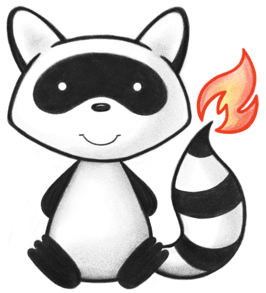
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * VirtualServiceDetail Type: Virtual Service Contact Details. 050 */ 051@DatatypeDef(name="VirtualServiceDetail") 052public class VirtualServiceDetail extends DataType implements ICompositeType { 053 054 /** 055 * The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp). 056 */ 057 @Child(name = "channelType", type = {Coding.class}, order=0, min=0, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Channel Type", formalDefinition="The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp)." ) 059 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/virtual-service-type") 060 protected Coding channelType; 061 062 /** 063 * What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type). 064 */ 065 @Child(name = "address", type = {UrlType.class, StringType.class, ContactPoint.class, ExtendedContactDetail.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Contact address/number", formalDefinition="What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type)." ) 067 protected DataType address; 068 069 /** 070 * Address to see alternative connection details. 071 */ 072 @Child(name = "additionalInfo", type = {UrlType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 073 @Description(shortDefinition="Address to see alternative connection details", formalDefinition="Address to see alternative connection details." ) 074 protected List<UrlType> additionalInfo; 075 076 /** 077 * Maximum number of participants supported by the virtual service. 078 */ 079 @Child(name = "maxParticipants", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Maximum number of participants supported by the virtual service", formalDefinition="Maximum number of participants supported by the virtual service." ) 081 protected PositiveIntType maxParticipants; 082 083 /** 084 * Session Key required by the virtual service. 085 */ 086 @Child(name = "sessionKey", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="Session Key required by the virtual service", formalDefinition="Session Key required by the virtual service." ) 088 protected StringType sessionKey; 089 090 private static final long serialVersionUID = -514931977L; 091 092 /** 093 * Constructor 094 */ 095 public VirtualServiceDetail() { 096 super(); 097 } 098 099 /** 100 * @return {@link #channelType} (The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp).) 101 */ 102 public Coding getChannelType() { 103 if (this.channelType == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create VirtualServiceDetail.channelType"); 106 else if (Configuration.doAutoCreate()) 107 this.channelType = new Coding(); // cc 108 return this.channelType; 109 } 110 111 public boolean hasChannelType() { 112 return this.channelType != null && !this.channelType.isEmpty(); 113 } 114 115 /** 116 * @param value {@link #channelType} (The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp).) 117 */ 118 public VirtualServiceDetail setChannelType(Coding value) { 119 this.channelType = value; 120 return this; 121 } 122 123 /** 124 * @return {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 125 */ 126 public DataType getAddress() { 127 return this.address; 128 } 129 130 /** 131 * @return {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 132 */ 133 public UrlType getAddressUrlType() throws FHIRException { 134 if (this.address == null) 135 this.address = new UrlType(); 136 if (!(this.address instanceof UrlType)) 137 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.address.getClass().getName()+" was encountered"); 138 return (UrlType) this.address; 139 } 140 141 public boolean hasAddressUrlType() { 142 return this != null && this.address instanceof UrlType; 143 } 144 145 /** 146 * @return {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 147 */ 148 public StringType getAddressStringType() throws FHIRException { 149 if (this.address == null) 150 this.address = new StringType(); 151 if (!(this.address instanceof StringType)) 152 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.address.getClass().getName()+" was encountered"); 153 return (StringType) this.address; 154 } 155 156 public boolean hasAddressStringType() { 157 return this != null && this.address instanceof StringType; 158 } 159 160 /** 161 * @return {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 162 */ 163 public ContactPoint getAddressContactPoint() throws FHIRException { 164 if (this.address == null) 165 this.address = new ContactPoint(); 166 if (!(this.address instanceof ContactPoint)) 167 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.address.getClass().getName()+" was encountered"); 168 return (ContactPoint) this.address; 169 } 170 171 public boolean hasAddressContactPoint() { 172 return this != null && this.address instanceof ContactPoint; 173 } 174 175 /** 176 * @return {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 177 */ 178 public ExtendedContactDetail getAddressExtendedContactDetail() throws FHIRException { 179 if (this.address == null) 180 this.address = new ExtendedContactDetail(); 181 if (!(this.address instanceof ExtendedContactDetail)) 182 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.address.getClass().getName()+" was encountered"); 183 return (ExtendedContactDetail) this.address; 184 } 185 186 public boolean hasAddressExtendedContactDetail() { 187 return this != null && this.address instanceof ExtendedContactDetail; 188 } 189 190 public boolean hasAddress() { 191 return this.address != null && !this.address.isEmpty(); 192 } 193 194 /** 195 * @param value {@link #address} (What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).) 196 */ 197 public VirtualServiceDetail setAddress(DataType value) { 198 if (value != null && !(value instanceof UrlType || value instanceof StringType || value instanceof ContactPoint || value instanceof ExtendedContactDetail)) 199 throw new FHIRException("Not the right type for VirtualServiceDetail.address[x]: "+value.fhirType()); 200 this.address = value; 201 return this; 202 } 203 204 /** 205 * @return {@link #additionalInfo} (Address to see alternative connection details.) 206 */ 207 public List<UrlType> getAdditionalInfo() { 208 if (this.additionalInfo == null) 209 this.additionalInfo = new ArrayList<UrlType>(); 210 return this.additionalInfo; 211 } 212 213 /** 214 * @return Returns a reference to <code>this</code> for easy method chaining 215 */ 216 public VirtualServiceDetail setAdditionalInfo(List<UrlType> theAdditionalInfo) { 217 this.additionalInfo = theAdditionalInfo; 218 return this; 219 } 220 221 public boolean hasAdditionalInfo() { 222 if (this.additionalInfo == null) 223 return false; 224 for (UrlType item : this.additionalInfo) 225 if (!item.isEmpty()) 226 return true; 227 return false; 228 } 229 230 /** 231 * @return {@link #additionalInfo} (Address to see alternative connection details.) 232 */ 233 public UrlType addAdditionalInfoElement() {//2 234 UrlType t = new UrlType(); 235 if (this.additionalInfo == null) 236 this.additionalInfo = new ArrayList<UrlType>(); 237 this.additionalInfo.add(t); 238 return t; 239 } 240 241 /** 242 * @param value {@link #additionalInfo} (Address to see alternative connection details.) 243 */ 244 public VirtualServiceDetail addAdditionalInfo(String value) { //1 245 UrlType t = new UrlType(); 246 t.setValue(value); 247 if (this.additionalInfo == null) 248 this.additionalInfo = new ArrayList<UrlType>(); 249 this.additionalInfo.add(t); 250 return this; 251 } 252 253 /** 254 * @param value {@link #additionalInfo} (Address to see alternative connection details.) 255 */ 256 public boolean hasAdditionalInfo(String value) { 257 if (this.additionalInfo == null) 258 return false; 259 for (UrlType v : this.additionalInfo) 260 if (v.getValue().equals(value)) // url 261 return true; 262 return false; 263 } 264 265 /** 266 * @return {@link #maxParticipants} (Maximum number of participants supported by the virtual service.). This is the underlying object with id, value and extensions. The accessor "getMaxParticipants" gives direct access to the value 267 */ 268 public PositiveIntType getMaxParticipantsElement() { 269 if (this.maxParticipants == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create VirtualServiceDetail.maxParticipants"); 272 else if (Configuration.doAutoCreate()) 273 this.maxParticipants = new PositiveIntType(); // bb 274 return this.maxParticipants; 275 } 276 277 public boolean hasMaxParticipantsElement() { 278 return this.maxParticipants != null && !this.maxParticipants.isEmpty(); 279 } 280 281 public boolean hasMaxParticipants() { 282 return this.maxParticipants != null && !this.maxParticipants.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #maxParticipants} (Maximum number of participants supported by the virtual service.). This is the underlying object with id, value and extensions. The accessor "getMaxParticipants" gives direct access to the value 287 */ 288 public VirtualServiceDetail setMaxParticipantsElement(PositiveIntType value) { 289 this.maxParticipants = value; 290 return this; 291 } 292 293 /** 294 * @return Maximum number of participants supported by the virtual service. 295 */ 296 public int getMaxParticipants() { 297 return this.maxParticipants == null || this.maxParticipants.isEmpty() ? 0 : this.maxParticipants.getValue(); 298 } 299 300 /** 301 * @param value Maximum number of participants supported by the virtual service. 302 */ 303 public VirtualServiceDetail setMaxParticipants(int value) { 304 if (this.maxParticipants == null) 305 this.maxParticipants = new PositiveIntType(); 306 this.maxParticipants.setValue(value); 307 return this; 308 } 309 310 /** 311 * @return {@link #sessionKey} (Session Key required by the virtual service.). This is the underlying object with id, value and extensions. The accessor "getSessionKey" gives direct access to the value 312 */ 313 public StringType getSessionKeyElement() { 314 if (this.sessionKey == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create VirtualServiceDetail.sessionKey"); 317 else if (Configuration.doAutoCreate()) 318 this.sessionKey = new StringType(); // bb 319 return this.sessionKey; 320 } 321 322 public boolean hasSessionKeyElement() { 323 return this.sessionKey != null && !this.sessionKey.isEmpty(); 324 } 325 326 public boolean hasSessionKey() { 327 return this.sessionKey != null && !this.sessionKey.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #sessionKey} (Session Key required by the virtual service.). This is the underlying object with id, value and extensions. The accessor "getSessionKey" gives direct access to the value 332 */ 333 public VirtualServiceDetail setSessionKeyElement(StringType value) { 334 this.sessionKey = value; 335 return this; 336 } 337 338 /** 339 * @return Session Key required by the virtual service. 340 */ 341 public String getSessionKey() { 342 return this.sessionKey == null ? null : this.sessionKey.getValue(); 343 } 344 345 /** 346 * @param value Session Key required by the virtual service. 347 */ 348 public VirtualServiceDetail setSessionKey(String value) { 349 if (Utilities.noString(value)) 350 this.sessionKey = null; 351 else { 352 if (this.sessionKey == null) 353 this.sessionKey = new StringType(); 354 this.sessionKey.setValue(value); 355 } 356 return this; 357 } 358 359 protected void listChildren(List<Property> children) { 360 super.listChildren(children); 361 children.add(new Property("channelType", "Coding", "The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp).", 0, 1, channelType)); 362 children.add(new Property("address[x]", "url|string|ContactPoint|ExtendedContactDetail", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address)); 363 children.add(new Property("additionalInfo", "url", "Address to see alternative connection details.", 0, java.lang.Integer.MAX_VALUE, additionalInfo)); 364 children.add(new Property("maxParticipants", "positiveInt", "Maximum number of participants supported by the virtual service.", 0, 1, maxParticipants)); 365 children.add(new Property("sessionKey", "string", "Session Key required by the virtual service.", 0, 1, sessionKey)); 366 } 367 368 @Override 369 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 370 switch (_hash) { 371 case 274155229: /*channelType*/ return new Property("channelType", "Coding", "The type of virtual service to connect to (i.e. Teams, Zoom, Specific VMR technology, WhatsApp).", 0, 1, channelType); 372 case 1341051916: /*address[x]*/ return new Property("address[x]", "url|string|ContactPoint|ExtendedContactDetail", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 373 case -1147692044: /*address*/ return new Property("address[x]", "url|string|ContactPoint|ExtendedContactDetail", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 374 case 1341045979: /*addressUrl*/ return new Property("address[x]", "url", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 375 case -740155099: /*addressString*/ return new Property("address[x]", "string", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 376 case 269121380: /*addressContactPoint*/ return new Property("address[x]", "ContactPoint", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 377 case -834417596: /*addressExtendedContactDetail*/ return new Property("address[x]", "ExtendedContactDetail", "What address or number needs to be used for a user to connect to the virtual service to join. The channelType informs as to which datatype is appropriate to use (requires knowledge of the specific type).", 0, 1, address); 378 case -974297739: /*additionalInfo*/ return new Property("additionalInfo", "url", "Address to see alternative connection details.", 0, java.lang.Integer.MAX_VALUE, additionalInfo); 379 case 950795044: /*maxParticipants*/ return new Property("maxParticipants", "positiveInt", "Maximum number of participants supported by the virtual service.", 0, 1, maxParticipants); 380 case 1661834217: /*sessionKey*/ return new Property("sessionKey", "string", "Session Key required by the virtual service.", 0, 1, sessionKey); 381 default: return super.getNamedProperty(_hash, _name, _checkValid); 382 } 383 384 } 385 386 @Override 387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 388 switch (hash) { 389 case 274155229: /*channelType*/ return this.channelType == null ? new Base[0] : new Base[] {this.channelType}; // Coding 390 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // DataType 391 case -974297739: /*additionalInfo*/ return this.additionalInfo == null ? new Base[0] : this.additionalInfo.toArray(new Base[this.additionalInfo.size()]); // UrlType 392 case 950795044: /*maxParticipants*/ return this.maxParticipants == null ? new Base[0] : new Base[] {this.maxParticipants}; // PositiveIntType 393 case 1661834217: /*sessionKey*/ return this.sessionKey == null ? new Base[0] : new Base[] {this.sessionKey}; // StringType 394 default: return super.getProperty(hash, name, checkValid); 395 } 396 397 } 398 399 @Override 400 public Base setProperty(int hash, String name, Base value) throws FHIRException { 401 switch (hash) { 402 case 274155229: // channelType 403 this.channelType = TypeConvertor.castToCoding(value); // Coding 404 return value; 405 case -1147692044: // address 406 this.address = TypeConvertor.castToType(value); // DataType 407 return value; 408 case -974297739: // additionalInfo 409 this.getAdditionalInfo().add(TypeConvertor.castToUrl(value)); // UrlType 410 return value; 411 case 950795044: // maxParticipants 412 this.maxParticipants = TypeConvertor.castToPositiveInt(value); // PositiveIntType 413 return value; 414 case 1661834217: // sessionKey 415 this.sessionKey = TypeConvertor.castToString(value); // StringType 416 return value; 417 default: return super.setProperty(hash, name, value); 418 } 419 420 } 421 422 @Override 423 public Base setProperty(String name, Base value) throws FHIRException { 424 if (name.equals("channelType")) { 425 this.channelType = TypeConvertor.castToCoding(value); // Coding 426 } else if (name.equals("address[x]")) { 427 this.address = TypeConvertor.castToType(value); // DataType 428 } else if (name.equals("additionalInfo")) { 429 this.getAdditionalInfo().add(TypeConvertor.castToUrl(value)); 430 } else if (name.equals("maxParticipants")) { 431 this.maxParticipants = TypeConvertor.castToPositiveInt(value); // PositiveIntType 432 } else if (name.equals("sessionKey")) { 433 this.sessionKey = TypeConvertor.castToString(value); // StringType 434 } else 435 return super.setProperty(name, value); 436 return value; 437 } 438 439 @Override 440 public void removeChild(String name, Base value) throws FHIRException { 441 if (name.equals("channelType")) { 442 this.channelType = null; 443 } else if (name.equals("address[x]")) { 444 this.address = null; 445 } else if (name.equals("additionalInfo")) { 446 this.getAdditionalInfo().remove(value); 447 } else if (name.equals("maxParticipants")) { 448 this.maxParticipants = null; 449 } else if (name.equals("sessionKey")) { 450 this.sessionKey = null; 451 } else 452 super.removeChild(name, value); 453 454 } 455 456 @Override 457 public Base makeProperty(int hash, String name) throws FHIRException { 458 switch (hash) { 459 case 274155229: return getChannelType(); 460 case 1341051916: return getAddress(); 461 case -1147692044: return getAddress(); 462 case -974297739: return addAdditionalInfoElement(); 463 case 950795044: return getMaxParticipantsElement(); 464 case 1661834217: return getSessionKeyElement(); 465 default: return super.makeProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case 274155229: /*channelType*/ return new String[] {"Coding"}; 474 case -1147692044: /*address*/ return new String[] {"url", "string", "ContactPoint", "ExtendedContactDetail"}; 475 case -974297739: /*additionalInfo*/ return new String[] {"url"}; 476 case 950795044: /*maxParticipants*/ return new String[] {"positiveInt"}; 477 case 1661834217: /*sessionKey*/ return new String[] {"string"}; 478 default: return super.getTypesForProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public Base addChild(String name) throws FHIRException { 485 if (name.equals("channelType")) { 486 this.channelType = new Coding(); 487 return this.channelType; 488 } 489 else if (name.equals("addressUrl")) { 490 this.address = new UrlType(); 491 return this.address; 492 } 493 else if (name.equals("addressString")) { 494 this.address = new StringType(); 495 return this.address; 496 } 497 else if (name.equals("addressContactPoint")) { 498 this.address = new ContactPoint(); 499 return this.address; 500 } 501 else if (name.equals("addressExtendedContactDetail")) { 502 this.address = new ExtendedContactDetail(); 503 return this.address; 504 } 505 else if (name.equals("additionalInfo")) { 506 throw new FHIRException("Cannot call addChild on a singleton property VirtualServiceDetail.additionalInfo"); 507 } 508 else if (name.equals("maxParticipants")) { 509 throw new FHIRException("Cannot call addChild on a singleton property VirtualServiceDetail.maxParticipants"); 510 } 511 else if (name.equals("sessionKey")) { 512 throw new FHIRException("Cannot call addChild on a singleton property VirtualServiceDetail.sessionKey"); 513 } 514 else 515 return super.addChild(name); 516 } 517 518 public String fhirType() { 519 return "VirtualServiceDetail"; 520 521 } 522 523 public VirtualServiceDetail copy() { 524 VirtualServiceDetail dst = new VirtualServiceDetail(); 525 copyValues(dst); 526 return dst; 527 } 528 529 public void copyValues(VirtualServiceDetail dst) { 530 super.copyValues(dst); 531 dst.channelType = channelType == null ? null : channelType.copy(); 532 dst.address = address == null ? null : address.copy(); 533 if (additionalInfo != null) { 534 dst.additionalInfo = new ArrayList<UrlType>(); 535 for (UrlType i : additionalInfo) 536 dst.additionalInfo.add(i.copy()); 537 }; 538 dst.maxParticipants = maxParticipants == null ? null : maxParticipants.copy(); 539 dst.sessionKey = sessionKey == null ? null : sessionKey.copy(); 540 } 541 542 protected VirtualServiceDetail typedCopy() { 543 return copy(); 544 } 545 546 @Override 547 public boolean equalsDeep(Base other_) { 548 if (!super.equalsDeep(other_)) 549 return false; 550 if (!(other_ instanceof VirtualServiceDetail)) 551 return false; 552 VirtualServiceDetail o = (VirtualServiceDetail) other_; 553 return compareDeep(channelType, o.channelType, true) && compareDeep(address, o.address, true) && compareDeep(additionalInfo, o.additionalInfo, true) 554 && compareDeep(maxParticipants, o.maxParticipants, true) && compareDeep(sessionKey, o.sessionKey, true) 555 ; 556 } 557 558 @Override 559 public boolean equalsShallow(Base other_) { 560 if (!super.equalsShallow(other_)) 561 return false; 562 if (!(other_ instanceof VirtualServiceDetail)) 563 return false; 564 VirtualServiceDetail o = (VirtualServiceDetail) other_; 565 return compareValues(additionalInfo, o.additionalInfo, true) && compareValues(maxParticipants, o.maxParticipants, true) 566 && compareValues(sessionKey, o.sessionKey, true); 567 } 568 569 public boolean isEmpty() { 570 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(channelType, address, additionalInfo 571 , maxParticipants, sessionKey); 572 } 573 574 575} 576