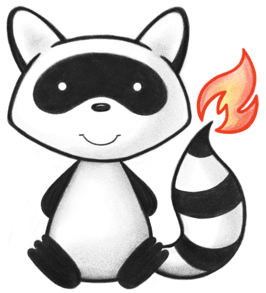
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes validation requirements, source(s), status and dates for one or more elements. 052 */ 053@ResourceDef(name="VerificationResult", profile="http://hl7.org/fhir/StructureDefinition/VerificationResult") 054public class VerificationResult extends DomainResource { 055 056 public enum VerificationResultStatus { 057 /** 058 * ***TODO*** 059 */ 060 ATTESTED, 061 /** 062 * ***TODO*** 063 */ 064 VALIDATED, 065 /** 066 * ***TODO*** 067 */ 068 INPROCESS, 069 /** 070 * ***TODO*** 071 */ 072 REQREVALID, 073 /** 074 * ***TODO*** 075 */ 076 VALFAIL, 077 /** 078 * ***TODO*** 079 */ 080 REVALFAIL, 081 /** 082 * The VerificationResult record was created erroneously and is not appropriated for use. 083 */ 084 ENTEREDINERROR, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static VerificationResultStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("attested".equals(codeString)) 093 return ATTESTED; 094 if ("validated".equals(codeString)) 095 return VALIDATED; 096 if ("in-process".equals(codeString)) 097 return INPROCESS; 098 if ("req-revalid".equals(codeString)) 099 return REQREVALID; 100 if ("val-fail".equals(codeString)) 101 return VALFAIL; 102 if ("reval-fail".equals(codeString)) 103 return REVALFAIL; 104 if ("entered-in-error".equals(codeString)) 105 return ENTEREDINERROR; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown VerificationResultStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case ATTESTED: return "attested"; 114 case VALIDATED: return "validated"; 115 case INPROCESS: return "in-process"; 116 case REQREVALID: return "req-revalid"; 117 case VALFAIL: return "val-fail"; 118 case REVALFAIL: return "reval-fail"; 119 case ENTEREDINERROR: return "entered-in-error"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case ATTESTED: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 127 case VALIDATED: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 128 case INPROCESS: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 129 case REQREVALID: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 130 case VALFAIL: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 131 case REVALFAIL: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 132 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/verificationresult-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case ATTESTED: return "***TODO***"; 140 case VALIDATED: return "***TODO***"; 141 case INPROCESS: return "***TODO***"; 142 case REQREVALID: return "***TODO***"; 143 case VALFAIL: return "***TODO***"; 144 case REVALFAIL: return "***TODO***"; 145 case ENTEREDINERROR: return "The VerificationResult record was created erroneously and is not appropriated for use."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case ATTESTED: return "Attested"; 153 case VALIDATED: return "Validated"; 154 case INPROCESS: return "In process"; 155 case REQREVALID: return "Requires revalidation"; 156 case VALFAIL: return "Validation failed"; 157 case REVALFAIL: return "Re-Validation failed"; 158 case ENTEREDINERROR: return "Entered in Error"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class VerificationResultStatusEnumFactory implements EnumFactory<VerificationResultStatus> { 166 public VerificationResultStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("attested".equals(codeString)) 171 return VerificationResultStatus.ATTESTED; 172 if ("validated".equals(codeString)) 173 return VerificationResultStatus.VALIDATED; 174 if ("in-process".equals(codeString)) 175 return VerificationResultStatus.INPROCESS; 176 if ("req-revalid".equals(codeString)) 177 return VerificationResultStatus.REQREVALID; 178 if ("val-fail".equals(codeString)) 179 return VerificationResultStatus.VALFAIL; 180 if ("reval-fail".equals(codeString)) 181 return VerificationResultStatus.REVALFAIL; 182 if ("entered-in-error".equals(codeString)) 183 return VerificationResultStatus.ENTEREDINERROR; 184 throw new IllegalArgumentException("Unknown VerificationResultStatus code '"+codeString+"'"); 185 } 186 public Enumeration<VerificationResultStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.NULL, code); 194 if ("attested".equals(codeString)) 195 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.ATTESTED, code); 196 if ("validated".equals(codeString)) 197 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.VALIDATED, code); 198 if ("in-process".equals(codeString)) 199 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.INPROCESS, code); 200 if ("req-revalid".equals(codeString)) 201 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.REQREVALID, code); 202 if ("val-fail".equals(codeString)) 203 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.VALFAIL, code); 204 if ("reval-fail".equals(codeString)) 205 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.REVALFAIL, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<VerificationResultStatus>(this, VerificationResultStatus.ENTEREDINERROR, code); 208 throw new FHIRException("Unknown VerificationResultStatus code '"+codeString+"'"); 209 } 210 public String toCode(VerificationResultStatus code) { 211 if (code == VerificationResultStatus.ATTESTED) 212 return "attested"; 213 if (code == VerificationResultStatus.VALIDATED) 214 return "validated"; 215 if (code == VerificationResultStatus.INPROCESS) 216 return "in-process"; 217 if (code == VerificationResultStatus.REQREVALID) 218 return "req-revalid"; 219 if (code == VerificationResultStatus.VALFAIL) 220 return "val-fail"; 221 if (code == VerificationResultStatus.REVALFAIL) 222 return "reval-fail"; 223 if (code == VerificationResultStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 return "?"; 226 } 227 public String toSystem(VerificationResultStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class VerificationResultPrimarySourceComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * Reference to the primary source. 236 */ 237 @Child(name = "who", type = {Organization.class, Practitioner.class, PractitionerRole.class}, order=1, min=0, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Reference to the primary source", formalDefinition="Reference to the primary source." ) 239 protected Reference who; 240 241 /** 242 * Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source). 243 */ 244 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 245 @Description(shortDefinition="Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source)", formalDefinition="Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source)." ) 246 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-primary-source-type") 247 protected List<CodeableConcept> type; 248 249 /** 250 * Method for communicating with the primary source (manual; API; Push). 251 */ 252 @Child(name = "communicationMethod", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 253 @Description(shortDefinition="Method for exchanging information with the primary source", formalDefinition="Method for communicating with the primary source (manual; API; Push)." ) 254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 255 protected List<CodeableConcept> communicationMethod; 256 257 /** 258 * Status of the validation of the target against the primary source (successful; failed; unknown). 259 */ 260 @Child(name = "validationStatus", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 261 @Description(shortDefinition="successful | failed | unknown", formalDefinition="Status of the validation of the target against the primary source (successful; failed; unknown)." ) 262 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-validation-status") 263 protected CodeableConcept validationStatus; 264 265 /** 266 * When the target was validated against the primary source. 267 */ 268 @Child(name = "validationDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 269 @Description(shortDefinition="When the target was validated against the primary source", formalDefinition="When the target was validated against the primary source." ) 270 protected DateTimeType validationDate; 271 272 /** 273 * Ability of the primary source to push updates/alerts (yes; no; undetermined). 274 */ 275 @Child(name = "canPushUpdates", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 276 @Description(shortDefinition="yes | no | undetermined", formalDefinition="Ability of the primary source to push updates/alerts (yes; no; undetermined)." ) 277 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-can-push-updates") 278 protected CodeableConcept canPushUpdates; 279 280 /** 281 * Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source). 282 */ 283 @Child(name = "pushTypeAvailable", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 284 @Description(shortDefinition="specific | any | source", formalDefinition="Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source)." ) 285 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-push-type-available") 286 protected List<CodeableConcept> pushTypeAvailable; 287 288 private static final long serialVersionUID = -266994197L; 289 290 /** 291 * Constructor 292 */ 293 public VerificationResultPrimarySourceComponent() { 294 super(); 295 } 296 297 /** 298 * @return {@link #who} (Reference to the primary source.) 299 */ 300 public Reference getWho() { 301 if (this.who == null) 302 if (Configuration.errorOnAutoCreate()) 303 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.who"); 304 else if (Configuration.doAutoCreate()) 305 this.who = new Reference(); // cc 306 return this.who; 307 } 308 309 public boolean hasWho() { 310 return this.who != null && !this.who.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #who} (Reference to the primary source.) 315 */ 316 public VerificationResultPrimarySourceComponent setWho(Reference value) { 317 this.who = value; 318 return this; 319 } 320 321 /** 322 * @return {@link #type} (Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).) 323 */ 324 public List<CodeableConcept> getType() { 325 if (this.type == null) 326 this.type = new ArrayList<CodeableConcept>(); 327 return this.type; 328 } 329 330 /** 331 * @return Returns a reference to <code>this</code> for easy method chaining 332 */ 333 public VerificationResultPrimarySourceComponent setType(List<CodeableConcept> theType) { 334 this.type = theType; 335 return this; 336 } 337 338 public boolean hasType() { 339 if (this.type == null) 340 return false; 341 for (CodeableConcept item : this.type) 342 if (!item.isEmpty()) 343 return true; 344 return false; 345 } 346 347 public CodeableConcept addType() { //3 348 CodeableConcept t = new CodeableConcept(); 349 if (this.type == null) 350 this.type = new ArrayList<CodeableConcept>(); 351 this.type.add(t); 352 return t; 353 } 354 355 public VerificationResultPrimarySourceComponent addType(CodeableConcept t) { //3 356 if (t == null) 357 return this; 358 if (this.type == null) 359 this.type = new ArrayList<CodeableConcept>(); 360 this.type.add(t); 361 return this; 362 } 363 364 /** 365 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 366 */ 367 public CodeableConcept getTypeFirstRep() { 368 if (getType().isEmpty()) { 369 addType(); 370 } 371 return getType().get(0); 372 } 373 374 /** 375 * @return {@link #communicationMethod} (Method for communicating with the primary source (manual; API; Push).) 376 */ 377 public List<CodeableConcept> getCommunicationMethod() { 378 if (this.communicationMethod == null) 379 this.communicationMethod = new ArrayList<CodeableConcept>(); 380 return this.communicationMethod; 381 } 382 383 /** 384 * @return Returns a reference to <code>this</code> for easy method chaining 385 */ 386 public VerificationResultPrimarySourceComponent setCommunicationMethod(List<CodeableConcept> theCommunicationMethod) { 387 this.communicationMethod = theCommunicationMethod; 388 return this; 389 } 390 391 public boolean hasCommunicationMethod() { 392 if (this.communicationMethod == null) 393 return false; 394 for (CodeableConcept item : this.communicationMethod) 395 if (!item.isEmpty()) 396 return true; 397 return false; 398 } 399 400 public CodeableConcept addCommunicationMethod() { //3 401 CodeableConcept t = new CodeableConcept(); 402 if (this.communicationMethod == null) 403 this.communicationMethod = new ArrayList<CodeableConcept>(); 404 this.communicationMethod.add(t); 405 return t; 406 } 407 408 public VerificationResultPrimarySourceComponent addCommunicationMethod(CodeableConcept t) { //3 409 if (t == null) 410 return this; 411 if (this.communicationMethod == null) 412 this.communicationMethod = new ArrayList<CodeableConcept>(); 413 this.communicationMethod.add(t); 414 return this; 415 } 416 417 /** 418 * @return The first repetition of repeating field {@link #communicationMethod}, creating it if it does not already exist {3} 419 */ 420 public CodeableConcept getCommunicationMethodFirstRep() { 421 if (getCommunicationMethod().isEmpty()) { 422 addCommunicationMethod(); 423 } 424 return getCommunicationMethod().get(0); 425 } 426 427 /** 428 * @return {@link #validationStatus} (Status of the validation of the target against the primary source (successful; failed; unknown).) 429 */ 430 public CodeableConcept getValidationStatus() { 431 if (this.validationStatus == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationStatus"); 434 else if (Configuration.doAutoCreate()) 435 this.validationStatus = new CodeableConcept(); // cc 436 return this.validationStatus; 437 } 438 439 public boolean hasValidationStatus() { 440 return this.validationStatus != null && !this.validationStatus.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #validationStatus} (Status of the validation of the target against the primary source (successful; failed; unknown).) 445 */ 446 public VerificationResultPrimarySourceComponent setValidationStatus(CodeableConcept value) { 447 this.validationStatus = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #validationDate} (When the target was validated against the primary source.). This is the underlying object with id, value and extensions. The accessor "getValidationDate" gives direct access to the value 453 */ 454 public DateTimeType getValidationDateElement() { 455 if (this.validationDate == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationDate"); 458 else if (Configuration.doAutoCreate()) 459 this.validationDate = new DateTimeType(); // bb 460 return this.validationDate; 461 } 462 463 public boolean hasValidationDateElement() { 464 return this.validationDate != null && !this.validationDate.isEmpty(); 465 } 466 467 public boolean hasValidationDate() { 468 return this.validationDate != null && !this.validationDate.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #validationDate} (When the target was validated against the primary source.). This is the underlying object with id, value and extensions. The accessor "getValidationDate" gives direct access to the value 473 */ 474 public VerificationResultPrimarySourceComponent setValidationDateElement(DateTimeType value) { 475 this.validationDate = value; 476 return this; 477 } 478 479 /** 480 * @return When the target was validated against the primary source. 481 */ 482 public Date getValidationDate() { 483 return this.validationDate == null ? null : this.validationDate.getValue(); 484 } 485 486 /** 487 * @param value When the target was validated against the primary source. 488 */ 489 public VerificationResultPrimarySourceComponent setValidationDate(Date value) { 490 if (value == null) 491 this.validationDate = null; 492 else { 493 if (this.validationDate == null) 494 this.validationDate = new DateTimeType(); 495 this.validationDate.setValue(value); 496 } 497 return this; 498 } 499 500 /** 501 * @return {@link #canPushUpdates} (Ability of the primary source to push updates/alerts (yes; no; undetermined).) 502 */ 503 public CodeableConcept getCanPushUpdates() { 504 if (this.canPushUpdates == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.canPushUpdates"); 507 else if (Configuration.doAutoCreate()) 508 this.canPushUpdates = new CodeableConcept(); // cc 509 return this.canPushUpdates; 510 } 511 512 public boolean hasCanPushUpdates() { 513 return this.canPushUpdates != null && !this.canPushUpdates.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #canPushUpdates} (Ability of the primary source to push updates/alerts (yes; no; undetermined).) 518 */ 519 public VerificationResultPrimarySourceComponent setCanPushUpdates(CodeableConcept value) { 520 this.canPushUpdates = value; 521 return this; 522 } 523 524 /** 525 * @return {@link #pushTypeAvailable} (Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).) 526 */ 527 public List<CodeableConcept> getPushTypeAvailable() { 528 if (this.pushTypeAvailable == null) 529 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 530 return this.pushTypeAvailable; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public VerificationResultPrimarySourceComponent setPushTypeAvailable(List<CodeableConcept> thePushTypeAvailable) { 537 this.pushTypeAvailable = thePushTypeAvailable; 538 return this; 539 } 540 541 public boolean hasPushTypeAvailable() { 542 if (this.pushTypeAvailable == null) 543 return false; 544 for (CodeableConcept item : this.pushTypeAvailable) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 public CodeableConcept addPushTypeAvailable() { //3 551 CodeableConcept t = new CodeableConcept(); 552 if (this.pushTypeAvailable == null) 553 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 554 this.pushTypeAvailable.add(t); 555 return t; 556 } 557 558 public VerificationResultPrimarySourceComponent addPushTypeAvailable(CodeableConcept t) { //3 559 if (t == null) 560 return this; 561 if (this.pushTypeAvailable == null) 562 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 563 this.pushTypeAvailable.add(t); 564 return this; 565 } 566 567 /** 568 * @return The first repetition of repeating field {@link #pushTypeAvailable}, creating it if it does not already exist {3} 569 */ 570 public CodeableConcept getPushTypeAvailableFirstRep() { 571 if (getPushTypeAvailable().isEmpty()) { 572 addPushTypeAvailable(); 573 } 574 return getPushTypeAvailable().get(0); 575 } 576 577 protected void listChildren(List<Property> children) { 578 super.listChildren(children); 579 children.add(new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", "Reference to the primary source.", 0, 1, who)); 580 children.add(new Property("type", "CodeableConcept", "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 0, java.lang.Integer.MAX_VALUE, type)); 581 children.add(new Property("communicationMethod", "CodeableConcept", "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, communicationMethod)); 582 children.add(new Property("validationStatus", "CodeableConcept", "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, validationStatus)); 583 children.add(new Property("validationDate", "dateTime", "When the target was validated against the primary source.", 0, 1, validationDate)); 584 children.add(new Property("canPushUpdates", "CodeableConcept", "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates)); 585 children.add(new Property("pushTypeAvailable", "CodeableConcept", "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable)); 586 } 587 588 @Override 589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 590 switch (_hash) { 591 case 117694: /*who*/ return new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", "Reference to the primary source.", 0, 1, who); 592 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 0, java.lang.Integer.MAX_VALUE, type); 593 case 1314116695: /*communicationMethod*/ return new Property("communicationMethod", "CodeableConcept", "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, communicationMethod); 594 case 1775633867: /*validationStatus*/ return new Property("validationStatus", "CodeableConcept", "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, validationStatus); 595 case -280180793: /*validationDate*/ return new Property("validationDate", "dateTime", "When the target was validated against the primary source.", 0, 1, validationDate); 596 case 1463787104: /*canPushUpdates*/ return new Property("canPushUpdates", "CodeableConcept", "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates); 597 case 945223605: /*pushTypeAvailable*/ return new Property("pushTypeAvailable", "CodeableConcept", "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable); 598 default: return super.getNamedProperty(_hash, _name, _checkValid); 599 } 600 601 } 602 603 @Override 604 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 605 switch (hash) { 606 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Reference 607 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 608 case 1314116695: /*communicationMethod*/ return this.communicationMethod == null ? new Base[0] : this.communicationMethod.toArray(new Base[this.communicationMethod.size()]); // CodeableConcept 609 case 1775633867: /*validationStatus*/ return this.validationStatus == null ? new Base[0] : new Base[] {this.validationStatus}; // CodeableConcept 610 case -280180793: /*validationDate*/ return this.validationDate == null ? new Base[0] : new Base[] {this.validationDate}; // DateTimeType 611 case 1463787104: /*canPushUpdates*/ return this.canPushUpdates == null ? new Base[0] : new Base[] {this.canPushUpdates}; // CodeableConcept 612 case 945223605: /*pushTypeAvailable*/ return this.pushTypeAvailable == null ? new Base[0] : this.pushTypeAvailable.toArray(new Base[this.pushTypeAvailable.size()]); // CodeableConcept 613 default: return super.getProperty(hash, name, checkValid); 614 } 615 616 } 617 618 @Override 619 public Base setProperty(int hash, String name, Base value) throws FHIRException { 620 switch (hash) { 621 case 117694: // who 622 this.who = TypeConvertor.castToReference(value); // Reference 623 return value; 624 case 3575610: // type 625 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 626 return value; 627 case 1314116695: // communicationMethod 628 this.getCommunicationMethod().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 629 return value; 630 case 1775633867: // validationStatus 631 this.validationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 632 return value; 633 case -280180793: // validationDate 634 this.validationDate = TypeConvertor.castToDateTime(value); // DateTimeType 635 return value; 636 case 1463787104: // canPushUpdates 637 this.canPushUpdates = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 638 return value; 639 case 945223605: // pushTypeAvailable 640 this.getPushTypeAvailable().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 641 return value; 642 default: return super.setProperty(hash, name, value); 643 } 644 645 } 646 647 @Override 648 public Base setProperty(String name, Base value) throws FHIRException { 649 if (name.equals("who")) { 650 this.who = TypeConvertor.castToReference(value); // Reference 651 } else if (name.equals("type")) { 652 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 653 } else if (name.equals("communicationMethod")) { 654 this.getCommunicationMethod().add(TypeConvertor.castToCodeableConcept(value)); 655 } else if (name.equals("validationStatus")) { 656 this.validationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 657 } else if (name.equals("validationDate")) { 658 this.validationDate = TypeConvertor.castToDateTime(value); // DateTimeType 659 } else if (name.equals("canPushUpdates")) { 660 this.canPushUpdates = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 661 } else if (name.equals("pushTypeAvailable")) { 662 this.getPushTypeAvailable().add(TypeConvertor.castToCodeableConcept(value)); 663 } else 664 return super.setProperty(name, value); 665 return value; 666 } 667 668 @Override 669 public void removeChild(String name, Base value) throws FHIRException { 670 if (name.equals("who")) { 671 this.who = null; 672 } else if (name.equals("type")) { 673 this.getType().remove(value); 674 } else if (name.equals("communicationMethod")) { 675 this.getCommunicationMethod().remove(value); 676 } else if (name.equals("validationStatus")) { 677 this.validationStatus = null; 678 } else if (name.equals("validationDate")) { 679 this.validationDate = null; 680 } else if (name.equals("canPushUpdates")) { 681 this.canPushUpdates = null; 682 } else if (name.equals("pushTypeAvailable")) { 683 this.getPushTypeAvailable().remove(value); 684 } else 685 super.removeChild(name, value); 686 687 } 688 689 @Override 690 public Base makeProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 117694: return getWho(); 693 case 3575610: return addType(); 694 case 1314116695: return addCommunicationMethod(); 695 case 1775633867: return getValidationStatus(); 696 case -280180793: return getValidationDateElement(); 697 case 1463787104: return getCanPushUpdates(); 698 case 945223605: return addPushTypeAvailable(); 699 default: return super.makeProperty(hash, name); 700 } 701 702 } 703 704 @Override 705 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 706 switch (hash) { 707 case 117694: /*who*/ return new String[] {"Reference"}; 708 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 709 case 1314116695: /*communicationMethod*/ return new String[] {"CodeableConcept"}; 710 case 1775633867: /*validationStatus*/ return new String[] {"CodeableConcept"}; 711 case -280180793: /*validationDate*/ return new String[] {"dateTime"}; 712 case 1463787104: /*canPushUpdates*/ return new String[] {"CodeableConcept"}; 713 case 945223605: /*pushTypeAvailable*/ return new String[] {"CodeableConcept"}; 714 default: return super.getTypesForProperty(hash, name); 715 } 716 717 } 718 719 @Override 720 public Base addChild(String name) throws FHIRException { 721 if (name.equals("who")) { 722 this.who = new Reference(); 723 return this.who; 724 } 725 else if (name.equals("type")) { 726 return addType(); 727 } 728 else if (name.equals("communicationMethod")) { 729 return addCommunicationMethod(); 730 } 731 else if (name.equals("validationStatus")) { 732 this.validationStatus = new CodeableConcept(); 733 return this.validationStatus; 734 } 735 else if (name.equals("validationDate")) { 736 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.primarySource.validationDate"); 737 } 738 else if (name.equals("canPushUpdates")) { 739 this.canPushUpdates = new CodeableConcept(); 740 return this.canPushUpdates; 741 } 742 else if (name.equals("pushTypeAvailable")) { 743 return addPushTypeAvailable(); 744 } 745 else 746 return super.addChild(name); 747 } 748 749 public VerificationResultPrimarySourceComponent copy() { 750 VerificationResultPrimarySourceComponent dst = new VerificationResultPrimarySourceComponent(); 751 copyValues(dst); 752 return dst; 753 } 754 755 public void copyValues(VerificationResultPrimarySourceComponent dst) { 756 super.copyValues(dst); 757 dst.who = who == null ? null : who.copy(); 758 if (type != null) { 759 dst.type = new ArrayList<CodeableConcept>(); 760 for (CodeableConcept i : type) 761 dst.type.add(i.copy()); 762 }; 763 if (communicationMethod != null) { 764 dst.communicationMethod = new ArrayList<CodeableConcept>(); 765 for (CodeableConcept i : communicationMethod) 766 dst.communicationMethod.add(i.copy()); 767 }; 768 dst.validationStatus = validationStatus == null ? null : validationStatus.copy(); 769 dst.validationDate = validationDate == null ? null : validationDate.copy(); 770 dst.canPushUpdates = canPushUpdates == null ? null : canPushUpdates.copy(); 771 if (pushTypeAvailable != null) { 772 dst.pushTypeAvailable = new ArrayList<CodeableConcept>(); 773 for (CodeableConcept i : pushTypeAvailable) 774 dst.pushTypeAvailable.add(i.copy()); 775 }; 776 } 777 778 @Override 779 public boolean equalsDeep(Base other_) { 780 if (!super.equalsDeep(other_)) 781 return false; 782 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 783 return false; 784 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 785 return compareDeep(who, o.who, true) && compareDeep(type, o.type, true) && compareDeep(communicationMethod, o.communicationMethod, true) 786 && compareDeep(validationStatus, o.validationStatus, true) && compareDeep(validationDate, o.validationDate, true) 787 && compareDeep(canPushUpdates, o.canPushUpdates, true) && compareDeep(pushTypeAvailable, o.pushTypeAvailable, true) 788 ; 789 } 790 791 @Override 792 public boolean equalsShallow(Base other_) { 793 if (!super.equalsShallow(other_)) 794 return false; 795 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 796 return false; 797 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 798 return compareValues(validationDate, o.validationDate, true); 799 } 800 801 public boolean isEmpty() { 802 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, type, communicationMethod 803 , validationStatus, validationDate, canPushUpdates, pushTypeAvailable); 804 } 805 806 public String fhirType() { 807 return "VerificationResult.primarySource"; 808 809 } 810 811 } 812 813 @Block() 814 public static class VerificationResultAttestationComponent extends BackboneElement implements IBaseBackboneElement { 815 /** 816 * The individual or organization attesting to information. 817 */ 818 @Child(name = "who", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=1, min=0, max=1, modifier=false, summary=true) 819 @Description(shortDefinition="The individual or organization attesting to information", formalDefinition="The individual or organization attesting to information." ) 820 protected Reference who; 821 822 /** 823 * When the who is asserting on behalf of another (organization or individual). 824 */ 825 @Child(name = "onBehalfOf", type = {Organization.class, Practitioner.class, PractitionerRole.class}, order=2, min=0, max=1, modifier=false, summary=true) 826 @Description(shortDefinition="When the who is asserting on behalf of another (organization or individual)", formalDefinition="When the who is asserting on behalf of another (organization or individual)." ) 827 protected Reference onBehalfOf; 828 829 /** 830 * The method by which attested information was submitted/retrieved (manual; API; Push). 831 */ 832 @Child(name = "communicationMethod", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 833 @Description(shortDefinition="The method by which attested information was submitted/retrieved", formalDefinition="The method by which attested information was submitted/retrieved (manual; API; Push)." ) 834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 835 protected CodeableConcept communicationMethod; 836 837 /** 838 * The date the information was attested to. 839 */ 840 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=true) 841 @Description(shortDefinition="The date the information was attested to", formalDefinition="The date the information was attested to." ) 842 protected DateType date; 843 844 /** 845 * A digital identity certificate associated with the attestation source. 846 */ 847 @Child(name = "sourceIdentityCertificate", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 848 @Description(shortDefinition="A digital identity certificate associated with the attestation source", formalDefinition="A digital identity certificate associated with the attestation source." ) 849 protected StringType sourceIdentityCertificate; 850 851 /** 852 * A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source. 853 */ 854 @Child(name = "proxyIdentityCertificate", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 855 @Description(shortDefinition="A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source", formalDefinition="A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source." ) 856 protected StringType proxyIdentityCertificate; 857 858 /** 859 * Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source. 860 */ 861 @Child(name = "proxySignature", type = {Signature.class}, order=7, min=0, max=1, modifier=false, summary=false) 862 @Description(shortDefinition="Proxy signature (digital or image)", formalDefinition="Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source." ) 863 protected Signature proxySignature; 864 865 /** 866 * Signed assertion by the attestation source that they have attested to the information. 867 */ 868 @Child(name = "sourceSignature", type = {Signature.class}, order=8, min=0, max=1, modifier=false, summary=false) 869 @Description(shortDefinition="Attester signature (digital or image)", formalDefinition="Signed assertion by the attestation source that they have attested to the information." ) 870 protected Signature sourceSignature; 871 872 private static final long serialVersionUID = -1110142980L; 873 874 /** 875 * Constructor 876 */ 877 public VerificationResultAttestationComponent() { 878 super(); 879 } 880 881 /** 882 * @return {@link #who} (The individual or organization attesting to information.) 883 */ 884 public Reference getWho() { 885 if (this.who == null) 886 if (Configuration.errorOnAutoCreate()) 887 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.who"); 888 else if (Configuration.doAutoCreate()) 889 this.who = new Reference(); // cc 890 return this.who; 891 } 892 893 public boolean hasWho() { 894 return this.who != null && !this.who.isEmpty(); 895 } 896 897 /** 898 * @param value {@link #who} (The individual or organization attesting to information.) 899 */ 900 public VerificationResultAttestationComponent setWho(Reference value) { 901 this.who = value; 902 return this; 903 } 904 905 /** 906 * @return {@link #onBehalfOf} (When the who is asserting on behalf of another (organization or individual).) 907 */ 908 public Reference getOnBehalfOf() { 909 if (this.onBehalfOf == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.onBehalfOf"); 912 else if (Configuration.doAutoCreate()) 913 this.onBehalfOf = new Reference(); // cc 914 return this.onBehalfOf; 915 } 916 917 public boolean hasOnBehalfOf() { 918 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #onBehalfOf} (When the who is asserting on behalf of another (organization or individual).) 923 */ 924 public VerificationResultAttestationComponent setOnBehalfOf(Reference value) { 925 this.onBehalfOf = value; 926 return this; 927 } 928 929 /** 930 * @return {@link #communicationMethod} (The method by which attested information was submitted/retrieved (manual; API; Push).) 931 */ 932 public CodeableConcept getCommunicationMethod() { 933 if (this.communicationMethod == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.communicationMethod"); 936 else if (Configuration.doAutoCreate()) 937 this.communicationMethod = new CodeableConcept(); // cc 938 return this.communicationMethod; 939 } 940 941 public boolean hasCommunicationMethod() { 942 return this.communicationMethod != null && !this.communicationMethod.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #communicationMethod} (The method by which attested information was submitted/retrieved (manual; API; Push).) 947 */ 948 public VerificationResultAttestationComponent setCommunicationMethod(CodeableConcept value) { 949 this.communicationMethod = value; 950 return this; 951 } 952 953 /** 954 * @return {@link #date} (The date the information was attested to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 955 */ 956 public DateType getDateElement() { 957 if (this.date == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.date"); 960 else if (Configuration.doAutoCreate()) 961 this.date = new DateType(); // bb 962 return this.date; 963 } 964 965 public boolean hasDateElement() { 966 return this.date != null && !this.date.isEmpty(); 967 } 968 969 public boolean hasDate() { 970 return this.date != null && !this.date.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #date} (The date the information was attested to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 975 */ 976 public VerificationResultAttestationComponent setDateElement(DateType value) { 977 this.date = value; 978 return this; 979 } 980 981 /** 982 * @return The date the information was attested to. 983 */ 984 public Date getDate() { 985 return this.date == null ? null : this.date.getValue(); 986 } 987 988 /** 989 * @param value The date the information was attested to. 990 */ 991 public VerificationResultAttestationComponent setDate(Date value) { 992 if (value == null) 993 this.date = null; 994 else { 995 if (this.date == null) 996 this.date = new DateType(); 997 this.date.setValue(value); 998 } 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #sourceIdentityCertificate} (A digital identity certificate associated with the attestation source.). This is the underlying object with id, value and extensions. The accessor "getSourceIdentityCertificate" gives direct access to the value 1004 */ 1005 public StringType getSourceIdentityCertificateElement() { 1006 if (this.sourceIdentityCertificate == null) 1007 if (Configuration.errorOnAutoCreate()) 1008 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceIdentityCertificate"); 1009 else if (Configuration.doAutoCreate()) 1010 this.sourceIdentityCertificate = new StringType(); // bb 1011 return this.sourceIdentityCertificate; 1012 } 1013 1014 public boolean hasSourceIdentityCertificateElement() { 1015 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1016 } 1017 1018 public boolean hasSourceIdentityCertificate() { 1019 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1020 } 1021 1022 /** 1023 * @param value {@link #sourceIdentityCertificate} (A digital identity certificate associated with the attestation source.). This is the underlying object with id, value and extensions. The accessor "getSourceIdentityCertificate" gives direct access to the value 1024 */ 1025 public VerificationResultAttestationComponent setSourceIdentityCertificateElement(StringType value) { 1026 this.sourceIdentityCertificate = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return A digital identity certificate associated with the attestation source. 1032 */ 1033 public String getSourceIdentityCertificate() { 1034 return this.sourceIdentityCertificate == null ? null : this.sourceIdentityCertificate.getValue(); 1035 } 1036 1037 /** 1038 * @param value A digital identity certificate associated with the attestation source. 1039 */ 1040 public VerificationResultAttestationComponent setSourceIdentityCertificate(String value) { 1041 if (Utilities.noString(value)) 1042 this.sourceIdentityCertificate = null; 1043 else { 1044 if (this.sourceIdentityCertificate == null) 1045 this.sourceIdentityCertificate = new StringType(); 1046 this.sourceIdentityCertificate.setValue(value); 1047 } 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #proxyIdentityCertificate} (A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.). This is the underlying object with id, value and extensions. The accessor "getProxyIdentityCertificate" gives direct access to the value 1053 */ 1054 public StringType getProxyIdentityCertificateElement() { 1055 if (this.proxyIdentityCertificate == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxyIdentityCertificate"); 1058 else if (Configuration.doAutoCreate()) 1059 this.proxyIdentityCertificate = new StringType(); // bb 1060 return this.proxyIdentityCertificate; 1061 } 1062 1063 public boolean hasProxyIdentityCertificateElement() { 1064 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1065 } 1066 1067 public boolean hasProxyIdentityCertificate() { 1068 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #proxyIdentityCertificate} (A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.). This is the underlying object with id, value and extensions. The accessor "getProxyIdentityCertificate" gives direct access to the value 1073 */ 1074 public VerificationResultAttestationComponent setProxyIdentityCertificateElement(StringType value) { 1075 this.proxyIdentityCertificate = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source. 1081 */ 1082 public String getProxyIdentityCertificate() { 1083 return this.proxyIdentityCertificate == null ? null : this.proxyIdentityCertificate.getValue(); 1084 } 1085 1086 /** 1087 * @param value A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source. 1088 */ 1089 public VerificationResultAttestationComponent setProxyIdentityCertificate(String value) { 1090 if (Utilities.noString(value)) 1091 this.proxyIdentityCertificate = null; 1092 else { 1093 if (this.proxyIdentityCertificate == null) 1094 this.proxyIdentityCertificate = new StringType(); 1095 this.proxyIdentityCertificate.setValue(value); 1096 } 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #proxySignature} (Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.) 1102 */ 1103 public Signature getProxySignature() { 1104 if (this.proxySignature == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxySignature"); 1107 else if (Configuration.doAutoCreate()) 1108 this.proxySignature = new Signature(); // cc 1109 return this.proxySignature; 1110 } 1111 1112 public boolean hasProxySignature() { 1113 return this.proxySignature != null && !this.proxySignature.isEmpty(); 1114 } 1115 1116 /** 1117 * @param value {@link #proxySignature} (Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.) 1118 */ 1119 public VerificationResultAttestationComponent setProxySignature(Signature value) { 1120 this.proxySignature = value; 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #sourceSignature} (Signed assertion by the attestation source that they have attested to the information.) 1126 */ 1127 public Signature getSourceSignature() { 1128 if (this.sourceSignature == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceSignature"); 1131 else if (Configuration.doAutoCreate()) 1132 this.sourceSignature = new Signature(); // cc 1133 return this.sourceSignature; 1134 } 1135 1136 public boolean hasSourceSignature() { 1137 return this.sourceSignature != null && !this.sourceSignature.isEmpty(); 1138 } 1139 1140 /** 1141 * @param value {@link #sourceSignature} (Signed assertion by the attestation source that they have attested to the information.) 1142 */ 1143 public VerificationResultAttestationComponent setSourceSignature(Signature value) { 1144 this.sourceSignature = value; 1145 return this; 1146 } 1147 1148 protected void listChildren(List<Property> children) { 1149 super.listChildren(children); 1150 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization attesting to information.", 0, 1, who)); 1151 children.add(new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf)); 1152 children.add(new Property("communicationMethod", "CodeableConcept", "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, communicationMethod)); 1153 children.add(new Property("date", "date", "The date the information was attested to.", 0, 1, date)); 1154 children.add(new Property("sourceIdentityCertificate", "string", "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate)); 1155 children.add(new Property("proxyIdentityCertificate", "string", "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 0, 1, proxyIdentityCertificate)); 1156 children.add(new Property("proxySignature", "Signature", "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 0, 1, proxySignature)); 1157 children.add(new Property("sourceSignature", "Signature", "Signed assertion by the attestation source that they have attested to the information.", 0, 1, sourceSignature)); 1158 } 1159 1160 @Override 1161 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1162 switch (_hash) { 1163 case 117694: /*who*/ return new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization attesting to information.", 0, 1, who); 1164 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf); 1165 case 1314116695: /*communicationMethod*/ return new Property("communicationMethod", "CodeableConcept", "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, communicationMethod); 1166 case 3076014: /*date*/ return new Property("date", "date", "The date the information was attested to.", 0, 1, date); 1167 case -799067682: /*sourceIdentityCertificate*/ return new Property("sourceIdentityCertificate", "string", "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate); 1168 case 431558827: /*proxyIdentityCertificate*/ return new Property("proxyIdentityCertificate", "string", "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 0, 1, proxyIdentityCertificate); 1169 case 1455540714: /*proxySignature*/ return new Property("proxySignature", "Signature", "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 0, 1, proxySignature); 1170 case 1754480349: /*sourceSignature*/ return new Property("sourceSignature", "Signature", "Signed assertion by the attestation source that they have attested to the information.", 0, 1, sourceSignature); 1171 default: return super.getNamedProperty(_hash, _name, _checkValid); 1172 } 1173 1174 } 1175 1176 @Override 1177 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1178 switch (hash) { 1179 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Reference 1180 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 1181 case 1314116695: /*communicationMethod*/ return this.communicationMethod == null ? new Base[0] : new Base[] {this.communicationMethod}; // CodeableConcept 1182 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 1183 case -799067682: /*sourceIdentityCertificate*/ return this.sourceIdentityCertificate == null ? new Base[0] : new Base[] {this.sourceIdentityCertificate}; // StringType 1184 case 431558827: /*proxyIdentityCertificate*/ return this.proxyIdentityCertificate == null ? new Base[0] : new Base[] {this.proxyIdentityCertificate}; // StringType 1185 case 1455540714: /*proxySignature*/ return this.proxySignature == null ? new Base[0] : new Base[] {this.proxySignature}; // Signature 1186 case 1754480349: /*sourceSignature*/ return this.sourceSignature == null ? new Base[0] : new Base[] {this.sourceSignature}; // Signature 1187 default: return super.getProperty(hash, name, checkValid); 1188 } 1189 1190 } 1191 1192 @Override 1193 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1194 switch (hash) { 1195 case 117694: // who 1196 this.who = TypeConvertor.castToReference(value); // Reference 1197 return value; 1198 case -14402964: // onBehalfOf 1199 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 1200 return value; 1201 case 1314116695: // communicationMethod 1202 this.communicationMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1203 return value; 1204 case 3076014: // date 1205 this.date = TypeConvertor.castToDate(value); // DateType 1206 return value; 1207 case -799067682: // sourceIdentityCertificate 1208 this.sourceIdentityCertificate = TypeConvertor.castToString(value); // StringType 1209 return value; 1210 case 431558827: // proxyIdentityCertificate 1211 this.proxyIdentityCertificate = TypeConvertor.castToString(value); // StringType 1212 return value; 1213 case 1455540714: // proxySignature 1214 this.proxySignature = TypeConvertor.castToSignature(value); // Signature 1215 return value; 1216 case 1754480349: // sourceSignature 1217 this.sourceSignature = TypeConvertor.castToSignature(value); // Signature 1218 return value; 1219 default: return super.setProperty(hash, name, value); 1220 } 1221 1222 } 1223 1224 @Override 1225 public Base setProperty(String name, Base value) throws FHIRException { 1226 if (name.equals("who")) { 1227 this.who = TypeConvertor.castToReference(value); // Reference 1228 } else if (name.equals("onBehalfOf")) { 1229 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 1230 } else if (name.equals("communicationMethod")) { 1231 this.communicationMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1232 } else if (name.equals("date")) { 1233 this.date = TypeConvertor.castToDate(value); // DateType 1234 } else if (name.equals("sourceIdentityCertificate")) { 1235 this.sourceIdentityCertificate = TypeConvertor.castToString(value); // StringType 1236 } else if (name.equals("proxyIdentityCertificate")) { 1237 this.proxyIdentityCertificate = TypeConvertor.castToString(value); // StringType 1238 } else if (name.equals("proxySignature")) { 1239 this.proxySignature = TypeConvertor.castToSignature(value); // Signature 1240 } else if (name.equals("sourceSignature")) { 1241 this.sourceSignature = TypeConvertor.castToSignature(value); // Signature 1242 } else 1243 return super.setProperty(name, value); 1244 return value; 1245 } 1246 1247 @Override 1248 public void removeChild(String name, Base value) throws FHIRException { 1249 if (name.equals("who")) { 1250 this.who = null; 1251 } else if (name.equals("onBehalfOf")) { 1252 this.onBehalfOf = null; 1253 } else if (name.equals("communicationMethod")) { 1254 this.communicationMethod = null; 1255 } else if (name.equals("date")) { 1256 this.date = null; 1257 } else if (name.equals("sourceIdentityCertificate")) { 1258 this.sourceIdentityCertificate = null; 1259 } else if (name.equals("proxyIdentityCertificate")) { 1260 this.proxyIdentityCertificate = null; 1261 } else if (name.equals("proxySignature")) { 1262 this.proxySignature = null; 1263 } else if (name.equals("sourceSignature")) { 1264 this.sourceSignature = null; 1265 } else 1266 super.removeChild(name, value); 1267 1268 } 1269 1270 @Override 1271 public Base makeProperty(int hash, String name) throws FHIRException { 1272 switch (hash) { 1273 case 117694: return getWho(); 1274 case -14402964: return getOnBehalfOf(); 1275 case 1314116695: return getCommunicationMethod(); 1276 case 3076014: return getDateElement(); 1277 case -799067682: return getSourceIdentityCertificateElement(); 1278 case 431558827: return getProxyIdentityCertificateElement(); 1279 case 1455540714: return getProxySignature(); 1280 case 1754480349: return getSourceSignature(); 1281 default: return super.makeProperty(hash, name); 1282 } 1283 1284 } 1285 1286 @Override 1287 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1288 switch (hash) { 1289 case 117694: /*who*/ return new String[] {"Reference"}; 1290 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 1291 case 1314116695: /*communicationMethod*/ return new String[] {"CodeableConcept"}; 1292 case 3076014: /*date*/ return new String[] {"date"}; 1293 case -799067682: /*sourceIdentityCertificate*/ return new String[] {"string"}; 1294 case 431558827: /*proxyIdentityCertificate*/ return new String[] {"string"}; 1295 case 1455540714: /*proxySignature*/ return new String[] {"Signature"}; 1296 case 1754480349: /*sourceSignature*/ return new String[] {"Signature"}; 1297 default: return super.getTypesForProperty(hash, name); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base addChild(String name) throws FHIRException { 1304 if (name.equals("who")) { 1305 this.who = new Reference(); 1306 return this.who; 1307 } 1308 else if (name.equals("onBehalfOf")) { 1309 this.onBehalfOf = new Reference(); 1310 return this.onBehalfOf; 1311 } 1312 else if (name.equals("communicationMethod")) { 1313 this.communicationMethod = new CodeableConcept(); 1314 return this.communicationMethod; 1315 } 1316 else if (name.equals("date")) { 1317 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.attestation.date"); 1318 } 1319 else if (name.equals("sourceIdentityCertificate")) { 1320 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.attestation.sourceIdentityCertificate"); 1321 } 1322 else if (name.equals("proxyIdentityCertificate")) { 1323 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.attestation.proxyIdentityCertificate"); 1324 } 1325 else if (name.equals("proxySignature")) { 1326 this.proxySignature = new Signature(); 1327 return this.proxySignature; 1328 } 1329 else if (name.equals("sourceSignature")) { 1330 this.sourceSignature = new Signature(); 1331 return this.sourceSignature; 1332 } 1333 else 1334 return super.addChild(name); 1335 } 1336 1337 public VerificationResultAttestationComponent copy() { 1338 VerificationResultAttestationComponent dst = new VerificationResultAttestationComponent(); 1339 copyValues(dst); 1340 return dst; 1341 } 1342 1343 public void copyValues(VerificationResultAttestationComponent dst) { 1344 super.copyValues(dst); 1345 dst.who = who == null ? null : who.copy(); 1346 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 1347 dst.communicationMethod = communicationMethod == null ? null : communicationMethod.copy(); 1348 dst.date = date == null ? null : date.copy(); 1349 dst.sourceIdentityCertificate = sourceIdentityCertificate == null ? null : sourceIdentityCertificate.copy(); 1350 dst.proxyIdentityCertificate = proxyIdentityCertificate == null ? null : proxyIdentityCertificate.copy(); 1351 dst.proxySignature = proxySignature == null ? null : proxySignature.copy(); 1352 dst.sourceSignature = sourceSignature == null ? null : sourceSignature.copy(); 1353 } 1354 1355 @Override 1356 public boolean equalsDeep(Base other_) { 1357 if (!super.equalsDeep(other_)) 1358 return false; 1359 if (!(other_ instanceof VerificationResultAttestationComponent)) 1360 return false; 1361 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1362 return compareDeep(who, o.who, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(communicationMethod, o.communicationMethod, true) 1363 && compareDeep(date, o.date, true) && compareDeep(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1364 && compareDeep(proxyIdentityCertificate, o.proxyIdentityCertificate, true) && compareDeep(proxySignature, o.proxySignature, true) 1365 && compareDeep(sourceSignature, o.sourceSignature, true); 1366 } 1367 1368 @Override 1369 public boolean equalsShallow(Base other_) { 1370 if (!super.equalsShallow(other_)) 1371 return false; 1372 if (!(other_ instanceof VerificationResultAttestationComponent)) 1373 return false; 1374 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1375 return compareValues(date, o.date, true) && compareValues(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1376 && compareValues(proxyIdentityCertificate, o.proxyIdentityCertificate, true); 1377 } 1378 1379 public boolean isEmpty() { 1380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, onBehalfOf, communicationMethod 1381 , date, sourceIdentityCertificate, proxyIdentityCertificate, proxySignature, sourceSignature 1382 ); 1383 } 1384 1385 public String fhirType() { 1386 return "VerificationResult.attestation"; 1387 1388 } 1389 1390 } 1391 1392 @Block() 1393 public static class VerificationResultValidatorComponent extends BackboneElement implements IBaseBackboneElement { 1394 /** 1395 * Reference to the organization validating information. 1396 */ 1397 @Child(name = "organization", type = {Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 1398 @Description(shortDefinition="Reference to the organization validating information", formalDefinition="Reference to the organization validating information." ) 1399 protected Reference organization; 1400 1401 /** 1402 * A digital identity certificate associated with the validator. 1403 */ 1404 @Child(name = "identityCertificate", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1405 @Description(shortDefinition="A digital identity certificate associated with the validator", formalDefinition="A digital identity certificate associated with the validator." ) 1406 protected StringType identityCertificate; 1407 1408 /** 1409 * Signed assertion by the validator that they have validated the information. 1410 */ 1411 @Child(name = "attestationSignature", type = {Signature.class}, order=3, min=0, max=1, modifier=false, summary=false) 1412 @Description(shortDefinition="Validator signature (digital or image)", formalDefinition="Signed assertion by the validator that they have validated the information." ) 1413 protected Signature attestationSignature; 1414 1415 private static final long serialVersionUID = 335952370L; 1416 1417 /** 1418 * Constructor 1419 */ 1420 public VerificationResultValidatorComponent() { 1421 super(); 1422 } 1423 1424 /** 1425 * Constructor 1426 */ 1427 public VerificationResultValidatorComponent(Reference organization) { 1428 super(); 1429 this.setOrganization(organization); 1430 } 1431 1432 /** 1433 * @return {@link #organization} (Reference to the organization validating information.) 1434 */ 1435 public Reference getOrganization() { 1436 if (this.organization == null) 1437 if (Configuration.errorOnAutoCreate()) 1438 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.organization"); 1439 else if (Configuration.doAutoCreate()) 1440 this.organization = new Reference(); // cc 1441 return this.organization; 1442 } 1443 1444 public boolean hasOrganization() { 1445 return this.organization != null && !this.organization.isEmpty(); 1446 } 1447 1448 /** 1449 * @param value {@link #organization} (Reference to the organization validating information.) 1450 */ 1451 public VerificationResultValidatorComponent setOrganization(Reference value) { 1452 this.organization = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #identityCertificate} (A digital identity certificate associated with the validator.). This is the underlying object with id, value and extensions. The accessor "getIdentityCertificate" gives direct access to the value 1458 */ 1459 public StringType getIdentityCertificateElement() { 1460 if (this.identityCertificate == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.identityCertificate"); 1463 else if (Configuration.doAutoCreate()) 1464 this.identityCertificate = new StringType(); // bb 1465 return this.identityCertificate; 1466 } 1467 1468 public boolean hasIdentityCertificateElement() { 1469 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1470 } 1471 1472 public boolean hasIdentityCertificate() { 1473 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1474 } 1475 1476 /** 1477 * @param value {@link #identityCertificate} (A digital identity certificate associated with the validator.). This is the underlying object with id, value and extensions. The accessor "getIdentityCertificate" gives direct access to the value 1478 */ 1479 public VerificationResultValidatorComponent setIdentityCertificateElement(StringType value) { 1480 this.identityCertificate = value; 1481 return this; 1482 } 1483 1484 /** 1485 * @return A digital identity certificate associated with the validator. 1486 */ 1487 public String getIdentityCertificate() { 1488 return this.identityCertificate == null ? null : this.identityCertificate.getValue(); 1489 } 1490 1491 /** 1492 * @param value A digital identity certificate associated with the validator. 1493 */ 1494 public VerificationResultValidatorComponent setIdentityCertificate(String value) { 1495 if (Utilities.noString(value)) 1496 this.identityCertificate = null; 1497 else { 1498 if (this.identityCertificate == null) 1499 this.identityCertificate = new StringType(); 1500 this.identityCertificate.setValue(value); 1501 } 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #attestationSignature} (Signed assertion by the validator that they have validated the information.) 1507 */ 1508 public Signature getAttestationSignature() { 1509 if (this.attestationSignature == null) 1510 if (Configuration.errorOnAutoCreate()) 1511 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.attestationSignature"); 1512 else if (Configuration.doAutoCreate()) 1513 this.attestationSignature = new Signature(); // cc 1514 return this.attestationSignature; 1515 } 1516 1517 public boolean hasAttestationSignature() { 1518 return this.attestationSignature != null && !this.attestationSignature.isEmpty(); 1519 } 1520 1521 /** 1522 * @param value {@link #attestationSignature} (Signed assertion by the validator that they have validated the information.) 1523 */ 1524 public VerificationResultValidatorComponent setAttestationSignature(Signature value) { 1525 this.attestationSignature = value; 1526 return this; 1527 } 1528 1529 protected void listChildren(List<Property> children) { 1530 super.listChildren(children); 1531 children.add(new Property("organization", "Reference(Organization)", "Reference to the organization validating information.", 0, 1, organization)); 1532 children.add(new Property("identityCertificate", "string", "A digital identity certificate associated with the validator.", 0, 1, identityCertificate)); 1533 children.add(new Property("attestationSignature", "Signature", "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature)); 1534 } 1535 1536 @Override 1537 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1538 switch (_hash) { 1539 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "Reference to the organization validating information.", 0, 1, organization); 1540 case -854379015: /*identityCertificate*/ return new Property("identityCertificate", "string", "A digital identity certificate associated with the validator.", 0, 1, identityCertificate); 1541 case -184196152: /*attestationSignature*/ return new Property("attestationSignature", "Signature", "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature); 1542 default: return super.getNamedProperty(_hash, _name, _checkValid); 1543 } 1544 1545 } 1546 1547 @Override 1548 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1549 switch (hash) { 1550 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1551 case -854379015: /*identityCertificate*/ return this.identityCertificate == null ? new Base[0] : new Base[] {this.identityCertificate}; // StringType 1552 case -184196152: /*attestationSignature*/ return this.attestationSignature == null ? new Base[0] : new Base[] {this.attestationSignature}; // Signature 1553 default: return super.getProperty(hash, name, checkValid); 1554 } 1555 1556 } 1557 1558 @Override 1559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1560 switch (hash) { 1561 case 1178922291: // organization 1562 this.organization = TypeConvertor.castToReference(value); // Reference 1563 return value; 1564 case -854379015: // identityCertificate 1565 this.identityCertificate = TypeConvertor.castToString(value); // StringType 1566 return value; 1567 case -184196152: // attestationSignature 1568 this.attestationSignature = TypeConvertor.castToSignature(value); // Signature 1569 return value; 1570 default: return super.setProperty(hash, name, value); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base setProperty(String name, Base value) throws FHIRException { 1577 if (name.equals("organization")) { 1578 this.organization = TypeConvertor.castToReference(value); // Reference 1579 } else if (name.equals("identityCertificate")) { 1580 this.identityCertificate = TypeConvertor.castToString(value); // StringType 1581 } else if (name.equals("attestationSignature")) { 1582 this.attestationSignature = TypeConvertor.castToSignature(value); // Signature 1583 } else 1584 return super.setProperty(name, value); 1585 return value; 1586 } 1587 1588 @Override 1589 public void removeChild(String name, Base value) throws FHIRException { 1590 if (name.equals("organization")) { 1591 this.organization = null; 1592 } else if (name.equals("identityCertificate")) { 1593 this.identityCertificate = null; 1594 } else if (name.equals("attestationSignature")) { 1595 this.attestationSignature = null; 1596 } else 1597 super.removeChild(name, value); 1598 1599 } 1600 1601 @Override 1602 public Base makeProperty(int hash, String name) throws FHIRException { 1603 switch (hash) { 1604 case 1178922291: return getOrganization(); 1605 case -854379015: return getIdentityCertificateElement(); 1606 case -184196152: return getAttestationSignature(); 1607 default: return super.makeProperty(hash, name); 1608 } 1609 1610 } 1611 1612 @Override 1613 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1614 switch (hash) { 1615 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1616 case -854379015: /*identityCertificate*/ return new String[] {"string"}; 1617 case -184196152: /*attestationSignature*/ return new String[] {"Signature"}; 1618 default: return super.getTypesForProperty(hash, name); 1619 } 1620 1621 } 1622 1623 @Override 1624 public Base addChild(String name) throws FHIRException { 1625 if (name.equals("organization")) { 1626 this.organization = new Reference(); 1627 return this.organization; 1628 } 1629 else if (name.equals("identityCertificate")) { 1630 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.validator.identityCertificate"); 1631 } 1632 else if (name.equals("attestationSignature")) { 1633 this.attestationSignature = new Signature(); 1634 return this.attestationSignature; 1635 } 1636 else 1637 return super.addChild(name); 1638 } 1639 1640 public VerificationResultValidatorComponent copy() { 1641 VerificationResultValidatorComponent dst = new VerificationResultValidatorComponent(); 1642 copyValues(dst); 1643 return dst; 1644 } 1645 1646 public void copyValues(VerificationResultValidatorComponent dst) { 1647 super.copyValues(dst); 1648 dst.organization = organization == null ? null : organization.copy(); 1649 dst.identityCertificate = identityCertificate == null ? null : identityCertificate.copy(); 1650 dst.attestationSignature = attestationSignature == null ? null : attestationSignature.copy(); 1651 } 1652 1653 @Override 1654 public boolean equalsDeep(Base other_) { 1655 if (!super.equalsDeep(other_)) 1656 return false; 1657 if (!(other_ instanceof VerificationResultValidatorComponent)) 1658 return false; 1659 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 1660 return compareDeep(organization, o.organization, true) && compareDeep(identityCertificate, o.identityCertificate, true) 1661 && compareDeep(attestationSignature, o.attestationSignature, true); 1662 } 1663 1664 @Override 1665 public boolean equalsShallow(Base other_) { 1666 if (!super.equalsShallow(other_)) 1667 return false; 1668 if (!(other_ instanceof VerificationResultValidatorComponent)) 1669 return false; 1670 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 1671 return compareValues(identityCertificate, o.identityCertificate, true); 1672 } 1673 1674 public boolean isEmpty() { 1675 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(organization, identityCertificate 1676 , attestationSignature); 1677 } 1678 1679 public String fhirType() { 1680 return "VerificationResult.validator"; 1681 1682 } 1683 1684 } 1685 1686 /** 1687 * A resource that was validated. 1688 */ 1689 @Child(name = "target", type = {Reference.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1690 @Description(shortDefinition="A resource that was validated", formalDefinition="A resource that was validated." ) 1691 protected List<Reference> target; 1692 1693 /** 1694 * The fhirpath location(s) within the resource that was validated. 1695 */ 1696 @Child(name = "targetLocation", type = {StringType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1697 @Description(shortDefinition="The fhirpath location(s) within the resource that was validated", formalDefinition="The fhirpath location(s) within the resource that was validated." ) 1698 protected List<StringType> targetLocation; 1699 1700 /** 1701 * The frequency with which the target must be validated (none; initial; periodic). 1702 */ 1703 @Child(name = "need", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1704 @Description(shortDefinition="none | initial | periodic", formalDefinition="The frequency with which the target must be validated (none; initial; periodic)." ) 1705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-need") 1706 protected CodeableConcept need; 1707 1708 /** 1709 * The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed). 1710 */ 1711 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1712 @Description(shortDefinition="attested | validated | in-process | req-revalid | val-fail | reval-fail | entered-in-error", formalDefinition="The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed)." ) 1713 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-status") 1714 protected Enumeration<VerificationResultStatus> status; 1715 1716 /** 1717 * When the validation status was updated. 1718 */ 1719 @Child(name = "statusDate", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1720 @Description(shortDefinition="When the validation status was updated", formalDefinition="When the validation status was updated." ) 1721 protected DateTimeType statusDate; 1722 1723 /** 1724 * What the target is validated against (nothing; primary source; multiple sources). 1725 */ 1726 @Child(name = "validationType", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 1727 @Description(shortDefinition="nothing | primary | multiple", formalDefinition="What the target is validated against (nothing; primary source; multiple sources)." ) 1728 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-validation-type") 1729 protected CodeableConcept validationType; 1730 1731 /** 1732 * The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context). 1733 */ 1734 @Child(name = "validationProcess", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1735 @Description(shortDefinition="The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context)", formalDefinition="The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context)." ) 1736 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-validation-process") 1737 protected List<CodeableConcept> validationProcess; 1738 1739 /** 1740 * Frequency of revalidation. 1741 */ 1742 @Child(name = "frequency", type = {Timing.class}, order=7, min=0, max=1, modifier=false, summary=false) 1743 @Description(shortDefinition="Frequency of revalidation", formalDefinition="Frequency of revalidation." ) 1744 protected Timing frequency; 1745 1746 /** 1747 * The date/time validation was last completed (including failed validations). 1748 */ 1749 @Child(name = "lastPerformed", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1750 @Description(shortDefinition="The date/time validation was last completed (including failed validations)", formalDefinition="The date/time validation was last completed (including failed validations)." ) 1751 protected DateTimeType lastPerformed; 1752 1753 /** 1754 * The date when target is next validated, if appropriate. 1755 */ 1756 @Child(name = "nextScheduled", type = {DateType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1757 @Description(shortDefinition="The date when target is next validated, if appropriate", formalDefinition="The date when target is next validated, if appropriate." ) 1758 protected DateType nextScheduled; 1759 1760 /** 1761 * The result if validation fails (fatal; warning; record only; none). 1762 */ 1763 @Child(name = "failureAction", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 1764 @Description(shortDefinition="fatal | warn | rec-only | none", formalDefinition="The result if validation fails (fatal; warning; record only; none)." ) 1765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/verificationresult-failure-action") 1766 protected CodeableConcept failureAction; 1767 1768 /** 1769 * Information about the primary source(s) involved in validation. 1770 */ 1771 @Child(name = "primarySource", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1772 @Description(shortDefinition="Information about the primary source(s) involved in validation", formalDefinition="Information about the primary source(s) involved in validation." ) 1773 protected List<VerificationResultPrimarySourceComponent> primarySource; 1774 1775 /** 1776 * Information about the entity attesting to information. 1777 */ 1778 @Child(name = "attestation", type = {}, order=12, min=0, max=1, modifier=false, summary=false) 1779 @Description(shortDefinition="Information about the entity attesting to information", formalDefinition="Information about the entity attesting to information." ) 1780 protected VerificationResultAttestationComponent attestation; 1781 1782 /** 1783 * Information about the entity validating information. 1784 */ 1785 @Child(name = "validator", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1786 @Description(shortDefinition="Information about the entity validating information", formalDefinition="Information about the entity validating information." ) 1787 protected List<VerificationResultValidatorComponent> validator; 1788 1789 private static final long serialVersionUID = -612905810L; 1790 1791 /** 1792 * Constructor 1793 */ 1794 public VerificationResult() { 1795 super(); 1796 } 1797 1798 /** 1799 * Constructor 1800 */ 1801 public VerificationResult(VerificationResultStatus status) { 1802 super(); 1803 this.setStatus(status); 1804 } 1805 1806 /** 1807 * @return {@link #target} (A resource that was validated.) 1808 */ 1809 public List<Reference> getTarget() { 1810 if (this.target == null) 1811 this.target = new ArrayList<Reference>(); 1812 return this.target; 1813 } 1814 1815 /** 1816 * @return Returns a reference to <code>this</code> for easy method chaining 1817 */ 1818 public VerificationResult setTarget(List<Reference> theTarget) { 1819 this.target = theTarget; 1820 return this; 1821 } 1822 1823 public boolean hasTarget() { 1824 if (this.target == null) 1825 return false; 1826 for (Reference item : this.target) 1827 if (!item.isEmpty()) 1828 return true; 1829 return false; 1830 } 1831 1832 public Reference addTarget() { //3 1833 Reference t = new Reference(); 1834 if (this.target == null) 1835 this.target = new ArrayList<Reference>(); 1836 this.target.add(t); 1837 return t; 1838 } 1839 1840 public VerificationResult addTarget(Reference t) { //3 1841 if (t == null) 1842 return this; 1843 if (this.target == null) 1844 this.target = new ArrayList<Reference>(); 1845 this.target.add(t); 1846 return this; 1847 } 1848 1849 /** 1850 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 1851 */ 1852 public Reference getTargetFirstRep() { 1853 if (getTarget().isEmpty()) { 1854 addTarget(); 1855 } 1856 return getTarget().get(0); 1857 } 1858 1859 /** 1860 * @return {@link #targetLocation} (The fhirpath location(s) within the resource that was validated.) 1861 */ 1862 public List<StringType> getTargetLocation() { 1863 if (this.targetLocation == null) 1864 this.targetLocation = new ArrayList<StringType>(); 1865 return this.targetLocation; 1866 } 1867 1868 /** 1869 * @return Returns a reference to <code>this</code> for easy method chaining 1870 */ 1871 public VerificationResult setTargetLocation(List<StringType> theTargetLocation) { 1872 this.targetLocation = theTargetLocation; 1873 return this; 1874 } 1875 1876 public boolean hasTargetLocation() { 1877 if (this.targetLocation == null) 1878 return false; 1879 for (StringType item : this.targetLocation) 1880 if (!item.isEmpty()) 1881 return true; 1882 return false; 1883 } 1884 1885 /** 1886 * @return {@link #targetLocation} (The fhirpath location(s) within the resource that was validated.) 1887 */ 1888 public StringType addTargetLocationElement() {//2 1889 StringType t = new StringType(); 1890 if (this.targetLocation == null) 1891 this.targetLocation = new ArrayList<StringType>(); 1892 this.targetLocation.add(t); 1893 return t; 1894 } 1895 1896 /** 1897 * @param value {@link #targetLocation} (The fhirpath location(s) within the resource that was validated.) 1898 */ 1899 public VerificationResult addTargetLocation(String value) { //1 1900 StringType t = new StringType(); 1901 t.setValue(value); 1902 if (this.targetLocation == null) 1903 this.targetLocation = new ArrayList<StringType>(); 1904 this.targetLocation.add(t); 1905 return this; 1906 } 1907 1908 /** 1909 * @param value {@link #targetLocation} (The fhirpath location(s) within the resource that was validated.) 1910 */ 1911 public boolean hasTargetLocation(String value) { 1912 if (this.targetLocation == null) 1913 return false; 1914 for (StringType v : this.targetLocation) 1915 if (v.getValue().equals(value)) // string 1916 return true; 1917 return false; 1918 } 1919 1920 /** 1921 * @return {@link #need} (The frequency with which the target must be validated (none; initial; periodic).) 1922 */ 1923 public CodeableConcept getNeed() { 1924 if (this.need == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create VerificationResult.need"); 1927 else if (Configuration.doAutoCreate()) 1928 this.need = new CodeableConcept(); // cc 1929 return this.need; 1930 } 1931 1932 public boolean hasNeed() { 1933 return this.need != null && !this.need.isEmpty(); 1934 } 1935 1936 /** 1937 * @param value {@link #need} (The frequency with which the target must be validated (none; initial; periodic).) 1938 */ 1939 public VerificationResult setNeed(CodeableConcept value) { 1940 this.need = value; 1941 return this; 1942 } 1943 1944 /** 1945 * @return {@link #status} (The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1946 */ 1947 public Enumeration<VerificationResultStatus> getStatusElement() { 1948 if (this.status == null) 1949 if (Configuration.errorOnAutoCreate()) 1950 throw new Error("Attempt to auto-create VerificationResult.status"); 1951 else if (Configuration.doAutoCreate()) 1952 this.status = new Enumeration<VerificationResultStatus>(new VerificationResultStatusEnumFactory()); // bb 1953 return this.status; 1954 } 1955 1956 public boolean hasStatusElement() { 1957 return this.status != null && !this.status.isEmpty(); 1958 } 1959 1960 public boolean hasStatus() { 1961 return this.status != null && !this.status.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #status} (The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1966 */ 1967 public VerificationResult setStatusElement(Enumeration<VerificationResultStatus> value) { 1968 this.status = value; 1969 return this; 1970 } 1971 1972 /** 1973 * @return The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed). 1974 */ 1975 public VerificationResultStatus getStatus() { 1976 return this.status == null ? null : this.status.getValue(); 1977 } 1978 1979 /** 1980 * @param value The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed). 1981 */ 1982 public VerificationResult setStatus(VerificationResultStatus value) { 1983 if (this.status == null) 1984 this.status = new Enumeration<VerificationResultStatus>(new VerificationResultStatusEnumFactory()); 1985 this.status.setValue(value); 1986 return this; 1987 } 1988 1989 /** 1990 * @return {@link #statusDate} (When the validation status was updated.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 1991 */ 1992 public DateTimeType getStatusDateElement() { 1993 if (this.statusDate == null) 1994 if (Configuration.errorOnAutoCreate()) 1995 throw new Error("Attempt to auto-create VerificationResult.statusDate"); 1996 else if (Configuration.doAutoCreate()) 1997 this.statusDate = new DateTimeType(); // bb 1998 return this.statusDate; 1999 } 2000 2001 public boolean hasStatusDateElement() { 2002 return this.statusDate != null && !this.statusDate.isEmpty(); 2003 } 2004 2005 public boolean hasStatusDate() { 2006 return this.statusDate != null && !this.statusDate.isEmpty(); 2007 } 2008 2009 /** 2010 * @param value {@link #statusDate} (When the validation status was updated.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2011 */ 2012 public VerificationResult setStatusDateElement(DateTimeType value) { 2013 this.statusDate = value; 2014 return this; 2015 } 2016 2017 /** 2018 * @return When the validation status was updated. 2019 */ 2020 public Date getStatusDate() { 2021 return this.statusDate == null ? null : this.statusDate.getValue(); 2022 } 2023 2024 /** 2025 * @param value When the validation status was updated. 2026 */ 2027 public VerificationResult setStatusDate(Date value) { 2028 if (value == null) 2029 this.statusDate = null; 2030 else { 2031 if (this.statusDate == null) 2032 this.statusDate = new DateTimeType(); 2033 this.statusDate.setValue(value); 2034 } 2035 return this; 2036 } 2037 2038 /** 2039 * @return {@link #validationType} (What the target is validated against (nothing; primary source; multiple sources).) 2040 */ 2041 public CodeableConcept getValidationType() { 2042 if (this.validationType == null) 2043 if (Configuration.errorOnAutoCreate()) 2044 throw new Error("Attempt to auto-create VerificationResult.validationType"); 2045 else if (Configuration.doAutoCreate()) 2046 this.validationType = new CodeableConcept(); // cc 2047 return this.validationType; 2048 } 2049 2050 public boolean hasValidationType() { 2051 return this.validationType != null && !this.validationType.isEmpty(); 2052 } 2053 2054 /** 2055 * @param value {@link #validationType} (What the target is validated against (nothing; primary source; multiple sources).) 2056 */ 2057 public VerificationResult setValidationType(CodeableConcept value) { 2058 this.validationType = value; 2059 return this; 2060 } 2061 2062 /** 2063 * @return {@link #validationProcess} (The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).) 2064 */ 2065 public List<CodeableConcept> getValidationProcess() { 2066 if (this.validationProcess == null) 2067 this.validationProcess = new ArrayList<CodeableConcept>(); 2068 return this.validationProcess; 2069 } 2070 2071 /** 2072 * @return Returns a reference to <code>this</code> for easy method chaining 2073 */ 2074 public VerificationResult setValidationProcess(List<CodeableConcept> theValidationProcess) { 2075 this.validationProcess = theValidationProcess; 2076 return this; 2077 } 2078 2079 public boolean hasValidationProcess() { 2080 if (this.validationProcess == null) 2081 return false; 2082 for (CodeableConcept item : this.validationProcess) 2083 if (!item.isEmpty()) 2084 return true; 2085 return false; 2086 } 2087 2088 public CodeableConcept addValidationProcess() { //3 2089 CodeableConcept t = new CodeableConcept(); 2090 if (this.validationProcess == null) 2091 this.validationProcess = new ArrayList<CodeableConcept>(); 2092 this.validationProcess.add(t); 2093 return t; 2094 } 2095 2096 public VerificationResult addValidationProcess(CodeableConcept t) { //3 2097 if (t == null) 2098 return this; 2099 if (this.validationProcess == null) 2100 this.validationProcess = new ArrayList<CodeableConcept>(); 2101 this.validationProcess.add(t); 2102 return this; 2103 } 2104 2105 /** 2106 * @return The first repetition of repeating field {@link #validationProcess}, creating it if it does not already exist {3} 2107 */ 2108 public CodeableConcept getValidationProcessFirstRep() { 2109 if (getValidationProcess().isEmpty()) { 2110 addValidationProcess(); 2111 } 2112 return getValidationProcess().get(0); 2113 } 2114 2115 /** 2116 * @return {@link #frequency} (Frequency of revalidation.) 2117 */ 2118 public Timing getFrequency() { 2119 if (this.frequency == null) 2120 if (Configuration.errorOnAutoCreate()) 2121 throw new Error("Attempt to auto-create VerificationResult.frequency"); 2122 else if (Configuration.doAutoCreate()) 2123 this.frequency = new Timing(); // cc 2124 return this.frequency; 2125 } 2126 2127 public boolean hasFrequency() { 2128 return this.frequency != null && !this.frequency.isEmpty(); 2129 } 2130 2131 /** 2132 * @param value {@link #frequency} (Frequency of revalidation.) 2133 */ 2134 public VerificationResult setFrequency(Timing value) { 2135 this.frequency = value; 2136 return this; 2137 } 2138 2139 /** 2140 * @return {@link #lastPerformed} (The date/time validation was last completed (including failed validations).). This is the underlying object with id, value and extensions. The accessor "getLastPerformed" gives direct access to the value 2141 */ 2142 public DateTimeType getLastPerformedElement() { 2143 if (this.lastPerformed == null) 2144 if (Configuration.errorOnAutoCreate()) 2145 throw new Error("Attempt to auto-create VerificationResult.lastPerformed"); 2146 else if (Configuration.doAutoCreate()) 2147 this.lastPerformed = new DateTimeType(); // bb 2148 return this.lastPerformed; 2149 } 2150 2151 public boolean hasLastPerformedElement() { 2152 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2153 } 2154 2155 public boolean hasLastPerformed() { 2156 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2157 } 2158 2159 /** 2160 * @param value {@link #lastPerformed} (The date/time validation was last completed (including failed validations).). This is the underlying object with id, value and extensions. The accessor "getLastPerformed" gives direct access to the value 2161 */ 2162 public VerificationResult setLastPerformedElement(DateTimeType value) { 2163 this.lastPerformed = value; 2164 return this; 2165 } 2166 2167 /** 2168 * @return The date/time validation was last completed (including failed validations). 2169 */ 2170 public Date getLastPerformed() { 2171 return this.lastPerformed == null ? null : this.lastPerformed.getValue(); 2172 } 2173 2174 /** 2175 * @param value The date/time validation was last completed (including failed validations). 2176 */ 2177 public VerificationResult setLastPerformed(Date value) { 2178 if (value == null) 2179 this.lastPerformed = null; 2180 else { 2181 if (this.lastPerformed == null) 2182 this.lastPerformed = new DateTimeType(); 2183 this.lastPerformed.setValue(value); 2184 } 2185 return this; 2186 } 2187 2188 /** 2189 * @return {@link #nextScheduled} (The date when target is next validated, if appropriate.). This is the underlying object with id, value and extensions. The accessor "getNextScheduled" gives direct access to the value 2190 */ 2191 public DateType getNextScheduledElement() { 2192 if (this.nextScheduled == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create VerificationResult.nextScheduled"); 2195 else if (Configuration.doAutoCreate()) 2196 this.nextScheduled = new DateType(); // bb 2197 return this.nextScheduled; 2198 } 2199 2200 public boolean hasNextScheduledElement() { 2201 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2202 } 2203 2204 public boolean hasNextScheduled() { 2205 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #nextScheduled} (The date when target is next validated, if appropriate.). This is the underlying object with id, value and extensions. The accessor "getNextScheduled" gives direct access to the value 2210 */ 2211 public VerificationResult setNextScheduledElement(DateType value) { 2212 this.nextScheduled = value; 2213 return this; 2214 } 2215 2216 /** 2217 * @return The date when target is next validated, if appropriate. 2218 */ 2219 public Date getNextScheduled() { 2220 return this.nextScheduled == null ? null : this.nextScheduled.getValue(); 2221 } 2222 2223 /** 2224 * @param value The date when target is next validated, if appropriate. 2225 */ 2226 public VerificationResult setNextScheduled(Date value) { 2227 if (value == null) 2228 this.nextScheduled = null; 2229 else { 2230 if (this.nextScheduled == null) 2231 this.nextScheduled = new DateType(); 2232 this.nextScheduled.setValue(value); 2233 } 2234 return this; 2235 } 2236 2237 /** 2238 * @return {@link #failureAction} (The result if validation fails (fatal; warning; record only; none).) 2239 */ 2240 public CodeableConcept getFailureAction() { 2241 if (this.failureAction == null) 2242 if (Configuration.errorOnAutoCreate()) 2243 throw new Error("Attempt to auto-create VerificationResult.failureAction"); 2244 else if (Configuration.doAutoCreate()) 2245 this.failureAction = new CodeableConcept(); // cc 2246 return this.failureAction; 2247 } 2248 2249 public boolean hasFailureAction() { 2250 return this.failureAction != null && !this.failureAction.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #failureAction} (The result if validation fails (fatal; warning; record only; none).) 2255 */ 2256 public VerificationResult setFailureAction(CodeableConcept value) { 2257 this.failureAction = value; 2258 return this; 2259 } 2260 2261 /** 2262 * @return {@link #primarySource} (Information about the primary source(s) involved in validation.) 2263 */ 2264 public List<VerificationResultPrimarySourceComponent> getPrimarySource() { 2265 if (this.primarySource == null) 2266 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2267 return this.primarySource; 2268 } 2269 2270 /** 2271 * @return Returns a reference to <code>this</code> for easy method chaining 2272 */ 2273 public VerificationResult setPrimarySource(List<VerificationResultPrimarySourceComponent> thePrimarySource) { 2274 this.primarySource = thePrimarySource; 2275 return this; 2276 } 2277 2278 public boolean hasPrimarySource() { 2279 if (this.primarySource == null) 2280 return false; 2281 for (VerificationResultPrimarySourceComponent item : this.primarySource) 2282 if (!item.isEmpty()) 2283 return true; 2284 return false; 2285 } 2286 2287 public VerificationResultPrimarySourceComponent addPrimarySource() { //3 2288 VerificationResultPrimarySourceComponent t = new VerificationResultPrimarySourceComponent(); 2289 if (this.primarySource == null) 2290 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2291 this.primarySource.add(t); 2292 return t; 2293 } 2294 2295 public VerificationResult addPrimarySource(VerificationResultPrimarySourceComponent t) { //3 2296 if (t == null) 2297 return this; 2298 if (this.primarySource == null) 2299 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2300 this.primarySource.add(t); 2301 return this; 2302 } 2303 2304 /** 2305 * @return The first repetition of repeating field {@link #primarySource}, creating it if it does not already exist {3} 2306 */ 2307 public VerificationResultPrimarySourceComponent getPrimarySourceFirstRep() { 2308 if (getPrimarySource().isEmpty()) { 2309 addPrimarySource(); 2310 } 2311 return getPrimarySource().get(0); 2312 } 2313 2314 /** 2315 * @return {@link #attestation} (Information about the entity attesting to information.) 2316 */ 2317 public VerificationResultAttestationComponent getAttestation() { 2318 if (this.attestation == null) 2319 if (Configuration.errorOnAutoCreate()) 2320 throw new Error("Attempt to auto-create VerificationResult.attestation"); 2321 else if (Configuration.doAutoCreate()) 2322 this.attestation = new VerificationResultAttestationComponent(); // cc 2323 return this.attestation; 2324 } 2325 2326 public boolean hasAttestation() { 2327 return this.attestation != null && !this.attestation.isEmpty(); 2328 } 2329 2330 /** 2331 * @param value {@link #attestation} (Information about the entity attesting to information.) 2332 */ 2333 public VerificationResult setAttestation(VerificationResultAttestationComponent value) { 2334 this.attestation = value; 2335 return this; 2336 } 2337 2338 /** 2339 * @return {@link #validator} (Information about the entity validating information.) 2340 */ 2341 public List<VerificationResultValidatorComponent> getValidator() { 2342 if (this.validator == null) 2343 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2344 return this.validator; 2345 } 2346 2347 /** 2348 * @return Returns a reference to <code>this</code> for easy method chaining 2349 */ 2350 public VerificationResult setValidator(List<VerificationResultValidatorComponent> theValidator) { 2351 this.validator = theValidator; 2352 return this; 2353 } 2354 2355 public boolean hasValidator() { 2356 if (this.validator == null) 2357 return false; 2358 for (VerificationResultValidatorComponent item : this.validator) 2359 if (!item.isEmpty()) 2360 return true; 2361 return false; 2362 } 2363 2364 public VerificationResultValidatorComponent addValidator() { //3 2365 VerificationResultValidatorComponent t = new VerificationResultValidatorComponent(); 2366 if (this.validator == null) 2367 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2368 this.validator.add(t); 2369 return t; 2370 } 2371 2372 public VerificationResult addValidator(VerificationResultValidatorComponent t) { //3 2373 if (t == null) 2374 return this; 2375 if (this.validator == null) 2376 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2377 this.validator.add(t); 2378 return this; 2379 } 2380 2381 /** 2382 * @return The first repetition of repeating field {@link #validator}, creating it if it does not already exist {3} 2383 */ 2384 public VerificationResultValidatorComponent getValidatorFirstRep() { 2385 if (getValidator().isEmpty()) { 2386 addValidator(); 2387 } 2388 return getValidator().get(0); 2389 } 2390 2391 protected void listChildren(List<Property> children) { 2392 super.listChildren(children); 2393 children.add(new Property("target", "Reference(Any)", "A resource that was validated.", 0, java.lang.Integer.MAX_VALUE, target)); 2394 children.add(new Property("targetLocation", "string", "The fhirpath location(s) within the resource that was validated.", 0, java.lang.Integer.MAX_VALUE, targetLocation)); 2395 children.add(new Property("need", "CodeableConcept", "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need)); 2396 children.add(new Property("status", "code", "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 0, 1, status)); 2397 children.add(new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, statusDate)); 2398 children.add(new Property("validationType", "CodeableConcept", "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType)); 2399 children.add(new Property("validationProcess", "CodeableConcept", "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 0, java.lang.Integer.MAX_VALUE, validationProcess)); 2400 children.add(new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency)); 2401 children.add(new Property("lastPerformed", "dateTime", "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed)); 2402 children.add(new Property("nextScheduled", "date", "The date when target is next validated, if appropriate.", 0, 1, nextScheduled)); 2403 children.add(new Property("failureAction", "CodeableConcept", "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction)); 2404 children.add(new Property("primarySource", "", "Information about the primary source(s) involved in validation.", 0, java.lang.Integer.MAX_VALUE, primarySource)); 2405 children.add(new Property("attestation", "", "Information about the entity attesting to information.", 0, 1, attestation)); 2406 children.add(new Property("validator", "", "Information about the entity validating information.", 0, java.lang.Integer.MAX_VALUE, validator)); 2407 } 2408 2409 @Override 2410 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2411 switch (_hash) { 2412 case -880905839: /*target*/ return new Property("target", "Reference(Any)", "A resource that was validated.", 0, java.lang.Integer.MAX_VALUE, target); 2413 case 308958310: /*targetLocation*/ return new Property("targetLocation", "string", "The fhirpath location(s) within the resource that was validated.", 0, java.lang.Integer.MAX_VALUE, targetLocation); 2414 case 3377302: /*need*/ return new Property("need", "CodeableConcept", "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need); 2415 case -892481550: /*status*/ return new Property("status", "code", "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 0, 1, status); 2416 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, statusDate); 2417 case -279681197: /*validationType*/ return new Property("validationType", "CodeableConcept", "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType); 2418 case 797680566: /*validationProcess*/ return new Property("validationProcess", "CodeableConcept", "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 0, java.lang.Integer.MAX_VALUE, validationProcess); 2419 case -70023844: /*frequency*/ return new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency); 2420 case -1313229366: /*lastPerformed*/ return new Property("lastPerformed", "dateTime", "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed); 2421 case 1874589434: /*nextScheduled*/ return new Property("nextScheduled", "date", "The date when target is next validated, if appropriate.", 0, 1, nextScheduled); 2422 case 1816382560: /*failureAction*/ return new Property("failureAction", "CodeableConcept", "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction); 2423 case -528721731: /*primarySource*/ return new Property("primarySource", "", "Information about the primary source(s) involved in validation.", 0, java.lang.Integer.MAX_VALUE, primarySource); 2424 case -709624112: /*attestation*/ return new Property("attestation", "", "Information about the entity attesting to information.", 0, 1, attestation); 2425 case -1109783726: /*validator*/ return new Property("validator", "", "Information about the entity validating information.", 0, java.lang.Integer.MAX_VALUE, validator); 2426 default: return super.getNamedProperty(_hash, _name, _checkValid); 2427 } 2428 2429 } 2430 2431 @Override 2432 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2433 switch (hash) { 2434 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 2435 case 308958310: /*targetLocation*/ return this.targetLocation == null ? new Base[0] : this.targetLocation.toArray(new Base[this.targetLocation.size()]); // StringType 2436 case 3377302: /*need*/ return this.need == null ? new Base[0] : new Base[] {this.need}; // CodeableConcept 2437 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<VerificationResultStatus> 2438 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 2439 case -279681197: /*validationType*/ return this.validationType == null ? new Base[0] : new Base[] {this.validationType}; // CodeableConcept 2440 case 797680566: /*validationProcess*/ return this.validationProcess == null ? new Base[0] : this.validationProcess.toArray(new Base[this.validationProcess.size()]); // CodeableConcept 2441 case -70023844: /*frequency*/ return this.frequency == null ? new Base[0] : new Base[] {this.frequency}; // Timing 2442 case -1313229366: /*lastPerformed*/ return this.lastPerformed == null ? new Base[0] : new Base[] {this.lastPerformed}; // DateTimeType 2443 case 1874589434: /*nextScheduled*/ return this.nextScheduled == null ? new Base[0] : new Base[] {this.nextScheduled}; // DateType 2444 case 1816382560: /*failureAction*/ return this.failureAction == null ? new Base[0] : new Base[] {this.failureAction}; // CodeableConcept 2445 case -528721731: /*primarySource*/ return this.primarySource == null ? new Base[0] : this.primarySource.toArray(new Base[this.primarySource.size()]); // VerificationResultPrimarySourceComponent 2446 case -709624112: /*attestation*/ return this.attestation == null ? new Base[0] : new Base[] {this.attestation}; // VerificationResultAttestationComponent 2447 case -1109783726: /*validator*/ return this.validator == null ? new Base[0] : this.validator.toArray(new Base[this.validator.size()]); // VerificationResultValidatorComponent 2448 default: return super.getProperty(hash, name, checkValid); 2449 } 2450 2451 } 2452 2453 @Override 2454 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2455 switch (hash) { 2456 case -880905839: // target 2457 this.getTarget().add(TypeConvertor.castToReference(value)); // Reference 2458 return value; 2459 case 308958310: // targetLocation 2460 this.getTargetLocation().add(TypeConvertor.castToString(value)); // StringType 2461 return value; 2462 case 3377302: // need 2463 this.need = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2464 return value; 2465 case -892481550: // status 2466 value = new VerificationResultStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2467 this.status = (Enumeration) value; // Enumeration<VerificationResultStatus> 2468 return value; 2469 case 247524032: // statusDate 2470 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2471 return value; 2472 case -279681197: // validationType 2473 this.validationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2474 return value; 2475 case 797680566: // validationProcess 2476 this.getValidationProcess().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2477 return value; 2478 case -70023844: // frequency 2479 this.frequency = TypeConvertor.castToTiming(value); // Timing 2480 return value; 2481 case -1313229366: // lastPerformed 2482 this.lastPerformed = TypeConvertor.castToDateTime(value); // DateTimeType 2483 return value; 2484 case 1874589434: // nextScheduled 2485 this.nextScheduled = TypeConvertor.castToDate(value); // DateType 2486 return value; 2487 case 1816382560: // failureAction 2488 this.failureAction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2489 return value; 2490 case -528721731: // primarySource 2491 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); // VerificationResultPrimarySourceComponent 2492 return value; 2493 case -709624112: // attestation 2494 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 2495 return value; 2496 case -1109783726: // validator 2497 this.getValidator().add((VerificationResultValidatorComponent) value); // VerificationResultValidatorComponent 2498 return value; 2499 default: return super.setProperty(hash, name, value); 2500 } 2501 2502 } 2503 2504 @Override 2505 public Base setProperty(String name, Base value) throws FHIRException { 2506 if (name.equals("target")) { 2507 this.getTarget().add(TypeConvertor.castToReference(value)); 2508 } else if (name.equals("targetLocation")) { 2509 this.getTargetLocation().add(TypeConvertor.castToString(value)); 2510 } else if (name.equals("need")) { 2511 this.need = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2512 } else if (name.equals("status")) { 2513 value = new VerificationResultStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2514 this.status = (Enumeration) value; // Enumeration<VerificationResultStatus> 2515 } else if (name.equals("statusDate")) { 2516 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2517 } else if (name.equals("validationType")) { 2518 this.validationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2519 } else if (name.equals("validationProcess")) { 2520 this.getValidationProcess().add(TypeConvertor.castToCodeableConcept(value)); 2521 } else if (name.equals("frequency")) { 2522 this.frequency = TypeConvertor.castToTiming(value); // Timing 2523 } else if (name.equals("lastPerformed")) { 2524 this.lastPerformed = TypeConvertor.castToDateTime(value); // DateTimeType 2525 } else if (name.equals("nextScheduled")) { 2526 this.nextScheduled = TypeConvertor.castToDate(value); // DateType 2527 } else if (name.equals("failureAction")) { 2528 this.failureAction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2529 } else if (name.equals("primarySource")) { 2530 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); 2531 } else if (name.equals("attestation")) { 2532 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 2533 } else if (name.equals("validator")) { 2534 this.getValidator().add((VerificationResultValidatorComponent) value); 2535 } else 2536 return super.setProperty(name, value); 2537 return value; 2538 } 2539 2540 @Override 2541 public void removeChild(String name, Base value) throws FHIRException { 2542 if (name.equals("target")) { 2543 this.getTarget().remove(value); 2544 } else if (name.equals("targetLocation")) { 2545 this.getTargetLocation().remove(value); 2546 } else if (name.equals("need")) { 2547 this.need = null; 2548 } else if (name.equals("status")) { 2549 value = new VerificationResultStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2550 this.status = (Enumeration) value; // Enumeration<VerificationResultStatus> 2551 } else if (name.equals("statusDate")) { 2552 this.statusDate = null; 2553 } else if (name.equals("validationType")) { 2554 this.validationType = null; 2555 } else if (name.equals("validationProcess")) { 2556 this.getValidationProcess().remove(value); 2557 } else if (name.equals("frequency")) { 2558 this.frequency = null; 2559 } else if (name.equals("lastPerformed")) { 2560 this.lastPerformed = null; 2561 } else if (name.equals("nextScheduled")) { 2562 this.nextScheduled = null; 2563 } else if (name.equals("failureAction")) { 2564 this.failureAction = null; 2565 } else if (name.equals("primarySource")) { 2566 this.getPrimarySource().remove((VerificationResultPrimarySourceComponent) value); 2567 } else if (name.equals("attestation")) { 2568 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 2569 } else if (name.equals("validator")) { 2570 this.getValidator().remove((VerificationResultValidatorComponent) value); 2571 } else 2572 super.removeChild(name, value); 2573 2574 } 2575 2576 @Override 2577 public Base makeProperty(int hash, String name) throws FHIRException { 2578 switch (hash) { 2579 case -880905839: return addTarget(); 2580 case 308958310: return addTargetLocationElement(); 2581 case 3377302: return getNeed(); 2582 case -892481550: return getStatusElement(); 2583 case 247524032: return getStatusDateElement(); 2584 case -279681197: return getValidationType(); 2585 case 797680566: return addValidationProcess(); 2586 case -70023844: return getFrequency(); 2587 case -1313229366: return getLastPerformedElement(); 2588 case 1874589434: return getNextScheduledElement(); 2589 case 1816382560: return getFailureAction(); 2590 case -528721731: return addPrimarySource(); 2591 case -709624112: return getAttestation(); 2592 case -1109783726: return addValidator(); 2593 default: return super.makeProperty(hash, name); 2594 } 2595 2596 } 2597 2598 @Override 2599 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2600 switch (hash) { 2601 case -880905839: /*target*/ return new String[] {"Reference"}; 2602 case 308958310: /*targetLocation*/ return new String[] {"string"}; 2603 case 3377302: /*need*/ return new String[] {"CodeableConcept"}; 2604 case -892481550: /*status*/ return new String[] {"code"}; 2605 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 2606 case -279681197: /*validationType*/ return new String[] {"CodeableConcept"}; 2607 case 797680566: /*validationProcess*/ return new String[] {"CodeableConcept"}; 2608 case -70023844: /*frequency*/ return new String[] {"Timing"}; 2609 case -1313229366: /*lastPerformed*/ return new String[] {"dateTime"}; 2610 case 1874589434: /*nextScheduled*/ return new String[] {"date"}; 2611 case 1816382560: /*failureAction*/ return new String[] {"CodeableConcept"}; 2612 case -528721731: /*primarySource*/ return new String[] {}; 2613 case -709624112: /*attestation*/ return new String[] {}; 2614 case -1109783726: /*validator*/ return new String[] {}; 2615 default: return super.getTypesForProperty(hash, name); 2616 } 2617 2618 } 2619 2620 @Override 2621 public Base addChild(String name) throws FHIRException { 2622 if (name.equals("target")) { 2623 return addTarget(); 2624 } 2625 else if (name.equals("targetLocation")) { 2626 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.targetLocation"); 2627 } 2628 else if (name.equals("need")) { 2629 this.need = new CodeableConcept(); 2630 return this.need; 2631 } 2632 else if (name.equals("status")) { 2633 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.status"); 2634 } 2635 else if (name.equals("statusDate")) { 2636 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.statusDate"); 2637 } 2638 else if (name.equals("validationType")) { 2639 this.validationType = new CodeableConcept(); 2640 return this.validationType; 2641 } 2642 else if (name.equals("validationProcess")) { 2643 return addValidationProcess(); 2644 } 2645 else if (name.equals("frequency")) { 2646 this.frequency = new Timing(); 2647 return this.frequency; 2648 } 2649 else if (name.equals("lastPerformed")) { 2650 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.lastPerformed"); 2651 } 2652 else if (name.equals("nextScheduled")) { 2653 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.nextScheduled"); 2654 } 2655 else if (name.equals("failureAction")) { 2656 this.failureAction = new CodeableConcept(); 2657 return this.failureAction; 2658 } 2659 else if (name.equals("primarySource")) { 2660 return addPrimarySource(); 2661 } 2662 else if (name.equals("attestation")) { 2663 this.attestation = new VerificationResultAttestationComponent(); 2664 return this.attestation; 2665 } 2666 else if (name.equals("validator")) { 2667 return addValidator(); 2668 } 2669 else 2670 return super.addChild(name); 2671 } 2672 2673 public String fhirType() { 2674 return "VerificationResult"; 2675 2676 } 2677 2678 public VerificationResult copy() { 2679 VerificationResult dst = new VerificationResult(); 2680 copyValues(dst); 2681 return dst; 2682 } 2683 2684 public void copyValues(VerificationResult dst) { 2685 super.copyValues(dst); 2686 if (target != null) { 2687 dst.target = new ArrayList<Reference>(); 2688 for (Reference i : target) 2689 dst.target.add(i.copy()); 2690 }; 2691 if (targetLocation != null) { 2692 dst.targetLocation = new ArrayList<StringType>(); 2693 for (StringType i : targetLocation) 2694 dst.targetLocation.add(i.copy()); 2695 }; 2696 dst.need = need == null ? null : need.copy(); 2697 dst.status = status == null ? null : status.copy(); 2698 dst.statusDate = statusDate == null ? null : statusDate.copy(); 2699 dst.validationType = validationType == null ? null : validationType.copy(); 2700 if (validationProcess != null) { 2701 dst.validationProcess = new ArrayList<CodeableConcept>(); 2702 for (CodeableConcept i : validationProcess) 2703 dst.validationProcess.add(i.copy()); 2704 }; 2705 dst.frequency = frequency == null ? null : frequency.copy(); 2706 dst.lastPerformed = lastPerformed == null ? null : lastPerformed.copy(); 2707 dst.nextScheduled = nextScheduled == null ? null : nextScheduled.copy(); 2708 dst.failureAction = failureAction == null ? null : failureAction.copy(); 2709 if (primarySource != null) { 2710 dst.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2711 for (VerificationResultPrimarySourceComponent i : primarySource) 2712 dst.primarySource.add(i.copy()); 2713 }; 2714 dst.attestation = attestation == null ? null : attestation.copy(); 2715 if (validator != null) { 2716 dst.validator = new ArrayList<VerificationResultValidatorComponent>(); 2717 for (VerificationResultValidatorComponent i : validator) 2718 dst.validator.add(i.copy()); 2719 }; 2720 } 2721 2722 protected VerificationResult typedCopy() { 2723 return copy(); 2724 } 2725 2726 @Override 2727 public boolean equalsDeep(Base other_) { 2728 if (!super.equalsDeep(other_)) 2729 return false; 2730 if (!(other_ instanceof VerificationResult)) 2731 return false; 2732 VerificationResult o = (VerificationResult) other_; 2733 return compareDeep(target, o.target, true) && compareDeep(targetLocation, o.targetLocation, true) 2734 && compareDeep(need, o.need, true) && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 2735 && compareDeep(validationType, o.validationType, true) && compareDeep(validationProcess, o.validationProcess, true) 2736 && compareDeep(frequency, o.frequency, true) && compareDeep(lastPerformed, o.lastPerformed, true) 2737 && compareDeep(nextScheduled, o.nextScheduled, true) && compareDeep(failureAction, o.failureAction, true) 2738 && compareDeep(primarySource, o.primarySource, true) && compareDeep(attestation, o.attestation, true) 2739 && compareDeep(validator, o.validator, true); 2740 } 2741 2742 @Override 2743 public boolean equalsShallow(Base other_) { 2744 if (!super.equalsShallow(other_)) 2745 return false; 2746 if (!(other_ instanceof VerificationResult)) 2747 return false; 2748 VerificationResult o = (VerificationResult) other_; 2749 return compareValues(targetLocation, o.targetLocation, true) && compareValues(status, o.status, true) 2750 && compareValues(statusDate, o.statusDate, true) && compareValues(lastPerformed, o.lastPerformed, true) 2751 && compareValues(nextScheduled, o.nextScheduled, true); 2752 } 2753 2754 public boolean isEmpty() { 2755 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, targetLocation, need 2756 , status, statusDate, validationType, validationProcess, frequency, lastPerformed 2757 , nextScheduled, failureAction, primarySource, attestation, validator); 2758 } 2759 2760 @Override 2761 public ResourceType getResourceType() { 2762 return ResourceType.VerificationResult; 2763 } 2764 2765 /** 2766 * Search parameter: <b>attestation-method</b> 2767 * <p> 2768 * Description: <b>Select validation information for data that was attested to using the specified method</b><br> 2769 * Type: <b>token</b><br> 2770 * Path: <b>VerificationResult.attestation.communicationMethod</b><br> 2771 * </p> 2772 */ 2773 @SearchParamDefinition(name="attestation-method", path="VerificationResult.attestation.communicationMethod", description="Select validation information for data that was attested to using the specified method", type="token" ) 2774 public static final String SP_ATTESTATION_METHOD = "attestation-method"; 2775 /** 2776 * <b>Fluent Client</b> search parameter constant for <b>attestation-method</b> 2777 * <p> 2778 * Description: <b>Select validation information for data that was attested to using the specified method</b><br> 2779 * Type: <b>token</b><br> 2780 * Path: <b>VerificationResult.attestation.communicationMethod</b><br> 2781 * </p> 2782 */ 2783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ATTESTATION_METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ATTESTATION_METHOD); 2784 2785 /** 2786 * Search parameter: <b>attestation-onbehalfof</b> 2787 * <p> 2788 * Description: <b>Select validation information for data that was attested to on behalf of the specified source</b><br> 2789 * Type: <b>reference</b><br> 2790 * Path: <b>VerificationResult.attestation.onBehalfOf</b><br> 2791 * </p> 2792 */ 2793 @SearchParamDefinition(name="attestation-onbehalfof", path="VerificationResult.attestation.onBehalfOf", description="Select validation information for data that was attested to on behalf of the specified source", type="reference", target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2794 public static final String SP_ATTESTATION_ONBEHALFOF = "attestation-onbehalfof"; 2795 /** 2796 * <b>Fluent Client</b> search parameter constant for <b>attestation-onbehalfof</b> 2797 * <p> 2798 * Description: <b>Select validation information for data that was attested to on behalf of the specified source</b><br> 2799 * Type: <b>reference</b><br> 2800 * Path: <b>VerificationResult.attestation.onBehalfOf</b><br> 2801 * </p> 2802 */ 2803 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTATION_ONBEHALFOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ATTESTATION_ONBEHALFOF); 2804 2805/** 2806 * Constant for fluent queries to be used to add include statements. Specifies 2807 * the path value of "<b>VerificationResult:attestation-onbehalfof</b>". 2808 */ 2809 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTATION_ONBEHALFOF = new ca.uhn.fhir.model.api.Include("VerificationResult:attestation-onbehalfof").toLocked(); 2810 2811 /** 2812 * Search parameter: <b>attestation-who</b> 2813 * <p> 2814 * Description: <b>Select validation information for data that was attested to by the specified source</b><br> 2815 * Type: <b>reference</b><br> 2816 * Path: <b>VerificationResult.attestation.who</b><br> 2817 * </p> 2818 */ 2819 @SearchParamDefinition(name="attestation-who", path="VerificationResult.attestation.who", description="Select validation information for data that was attested to by the specified source", type="reference", target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2820 public static final String SP_ATTESTATION_WHO = "attestation-who"; 2821 /** 2822 * <b>Fluent Client</b> search parameter constant for <b>attestation-who</b> 2823 * <p> 2824 * Description: <b>Select validation information for data that was attested to by the specified source</b><br> 2825 * Type: <b>reference</b><br> 2826 * Path: <b>VerificationResult.attestation.who</b><br> 2827 * </p> 2828 */ 2829 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTATION_WHO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ATTESTATION_WHO); 2830 2831/** 2832 * Constant for fluent queries to be used to add include statements. Specifies 2833 * the path value of "<b>VerificationResult:attestation-who</b>". 2834 */ 2835 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTATION_WHO = new ca.uhn.fhir.model.api.Include("VerificationResult:attestation-who").toLocked(); 2836 2837 /** 2838 * Search parameter: <b>primarysource-date</b> 2839 * <p> 2840 * Description: <b>Select validation information for data that was validated against a primary source on the specified date</b><br> 2841 * Type: <b>date</b><br> 2842 * Path: <b>VerificationResult.primarySource.validationDate</b><br> 2843 * </p> 2844 */ 2845 @SearchParamDefinition(name="primarysource-date", path="VerificationResult.primarySource.validationDate", description="Select validation information for data that was validated against a primary source on the specified date", type="date" ) 2846 public static final String SP_PRIMARYSOURCE_DATE = "primarysource-date"; 2847 /** 2848 * <b>Fluent Client</b> search parameter constant for <b>primarysource-date</b> 2849 * <p> 2850 * Description: <b>Select validation information for data that was validated against a primary source on the specified date</b><br> 2851 * Type: <b>date</b><br> 2852 * Path: <b>VerificationResult.primarySource.validationDate</b><br> 2853 * </p> 2854 */ 2855 public static final ca.uhn.fhir.rest.gclient.DateClientParam PRIMARYSOURCE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PRIMARYSOURCE_DATE); 2856 2857 /** 2858 * Search parameter: <b>primarysource-type</b> 2859 * <p> 2860 * Description: <b>Select validation information for data that was validated against the specified type of primary source organization</b><br> 2861 * Type: <b>token</b><br> 2862 * Path: <b>VerificationResult.primarySource.type</b><br> 2863 * </p> 2864 */ 2865 @SearchParamDefinition(name="primarysource-type", path="VerificationResult.primarySource.type", description="Select validation information for data that was validated against the specified type of primary source organization", type="token" ) 2866 public static final String SP_PRIMARYSOURCE_TYPE = "primarysource-type"; 2867 /** 2868 * <b>Fluent Client</b> search parameter constant for <b>primarysource-type</b> 2869 * <p> 2870 * Description: <b>Select validation information for data that was validated against the specified type of primary source organization</b><br> 2871 * Type: <b>token</b><br> 2872 * Path: <b>VerificationResult.primarySource.type</b><br> 2873 * </p> 2874 */ 2875 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIMARYSOURCE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIMARYSOURCE_TYPE); 2876 2877 /** 2878 * Search parameter: <b>primarysource-who</b> 2879 * <p> 2880 * Description: <b>Select validation information for data that was validated against the specified primary source</b><br> 2881 * Type: <b>reference</b><br> 2882 * Path: <b>VerificationResult.primarySource.who</b><br> 2883 * </p> 2884 */ 2885 @SearchParamDefinition(name="primarysource-who", path="VerificationResult.primarySource.who", description="Select validation information for data that was validated against the specified primary source", type="reference", target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2886 public static final String SP_PRIMARYSOURCE_WHO = "primarysource-who"; 2887 /** 2888 * <b>Fluent Client</b> search parameter constant for <b>primarysource-who</b> 2889 * <p> 2890 * Description: <b>Select validation information for data that was validated against the specified primary source</b><br> 2891 * Type: <b>reference</b><br> 2892 * Path: <b>VerificationResult.primarySource.who</b><br> 2893 * </p> 2894 */ 2895 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIMARYSOURCE_WHO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRIMARYSOURCE_WHO); 2896 2897/** 2898 * Constant for fluent queries to be used to add include statements. Specifies 2899 * the path value of "<b>VerificationResult:primarysource-who</b>". 2900 */ 2901 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIMARYSOURCE_WHO = new ca.uhn.fhir.model.api.Include("VerificationResult:primarysource-who").toLocked(); 2902 2903 /** 2904 * Search parameter: <b>status-date</b> 2905 * <p> 2906 * Description: <b>Select validation information with a validation status that occurred at the specified date/time</b><br> 2907 * Type: <b>date</b><br> 2908 * Path: <b>VerificationResult.statusDate</b><br> 2909 * </p> 2910 */ 2911 @SearchParamDefinition(name="status-date", path="VerificationResult.statusDate", description="Select validation information with a validation status that occurred at the specified date/time", type="date" ) 2912 public static final String SP_STATUS_DATE = "status-date"; 2913 /** 2914 * <b>Fluent Client</b> search parameter constant for <b>status-date</b> 2915 * <p> 2916 * Description: <b>Select validation information with a validation status that occurred at the specified date/time</b><br> 2917 * Type: <b>date</b><br> 2918 * Path: <b>VerificationResult.statusDate</b><br> 2919 * </p> 2920 */ 2921 public static final ca.uhn.fhir.rest.gclient.DateClientParam STATUS_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_STATUS_DATE); 2922 2923 /** 2924 * Search parameter: <b>status</b> 2925 * <p> 2926 * Description: <b>Select validation information with the specified status</b><br> 2927 * Type: <b>token</b><br> 2928 * Path: <b>VerificationResult.status</b><br> 2929 * </p> 2930 */ 2931 @SearchParamDefinition(name="status", path="VerificationResult.status", description="Select validation information with the specified status", type="token" ) 2932 public static final String SP_STATUS = "status"; 2933 /** 2934 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2935 * <p> 2936 * Description: <b>Select validation information with the specified status</b><br> 2937 * Type: <b>token</b><br> 2938 * Path: <b>VerificationResult.status</b><br> 2939 * </p> 2940 */ 2941 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2942 2943 /** 2944 * Search parameter: <b>target</b> 2945 * <p> 2946 * Description: <b>A resource that was validated</b><br> 2947 * Type: <b>reference</b><br> 2948 * Path: <b>VerificationResult.target</b><br> 2949 * </p> 2950 */ 2951 @SearchParamDefinition(name="target", path="VerificationResult.target", description="A resource that was validated", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2952 public static final String SP_TARGET = "target"; 2953 /** 2954 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2955 * <p> 2956 * Description: <b>A resource that was validated</b><br> 2957 * Type: <b>reference</b><br> 2958 * Path: <b>VerificationResult.target</b><br> 2959 * </p> 2960 */ 2961 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 2962 2963/** 2964 * Constant for fluent queries to be used to add include statements. Specifies 2965 * the path value of "<b>VerificationResult:target</b>". 2966 */ 2967 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("VerificationResult:target").toLocked(); 2968 2969 /** 2970 * Search parameter: <b>validator-organization</b> 2971 * <p> 2972 * Description: <b>Select validation information for data that was validated by the specified organization</b><br> 2973 * Type: <b>reference</b><br> 2974 * Path: <b>VerificationResult.validator.organization</b><br> 2975 * </p> 2976 */ 2977 @SearchParamDefinition(name="validator-organization", path="VerificationResult.validator.organization", description="Select validation information for data that was validated by the specified organization", type="reference", target={Organization.class } ) 2978 public static final String SP_VALIDATOR_ORGANIZATION = "validator-organization"; 2979 /** 2980 * <b>Fluent Client</b> search parameter constant for <b>validator-organization</b> 2981 * <p> 2982 * Description: <b>Select validation information for data that was validated by the specified organization</b><br> 2983 * Type: <b>reference</b><br> 2984 * Path: <b>VerificationResult.validator.organization</b><br> 2985 * </p> 2986 */ 2987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALIDATOR_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALIDATOR_ORGANIZATION); 2988 2989/** 2990 * Constant for fluent queries to be used to add include statements. Specifies 2991 * the path value of "<b>VerificationResult:validator-organization</b>". 2992 */ 2993 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALIDATOR_ORGANIZATION = new ca.uhn.fhir.model.api.Include("VerificationResult:validator-organization").toLocked(); 2994 2995 2996} 2997