if (getContains().isEmpty()) { 2705 addContains(); 2706 } 2707 return getContains().get(0); 2708 } 2709 2710 protected void listChildren(List<Property> children) { 2711 super.listChildren(children); 2712 children.add(new Property("identifier", "uri", "An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.", 0, 1, identifier)); 2713 children.add(new Property("next", "uri", "As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them.", 0, 1, next)); 2714 children.add(new Property("timestamp", "dateTime", "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp)); 2715 children.add(new Property("total", "integer", "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 0, 1, total)); 2716 children.add(new Property("offset", "integer", "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.", 0, 1, offset)); 2717 children.add(new Property("parameter", "", "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2718 children.add(new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property)); 2719 children.add(new Property("contains", "", "The codes that are contained in the value set expansion.", 0, java.lang.Integer.MAX_VALUE, contains)); 2720 } 2721 2722 @Override 2723 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2724 switch (_hash) { 2725 case -1618432855: /*identifier*/ return new Property("identifier", "uri", "An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.", 0, 1, identifier); 2726 case 3377907: /*next*/ return new Property("next", "uri", "As per paging Search results, the next URLs are opaque to the client, have no dictated structure, and only the server understands them.", 0, 1, next); 2727 case 55126294: /*timestamp*/ return new Property("timestamp", "dateTime", "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp); 2728 case 110549828: /*total*/ return new Property("total", "integer", "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 0, 1, total); 2729 case -1019779949: /*offset*/ return new Property("offset", "integer", "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.", 0, 1, offset); 2730 case 1954460585: /*parameter*/ return new Property("parameter", "", "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 0, java.lang.Integer.MAX_VALUE, parameter); 2731 case -993141291: /*property*/ return new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property); 2732 case -567445985: /*contains*/ return new Property("contains", "", "The codes that are contained in the value set expansion.", 0, java.lang.Integer.MAX_VALUE, contains); 2733 default: return super.getNamedProperty(_hash, _name, _checkValid); 2734 } 2735 2736 } 2737 2738 @Override 2739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2740 switch (hash) { 2741 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // UriType 2742 case 3377907: /*next*/ return this.next == null ? new Base[0] : new Base[] {this.next}; // UriType 2743 case 55126294: /*timestamp*/ return this.timestamp == null ? new Base[0] : new Base[] {this.timestamp}; // DateTimeType 2744 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // IntegerType 2745 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // IntegerType 2746 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ValueSetExpansionParameterComponent 2747 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ValueSetExpansionPropertyComponent 2748 case -567445985: /*contains*/ return this.contains == null ? new Base[0] : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 2749 default: return super.getProperty(hash, name, checkValid); 2750 } 2751 2752 } 2753 2754 @Override 2755 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2756 switch (hash) { 2757 case -1618432855: // identifier 2758 this.identifier = TypeConvertor.castToUri(value); // UriType 2759 return value; 2760 case 3377907: // next 2761 this.next = TypeConvertor.castToUri(value); // UriType 2762 return value; 2763 case 55126294: // timestamp 2764 this.timestamp = TypeConvertor.castToDateTime(value); // DateTimeType 2765 return value; 2766 case 110549828: // total 2767 this.total = TypeConvertor.castToInteger(value); // IntegerType 2768 return value; 2769 case -1019779949: // offset 2770 this.offset = TypeConvertor.castToInteger(value); // IntegerType 2771 return value; 2772 case 1954460585: // parameter 2773 this.getParameter().add((ValueSetExpansionParameterComponent) value); // ValueSetExpansionParameterComponent 2774 return value; 2775 case -993141291: // property 2776 this.getProperty().add((ValueSetExpansionPropertyComponent) value); // ValueSetExpansionPropertyComponent 2777 return value; 2778 case -567445985: // contains 2779 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 2780 return value; 2781 default: return super.setProperty(hash, name, value); 2782 } 2783 2784 } 2785 2786 @Override 2787 public Base setProperty(String name, Base value) throws FHIRException { 2788 if (name.equals("identifier")) { 2789 this.identifier = TypeConvertor.castToUri(value); // UriType 2790 } else if (name.equals("next")) { 2791 this.next = TypeConvertor.castToUri(value); // UriType 2792 } else if (name.equals("timestamp")) { 2793 this.timestamp = TypeConvertor.castToDateTime(value); // DateTimeType 2794 } else if (name.equals("total")) { 2795 this.total = TypeConvertor.castToInteger(value); // IntegerType 2796 } else if (name.equals("offset")) { 2797 this.offset = TypeConvertor.castToInteger(value); // IntegerType 2798 } else if (name.equals("parameter")) { 2799 this.getParameter().add((ValueSetExpansionParameterComponent) value); 2800 } else if (name.equals("property")) { 2801 this.getProperty().add((ValueSetExpansionPropertyComponent) value); 2802 } else if (name.equals("contains")) { 2803 this.getContains().add((ValueSetExpansionContainsComponent) value); 2804 } else 2805 return super.setProperty(name, value); 2806 return value; 2807 } 2808 2809 @Override 2810 public void removeChild(String name, Base value) throws FHIRException { 2811 if (name.equals("identifier")) { 2812 this.identifier = null; 2813 } else if (name.equals("next")) { 2814 this.next = null; 2815 } else if (name.equals("timestamp")) { 2816 this.timestamp = null; 2817 } else if (name.equals("total")) { 2818 this.total = null; 2819 } else if (name.equals("offset")) { 2820 this.offset = null; 2821 } else if (name.equals("parameter")) { 2822 this.getParameter().remove((ValueSetExpansionParameterComponent) value); 2823 } else if (name.equals("property")) { 2824 this.getProperty().remove((ValueSetExpansionPropertyComponent) value); 2825 } else if (name.equals("contains")) { 2826 this.getContains().remove((ValueSetExpansionContainsComponent) value); 2827 } else 2828 super.removeChild(name, value); 2829 2830 } 2831 2832 @Override 2833 public Base makeProperty(int hash, String name) throws FHIRException { 2834 switch (hash) { 2835 case -1618432855: return getIdentifierElement(); 2836 case 3377907: return getNextElement(); 2837 case 55126294: return getTimestampElement(); 2838 case 110549828: return getTotalElement(); 2839 case -1019779949: return getOffsetElement(); 2840 case 1954460585: return addParameter(); 2841 case -993141291: return addProperty(); 2842 case -567445985: return addContains(); 2843 default: return super.makeProperty(hash, name); 2844 } 2845 2846 } 2847 2848 @Override 2849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2850 switch (hash) { 2851 case -1618432855: /*identifier*/ return new String[] {"uri"}; 2852 case 3377907: /*next*/ return new String[] {"uri"}; 2853 case 55126294: /*timestamp*/ return new String[] {"dateTime"}; 2854 case 110549828: /*total*/ return new String[] {"integer"}; 2855 case -1019779949: /*offset*/ return new String[] {"integer"}; 2856 case 1954460585: /*parameter*/ return new String[] {}; 2857 case -993141291: /*property*/ return new String[] {}; 2858 case -567445985: /*contains*/ return new String[] {}; 2859 default: return super.getTypesForProperty(hash, name); 2860 } 2861 2862 } 2863 2864 @Override 2865 public Base addChild(String name) throws FHIRException { 2866 if (name.equals("identifier")) { 2867 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.identifier"); 2868 } 2869 else if (name.equals("next")) { 2870 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.next"); 2871 } 2872 else if (name.equals("timestamp")) { 2873 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.timestamp"); 2874 } 2875 else if (name.equals("total")) { 2876 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.total"); 2877 } 2878 else if (name.equals("offset")) { 2879 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.offset"); 2880 } 2881 else if (name.equals("parameter")) { 2882 return addParameter(); 2883 } 2884 else if (name.equals("property")) { 2885 return addProperty(); 2886 } 2887 else if (name.equals("contains")) { 2888 return addContains(); 2889 } 2890 else 2891 return super.addChild(name); 2892 } 2893 2894 public ValueSetExpansionComponent copy() { 2895 ValueSetExpansionComponent dst = new ValueSetExpansionComponent(); 2896 copyValues(dst); 2897 return dst; 2898 } 2899 2900 public void copyValues(ValueSetExpansionComponent dst) { 2901 super.copyValues(dst); 2902 dst.identifier = identifier == null ? null : identifier.copy(); 2903 dst.next = next == null ? null : next.copy(); 2904 dst.timestamp = timestamp == null ? null : timestamp.copy(); 2905 dst.total = total == null ? null : total.copy(); 2906 dst.offset = offset == null ? null : offset.copy(); 2907 if (parameter != null) { 2908 dst.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2909 for (ValueSetExpansionParameterComponent i : parameter) 2910 dst.parameter.add(i.copy()); 2911 }; 2912 if (property != null) { 2913 dst.property = new ArrayList<ValueSetExpansionPropertyComponent>(); 2914 for (ValueSetExpansionPropertyComponent i : property) 2915 dst.property.add(i.copy()); 2916 }; 2917 if (contains != null) { 2918 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2919 for (ValueSetExpansionContainsComponent i : contains) 2920 dst.contains.add(i.copy()); 2921 }; 2922 } 2923 2924 @Override 2925 public boolean equalsDeep(Base other_) { 2926 if (!super.equalsDeep(other_)) 2927 return false; 2928 if (!(other_ instanceof ValueSetExpansionComponent)) 2929 return false; 2930 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 2931 return compareDeep(identifier, o.identifier, true) && compareDeep(next, o.next, true) && compareDeep(timestamp, o.timestamp, true) 2932 && compareDeep(total, o.total, true) && compareDeep(offset, o.offset, true) && compareDeep(parameter, o.parameter, true) 2933 && compareDeep(property, o.property, true) && compareDeep(contains, o.contains, true); 2934 } 2935 2936 @Override 2937 public boolean equalsShallow(Base other_) { 2938 if (!super.equalsShallow(other_)) 2939 return false; 2940 if (!(other_ instanceof ValueSetExpansionComponent)) 2941 return false; 2942 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 2943 return compareValues(identifier, o.identifier, true) && compareValues(next, o.next, true) && compareValues(timestamp, o.timestamp, true) 2944 && compareValues(total, o.total, true) && compareValues(offset, o.offset, true); 2945 } 2946 2947 public boolean isEmpty() { 2948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, next, timestamp 2949 , total, offset, parameter, property, contains); 2950 } 2951 2952 public boolean hasParameterValue(String name, String value) { 2953 for (ValueSetExpansionParameterComponent p : getParameter()) { 2954 if (name.equals(p.getName()) && p.hasValue() && value.equals(p.getValue().primitiveValue())) { 2955 return true; 2956 } 2957 } 2958 return false; 2959 } 2960 2961 public void addParameter(String name, DataType value) { 2962 getParameter().add(new ValueSetExpansionParameterComponent(name).setValue(value)); 2963 } 2964 2965 public String fhirType() { 2966 return "ValueSet.expansion"; 2967 } 2968 2969 } 2970 2971 @Block() 2972 public static class ValueSetExpansionParameterComponent extends BackboneElement implements IBaseBackboneElement { 2973 /** 2974 * Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process. 2975 */ 2976 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2977 @Description(shortDefinition="Name as assigned by the client or server", formalDefinition="Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process." ) 2978 protected StringType name; 2979 2980 /** 2981 * The value of the parameter. 2982 */ 2983 @Child(name = "value", type = {StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, UriType.class, CodeType.class, DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2984 @Description(shortDefinition="Value of the named parameter", formalDefinition="The value of the parameter." ) 2985 protected DataType value; 2986 2987 private static final long serialVersionUID = 1839679495L; 2988 2989 /** 2990 * Constructor 2991 */ 2992 public ValueSetExpansionParameterComponent() { 2993 super(); 2994 } 2995 2996 /** 2997 * Constructor 2998 */ 2999 public ValueSetExpansionParameterComponent(String name) { 3000 super(); 3001 this.setName(name); 3002 } 3003 3004 /** 3005 * @return {@link #name} (Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3006 */ 3007 public StringType getNameElement() { 3008 if (this.name == null) 3009 if (Configuration.errorOnAutoCreate()) 3010 throw new Error("Attempt to auto-create ValueSetExpansionParameterComponent.name"); 3011 else if (Configuration.doAutoCreate()) 3012 this.name = new StringType(); // bb 3013 return this.name; 3014 } 3015 3016 public boolean hasNameElement() { 3017 return this.name != null && !this.name.isEmpty(); 3018 } 3019 3020 public boolean hasName() { 3021 return this.name != null && !this.name.isEmpty(); 3022 } 3023 3024 /** 3025 * @param value {@link #name} (Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3026 */ 3027 public ValueSetExpansionParameterComponent setNameElement(StringType value) { 3028 this.name = value; 3029 return this; 3030 } 3031 3032 /** 3033 * @return Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process. 3034 */ 3035 public String getName() { 3036 return this.name == null ? null : this.name.getValue(); 3037 } 3038 3039 /** 3040 * @param value Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process. 3041 */ 3042 public ValueSetExpansionParameterComponent setName(String value) { 3043 if (this.name == null) 3044 this.name = new StringType(); 3045 this.name.setValue(value); 3046 return this; 3047 } 3048 3049 /** 3050 * @return {@link #value} (The value of the parameter.) 3051 */ 3052 public DataType getValue() { 3053 return this.value; 3054 } 3055 3056 /** 3057 * @return {@link #value} (The value of the parameter.) 3058 */ 3059 public StringType getValueStringType() throws FHIRException { 3060 if (this.value == null) 3061 this.value = new StringType(); 3062 if (!(this.value instanceof StringType)) 3063 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3064 return (StringType) this.value; 3065 } 3066 3067 public boolean hasValueStringType() { 3068 return this != null && this.value instanceof StringType; 3069 } 3070 3071 /** 3072 * @return {@link #value} (The value of the parameter.) 3073 */ 3074 public BooleanType getValueBooleanType() throws FHIRException { 3075 if (this.value == null) 3076 this.value = new BooleanType(); 3077 if (!(this.value instanceof BooleanType)) 3078 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3079 return (BooleanType) this.value; 3080 } 3081 3082 public boolean hasValueBooleanType() { 3083 return this != null && this.value instanceof BooleanType; 3084 } 3085 3086 /** 3087 * @return {@link #value} (The value of the parameter.) 3088 */ 3089 public IntegerType getValueIntegerType() throws FHIRException { 3090 if (this.value == null) 3091 this.value = new IntegerType(); 3092 if (!(this.value instanceof IntegerType)) 3093 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 3094 return (IntegerType) this.value; 3095 } 3096 3097 public boolean hasValueIntegerType() { 3098 return this != null && this.value instanceof IntegerType; 3099 } 3100 3101 /** 3102 * @return {@link #value} (The value of the parameter.) 3103 */ 3104 public DecimalType getValueDecimalType() throws FHIRException { 3105 if (this.value == null) 3106 this.value = new DecimalType(); 3107 if (!(this.value instanceof DecimalType)) 3108 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 3109 return (DecimalType) this.value; 3110 } 3111 3112 public boolean hasValueDecimalType() { 3113 return this != null && this.value instanceof DecimalType; 3114 } 3115 3116 /** 3117 * @return {@link #value} (The value of the parameter.) 3118 */ 3119 public UriType getValueUriType() throws FHIRException { 3120 if (this.value == null) 3121 this.value = new UriType(); 3122 if (!(this.value instanceof UriType)) 3123 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 3124 return (UriType) this.value; 3125 } 3126 3127 public boolean hasValueUriType() { 3128 return this != null && this.value instanceof UriType; 3129 } 3130 3131 /** 3132 * @return {@link #value} (The value of the parameter.) 3133 */ 3134 public CodeType getValueCodeType() throws FHIRException { 3135 if (this.value == null) 3136 this.value = new CodeType(); 3137 if (!(this.value instanceof CodeType)) 3138 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3139 return (CodeType) this.value; 3140 } 3141 3142 public boolean hasValueCodeType() { 3143 return this != null && this.value instanceof CodeType; 3144 } 3145 3146 /** 3147 * @return {@link #value} (The value of the parameter.) 3148 */ 3149 public DateTimeType getValueDateTimeType() throws FHIRException { 3150 if (this.value == null) 3151 this.value = new DateTimeType(); 3152 if (!(this.value instanceof DateTimeType)) 3153 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3154 return (DateTimeType) this.value; 3155 } 3156 3157 public boolean hasValueDateTimeType() { 3158 return this != null && this.value instanceof DateTimeType; 3159 } 3160 3161 public boolean hasValue() { 3162 return this.value != null && !this.value.isEmpty(); 3163 } 3164 3165 /** 3166 * @param value {@link #value} (The value of the parameter.) 3167 */ 3168 public ValueSetExpansionParameterComponent setValue(DataType value) { 3169 if (value != null && !(value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType || value instanceof UriType || value instanceof CodeType || value instanceof DateTimeType)) 3170 throw new FHIRException("Not the right type for ValueSet.expansion.parameter.value[x]: "+value.fhirType()); 3171 this.value = value; 3172 return this; 3173 } 3174 3175 protected void listChildren(List<Property> children) { 3176 super.listChildren(children); 3177 children.add(new Property("name", "string", "Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.", 0, 1, name)); 3178 children.add(new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", "The value of the parameter.", 0, 1, value)); 3179 } 3180 3181 @Override 3182 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3183 switch (_hash) { 3184 case 3373707: /*name*/ return new Property("name", "string", "Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.", 0, 1, name); 3185 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", "The value of the parameter.", 0, 1, value); 3186 case 111972721: /*value*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", "The value of the parameter.", 0, 1, value); 3187 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the parameter.", 0, 1, value); 3188 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the parameter.", 0, 1, value); 3189 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the parameter.", 0, 1, value); 3190 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the parameter.", 0, 1, value); 3191 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the parameter.", 0, 1, value); 3192 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of the parameter.", 0, 1, value); 3193 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the parameter.", 0, 1, value); 3194 default: return super.getNamedProperty(_hash, _name, _checkValid); 3195 } 3196 3197 } 3198 3199 @Override 3200 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3201 switch (hash) { 3202 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3203 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3204 default: return super.getProperty(hash, name, checkValid); 3205 } 3206 3207 } 3208 3209 @Override 3210 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3211 switch (hash) { 3212 case 3373707: // name 3213 this.name = TypeConvertor.castToString(value); // StringType 3214 return value; 3215 case 111972721: // value 3216 this.value = TypeConvertor.castToType(value); // DataType 3217 return value; 3218 default: return super.setProperty(hash, name, value); 3219 } 3220 3221 } 3222 3223 @Override 3224 public Base setProperty(String name, Base value) throws FHIRException { 3225 if (name.equals("name")) { 3226 this.name = TypeConvertor.castToString(value); // StringType 3227 } else if (name.equals("value[x]")) { 3228 this.value = TypeConvertor.castToType(value); // DataType 3229 } else 3230 return super.setProperty(name, value); 3231 return value; 3232 } 3233 3234 @Override 3235 public void removeChild(String name, Base value) throws FHIRException { 3236 if (name.equals("name")) { 3237 this.name = null; 3238 } else if (name.equals("value[x]")) { 3239 this.value = null; 3240 } else 3241 super.removeChild(name, value); 3242 3243 } 3244 3245 @Override 3246 public Base makeProperty(int hash, String name) throws FHIRException { 3247 switch (hash) { 3248 case 3373707: return getNameElement(); 3249 case -1410166417: return getValue(); 3250 case 111972721: return getValue(); 3251 default: return super.makeProperty(hash, name); 3252 } 3253 3254 } 3255 3256 @Override 3257 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3258 switch (hash) { 3259 case 3373707: /*name*/ return new String[] {"string"}; 3260 case 111972721: /*value*/ return new String[] {"string", "boolean", "integer", "decimal", "uri", "code", "dateTime"}; 3261 default: return super.getTypesForProperty(hash, name); 3262 } 3263 3264 } 3265 3266 @Override 3267 public Base addChild(String name) throws FHIRException { 3268 if (name.equals("name")) { 3269 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.parameter.name"); 3270 } 3271 else if (name.equals("valueString")) { 3272 this.value = new StringType(); 3273 return this.value; 3274 } 3275 else if (name.equals("valueBoolean")) { 3276 this.value = new BooleanType(); 3277 return this.value; 3278 } 3279 else if (name.equals("valueInteger")) { 3280 this.value = new IntegerType(); 3281 return this.value; 3282 } 3283 else if (name.equals("valueDecimal")) { 3284 this.value = new DecimalType(); 3285 return this.value; 3286 } 3287 else if (name.equals("valueUri")) { 3288 this.value = new UriType(); 3289 return this.value; 3290 } 3291 else if (name.equals("valueCode")) { 3292 this.value = new CodeType(); 3293 return this.value; 3294 } 3295 else if (name.equals("valueDateTime")) { 3296 this.value = new DateTimeType(); 3297 return this.value; 3298 } 3299 else 3300 return super.addChild(name); 3301 } 3302 3303 public ValueSetExpansionParameterComponent copy() { 3304 ValueSetExpansionParameterComponent dst = new ValueSetExpansionParameterComponent(); 3305 copyValues(dst); 3306 return dst; 3307 } 3308 3309 public void copyValues(ValueSetExpansionParameterComponent dst) { 3310 super.copyValues(dst); 3311 dst.name = name == null ? null : name.copy(); 3312 dst.value = value == null ? null : value.copy(); 3313 } 3314 3315 @Override 3316 public boolean equalsDeep(Base other_) { 3317 if (!super.equalsDeep(other_)) 3318 return false; 3319 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 3320 return false; 3321 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 3322 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 3323 } 3324 3325 @Override 3326 public boolean equalsShallow(Base other_) { 3327 if (!super.equalsShallow(other_)) 3328 return false; 3329 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 3330 return false; 3331 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 3332 return compareValues(name, o.name, true); 3333 } 3334 3335 public boolean isEmpty() { 3336 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 3337 } 3338 3339 public String fhirType() { 3340 return "ValueSet.expansion.parameter"; 3341 3342 } 3343 3344 @Override 3345 public String toString() { 3346 var s = (hasValue() ? getValue().primitiveValue() : ""); 3347 return getName() + "=" + s; 3348 } 3349 3350 } 3351 3352 @Block() 3353 public static class ValueSetExpansionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 3354 /** 3355 * A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code. 3356 */ 3357 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3358 @Description(shortDefinition="Identifies the property on the concepts, and when referred to in operations", formalDefinition="A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code." ) 3359 protected CodeType code; 3360 3361 /** 3362 * Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 3363 */ 3364 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3365 @Description(shortDefinition="Formal identifier for the property", formalDefinition="Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system." ) 3366 protected UriType uri; 3367 3368 private static final long serialVersionUID = 929575836L; 3369 3370 /** 3371 * Constructor 3372 */ 3373 public ValueSetExpansionPropertyComponent() { 3374 super(); 3375 } 3376 3377 /** 3378 * Constructor 3379 */ 3380 public ValueSetExpansionPropertyComponent(String code) { 3381 super(); 3382 this.setCode(code); 3383 } 3384 3385 /** 3386 * @return {@link #code} (A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3387 */ 3388 public CodeType getCodeElement() { 3389 if (this.code == null) 3390 if (Configuration.errorOnAutoCreate()) 3391 throw new Error("Attempt to auto-create ValueSetExpansionPropertyComponent.code"); 3392 else if (Configuration.doAutoCreate()) 3393 this.code = new CodeType(); // bb 3394 return this.code; 3395 } 3396 3397 public boolean hasCodeElement() { 3398 return this.code != null && !this.code.isEmpty(); 3399 } 3400 3401 public boolean hasCode() { 3402 return this.code != null && !this.code.isEmpty(); 3403 } 3404 3405 /** 3406 * @param value {@link #code} (A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3407 */ 3408 public ValueSetExpansionPropertyComponent setCodeElement(CodeType value) { 3409 this.code = value; 3410 return this; 3411 } 3412 3413 /** 3414 * @return A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code. 3415 */ 3416 public String getCode() { 3417 return this.code == null ? null : this.code.getValue(); 3418 } 3419 3420 /** 3421 * @param value A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code. 3422 */ 3423 public ValueSetExpansionPropertyComponent setCode(String value) { 3424 if (this.code == null) 3425 this.code = new CodeType(); 3426 this.code.setValue(value); 3427 return this; 3428 } 3429 3430 /** 3431 * @return {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 3432 */ 3433 public UriType getUriElement() { 3434 if (this.uri == null) 3435 if (Configuration.errorOnAutoCreate()) 3436 throw new Error("Attempt to auto-create ValueSetExpansionPropertyComponent.uri"); 3437 else if (Configuration.doAutoCreate()) 3438 this.uri = new UriType(); // bb 3439 return this.uri; 3440 } 3441 3442 public boolean hasUriElement() { 3443 return this.uri != null && !this.uri.isEmpty(); 3444 } 3445 3446 public boolean hasUri() { 3447 return this.uri != null && !this.uri.isEmpty(); 3448 } 3449 3450 /** 3451 * @param value {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 3452 */ 3453 public ValueSetExpansionPropertyComponent setUriElement(UriType value) { 3454 this.uri = value; 3455 return this; 3456 } 3457 3458 /** 3459 * @return Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 3460 */ 3461 public String getUri() { 3462 return this.uri == null ? null : this.uri.getValue(); 3463 } 3464 3465 /** 3466 * @param value Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 3467 */ 3468 public ValueSetExpansionPropertyComponent setUri(String value) { 3469 if (Utilities.noString(value)) 3470 this.uri = null; 3471 else { 3472 if (this.uri == null) 3473 this.uri = new UriType(); 3474 this.uri.setValue(value); 3475 } 3476 return this; 3477 } 3478 3479 protected void listChildren(List<Property> children) { 3480 super.listChildren(children); 3481 children.add(new Property("code", "code", "A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code.", 0, 1, code)); 3482 children.add(new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri)); 3483 } 3484 3485 @Override 3486 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3487 switch (_hash) { 3488 case 3059181: /*code*/ return new Property("code", "code", "A code that is used to identify the property. The code is used in ValueSet.expansion.contains.property.code.", 0, 1, code); 3489 case 116076: /*uri*/ return new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri); 3490 default: return super.getNamedProperty(_hash, _name, _checkValid); 3491 } 3492 3493 } 3494 3495 @Override 3496 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3497 switch (hash) { 3498 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 3499 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 3500 default: return super.getProperty(hash, name, checkValid); 3501 } 3502 3503 } 3504 3505 @Override 3506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3507 switch (hash) { 3508 case 3059181: // code 3509 this.code = TypeConvertor.castToCode(value); // CodeType 3510 return value; 3511 case 116076: // uri 3512 this.uri = TypeConvertor.castToUri(value); // UriType 3513 return value; 3514 default: return super.setProperty(hash, name, value); 3515 } 3516 3517 } 3518 3519 @Override 3520 public Base setProperty(String name, Base value) throws FHIRException { 3521 if (name.equals("code")) { 3522 this.code = TypeConvertor.castToCode(value); // CodeType 3523 } else if (name.equals("uri")) { 3524 this.uri = TypeConvertor.castToUri(value); // UriType 3525 } else 3526 return super.setProperty(name, value); 3527 return value; 3528 } 3529 3530 @Override 3531 public void removeChild(String name, Base value) throws FHIRException { 3532 if (name.equals("code")) { 3533 this.code = null; 3534 } else if (name.equals("uri")) { 3535 this.uri = null; 3536 } else 3537 super.removeChild(name, value); 3538 3539 } 3540 3541 @Override 3542 public Base makeProperty(int hash, String name) throws FHIRException { 3543 switch (hash) { 3544 case 3059181: return getCodeElement(); 3545 case 116076: return getUriElement(); 3546 default: return super.makeProperty(hash, name); 3547 } 3548 3549 } 3550 3551 @Override 3552 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3553 switch (hash) { 3554 case 3059181: /*code*/ return new String[] {"code"}; 3555 case 116076: /*uri*/ return new String[] {"uri"}; 3556 default: return super.getTypesForProperty(hash, name); 3557 } 3558 3559 } 3560 3561 @Override 3562 public Base addChild(String name) throws FHIRException { 3563 if (name.equals("code")) { 3564 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.property.code"); 3565 } 3566 else if (name.equals("uri")) { 3567 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.property.uri"); 3568 } 3569 else 3570 return super.addChild(name); 3571 } 3572 3573 public ValueSetExpansionPropertyComponent copy() { 3574 ValueSetExpansionPropertyComponent dst = new ValueSetExpansionPropertyComponent(); 3575 copyValues(dst); 3576 return dst; 3577 } 3578 3579 public void copyValues(ValueSetExpansionPropertyComponent dst) { 3580 super.copyValues(dst); 3581 dst.code = code == null ? null : code.copy(); 3582 dst.uri = uri == null ? null : uri.copy(); 3583 } 3584 3585 @Override 3586 public boolean equalsDeep(Base other_) { 3587 if (!super.equalsDeep(other_)) 3588 return false; 3589 if (!(other_ instanceof ValueSetExpansionPropertyComponent)) 3590 return false; 3591 ValueSetExpansionPropertyComponent o = (ValueSetExpansionPropertyComponent) other_; 3592 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true); 3593 } 3594 3595 @Override 3596 public boolean equalsShallow(Base other_) { 3597 if (!super.equalsShallow(other_)) 3598 return false; 3599 if (!(other_ instanceof ValueSetExpansionPropertyComponent)) 3600 return false; 3601 ValueSetExpansionPropertyComponent o = (ValueSetExpansionPropertyComponent) other_; 3602 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true); 3603 } 3604 3605 public boolean isEmpty() { 3606 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri); 3607 } 3608 3609 public String fhirType() { 3610 return "ValueSet.expansion.property"; 3611 3612 } 3613 3614 } 3615 3616 @Block() 3617 public static class ValueSetExpansionContainsComponent extends BackboneElement implements IBaseBackboneElement { 3618 /** 3619 * An absolute URI which is the code system in which the code for this item in the expansion is defined. 3620 */ 3621 @Child(name = "system", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3622 @Description(shortDefinition="System value for the code", formalDefinition="An absolute URI which is the code system in which the code for this item in the expansion is defined." ) 3623 protected UriType system; 3624 3625 /** 3626 * If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 3627 */ 3628 @Child(name = "abstract", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3629 @Description(shortDefinition="If user cannot select this entry", formalDefinition="If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value." ) 3630 protected BooleanType abstract_; 3631 3632 /** 3633 * If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use). 3634 */ 3635 @Child(name = "inactive", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3636 @Description(shortDefinition="If concept is inactive in the code system", formalDefinition="If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use)." ) 3637 protected BooleanType inactive; 3638 3639 /** 3640 * The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 3641 */ 3642 @Child(name = "version", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3643 @Description(shortDefinition="Version in which this code/display is defined", formalDefinition="The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged." ) 3644 protected StringType version; 3645 3646 /** 3647 * The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3648 */ 3649 @Child(name = "code", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 3650 @Description(shortDefinition="Code - if blank, this is not a selectable code", formalDefinition="The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set." ) 3651 protected CodeType code; 3652 3653 /** 3654 * The recommended display for this item in the expansion. 3655 */ 3656 @Child(name = "display", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3657 @Description(shortDefinition="User display for the concept", formalDefinition="The recommended display for this item in the expansion." ) 3658 protected StringType display; 3659 3660 /** 3661 * Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation. 3662 */ 3663 @Child(name = "designation", type = {ConceptReferenceDesignationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3664 @Description(shortDefinition="Additional representations for this item", formalDefinition="Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation." ) 3665 protected List<ConceptReferenceDesignationComponent> designation; 3666 3667 /** 3668 * A property value for this concept. 3669 */ 3670 @Child(name = "property", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3671 @Description(shortDefinition="Property value for the concept", formalDefinition="A property value for this concept." ) 3672 protected List<ConceptPropertyComponent> property; 3673 3674 /** 3675 * Other codes and entries contained under this entry in the hierarchy. 3676 */ 3677 @Child(name = "contains", type = {ValueSetExpansionContainsComponent.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3678 @Description(shortDefinition="Codes contained under this entry", formalDefinition="Other codes and entries contained under this entry in the hierarchy." ) 3679 protected List<ValueSetExpansionContainsComponent> contains; 3680 3681 private static final long serialVersionUID = -1370569439L; 3682 3683 /** 3684 * Constructor 3685 */ 3686 public ValueSetExpansionContainsComponent() { 3687 super(); 3688 } 3689 3690 /** 3691 * @return {@link #system} (An absolute URI which is the code system in which the code for this item in the expansion is defined.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3692 */ 3693 public UriType getSystemElement() { 3694 if (this.system == null) 3695 if (Configuration.errorOnAutoCreate()) 3696 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.system"); 3697 else if (Configuration.doAutoCreate()) 3698 this.system = new UriType(); // bb 3699 return this.system; 3700 } 3701 3702 public boolean hasSystemElement() { 3703 return this.system != null && !this.system.isEmpty(); 3704 } 3705 3706 public boolean hasSystem() { 3707 return this.system != null && !this.system.isEmpty(); 3708 } 3709 3710 /** 3711 * @param value {@link #system} (An absolute URI which is the code system in which the code for this item in the expansion is defined.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3712 */ 3713 public ValueSetExpansionContainsComponent setSystemElement(UriType value) { 3714 this.system = value; 3715 return this; 3716 } 3717 3718 /** 3719 * @return An absolute URI which is the code system in which the code for this item in the expansion is defined. 3720 */ 3721 public String getSystem() { 3722 return this.system == null ? null : this.system.getValue(); 3723 } 3724 3725 /** 3726 * @param value An absolute URI which is the code system in which the code for this item in the expansion is defined. 3727 */ 3728 public ValueSetExpansionContainsComponent setSystem(String value) { 3729 if (Utilities.noString(value)) 3730 this.system = null; 3731 else { 3732 if (this.system == null) 3733 this.system = new UriType(); 3734 this.system.setValue(value); 3735 } 3736 return this; 3737 } 3738 3739 /** 3740 * @return {@link #abstract_} (If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 3741 */ 3742 public BooleanType getAbstractElement() { 3743 if (this.abstract_ == null) 3744 if (Configuration.errorOnAutoCreate()) 3745 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.abstract_"); 3746 else if (Configuration.doAutoCreate()) 3747 this.abstract_ = new BooleanType(); // bb 3748 return this.abstract_; 3749 } 3750 3751 public boolean hasAbstractElement() { 3752 return this.abstract_ != null && !this.abstract_.isEmpty(); 3753 } 3754 3755 public boolean hasAbstract() { 3756 return this.abstract_ != null && !this.abstract_.isEmpty(); 3757 } 3758 3759 /** 3760 * @param value {@link #abstract_} (If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 3761 */ 3762 public ValueSetExpansionContainsComponent setAbstractElement(BooleanType value) { 3763 this.abstract_ = value; 3764 return this; 3765 } 3766 3767 /** 3768 * @return If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 3769 */ 3770 public boolean getAbstract() { 3771 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 3772 } 3773 3774 /** 3775 * @param value If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 3776 */ 3777 public ValueSetExpansionContainsComponent setAbstract(boolean value) { 3778 if (this.abstract_ == null) 3779 this.abstract_ = new BooleanType(); 3780 this.abstract_.setValue(value); 3781 return this; 3782 } 3783 3784 /** 3785 * @return {@link #inactive} (If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 3786 */ 3787 public BooleanType getInactiveElement() { 3788 if (this.inactive == null) 3789 if (Configuration.errorOnAutoCreate()) 3790 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.inactive"); 3791 else if (Configuration.doAutoCreate()) 3792 this.inactive = new BooleanType(); // bb 3793 return this.inactive; 3794 } 3795 3796 public boolean hasInactiveElement() { 3797 return this.inactive != null && !this.inactive.isEmpty(); 3798 } 3799 3800 public boolean hasInactive() { 3801 return this.inactive != null && !this.inactive.isEmpty(); 3802 } 3803 3804 /** 3805 * @param value {@link #inactive} (If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 3806 */ 3807 public ValueSetExpansionContainsComponent setInactiveElement(BooleanType value) { 3808 this.inactive = value; 3809 return this; 3810 } 3811 3812 /** 3813 * @return If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use). 3814 */ 3815 public boolean getInactive() { 3816 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 3817 } 3818 3819 /** 3820 * @param value If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use). 3821 */ 3822 public ValueSetExpansionContainsComponent setInactive(boolean value) { 3823 if (this.inactive == null) 3824 this.inactive = new BooleanType(); 3825 this.inactive.setValue(value); 3826 return this; 3827 } 3828 3829 /** 3830 * @return {@link #version} (The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3831 */ 3832 public StringType getVersionElement() { 3833 if (this.version == null) 3834 if (Configuration.errorOnAutoCreate()) 3835 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.version"); 3836 else if (Configuration.doAutoCreate()) 3837 this.version = new StringType(); // bb 3838 return this.version; 3839 } 3840 3841 public boolean hasVersionElement() { 3842 return this.version != null && !this.version.isEmpty(); 3843 } 3844 3845 public boolean hasVersion() { 3846 return this.version != null && !this.version.isEmpty(); 3847 } 3848 3849 /** 3850 * @param value {@link #version} (The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3851 */ 3852 public ValueSetExpansionContainsComponent setVersionElement(StringType value) { 3853 this.version = value; 3854 return this; 3855 } 3856 3857 /** 3858 * @return The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 3859 */ 3860 public String getVersion() { 3861 return this.version == null ? null : this.version.getValue(); 3862 } 3863 3864 /** 3865 * @param value The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 3866 */ 3867 public ValueSetExpansionContainsComponent setVersion(String value) { 3868 if (Utilities.noString(value)) 3869 this.version = null; 3870 else { 3871 if (this.version == null) 3872 this.version = new StringType(); 3873 this.version.setValue(value); 3874 } 3875 return this; 3876 } 3877 3878 /** 3879 * @return {@link #code} (The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3880 */ 3881 public CodeType getCodeElement() { 3882 if (this.code == null) 3883 if (Configuration.errorOnAutoCreate()) 3884 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.code"); 3885 else if (Configuration.doAutoCreate()) 3886 this.code = new CodeType(); // bb 3887 return this.code; 3888 } 3889 3890 public boolean hasCodeElement() { 3891 return this.code != null && !this.code.isEmpty(); 3892 } 3893 3894 public boolean hasCode() { 3895 return this.code != null && !this.code.isEmpty(); 3896 } 3897 3898 /** 3899 * @param value {@link #code} (The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3900 */ 3901 public ValueSetExpansionContainsComponent setCodeElement(CodeType value) { 3902 this.code = value; 3903 return this; 3904 } 3905 3906 /** 3907 * @return The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3908 */ 3909 public String getCode() { 3910 return this.code == null ? null : this.code.getValue(); 3911 } 3912 3913 /** 3914 * @param value The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3915 */ 3916 public ValueSetExpansionContainsComponent setCode(String value) { 3917 if (Utilities.noString(value)) 3918 this.code = null; 3919 else { 3920 if (this.code == null) 3921 this.code = new CodeType(); 3922 this.code.setValue(value); 3923 } 3924 return this; 3925 } 3926 3927 /** 3928 * @return {@link #display} (The recommended display for this item in the expansion.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3929 */ 3930 public StringType getDisplayElement() { 3931 if (this.display == null) 3932 if (Configuration.errorOnAutoCreate()) 3933 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.display"); 3934 else if (Configuration.doAutoCreate()) 3935 this.display = new StringType(); // bb 3936 return this.display; 3937 } 3938 3939 public boolean hasDisplayElement() { 3940 return this.display != null && !this.display.isEmpty(); 3941 } 3942 3943 public boolean hasDisplay() { 3944 return this.display != null && !this.display.isEmpty(); 3945 } 3946 3947 /** 3948 * @param value {@link #display} (The recommended display for this item in the expansion.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3949 */ 3950 public ValueSetExpansionContainsComponent setDisplayElement(StringType value) { 3951 this.display = value; 3952 return this; 3953 } 3954 3955 /** 3956 * @return The recommended display for this item in the expansion. 3957 */ 3958 public String getDisplay() { 3959 return this.display == null ? null : this.display.getValue(); 3960 } 3961 3962 /** 3963 * @param value The recommended display for this item in the expansion. 3964 */ 3965 public ValueSetExpansionContainsComponent setDisplay(String value) { 3966 if (Utilities.noString(value)) 3967 this.display = null; 3968 else { 3969 if (this.display == null) 3970 this.display = new StringType(); 3971 this.display.setValue(value); 3972 } 3973 return this; 3974 } 3975 3976 /** 3977 * @return {@link #designation} (Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.) 3978 */ 3979 public List<ConceptReferenceDesignationComponent> getDesignation() { 3980 if (this.designation == null) 3981 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 3982 return this.designation; 3983 } 3984 3985 /** 3986 * @return Returns a reference to <code>this</code> for easy method chaining 3987 */ 3988 public ValueSetExpansionContainsComponent setDesignation(List<ConceptReferenceDesignationComponent> theDesignation) { 3989 this.designation = theDesignation; 3990 return this; 3991 } 3992 3993 public boolean hasDesignation() { 3994 if (this.designation == null) 3995 return false; 3996 for (ConceptReferenceDesignationComponent item : this.designation) 3997 if (!item.isEmpty()) 3998 return true; 3999 return false; 4000 } 4001 4002 public ConceptReferenceDesignationComponent addDesignation() { //3 4003 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 4004 if (this.designation == null) 4005 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4006 this.designation.add(t); 4007 return t; 4008 } 4009 4010 public ValueSetExpansionContainsComponent addDesignation(ConceptReferenceDesignationComponent t) { //3 4011 if (t == null) 4012 return this; 4013 if (this.designation == null) 4014 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4015 this.designation.add(t); 4016 return this; 4017 } 4018 4019 /** 4020 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist {3} 4021 */ 4022 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 4023 if (getDesignation().isEmpty()) { 4024 addDesignation(); 4025 } 4026 return getDesignation().get(0); 4027 } 4028 4029 /** 4030 * @return {@link #property} (A property value for this concept.) 4031 */ 4032 public List<ConceptPropertyComponent> getProperty() { 4033 if (this.property == null) 4034 this.property = new ArrayList<ConceptPropertyComponent>(); 4035 return this.property; 4036 } 4037 4038 /** 4039 * @return Returns a reference to <code>this</code> for easy method chaining 4040 */ 4041 public ValueSetExpansionContainsComponent setProperty(List<ConceptPropertyComponent> theProperty) { 4042 this.property = theProperty; 4043 return this; 4044 } 4045 4046 public boolean hasProperty() { 4047 if (this.property == null) 4048 return false; 4049 for (ConceptPropertyComponent item : this.property) 4050 if (!item.isEmpty()) 4051 return true; 4052 return false; 4053 } 4054 4055 public ConceptPropertyComponent addProperty() { //3 4056 ConceptPropertyComponent t = new ConceptPropertyComponent(); 4057 if (this.property == null) 4058 this.property = new ArrayList<ConceptPropertyComponent>(); 4059 this.property.add(t); 4060 return t; 4061 } 4062 4063 public ValueSetExpansionContainsComponent addProperty(ConceptPropertyComponent t) { //3 4064 if (t == null) 4065 return this; 4066 if (this.property == null) 4067 this.property = new ArrayList<ConceptPropertyComponent>(); 4068 this.property.add(t); 4069 return this; 4070 } 4071 4072 /** 4073 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 4074 */ 4075 public ConceptPropertyComponent getPropertyFirstRep() { 4076 if (getProperty().isEmpty()) { 4077 addProperty(); 4078 } 4079 return getProperty().get(0); 4080 } 4081 4082 /** 4083 * @return {@link #contains} (Other codes and entries contained under this entry in the hierarchy.) 4084 */ 4085 public List<ValueSetExpansionContainsComponent> getContains() { 4086 if (this.contains == null) 4087 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4088 return this.contains; 4089 } 4090 4091 /** 4092 * @return Returns a reference to <code>this</code> for easy method chaining 4093 */ 4094 public ValueSetExpansionContainsComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 4095 this.contains = theContains; 4096 return this; 4097 } 4098 4099 public boolean hasContains() { 4100 if (this.contains == null) 4101 return false; 4102 for (ValueSetExpansionContainsComponent item : this.contains) 4103 if (!item.isEmpty()) 4104 return true; 4105 return false; 4106 } 4107 4108 public ValueSetExpansionContainsComponent addContains() { //3 4109 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 4110 if (this.contains == null) 4111 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4112 this.contains.add(t); 4113 return t; 4114 } 4115 4116 public ValueSetExpansionContainsComponent addContains(ValueSetExpansionContainsComponent t) { //3 4117 if (t == null) 4118 return this; 4119 if (this.contains == null) 4120 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4121 this.contains.add(t); 4122 return this; 4123 } 4124 4125 /** 4126 * @return The first repetition of repeating field {@link #contains}, creating it if it does not already exist {3} 4127 */ 4128 public ValueSetExpansionContainsComponent getContainsFirstRep() { 4129 if (getContains().isEmpty()) { 4130 addContains(); 4131 } 4132 return getContains().get(0); 4133 } 4134 4135 protected void listChildren(List<Property> children) { 4136 super.listChildren(children); 4137 children.add(new Property("system", "uri", "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 1, system)); 4138 children.add(new Property("abstract", "boolean", "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 0, 1, abstract_)); 4139 children.add(new Property("inactive", "boolean", "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use).", 0, 1, inactive)); 4140 children.add(new Property("version", "string", "The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version)); 4141 children.add(new Property("code", "code", "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 0, 1, code)); 4142 children.add(new Property("display", "string", "The recommended display for this item in the expansion.", 0, 1, display)); 4143 children.add(new Property("designation", "@ValueSet.compose.include.concept.designation", "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 0, java.lang.Integer.MAX_VALUE, designation)); 4144 children.add(new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property)); 4145 children.add(new Property("contains", "@ValueSet.expansion.contains", "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, contains)); 4146 } 4147 4148 @Override 4149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4150 switch (_hash) { 4151 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 1, system); 4152 case 1732898850: /*abstract*/ return new Property("abstract", "boolean", "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 0, 1, abstract_); 4153 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether a concept is inactive (and it may depend on the context of use).", 0, 1, inactive); 4154 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version); 4155 case 3059181: /*code*/ return new Property("code", "code", "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 0, 1, code); 4156 case 1671764162: /*display*/ return new Property("display", "string", "The recommended display for this item in the expansion.", 0, 1, display); 4157 case -900931593: /*designation*/ return new Property("designation", "@ValueSet.compose.include.concept.designation", "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 0, java.lang.Integer.MAX_VALUE, designation); 4158 case -993141291: /*property*/ return new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property); 4159 case -567445985: /*contains*/ return new Property("contains", "@ValueSet.expansion.contains", "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, contains); 4160 default: return super.getNamedProperty(_hash, _name, _checkValid); 4161 } 4162 4163 } 4164 4165 @Override 4166 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4167 switch (hash) { 4168 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 4169 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : new Base[] {this.abstract_}; // BooleanType 4170 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 4171 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4172 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 4173 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 4174 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 4175 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ConceptPropertyComponent 4176 case -567445985: /*contains*/ return this.contains == null ? new Base[0] : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 4177 default: return super.getProperty(hash, name, checkValid); 4178 } 4179 4180 } 4181 4182 @Override 4183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4184 switch (hash) { 4185 case -887328209: // system 4186 this.system = TypeConvertor.castToUri(value); // UriType 4187 return value; 4188 case 1732898850: // abstract 4189 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 4190 return value; 4191 case 24665195: // inactive 4192 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 4193 return value; 4194 case 351608024: // version 4195 this.version = TypeConvertor.castToString(value); // StringType 4196 return value; 4197 case 3059181: // code 4198 this.code = TypeConvertor.castToCode(value); // CodeType 4199 return value; 4200 case 1671764162: // display 4201 this.display = TypeConvertor.castToString(value); // StringType 4202 return value; 4203 case -900931593: // designation 4204 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 4205 return value; 4206 case -993141291: // property 4207 this.getProperty().add((ConceptPropertyComponent) value); // ConceptPropertyComponent 4208 return value; 4209 case -567445985: // contains 4210 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 4211 return value; 4212 default: return super.setProperty(hash, name, value); 4213 } 4214 4215 } 4216 4217 @Override 4218 public Base setProperty(String name, Base value) throws FHIRException { 4219 if (name.equals("system")) { 4220 this.system = TypeConvertor.castToUri(value); // UriType 4221 } else if (name.equals("abstract")) { 4222 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 4223 } else if (name.equals("inactive")) { 4224 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 4225 } else if (name.equals("version")) { 4226 this.version = TypeConvertor.castToString(value); // StringType 4227 } else if (name.equals("code")) { 4228 this.code = TypeConvertor.castToCode(value); // CodeType 4229 } else if (name.equals("display")) { 4230 this.display = TypeConvertor.castToString(value); // StringType 4231 } else if (name.equals("designation")) { 4232 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 4233 } else if (name.equals("property")) { 4234 this.getProperty().add((ConceptPropertyComponent) value); 4235 } else if (name.equals("contains")) { 4236 this.getContains().add((ValueSetExpansionContainsComponent) value); 4237 } else 4238 return super.setProperty(name, value); 4239 return value; 4240 } 4241 4242 @Override 4243 public void removeChild(String name, Base value) throws FHIRException { 4244 if (name.equals("system")) { 4245 this.system = null; 4246 } else if (name.equals("abstract")) { 4247 this.abstract_ = null; 4248 } else if (name.equals("inactive")) { 4249 this.inactive = null; 4250 } else if (name.equals("version")) { 4251 this.version = null; 4252 } else if (name.equals("code")) { 4253 this.code = null; 4254 } else if (name.equals("display")) { 4255 this.display = null; 4256 } else if (name.equals("designation")) { 4257 this.getDesignation().remove((ConceptReferenceDesignationComponent) value); 4258 } else if (name.equals("property")) { 4259 this.getProperty().remove((ConceptPropertyComponent) value); 4260 } else if (name.equals("contains")) { 4261 this.getContains().remove((ValueSetExpansionContainsComponent) value); 4262 } else 4263 super.removeChild(name, value); 4264 4265 } 4266 4267 @Override 4268 public Base makeProperty(int hash, String name) throws FHIRException { 4269 switch (hash) { 4270 case -887328209: return getSystemElement(); 4271 case 1732898850: return getAbstractElement(); 4272 case 24665195: return getInactiveElement(); 4273 case 351608024: return getVersionElement(); 4274 case 3059181: return getCodeElement(); 4275 case 1671764162: return getDisplayElement(); 4276 case -900931593: return addDesignation(); 4277 case -993141291: return addProperty(); 4278 case -567445985: return addContains(); 4279 default: return super.makeProperty(hash, name); 4280 } 4281 4282 } 4283 4284 @Override 4285 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4286 switch (hash) { 4287 case -887328209: /*system*/ return new String[] {"uri"}; 4288 case 1732898850: /*abstract*/ return new String[] {"boolean"}; 4289 case 24665195: /*inactive*/ return new String[] {"boolean"}; 4290 case 351608024: /*version*/ return new String[] {"string"}; 4291 case 3059181: /*code*/ return new String[] {"code"}; 4292 case 1671764162: /*display*/ return new String[] {"string"}; 4293 case -900931593: /*designation*/ return new String[] {"@ValueSet.compose.include.concept.designation"}; 4294 case -993141291: /*property*/ return new String[] {}; 4295 case -567445985: /*contains*/ return new String[] {"@ValueSet.expansion.contains"}; 4296 default: return super.getTypesForProperty(hash, name); 4297 } 4298 4299 } 4300 4301 @Override 4302 public Base addChild(String name) throws FHIRException { 4303 if (name.equals("system")) { 4304 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.system"); 4305 } 4306 else if (name.equals("abstract")) { 4307 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.abstract"); 4308 } 4309 else if (name.equals("inactive")) { 4310 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.inactive"); 4311 } 4312 else if (name.equals("version")) { 4313 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.version"); 4314 } 4315 else if (name.equals("code")) { 4316 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.code"); 4317 } 4318 else if (name.equals("display")) { 4319 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.display"); 4320 } 4321 else if (name.equals("designation")) { 4322 return addDesignation(); 4323 } 4324 else if (name.equals("property")) { 4325 return addProperty(); 4326 } 4327 else if (name.equals("contains")) { 4328 return addContains(); 4329 } 4330 else 4331 return super.addChild(name); 4332 } 4333 4334 public ValueSetExpansionContainsComponent copy() { 4335 ValueSetExpansionContainsComponent dst = new ValueSetExpansionContainsComponent(); 4336 copyValues(dst); 4337 return dst; 4338 } 4339 4340 public void copyValues(ValueSetExpansionContainsComponent dst) { 4341 super.copyValues(dst); 4342 dst.system = system == null ? null : system.copy(); 4343 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 4344 dst.inactive = inactive == null ? null : inactive.copy(); 4345 dst.version = version == null ? null : version.copy(); 4346 dst.code = code == null ? null : code.copy(); 4347 dst.display = display == null ? null : display.copy(); 4348 if (designation != null) { 4349 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4350 for (ConceptReferenceDesignationComponent i : designation) 4351 dst.designation.add(i.copy()); 4352 }; 4353 if (property != null) { 4354 dst.property = new ArrayList<ConceptPropertyComponent>(); 4355 for (ConceptPropertyComponent i : property) 4356 dst.property.add(i.copy()); 4357 }; 4358 if (contains != null) { 4359 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4360 for (ValueSetExpansionContainsComponent i : contains) 4361 dst.contains.add(i.copy()); 4362 }; 4363 } 4364 4365 @Override 4366 public boolean equalsDeep(Base other_) { 4367 if (!super.equalsDeep(other_)) 4368 return false; 4369 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 4370 return false; 4371 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 4372 return compareDeep(system, o.system, true) && compareDeep(abstract_, o.abstract_, true) && compareDeep(inactive, o.inactive, true) 4373 && compareDeep(version, o.version, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 4374 && compareDeep(designation, o.designation, true) && compareDeep(property, o.property, true) && compareDeep(contains, o.contains, true) 4375 ; 4376 } 4377 4378 @Override 4379 public boolean equalsShallow(Base other_) { 4380 if (!super.equalsShallow(other_)) 4381 return false; 4382 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 4383 return false; 4384 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 4385 return compareValues(system, o.system, true) && compareValues(abstract_, o.abstract_, true) && compareValues(inactive, o.inactive, true) 4386 && compareValues(version, o.version, true) && compareValues(code, o.code, true) && compareValues(display, o.display, true) 4387 ; 4388 } 4389 4390 public boolean isEmpty() { 4391 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, abstract_, inactive 4392 , version, code, display, designation, property, contains); 4393 } 4394 4395 public String fhirType() { 4396 return "ValueSet.expansion.contains"; 4397 4398 } 4399 4400 } 4401 4402 @Block() 4403 public static class ConceptPropertyComponent extends BackboneElement implements IBaseBackboneElement { 4404 /** 4405 * A code that is a reference to ValueSet.expansion.property.code. 4406 */ 4407 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4408 @Description(shortDefinition="Reference to ValueSet.expansion.property.code", formalDefinition="A code that is a reference to ValueSet.expansion.property.code." ) 4409 protected CodeType code; 4410 4411 /** 4412 * The value of this property. 4413 */ 4414 @Child(name = "value", type = {CodeType.class, Coding.class, StringType.class, IntegerType.class, BooleanType.class, DateTimeType.class, DecimalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4415 @Description(shortDefinition="Value of the property for this concept", formalDefinition="The value of this property." ) 4416 protected DataType value; 4417 4418 /** 4419 * A subproperty value for this concept. 4420 */ 4421 @Child(name = "subProperty", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4422 @Description(shortDefinition="SubProperty value for the concept", formalDefinition="A subproperty value for this concept." ) 4423 protected List<ConceptSubPropertyComponent> subProperty; 4424 4425 private static final long serialVersionUID = -948620650L; 4426 4427 /** 4428 * Constructor 4429 */ 4430 public ConceptPropertyComponent() { 4431 super(); 4432 } 4433 4434 /** 4435 * Constructor 4436 */ 4437 public ConceptPropertyComponent(String code, DataType value) { 4438 super(); 4439 this.setCode(code); 4440 this.setValue(value); 4441 } 4442 4443 /** 4444 * @return {@link #code} (A code that is a reference to ValueSet.expansion.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4445 */ 4446 public CodeType getCodeElement() { 4447 if (this.code == null) 4448 if (Configuration.errorOnAutoCreate()) 4449 throw new Error("Attempt to auto-create ConceptPropertyComponent.code"); 4450 else if (Configuration.doAutoCreate()) 4451 this.code = new CodeType(); // bb 4452 return this.code; 4453 } 4454 4455 public boolean hasCodeElement() { 4456 return this.code != null && !this.code.isEmpty(); 4457 } 4458 4459 public boolean hasCode() { 4460 return this.code != null && !this.code.isEmpty(); 4461 } 4462 4463 /** 4464 * @param value {@link #code} (A code that is a reference to ValueSet.expansion.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4465 */ 4466 public ConceptPropertyComponent setCodeElement(CodeType value) { 4467 this.code = value; 4468 return this; 4469 } 4470 4471 /** 4472 * @return A code that is a reference to ValueSet.expansion.property.code. 4473 */ 4474 public String getCode() { 4475 return this.code == null ? null : this.code.getValue(); 4476 } 4477 4478 /** 4479 * @param value A code that is a reference to ValueSet.expansion.property.code. 4480 */ 4481 public ConceptPropertyComponent setCode(String value) { 4482 if (this.code == null) 4483 this.code = new CodeType(); 4484 this.code.setValue(value); 4485 return this; 4486 } 4487 4488 /** 4489 * @return {@link #value} (The value of this property.) 4490 */ 4491 public DataType getValue() { 4492 return this.value; 4493 } 4494 4495 /** 4496 * @return {@link #value} (The value of this property.) 4497 */ 4498 public CodeType getValueCodeType() throws FHIRException { 4499 if (this.value == null) 4500 this.value = new CodeType(); 4501 if (!(this.value instanceof CodeType)) 4502 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4503 return (CodeType) this.value; 4504 } 4505 4506 public boolean hasValueCodeType() { 4507 return this != null && this.value instanceof CodeType; 4508 } 4509 4510 /** 4511 * @return {@link #value} (The value of this property.) 4512 */ 4513 public Coding getValueCoding() throws FHIRException { 4514 if (this.value == null) 4515 this.value = new Coding(); 4516 if (!(this.value instanceof Coding)) 4517 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 4518 return (Coding) this.value; 4519 } 4520 4521 public boolean hasValueCoding() { 4522 return this != null && this.value instanceof Coding; 4523 } 4524 4525 /** 4526 * @return {@link #value} (The value of this property.) 4527 */ 4528 public StringType getValueStringType() throws FHIRException { 4529 if (this.value == null) 4530 this.value = new StringType(); 4531 if (!(this.value instanceof StringType)) 4532 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 4533 return (StringType) this.value; 4534 } 4535 4536 public boolean hasValueStringType() { 4537 return this != null && this.value instanceof StringType; 4538 } 4539 4540 /** 4541 * @return {@link #value} (The value of this property.) 4542 */ 4543 public IntegerType getValueIntegerType() throws FHIRException { 4544 if (this.value == null) 4545 this.value = new IntegerType(); 4546 if (!(this.value instanceof IntegerType)) 4547 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 4548 return (IntegerType) this.value; 4549 } 4550 4551 public boolean hasValueIntegerType() { 4552 return this != null && this.value instanceof IntegerType; 4553 } 4554 4555 /** 4556 * @return {@link #value} (The value of this property.) 4557 */ 4558 public BooleanType getValueBooleanType() throws FHIRException { 4559 if (this.value == null) 4560 this.value = new BooleanType(); 4561 if (!(this.value instanceof BooleanType)) 4562 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 4563 return (BooleanType) this.value; 4564 } 4565 4566 public boolean hasValueBooleanType() { 4567 return this != null && this.value instanceof BooleanType; 4568 } 4569 4570 /** 4571 * @return {@link #value} (The value of this property.) 4572 */ 4573 public DateTimeType getValueDateTimeType() throws FHIRException { 4574 if (this.value == null) 4575 this.value = new DateTimeType(); 4576 if (!(this.value instanceof DateTimeType)) 4577 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4578 return (DateTimeType) this.value; 4579 } 4580 4581 public boolean hasValueDateTimeType() { 4582 return this != null && this.value instanceof DateTimeType; 4583 } 4584 4585 /** 4586 * @return {@link #value} (The value of this property.) 4587 */ 4588 public DecimalType getValueDecimalType() throws FHIRException { 4589 if (this.value == null) 4590 this.value = new DecimalType(); 4591 if (!(this.value instanceof DecimalType)) 4592 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 4593 return (DecimalType) this.value; 4594 } 4595 4596 public boolean hasValueDecimalType() { 4597 return this != null && this.value instanceof DecimalType; 4598 } 4599 4600 public boolean hasValue() { 4601 return this.value != null && !this.value.isEmpty(); 4602 } 4603 4604 /** 4605 * @param value {@link #value} (The value of this property.) 4606 */ 4607 public ConceptPropertyComponent setValue(DataType value) { 4608 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType || value instanceof DecimalType)) 4609 throw new FHIRException("Not the right type for ValueSet.expansion.contains.property.value[x]: "+value.fhirType()); 4610 this.value = value; 4611 return this; 4612 } 4613 4614 /** 4615 * @return {@link #subProperty} (A subproperty value for this concept.) 4616 */ 4617 public List<ConceptSubPropertyComponent> getSubProperty() { 4618 if (this.subProperty == null) 4619 this.subProperty = new ArrayList<ConceptSubPropertyComponent>(); 4620 return this.subProperty; 4621 } 4622 4623 /** 4624 * @return Returns a reference to <code>this</code> for easy method chaining 4625 */ 4626 public ConceptPropertyComponent setSubProperty(List<ConceptSubPropertyComponent> theSubProperty) { 4627 this.subProperty = theSubProperty; 4628 return this; 4629 } 4630 4631 public boolean hasSubProperty() { 4632 if (this.subProperty == null) 4633 return false; 4634 for (ConceptSubPropertyComponent item : this.subProperty) 4635 if (!item.isEmpty()) 4636 return true; 4637 return false; 4638 } 4639 4640 public ConceptSubPropertyComponent addSubProperty() { //3 4641 ConceptSubPropertyComponent t = new ConceptSubPropertyComponent(); 4642 if (this.subProperty == null) 4643 this.subProperty = new ArrayList<ConceptSubPropertyComponent>(); 4644 this.subProperty.add(t); 4645 return t; 4646 } 4647 4648 public ConceptPropertyComponent addSubProperty(ConceptSubPropertyComponent t) { //3 4649 if (t == null) 4650 return this; 4651 if (this.subProperty == null) 4652 this.subProperty = new ArrayList<ConceptSubPropertyComponent>(); 4653 this.subProperty.add(t); 4654 return this; 4655 } 4656 4657 /** 4658 * @return The first repetition of repeating field {@link #subProperty}, creating it if it does not already exist {3} 4659 */ 4660 public ConceptSubPropertyComponent getSubPropertyFirstRep() { 4661 if (getSubProperty().isEmpty()) { 4662 addSubProperty(); 4663 } 4664 return getSubProperty().get(0); 4665 } 4666 4667 protected void listChildren(List<Property> children) { 4668 super.listChildren(children); 4669 children.add(new Property("code", "code", "A code that is a reference to ValueSet.expansion.property.code.", 0, 1, code)); 4670 children.add(new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value)); 4671 children.add(new Property("subProperty", "", "A subproperty value for this concept.", 0, java.lang.Integer.MAX_VALUE, subProperty)); 4672 } 4673 4674 @Override 4675 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4676 switch (_hash) { 4677 case 3059181: /*code*/ return new Property("code", "code", "A code that is a reference to ValueSet.expansion.property.code.", 0, 1, code); 4678 case -1410166417: /*value[x]*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value); 4679 case 111972721: /*value*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value); 4680 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of this property.", 0, 1, value); 4681 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of this property.", 0, 1, value); 4682 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of this property.", 0, 1, value); 4683 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of this property.", 0, 1, value); 4684 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of this property.", 0, 1, value); 4685 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of this property.", 0, 1, value); 4686 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of this property.", 0, 1, value); 4687 case 321372213: /*subProperty*/ return new Property("subProperty", "", "A subproperty value for this concept.", 0, java.lang.Integer.MAX_VALUE, subProperty); 4688 default: return super.getNamedProperty(_hash, _name, _checkValid); 4689 } 4690 4691 } 4692 4693 @Override 4694 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4695 switch (hash) { 4696 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 4697 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 4698 case 321372213: /*subProperty*/ return this.subProperty == null ? new Base[0] : this.subProperty.toArray(new Base[this.subProperty.size()]); // ConceptSubPropertyComponent 4699 default: return super.getProperty(hash, name, checkValid); 4700 } 4701 4702 } 4703 4704 @Override 4705 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4706 switch (hash) { 4707 case 3059181: // code 4708 this.code = TypeConvertor.castToCode(value); // CodeType 4709 return value; 4710 case 111972721: // value 4711 this.value = TypeConvertor.castToType(value); // DataType 4712 return value; 4713 case 321372213: // subProperty 4714 this.getSubProperty().add((ConceptSubPropertyComponent) value); // ConceptSubPropertyComponent 4715 return value; 4716 default: return super.setProperty(hash, name, value); 4717 } 4718 4719 } 4720 4721 @Override 4722 public Base setProperty(String name, Base value) throws FHIRException { 4723 if (name.equals("code")) { 4724 this.code = TypeConvertor.castToCode(value); // CodeType 4725 } else if (name.equals("value[x]")) { 4726 this.value = TypeConvertor.castToType(value); // DataType 4727 } else if (name.equals("subProperty")) { 4728 this.getSubProperty().add((ConceptSubPropertyComponent) value); 4729 } else 4730 return super.setProperty(name, value); 4731 return value; 4732 } 4733 4734 @Override 4735 public void removeChild(String name, Base value) throws FHIRException { 4736 if (name.equals("code")) { 4737 this.code = null; 4738 } else if (name.equals("value[x]")) { 4739 this.value = null; 4740 } else if (name.equals("subProperty")) { 4741 this.getSubProperty().remove((ConceptSubPropertyComponent) value); 4742 } else 4743 super.removeChild(name, value); 4744 4745 } 4746 4747 @Override 4748 public Base makeProperty(int hash, String name) throws FHIRException { 4749 switch (hash) { 4750 case 3059181: return getCodeElement(); 4751 case -1410166417: return getValue(); 4752 case 111972721: return getValue(); 4753 case 321372213: return addSubProperty(); 4754 default: return super.makeProperty(hash, name); 4755 } 4756 4757 } 4758 4759 @Override 4760 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4761 switch (hash) { 4762 case 3059181: /*code*/ return new String[] {"code"}; 4763 case 111972721: /*value*/ return new String[] {"code", "Coding", "string", "integer", "boolean", "dateTime", "decimal"}; 4764 case 321372213: /*subProperty*/ return new String[] {}; 4765 default: return super.getTypesForProperty(hash, name); 4766 } 4767 4768 } 4769 4770 @Override 4771 public Base addChild(String name) throws FHIRException { 4772 if (name.equals("code")) { 4773 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.property.code"); 4774 } 4775 else if (name.equals("valueCode")) { 4776 this.value = new CodeType(); 4777 return this.value; 4778 } 4779 else if (name.equals("valueCoding")) { 4780 this.value = new Coding(); 4781 return this.value; 4782 } 4783 else if (name.equals("valueString")) { 4784 this.value = new StringType(); 4785 return this.value; 4786 } 4787 else if (name.equals("valueInteger")) { 4788 this.value = new IntegerType(); 4789 return this.value; 4790 } 4791 else if (name.equals("valueBoolean")) { 4792 this.value = new BooleanType(); 4793 return this.value; 4794 } 4795 else if (name.equals("valueDateTime")) { 4796 this.value = new DateTimeType(); 4797 return this.value; 4798 } 4799 else if (name.equals("valueDecimal")) { 4800 this.value = new DecimalType(); 4801 return this.value; 4802 } 4803 else if (name.equals("subProperty")) { 4804 return addSubProperty(); 4805 } 4806 else 4807 return super.addChild(name); 4808 } 4809 4810 public ConceptPropertyComponent copy() { 4811 ConceptPropertyComponent dst = new ConceptPropertyComponent(); 4812 copyValues(dst); 4813 return dst; 4814 } 4815 4816 public void copyValues(ConceptPropertyComponent dst) { 4817 super.copyValues(dst); 4818 dst.code = code == null ? null : code.copy(); 4819 dst.value = value == null ? null : value.copy(); 4820 if (subProperty != null) { 4821 dst.subProperty = new ArrayList<ConceptSubPropertyComponent>(); 4822 for (ConceptSubPropertyComponent i : subProperty) 4823 dst.subProperty.add(i.copy()); 4824 }; 4825 } 4826 4827 @Override 4828 public boolean equalsDeep(Base other_) { 4829 if (!super.equalsDeep(other_)) 4830 return false; 4831 if (!(other_ instanceof ConceptPropertyComponent)) 4832 return false; 4833 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 4834 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(subProperty, o.subProperty, true) 4835 ; 4836 } 4837 4838 @Override 4839 public boolean equalsShallow(Base other_) { 4840 if (!super.equalsShallow(other_)) 4841 return false; 4842 if (!(other_ instanceof ConceptPropertyComponent)) 4843 return false; 4844 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 4845 return compareValues(code, o.code, true); 4846 } 4847 4848 public boolean isEmpty() { 4849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, subProperty 4850 ); 4851 } 4852 4853 public String fhirType() { 4854 return "ValueSet.expansion.contains.property"; 4855 4856 } 4857 4858 } 4859 4860 @Block() 4861 public static class ConceptSubPropertyComponent extends BackboneElement implements IBaseBackboneElement { 4862 /** 4863 * A code that is a reference to ValueSet.expansion.property.code. 4864 */ 4865 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4866 @Description(shortDefinition="Reference to ValueSet.expansion.property.code", formalDefinition="A code that is a reference to ValueSet.expansion.property.code." ) 4867 protected CodeType code; 4868 4869 /** 4870 * The value of this subproperty. 4871 */ 4872 @Child(name = "value", type = {CodeType.class, Coding.class, StringType.class, IntegerType.class, BooleanType.class, DateTimeType.class, DecimalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4873 @Description(shortDefinition="Value of the subproperty for this concept", formalDefinition="The value of this subproperty." ) 4874 protected DataType value; 4875 4876 private static final long serialVersionUID = -422546419L; 4877 4878 /** 4879 * Constructor 4880 */ 4881 public ConceptSubPropertyComponent() { 4882 super(); 4883 } 4884 4885 /** 4886 * Constructor 4887 */ 4888 public ConceptSubPropertyComponent(String code, DataType value) { 4889 super(); 4890 this.setCode(code); 4891 this.setValue(value); 4892 } 4893 4894 /** 4895 * @return {@link #code} (A code that is a reference to ValueSet.expansion.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4896 */ 4897 public CodeType getCodeElement() { 4898 if (this.code == null) 4899 if (Configuration.errorOnAutoCreate()) 4900 throw new Error("Attempt to auto-create ConceptSubPropertyComponent.code"); 4901 else if (Configuration.doAutoCreate()) 4902 this.code = new CodeType(); // bb 4903 return this.code; 4904 } 4905 4906 public boolean hasCodeElement() { 4907 return this.code != null && !this.code.isEmpty(); 4908 } 4909 4910 public boolean hasCode() { 4911 return this.code != null && !this.code.isEmpty(); 4912 } 4913 4914 /** 4915 * @param value {@link #code} (A code that is a reference to ValueSet.expansion.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4916 */ 4917 public ConceptSubPropertyComponent setCodeElement(CodeType value) { 4918 this.code = value; 4919 return this; 4920 } 4921 4922 /** 4923 * @return A code that is a reference to ValueSet.expansion.property.code. 4924 */ 4925 public String getCode() { 4926 return this.code == null ? null : this.code.getValue(); 4927 } 4928 4929 /** 4930 * @param value A code that is a reference to ValueSet.expansion.property.code. 4931 */ 4932 public ConceptSubPropertyComponent setCode(String value) { 4933 if (this.code == null) 4934 this.code = new CodeType(); 4935 this.code.setValue(value); 4936 return this; 4937 } 4938 4939 /** 4940 * @return {@link #value} (The value of this subproperty.) 4941 */ 4942 public DataType getValue() { 4943 return this.value; 4944 } 4945 4946 /** 4947 * @return {@link #value} (The value of this subproperty.) 4948 */ 4949 public CodeType getValueCodeType() throws FHIRException { 4950 if (this.value == null) 4951 this.value = new CodeType(); 4952 if (!(this.value instanceof CodeType)) 4953 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4954 return (CodeType) this.value; 4955 } 4956 4957 public boolean hasValueCodeType() { 4958 return this != null && this.value instanceof CodeType; 4959 } 4960 4961 /** 4962 * @return {@link #value} (The value of this subproperty.) 4963 */ 4964 public Coding getValueCoding() throws FHIRException { 4965 if (this.value == null) 4966 this.value = new Coding(); 4967 if (!(this.value instanceof Coding)) 4968 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 4969 return (Coding) this.value; 4970 } 4971 4972 public boolean hasValueCoding() { 4973 return this != null && this.value instanceof Coding; 4974 } 4975 4976 /** 4977 * @return {@link #value} (The value of this subproperty.) 4978 */ 4979 public StringType getValueStringType() throws FHIRException { 4980 if (this.value == null) 4981 this.value = new StringType(); 4982 if (!(this.value instanceof StringType)) 4983 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 4984 return (StringType) this.value; 4985 } 4986 4987 public boolean hasValueStringType() { 4988 return this != null && this.value instanceof StringType; 4989 } 4990 4991 /** 4992 * @return {@link #value} (The value of this subproperty.) 4993 */ 4994 public IntegerType getValueIntegerType() throws FHIRException { 4995 if (this.value == null) 4996 this.value = new IntegerType(); 4997 if (!(this.value instanceof IntegerType)) 4998 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 4999 return (IntegerType) this.value; 5000 } 5001 5002 public boolean hasValueIntegerType() { 5003 return this != null && this.value instanceof IntegerType; 5004 } 5005 5006 /** 5007 * @return {@link #value} (The value of this subproperty.) 5008 */ 5009 public BooleanType getValueBooleanType() throws FHIRException { 5010 if (this.value == null) 5011 this.value = new BooleanType(); 5012 if (!(this.value instanceof BooleanType)) 5013 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 5014 return (BooleanType) this.value; 5015 } 5016 5017 public boolean hasValueBooleanType() { 5018 return this != null && this.value instanceof BooleanType; 5019 } 5020 5021 /** 5022 * @return {@link #value} (The value of this subproperty.) 5023 */ 5024 public DateTimeType getValueDateTimeType() throws FHIRException { 5025 if (this.value == null) 5026 this.value = new DateTimeType(); 5027 if (!(this.value instanceof DateTimeType)) 5028 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 5029 return (DateTimeType) this.value; 5030 } 5031 5032 public boolean hasValueDateTimeType() { 5033 return this != null && this.value instanceof DateTimeType; 5034 } 5035 5036 /** 5037 * @return {@link #value} (The value of this subproperty.) 5038 */ 5039 public DecimalType getValueDecimalType() throws FHIRException { 5040 if (this.value == null) 5041 this.value = new DecimalType(); 5042 if (!(this.value instanceof DecimalType)) 5043 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 5044 return (DecimalType) this.value; 5045 } 5046 5047 public boolean hasValueDecimalType() { 5048 return this != null && this.value instanceof DecimalType; 5049 } 5050 5051 public boolean hasValue() { 5052 return this.value != null && !this.value.isEmpty(); 5053 } 5054 5055 /** 5056 * @param value {@link #value} (The value of this subproperty.) 5057 */ 5058 public ConceptSubPropertyComponent setValue(DataType value) { 5059 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType || value instanceof DecimalType)) 5060 throw new FHIRException("Not the right type for ValueSet.expansion.contains.property.subProperty.value[x]: "+value.fhirType()); 5061 this.value = value; 5062 return this; 5063 } 5064 5065 protected void listChildren(List<Property> children) { 5066 super.listChildren(children); 5067 children.add(new Property("code", "code", "A code that is a reference to ValueSet.expansion.property.code.", 0, 1, code)); 5068 children.add(new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this subproperty.", 0, 1, value)); 5069 } 5070 5071 @Override 5072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5073 switch (_hash) { 5074 case 3059181: /*code*/ return new Property("code", "code", "A code that is a reference to ValueSet.expansion.property.code.", 0, 1, code); 5075 case -1410166417: /*value[x]*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this subproperty.", 0, 1, value); 5076 case 111972721: /*value*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this subproperty.", 0, 1, value); 5077 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of this subproperty.", 0, 1, value); 5078 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of this subproperty.", 0, 1, value); 5079 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of this subproperty.", 0, 1, value); 5080 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of this subproperty.", 0, 1, value); 5081 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of this subproperty.", 0, 1, value); 5082 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of this subproperty.", 0, 1, value); 5083 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of this subproperty.", 0, 1, value); 5084 default: return super.getNamedProperty(_hash, _name, _checkValid); 5085 } 5086 5087 } 5088 5089 @Override 5090 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5091 switch (hash) { 5092 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 5093 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 5094 default: return super.getProperty(hash, name, checkValid); 5095 } 5096 5097 } 5098 5099 @Override 5100 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5101 switch (hash) { 5102 case 3059181: // code 5103 this.code = TypeConvertor.castToCode(value); // CodeType 5104 return value; 5105 case 111972721: // value 5106 this.value = TypeConvertor.castToType(value); // DataType 5107 return value; 5108 default: return super.setProperty(hash, name, value); 5109 } 5110 5111 } 5112 5113 @Override 5114 public Base setProperty(String name, Base value) throws FHIRException { 5115 if (name.equals("code")) { 5116 this.code = TypeConvertor.castToCode(value); // CodeType 5117 } else if (name.equals("value[x]")) { 5118 this.value = TypeConvertor.castToType(value); // DataType 5119 } else 5120 return super.setProperty(name, value); 5121 return value; 5122 } 5123 5124 @Override 5125 public void removeChild(String name, Base value) throws FHIRException { 5126 if (name.equals("code")) { 5127 this.code = null; 5128 } else if (name.equals("value[x]")) { 5129 this.value = null; 5130 } else 5131 super.removeChild(name, value); 5132 5133 } 5134 5135 @Override 5136 public Base makeProperty(int hash, String name) throws FHIRException { 5137 switch (hash) { 5138 case 3059181: return getCodeElement(); 5139 case -1410166417: return getValue(); 5140 case 111972721: return getValue(); 5141 default: return super.makeProperty(hash, name); 5142 } 5143 5144 } 5145 5146 @Override 5147 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5148 switch (hash) { 5149 case 3059181: /*code*/ return new String[] {"code"}; 5150 case 111972721: /*value*/ return new String[] {"code", "Coding", "string", "integer", "boolean", "dateTime", "decimal"}; 5151 default: return super.getTypesForProperty(hash, name); 5152 } 5153 5154 } 5155 5156 @Override 5157 public Base addChild(String name) throws FHIRException { 5158 if (name.equals("code")) { 5159 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.expansion.contains.property.subProperty.code"); 5160 } 5161 else if (name.equals("valueCode")) { 5162 this.value = new CodeType(); 5163 return this.value; 5164 } 5165 else if (name.equals("valueCoding")) { 5166 this.value = new Coding(); 5167 return this.value; 5168 } 5169 else if (name.equals("valueString")) { 5170 this.value = new StringType(); 5171 return this.value; 5172 } 5173 else if (name.equals("valueInteger")) { 5174 this.value = new IntegerType(); 5175 return this.value; 5176 } 5177 else if (name.equals("valueBoolean")) { 5178 this.value = new BooleanType(); 5179 return this.value; 5180 } 5181 else if (name.equals("valueDateTime")) { 5182 this.value = new DateTimeType(); 5183 return this.value; 5184 } 5185 else if (name.equals("valueDecimal")) { 5186 this.value = new DecimalType(); 5187 return this.value; 5188 } 5189 else 5190 return super.addChild(name); 5191 } 5192 5193 public ConceptSubPropertyComponent copy() { 5194 ConceptSubPropertyComponent dst = new ConceptSubPropertyComponent(); 5195 copyValues(dst); 5196 return dst; 5197 } 5198 5199 public void copyValues(ConceptSubPropertyComponent dst) { 5200 super.copyValues(dst); 5201 dst.code = code == null ? null : code.copy(); 5202 dst.value = value == null ? null : value.copy(); 5203 } 5204 5205 @Override 5206 public boolean equalsDeep(Base other_) { 5207 if (!super.equalsDeep(other_)) 5208 return false; 5209 if (!(other_ instanceof ConceptSubPropertyComponent)) 5210 return false; 5211 ConceptSubPropertyComponent o = (ConceptSubPropertyComponent) other_; 5212 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 5213 } 5214 5215 @Override 5216 public boolean equalsShallow(Base other_) { 5217 if (!super.equalsShallow(other_)) 5218 return false; 5219 if (!(other_ instanceof ConceptSubPropertyComponent)) 5220 return false; 5221 ConceptSubPropertyComponent o = (ConceptSubPropertyComponent) other_; 5222 return compareValues(code, o.code, true); 5223 } 5224 5225 public boolean isEmpty() { 5226 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 5227 } 5228 5229 public String fhirType() { 5230 return "ValueSet.expansion.contains.property.subProperty"; 5231 5232 } 5233 5234 } 5235 5236 @Block() 5237 public static class ValueSetScopeComponent extends BackboneElement implements IBaseBackboneElement { 5238 /** 5239 * Criteria describing which concepts or codes should be included and why. 5240 */ 5241 @Child(name = "inclusionCriteria", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 5242 @Description(shortDefinition="Criteria describing which concepts or codes should be included and why", formalDefinition="Criteria describing which concepts or codes should be included and why." ) 5243 protected StringType inclusionCriteria; 5244 5245 /** 5246 * Criteria describing which concepts or codes should be excluded and why. 5247 */ 5248 @Child(name = "exclusionCriteria", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5249 @Description(shortDefinition="Criteria describing which concepts or codes should be excluded and why", formalDefinition="Criteria describing which concepts or codes should be excluded and why." ) 5250 protected StringType exclusionCriteria; 5251 5252 private static final long serialVersionUID = -641434610L; 5253 5254 /** 5255 * Constructor 5256 */ 5257 public ValueSetScopeComponent() { 5258 super(); 5259 } 5260 5261 /** 5262 * @return {@link #inclusionCriteria} (Criteria describing which concepts or codes should be included and why.). This is the underlying object with id, value and extensions. The accessor "getInclusionCriteria" gives direct access to the value 5263 */ 5264 public StringType getInclusionCriteriaElement() { 5265 if (this.inclusionCriteria == null) 5266 if (Configuration.errorOnAutoCreate()) 5267 throw new Error("Attempt to auto-create ValueSetScopeComponent.inclusionCriteria"); 5268 else if (Configuration.doAutoCreate()) 5269 this.inclusionCriteria = new StringType(); // bb 5270 return this.inclusionCriteria; 5271 } 5272 5273 public boolean hasInclusionCriteriaElement() { 5274 return this.inclusionCriteria != null && !this.inclusionCriteria.isEmpty(); 5275 } 5276 5277 public boolean hasInclusionCriteria() { 5278 return this.inclusionCriteria != null && !this.inclusionCriteria.isEmpty(); 5279 } 5280 5281 /** 5282 * @param value {@link #inclusionCriteria} (Criteria describing which concepts or codes should be included and why.). This is the underlying object with id, value and extensions. The accessor "getInclusionCriteria" gives direct access to the value 5283 */ 5284 public ValueSetScopeComponent setInclusionCriteriaElement(StringType value) { 5285 this.inclusionCriteria = value; 5286 return this; 5287 } 5288 5289 /** 5290 * @return Criteria describing which concepts or codes should be included and why. 5291 */ 5292 public String getInclusionCriteria() { 5293 return this.inclusionCriteria == null ? null : this.inclusionCriteria.getValue(); 5294 } 5295 5296 /** 5297 * @param value Criteria describing which concepts or codes should be included and why. 5298 */ 5299 public ValueSetScopeComponent setInclusionCriteria(String value) { 5300 if (Utilities.noString(value)) 5301 this.inclusionCriteria = null; 5302 else { 5303 if (this.inclusionCriteria == null) 5304 this.inclusionCriteria = new StringType(); 5305 this.inclusionCriteria.setValue(value); 5306 } 5307 return this; 5308 } 5309 5310 /** 5311 * @return {@link #exclusionCriteria} (Criteria describing which concepts or codes should be excluded and why.). This is the underlying object with id, value and extensions. The accessor "getExclusionCriteria" gives direct access to the value 5312 */ 5313 public StringType getExclusionCriteriaElement() { 5314 if (this.exclusionCriteria == null) 5315 if (Configuration.errorOnAutoCreate()) 5316 throw new Error("Attempt to auto-create ValueSetScopeComponent.exclusionCriteria"); 5317 else if (Configuration.doAutoCreate()) 5318 this.exclusionCriteria = new StringType(); // bb 5319 return this.exclusionCriteria; 5320 } 5321 5322 public boolean hasExclusionCriteriaElement() { 5323 return this.exclusionCriteria != null && !this.exclusionCriteria.isEmpty(); 5324 } 5325 5326 public boolean hasExclusionCriteria() { 5327 return this.exclusionCriteria != null && !this.exclusionCriteria.isEmpty(); 5328 } 5329 5330 /** 5331 * @param value {@link #exclusionCriteria} (Criteria describing which concepts or codes should be excluded and why.). This is the underlying object with id, value and extensions. The accessor "getExclusionCriteria" gives direct access to the value 5332 */ 5333 public ValueSetScopeComponent setExclusionCriteriaElement(StringType value) { 5334 this.exclusionCriteria = value; 5335 return this; 5336 } 5337 5338 /** 5339 * @return Criteria describing which concepts or codes should be excluded and why. 5340 */ 5341 public String getExclusionCriteria() { 5342 return this.exclusionCriteria == null ? null : this.exclusionCriteria.getValue(); 5343 } 5344 5345 /** 5346 * @param value Criteria describing which concepts or codes should be excluded and why. 5347 */ 5348 public ValueSetScopeComponent setExclusionCriteria(String value) { 5349 if (Utilities.noString(value)) 5350 this.exclusionCriteria = null; 5351 else { 5352 if (this.exclusionCriteria == null) 5353 this.exclusionCriteria = new StringType(); 5354 this.exclusionCriteria.setValue(value); 5355 } 5356 return this; 5357 } 5358 5359 protected void listChildren(List<Property> children) { 5360 super.listChildren(children); 5361 children.add(new Property("inclusionCriteria", "string", "Criteria describing which concepts or codes should be included and why.", 0, 1, inclusionCriteria)); 5362 children.add(new Property("exclusionCriteria", "string", "Criteria describing which concepts or codes should be excluded and why.", 0, 1, exclusionCriteria)); 5363 } 5364 5365 @Override 5366 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5367 switch (_hash) { 5368 case -1380638565: /*inclusionCriteria*/ return new Property("inclusionCriteria", "string", "Criteria describing which concepts or codes should be included and why.", 0, 1, inclusionCriteria); 5369 case 985682765: /*exclusionCriteria*/ return new Property("exclusionCriteria", "string", "Criteria describing which concepts or codes should be excluded and why.", 0, 1, exclusionCriteria); 5370 default: return super.getNamedProperty(_hash, _name, _checkValid); 5371 } 5372 5373 } 5374 5375 @Override 5376 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5377 switch (hash) { 5378 case -1380638565: /*inclusionCriteria*/ return this.inclusionCriteria == null ? new Base[0] : new Base[] {this.inclusionCriteria}; // StringType 5379 case 985682765: /*exclusionCriteria*/ return this.exclusionCriteria == null ? new Base[0] : new Base[] {this.exclusionCriteria}; // StringType 5380 default: return super.getProperty(hash, name, checkValid); 5381 } 5382 5383 } 5384 5385 @Override 5386 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5387 switch (hash) { 5388 case -1380638565: // inclusionCriteria 5389 this.inclusionCriteria = TypeConvertor.castToString(value); // StringType 5390 return value; 5391 case 985682765: // exclusionCriteria 5392 this.exclusionCriteria = TypeConvertor.castToString(value); // StringType 5393 return value; 5394 default: return super.setProperty(hash, name, value); 5395 } 5396 5397 } 5398 5399 @Override 5400 public Base setProperty(String name, Base value) throws FHIRException { 5401 if (name.equals("inclusionCriteria")) { 5402 this.inclusionCriteria = TypeConvertor.castToString(value); // StringType 5403 } else if (name.equals("exclusionCriteria")) { 5404 this.exclusionCriteria = TypeConvertor.castToString(value); // StringType 5405 } else 5406 return super.setProperty(name, value); 5407 return value; 5408 } 5409 5410 @Override 5411 public void removeChild(String name, Base value) throws FHIRException { 5412 if (name.equals("inclusionCriteria")) { 5413 this.inclusionCriteria = null; 5414 } else if (name.equals("exclusionCriteria")) { 5415 this.exclusionCriteria = null; 5416 } else 5417 super.removeChild(name, value); 5418 5419 } 5420 5421 @Override 5422 public Base makeProperty(int hash, String name) throws FHIRException { 5423 switch (hash) { 5424 case -1380638565: return getInclusionCriteriaElement(); 5425 case 985682765: return getExclusionCriteriaElement(); 5426 default: return super.makeProperty(hash, name); 5427 } 5428 5429 } 5430 5431 @Override 5432 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5433 switch (hash) { 5434 case -1380638565: /*inclusionCriteria*/ return new String[] {"string"}; 5435 case 985682765: /*exclusionCriteria*/ return new String[] {"string"}; 5436 default: return super.getTypesForProperty(hash, name); 5437 } 5438 5439 } 5440 5441 @Override 5442 public Base addChild(String name) throws FHIRException { 5443 if (name.equals("inclusionCriteria")) { 5444 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.scope.inclusionCriteria"); 5445 } 5446 else if (name.equals("exclusionCriteria")) { 5447 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.scope.exclusionCriteria"); 5448 } 5449 else 5450 return super.addChild(name); 5451 } 5452 5453 public ValueSetScopeComponent copy() { 5454 ValueSetScopeComponent dst = new ValueSetScopeComponent(); 5455 copyValues(dst); 5456 return dst; 5457 } 5458 5459 public void copyValues(ValueSetScopeComponent dst) { 5460 super.copyValues(dst); 5461 dst.inclusionCriteria = inclusionCriteria == null ? null : inclusionCriteria.copy(); 5462 dst.exclusionCriteria = exclusionCriteria == null ? null : exclusionCriteria.copy(); 5463 } 5464 5465 @Override 5466 public boolean equalsDeep(Base other_) { 5467 if (!super.equalsDeep(other_)) 5468 return false; 5469 if (!(other_ instanceof ValueSetScopeComponent)) 5470 return false; 5471 ValueSetScopeComponent o = (ValueSetScopeComponent) other_; 5472 return compareDeep(inclusionCriteria, o.inclusionCriteria, true) && compareDeep(exclusionCriteria, o.exclusionCriteria, true) 5473 ; 5474 } 5475 5476 @Override 5477 public boolean equalsShallow(Base other_) { 5478 if (!super.equalsShallow(other_)) 5479 return false; 5480 if (!(other_ instanceof ValueSetScopeComponent)) 5481 return false; 5482 ValueSetScopeComponent o = (ValueSetScopeComponent) other_; 5483 return compareValues(inclusionCriteria, o.inclusionCriteria, true) && compareValues(exclusionCriteria, o.exclusionCriteria, true) 5484 ; 5485 } 5486 5487 public boolean isEmpty() { 5488 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(inclusionCriteria, exclusionCriteria 5489 ); 5490 } 5491 5492 public String fhirType() { 5493 return "ValueSet.scope"; 5494 5495 } 5496 5497 } 5498 5499 /** 5500 * An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers. 5501 */ 5502 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 5503 @Description(shortDefinition="Canonical identifier for this value set, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers." ) 5504 protected UriType url; 5505 5506 /** 5507 * A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance. 5508 */ 5509 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5510 @Description(shortDefinition="Additional identifier for the value set (business identifier)", formalDefinition="A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 5511 protected List<Identifier> identifier; 5512 5513 /** 5514 * The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5515 */ 5516 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 5517 @Description(shortDefinition="Business version of the value set", formalDefinition="The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 5518 protected StringType version; 5519 5520 /** 5521 * Indicates the mechanism used to compare versions to determine which ValueSet is more current. 5522 */ 5523 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 5524 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which ValueSet is more current." ) 5525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 5526 protected DataType versionAlgorithm; 5527 5528 /** 5529 * A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5530 */ 5531 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 5532 @Description(shortDefinition="Name for this value set (computer friendly)", formalDefinition="A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 5533 protected StringType name; 5534 5535 /** 5536 * A short, descriptive, user-friendly title for the value set. 5537 */ 5538 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 5539 @Description(shortDefinition="Name for this value set (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the value set." ) 5540 protected StringType title; 5541 5542 /** 5543 * The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state. 5544 */ 5545 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 5546 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state." ) 5547 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 5548 protected Enumeration<PublicationStatus> status; 5549 5550 /** 5551 * A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5552 */ 5553 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 5554 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 5555 protected BooleanType experimental; 5556 5557 /** 5558 * The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised. 5559 */ 5560 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 5561 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised." ) 5562 protected DateTimeType date; 5563 5564 /** 5565 * The name of the organization or individual responsible for the release and ongoing maintenance of the value set. 5566 */ 5567 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 5568 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the value set." ) 5569 protected StringType publisher; 5570 5571 /** 5572 * Contact details to assist a user in finding and communicating with the publisher. 5573 */ 5574 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5575 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 5576 protected List<ContactDetail> contact; 5577 5578 /** 5579 * A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set. 5580 */ 5581 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 5582 @Description(shortDefinition="Natural language description of the value set", formalDefinition="A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set." ) 5583 protected MarkdownType description; 5584 5585 /** 5586 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances. 5587 */ 5588 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5589 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances." ) 5590 protected List<UsageContext> useContext; 5591 5592 /** 5593 * A legal or geographic region in which the value set is intended to be used. 5594 */ 5595 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5596 @Description(shortDefinition="Intended jurisdiction for value set (if applicable)", formalDefinition="A legal or geographic region in which the value set is intended to be used." ) 5597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 5598 protected List<CodeableConcept> jurisdiction; 5599 5600 /** 5601 * If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 5602 */ 5603 @Child(name = "immutable", type = {BooleanType.class}, order=14, min=0, max=1, modifier=false, summary=true) 5604 @Description(shortDefinition="Indicates whether or not any change to the content logical definition may occur", formalDefinition="If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change." ) 5605 protected BooleanType immutable; 5606 5607 /** 5608 * Explanation of why this value set is needed and why it has been designed as it has. 5609 */ 5610 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5611 @Description(shortDefinition="Why this value set is defined", formalDefinition="Explanation of why this value set is needed and why it has been designed as it has." ) 5612 protected MarkdownType purpose; 5613 5614 /** 5615 * A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 5616 */ 5617 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 5618 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set." ) 5619 protected MarkdownType copyright; 5620 5621 /** 5622 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5623 */ 5624 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 5625 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 5626 protected StringType copyrightLabel; 5627 5628 /** 5629 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 5630 */ 5631 @Child(name = "approvalDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 5632 @Description(shortDefinition="When the ValueSet was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 5633 protected DateType approvalDate; 5634 5635 /** 5636 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 5637 */ 5638 @Child(name = "lastReviewDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 5639 @Description(shortDefinition="When the ValueSet was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 5640 protected DateType lastReviewDate; 5641 5642 /** 5643 * The period during which the ValueSet content was or is planned to be in active use. 5644 */ 5645 @Child(name = "effectivePeriod", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=true) 5646 @Description(shortDefinition="When the ValueSet is expected to be used", formalDefinition="The period during which the ValueSet content was or is planned to be in active use." ) 5647 protected Period effectivePeriod; 5648 5649 /** 5650 * Descriptions related to the content of the ValueSet. Topics provide a high-level categorization as well as keywords for the ValueSet that can be useful for filtering and searching. 5651 */ 5652 @Child(name = "topic", type = {CodeableConcept.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5653 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptions related to the content of the ValueSet. Topics provide a high-level categorization as well as keywords for the ValueSet that can be useful for filtering and searching." ) 5654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 5655 protected List<CodeableConcept> topic; 5656 5657 /** 5658 * An individiual or organization primarily involved in the creation and maintenance of the ValueSet. 5659 */ 5660 @Child(name = "author", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5661 @Description(shortDefinition="Who authored the ValueSet", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the ValueSet." ) 5662 protected List<ContactDetail> author; 5663 5664 /** 5665 * An individual or organization primarily responsible for internal coherence of the ValueSet. 5666 */ 5667 @Child(name = "editor", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5668 @Description(shortDefinition="Who edited the ValueSet", formalDefinition="An individual or organization primarily responsible for internal coherence of the ValueSet." ) 5669 protected List<ContactDetail> editor; 5670 5671 /** 5672 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ValueSet. 5673 */ 5674 @Child(name = "reviewer", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5675 @Description(shortDefinition="Who reviewed the ValueSet", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ValueSet." ) 5676 protected List<ContactDetail> reviewer; 5677 5678 /** 5679 * An individual or organization asserted by the publisher to be responsible for officially endorsing the ValueSet for use in some setting. 5680 */ 5681 @Child(name = "endorser", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5682 @Description(shortDefinition="Who endorsed the ValueSet", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the ValueSet for use in some setting." ) 5683 protected List<ContactDetail> endorser; 5684 5685 /** 5686 * Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts. 5687 */ 5688 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5689 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts." ) 5690 protected List<RelatedArtifact> relatedArtifact; 5691 5692 /** 5693 * A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD). 5694 */ 5695 @Child(name = "compose", type = {}, order=27, min=0, max=1, modifier=false, summary=false) 5696 @Description(shortDefinition="Content logical definition of the value set (CLD)", formalDefinition="A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD)." ) 5697 protected ValueSetComposeComponent compose; 5698 5699 /** 5700 * A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed. 5701 */ 5702 @Child(name = "expansion", type = {}, order=28, min=0, max=1, modifier=false, summary=false) 5703 @Description(shortDefinition="Used when the value set is \"expanded\"", formalDefinition="A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed." ) 5704 protected ValueSetExpansionComponent expansion; 5705 5706 /** 5707 * Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description. 5708 */ 5709 @Child(name = "scope", type = {}, order=29, min=0, max=1, modifier=false, summary=false) 5710 @Description(shortDefinition="Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description", formalDefinition="Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description." ) 5711 protected ValueSetScopeComponent scope; 5712 5713 private static final long serialVersionUID = -1050676231L; 5714 5715 /** 5716 * Constructor 5717 */ 5718 public ValueSet() { 5719 super(); 5720 } 5721 5722 /** 5723 * Constructor 5724 */ 5725 public ValueSet(PublicationStatus status) { 5726 super(); 5727 this.setStatus(status); 5728 } 5729 5730 /** 5731 * @return {@link #url} (An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5732 */ 5733 public UriType getUrlElement() { 5734 if (this.url == null) 5735 if (Configuration.errorOnAutoCreate()) 5736 throw new Error("Attempt to auto-create ValueSet.url"); 5737 else if (Configuration.doAutoCreate()) 5738 this.url = new UriType(); // bb 5739 return this.url; 5740 } 5741 5742 public boolean hasUrlElement() { 5743 return this.url != null && !this.url.isEmpty(); 5744 } 5745 5746 public boolean hasUrl() { 5747 return this.url != null && !this.url.isEmpty(); 5748 } 5749 5750 /** 5751 * @param value {@link #url} (An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5752 */ 5753 public ValueSet setUrlElement(UriType value) { 5754 this.url = value; 5755 return this; 5756 } 5757 5758 /** 5759 * @return An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers. 5760 */ 5761 public String getUrl() { 5762 return this.url == null ? null : this.url.getValue(); 5763 } 5764 5765 /** 5766 * @param value An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers. 5767 */ 5768 public ValueSet setUrl(String value) { 5769 if (Utilities.noString(value)) 5770 this.url = null; 5771 else { 5772 if (this.url == null) 5773 this.url = new UriType(); 5774 this.url.setValue(value); 5775 } 5776 return this; 5777 } 5778 5779 /** 5780 * @return {@link #identifier} (A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.) 5781 */ 5782 public List<Identifier> getIdentifier() { 5783 if (this.identifier == null) 5784 this.identifier = new ArrayList<Identifier>(); 5785 return this.identifier; 5786 } 5787 5788 /** 5789 * @return Returns a reference to <code>this</code> for easy method chaining 5790 */ 5791 public ValueSet setIdentifier(List<Identifier> theIdentifier) { 5792 this.identifier = theIdentifier; 5793 return this; 5794 } 5795 5796 public boolean hasIdentifier() { 5797 if (this.identifier == null) 5798 return false; 5799 for (Identifier item : this.identifier) 5800 if (!item.isEmpty()) 5801 return true; 5802 return false; 5803 } 5804 5805 public Identifier addIdentifier() { //3 5806 Identifier t = new Identifier(); 5807 if (this.identifier == null) 5808 this.identifier = new ArrayList<Identifier>(); 5809 this.identifier.add(t); 5810 return t; 5811 } 5812 5813 public ValueSet addIdentifier(Identifier t) { //3 5814 if (t == null) 5815 return this; 5816 if (this.identifier == null) 5817 this.identifier = new ArrayList<Identifier>(); 5818 this.identifier.add(t); 5819 return this; 5820 } 5821 5822 /** 5823 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5824 */ 5825 public Identifier getIdentifierFirstRep() { 5826 if (getIdentifier().isEmpty()) { 5827 addIdentifier(); 5828 } 5829 return getIdentifier().get(0); 5830 } 5831 5832 /** 5833 * @return {@link #version} (The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5834 */ 5835 public StringType getVersionElement() { 5836 if (this.version == null) 5837 if (Configuration.errorOnAutoCreate()) 5838 throw new Error("Attempt to auto-create ValueSet.version"); 5839 else if (Configuration.doAutoCreate()) 5840 this.version = new StringType(); // bb 5841 return this.version; 5842 } 5843 5844 public boolean hasVersionElement() { 5845 return this.version != null && !this.version.isEmpty(); 5846 } 5847 5848 public boolean hasVersion() { 5849 return this.version != null && !this.version.isEmpty(); 5850 } 5851 5852 /** 5853 * @param value {@link #version} (The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5854 */ 5855 public ValueSet setVersionElement(StringType value) { 5856 this.version = value; 5857 return this; 5858 } 5859 5860 /** 5861 * @return The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5862 */ 5863 public String getVersion() { 5864 return this.version == null ? null : this.version.getValue(); 5865 } 5866 5867 /** 5868 * @param value The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5869 */ 5870 public ValueSet setVersion(String value) { 5871 if (Utilities.noString(value)) 5872 this.version = null; 5873 else { 5874 if (this.version == null) 5875 this.version = new StringType(); 5876 this.version.setValue(value); 5877 } 5878 return this; 5879 } 5880 5881 /** 5882 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ValueSet is more current.) 5883 */ 5884 public DataType getVersionAlgorithm() { 5885 return this.versionAlgorithm; 5886 } 5887 5888 /** 5889 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ValueSet is more current.) 5890 */ 5891 public StringType getVersionAlgorithmStringType() throws FHIRException { 5892 if (this.versionAlgorithm == null) 5893 this.versionAlgorithm = new StringType(); 5894 if (!(this.versionAlgorithm instanceof StringType)) 5895 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5896 return (StringType) this.versionAlgorithm; 5897 } 5898 5899 public boolean hasVersionAlgorithmStringType() { 5900 return this != null && this.versionAlgorithm instanceof StringType; 5901 } 5902 5903 /** 5904 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ValueSet is more current.) 5905 */ 5906 public Coding getVersionAlgorithmCoding() throws FHIRException { 5907 if (this.versionAlgorithm == null) 5908 this.versionAlgorithm = new Coding(); 5909 if (!(this.versionAlgorithm instanceof Coding)) 5910 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5911 return (Coding) this.versionAlgorithm; 5912 } 5913 5914 public boolean hasVersionAlgorithmCoding() { 5915 return this != null && this.versionAlgorithm instanceof Coding; 5916 } 5917 5918 public boolean hasVersionAlgorithm() { 5919 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 5920 } 5921 5922 /** 5923 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ValueSet is more current.) 5924 */ 5925 public ValueSet setVersionAlgorithm(DataType value) { 5926 if (value != null && !(value instanceof StringType || value instanceof Coding)) 5927 throw new FHIRException("Not the right type for ValueSet.versionAlgorithm[x]: "+value.fhirType()); 5928 this.versionAlgorithm = value; 5929 return this; 5930 } 5931 5932 /** 5933 * @return {@link #name} (A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5934 */ 5935 public StringType getNameElement() { 5936 if (this.name == null) 5937 if (Configuration.errorOnAutoCreate()) 5938 throw new Error("Attempt to auto-create ValueSet.name"); 5939 else if (Configuration.doAutoCreate()) 5940 this.name = new StringType(); // bb 5941 return this.name; 5942 } 5943 5944 public boolean hasNameElement() { 5945 return this.name != null && !this.name.isEmpty(); 5946 } 5947 5948 public boolean hasName() { 5949 return this.name != null && !this.name.isEmpty(); 5950 } 5951 5952 /** 5953 * @param value {@link #name} (A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5954 */ 5955 public ValueSet setNameElement(StringType value) { 5956 this.name = value; 5957 return this; 5958 } 5959 5960 /** 5961 * @return A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5962 */ 5963 public String getName() { 5964 return this.name == null ? null : this.name.getValue(); 5965 } 5966 5967 /** 5968 * @param value A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5969 */ 5970 public ValueSet setName(String value) { 5971 if (Utilities.noString(value)) 5972 this.name = null; 5973 else { 5974 if (this.name == null) 5975 this.name = new StringType(); 5976 this.name.setValue(value); 5977 } 5978 return this; 5979 } 5980 5981 /** 5982 * @return {@link #title} (A short, descriptive, user-friendly title for the value set.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5983 */ 5984 public StringType getTitleElement() { 5985 if (this.title == null) 5986 if (Configuration.errorOnAutoCreate()) 5987 throw new Error("Attempt to auto-create ValueSet.title"); 5988 else if (Configuration.doAutoCreate()) 5989 this.title = new StringType(); // bb 5990 return this.title; 5991 } 5992 5993 public boolean hasTitleElement() { 5994 return this.title != null && !this.title.isEmpty(); 5995 } 5996 5997 public boolean hasTitle() { 5998 return this.title != null && !this.title.isEmpty(); 5999 } 6000 6001 /** 6002 * @param value {@link #title} (A short, descriptive, user-friendly title for the value set.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 6003 */ 6004 public ValueSet setTitleElement(StringType value) { 6005 this.title = value; 6006 return this; 6007 } 6008 6009 /** 6010 * @return A short, descriptive, user-friendly title for the value set. 6011 */ 6012 public String getTitle() { 6013 return this.title == null ? null : this.title.getValue(); 6014 } 6015 6016 /** 6017 * @param value A short, descriptive, user-friendly title for the value set. 6018 */ 6019 public ValueSet setTitle(String value) { 6020 if (Utilities.noString(value)) 6021 this.title = null; 6022 else { 6023 if (this.title == null) 6024 this.title = new StringType(); 6025 this.title.setValue(value); 6026 } 6027 return this; 6028 } 6029 6030 /** 6031 * @return {@link #status} (The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6032 */ 6033 public Enumeration<PublicationStatus> getStatusElement() { 6034 if (this.status == null) 6035 if (Configuration.errorOnAutoCreate()) 6036 throw new Error("Attempt to auto-create ValueSet.status"); 6037 else if (Configuration.doAutoCreate()) 6038 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 6039 return this.status; 6040 } 6041 6042 public boolean hasStatusElement() { 6043 return this.status != null && !this.status.isEmpty(); 6044 } 6045 6046 public boolean hasStatus() { 6047 return this.status != null && !this.status.isEmpty(); 6048 } 6049 6050 /** 6051 * @param value {@link #status} (The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6052 */ 6053 public ValueSet setStatusElement(Enumeration<PublicationStatus> value) { 6054 this.status = value; 6055 return this; 6056 } 6057 6058 /** 6059 * @return The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state. 6060 */ 6061 public PublicationStatus getStatus() { 6062 return this.status == null ? null : this.status.getValue(); 6063 } 6064 6065 /** 6066 * @param value The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state. 6067 */ 6068 public ValueSet setStatus(PublicationStatus value) { 6069 if (this.status == null) 6070 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 6071 this.status.setValue(value); 6072 return this; 6073 } 6074 6075 /** 6076 * @return {@link #experimental} (A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 6077 */ 6078 public BooleanType getExperimentalElement() { 6079 if (this.experimental == null) 6080 if (Configuration.errorOnAutoCreate()) 6081 throw new Error("Attempt to auto-create ValueSet.experimental"); 6082 else if (Configuration.doAutoCreate()) 6083 this.experimental = new BooleanType(); // bb 6084 return this.experimental; 6085 } 6086 6087 public boolean hasExperimentalElement() { 6088 return this.experimental != null && !this.experimental.isEmpty(); 6089 } 6090 6091 public boolean hasExperimental() { 6092 return this.experimental != null && !this.experimental.isEmpty(); 6093 } 6094 6095 /** 6096 * @param value {@link #experimental} (A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 6097 */ 6098 public ValueSet setExperimentalElement(BooleanType value) { 6099 this.experimental = value; 6100 return this; 6101 } 6102 6103 /** 6104 * @return A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 6105 */ 6106 public boolean getExperimental() { 6107 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 6108 } 6109 6110 /** 6111 * @param value A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 6112 */ 6113 public ValueSet setExperimental(boolean value) { 6114 if (this.experimental == null) 6115 this.experimental = new BooleanType(); 6116 this.experimental.setValue(value); 6117 return this; 6118 } 6119 6120 /** 6121 * @return {@link #date} (The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6122 */ 6123 public DateTimeType getDateElement() { 6124 if (this.date == null) 6125 if (Configuration.errorOnAutoCreate()) 6126 throw new Error("Attempt to auto-create ValueSet.date"); 6127 else if (Configuration.doAutoCreate()) 6128 this.date = new DateTimeType(); // bb 6129 return this.date; 6130 } 6131 6132 public boolean hasDateElement() { 6133 return this.date != null && !this.date.isEmpty(); 6134 } 6135 6136 public boolean hasDate() { 6137 return this.date != null && !this.date.isEmpty(); 6138 } 6139 6140 /** 6141 * @param value {@link #date} (The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6142 */ 6143 public ValueSet setDateElement(DateTimeType value) { 6144 this.date = value; 6145 return this; 6146 } 6147 6148 /** 6149 * @return The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised. 6150 */ 6151 public Date getDate() { 6152 return this.date == null ? null : this.date.getValue(); 6153 } 6154 6155 /** 6156 * @param value The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised. 6157 */ 6158 public ValueSet setDate(Date value) { 6159 if (value == null) 6160 this.date = null; 6161 else { 6162 if (this.date == null) 6163 this.date = new DateTimeType(); 6164 this.date.setValue(value); 6165 } 6166 return this; 6167 } 6168 6169 /** 6170 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the value set.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6171 */ 6172 public StringType getPublisherElement() { 6173 if (this.publisher == null) 6174 if (Configuration.errorOnAutoCreate()) 6175 throw new Error("Attempt to auto-create ValueSet.publisher"); 6176 else if (Configuration.doAutoCreate()) 6177 this.publisher = new StringType(); // bb 6178 return this.publisher; 6179 } 6180 6181 public boolean hasPublisherElement() { 6182 return this.publisher != null && !this.publisher.isEmpty(); 6183 } 6184 6185 public boolean hasPublisher() { 6186 return this.publisher != null && !this.publisher.isEmpty(); 6187 } 6188 6189 /** 6190 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the value set.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6191 */ 6192 public ValueSet setPublisherElement(StringType value) { 6193 this.publisher = value; 6194 return this; 6195 } 6196 6197 /** 6198 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the value set. 6199 */ 6200 public String getPublisher() { 6201 return this.publisher == null ? null : this.publisher.getValue(); 6202 } 6203 6204 /** 6205 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the value set. 6206 */ 6207 public ValueSet setPublisher(String value) { 6208 if (Utilities.noString(value)) 6209 this.publisher = null; 6210 else { 6211 if (this.publisher == null) 6212 this.publisher = new StringType(); 6213 this.publisher.setValue(value); 6214 } 6215 return this; 6216 } 6217 6218 /** 6219 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 6220 */ 6221 public List<ContactDetail> getContact() { 6222 if (this.contact == null) 6223 this.contact = new ArrayList<ContactDetail>(); 6224 return this.contact; 6225 } 6226 6227 /** 6228 * @return Returns a reference to <code>this</code> for easy method chaining 6229 */ 6230 public ValueSet setContact(List<ContactDetail> theContact) { 6231 this.contact = theContact; 6232 return this; 6233 } 6234 6235 public boolean hasContact() { 6236 if (this.contact == null) 6237 return false; 6238 for (ContactDetail item : this.contact) 6239 if (!item.isEmpty()) 6240 return true; 6241 return false; 6242 } 6243 6244 public ContactDetail addContact() { //3 6245 ContactDetail t = new ContactDetail(); 6246 if (this.contact == null) 6247 this.contact = new ArrayList<ContactDetail>(); 6248 this.contact.add(t); 6249 return t; 6250 } 6251 6252 public ValueSet addContact(ContactDetail t) { //3 6253 if (t == null) 6254 return this; 6255 if (this.contact == null) 6256 this.contact = new ArrayList<ContactDetail>(); 6257 this.contact.add(t); 6258 return this; 6259 } 6260 6261 /** 6262 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 6263 */ 6264 public ContactDetail getContactFirstRep() { 6265 if (getContact().isEmpty()) { 6266 addContact(); 6267 } 6268 return getContact().get(0); 6269 } 6270 6271 /** 6272 * @return {@link #description} (A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6273 */ 6274 public MarkdownType getDescriptionElement() { 6275 if (this.description == null) 6276 if (Configuration.errorOnAutoCreate()) 6277 throw new Error("Attempt to auto-create ValueSet.description"); 6278 else if (Configuration.doAutoCreate()) 6279 this.description = new MarkdownType(); // bb 6280 return this.description; 6281 } 6282 6283 public boolean hasDescriptionElement() { 6284 return this.description != null && !this.description.isEmpty(); 6285 } 6286 6287 public boolean hasDescription() { 6288 return this.description != null && !this.description.isEmpty(); 6289 } 6290 6291 /** 6292 * @param value {@link #description} (A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6293 */ 6294 public ValueSet setDescriptionElement(MarkdownType value) { 6295 this.description = value; 6296 return this; 6297 } 6298 6299 /** 6300 * @return A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set. 6301 */ 6302 public String getDescription() { 6303 return this.description == null ? null : this.description.getValue(); 6304 } 6305 6306 /** 6307 * @param value A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set. 6308 */ 6309 public ValueSet setDescription(String value) { 6310 if (Utilities.noString(value)) 6311 this.description = null; 6312 else { 6313 if (this.description == null) 6314 this.description = new MarkdownType(); 6315 this.description.setValue(value); 6316 } 6317 return this; 6318 } 6319 6320 /** 6321 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances.) 6322 */ 6323 public List<UsageContext> getUseContext() { 6324 if (this.useContext == null) 6325 this.useContext = new ArrayList<UsageContext>(); 6326 return this.useContext; 6327 } 6328 6329 /** 6330 * @return Returns a reference to <code>this</code> for easy method chaining 6331 */ 6332 public ValueSet setUseContext(List<UsageContext> theUseContext) { 6333 this.useContext = theUseContext; 6334 return this; 6335 } 6336 6337 public boolean hasUseContext() { 6338 if (this.useContext == null) 6339 return false; 6340 for (UsageContext item : this.useContext) 6341 if (!item.isEmpty()) 6342 return true; 6343 return false; 6344 } 6345 6346 public UsageContext addUseContext() { //3 6347 UsageContext t = new UsageContext(); 6348 if (this.useContext == null) 6349 this.useContext = new ArrayList<UsageContext>(); 6350 this.useContext.add(t); 6351 return t; 6352 } 6353 6354 public ValueSet addUseContext(UsageContext t) { //3 6355 if (t == null) 6356 return this; 6357 if (this.useContext == null) 6358 this.useContext = new ArrayList<UsageContext>(); 6359 this.useContext.add(t); 6360 return this; 6361 } 6362 6363 /** 6364 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 6365 */ 6366 public UsageContext getUseContextFirstRep() { 6367 if (getUseContext().isEmpty()) { 6368 addUseContext(); 6369 } 6370 return getUseContext().get(0); 6371 } 6372 6373 /** 6374 * @return {@link #jurisdiction} (A legal or geographic region in which the value set is intended to be used.) 6375 */ 6376 public List<CodeableConcept> getJurisdiction() { 6377 if (this.jurisdiction == null) 6378 this.jurisdiction = new ArrayList<CodeableConcept>(); 6379 return this.jurisdiction; 6380 } 6381 6382 /** 6383 * @return Returns a reference to <code>this</code> for easy method chaining 6384 */ 6385 public ValueSet setJurisdiction(List<CodeableConcept> theJurisdiction) { 6386 this.jurisdiction = theJurisdiction; 6387 return this; 6388 } 6389 6390 public boolean hasJurisdiction() { 6391 if (this.jurisdiction == null) 6392 return false; 6393 for (CodeableConcept item : this.jurisdiction) 6394 if (!item.isEmpty()) 6395 return true; 6396 return false; 6397 } 6398 6399 public CodeableConcept addJurisdiction() { //3 6400 CodeableConcept t = new CodeableConcept(); 6401 if (this.jurisdiction == null) 6402 this.jurisdiction = new ArrayList<CodeableConcept>(); 6403 this.jurisdiction.add(t); 6404 return t; 6405 } 6406 6407 public ValueSet addJurisdiction(CodeableConcept t) { //3 6408 if (t == null) 6409 return this; 6410 if (this.jurisdiction == null) 6411 this.jurisdiction = new ArrayList<CodeableConcept>(); 6412 this.jurisdiction.add(t); 6413 return this; 6414 } 6415 6416 /** 6417 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 6418 */ 6419 public CodeableConcept getJurisdictionFirstRep() { 6420 if (getJurisdiction().isEmpty()) { 6421 addJurisdiction(); 6422 } 6423 return getJurisdiction().get(0); 6424 } 6425 6426 /** 6427 * @return {@link #immutable} (If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.). This is the underlying object with id, value and extensions. The accessor "getImmutable" gives direct access to the value 6428 */ 6429 public BooleanType getImmutableElement() { 6430 if (this.immutable == null) 6431 if (Configuration.errorOnAutoCreate()) 6432 throw new Error("Attempt to auto-create ValueSet.immutable"); 6433 else if (Configuration.doAutoCreate()) 6434 this.immutable = new BooleanType(); // bb 6435 return this.immutable; 6436 } 6437 6438 public boolean hasImmutableElement() { 6439 return this.immutable != null && !this.immutable.isEmpty(); 6440 } 6441 6442 public boolean hasImmutable() { 6443 return this.immutable != null && !this.immutable.isEmpty(); 6444 } 6445 6446 /** 6447 * @param value {@link #immutable} (If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.). This is the underlying object with id, value and extensions. The accessor "getImmutable" gives direct access to the value 6448 */ 6449 public ValueSet setImmutableElement(BooleanType value) { 6450 this.immutable = value; 6451 return this; 6452 } 6453 6454 /** 6455 * @return If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 6456 */ 6457 public boolean getImmutable() { 6458 return this.immutable == null || this.immutable.isEmpty() ? false : this.immutable.getValue(); 6459 } 6460 6461 /** 6462 * @param value If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 6463 */ 6464 public ValueSet setImmutable(boolean value) { 6465 if (this.immutable == null) 6466 this.immutable = new BooleanType(); 6467 this.immutable.setValue(value); 6468 return this; 6469 } 6470 6471 /** 6472 * @return {@link #purpose} (Explanation of why this value set is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6473 */ 6474 public MarkdownType getPurposeElement() { 6475 if (this.purpose == null) 6476 if (Configuration.errorOnAutoCreate()) 6477 throw new Error("Attempt to auto-create ValueSet.purpose"); 6478 else if (Configuration.doAutoCreate()) 6479 this.purpose = new MarkdownType(); // bb 6480 return this.purpose; 6481 } 6482 6483 public boolean hasPurposeElement() { 6484 return this.purpose != null && !this.purpose.isEmpty(); 6485 } 6486 6487 public boolean hasPurpose() { 6488 return this.purpose != null && !this.purpose.isEmpty(); 6489 } 6490 6491 /** 6492 * @param value {@link #purpose} (Explanation of why this value set is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6493 */ 6494 public ValueSet setPurposeElement(MarkdownType value) { 6495 this.purpose = value; 6496 return this; 6497 } 6498 6499 /** 6500 * @return Explanation of why this value set is needed and why it has been designed as it has. 6501 */ 6502 public String getPurpose() { 6503 return this.purpose == null ? null : this.purpose.getValue(); 6504 } 6505 6506 /** 6507 * @param value Explanation of why this value set is needed and why it has been designed as it has. 6508 */ 6509 public ValueSet setPurpose(String value) { 6510 if (Utilities.noString(value)) 6511 this.purpose = null; 6512 else { 6513 if (this.purpose == null) 6514 this.purpose = new MarkdownType(); 6515 this.purpose.setValue(value); 6516 } 6517 return this; 6518 } 6519 6520 /** 6521 * @return {@link #copyright} (A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6522 */ 6523 public MarkdownType getCopyrightElement() { 6524 if (this.copyright == null) 6525 if (Configuration.errorOnAutoCreate()) 6526 throw new Error("Attempt to auto-create ValueSet.copyright"); 6527 else if (Configuration.doAutoCreate()) 6528 this.copyright = new MarkdownType(); // bb 6529 return this.copyright; 6530 } 6531 6532 public boolean hasCopyrightElement() { 6533 return this.copyright != null && !this.copyright.isEmpty(); 6534 } 6535 6536 public boolean hasCopyright() { 6537 return this.copyright != null && !this.copyright.isEmpty(); 6538 } 6539 6540 /** 6541 * @param value {@link #copyright} (A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6542 */ 6543 public ValueSet setCopyrightElement(MarkdownType value) { 6544 this.copyright = value; 6545 return this; 6546 } 6547 6548 /** 6549 * @return A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 6550 */ 6551 public String getCopyright() { 6552 return this.copyright == null ? null : this.copyright.getValue(); 6553 } 6554 6555 /** 6556 * @param value A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 6557 */ 6558 public ValueSet setCopyright(String value) { 6559 if (Utilities.noString(value)) 6560 this.copyright = null; 6561 else { 6562 if (this.copyright == null) 6563 this.copyright = new MarkdownType(); 6564 this.copyright.setValue(value); 6565 } 6566 return this; 6567 } 6568 6569 /** 6570 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6571 */ 6572 public StringType getCopyrightLabelElement() { 6573 if (this.copyrightLabel == null) 6574 if (Configuration.errorOnAutoCreate()) 6575 throw new Error("Attempt to auto-create ValueSet.copyrightLabel"); 6576 else if (Configuration.doAutoCreate()) 6577 this.copyrightLabel = new StringType(); // bb 6578 return this.copyrightLabel; 6579 } 6580 6581 public boolean hasCopyrightLabelElement() { 6582 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6583 } 6584 6585 public boolean hasCopyrightLabel() { 6586 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6587 } 6588 6589 /** 6590 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6591 */ 6592 public ValueSet setCopyrightLabelElement(StringType value) { 6593 this.copyrightLabel = value; 6594 return this; 6595 } 6596 6597 /** 6598 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6599 */ 6600 public String getCopyrightLabel() { 6601 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 6602 } 6603 6604 /** 6605 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6606 */ 6607 public ValueSet setCopyrightLabel(String value) { 6608 if (Utilities.noString(value)) 6609 this.copyrightLabel = null; 6610 else { 6611 if (this.copyrightLabel == null) 6612 this.copyrightLabel = new StringType(); 6613 this.copyrightLabel.setValue(value); 6614 } 6615 return this; 6616 } 6617 6618 /** 6619 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 6620 */ 6621 public DateType getApprovalDateElement() { 6622 if (this.approvalDate == null) 6623 if (Configuration.errorOnAutoCreate()) 6624 throw new Error("Attempt to auto-create ValueSet.approvalDate"); 6625 else if (Configuration.doAutoCreate()) 6626 this.approvalDate = new DateType(); // bb 6627 return this.approvalDate; 6628 } 6629 6630 public boolean hasApprovalDateElement() { 6631 return this.approvalDate != null && !this.approvalDate.isEmpty(); 6632 } 6633 6634 public boolean hasApprovalDate() { 6635 return this.approvalDate != null && !this.approvalDate.isEmpty(); 6636 } 6637 6638 /** 6639 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 6640 */ 6641 public ValueSet setApprovalDateElement(DateType value) { 6642 this.approvalDate = value; 6643 return this; 6644 } 6645 6646 /** 6647 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 6648 */ 6649 public Date getApprovalDate() { 6650 return this.approvalDate == null ? null : this.approvalDate.getValue(); 6651 } 6652 6653 /** 6654 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 6655 */ 6656 public ValueSet setApprovalDate(Date value) { 6657 if (value == null) 6658 this.approvalDate = null; 6659 else { 6660 if (this.approvalDate == null) 6661 this.approvalDate = new DateType(); 6662 this.approvalDate.setValue(value); 6663 } 6664 return this; 6665 } 6666 6667 /** 6668 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 6669 */ 6670 public DateType getLastReviewDateElement() { 6671 if (this.lastReviewDate == null) 6672 if (Configuration.errorOnAutoCreate()) 6673 throw new Error("Attempt to auto-create ValueSet.lastReviewDate"); 6674 else if (Configuration.doAutoCreate()) 6675 this.lastReviewDate = new DateType(); // bb 6676 return this.lastReviewDate; 6677 } 6678 6679 public boolean hasLastReviewDateElement() { 6680 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 6681 } 6682 6683 public boolean hasLastReviewDate() { 6684 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 6685 } 6686 6687 /** 6688 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 6689 */ 6690 public ValueSet setLastReviewDateElement(DateType value) { 6691 this.lastReviewDate = value; 6692 return this; 6693 } 6694 6695 /** 6696 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 6697 */ 6698 public Date getLastReviewDate() { 6699 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 6700 } 6701 6702 /** 6703 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 6704 */ 6705 public ValueSet setLastReviewDate(Date value) { 6706 if (value == null) 6707 this.lastReviewDate = null; 6708 else { 6709 if (this.lastReviewDate == null) 6710 this.lastReviewDate = new DateType(); 6711 this.lastReviewDate.setValue(value); 6712 } 6713 return this; 6714 } 6715 6716 /** 6717 * @return {@link #effectivePeriod} (The period during which the ValueSet content was or is planned to be in active use.) 6718 */ 6719 public Period getEffectivePeriod() { 6720 if (this.effectivePeriod == null) 6721 if (Configuration.errorOnAutoCreate()) 6722 throw new Error("Attempt to auto-create ValueSet.effectivePeriod"); 6723 else if (Configuration.doAutoCreate()) 6724 this.effectivePeriod = new Period(); // cc 6725 return this.effectivePeriod; 6726 } 6727 6728 public boolean hasEffectivePeriod() { 6729 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 6730 } 6731 6732 /** 6733 * @param value {@link #effectivePeriod} (The period during which the ValueSet content was or is planned to be in active use.) 6734 */ 6735 public ValueSet setEffectivePeriod(Period value) { 6736 this.effectivePeriod = value; 6737 return this; 6738 } 6739 6740 /** 6741 * @return {@link #topic} (Descriptions related to the content of the ValueSet. Topics provide a high-level categorization as well as keywords for the ValueSet that can be useful for filtering and searching.) 6742 */ 6743 public List<CodeableConcept> getTopic() { 6744 if (this.topic == null) 6745 this.topic = new ArrayList<CodeableConcept>(); 6746 return this.topic; 6747 } 6748 6749 /** 6750 * @return Returns a reference to <code>this</code> for easy method chaining 6751 */ 6752 public ValueSet setTopic(List<CodeableConcept> theTopic) { 6753 this.topic = theTopic; 6754 return this; 6755 } 6756 6757 public boolean hasTopic() { 6758 if (this.topic == null) 6759 return false; 6760 for (CodeableConcept item : this.topic) 6761 if (!item.isEmpty()) 6762 return true; 6763 return false; 6764 } 6765 6766 public CodeableConcept addTopic() { //3 6767 CodeableConcept t = new CodeableConcept(); 6768 if (this.topic == null) 6769 this.topic = new ArrayList<CodeableConcept>(); 6770 this.topic.add(t); 6771 return t; 6772 } 6773 6774 public ValueSet addTopic(CodeableConcept t) { //3 6775 if (t == null) 6776 return this; 6777 if (this.topic == null) 6778 this.topic = new ArrayList<CodeableConcept>(); 6779 this.topic.add(t); 6780 return this; 6781 } 6782 6783 /** 6784 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 6785 */ 6786 public CodeableConcept getTopicFirstRep() { 6787 if (getTopic().isEmpty()) { 6788 addTopic(); 6789 } 6790 return getTopic().get(0); 6791 } 6792 6793 /** 6794 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the ValueSet.) 6795 */ 6796 public List<ContactDetail> getAuthor() { 6797 if (this.author == null) 6798 this.author = new ArrayList<ContactDetail>(); 6799 return this.author; 6800 } 6801 6802 /** 6803 * @return Returns a reference to <code>this</code> for easy method chaining 6804 */ 6805 public ValueSet setAuthor(List<ContactDetail> theAuthor) { 6806 this.author = theAuthor; 6807 return this; 6808 } 6809 6810 public boolean hasAuthor() { 6811 if (this.author == null) 6812 return false; 6813 for (ContactDetail item : this.author) 6814 if (!item.isEmpty()) 6815 return true; 6816 return false; 6817 } 6818 6819 public ContactDetail addAuthor() { //3 6820 ContactDetail t = new ContactDetail(); 6821 if (this.author == null) 6822 this.author = new ArrayList<ContactDetail>(); 6823 this.author.add(t); 6824 return t; 6825 } 6826 6827 public ValueSet addAuthor(ContactDetail t) { //3 6828 if (t == null) 6829 return this; 6830 if (this.author == null) 6831 this.author = new ArrayList<ContactDetail>(); 6832 this.author.add(t); 6833 return this; 6834 } 6835 6836 /** 6837 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 6838 */ 6839 public ContactDetail getAuthorFirstRep() { 6840 if (getAuthor().isEmpty()) { 6841 addAuthor(); 6842 } 6843 return getAuthor().get(0); 6844 } 6845 6846 /** 6847 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the ValueSet.) 6848 */ 6849 public List<ContactDetail> getEditor() { 6850 if (this.editor == null) 6851 this.editor = new ArrayList<ContactDetail>(); 6852 return this.editor; 6853 } 6854 6855 /** 6856 * @return Returns a reference to <code>this</code> for easy method chaining 6857 */ 6858 public ValueSet setEditor(List<ContactDetail> theEditor) { 6859 this.editor = theEditor; 6860 return this; 6861 } 6862 6863 public boolean hasEditor() { 6864 if (this.editor == null) 6865 return false; 6866 for (ContactDetail item : this.editor) 6867 if (!item.isEmpty()) 6868 return true; 6869 return false; 6870 } 6871 6872 public ContactDetail addEditor() { //3 6873 ContactDetail t = new ContactDetail(); 6874 if (this.editor == null) 6875 this.editor = new ArrayList<ContactDetail>(); 6876 this.editor.add(t); 6877 return t; 6878 } 6879 6880 public ValueSet addEditor(ContactDetail t) { //3 6881 if (t == null) 6882 return this; 6883 if (this.editor == null) 6884 this.editor = new ArrayList<ContactDetail>(); 6885 this.editor.add(t); 6886 return this; 6887 } 6888 6889 /** 6890 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 6891 */ 6892 public ContactDetail getEditorFirstRep() { 6893 if (getEditor().isEmpty()) { 6894 addEditor(); 6895 } 6896 return getEditor().get(0); 6897 } 6898 6899 /** 6900 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ValueSet.) 6901 */ 6902 public List<ContactDetail> getReviewer() { 6903 if (this.reviewer == null) 6904 this.reviewer = new ArrayList<ContactDetail>(); 6905 return this.reviewer; 6906 } 6907 6908 /** 6909 * @return Returns a reference to <code>this</code> for easy method chaining 6910 */ 6911 public ValueSet setReviewer(List<ContactDetail> theReviewer) { 6912 this.reviewer = theReviewer; 6913 return this; 6914 } 6915 6916 public boolean hasReviewer() { 6917 if (this.reviewer == null) 6918 return false; 6919 for (ContactDetail item : this.reviewer) 6920 if (!item.isEmpty()) 6921 return true; 6922 return false; 6923 } 6924 6925 public ContactDetail addReviewer() { //3 6926 ContactDetail t = new ContactDetail(); 6927 if (this.reviewer == null) 6928 this.reviewer = new ArrayList<ContactDetail>(); 6929 this.reviewer.add(t); 6930 return t; 6931 } 6932 6933 public ValueSet addReviewer(ContactDetail t) { //3 6934 if (t == null) 6935 return this; 6936 if (this.reviewer == null) 6937 this.reviewer = new ArrayList<ContactDetail>(); 6938 this.reviewer.add(t); 6939 return this; 6940 } 6941 6942 /** 6943 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 6944 */ 6945 public ContactDetail getReviewerFirstRep() { 6946 if (getReviewer().isEmpty()) { 6947 addReviewer(); 6948 } 6949 return getReviewer().get(0); 6950 } 6951 6952 /** 6953 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the ValueSet for use in some setting.) 6954 */ 6955 public List<ContactDetail> getEndorser() { 6956 if (this.endorser == null) 6957 this.endorser = new ArrayList<ContactDetail>(); 6958 return this.endorser; 6959 } 6960 6961 /** 6962 * @return Returns a reference to <code>this</code> for easy method chaining 6963 */ 6964 public ValueSet setEndorser(List<ContactDetail> theEndorser) { 6965 this.endorser = theEndorser; 6966 return this; 6967 } 6968 6969 public boolean hasEndorser() { 6970 if (this.endorser == null) 6971 return false; 6972 for (ContactDetail item : this.endorser) 6973 if (!item.isEmpty()) 6974 return true; 6975 return false; 6976 } 6977 6978 public ContactDetail addEndorser() { //3 6979 ContactDetail t = new ContactDetail(); 6980 if (this.endorser == null) 6981 this.endorser = new ArrayList<ContactDetail>(); 6982 this.endorser.add(t); 6983 return t; 6984 } 6985 6986 public ValueSet addEndorser(ContactDetail t) { //3 6987 if (t == null) 6988 return this; 6989 if (this.endorser == null) 6990 this.endorser = new ArrayList<ContactDetail>(); 6991 this.endorser.add(t); 6992 return this; 6993 } 6994 6995 /** 6996 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 6997 */ 6998 public ContactDetail getEndorserFirstRep() { 6999 if (getEndorser().isEmpty()) { 7000 addEndorser(); 7001 } 7002 return getEndorser().get(0); 7003 } 7004 7005 /** 7006 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 7007 */ 7008 public List<RelatedArtifact> getRelatedArtifact() { 7009 if (this.relatedArtifact == null) 7010 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7011 return this.relatedArtifact; 7012 } 7013 7014 /** 7015 * @return Returns a reference to <code>this</code> for easy method chaining 7016 */ 7017 public ValueSet setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 7018 this.relatedArtifact = theRelatedArtifact; 7019 return this; 7020 } 7021 7022 public boolean hasRelatedArtifact() { 7023 if (this.relatedArtifact == null) 7024 return false; 7025 for (RelatedArtifact item : this.relatedArtifact) 7026 if (!item.isEmpty()) 7027 return true; 7028 return false; 7029 } 7030 7031 public RelatedArtifact addRelatedArtifact() { //3 7032 RelatedArtifact t = new RelatedArtifact(); 7033 if (this.relatedArtifact == null) 7034 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7035 this.relatedArtifact.add(t); 7036 return t; 7037 } 7038 7039 public ValueSet addRelatedArtifact(RelatedArtifact t) { //3 7040 if (t == null) 7041 return this; 7042 if (this.relatedArtifact == null) 7043 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 7044 this.relatedArtifact.add(t); 7045 return this; 7046 } 7047 7048 /** 7049 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 7050 */ 7051 public RelatedArtifact getRelatedArtifactFirstRep() { 7052 if (getRelatedArtifact().isEmpty()) { 7053 addRelatedArtifact(); 7054 } 7055 return getRelatedArtifact().get(0); 7056 } 7057 7058 /** 7059 * @return {@link #compose} (A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).) 7060 */ 7061 public ValueSetComposeComponent getCompose() { 7062 if (this.compose == null) 7063 if (Configuration.errorOnAutoCreate()) 7064 throw new Error("Attempt to auto-create ValueSet.compose"); 7065 else if (Configuration.doAutoCreate()) 7066 this.compose = new ValueSetComposeComponent(); // cc 7067 return this.compose; 7068 } 7069 7070 public boolean hasCompose() { 7071 return this.compose != null && !this.compose.isEmpty(); 7072 } 7073 7074 /** 7075 * @param value {@link #compose} (A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).) 7076 */ 7077 public ValueSet setCompose(ValueSetComposeComponent value) { 7078 this.compose = value; 7079 return this; 7080 } 7081 7082 /** 7083 * @return {@link #expansion} (A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.) 7084 */ 7085 public ValueSetExpansionComponent getExpansion() { 7086 if (this.expansion == null) 7087 if (Configuration.errorOnAutoCreate()) 7088 throw new Error("Attempt to auto-create ValueSet.expansion"); 7089 else if (Configuration.doAutoCreate()) 7090 this.expansion = new ValueSetExpansionComponent(); // cc 7091 return this.expansion; 7092 } 7093 7094 public boolean hasExpansion() { 7095 return this.expansion != null && !this.expansion.isEmpty(); 7096 } 7097 7098 /** 7099 * @param value {@link #expansion} (A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.) 7100 */ 7101 public ValueSet setExpansion(ValueSetExpansionComponent value) { 7102 this.expansion = value; 7103 return this; 7104 } 7105 7106 /** 7107 * @return {@link #scope} (Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description.) 7108 */ 7109 public ValueSetScopeComponent getScope() { 7110 if (this.scope == null) 7111 if (Configuration.errorOnAutoCreate()) 7112 throw new Error("Attempt to auto-create ValueSet.scope"); 7113 else if (Configuration.doAutoCreate()) 7114 this.scope = new ValueSetScopeComponent(); // cc 7115 return this.scope; 7116 } 7117 7118 public boolean hasScope() { 7119 return this.scope != null && !this.scope.isEmpty(); 7120 } 7121 7122 /** 7123 * @param value {@link #scope} (Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description.) 7124 */ 7125 public ValueSet setScope(ValueSetScopeComponent value) { 7126 this.scope = value; 7127 return this; 7128 } 7129 7130 protected void listChildren(List<Property> children) { 7131 super.listChildren(children); 7132 children.add(new Property("url", "uri", "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.", 0, 1, url)); 7133 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 7134 children.add(new Property("version", "string", "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 7135 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ValueSet is more current.", 0, 1, versionAlgorithm)); 7136 children.add(new Property("name", "string", "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 7137 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 0, 1, title)); 7138 children.add(new Property("status", "code", "The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.", 0, 1, status)); 7139 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 7140 children.add(new Property("date", "dateTime", "The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised.", 0, 1, date)); 7141 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the value set.", 0, 1, publisher)); 7142 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 7143 children.add(new Property("description", "markdown", "A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.", 0, 1, description)); 7144 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 7145 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 7146 children.add(new Property("immutable", "boolean", "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 0, 1, immutable)); 7147 children.add(new Property("purpose", "markdown", "Explanation of why this value set is needed and why it has been designed as it has.", 0, 1, purpose)); 7148 children.add(new Property("copyright", "markdown", "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 0, 1, copyright)); 7149 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 7150 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 7151 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 7152 children.add(new Property("effectivePeriod", "Period", "The period during which the ValueSet content was or is planned to be in active use.", 0, 1, effectivePeriod)); 7153 children.add(new Property("topic", "CodeableConcept", "Descriptions related to the content of the ValueSet. Topics provide a high-level categorization as well as keywords for the ValueSet that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 7154 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, author)); 7155 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, editor)); 7156 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 7157 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the ValueSet for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 7158 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 7159 children.add(new Property("compose", "", "A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).", 0, 1, compose)); 7160 children.add(new Property("expansion", "", "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 0, 1, expansion)); 7161 children.add(new Property("scope", "", "Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description.", 0, 1, scope)); 7162 } 7163 7164 @Override 7165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7166 switch (_hash) { 7167 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.", 0, 1, url); 7168 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 7169 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 7170 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ValueSet is more current.", 0, 1, versionAlgorithm); 7171 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ValueSet is more current.", 0, 1, versionAlgorithm); 7172 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which ValueSet is more current.", 0, 1, versionAlgorithm); 7173 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which ValueSet is more current.", 0, 1, versionAlgorithm); 7174 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 7175 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 0, 1, title); 7176 case -892481550: /*status*/ return new Property("status", "code", "The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.", 0, 1, status); 7177 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 7178 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the value set metadata or content logical definition (.compose) was created or revised.", 0, 1, date); 7179 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the value set.", 0, 1, publisher); 7180 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 7181 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.", 0, 1, description); 7182 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 7183 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 7184 case 1596987778: /*immutable*/ return new Property("immutable", "boolean", "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 0, 1, immutable); 7185 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this value set is needed and why it has been designed as it has.", 0, 1, purpose); 7186 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 0, 1, copyright); 7187 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 7188 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 7189 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 7190 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the ValueSet content was or is planned to be in active use.", 0, 1, effectivePeriod); 7191 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptions related to the content of the ValueSet. Topics provide a high-level categorization as well as keywords for the ValueSet that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 7192 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, author); 7193 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, editor); 7194 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ValueSet.", 0, java.lang.Integer.MAX_VALUE, reviewer); 7195 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the ValueSet for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 7196 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 7197 case 950497682: /*compose*/ return new Property("compose", "", "A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).", 0, 1, compose); 7198 case 17878207: /*expansion*/ return new Property("expansion", "", "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 0, 1, expansion); 7199 case 109264468: /*scope*/ return new Property("scope", "", "Description of the semantic space the Value Set Expansion is intended to cover and should further clarify the text in ValueSet.description.", 0, 1, scope); 7200 default: return super.getNamedProperty(_hash, _name, _checkValid); 7201 } 7202 7203 } 7204 7205 @Override 7206 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7207 switch (hash) { 7208 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 7209 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 7210 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 7211 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 7212 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 7213 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 7214 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 7215 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 7216 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 7217 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 7218 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 7219 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 7220 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 7221 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 7222 case 1596987778: /*immutable*/ return this.immutable == null ? new Base[0] : new Base[] {this.immutable}; // BooleanType 7223 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 7224 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 7225 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 7226 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 7227 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 7228 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 7229 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 7230 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 7231 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 7232 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 7233 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 7234 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 7235 case 950497682: /*compose*/ return this.compose == null ? new Base[0] : new Base[] {this.compose}; // ValueSetComposeComponent 7236 case 17878207: /*expansion*/ return this.expansion == null ? new Base[0] : new Base[] {this.expansion}; // ValueSetExpansionComponent 7237 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // ValueSetScopeComponent 7238 default: return super.getProperty(hash, name, checkValid); 7239 } 7240 7241 } 7242 7243 @Override 7244 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7245 switch (hash) { 7246 case 116079: // url 7247 this.url = TypeConvertor.castToUri(value); // UriType 7248 return value; 7249 case -1618432855: // identifier 7250 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 7251 return value; 7252 case 351608024: // version 7253 this.version = TypeConvertor.castToString(value); // StringType 7254 return value; 7255 case 1508158071: // versionAlgorithm 7256 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 7257 return value; 7258 case 3373707: // name 7259 this.name = TypeConvertor.castToString(value); // StringType 7260 return value; 7261 case 110371416: // title 7262 this.title = TypeConvertor.castToString(value); // StringType 7263 return value; 7264 case -892481550: // status 7265 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 7266 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 7267 return value; 7268 case -404562712: // experimental 7269 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 7270 return value; 7271 case 3076014: // date 7272 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 7273 return value; 7274 case 1447404028: // publisher 7275 this.publisher = TypeConvertor.castToString(value); // StringType 7276 return value; 7277 case 951526432: // contact 7278 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 7279 return value; 7280 case -1724546052: // description 7281 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 7282 return value; 7283 case -669707736: // useContext 7284 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 7285 return value; 7286 case -507075711: // jurisdiction 7287 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7288 return value; 7289 case 1596987778: // immutable 7290 this.immutable = TypeConvertor.castToBoolean(value); // BooleanType 7291 return value; 7292 case -220463842: // purpose 7293 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 7294 return value; 7295 case 1522889671: // copyright 7296 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 7297 return value; 7298 case 765157229: // copyrightLabel 7299 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 7300 return value; 7301 case 223539345: // approvalDate 7302 this.approvalDate = TypeConvertor.castToDate(value); // DateType 7303 return value; 7304 case -1687512484: // lastReviewDate 7305 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 7306 return value; 7307 case -403934648: // effectivePeriod 7308 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 7309 return value; 7310 case 110546223: // topic 7311 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7312 return value; 7313 case -1406328437: // author 7314 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 7315 return value; 7316 case -1307827859: // editor 7317 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 7318 return value; 7319 case -261190139: // reviewer 7320 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 7321 return value; 7322 case 1740277666: // endorser 7323 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 7324 return value; 7325 case 666807069: // relatedArtifact 7326 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 7327 return value; 7328 case 950497682: // compose 7329 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 7330 return value; 7331 case 17878207: // expansion 7332 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 7333 return value; 7334 case 109264468: // scope 7335 this.scope = (ValueSetScopeComponent) value; // ValueSetScopeComponent 7336 return value; 7337 default: return super.setProperty(hash, name, value); 7338 } 7339 7340 } 7341 7342 @Override 7343 public Base setProperty(String name, Base value) throws FHIRException { 7344 if (name.equals("url")) { 7345 this.url = TypeConvertor.castToUri(value); // UriType 7346 } else if (name.equals("identifier")) { 7347 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 7348 } else if (name.equals("version")) { 7349 this.version = TypeConvertor.castToString(value); // StringType 7350 } else if (name.equals("versionAlgorithm[x]")) { 7351 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 7352 } else if (name.equals("name")) { 7353 this.name = TypeConvertor.castToString(value); // StringType 7354 } else if (name.equals("title")) { 7355 this.title = TypeConvertor.castToString(value); // StringType 7356 } else if (name.equals("status")) { 7357 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 7358 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 7359 } else if (name.equals("experimental")) { 7360 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 7361 } else if (name.equals("date")) { 7362 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 7363 } else if (name.equals("publisher")) { 7364 this.publisher = TypeConvertor.castToString(value); // StringType 7365 } else if (name.equals("contact")) { 7366 this.getContact().add(TypeConvertor.castToContactDetail(value)); 7367 } else if (name.equals("description")) { 7368 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 7369 } else if (name.equals("useContext")) { 7370 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 7371 } else if (name.equals("jurisdiction")) { 7372 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 7373 } else if (name.equals("immutable")) { 7374 this.immutable = TypeConvertor.castToBoolean(value); // BooleanType 7375 } else if (name.equals("purpose")) { 7376 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 7377 } else if (name.equals("copyright")) { 7378 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 7379 } else if (name.equals("copyrightLabel")) { 7380 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 7381 } else if (name.equals("approvalDate")) { 7382 this.approvalDate = TypeConvertor.castToDate(value); // DateType 7383 } else if (name.equals("lastReviewDate")) { 7384 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 7385 } else if (name.equals("effectivePeriod")) { 7386 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 7387 } else if (name.equals("topic")) { 7388 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 7389 } else if (name.equals("author")) { 7390 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 7391 } else if (name.equals("editor")) { 7392 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 7393 } else if (name.equals("reviewer")) { 7394 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 7395 } else if (name.equals("endorser")) { 7396 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 7397 } else if (name.equals("relatedArtifact")) { 7398 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 7399 } else if (name.equals("compose")) { 7400 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 7401 } else if (name.equals("expansion")) { 7402 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 7403 } else if (name.equals("scope")) { 7404 this.scope = (ValueSetScopeComponent) value; // ValueSetScopeComponent 7405 } else 7406 return super.setProperty(name, value); 7407 return value; 7408 } 7409 7410 @Override 7411 public void removeChild(String name, Base value) throws FHIRException { 7412 if (name.equals("url")) { 7413 this.url = null; 7414 } else if (name.equals("identifier")) { 7415 this.getIdentifier().remove(value); 7416 } else if (name.equals("version")) { 7417 this.version = null; 7418 } else if (name.equals("versionAlgorithm[x]")) { 7419 this.versionAlgorithm = null; 7420 } else if (name.equals("name")) { 7421 this.name = null; 7422 } else if (name.equals("title")) { 7423 this.title = null; 7424 } else if (name.equals("status")) { 7425 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 7426 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 7427 } else if (name.equals("experimental")) { 7428 this.experimental = null; 7429 } else if (name.equals("date")) { 7430 this.date = null; 7431 } else if (name.equals("publisher")) { 7432 this.publisher = null; 7433 } else if (name.equals("contact")) { 7434 this.getContact().remove(value); 7435 } else if (name.equals("description")) { 7436 this.description = null; 7437 } else if (name.equals("useContext")) { 7438 this.getUseContext().remove(value); 7439 } else if (name.equals("jurisdiction")) { 7440 this.getJurisdiction().remove(value); 7441 } else if (name.equals("immutable")) { 7442 this.immutable = null; 7443 } else if (name.equals("purpose")) { 7444 this.purpose = null; 7445 } else if (name.equals("copyright")) { 7446 this.copyright = null; 7447 } else if (name.equals("copyrightLabel")) { 7448 this.copyrightLabel = null; 7449 } else if (name.equals("approvalDate")) { 7450 this.approvalDate = null; 7451 } else if (name.equals("lastReviewDate")) { 7452 this.lastReviewDate = null; 7453 } else if (name.equals("effectivePeriod")) { 7454 this.effectivePeriod = null; 7455 } else if (name.equals("topic")) { 7456 this.getTopic().remove(value); 7457 } else if (name.equals("author")) { 7458 this.getAuthor().remove(value); 7459 } else if (name.equals("editor")) { 7460 this.getEditor().remove(value); 7461 } else if (name.equals("reviewer")) { 7462 this.getReviewer().remove(value); 7463 } else if (name.equals("endorser")) { 7464 this.getEndorser().remove(value); 7465 } else if (name.equals("relatedArtifact")) { 7466 this.getRelatedArtifact().remove(value); 7467 } else if (name.equals("compose")) { 7468 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 7469 } else if (name.equals("expansion")) { 7470 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 7471 } else if (name.equals("scope")) { 7472 this.scope = (ValueSetScopeComponent) value; // ValueSetScopeComponent 7473 } else 7474 super.removeChild(name, value); 7475 7476 } 7477 7478 @Override 7479 public Base makeProperty(int hash, String name) throws FHIRException { 7480 switch (hash) { 7481 case 116079: return getUrlElement(); 7482 case -1618432855: return addIdentifier(); 7483 case 351608024: return getVersionElement(); 7484 case -115699031: return getVersionAlgorithm(); 7485 case 1508158071: return getVersionAlgorithm(); 7486 case 3373707: return getNameElement(); 7487 case 110371416: return getTitleElement(); 7488 case -892481550: return getStatusElement(); 7489 case -404562712: return getExperimentalElement(); 7490 case 3076014: return getDateElement(); 7491 case 1447404028: return getPublisherElement(); 7492 case 951526432: return addContact(); 7493 case -1724546052: return getDescriptionElement(); 7494 case -669707736: return addUseContext(); 7495 case -507075711: return addJurisdiction(); 7496 case 1596987778: return getImmutableElement(); 7497 case -220463842: return getPurposeElement(); 7498 case 1522889671: return getCopyrightElement(); 7499 case 765157229: return getCopyrightLabelElement(); 7500 case 223539345: return getApprovalDateElement(); 7501 case -1687512484: return getLastReviewDateElement(); 7502 case -403934648: return getEffectivePeriod(); 7503 case 110546223: return addTopic(); 7504 case -1406328437: return addAuthor(); 7505 case -1307827859: return addEditor(); 7506 case -261190139: return addReviewer(); 7507 case 1740277666: return addEndorser(); 7508 case 666807069: return addRelatedArtifact(); 7509 case 950497682: return getCompose(); 7510 case 17878207: return getExpansion(); 7511 case 109264468: return getScope(); 7512 default: return super.makeProperty(hash, name); 7513 } 7514 7515 } 7516 7517 @Override 7518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7519 switch (hash) { 7520 case 116079: /*url*/ return new String[] {"uri"}; 7521 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 7522 case 351608024: /*version*/ return new String[] {"string"}; 7523 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 7524 case 3373707: /*name*/ return new String[] {"string"}; 7525 case 110371416: /*title*/ return new String[] {"string"}; 7526 case -892481550: /*status*/ return new String[] {"code"}; 7527 case -404562712: /*experimental*/ return new String[] {"boolean"}; 7528 case 3076014: /*date*/ return new String[] {"dateTime"}; 7529 case 1447404028: /*publisher*/ return new String[] {"string"}; 7530 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 7531 case -1724546052: /*description*/ return new String[] {"markdown"}; 7532 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 7533 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 7534 case 1596987778: /*immutable*/ return new String[] {"boolean"}; 7535 case -220463842: /*purpose*/ return new String[] {"markdown"}; 7536 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 7537 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 7538 case 223539345: /*approvalDate*/ return new String[] {"date"}; 7539 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 7540 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 7541 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 7542 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 7543 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 7544 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 7545 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 7546 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 7547 case 950497682: /*compose*/ return new String[] {}; 7548 case 17878207: /*expansion*/ return new String[] {}; 7549 case 109264468: /*scope*/ return new String[] {}; 7550 default: return super.getTypesForProperty(hash, name); 7551 } 7552 7553 } 7554 7555 @Override 7556 public Base addChild(String name) throws FHIRException { 7557 if (name.equals("url")) { 7558 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.url"); 7559 } 7560 else if (name.equals("identifier")) { 7561 return addIdentifier(); 7562 } 7563 else if (name.equals("version")) { 7564 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 7565 } 7566 else if (name.equals("versionAlgorithmString")) { 7567 this.versionAlgorithm = new StringType(); 7568 return this.versionAlgorithm; 7569 } 7570 else if (name.equals("versionAlgorithmCoding")) { 7571 this.versionAlgorithm = new Coding(); 7572 return this.versionAlgorithm; 7573 } 7574 else if (name.equals("name")) { 7575 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 7576 } 7577 else if (name.equals("title")) { 7578 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.title"); 7579 } 7580 else if (name.equals("status")) { 7581 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.status"); 7582 } 7583 else if (name.equals("experimental")) { 7584 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.experimental"); 7585 } 7586 else if (name.equals("date")) { 7587 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.date"); 7588 } 7589 else if (name.equals("publisher")) { 7590 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.publisher"); 7591 } 7592 else if (name.equals("contact")) { 7593 return addContact(); 7594 } 7595 else if (name.equals("description")) { 7596 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.description"); 7597 } 7598 else if (name.equals("useContext")) { 7599 return addUseContext(); 7600 } 7601 else if (name.equals("jurisdiction")) { 7602 return addJurisdiction(); 7603 } 7604 else if (name.equals("immutable")) { 7605 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.immutable"); 7606 } 7607 else if (name.equals("purpose")) { 7608 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.purpose"); 7609 } 7610 else if (name.equals("copyright")) { 7611 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.copyright"); 7612 } 7613 else if (name.equals("copyrightLabel")) { 7614 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.copyrightLabel"); 7615 } 7616 else if (name.equals("approvalDate")) { 7617 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.approvalDate"); 7618 } 7619 else if (name.equals("lastReviewDate")) { 7620 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.lastReviewDate"); 7621 } 7622 else if (name.equals("effectivePeriod")) { 7623 this.effectivePeriod = new Period(); 7624 return this.effectivePeriod; 7625 } 7626 else if (name.equals("topic")) { 7627 return addTopic(); 7628 } 7629 else if (name.equals("author")) { 7630 return addAuthor(); 7631 } 7632 else if (name.equals("editor")) { 7633 return addEditor(); 7634 } 7635 else if (name.equals("reviewer")) { 7636 return addReviewer(); 7637 } 7638 else if (name.equals("endorser")) { 7639 return addEndorser(); 7640 } 7641 else if (name.equals("relatedArtifact")) { 7642 return addRelatedArtifact(); 7643 } 7644 else if (name.equals("compose")) { 7645 this.compose = new ValueSetComposeComponent(); 7646 return this.compose; 7647 } 7648 else if (name.equals("expansion")) { 7649 this.expansion = new ValueSetExpansionComponent(); 7650 return this.expansion; 7651 } 7652 else if (name.equals("scope")) { 7653 this.scope = new ValueSetScopeComponent(); 7654 return this.scope; 7655 } 7656 else 7657 return super.addChild(name); 7658 } 7659 7660 public String fhirType() { 7661 return "ValueSet"; 7662 7663 } 7664 7665 public ValueSet copy() { 7666 ValueSet dst = new ValueSet(); 7667 copyValues(dst); 7668 return dst; 7669 } 7670 7671 public void copyValues(ValueSet dst) { 7672 super.copyValues(dst); 7673 dst.url = url == null ? null : url.copy(); 7674 if (identifier != null) { 7675 dst.identifier = new ArrayList<Identifier>(); 7676 for (Identifier i : identifier) 7677 dst.identifier.add(i.copy()); 7678 }; 7679 dst.version = version == null ? null : version.copy(); 7680 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 7681 dst.name = name == null ? null : name.copy(); 7682 dst.title = title == null ? null : title.copy(); 7683 dst.status = status == null ? null : status.copy(); 7684 dst.experimental = experimental == null ? null : experimental.copy(); 7685 dst.date = date == null ? null : date.copy(); 7686 dst.publisher = publisher == null ? null : publisher.copy(); 7687 if (contact != null) { 7688 dst.contact = new ArrayList<ContactDetail>(); 7689 for (ContactDetail i : contact) 7690 dst.contact.add(i.copy()); 7691 }; 7692 dst.description = description == null ? null : description.copy(); 7693 if (useContext != null) { 7694 dst.useContext = new ArrayList<UsageContext>(); 7695 for (UsageContext i : useContext) 7696 dst.useContext.add(i.copy()); 7697 }; 7698 if (jurisdiction != null) { 7699 dst.jurisdiction = new ArrayList<CodeableConcept>(); 7700 for (CodeableConcept i : jurisdiction) 7701 dst.jurisdiction.add(i.copy()); 7702 }; 7703 dst.immutable = immutable == null ? null : immutable.copy(); 7704 dst.purpose = purpose == null ? null : purpose.copy(); 7705 dst.copyright = copyright == null ? null : copyright.copy(); 7706 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 7707 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 7708 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 7709 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 7710 if (topic != null) { 7711 dst.topic = new ArrayList<CodeableConcept>(); 7712 for (CodeableConcept i : topic) 7713 dst.topic.add(i.copy()); 7714 }; 7715 if (author != null) { 7716 dst.author = new ArrayList<ContactDetail>(); 7717 for (ContactDetail i : author) 7718 dst.author.add(i.copy()); 7719 }; 7720 if (editor != null) { 7721 dst.editor = new ArrayList<ContactDetail>(); 7722 for (ContactDetail i : editor) 7723 dst.editor.add(i.copy()); 7724 }; 7725 if (reviewer != null) { 7726 dst.reviewer = new ArrayList<ContactDetail>(); 7727 for (ContactDetail i : reviewer) 7728 dst.reviewer.add(i.copy()); 7729 }; 7730 if (endorser != null) { 7731 dst.endorser = new ArrayList<ContactDetail>(); 7732 for (ContactDetail i : endorser) 7733 dst.endorser.add(i.copy()); 7734 }; 7735 if (relatedArtifact != null) { 7736 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 7737 for (RelatedArtifact i : relatedArtifact) 7738 dst.relatedArtifact.add(i.copy()); 7739 }; 7740 dst.compose = compose == null ? null : compose.copy(); 7741 dst.expansion = expansion == null ? null : expansion.copy(); 7742 dst.scope = scope == null ? null : scope.copy(); 7743 } 7744 7745 protected ValueSet typedCopy() { 7746 return copy(); 7747 } 7748 7749 @Override 7750 public boolean equalsDeep(Base other_) { 7751 if (!super.equalsDeep(other_)) 7752 return false; 7753 if (!(other_ instanceof ValueSet)) 7754 return false; 7755 ValueSet o = (ValueSet) other_; 7756 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 7757 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 7758 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 7759 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 7760 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 7761 && compareDeep(immutable, o.immutable, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 7762 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 7763 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 7764 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 7765 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 7766 && compareDeep(compose, o.compose, true) && compareDeep(expansion, o.expansion, true) && compareDeep(scope, o.scope, true) 7767 ; 7768 } 7769 7770 @Override 7771 public boolean equalsShallow(Base other_) { 7772 if (!super.equalsShallow(other_)) 7773 return false; 7774 if (!(other_ instanceof ValueSet)) 7775 return false; 7776 ValueSet o = (ValueSet) other_; 7777 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 7778 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 7779 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 7780 && compareValues(immutable, o.immutable, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 7781 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(approvalDate, o.approvalDate, true) 7782 && compareValues(lastReviewDate, o.lastReviewDate, true); 7783 } 7784 7785 public boolean isEmpty() { 7786 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 7787 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 7788 , description, useContext, jurisdiction, immutable, purpose, copyright, copyrightLabel 7789 , approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer 7790 , endorser, relatedArtifact, compose, expansion, scope); 7791 } 7792 7793 @Override 7794 public ResourceType getResourceType() { 7795 return ResourceType.ValueSet; 7796 } 7797 7798 /** 7799 * Search parameter: <b>context-quantity</b> 7800 * <p> 7801 * Description: <b>Multiple Resources: 7802 7803* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7804* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7805* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7806* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7807* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7808* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7809* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7810* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7811* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7812* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7813* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7814* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7815* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7816* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7817* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7818* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7819* [Library](library.html): A quantity- or range-valued use context assigned to the library 7820* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7821* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7822* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7823* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7824* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7825* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7826* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7827* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7828* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7829* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7830* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7831* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7832* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7833</b><br> 7834 * Type: <b>quantity</b><br> 7835 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7836 * </p> 7837 */ 7838 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 7839 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 7840 /** 7841 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 7842 * <p> 7843 * Description: <b>Multiple Resources: 7844 7845* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7846* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7847* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7848* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7849* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7850* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7851* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7852* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7853* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7854* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7855* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7856* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7857* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7858* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7859* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7860* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7861* [Library](library.html): A quantity- or range-valued use context assigned to the library 7862* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7863* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7864* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7865* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7866* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7867* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7868* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7869* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7870* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7871* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7872* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7873* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7874* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7875</b><br> 7876 * Type: <b>quantity</b><br> 7877 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7878 * </p> 7879 */ 7880 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 7881 7882 /** 7883 * Search parameter: <b>context-type-quantity</b> 7884 * <p> 7885 * Description: <b>Multiple Resources: 7886 7887* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7888* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7889* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7890* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7891* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7892* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7893* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7894* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7895* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7896* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7897* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7898* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7899* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7900* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7901* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7902* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7903* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7904* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7905* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7906* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7907* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7908* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7909* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7910* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7911* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7912* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7913* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7914* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7915* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7916* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7917</b><br> 7918 * Type: <b>composite</b><br> 7919 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7920 * </p> 7921 */ 7922 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 7923 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 7924 /** 7925 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 7926 * <p> 7927 * Description: <b>Multiple Resources: 7928 7929* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7930* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7931* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7932* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7933* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7934* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7935* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7936* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7937* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7938* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7939* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7940* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7941* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7942* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7943* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7944* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7945* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7946* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7947* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7948* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7949* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7950* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7951* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7952* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7953* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7954* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7955* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7956* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7957* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7958* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7959</b><br> 7960 * Type: <b>composite</b><br> 7961 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7962 * </p> 7963 */ 7964 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 7965 7966 /** 7967 * Search parameter: <b>context-type-value</b> 7968 * <p> 7969 * Description: <b>Multiple Resources: 7970 7971* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7972* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7973* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7974* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7975* [Citation](citation.html): A use context type and value assigned to the citation 7976* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7977* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7978* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7979* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7980* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7981* [Evidence](evidence.html): A use context type and value assigned to the evidence 7982* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7983* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7984* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7985* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7986* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7987* [Library](library.html): A use context type and value assigned to the library 7988* [Measure](measure.html): A use context type and value assigned to the measure 7989* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7990* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7991* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7992* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7993* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7994* [Requirements](requirements.html): A use context type and value assigned to the requirements 7995* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7996* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7997* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7998* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7999* [TestScript](testscript.html): A use context type and value assigned to the test script 8000* [ValueSet](valueset.html): A use context type and value assigned to the value set 8001</b><br> 8002 * Type: <b>composite</b><br> 8003 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 8004 * </p> 8005 */ 8006 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 8007 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 8008 /** 8009 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 8010 * <p> 8011 * Description: <b>Multiple Resources: 8012 8013* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 8014* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 8015* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 8016* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 8017* [Citation](citation.html): A use context type and value assigned to the citation 8018* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 8019* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 8020* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 8021* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 8022* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 8023* [Evidence](evidence.html): A use context type and value assigned to the evidence 8024* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 8025* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 8026* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 8027* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 8028* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 8029* [Library](library.html): A use context type and value assigned to the library 8030* [Measure](measure.html): A use context type and value assigned to the measure 8031* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 8032* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 8033* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 8034* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 8035* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 8036* [Requirements](requirements.html): A use context type and value assigned to the requirements 8037* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 8038* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 8039* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 8040* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 8041* [TestScript](testscript.html): A use context type and value assigned to the test script 8042* [ValueSet](valueset.html): A use context type and value assigned to the value set 8043</b><br> 8044 * Type: <b>composite</b><br> 8045 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 8046 * </p> 8047 */ 8048 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 8049 8050 /** 8051 * Search parameter: <b>context-type</b> 8052 * <p> 8053 * Description: <b>Multiple Resources: 8054 8055* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 8056* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 8057* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 8058* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 8059* [Citation](citation.html): A type of use context assigned to the citation 8060* [CodeSystem](codesystem.html): A type of use context assigned to the code system 8061* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 8062* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 8063* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 8064* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 8065* [Evidence](evidence.html): A type of use context assigned to the evidence 8066* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 8067* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 8068* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 8069* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 8070* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 8071* [Library](library.html): A type of use context assigned to the library 8072* [Measure](measure.html): A type of use context assigned to the measure 8073* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 8074* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 8075* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 8076* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 8077* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 8078* [Requirements](requirements.html): A type of use context assigned to the requirements 8079* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 8080* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 8081* [StructureMap](structuremap.html): A type of use context assigned to the structure map 8082* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 8083* [TestScript](testscript.html): A type of use context assigned to the test script 8084* [ValueSet](valueset.html): A type of use context assigned to the value set 8085</b><br> 8086 * Type: <b>token</b><br> 8087 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 8088 * </p> 8089 */ 8090 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 8091 public static final String SP_CONTEXT_TYPE = "context-type"; 8092 /** 8093 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 8094 * <p> 8095 * Description: <b>Multiple Resources: 8096 8097* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 8098* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 8099* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 8100* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 8101* [Citation](citation.html): A type of use context assigned to the citation 8102* [CodeSystem](codesystem.html): A type of use context assigned to the code system 8103* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 8104* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 8105* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 8106* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 8107* [Evidence](evidence.html): A type of use context assigned to the evidence 8108* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 8109* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 8110* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 8111* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 8112* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 8113* [Library](library.html): A type of use context assigned to the library 8114* [Measure](measure.html): A type of use context assigned to the measure 8115* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 8116* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 8117* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 8118* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 8119* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 8120* [Requirements](requirements.html): A type of use context assigned to the requirements 8121* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 8122* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 8123* [StructureMap](structuremap.html): A type of use context assigned to the structure map 8124* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 8125* [TestScript](testscript.html): A type of use context assigned to the test script 8126* [ValueSet](valueset.html): A type of use context assigned to the value set 8127</b><br> 8128 * Type: <b>token</b><br> 8129 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 8130 * </p> 8131 */ 8132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 8133 8134 /** 8135 * Search parameter: <b>context</b> 8136 * <p> 8137 * Description: <b>Multiple Resources: 8138 8139* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 8140* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 8141* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 8142* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 8143* [Citation](citation.html): A use context assigned to the citation 8144* [CodeSystem](codesystem.html): A use context assigned to the code system 8145* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 8146* [ConceptMap](conceptmap.html): A use context assigned to the concept map 8147* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 8148* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 8149* [Evidence](evidence.html): A use context assigned to the evidence 8150* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 8151* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 8152* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 8153* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 8154* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 8155* [Library](library.html): A use context assigned to the library 8156* [Measure](measure.html): A use context assigned to the measure 8157* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 8158* [NamingSystem](namingsystem.html): A use context assigned to the naming system 8159* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 8160* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 8161* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 8162* [Requirements](requirements.html): A use context assigned to the requirements 8163* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 8164* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 8165* [StructureMap](structuremap.html): A use context assigned to the structure map 8166* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 8167* [TestScript](testscript.html): A use context assigned to the test script 8168* [ValueSet](valueset.html): A use context assigned to the value set 8169</b><br> 8170 * Type: <b>token</b><br> 8171 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 8172 * </p> 8173 */ 8174 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 8175 public static final String SP_CONTEXT = "context"; 8176 /** 8177 * <b>Fluent Client</b> search parameter constant for <b>context</b> 8178 * <p> 8179 * Description: <b>Multiple Resources: 8180 8181* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 8182* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 8183* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 8184* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 8185* [Citation](citation.html): A use context assigned to the citation 8186* [CodeSystem](codesystem.html): A use context assigned to the code system 8187* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 8188* [ConceptMap](conceptmap.html): A use context assigned to the concept map 8189* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 8190* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 8191* [Evidence](evidence.html): A use context assigned to the evidence 8192* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 8193* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 8194* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 8195* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 8196* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 8197* [Library](library.html): A use context assigned to the library 8198* [Measure](measure.html): A use context assigned to the measure 8199* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 8200* [NamingSystem](namingsystem.html): A use context assigned to the naming system 8201* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 8202* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 8203* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 8204* [Requirements](requirements.html): A use context assigned to the requirements 8205* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 8206* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 8207* [StructureMap](structuremap.html): A use context assigned to the structure map 8208* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 8209* [TestScript](testscript.html): A use context assigned to the test script 8210* [ValueSet](valueset.html): A use context assigned to the value set 8211</b><br> 8212 * Type: <b>token</b><br> 8213 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 8214 * </p> 8215 */ 8216 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 8217 8218 /** 8219 * Search parameter: <b>date</b> 8220 * <p> 8221 * Description: <b>Multiple Resources: 8222 8223* [ActivityDefinition](activitydefinition.html): The activity definition publication date 8224* [ActorDefinition](actordefinition.html): The Actor Definition publication date 8225* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 8226* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 8227* [Citation](citation.html): The citation publication date 8228* [CodeSystem](codesystem.html): The code system publication date 8229* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 8230* [ConceptMap](conceptmap.html): The concept map publication date 8231* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 8232* [EventDefinition](eventdefinition.html): The event definition publication date 8233* [Evidence](evidence.html): The evidence publication date 8234* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 8235* [ExampleScenario](examplescenario.html): The example scenario publication date 8236* [GraphDefinition](graphdefinition.html): The graph definition publication date 8237* [ImplementationGuide](implementationguide.html): The implementation guide publication date 8238* [Library](library.html): The library publication date 8239* [Measure](measure.html): The measure publication date 8240* [MessageDefinition](messagedefinition.html): The message definition publication date 8241* [NamingSystem](namingsystem.html): The naming system publication date 8242* [OperationDefinition](operationdefinition.html): The operation definition publication date 8243* [PlanDefinition](plandefinition.html): The plan definition publication date 8244* [Questionnaire](questionnaire.html): The questionnaire publication date 8245* [Requirements](requirements.html): The requirements publication date 8246* [SearchParameter](searchparameter.html): The search parameter publication date 8247* [StructureDefinition](structuredefinition.html): The structure definition publication date 8248* [StructureMap](structuremap.html): The structure map publication date 8249* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 8250* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 8251* [TestScript](testscript.html): The test script publication date 8252* [ValueSet](valueset.html): The value set publication date 8253</b><br> 8254 * Type: <b>date</b><br> 8255 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 8256 * </p> 8257 */ 8258 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 8259 public static final String SP_DATE = "date"; 8260 /** 8261 * <b>Fluent Client</b> search parameter constant for <b>date</b> 8262 * <p> 8263 * Description: <b>Multiple Resources: 8264 8265* [ActivityDefinition](activitydefinition.html): The activity definition publication date 8266* [ActorDefinition](actordefinition.html): The Actor Definition publication date 8267* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 8268* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 8269* [Citation](citation.html): The citation publication date 8270* [CodeSystem](codesystem.html): The code system publication date 8271* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 8272* [ConceptMap](conceptmap.html): The concept map publication date 8273* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 8274* [EventDefinition](eventdefinition.html): The event definition publication date 8275* [Evidence](evidence.html): The evidence publication date 8276* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 8277* [ExampleScenario](examplescenario.html): The example scenario publication date 8278* [GraphDefinition](graphdefinition.html): The graph definition publication date 8279* [ImplementationGuide](implementationguide.html): The implementation guide publication date 8280* [Library](library.html): The library publication date 8281* [Measure](measure.html): The measure publication date 8282* [MessageDefinition](messagedefinition.html): The message definition publication date 8283* [NamingSystem](namingsystem.html): The naming system publication date 8284* [OperationDefinition](operationdefinition.html): The operation definition publication date 8285* [PlanDefinition](plandefinition.html): The plan definition publication date 8286* [Questionnaire](questionnaire.html): The questionnaire publication date 8287* [Requirements](requirements.html): The requirements publication date 8288* [SearchParameter](searchparameter.html): The search parameter publication date 8289* [StructureDefinition](structuredefinition.html): The structure definition publication date 8290* [StructureMap](structuremap.html): The structure map publication date 8291* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 8292* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 8293* [TestScript](testscript.html): The test script publication date 8294* [ValueSet](valueset.html): The value set publication date 8295</b><br> 8296 * Type: <b>date</b><br> 8297 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 8298 * </p> 8299 */ 8300 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 8301 8302 /** 8303 * Search parameter: <b>description</b> 8304 * <p> 8305 * Description: <b>Multiple Resources: 8306 8307* [ActivityDefinition](activitydefinition.html): The description of the activity definition 8308* [ActorDefinition](actordefinition.html): The description of the Actor Definition 8309* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 8310* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 8311* [Citation](citation.html): The description of the citation 8312* [CodeSystem](codesystem.html): The description of the code system 8313* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 8314* [ConceptMap](conceptmap.html): The description of the concept map 8315* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 8316* [EventDefinition](eventdefinition.html): The description of the event definition 8317* [Evidence](evidence.html): The description of the evidence 8318* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 8319* [GraphDefinition](graphdefinition.html): The description of the graph definition 8320* [ImplementationGuide](implementationguide.html): The description of the implementation guide 8321* [Library](library.html): The description of the library 8322* [Measure](measure.html): The description of the measure 8323* [MessageDefinition](messagedefinition.html): The description of the message definition 8324* [NamingSystem](namingsystem.html): The description of the naming system 8325* [OperationDefinition](operationdefinition.html): The description of the operation definition 8326* [PlanDefinition](plandefinition.html): The description of the plan definition 8327* [Questionnaire](questionnaire.html): The description of the questionnaire 8328* [Requirements](requirements.html): The description of the requirements 8329* [SearchParameter](searchparameter.html): The description of the search parameter 8330* [StructureDefinition](structuredefinition.html): The description of the structure definition 8331* [StructureMap](structuremap.html): The description of the structure map 8332* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 8333* [TestScript](testscript.html): The description of the test script 8334* [ValueSet](valueset.html): The description of the value set 8335</b><br> 8336 * Type: <b>string</b><br> 8337 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 8338 * </p> 8339 */ 8340 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 8341 public static final String SP_DESCRIPTION = "description"; 8342 /** 8343 * <b>Fluent Client</b> search parameter constant for <b>description</b> 8344 * <p> 8345 * Description: <b>Multiple Resources: 8346 8347* [ActivityDefinition](activitydefinition.html): The description of the activity definition 8348* [ActorDefinition](actordefinition.html): The description of the Actor Definition 8349* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 8350* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 8351* [Citation](citation.html): The description of the citation 8352* [CodeSystem](codesystem.html): The description of the code system 8353* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 8354* [ConceptMap](conceptmap.html): The description of the concept map 8355* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 8356* [EventDefinition](eventdefinition.html): The description of the event definition 8357* [Evidence](evidence.html): The description of the evidence 8358* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 8359* [GraphDefinition](graphdefinition.html): The description of the graph definition 8360* [ImplementationGuide](implementationguide.html): The description of the implementation guide 8361* [Library](library.html): The description of the library 8362* [Measure](measure.html): The description of the measure 8363* [MessageDefinition](messagedefinition.html): The description of the message definition 8364* [NamingSystem](namingsystem.html): The description of the naming system 8365* [OperationDefinition](operationdefinition.html): The description of the operation definition 8366* [PlanDefinition](plandefinition.html): The description of the plan definition 8367* [Questionnaire](questionnaire.html): The description of the questionnaire 8368* [Requirements](requirements.html): The description of the requirements 8369* [SearchParameter](searchparameter.html): The description of the search parameter 8370* [StructureDefinition](structuredefinition.html): The description of the structure definition 8371* [StructureMap](structuremap.html): The description of the structure map 8372* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 8373* [TestScript](testscript.html): The description of the test script 8374* [ValueSet](valueset.html): The description of the value set 8375</b><br> 8376 * Type: <b>string</b><br> 8377 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 8378 * </p> 8379 */ 8380 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 8381 8382 /** 8383 * Search parameter: <b>identifier</b> 8384 * <p> 8385 * Description: <b>Multiple Resources: 8386 8387* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 8388* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 8389* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 8390* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 8391* [Citation](citation.html): External identifier for the citation 8392* [CodeSystem](codesystem.html): External identifier for the code system 8393* [ConceptMap](conceptmap.html): External identifier for the concept map 8394* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 8395* [EventDefinition](eventdefinition.html): External identifier for the event definition 8396* [Evidence](evidence.html): External identifier for the evidence 8397* [EvidenceReport](evidencereport.html): External identifier for the evidence report 8398* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 8399* [ExampleScenario](examplescenario.html): External identifier for the example scenario 8400* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 8401* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 8402* [Library](library.html): External identifier for the library 8403* [Measure](measure.html): External identifier for the measure 8404* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 8405* [MessageDefinition](messagedefinition.html): External identifier for the message definition 8406* [NamingSystem](namingsystem.html): External identifier for the naming system 8407* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 8408* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 8409* [PlanDefinition](plandefinition.html): External identifier for the plan definition 8410* [Questionnaire](questionnaire.html): External identifier for the questionnaire 8411* [Requirements](requirements.html): External identifier for the requirements 8412* [SearchParameter](searchparameter.html): External identifier for the search parameter 8413* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 8414* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 8415* [StructureMap](structuremap.html): External identifier for the structure map 8416* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 8417* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 8418* [TestPlan](testplan.html): An identifier for the test plan 8419* [TestScript](testscript.html): External identifier for the test script 8420* [ValueSet](valueset.html): External identifier for the value set 8421</b><br> 8422 * Type: <b>token</b><br> 8423 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 8424 * </p> 8425 */ 8426 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 8427 public static final String SP_IDENTIFIER = "identifier"; 8428 /** 8429 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8430 * <p> 8431 * Description: <b>Multiple Resources: 8432 8433* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 8434* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 8435* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 8436* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 8437* [Citation](citation.html): External identifier for the citation 8438* [CodeSystem](codesystem.html): External identifier for the code system 8439* [ConceptMap](conceptmap.html): External identifier for the concept map 8440* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 8441* [EventDefinition](eventdefinition.html): External identifier for the event definition 8442* [Evidence](evidence.html): External identifier for the evidence 8443* [EvidenceReport](evidencereport.html): External identifier for the evidence report 8444* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 8445* [ExampleScenario](examplescenario.html): External identifier for the example scenario 8446* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 8447* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 8448* [Library](library.html): External identifier for the library 8449* [Measure](measure.html): External identifier for the measure 8450* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 8451* [MessageDefinition](messagedefinition.html): External identifier for the message definition 8452* [NamingSystem](namingsystem.html): External identifier for the naming system 8453* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 8454* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 8455* [PlanDefinition](plandefinition.html): External identifier for the plan definition 8456* [Questionnaire](questionnaire.html): External identifier for the questionnaire 8457* [Requirements](requirements.html): External identifier for the requirements 8458* [SearchParameter](searchparameter.html): External identifier for the search parameter 8459* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 8460* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 8461* [StructureMap](structuremap.html): External identifier for the structure map 8462* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 8463* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 8464* [TestPlan](testplan.html): An identifier for the test plan 8465* [TestScript](testscript.html): External identifier for the test script 8466* [ValueSet](valueset.html): External identifier for the value set 8467</b><br> 8468 * Type: <b>token</b><br> 8469 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 8470 * </p> 8471 */ 8472 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 8473 8474 /** 8475 * Search parameter: <b>jurisdiction</b> 8476 * <p> 8477 * Description: <b>Multiple Resources: 8478 8479* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 8480* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 8481* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 8482* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 8483* [Citation](citation.html): Intended jurisdiction for the citation 8484* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 8485* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 8486* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 8487* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 8488* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 8489* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 8490* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 8491* [Library](library.html): Intended jurisdiction for the library 8492* [Measure](measure.html): Intended jurisdiction for the measure 8493* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 8494* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 8495* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 8496* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 8497* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 8498* [Requirements](requirements.html): Intended jurisdiction for the requirements 8499* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 8500* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 8501* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 8502* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 8503* [TestScript](testscript.html): Intended jurisdiction for the test script 8504* [ValueSet](valueset.html): Intended jurisdiction for the value set 8505</b><br> 8506 * Type: <b>token</b><br> 8507 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 8508 * </p> 8509 */ 8510 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 8511 public static final String SP_JURISDICTION = "jurisdiction"; 8512 /** 8513 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 8514 * <p> 8515 * Description: <b>Multiple Resources: 8516 8517* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 8518* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 8519* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 8520* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 8521* [Citation](citation.html): Intended jurisdiction for the citation 8522* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 8523* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 8524* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 8525* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 8526* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 8527* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 8528* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 8529* [Library](library.html): Intended jurisdiction for the library 8530* [Measure](measure.html): Intended jurisdiction for the measure 8531* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 8532* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 8533* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 8534* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 8535* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 8536* [Requirements](requirements.html): Intended jurisdiction for the requirements 8537* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 8538* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 8539* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 8540* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 8541* [TestScript](testscript.html): Intended jurisdiction for the test script 8542* [ValueSet](valueset.html): Intended jurisdiction for the value set 8543</b><br> 8544 * Type: <b>token</b><br> 8545 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 8546 * </p> 8547 */ 8548 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 8549 8550 /** 8551 * Search parameter: <b>name</b> 8552 * <p> 8553 * Description: <b>Multiple Resources: 8554 8555* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 8556* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 8557* [Citation](citation.html): Computationally friendly name of the citation 8558* [CodeSystem](codesystem.html): Computationally friendly name of the code system 8559* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 8560* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 8561* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 8562* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 8563* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 8564* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 8565* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 8566* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 8567* [Library](library.html): Computationally friendly name of the library 8568* [Measure](measure.html): Computationally friendly name of the measure 8569* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 8570* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 8571* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 8572* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 8573* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 8574* [Requirements](requirements.html): Computationally friendly name of the requirements 8575* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 8576* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 8577* [StructureMap](structuremap.html): Computationally friendly name of the structure map 8578* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 8579* [TestScript](testscript.html): Computationally friendly name of the test script 8580* [ValueSet](valueset.html): Computationally friendly name of the value set 8581</b><br> 8582 * Type: <b>string</b><br> 8583 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 8584 * </p> 8585 */ 8586 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 8587 public static final String SP_NAME = "name"; 8588 /** 8589 * <b>Fluent Client</b> search parameter constant for <b>name</b> 8590 * <p> 8591 * Description: <b>Multiple Resources: 8592 8593* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 8594* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 8595* [Citation](citation.html): Computationally friendly name of the citation 8596* [CodeSystem](codesystem.html): Computationally friendly name of the code system 8597* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 8598* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 8599* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 8600* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 8601* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 8602* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 8603* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 8604* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 8605* [Library](library.html): Computationally friendly name of the library 8606* [Measure](measure.html): Computationally friendly name of the measure 8607* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 8608* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 8609* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 8610* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 8611* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 8612* [Requirements](requirements.html): Computationally friendly name of the requirements 8613* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 8614* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 8615* [StructureMap](structuremap.html): Computationally friendly name of the structure map 8616* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 8617* [TestScript](testscript.html): Computationally friendly name of the test script 8618* [ValueSet](valueset.html): Computationally friendly name of the value set 8619</b><br> 8620 * Type: <b>string</b><br> 8621 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 8622 * </p> 8623 */ 8624 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 8625 8626 /** 8627 * Search parameter: <b>publisher</b> 8628 * <p> 8629 * Description: <b>Multiple Resources: 8630 8631* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8632* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8633* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8634* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8635* [Citation](citation.html): Name of the publisher of the citation 8636* [CodeSystem](codesystem.html): Name of the publisher of the code system 8637* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8638* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8639* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8640* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8641* [Evidence](evidence.html): Name of the publisher of the evidence 8642* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8643* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8644* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8645* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8646* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8647* [Library](library.html): Name of the publisher of the library 8648* [Measure](measure.html): Name of the publisher of the measure 8649* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8650* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8651* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8652* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8653* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8654* [Requirements](requirements.html): Name of the publisher of the requirements 8655* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8656* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8657* [StructureMap](structuremap.html): Name of the publisher of the structure map 8658* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8659* [TestScript](testscript.html): Name of the publisher of the test script 8660* [ValueSet](valueset.html): Name of the publisher of the value set 8661</b><br> 8662 * Type: <b>string</b><br> 8663 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8664 * </p> 8665 */ 8666 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 8667 public static final String SP_PUBLISHER = "publisher"; 8668 /** 8669 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 8670 * <p> 8671 * Description: <b>Multiple Resources: 8672 8673* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8674* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8675* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8676* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8677* [Citation](citation.html): Name of the publisher of the citation 8678* [CodeSystem](codesystem.html): Name of the publisher of the code system 8679* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8680* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8681* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8682* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8683* [Evidence](evidence.html): Name of the publisher of the evidence 8684* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8685* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8686* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8687* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8688* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8689* [Library](library.html): Name of the publisher of the library 8690* [Measure](measure.html): Name of the publisher of the measure 8691* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8692* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8693* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8694* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8695* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8696* [Requirements](requirements.html): Name of the publisher of the requirements 8697* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8698* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8699* [StructureMap](structuremap.html): Name of the publisher of the structure map 8700* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8701* [TestScript](testscript.html): Name of the publisher of the test script 8702* [ValueSet](valueset.html): Name of the publisher of the value set 8703</b><br> 8704 * Type: <b>string</b><br> 8705 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8706 * </p> 8707 */ 8708 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 8709 8710 /** 8711 * Search parameter: <b>status</b> 8712 * <p> 8713 * Description: <b>Multiple Resources: 8714 8715* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8716* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8717* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8718* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8719* [Citation](citation.html): The current status of the citation 8720* [CodeSystem](codesystem.html): The current status of the code system 8721* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8722* [ConceptMap](conceptmap.html): The current status of the concept map 8723* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8724* [EventDefinition](eventdefinition.html): The current status of the event definition 8725* [Evidence](evidence.html): The current status of the evidence 8726* [EvidenceReport](evidencereport.html): The current status of the evidence report 8727* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8728* [ExampleScenario](examplescenario.html): The current status of the example scenario 8729* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8730* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8731* [Library](library.html): The current status of the library 8732* [Measure](measure.html): The current status of the measure 8733* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8734* [MessageDefinition](messagedefinition.html): The current status of the message definition 8735* [NamingSystem](namingsystem.html): The current status of the naming system 8736* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8737* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8738* [PlanDefinition](plandefinition.html): The current status of the plan definition 8739* [Questionnaire](questionnaire.html): The current status of the questionnaire 8740* [Requirements](requirements.html): The current status of the requirements 8741* [SearchParameter](searchparameter.html): The current status of the search parameter 8742* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8743* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8744* [StructureMap](structuremap.html): The current status of the structure map 8745* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8746* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8747* [TestPlan](testplan.html): The current status of the test plan 8748* [TestScript](testscript.html): The current status of the test script 8749* [ValueSet](valueset.html): The current status of the value set 8750</b><br> 8751 * Type: <b>token</b><br> 8752 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8753 * </p> 8754 */ 8755 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 8756 public static final String SP_STATUS = "status"; 8757 /** 8758 * <b>Fluent Client</b> search parameter constant for <b>status</b> 8759 * <p> 8760 * Description: <b>Multiple Resources: 8761 8762* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8763* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8764* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8765* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8766* [Citation](citation.html): The current status of the citation 8767* [CodeSystem](codesystem.html): The current status of the code system 8768* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8769* [ConceptMap](conceptmap.html): The current status of the concept map 8770* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8771* [EventDefinition](eventdefinition.html): The current status of the event definition 8772* [Evidence](evidence.html): The current status of the evidence 8773* [EvidenceReport](evidencereport.html): The current status of the evidence report 8774* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8775* [ExampleScenario](examplescenario.html): The current status of the example scenario 8776* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8777* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8778* [Library](library.html): The current status of the library 8779* [Measure](measure.html): The current status of the measure 8780* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8781* [MessageDefinition](messagedefinition.html): The current status of the message definition 8782* [NamingSystem](namingsystem.html): The current status of the naming system 8783* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8784* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8785* [PlanDefinition](plandefinition.html): The current status of the plan definition 8786* [Questionnaire](questionnaire.html): The current status of the questionnaire 8787* [Requirements](requirements.html): The current status of the requirements 8788* [SearchParameter](searchparameter.html): The current status of the search parameter 8789* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8790* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8791* [StructureMap](structuremap.html): The current status of the structure map 8792* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8793* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8794* [TestPlan](testplan.html): The current status of the test plan 8795* [TestScript](testscript.html): The current status of the test script 8796* [ValueSet](valueset.html): The current status of the value set 8797</b><br> 8798 * Type: <b>token</b><br> 8799 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8800 * </p> 8801 */ 8802 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 8803 8804 /** 8805 * Search parameter: <b>title</b> 8806 * <p> 8807 * Description: <b>Multiple Resources: 8808 8809* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8810* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8811* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8812* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8813* [Citation](citation.html): The human-friendly name of the citation 8814* [CodeSystem](codesystem.html): The human-friendly name of the code system 8815* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8816* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8817* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8818* [Evidence](evidence.html): The human-friendly name of the evidence 8819* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8820* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8821* [Library](library.html): The human-friendly name of the library 8822* [Measure](measure.html): The human-friendly name of the measure 8823* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8824* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8825* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8826* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8827* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8828* [Requirements](requirements.html): The human-friendly name of the requirements 8829* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8830* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8831* [StructureMap](structuremap.html): The human-friendly name of the structure map 8832* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8833* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8834* [TestScript](testscript.html): The human-friendly name of the test script 8835* [ValueSet](valueset.html): The human-friendly name of the value set 8836</b><br> 8837 * Type: <b>string</b><br> 8838 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8839 * </p> 8840 */ 8841 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 8842 public static final String SP_TITLE = "title"; 8843 /** 8844 * <b>Fluent Client</b> search parameter constant for <b>title</b> 8845 * <p> 8846 * Description: <b>Multiple Resources: 8847 8848* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8849* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8850* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8851* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8852* [Citation](citation.html): The human-friendly name of the citation 8853* [CodeSystem](codesystem.html): The human-friendly name of the code system 8854* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8855* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8856* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8857* [Evidence](evidence.html): The human-friendly name of the evidence 8858* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8859* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8860* [Library](library.html): The human-friendly name of the library 8861* [Measure](measure.html): The human-friendly name of the measure 8862* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8863* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8864* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8865* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8866* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8867* [Requirements](requirements.html): The human-friendly name of the requirements 8868* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8869* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8870* [StructureMap](structuremap.html): The human-friendly name of the structure map 8871* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8872* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8873* [TestScript](testscript.html): The human-friendly name of the test script 8874* [ValueSet](valueset.html): The human-friendly name of the value set 8875</b><br> 8876 * Type: <b>string</b><br> 8877 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8878 * </p> 8879 */ 8880 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 8881 8882 /** 8883 * Search parameter: <b>url</b> 8884 * <p> 8885 * Description: <b>Multiple Resources: 8886 8887* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8888* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8889* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8890* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8891* [Citation](citation.html): The uri that identifies the citation 8892* [CodeSystem](codesystem.html): The uri that identifies the code system 8893* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8894* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8895* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8896* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8897* [Evidence](evidence.html): The uri that identifies the evidence 8898* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8899* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8900* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8901* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8902* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8903* [Library](library.html): The uri that identifies the library 8904* [Measure](measure.html): The uri that identifies the measure 8905* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8906* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8907* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8908* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8909* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8910* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8911* [Requirements](requirements.html): The uri that identifies the requirements 8912* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8913* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8914* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8915* [StructureMap](structuremap.html): The uri that identifies the structure map 8916* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8917* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8918* [TestPlan](testplan.html): The uri that identifies the test plan 8919* [TestScript](testscript.html): The uri that identifies the test script 8920* [ValueSet](valueset.html): The uri that identifies the value set 8921</b><br> 8922 * Type: <b>uri</b><br> 8923 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8924 * </p> 8925 */ 8926 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 8927 public static final String SP_URL = "url"; 8928 /** 8929 * <b>Fluent Client</b> search parameter constant for <b>url</b> 8930 * <p> 8931 * Description: <b>Multiple Resources: 8932 8933* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8934* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8935* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8936* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8937* [Citation](citation.html): The uri that identifies the citation 8938* [CodeSystem](codesystem.html): The uri that identifies the code system 8939* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8940* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8941* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8942* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8943* [Evidence](evidence.html): The uri that identifies the evidence 8944* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8945* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8946* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8947* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8948* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8949* [Library](library.html): The uri that identifies the library 8950* [Measure](measure.html): The uri that identifies the measure 8951* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8952* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8953* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8954* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8955* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8956* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8957* [Requirements](requirements.html): The uri that identifies the requirements 8958* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8959* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8960* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8961* [StructureMap](structuremap.html): The uri that identifies the structure map 8962* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8963* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8964* [TestPlan](testplan.html): The uri that identifies the test plan 8965* [TestScript](testscript.html): The uri that identifies the test script 8966* [ValueSet](valueset.html): The uri that identifies the value set 8967</b><br> 8968 * Type: <b>uri</b><br> 8969 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8970 * </p> 8971 */ 8972 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 8973 8974 /** 8975 * Search parameter: <b>version</b> 8976 * <p> 8977 * Description: <b>Multiple Resources: 8978 8979* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8980* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8981* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8982* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8983* [Citation](citation.html): The business version of the citation 8984* [CodeSystem](codesystem.html): The business version of the code system 8985* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8986* [ConceptMap](conceptmap.html): The business version of the concept map 8987* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8988* [EventDefinition](eventdefinition.html): The business version of the event definition 8989* [Evidence](evidence.html): The business version of the evidence 8990* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8991* [ExampleScenario](examplescenario.html): The business version of the example scenario 8992* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8993* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8994* [Library](library.html): The business version of the library 8995* [Measure](measure.html): The business version of the measure 8996* [MessageDefinition](messagedefinition.html): The business version of the message definition 8997* [NamingSystem](namingsystem.html): The business version of the naming system 8998* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8999* [PlanDefinition](plandefinition.html): The business version of the plan definition 9000* [Questionnaire](questionnaire.html): The business version of the questionnaire 9001* [Requirements](requirements.html): The business version of the requirements 9002* [SearchParameter](searchparameter.html): The business version of the search parameter 9003* [StructureDefinition](structuredefinition.html): The business version of the structure definition 9004* [StructureMap](structuremap.html): The business version of the structure map 9005* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 9006* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 9007* [TestScript](testscript.html): The business version of the test script 9008* [ValueSet](valueset.html): The business version of the value set 9009</b><br> 9010 * Type: <b>token</b><br> 9011 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 9012 * </p> 9013 */ 9014 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 9015 public static final String SP_VERSION = "version"; 9016 /** 9017 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9018 * <p> 9019 * Description: <b>Multiple Resources: 9020 9021* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 9022* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 9023* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 9024* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 9025* [Citation](citation.html): The business version of the citation 9026* [CodeSystem](codesystem.html): The business version of the code system 9027* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 9028* [ConceptMap](conceptmap.html): The business version of the concept map 9029* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 9030* [EventDefinition](eventdefinition.html): The business version of the event definition 9031* [Evidence](evidence.html): The business version of the evidence 9032* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 9033* [ExampleScenario](examplescenario.html): The business version of the example scenario 9034* [GraphDefinition](graphdefinition.html): The business version of the graph definition 9035* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 9036* [Library](library.html): The business version of the library 9037* [Measure](measure.html): The business version of the measure 9038* [MessageDefinition](messagedefinition.html): The business version of the message definition 9039* [NamingSystem](namingsystem.html): The business version of the naming system 9040* [OperationDefinition](operationdefinition.html): The business version of the operation definition 9041* [PlanDefinition](plandefinition.html): The business version of the plan definition 9042* [Questionnaire](questionnaire.html): The business version of the questionnaire 9043* [Requirements](requirements.html): The business version of the requirements 9044* [SearchParameter](searchparameter.html): The business version of the search parameter 9045* [StructureDefinition](structuredefinition.html): The business version of the structure definition 9046* [StructureMap](structuremap.html): The business version of the structure map 9047* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 9048* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 9049* [TestScript](testscript.html): The business version of the test script 9050* [ValueSet](valueset.html): The business version of the value set 9051</b><br> 9052 * Type: <b>token</b><br> 9053 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 9054 * </p> 9055 */ 9056 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 9057 9058 /** 9059 * Search parameter: <b>derived-from</b> 9060 * <p> 9061 * Description: <b>Multiple Resources: 9062 9063* [ActivityDefinition](activitydefinition.html): What resource is being referenced 9064* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 9065* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 9066* [EventDefinition](eventdefinition.html): What resource is being referenced 9067* [EvidenceVariable](evidencevariable.html): What resource is being referenced 9068* [Library](library.html): What resource is being referenced 9069* [Measure](measure.html): What resource is being referenced 9070* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 9071* [PlanDefinition](plandefinition.html): What resource is being referenced 9072* [ValueSet](valueset.html): A resource that the ValueSet is derived from 9073</b><br> 9074 * Type: <b>reference</b><br> 9075 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 9076 * </p> 9077 */ 9078 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 9079 public static final String SP_DERIVED_FROM = "derived-from"; 9080 /** 9081 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 9082 * <p> 9083 * Description: <b>Multiple Resources: 9084 9085* [ActivityDefinition](activitydefinition.html): What resource is being referenced 9086* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 9087* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 9088* [EventDefinition](eventdefinition.html): What resource is being referenced 9089* [EvidenceVariable](evidencevariable.html): What resource is being referenced 9090* [Library](library.html): What resource is being referenced 9091* [Measure](measure.html): What resource is being referenced 9092* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 9093* [PlanDefinition](plandefinition.html): What resource is being referenced 9094* [ValueSet](valueset.html): A resource that the ValueSet is derived from 9095</b><br> 9096 * Type: <b>reference</b><br> 9097 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 9098 * </p> 9099 */ 9100 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 9101 9102/** 9103 * Constant for fluent queries to be used to add include statements. Specifies 9104 * the path value of "<b>ValueSet:derived-from</b>". 9105 */ 9106 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ValueSet:derived-from").toLocked(); 9107 9108 /** 9109 * Search parameter: <b>effective</b> 9110 * <p> 9111 * Description: <b>Multiple Resources: 9112 9113* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 9114* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 9115* [Citation](citation.html): The time during which the citation is intended to be in use 9116* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 9117* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 9118* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 9119* [Library](library.html): The time during which the library is intended to be in use 9120* [Measure](measure.html): The time during which the measure is intended to be in use 9121* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 9122* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 9123* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 9124* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 9125</b><br> 9126 * Type: <b>date</b><br> 9127 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 9128 * </p> 9129 */ 9130 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 9131 public static final String SP_EFFECTIVE = "effective"; 9132 /** 9133 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 9134 * <p> 9135 * Description: <b>Multiple Resources: 9136 9137* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 9138* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 9139* [Citation](citation.html): The time during which the citation is intended to be in use 9140* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 9141* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 9142* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 9143* [Library](library.html): The time during which the library is intended to be in use 9144* [Measure](measure.html): The time during which the measure is intended to be in use 9145* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 9146* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 9147* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 9148* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 9149</b><br> 9150 * Type: <b>date</b><br> 9151 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 9152 * </p> 9153 */ 9154 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 9155 9156 /** 9157 * Search parameter: <b>predecessor</b> 9158 * <p> 9159 * Description: <b>Multiple Resources: 9160 9161* [ActivityDefinition](activitydefinition.html): What resource is being referenced 9162* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 9163* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 9164* [EventDefinition](eventdefinition.html): What resource is being referenced 9165* [EvidenceVariable](evidencevariable.html): What resource is being referenced 9166* [Library](library.html): What resource is being referenced 9167* [Measure](measure.html): What resource is being referenced 9168* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 9169* [PlanDefinition](plandefinition.html): What resource is being referenced 9170* [ValueSet](valueset.html): The predecessor of the ValueSet 9171</b><br> 9172 * Type: <b>reference</b><br> 9173 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 9174 * </p> 9175 */ 9176 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 9177 public static final String SP_PREDECESSOR = "predecessor"; 9178 /** 9179 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 9180 * <p> 9181 * Description: <b>Multiple Resources: 9182 9183* [ActivityDefinition](activitydefinition.html): What resource is being referenced 9184* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 9185* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 9186* [EventDefinition](eventdefinition.html): What resource is being referenced 9187* [EvidenceVariable](evidencevariable.html): What resource is being referenced 9188* [Library](library.html): What resource is being referenced 9189* [Measure](measure.html): What resource is being referenced 9190* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 9191* [PlanDefinition](plandefinition.html): What resource is being referenced 9192* [ValueSet](valueset.html): The predecessor of the ValueSet 9193</b><br> 9194 * Type: <b>reference</b><br> 9195 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 9196 * </p> 9197 */ 9198 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 9199 9200/** 9201 * Constant for fluent queries to be used to add include statements. Specifies 9202 * the path value of "<b>ValueSet:predecessor</b>". 9203 */ 9204 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("ValueSet:predecessor").toLocked(); 9205 9206 /** 9207 * Search parameter: <b>topic</b> 9208 * <p> 9209 * Description: <b>Multiple Resources: 9210 9211* [ActivityDefinition](activitydefinition.html): Topics associated with the module 9212* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 9213* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 9214* [EventDefinition](eventdefinition.html): Topics associated with the module 9215* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 9216* [Library](library.html): Topics associated with the module 9217* [Measure](measure.html): Topics associated with the measure 9218* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 9219* [PlanDefinition](plandefinition.html): Topics associated with the module 9220* [ValueSet](valueset.html): Topics associated with the ValueSet 9221</b><br> 9222 * Type: <b>token</b><br> 9223 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 9224 * </p> 9225 */ 9226 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 9227 public static final String SP_TOPIC = "topic"; 9228 /** 9229 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 9230 * <p> 9231 * Description: <b>Multiple Resources: 9232 9233* [ActivityDefinition](activitydefinition.html): Topics associated with the module 9234* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 9235* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 9236* [EventDefinition](eventdefinition.html): Topics associated with the module 9237* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 9238* [Library](library.html): Topics associated with the module 9239* [Measure](measure.html): Topics associated with the measure 9240* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 9241* [PlanDefinition](plandefinition.html): Topics associated with the module 9242* [ValueSet](valueset.html): Topics associated with the ValueSet 9243</b><br> 9244 * Type: <b>token</b><br> 9245 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 9246 * </p> 9247 */ 9248 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 9249 9250 /** 9251 * Search parameter: <b>code</b> 9252 * <p> 9253 * Description: <b>This special parameter searches for codes in the value set. See additional notes on the ValueSet resource</b><br> 9254 * Type: <b>token</b><br> 9255 * Path: <b>ValueSet.expansion.contains.code | ValueSet.compose.include.concept.code</b><br> 9256 * </p> 9257 */ 9258 @SearchParamDefinition(name="code", path="ValueSet.expansion.contains.code | ValueSet.compose.include.concept.code", description="This special parameter searches for codes in the value set. See additional notes on the ValueSet resource", type="token" ) 9259 public static final String SP_CODE = "code"; 9260 /** 9261 * <b>Fluent Client</b> search parameter constant for <b>code</b> 9262 * <p> 9263 * Description: <b>This special parameter searches for codes in the value set. See additional notes on the ValueSet resource</b><br> 9264 * Type: <b>token</b><br> 9265 * Path: <b>ValueSet.expansion.contains.code | ValueSet.compose.include.concept.code</b><br> 9266 * </p> 9267 */ 9268 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 9269 9270 /** 9271 * Search parameter: <b>expansion</b> 9272 * <p> 9273 * Description: <b>Identifies the value set expansion (business identifier)</b><br> 9274 * Type: <b>uri</b><br> 9275 * Path: <b>ValueSet.expansion.identifier</b><br> 9276 * </p> 9277 */ 9278 @SearchParamDefinition(name="expansion", path="ValueSet.expansion.identifier", description="Identifies the value set expansion (business identifier)", type="uri" ) 9279 public static final String SP_EXPANSION = "expansion"; 9280 /** 9281 * <b>Fluent Client</b> search parameter constant for <b>expansion</b> 9282 * <p> 9283 * Description: <b>Identifies the value set expansion (business identifier)</b><br> 9284 * Type: <b>uri</b><br> 9285 * Path: <b>ValueSet.expansion.identifier</b><br> 9286 * </p> 9287 */ 9288 public static final ca.uhn.fhir.rest.gclient.UriClientParam EXPANSION = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_EXPANSION); 9289 9290 /** 9291 * Search parameter: <b>reference</b> 9292 * <p> 9293 * Description: <b>A code system included or excluded in the value set or an imported value set</b><br> 9294 * Type: <b>uri</b><br> 9295 * Path: <b>ValueSet.compose.include.system</b><br> 9296 * </p> 9297 */ 9298 @SearchParamDefinition(name="reference", path="ValueSet.compose.include.system", description="A code system included or excluded in the value set or an imported value set", type="uri" ) 9299 public static final String SP_REFERENCE = "reference"; 9300 /** 9301 * <b>Fluent Client</b> search parameter constant for <b>reference</b> 9302 * <p> 9303 * Description: <b>A code system included or excluded in the value set or an imported value set</b><br> 9304 * Type: <b>uri</b><br> 9305 * Path: <b>ValueSet.compose.include.system</b><br> 9306 * </p> 9307 */ 9308 public static final ca.uhn.fhir.rest.gclient.UriClientParam REFERENCE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_REFERENCE); 9309 9310 9311} 9312