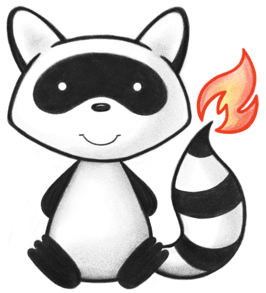
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * An authorization for the provision of glasses and/or contact lenses to a patient. 053 */ 054@ResourceDef(name="VisionPrescription", profile="http://hl7.org/fhir/StructureDefinition/VisionPrescription") 055public class VisionPrescription extends DomainResource { 056 057 public enum VisionBase { 058 /** 059 * top. 060 */ 061 UP, 062 /** 063 * bottom. 064 */ 065 DOWN, 066 /** 067 * inner edge. 068 */ 069 IN, 070 /** 071 * outer edge. 072 */ 073 OUT, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static VisionBase fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("up".equals(codeString)) 082 return UP; 083 if ("down".equals(codeString)) 084 return DOWN; 085 if ("in".equals(codeString)) 086 return IN; 087 if ("out".equals(codeString)) 088 return OUT; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown VisionBase code '"+codeString+"'"); 093 } 094 public String toCode() { 095 switch (this) { 096 case UP: return "up"; 097 case DOWN: return "down"; 098 case IN: return "in"; 099 case OUT: return "out"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getSystem() { 105 switch (this) { 106 case UP: return "http://hl7.org/fhir/vision-base-codes"; 107 case DOWN: return "http://hl7.org/fhir/vision-base-codes"; 108 case IN: return "http://hl7.org/fhir/vision-base-codes"; 109 case OUT: return "http://hl7.org/fhir/vision-base-codes"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case UP: return "top."; 117 case DOWN: return "bottom."; 118 case IN: return "inner edge."; 119 case OUT: return "outer edge."; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDisplay() { 125 switch (this) { 126 case UP: return "Up"; 127 case DOWN: return "Down"; 128 case IN: return "In"; 129 case OUT: return "Out"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 } 135 136 public static class VisionBaseEnumFactory implements EnumFactory<VisionBase> { 137 public VisionBase fromCode(String codeString) throws IllegalArgumentException { 138 if (codeString == null || "".equals(codeString)) 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("up".equals(codeString)) 142 return VisionBase.UP; 143 if ("down".equals(codeString)) 144 return VisionBase.DOWN; 145 if ("in".equals(codeString)) 146 return VisionBase.IN; 147 if ("out".equals(codeString)) 148 return VisionBase.OUT; 149 throw new IllegalArgumentException("Unknown VisionBase code '"+codeString+"'"); 150 } 151 public Enumeration<VisionBase> fromType(PrimitiveType<?> code) throws FHIRException { 152 if (code == null) 153 return null; 154 if (code.isEmpty()) 155 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 156 String codeString = ((PrimitiveType) code).asStringValue(); 157 if (codeString == null || "".equals(codeString)) 158 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 159 if ("up".equals(codeString)) 160 return new Enumeration<VisionBase>(this, VisionBase.UP, code); 161 if ("down".equals(codeString)) 162 return new Enumeration<VisionBase>(this, VisionBase.DOWN, code); 163 if ("in".equals(codeString)) 164 return new Enumeration<VisionBase>(this, VisionBase.IN, code); 165 if ("out".equals(codeString)) 166 return new Enumeration<VisionBase>(this, VisionBase.OUT, code); 167 throw new FHIRException("Unknown VisionBase code '"+codeString+"'"); 168 } 169 public String toCode(VisionBase code) { 170 if (code == VisionBase.NULL) 171 return null; 172 if (code == VisionBase.UP) 173 return "up"; 174 if (code == VisionBase.DOWN) 175 return "down"; 176 if (code == VisionBase.IN) 177 return "in"; 178 if (code == VisionBase.OUT) 179 return "out"; 180 return "?"; 181 } 182 public String toSystem(VisionBase code) { 183 return code.getSystem(); 184 } 185 } 186 187 public enum VisionEyes { 188 /** 189 * Right Eye. 190 */ 191 RIGHT, 192 /** 193 * Left Eye. 194 */ 195 LEFT, 196 /** 197 * added to help the parsers with the generic types 198 */ 199 NULL; 200 public static VisionEyes fromCode(String codeString) throws FHIRException { 201 if (codeString == null || "".equals(codeString)) 202 return null; 203 if ("right".equals(codeString)) 204 return RIGHT; 205 if ("left".equals(codeString)) 206 return LEFT; 207 if (Configuration.isAcceptInvalidEnums()) 208 return null; 209 else 210 throw new FHIRException("Unknown VisionEyes code '"+codeString+"'"); 211 } 212 public String toCode() { 213 switch (this) { 214 case RIGHT: return "right"; 215 case LEFT: return "left"; 216 case NULL: return null; 217 default: return "?"; 218 } 219 } 220 public String getSystem() { 221 switch (this) { 222 case RIGHT: return "http://hl7.org/fhir/vision-eye-codes"; 223 case LEFT: return "http://hl7.org/fhir/vision-eye-codes"; 224 case NULL: return null; 225 default: return "?"; 226 } 227 } 228 public String getDefinition() { 229 switch (this) { 230 case RIGHT: return "Right Eye."; 231 case LEFT: return "Left Eye."; 232 case NULL: return null; 233 default: return "?"; 234 } 235 } 236 public String getDisplay() { 237 switch (this) { 238 case RIGHT: return "Right Eye"; 239 case LEFT: return "Left Eye"; 240 case NULL: return null; 241 default: return "?"; 242 } 243 } 244 } 245 246 public static class VisionEyesEnumFactory implements EnumFactory<VisionEyes> { 247 public VisionEyes fromCode(String codeString) throws IllegalArgumentException { 248 if (codeString == null || "".equals(codeString)) 249 if (codeString == null || "".equals(codeString)) 250 return null; 251 if ("right".equals(codeString)) 252 return VisionEyes.RIGHT; 253 if ("left".equals(codeString)) 254 return VisionEyes.LEFT; 255 throw new IllegalArgumentException("Unknown VisionEyes code '"+codeString+"'"); 256 } 257 public Enumeration<VisionEyes> fromType(PrimitiveType<?> code) throws FHIRException { 258 if (code == null) 259 return null; 260 if (code.isEmpty()) 261 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 262 String codeString = ((PrimitiveType) code).asStringValue(); 263 if (codeString == null || "".equals(codeString)) 264 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 265 if ("right".equals(codeString)) 266 return new Enumeration<VisionEyes>(this, VisionEyes.RIGHT, code); 267 if ("left".equals(codeString)) 268 return new Enumeration<VisionEyes>(this, VisionEyes.LEFT, code); 269 throw new FHIRException("Unknown VisionEyes code '"+codeString+"'"); 270 } 271 public String toCode(VisionEyes code) { 272 if (code == VisionEyes.NULL) 273 return null; 274 if (code == VisionEyes.RIGHT) 275 return "right"; 276 if (code == VisionEyes.LEFT) 277 return "left"; 278 return "?"; 279 } 280 public String toSystem(VisionEyes code) { 281 return code.getSystem(); 282 } 283 } 284 285 @Block() 286 public static class VisionPrescriptionLensSpecificationComponent extends BackboneElement implements IBaseBackboneElement { 287 /** 288 * Identifies the type of vision correction product which is required for the patient. 289 */ 290 @Child(name = "product", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 291 @Description(shortDefinition="Product to be supplied", formalDefinition="Identifies the type of vision correction product which is required for the patient." ) 292 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-product") 293 protected CodeableConcept product; 294 295 /** 296 * The eye for which the lens specification applies. 297 */ 298 @Child(name = "eye", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 299 @Description(shortDefinition="right | left", formalDefinition="The eye for which the lens specification applies." ) 300 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-eye-codes") 301 protected Enumeration<VisionEyes> eye; 302 303 /** 304 * Lens power measured in dioptres (0.25 units). 305 */ 306 @Child(name = "sphere", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 307 @Description(shortDefinition="Power of the lens", formalDefinition="Lens power measured in dioptres (0.25 units)." ) 308 protected DecimalType sphere; 309 310 /** 311 * Power adjustment for astigmatism measured in dioptres (0.25 units). 312 */ 313 @Child(name = "cylinder", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 314 @Description(shortDefinition="Lens power for astigmatism", formalDefinition="Power adjustment for astigmatism measured in dioptres (0.25 units)." ) 315 protected DecimalType cylinder; 316 317 /** 318 * Adjustment for astigmatism measured in integer degrees. 319 */ 320 @Child(name = "axis", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=false) 321 @Description(shortDefinition="Lens meridian which contain no power for astigmatism", formalDefinition="Adjustment for astigmatism measured in integer degrees." ) 322 protected IntegerType axis; 323 324 /** 325 * Allows for adjustment on two axis. 326 */ 327 @Child(name = "prism", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 328 @Description(shortDefinition="Eye alignment compensation", formalDefinition="Allows for adjustment on two axis." ) 329 protected List<PrismComponent> prism; 330 331 /** 332 * Power adjustment for multifocal lenses measured in dioptres (0.25 units). 333 */ 334 @Child(name = "add", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 335 @Description(shortDefinition="Added power for multifocal levels", formalDefinition="Power adjustment for multifocal lenses measured in dioptres (0.25 units)." ) 336 protected DecimalType add; 337 338 /** 339 * Contact lens power measured in dioptres (0.25 units). 340 */ 341 @Child(name = "power", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=false) 342 @Description(shortDefinition="Contact lens power", formalDefinition="Contact lens power measured in dioptres (0.25 units)." ) 343 protected DecimalType power; 344 345 /** 346 * Back curvature measured in millimetres. 347 */ 348 @Child(name = "backCurve", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 349 @Description(shortDefinition="Contact lens back curvature", formalDefinition="Back curvature measured in millimetres." ) 350 protected DecimalType backCurve; 351 352 /** 353 * Contact lens diameter measured in millimetres. 354 */ 355 @Child(name = "diameter", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 356 @Description(shortDefinition="Contact lens diameter", formalDefinition="Contact lens diameter measured in millimetres." ) 357 protected DecimalType diameter; 358 359 /** 360 * The recommended maximum wear period for the lens. 361 */ 362 @Child(name = "duration", type = {Quantity.class}, order=11, min=0, max=1, modifier=false, summary=false) 363 @Description(shortDefinition="Lens wear duration", formalDefinition="The recommended maximum wear period for the lens." ) 364 protected Quantity duration; 365 366 /** 367 * Special color or pattern. 368 */ 369 @Child(name = "color", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 370 @Description(shortDefinition="Color required", formalDefinition="Special color or pattern." ) 371 protected StringType color; 372 373 /** 374 * Brand recommendations or restrictions. 375 */ 376 @Child(name = "brand", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 377 @Description(shortDefinition="Brand required", formalDefinition="Brand recommendations or restrictions." ) 378 protected StringType brand; 379 380 /** 381 * Notes for special requirements such as coatings and lens materials. 382 */ 383 @Child(name = "note", type = {Annotation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 384 @Description(shortDefinition="Notes for coatings", formalDefinition="Notes for special requirements such as coatings and lens materials." ) 385 protected List<Annotation> note; 386 387 private static final long serialVersionUID = 688924460L; 388 389 /** 390 * Constructor 391 */ 392 public VisionPrescriptionLensSpecificationComponent() { 393 super(); 394 } 395 396 /** 397 * Constructor 398 */ 399 public VisionPrescriptionLensSpecificationComponent(CodeableConcept product, VisionEyes eye) { 400 super(); 401 this.setProduct(product); 402 this.setEye(eye); 403 } 404 405 /** 406 * @return {@link #product} (Identifies the type of vision correction product which is required for the patient.) 407 */ 408 public CodeableConcept getProduct() { 409 if (this.product == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.product"); 412 else if (Configuration.doAutoCreate()) 413 this.product = new CodeableConcept(); // cc 414 return this.product; 415 } 416 417 public boolean hasProduct() { 418 return this.product != null && !this.product.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #product} (Identifies the type of vision correction product which is required for the patient.) 423 */ 424 public VisionPrescriptionLensSpecificationComponent setProduct(CodeableConcept value) { 425 this.product = value; 426 return this; 427 } 428 429 /** 430 * @return {@link #eye} (The eye for which the lens specification applies.). This is the underlying object with id, value and extensions. The accessor "getEye" gives direct access to the value 431 */ 432 public Enumeration<VisionEyes> getEyeElement() { 433 if (this.eye == null) 434 if (Configuration.errorOnAutoCreate()) 435 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.eye"); 436 else if (Configuration.doAutoCreate()) 437 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); // bb 438 return this.eye; 439 } 440 441 public boolean hasEyeElement() { 442 return this.eye != null && !this.eye.isEmpty(); 443 } 444 445 public boolean hasEye() { 446 return this.eye != null && !this.eye.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #eye} (The eye for which the lens specification applies.). This is the underlying object with id, value and extensions. The accessor "getEye" gives direct access to the value 451 */ 452 public VisionPrescriptionLensSpecificationComponent setEyeElement(Enumeration<VisionEyes> value) { 453 this.eye = value; 454 return this; 455 } 456 457 /** 458 * @return The eye for which the lens specification applies. 459 */ 460 public VisionEyes getEye() { 461 return this.eye == null ? null : this.eye.getValue(); 462 } 463 464 /** 465 * @param value The eye for which the lens specification applies. 466 */ 467 public VisionPrescriptionLensSpecificationComponent setEye(VisionEyes value) { 468 if (this.eye == null) 469 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); 470 this.eye.setValue(value); 471 return this; 472 } 473 474 /** 475 * @return {@link #sphere} (Lens power measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getSphere" gives direct access to the value 476 */ 477 public DecimalType getSphereElement() { 478 if (this.sphere == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.sphere"); 481 else if (Configuration.doAutoCreate()) 482 this.sphere = new DecimalType(); // bb 483 return this.sphere; 484 } 485 486 public boolean hasSphereElement() { 487 return this.sphere != null && !this.sphere.isEmpty(); 488 } 489 490 public boolean hasSphere() { 491 return this.sphere != null && !this.sphere.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #sphere} (Lens power measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getSphere" gives direct access to the value 496 */ 497 public VisionPrescriptionLensSpecificationComponent setSphereElement(DecimalType value) { 498 this.sphere = value; 499 return this; 500 } 501 502 /** 503 * @return Lens power measured in dioptres (0.25 units). 504 */ 505 public BigDecimal getSphere() { 506 return this.sphere == null ? null : this.sphere.getValue(); 507 } 508 509 /** 510 * @param value Lens power measured in dioptres (0.25 units). 511 */ 512 public VisionPrescriptionLensSpecificationComponent setSphere(BigDecimal value) { 513 if (value == null) 514 this.sphere = null; 515 else { 516 if (this.sphere == null) 517 this.sphere = new DecimalType(); 518 this.sphere.setValue(value); 519 } 520 return this; 521 } 522 523 /** 524 * @param value Lens power measured in dioptres (0.25 units). 525 */ 526 public VisionPrescriptionLensSpecificationComponent setSphere(long value) { 527 this.sphere = new DecimalType(); 528 this.sphere.setValue(value); 529 return this; 530 } 531 532 /** 533 * @param value Lens power measured in dioptres (0.25 units). 534 */ 535 public VisionPrescriptionLensSpecificationComponent setSphere(double value) { 536 this.sphere = new DecimalType(); 537 this.sphere.setValue(value); 538 return this; 539 } 540 541 /** 542 * @return {@link #cylinder} (Power adjustment for astigmatism measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getCylinder" gives direct access to the value 543 */ 544 public DecimalType getCylinderElement() { 545 if (this.cylinder == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.cylinder"); 548 else if (Configuration.doAutoCreate()) 549 this.cylinder = new DecimalType(); // bb 550 return this.cylinder; 551 } 552 553 public boolean hasCylinderElement() { 554 return this.cylinder != null && !this.cylinder.isEmpty(); 555 } 556 557 public boolean hasCylinder() { 558 return this.cylinder != null && !this.cylinder.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #cylinder} (Power adjustment for astigmatism measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getCylinder" gives direct access to the value 563 */ 564 public VisionPrescriptionLensSpecificationComponent setCylinderElement(DecimalType value) { 565 this.cylinder = value; 566 return this; 567 } 568 569 /** 570 * @return Power adjustment for astigmatism measured in dioptres (0.25 units). 571 */ 572 public BigDecimal getCylinder() { 573 return this.cylinder == null ? null : this.cylinder.getValue(); 574 } 575 576 /** 577 * @param value Power adjustment for astigmatism measured in dioptres (0.25 units). 578 */ 579 public VisionPrescriptionLensSpecificationComponent setCylinder(BigDecimal value) { 580 if (value == null) 581 this.cylinder = null; 582 else { 583 if (this.cylinder == null) 584 this.cylinder = new DecimalType(); 585 this.cylinder.setValue(value); 586 } 587 return this; 588 } 589 590 /** 591 * @param value Power adjustment for astigmatism measured in dioptres (0.25 units). 592 */ 593 public VisionPrescriptionLensSpecificationComponent setCylinder(long value) { 594 this.cylinder = new DecimalType(); 595 this.cylinder.setValue(value); 596 return this; 597 } 598 599 /** 600 * @param value Power adjustment for astigmatism measured in dioptres (0.25 units). 601 */ 602 public VisionPrescriptionLensSpecificationComponent setCylinder(double value) { 603 this.cylinder = new DecimalType(); 604 this.cylinder.setValue(value); 605 return this; 606 } 607 608 /** 609 * @return {@link #axis} (Adjustment for astigmatism measured in integer degrees.). This is the underlying object with id, value and extensions. The accessor "getAxis" gives direct access to the value 610 */ 611 public IntegerType getAxisElement() { 612 if (this.axis == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.axis"); 615 else if (Configuration.doAutoCreate()) 616 this.axis = new IntegerType(); // bb 617 return this.axis; 618 } 619 620 public boolean hasAxisElement() { 621 return this.axis != null && !this.axis.isEmpty(); 622 } 623 624 public boolean hasAxis() { 625 return this.axis != null && !this.axis.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #axis} (Adjustment for astigmatism measured in integer degrees.). This is the underlying object with id, value and extensions. The accessor "getAxis" gives direct access to the value 630 */ 631 public VisionPrescriptionLensSpecificationComponent setAxisElement(IntegerType value) { 632 this.axis = value; 633 return this; 634 } 635 636 /** 637 * @return Adjustment for astigmatism measured in integer degrees. 638 */ 639 public int getAxis() { 640 return this.axis == null || this.axis.isEmpty() ? 0 : this.axis.getValue(); 641 } 642 643 /** 644 * @param value Adjustment for astigmatism measured in integer degrees. 645 */ 646 public VisionPrescriptionLensSpecificationComponent setAxis(int value) { 647 if (this.axis == null) 648 this.axis = new IntegerType(); 649 this.axis.setValue(value); 650 return this; 651 } 652 653 /** 654 * @return {@link #prism} (Allows for adjustment on two axis.) 655 */ 656 public List<PrismComponent> getPrism() { 657 if (this.prism == null) 658 this.prism = new ArrayList<PrismComponent>(); 659 return this.prism; 660 } 661 662 /** 663 * @return Returns a reference to <code>this</code> for easy method chaining 664 */ 665 public VisionPrescriptionLensSpecificationComponent setPrism(List<PrismComponent> thePrism) { 666 this.prism = thePrism; 667 return this; 668 } 669 670 public boolean hasPrism() { 671 if (this.prism == null) 672 return false; 673 for (PrismComponent item : this.prism) 674 if (!item.isEmpty()) 675 return true; 676 return false; 677 } 678 679 public PrismComponent addPrism() { //3 680 PrismComponent t = new PrismComponent(); 681 if (this.prism == null) 682 this.prism = new ArrayList<PrismComponent>(); 683 this.prism.add(t); 684 return t; 685 } 686 687 public VisionPrescriptionLensSpecificationComponent addPrism(PrismComponent t) { //3 688 if (t == null) 689 return this; 690 if (this.prism == null) 691 this.prism = new ArrayList<PrismComponent>(); 692 this.prism.add(t); 693 return this; 694 } 695 696 /** 697 * @return The first repetition of repeating field {@link #prism}, creating it if it does not already exist {3} 698 */ 699 public PrismComponent getPrismFirstRep() { 700 if (getPrism().isEmpty()) { 701 addPrism(); 702 } 703 return getPrism().get(0); 704 } 705 706 /** 707 * @return {@link #add} (Power adjustment for multifocal lenses measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getAdd" gives direct access to the value 708 */ 709 public DecimalType getAddElement() { 710 if (this.add == null) 711 if (Configuration.errorOnAutoCreate()) 712 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.add"); 713 else if (Configuration.doAutoCreate()) 714 this.add = new DecimalType(); // bb 715 return this.add; 716 } 717 718 public boolean hasAddElement() { 719 return this.add != null && !this.add.isEmpty(); 720 } 721 722 public boolean hasAdd() { 723 return this.add != null && !this.add.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #add} (Power adjustment for multifocal lenses measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getAdd" gives direct access to the value 728 */ 729 public VisionPrescriptionLensSpecificationComponent setAddElement(DecimalType value) { 730 this.add = value; 731 return this; 732 } 733 734 /** 735 * @return Power adjustment for multifocal lenses measured in dioptres (0.25 units). 736 */ 737 public BigDecimal getAdd() { 738 return this.add == null ? null : this.add.getValue(); 739 } 740 741 /** 742 * @param value Power adjustment for multifocal lenses measured in dioptres (0.25 units). 743 */ 744 public VisionPrescriptionLensSpecificationComponent setAdd(BigDecimal value) { 745 if (value == null) 746 this.add = null; 747 else { 748 if (this.add == null) 749 this.add = new DecimalType(); 750 this.add.setValue(value); 751 } 752 return this; 753 } 754 755 /** 756 * @param value Power adjustment for multifocal lenses measured in dioptres (0.25 units). 757 */ 758 public VisionPrescriptionLensSpecificationComponent setAdd(long value) { 759 this.add = new DecimalType(); 760 this.add.setValue(value); 761 return this; 762 } 763 764 /** 765 * @param value Power adjustment for multifocal lenses measured in dioptres (0.25 units). 766 */ 767 public VisionPrescriptionLensSpecificationComponent setAdd(double value) { 768 this.add = new DecimalType(); 769 this.add.setValue(value); 770 return this; 771 } 772 773 /** 774 * @return {@link #power} (Contact lens power measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getPower" gives direct access to the value 775 */ 776 public DecimalType getPowerElement() { 777 if (this.power == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.power"); 780 else if (Configuration.doAutoCreate()) 781 this.power = new DecimalType(); // bb 782 return this.power; 783 } 784 785 public boolean hasPowerElement() { 786 return this.power != null && !this.power.isEmpty(); 787 } 788 789 public boolean hasPower() { 790 return this.power != null && !this.power.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #power} (Contact lens power measured in dioptres (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getPower" gives direct access to the value 795 */ 796 public VisionPrescriptionLensSpecificationComponent setPowerElement(DecimalType value) { 797 this.power = value; 798 return this; 799 } 800 801 /** 802 * @return Contact lens power measured in dioptres (0.25 units). 803 */ 804 public BigDecimal getPower() { 805 return this.power == null ? null : this.power.getValue(); 806 } 807 808 /** 809 * @param value Contact lens power measured in dioptres (0.25 units). 810 */ 811 public VisionPrescriptionLensSpecificationComponent setPower(BigDecimal value) { 812 if (value == null) 813 this.power = null; 814 else { 815 if (this.power == null) 816 this.power = new DecimalType(); 817 this.power.setValue(value); 818 } 819 return this; 820 } 821 822 /** 823 * @param value Contact lens power measured in dioptres (0.25 units). 824 */ 825 public VisionPrescriptionLensSpecificationComponent setPower(long value) { 826 this.power = new DecimalType(); 827 this.power.setValue(value); 828 return this; 829 } 830 831 /** 832 * @param value Contact lens power measured in dioptres (0.25 units). 833 */ 834 public VisionPrescriptionLensSpecificationComponent setPower(double value) { 835 this.power = new DecimalType(); 836 this.power.setValue(value); 837 return this; 838 } 839 840 /** 841 * @return {@link #backCurve} (Back curvature measured in millimetres.). This is the underlying object with id, value and extensions. The accessor "getBackCurve" gives direct access to the value 842 */ 843 public DecimalType getBackCurveElement() { 844 if (this.backCurve == null) 845 if (Configuration.errorOnAutoCreate()) 846 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.backCurve"); 847 else if (Configuration.doAutoCreate()) 848 this.backCurve = new DecimalType(); // bb 849 return this.backCurve; 850 } 851 852 public boolean hasBackCurveElement() { 853 return this.backCurve != null && !this.backCurve.isEmpty(); 854 } 855 856 public boolean hasBackCurve() { 857 return this.backCurve != null && !this.backCurve.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #backCurve} (Back curvature measured in millimetres.). This is the underlying object with id, value and extensions. The accessor "getBackCurve" gives direct access to the value 862 */ 863 public VisionPrescriptionLensSpecificationComponent setBackCurveElement(DecimalType value) { 864 this.backCurve = value; 865 return this; 866 } 867 868 /** 869 * @return Back curvature measured in millimetres. 870 */ 871 public BigDecimal getBackCurve() { 872 return this.backCurve == null ? null : this.backCurve.getValue(); 873 } 874 875 /** 876 * @param value Back curvature measured in millimetres. 877 */ 878 public VisionPrescriptionLensSpecificationComponent setBackCurve(BigDecimal value) { 879 if (value == null) 880 this.backCurve = null; 881 else { 882 if (this.backCurve == null) 883 this.backCurve = new DecimalType(); 884 this.backCurve.setValue(value); 885 } 886 return this; 887 } 888 889 /** 890 * @param value Back curvature measured in millimetres. 891 */ 892 public VisionPrescriptionLensSpecificationComponent setBackCurve(long value) { 893 this.backCurve = new DecimalType(); 894 this.backCurve.setValue(value); 895 return this; 896 } 897 898 /** 899 * @param value Back curvature measured in millimetres. 900 */ 901 public VisionPrescriptionLensSpecificationComponent setBackCurve(double value) { 902 this.backCurve = new DecimalType(); 903 this.backCurve.setValue(value); 904 return this; 905 } 906 907 /** 908 * @return {@link #diameter} (Contact lens diameter measured in millimetres.). This is the underlying object with id, value and extensions. The accessor "getDiameter" gives direct access to the value 909 */ 910 public DecimalType getDiameterElement() { 911 if (this.diameter == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.diameter"); 914 else if (Configuration.doAutoCreate()) 915 this.diameter = new DecimalType(); // bb 916 return this.diameter; 917 } 918 919 public boolean hasDiameterElement() { 920 return this.diameter != null && !this.diameter.isEmpty(); 921 } 922 923 public boolean hasDiameter() { 924 return this.diameter != null && !this.diameter.isEmpty(); 925 } 926 927 /** 928 * @param value {@link #diameter} (Contact lens diameter measured in millimetres.). This is the underlying object with id, value and extensions. The accessor "getDiameter" gives direct access to the value 929 */ 930 public VisionPrescriptionLensSpecificationComponent setDiameterElement(DecimalType value) { 931 this.diameter = value; 932 return this; 933 } 934 935 /** 936 * @return Contact lens diameter measured in millimetres. 937 */ 938 public BigDecimal getDiameter() { 939 return this.diameter == null ? null : this.diameter.getValue(); 940 } 941 942 /** 943 * @param value Contact lens diameter measured in millimetres. 944 */ 945 public VisionPrescriptionLensSpecificationComponent setDiameter(BigDecimal value) { 946 if (value == null) 947 this.diameter = null; 948 else { 949 if (this.diameter == null) 950 this.diameter = new DecimalType(); 951 this.diameter.setValue(value); 952 } 953 return this; 954 } 955 956 /** 957 * @param value Contact lens diameter measured in millimetres. 958 */ 959 public VisionPrescriptionLensSpecificationComponent setDiameter(long value) { 960 this.diameter = new DecimalType(); 961 this.diameter.setValue(value); 962 return this; 963 } 964 965 /** 966 * @param value Contact lens diameter measured in millimetres. 967 */ 968 public VisionPrescriptionLensSpecificationComponent setDiameter(double value) { 969 this.diameter = new DecimalType(); 970 this.diameter.setValue(value); 971 return this; 972 } 973 974 /** 975 * @return {@link #duration} (The recommended maximum wear period for the lens.) 976 */ 977 public Quantity getDuration() { 978 if (this.duration == null) 979 if (Configuration.errorOnAutoCreate()) 980 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.duration"); 981 else if (Configuration.doAutoCreate()) 982 this.duration = new Quantity(); // cc 983 return this.duration; 984 } 985 986 public boolean hasDuration() { 987 return this.duration != null && !this.duration.isEmpty(); 988 } 989 990 /** 991 * @param value {@link #duration} (The recommended maximum wear period for the lens.) 992 */ 993 public VisionPrescriptionLensSpecificationComponent setDuration(Quantity value) { 994 this.duration = value; 995 return this; 996 } 997 998 /** 999 * @return {@link #color} (Special color or pattern.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1000 */ 1001 public StringType getColorElement() { 1002 if (this.color == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.color"); 1005 else if (Configuration.doAutoCreate()) 1006 this.color = new StringType(); // bb 1007 return this.color; 1008 } 1009 1010 public boolean hasColorElement() { 1011 return this.color != null && !this.color.isEmpty(); 1012 } 1013 1014 public boolean hasColor() { 1015 return this.color != null && !this.color.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #color} (Special color or pattern.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1020 */ 1021 public VisionPrescriptionLensSpecificationComponent setColorElement(StringType value) { 1022 this.color = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return Special color or pattern. 1028 */ 1029 public String getColor() { 1030 return this.color == null ? null : this.color.getValue(); 1031 } 1032 1033 /** 1034 * @param value Special color or pattern. 1035 */ 1036 public VisionPrescriptionLensSpecificationComponent setColor(String value) { 1037 if (Utilities.noString(value)) 1038 this.color = null; 1039 else { 1040 if (this.color == null) 1041 this.color = new StringType(); 1042 this.color.setValue(value); 1043 } 1044 return this; 1045 } 1046 1047 /** 1048 * @return {@link #brand} (Brand recommendations or restrictions.). This is the underlying object with id, value and extensions. The accessor "getBrand" gives direct access to the value 1049 */ 1050 public StringType getBrandElement() { 1051 if (this.brand == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.brand"); 1054 else if (Configuration.doAutoCreate()) 1055 this.brand = new StringType(); // bb 1056 return this.brand; 1057 } 1058 1059 public boolean hasBrandElement() { 1060 return this.brand != null && !this.brand.isEmpty(); 1061 } 1062 1063 public boolean hasBrand() { 1064 return this.brand != null && !this.brand.isEmpty(); 1065 } 1066 1067 /** 1068 * @param value {@link #brand} (Brand recommendations or restrictions.). This is the underlying object with id, value and extensions. The accessor "getBrand" gives direct access to the value 1069 */ 1070 public VisionPrescriptionLensSpecificationComponent setBrandElement(StringType value) { 1071 this.brand = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return Brand recommendations or restrictions. 1077 */ 1078 public String getBrand() { 1079 return this.brand == null ? null : this.brand.getValue(); 1080 } 1081 1082 /** 1083 * @param value Brand recommendations or restrictions. 1084 */ 1085 public VisionPrescriptionLensSpecificationComponent setBrand(String value) { 1086 if (Utilities.noString(value)) 1087 this.brand = null; 1088 else { 1089 if (this.brand == null) 1090 this.brand = new StringType(); 1091 this.brand.setValue(value); 1092 } 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #note} (Notes for special requirements such as coatings and lens materials.) 1098 */ 1099 public List<Annotation> getNote() { 1100 if (this.note == null) 1101 this.note = new ArrayList<Annotation>(); 1102 return this.note; 1103 } 1104 1105 /** 1106 * @return Returns a reference to <code>this</code> for easy method chaining 1107 */ 1108 public VisionPrescriptionLensSpecificationComponent setNote(List<Annotation> theNote) { 1109 this.note = theNote; 1110 return this; 1111 } 1112 1113 public boolean hasNote() { 1114 if (this.note == null) 1115 return false; 1116 for (Annotation item : this.note) 1117 if (!item.isEmpty()) 1118 return true; 1119 return false; 1120 } 1121 1122 public Annotation addNote() { //3 1123 Annotation t = new Annotation(); 1124 if (this.note == null) 1125 this.note = new ArrayList<Annotation>(); 1126 this.note.add(t); 1127 return t; 1128 } 1129 1130 public VisionPrescriptionLensSpecificationComponent addNote(Annotation t) { //3 1131 if (t == null) 1132 return this; 1133 if (this.note == null) 1134 this.note = new ArrayList<Annotation>(); 1135 this.note.add(t); 1136 return this; 1137 } 1138 1139 /** 1140 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1141 */ 1142 public Annotation getNoteFirstRep() { 1143 if (getNote().isEmpty()) { 1144 addNote(); 1145 } 1146 return getNote().get(0); 1147 } 1148 1149 protected void listChildren(List<Property> children) { 1150 super.listChildren(children); 1151 children.add(new Property("product", "CodeableConcept", "Identifies the type of vision correction product which is required for the patient.", 0, 1, product)); 1152 children.add(new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye)); 1153 children.add(new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, sphere)); 1154 children.add(new Property("cylinder", "decimal", "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder)); 1155 children.add(new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis)); 1156 children.add(new Property("prism", "", "Allows for adjustment on two axis.", 0, java.lang.Integer.MAX_VALUE, prism)); 1157 children.add(new Property("add", "decimal", "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add)); 1158 children.add(new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1, power)); 1159 children.add(new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, backCurve)); 1160 children.add(new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1, diameter)); 1161 children.add(new Property("duration", "Quantity", "The recommended maximum wear period for the lens.", 0, 1, duration)); 1162 children.add(new Property("color", "string", "Special color or pattern.", 0, 1, color)); 1163 children.add(new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand)); 1164 children.add(new Property("note", "Annotation", "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note)); 1165 } 1166 1167 @Override 1168 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1169 switch (_hash) { 1170 case -309474065: /*product*/ return new Property("product", "CodeableConcept", "Identifies the type of vision correction product which is required for the patient.", 0, 1, product); 1171 case 100913: /*eye*/ return new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye); 1172 case -895981619: /*sphere*/ return new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, sphere); 1173 case -349378602: /*cylinder*/ return new Property("cylinder", "decimal", "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder); 1174 case 3008417: /*axis*/ return new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis); 1175 case 106935105: /*prism*/ return new Property("prism", "", "Allows for adjustment on two axis.", 0, java.lang.Integer.MAX_VALUE, prism); 1176 case 96417: /*add*/ return new Property("add", "decimal", "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add); 1177 case 106858757: /*power*/ return new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1, power); 1178 case 1309344840: /*backCurve*/ return new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, backCurve); 1179 case -233204595: /*diameter*/ return new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1, diameter); 1180 case -1992012396: /*duration*/ return new Property("duration", "Quantity", "The recommended maximum wear period for the lens.", 0, 1, duration); 1181 case 94842723: /*color*/ return new Property("color", "string", "Special color or pattern.", 0, 1, color); 1182 case 93997959: /*brand*/ return new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand); 1183 case 3387378: /*note*/ return new Property("note", "Annotation", "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note); 1184 default: return super.getNamedProperty(_hash, _name, _checkValid); 1185 } 1186 1187 } 1188 1189 @Override 1190 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1191 switch (hash) { 1192 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // CodeableConcept 1193 case 100913: /*eye*/ return this.eye == null ? new Base[0] : new Base[] {this.eye}; // Enumeration<VisionEyes> 1194 case -895981619: /*sphere*/ return this.sphere == null ? new Base[0] : new Base[] {this.sphere}; // DecimalType 1195 case -349378602: /*cylinder*/ return this.cylinder == null ? new Base[0] : new Base[] {this.cylinder}; // DecimalType 1196 case 3008417: /*axis*/ return this.axis == null ? new Base[0] : new Base[] {this.axis}; // IntegerType 1197 case 106935105: /*prism*/ return this.prism == null ? new Base[0] : this.prism.toArray(new Base[this.prism.size()]); // PrismComponent 1198 case 96417: /*add*/ return this.add == null ? new Base[0] : new Base[] {this.add}; // DecimalType 1199 case 106858757: /*power*/ return this.power == null ? new Base[0] : new Base[] {this.power}; // DecimalType 1200 case 1309344840: /*backCurve*/ return this.backCurve == null ? new Base[0] : new Base[] {this.backCurve}; // DecimalType 1201 case -233204595: /*diameter*/ return this.diameter == null ? new Base[0] : new Base[] {this.diameter}; // DecimalType 1202 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Quantity 1203 case 94842723: /*color*/ return this.color == null ? new Base[0] : new Base[] {this.color}; // StringType 1204 case 93997959: /*brand*/ return this.brand == null ? new Base[0] : new Base[] {this.brand}; // StringType 1205 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1206 default: return super.getProperty(hash, name, checkValid); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1213 switch (hash) { 1214 case -309474065: // product 1215 this.product = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1216 return value; 1217 case 100913: // eye 1218 value = new VisionEyesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1219 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1220 return value; 1221 case -895981619: // sphere 1222 this.sphere = TypeConvertor.castToDecimal(value); // DecimalType 1223 return value; 1224 case -349378602: // cylinder 1225 this.cylinder = TypeConvertor.castToDecimal(value); // DecimalType 1226 return value; 1227 case 3008417: // axis 1228 this.axis = TypeConvertor.castToInteger(value); // IntegerType 1229 return value; 1230 case 106935105: // prism 1231 this.getPrism().add((PrismComponent) value); // PrismComponent 1232 return value; 1233 case 96417: // add 1234 this.add = TypeConvertor.castToDecimal(value); // DecimalType 1235 return value; 1236 case 106858757: // power 1237 this.power = TypeConvertor.castToDecimal(value); // DecimalType 1238 return value; 1239 case 1309344840: // backCurve 1240 this.backCurve = TypeConvertor.castToDecimal(value); // DecimalType 1241 return value; 1242 case -233204595: // diameter 1243 this.diameter = TypeConvertor.castToDecimal(value); // DecimalType 1244 return value; 1245 case -1992012396: // duration 1246 this.duration = TypeConvertor.castToQuantity(value); // Quantity 1247 return value; 1248 case 94842723: // color 1249 this.color = TypeConvertor.castToString(value); // StringType 1250 return value; 1251 case 93997959: // brand 1252 this.brand = TypeConvertor.castToString(value); // StringType 1253 return value; 1254 case 3387378: // note 1255 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1256 return value; 1257 default: return super.setProperty(hash, name, value); 1258 } 1259 1260 } 1261 1262 @Override 1263 public Base setProperty(String name, Base value) throws FHIRException { 1264 if (name.equals("product")) { 1265 this.product = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1266 } else if (name.equals("eye")) { 1267 value = new VisionEyesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1268 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1269 } else if (name.equals("sphere")) { 1270 this.sphere = TypeConvertor.castToDecimal(value); // DecimalType 1271 } else if (name.equals("cylinder")) { 1272 this.cylinder = TypeConvertor.castToDecimal(value); // DecimalType 1273 } else if (name.equals("axis")) { 1274 this.axis = TypeConvertor.castToInteger(value); // IntegerType 1275 } else if (name.equals("prism")) { 1276 this.getPrism().add((PrismComponent) value); 1277 } else if (name.equals("add")) { 1278 this.add = TypeConvertor.castToDecimal(value); // DecimalType 1279 } else if (name.equals("power")) { 1280 this.power = TypeConvertor.castToDecimal(value); // DecimalType 1281 } else if (name.equals("backCurve")) { 1282 this.backCurve = TypeConvertor.castToDecimal(value); // DecimalType 1283 } else if (name.equals("diameter")) { 1284 this.diameter = TypeConvertor.castToDecimal(value); // DecimalType 1285 } else if (name.equals("duration")) { 1286 this.duration = TypeConvertor.castToQuantity(value); // Quantity 1287 } else if (name.equals("color")) { 1288 this.color = TypeConvertor.castToString(value); // StringType 1289 } else if (name.equals("brand")) { 1290 this.brand = TypeConvertor.castToString(value); // StringType 1291 } else if (name.equals("note")) { 1292 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1293 } else 1294 return super.setProperty(name, value); 1295 return value; 1296 } 1297 1298 @Override 1299 public void removeChild(String name, Base value) throws FHIRException { 1300 if (name.equals("product")) { 1301 this.product = null; 1302 } else if (name.equals("eye")) { 1303 value = new VisionEyesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1304 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1305 } else if (name.equals("sphere")) { 1306 this.sphere = null; 1307 } else if (name.equals("cylinder")) { 1308 this.cylinder = null; 1309 } else if (name.equals("axis")) { 1310 this.axis = null; 1311 } else if (name.equals("prism")) { 1312 this.getPrism().remove((PrismComponent) value); 1313 } else if (name.equals("add")) { 1314 this.add = null; 1315 } else if (name.equals("power")) { 1316 this.power = null; 1317 } else if (name.equals("backCurve")) { 1318 this.backCurve = null; 1319 } else if (name.equals("diameter")) { 1320 this.diameter = null; 1321 } else if (name.equals("duration")) { 1322 this.duration = null; 1323 } else if (name.equals("color")) { 1324 this.color = null; 1325 } else if (name.equals("brand")) { 1326 this.brand = null; 1327 } else if (name.equals("note")) { 1328 this.getNote().remove(value); 1329 } else 1330 super.removeChild(name, value); 1331 1332 } 1333 1334 @Override 1335 public Base makeProperty(int hash, String name) throws FHIRException { 1336 switch (hash) { 1337 case -309474065: return getProduct(); 1338 case 100913: return getEyeElement(); 1339 case -895981619: return getSphereElement(); 1340 case -349378602: return getCylinderElement(); 1341 case 3008417: return getAxisElement(); 1342 case 106935105: return addPrism(); 1343 case 96417: return getAddElement(); 1344 case 106858757: return getPowerElement(); 1345 case 1309344840: return getBackCurveElement(); 1346 case -233204595: return getDiameterElement(); 1347 case -1992012396: return getDuration(); 1348 case 94842723: return getColorElement(); 1349 case 93997959: return getBrandElement(); 1350 case 3387378: return addNote(); 1351 default: return super.makeProperty(hash, name); 1352 } 1353 1354 } 1355 1356 @Override 1357 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1358 switch (hash) { 1359 case -309474065: /*product*/ return new String[] {"CodeableConcept"}; 1360 case 100913: /*eye*/ return new String[] {"code"}; 1361 case -895981619: /*sphere*/ return new String[] {"decimal"}; 1362 case -349378602: /*cylinder*/ return new String[] {"decimal"}; 1363 case 3008417: /*axis*/ return new String[] {"integer"}; 1364 case 106935105: /*prism*/ return new String[] {}; 1365 case 96417: /*add*/ return new String[] {"decimal"}; 1366 case 106858757: /*power*/ return new String[] {"decimal"}; 1367 case 1309344840: /*backCurve*/ return new String[] {"decimal"}; 1368 case -233204595: /*diameter*/ return new String[] {"decimal"}; 1369 case -1992012396: /*duration*/ return new String[] {"Quantity"}; 1370 case 94842723: /*color*/ return new String[] {"string"}; 1371 case 93997959: /*brand*/ return new String[] {"string"}; 1372 case 3387378: /*note*/ return new String[] {"Annotation"}; 1373 default: return super.getTypesForProperty(hash, name); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base addChild(String name) throws FHIRException { 1380 if (name.equals("product")) { 1381 this.product = new CodeableConcept(); 1382 return this.product; 1383 } 1384 else if (name.equals("eye")) { 1385 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.eye"); 1386 } 1387 else if (name.equals("sphere")) { 1388 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.sphere"); 1389 } 1390 else if (name.equals("cylinder")) { 1391 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.cylinder"); 1392 } 1393 else if (name.equals("axis")) { 1394 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.axis"); 1395 } 1396 else if (name.equals("prism")) { 1397 return addPrism(); 1398 } 1399 else if (name.equals("add")) { 1400 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.add"); 1401 } 1402 else if (name.equals("power")) { 1403 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.power"); 1404 } 1405 else if (name.equals("backCurve")) { 1406 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.backCurve"); 1407 } 1408 else if (name.equals("diameter")) { 1409 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.diameter"); 1410 } 1411 else if (name.equals("duration")) { 1412 this.duration = new Quantity(); 1413 return this.duration; 1414 } 1415 else if (name.equals("color")) { 1416 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.color"); 1417 } 1418 else if (name.equals("brand")) { 1419 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.brand"); 1420 } 1421 else if (name.equals("note")) { 1422 return addNote(); 1423 } 1424 else 1425 return super.addChild(name); 1426 } 1427 1428 public VisionPrescriptionLensSpecificationComponent copy() { 1429 VisionPrescriptionLensSpecificationComponent dst = new VisionPrescriptionLensSpecificationComponent(); 1430 copyValues(dst); 1431 return dst; 1432 } 1433 1434 public void copyValues(VisionPrescriptionLensSpecificationComponent dst) { 1435 super.copyValues(dst); 1436 dst.product = product == null ? null : product.copy(); 1437 dst.eye = eye == null ? null : eye.copy(); 1438 dst.sphere = sphere == null ? null : sphere.copy(); 1439 dst.cylinder = cylinder == null ? null : cylinder.copy(); 1440 dst.axis = axis == null ? null : axis.copy(); 1441 if (prism != null) { 1442 dst.prism = new ArrayList<PrismComponent>(); 1443 for (PrismComponent i : prism) 1444 dst.prism.add(i.copy()); 1445 }; 1446 dst.add = add == null ? null : add.copy(); 1447 dst.power = power == null ? null : power.copy(); 1448 dst.backCurve = backCurve == null ? null : backCurve.copy(); 1449 dst.diameter = diameter == null ? null : diameter.copy(); 1450 dst.duration = duration == null ? null : duration.copy(); 1451 dst.color = color == null ? null : color.copy(); 1452 dst.brand = brand == null ? null : brand.copy(); 1453 if (note != null) { 1454 dst.note = new ArrayList<Annotation>(); 1455 for (Annotation i : note) 1456 dst.note.add(i.copy()); 1457 }; 1458 } 1459 1460 @Override 1461 public boolean equalsDeep(Base other_) { 1462 if (!super.equalsDeep(other_)) 1463 return false; 1464 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1465 return false; 1466 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1467 return compareDeep(product, o.product, true) && compareDeep(eye, o.eye, true) && compareDeep(sphere, o.sphere, true) 1468 && compareDeep(cylinder, o.cylinder, true) && compareDeep(axis, o.axis, true) && compareDeep(prism, o.prism, true) 1469 && compareDeep(add, o.add, true) && compareDeep(power, o.power, true) && compareDeep(backCurve, o.backCurve, true) 1470 && compareDeep(diameter, o.diameter, true) && compareDeep(duration, o.duration, true) && compareDeep(color, o.color, true) 1471 && compareDeep(brand, o.brand, true) && compareDeep(note, o.note, true); 1472 } 1473 1474 @Override 1475 public boolean equalsShallow(Base other_) { 1476 if (!super.equalsShallow(other_)) 1477 return false; 1478 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1479 return false; 1480 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1481 return compareValues(eye, o.eye, true) && compareValues(sphere, o.sphere, true) && compareValues(cylinder, o.cylinder, true) 1482 && compareValues(axis, o.axis, true) && compareValues(add, o.add, true) && compareValues(power, o.power, true) 1483 && compareValues(backCurve, o.backCurve, true) && compareValues(diameter, o.diameter, true) && compareValues(color, o.color, true) 1484 && compareValues(brand, o.brand, true); 1485 } 1486 1487 public boolean isEmpty() { 1488 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(product, eye, sphere, cylinder 1489 , axis, prism, add, power, backCurve, diameter, duration, color, brand, note 1490 ); 1491 } 1492 1493 public String fhirType() { 1494 return "VisionPrescription.lensSpecification"; 1495 1496 } 1497 1498 } 1499 1500 @Block() 1501 public static class PrismComponent extends BackboneElement implements IBaseBackboneElement { 1502 /** 1503 * Amount of prism to compensate for eye alignment in fractional units. 1504 */ 1505 @Child(name = "amount", type = {DecimalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1506 @Description(shortDefinition="Amount of adjustment", formalDefinition="Amount of prism to compensate for eye alignment in fractional units." ) 1507 protected DecimalType amount; 1508 1509 /** 1510 * The relative base, or reference lens edge, for the prism. 1511 */ 1512 @Child(name = "base", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1513 @Description(shortDefinition="up | down | in | out", formalDefinition="The relative base, or reference lens edge, for the prism." ) 1514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-base-codes") 1515 protected Enumeration<VisionBase> base; 1516 1517 private static final long serialVersionUID = 1677247628L; 1518 1519 /** 1520 * Constructor 1521 */ 1522 public PrismComponent() { 1523 super(); 1524 } 1525 1526 /** 1527 * Constructor 1528 */ 1529 public PrismComponent(BigDecimal amount, VisionBase base) { 1530 super(); 1531 this.setAmount(amount); 1532 this.setBase(base); 1533 } 1534 1535 /** 1536 * @return {@link #amount} (Amount of prism to compensate for eye alignment in fractional units.). This is the underlying object with id, value and extensions. The accessor "getAmount" gives direct access to the value 1537 */ 1538 public DecimalType getAmountElement() { 1539 if (this.amount == null) 1540 if (Configuration.errorOnAutoCreate()) 1541 throw new Error("Attempt to auto-create PrismComponent.amount"); 1542 else if (Configuration.doAutoCreate()) 1543 this.amount = new DecimalType(); // bb 1544 return this.amount; 1545 } 1546 1547 public boolean hasAmountElement() { 1548 return this.amount != null && !this.amount.isEmpty(); 1549 } 1550 1551 public boolean hasAmount() { 1552 return this.amount != null && !this.amount.isEmpty(); 1553 } 1554 1555 /** 1556 * @param value {@link #amount} (Amount of prism to compensate for eye alignment in fractional units.). This is the underlying object with id, value and extensions. The accessor "getAmount" gives direct access to the value 1557 */ 1558 public PrismComponent setAmountElement(DecimalType value) { 1559 this.amount = value; 1560 return this; 1561 } 1562 1563 /** 1564 * @return Amount of prism to compensate for eye alignment in fractional units. 1565 */ 1566 public BigDecimal getAmount() { 1567 return this.amount == null ? null : this.amount.getValue(); 1568 } 1569 1570 /** 1571 * @param value Amount of prism to compensate for eye alignment in fractional units. 1572 */ 1573 public PrismComponent setAmount(BigDecimal value) { 1574 if (this.amount == null) 1575 this.amount = new DecimalType(); 1576 this.amount.setValue(value); 1577 return this; 1578 } 1579 1580 /** 1581 * @param value Amount of prism to compensate for eye alignment in fractional units. 1582 */ 1583 public PrismComponent setAmount(long value) { 1584 this.amount = new DecimalType(); 1585 this.amount.setValue(value); 1586 return this; 1587 } 1588 1589 /** 1590 * @param value Amount of prism to compensate for eye alignment in fractional units. 1591 */ 1592 public PrismComponent setAmount(double value) { 1593 this.amount = new DecimalType(); 1594 this.amount.setValue(value); 1595 return this; 1596 } 1597 1598 /** 1599 * @return {@link #base} (The relative base, or reference lens edge, for the prism.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 1600 */ 1601 public Enumeration<VisionBase> getBaseElement() { 1602 if (this.base == null) 1603 if (Configuration.errorOnAutoCreate()) 1604 throw new Error("Attempt to auto-create PrismComponent.base"); 1605 else if (Configuration.doAutoCreate()) 1606 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); // bb 1607 return this.base; 1608 } 1609 1610 public boolean hasBaseElement() { 1611 return this.base != null && !this.base.isEmpty(); 1612 } 1613 1614 public boolean hasBase() { 1615 return this.base != null && !this.base.isEmpty(); 1616 } 1617 1618 /** 1619 * @param value {@link #base} (The relative base, or reference lens edge, for the prism.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 1620 */ 1621 public PrismComponent setBaseElement(Enumeration<VisionBase> value) { 1622 this.base = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return The relative base, or reference lens edge, for the prism. 1628 */ 1629 public VisionBase getBase() { 1630 return this.base == null ? null : this.base.getValue(); 1631 } 1632 1633 /** 1634 * @param value The relative base, or reference lens edge, for the prism. 1635 */ 1636 public PrismComponent setBase(VisionBase value) { 1637 if (this.base == null) 1638 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); 1639 this.base.setValue(value); 1640 return this; 1641 } 1642 1643 protected void listChildren(List<Property> children) { 1644 super.listChildren(children); 1645 children.add(new Property("amount", "decimal", "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount)); 1646 children.add(new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base)); 1647 } 1648 1649 @Override 1650 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1651 switch (_hash) { 1652 case -1413853096: /*amount*/ return new Property("amount", "decimal", "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount); 1653 case 3016401: /*base*/ return new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base); 1654 default: return super.getNamedProperty(_hash, _name, _checkValid); 1655 } 1656 1657 } 1658 1659 @Override 1660 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1661 switch (hash) { 1662 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // DecimalType 1663 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // Enumeration<VisionBase> 1664 default: return super.getProperty(hash, name, checkValid); 1665 } 1666 1667 } 1668 1669 @Override 1670 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1671 switch (hash) { 1672 case -1413853096: // amount 1673 this.amount = TypeConvertor.castToDecimal(value); // DecimalType 1674 return value; 1675 case 3016401: // base 1676 value = new VisionBaseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1677 this.base = (Enumeration) value; // Enumeration<VisionBase> 1678 return value; 1679 default: return super.setProperty(hash, name, value); 1680 } 1681 1682 } 1683 1684 @Override 1685 public Base setProperty(String name, Base value) throws FHIRException { 1686 if (name.equals("amount")) { 1687 this.amount = TypeConvertor.castToDecimal(value); // DecimalType 1688 } else if (name.equals("base")) { 1689 value = new VisionBaseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1690 this.base = (Enumeration) value; // Enumeration<VisionBase> 1691 } else 1692 return super.setProperty(name, value); 1693 return value; 1694 } 1695 1696 @Override 1697 public void removeChild(String name, Base value) throws FHIRException { 1698 if (name.equals("amount")) { 1699 this.amount = null; 1700 } else if (name.equals("base")) { 1701 value = new VisionBaseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1702 this.base = (Enumeration) value; // Enumeration<VisionBase> 1703 } else 1704 super.removeChild(name, value); 1705 1706 } 1707 1708 @Override 1709 public Base makeProperty(int hash, String name) throws FHIRException { 1710 switch (hash) { 1711 case -1413853096: return getAmountElement(); 1712 case 3016401: return getBaseElement(); 1713 default: return super.makeProperty(hash, name); 1714 } 1715 1716 } 1717 1718 @Override 1719 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1720 switch (hash) { 1721 case -1413853096: /*amount*/ return new String[] {"decimal"}; 1722 case 3016401: /*base*/ return new String[] {"code"}; 1723 default: return super.getTypesForProperty(hash, name); 1724 } 1725 1726 } 1727 1728 @Override 1729 public Base addChild(String name) throws FHIRException { 1730 if (name.equals("amount")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.prism.amount"); 1732 } 1733 else if (name.equals("base")) { 1734 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.lensSpecification.prism.base"); 1735 } 1736 else 1737 return super.addChild(name); 1738 } 1739 1740 public PrismComponent copy() { 1741 PrismComponent dst = new PrismComponent(); 1742 copyValues(dst); 1743 return dst; 1744 } 1745 1746 public void copyValues(PrismComponent dst) { 1747 super.copyValues(dst); 1748 dst.amount = amount == null ? null : amount.copy(); 1749 dst.base = base == null ? null : base.copy(); 1750 } 1751 1752 @Override 1753 public boolean equalsDeep(Base other_) { 1754 if (!super.equalsDeep(other_)) 1755 return false; 1756 if (!(other_ instanceof PrismComponent)) 1757 return false; 1758 PrismComponent o = (PrismComponent) other_; 1759 return compareDeep(amount, o.amount, true) && compareDeep(base, o.base, true); 1760 } 1761 1762 @Override 1763 public boolean equalsShallow(Base other_) { 1764 if (!super.equalsShallow(other_)) 1765 return false; 1766 if (!(other_ instanceof PrismComponent)) 1767 return false; 1768 PrismComponent o = (PrismComponent) other_; 1769 return compareValues(amount, o.amount, true) && compareValues(base, o.base, true); 1770 } 1771 1772 public boolean isEmpty() { 1773 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, base); 1774 } 1775 1776 public String fhirType() { 1777 return "VisionPrescription.lensSpecification.prism"; 1778 1779 } 1780 1781 } 1782 1783 /** 1784 * A unique identifier assigned to this vision prescription. 1785 */ 1786 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1787 @Description(shortDefinition="Business Identifier for vision prescription", formalDefinition="A unique identifier assigned to this vision prescription." ) 1788 protected List<Identifier> identifier; 1789 1790 /** 1791 * The status of the resource instance. 1792 */ 1793 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1794 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1796 protected Enumeration<FinancialResourceStatusCodes> status; 1797 1798 /** 1799 * The date this resource was created. 1800 */ 1801 @Child(name = "created", type = {DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1802 @Description(shortDefinition="Response creation date", formalDefinition="The date this resource was created." ) 1803 protected DateTimeType created; 1804 1805 /** 1806 * A resource reference to the person to whom the vision prescription applies. 1807 */ 1808 @Child(name = "patient", type = {Patient.class}, order=3, min=1, max=1, modifier=false, summary=true) 1809 @Description(shortDefinition="Who prescription is for", formalDefinition="A resource reference to the person to whom the vision prescription applies." ) 1810 protected Reference patient; 1811 1812 /** 1813 * A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued. 1814 */ 1815 @Child(name = "encounter", type = {Encounter.class}, order=4, min=0, max=1, modifier=false, summary=false) 1816 @Description(shortDefinition="Created during encounter / admission / stay", formalDefinition="A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued." ) 1817 protected Reference encounter; 1818 1819 /** 1820 * The date (and perhaps time) when the prescription was written. 1821 */ 1822 @Child(name = "dateWritten", type = {DateTimeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 1823 @Description(shortDefinition="When prescription was authorized", formalDefinition="The date (and perhaps time) when the prescription was written." ) 1824 protected DateTimeType dateWritten; 1825 1826 /** 1827 * The healthcare professional responsible for authorizing the prescription. 1828 */ 1829 @Child(name = "prescriber", type = {Practitioner.class, PractitionerRole.class}, order=6, min=1, max=1, modifier=false, summary=true) 1830 @Description(shortDefinition="Who authorized the vision prescription", formalDefinition="The healthcare professional responsible for authorizing the prescription." ) 1831 protected Reference prescriber; 1832 1833 /** 1834 * Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals. 1835 */ 1836 @Child(name = "lensSpecification", type = {}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1837 @Description(shortDefinition="Vision lens authorization", formalDefinition="Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals." ) 1838 protected List<VisionPrescriptionLensSpecificationComponent> lensSpecification; 1839 1840 private static final long serialVersionUID = -194296688L; 1841 1842 /** 1843 * Constructor 1844 */ 1845 public VisionPrescription() { 1846 super(); 1847 } 1848 1849 /** 1850 * Constructor 1851 */ 1852 public VisionPrescription(FinancialResourceStatusCodes status, Date created, Reference patient, Date dateWritten, Reference prescriber, VisionPrescriptionLensSpecificationComponent lensSpecification) { 1853 super(); 1854 this.setStatus(status); 1855 this.setCreated(created); 1856 this.setPatient(patient); 1857 this.setDateWritten(dateWritten); 1858 this.setPrescriber(prescriber); 1859 this.addLensSpecification(lensSpecification); 1860 } 1861 1862 /** 1863 * @return {@link #identifier} (A unique identifier assigned to this vision prescription.) 1864 */ 1865 public List<Identifier> getIdentifier() { 1866 if (this.identifier == null) 1867 this.identifier = new ArrayList<Identifier>(); 1868 return this.identifier; 1869 } 1870 1871 /** 1872 * @return Returns a reference to <code>this</code> for easy method chaining 1873 */ 1874 public VisionPrescription setIdentifier(List<Identifier> theIdentifier) { 1875 this.identifier = theIdentifier; 1876 return this; 1877 } 1878 1879 public boolean hasIdentifier() { 1880 if (this.identifier == null) 1881 return false; 1882 for (Identifier item : this.identifier) 1883 if (!item.isEmpty()) 1884 return true; 1885 return false; 1886 } 1887 1888 public Identifier addIdentifier() { //3 1889 Identifier t = new Identifier(); 1890 if (this.identifier == null) 1891 this.identifier = new ArrayList<Identifier>(); 1892 this.identifier.add(t); 1893 return t; 1894 } 1895 1896 public VisionPrescription addIdentifier(Identifier t) { //3 1897 if (t == null) 1898 return this; 1899 if (this.identifier == null) 1900 this.identifier = new ArrayList<Identifier>(); 1901 this.identifier.add(t); 1902 return this; 1903 } 1904 1905 /** 1906 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1907 */ 1908 public Identifier getIdentifierFirstRep() { 1909 if (getIdentifier().isEmpty()) { 1910 addIdentifier(); 1911 } 1912 return getIdentifier().get(0); 1913 } 1914 1915 /** 1916 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1917 */ 1918 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 1919 if (this.status == null) 1920 if (Configuration.errorOnAutoCreate()) 1921 throw new Error("Attempt to auto-create VisionPrescription.status"); 1922 else if (Configuration.doAutoCreate()) 1923 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 1924 return this.status; 1925 } 1926 1927 public boolean hasStatusElement() { 1928 return this.status != null && !this.status.isEmpty(); 1929 } 1930 1931 public boolean hasStatus() { 1932 return this.status != null && !this.status.isEmpty(); 1933 } 1934 1935 /** 1936 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1937 */ 1938 public VisionPrescription setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 1939 this.status = value; 1940 return this; 1941 } 1942 1943 /** 1944 * @return The status of the resource instance. 1945 */ 1946 public FinancialResourceStatusCodes getStatus() { 1947 return this.status == null ? null : this.status.getValue(); 1948 } 1949 1950 /** 1951 * @param value The status of the resource instance. 1952 */ 1953 public VisionPrescription setStatus(FinancialResourceStatusCodes value) { 1954 if (this.status == null) 1955 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 1956 this.status.setValue(value); 1957 return this; 1958 } 1959 1960 /** 1961 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1962 */ 1963 public DateTimeType getCreatedElement() { 1964 if (this.created == null) 1965 if (Configuration.errorOnAutoCreate()) 1966 throw new Error("Attempt to auto-create VisionPrescription.created"); 1967 else if (Configuration.doAutoCreate()) 1968 this.created = new DateTimeType(); // bb 1969 return this.created; 1970 } 1971 1972 public boolean hasCreatedElement() { 1973 return this.created != null && !this.created.isEmpty(); 1974 } 1975 1976 public boolean hasCreated() { 1977 return this.created != null && !this.created.isEmpty(); 1978 } 1979 1980 /** 1981 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1982 */ 1983 public VisionPrescription setCreatedElement(DateTimeType value) { 1984 this.created = value; 1985 return this; 1986 } 1987 1988 /** 1989 * @return The date this resource was created. 1990 */ 1991 public Date getCreated() { 1992 return this.created == null ? null : this.created.getValue(); 1993 } 1994 1995 /** 1996 * @param value The date this resource was created. 1997 */ 1998 public VisionPrescription setCreated(Date value) { 1999 if (this.created == null) 2000 this.created = new DateTimeType(); 2001 this.created.setValue(value); 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #patient} (A resource reference to the person to whom the vision prescription applies.) 2007 */ 2008 public Reference getPatient() { 2009 if (this.patient == null) 2010 if (Configuration.errorOnAutoCreate()) 2011 throw new Error("Attempt to auto-create VisionPrescription.patient"); 2012 else if (Configuration.doAutoCreate()) 2013 this.patient = new Reference(); // cc 2014 return this.patient; 2015 } 2016 2017 public boolean hasPatient() { 2018 return this.patient != null && !this.patient.isEmpty(); 2019 } 2020 2021 /** 2022 * @param value {@link #patient} (A resource reference to the person to whom the vision prescription applies.) 2023 */ 2024 public VisionPrescription setPatient(Reference value) { 2025 this.patient = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return {@link #encounter} (A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.) 2031 */ 2032 public Reference getEncounter() { 2033 if (this.encounter == null) 2034 if (Configuration.errorOnAutoCreate()) 2035 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 2036 else if (Configuration.doAutoCreate()) 2037 this.encounter = new Reference(); // cc 2038 return this.encounter; 2039 } 2040 2041 public boolean hasEncounter() { 2042 return this.encounter != null && !this.encounter.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #encounter} (A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.) 2047 */ 2048 public VisionPrescription setEncounter(Reference value) { 2049 this.encounter = value; 2050 return this; 2051 } 2052 2053 /** 2054 * @return {@link #dateWritten} (The date (and perhaps time) when the prescription was written.). This is the underlying object with id, value and extensions. The accessor "getDateWritten" gives direct access to the value 2055 */ 2056 public DateTimeType getDateWrittenElement() { 2057 if (this.dateWritten == null) 2058 if (Configuration.errorOnAutoCreate()) 2059 throw new Error("Attempt to auto-create VisionPrescription.dateWritten"); 2060 else if (Configuration.doAutoCreate()) 2061 this.dateWritten = new DateTimeType(); // bb 2062 return this.dateWritten; 2063 } 2064 2065 public boolean hasDateWrittenElement() { 2066 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2067 } 2068 2069 public boolean hasDateWritten() { 2070 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2071 } 2072 2073 /** 2074 * @param value {@link #dateWritten} (The date (and perhaps time) when the prescription was written.). This is the underlying object with id, value and extensions. The accessor "getDateWritten" gives direct access to the value 2075 */ 2076 public VisionPrescription setDateWrittenElement(DateTimeType value) { 2077 this.dateWritten = value; 2078 return this; 2079 } 2080 2081 /** 2082 * @return The date (and perhaps time) when the prescription was written. 2083 */ 2084 public Date getDateWritten() { 2085 return this.dateWritten == null ? null : this.dateWritten.getValue(); 2086 } 2087 2088 /** 2089 * @param value The date (and perhaps time) when the prescription was written. 2090 */ 2091 public VisionPrescription setDateWritten(Date value) { 2092 if (this.dateWritten == null) 2093 this.dateWritten = new DateTimeType(); 2094 this.dateWritten.setValue(value); 2095 return this; 2096 } 2097 2098 /** 2099 * @return {@link #prescriber} (The healthcare professional responsible for authorizing the prescription.) 2100 */ 2101 public Reference getPrescriber() { 2102 if (this.prescriber == null) 2103 if (Configuration.errorOnAutoCreate()) 2104 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 2105 else if (Configuration.doAutoCreate()) 2106 this.prescriber = new Reference(); // cc 2107 return this.prescriber; 2108 } 2109 2110 public boolean hasPrescriber() { 2111 return this.prescriber != null && !this.prescriber.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #prescriber} (The healthcare professional responsible for authorizing the prescription.) 2116 */ 2117 public VisionPrescription setPrescriber(Reference value) { 2118 this.prescriber = value; 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #lensSpecification} (Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.) 2124 */ 2125 public List<VisionPrescriptionLensSpecificationComponent> getLensSpecification() { 2126 if (this.lensSpecification == null) 2127 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2128 return this.lensSpecification; 2129 } 2130 2131 /** 2132 * @return Returns a reference to <code>this</code> for easy method chaining 2133 */ 2134 public VisionPrescription setLensSpecification(List<VisionPrescriptionLensSpecificationComponent> theLensSpecification) { 2135 this.lensSpecification = theLensSpecification; 2136 return this; 2137 } 2138 2139 public boolean hasLensSpecification() { 2140 if (this.lensSpecification == null) 2141 return false; 2142 for (VisionPrescriptionLensSpecificationComponent item : this.lensSpecification) 2143 if (!item.isEmpty()) 2144 return true; 2145 return false; 2146 } 2147 2148 public VisionPrescriptionLensSpecificationComponent addLensSpecification() { //3 2149 VisionPrescriptionLensSpecificationComponent t = new VisionPrescriptionLensSpecificationComponent(); 2150 if (this.lensSpecification == null) 2151 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2152 this.lensSpecification.add(t); 2153 return t; 2154 } 2155 2156 public VisionPrescription addLensSpecification(VisionPrescriptionLensSpecificationComponent t) { //3 2157 if (t == null) 2158 return this; 2159 if (this.lensSpecification == null) 2160 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2161 this.lensSpecification.add(t); 2162 return this; 2163 } 2164 2165 /** 2166 * @return The first repetition of repeating field {@link #lensSpecification}, creating it if it does not already exist {3} 2167 */ 2168 public VisionPrescriptionLensSpecificationComponent getLensSpecificationFirstRep() { 2169 if (getLensSpecification().isEmpty()) { 2170 addLensSpecification(); 2171 } 2172 return getLensSpecification().get(0); 2173 } 2174 2175 protected void listChildren(List<Property> children) { 2176 super.listChildren(children); 2177 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this vision prescription.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2178 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2179 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 2180 children.add(new Property("patient", "Reference(Patient)", "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient)); 2181 children.add(new Property("encounter", "Reference(Encounter)", "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 0, 1, encounter)); 2182 children.add(new Property("dateWritten", "dateTime", "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten)); 2183 children.add(new Property("prescriber", "Reference(Practitioner|PractitionerRole)", "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber)); 2184 children.add(new Property("lensSpecification", "", "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 0, java.lang.Integer.MAX_VALUE, lensSpecification)); 2185 } 2186 2187 @Override 2188 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2189 switch (_hash) { 2190 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this vision prescription.", 0, java.lang.Integer.MAX_VALUE, identifier); 2191 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2192 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 2193 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient); 2194 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 0, 1, encounter); 2195 case -1496880759: /*dateWritten*/ return new Property("dateWritten", "dateTime", "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten); 2196 case 1430631077: /*prescriber*/ return new Property("prescriber", "Reference(Practitioner|PractitionerRole)", "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber); 2197 case -1767318363: /*lensSpecification*/ return new Property("lensSpecification", "", "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 0, java.lang.Integer.MAX_VALUE, lensSpecification); 2198 default: return super.getNamedProperty(_hash, _name, _checkValid); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2205 switch (hash) { 2206 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2207 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 2208 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2209 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2210 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2211 case -1496880759: /*dateWritten*/ return this.dateWritten == null ? new Base[0] : new Base[] {this.dateWritten}; // DateTimeType 2212 case 1430631077: /*prescriber*/ return this.prescriber == null ? new Base[0] : new Base[] {this.prescriber}; // Reference 2213 case -1767318363: /*lensSpecification*/ return this.lensSpecification == null ? new Base[0] : this.lensSpecification.toArray(new Base[this.lensSpecification.size()]); // VisionPrescriptionLensSpecificationComponent 2214 default: return super.getProperty(hash, name, checkValid); 2215 } 2216 2217 } 2218 2219 @Override 2220 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2221 switch (hash) { 2222 case -1618432855: // identifier 2223 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2224 return value; 2225 case -892481550: // status 2226 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2227 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2228 return value; 2229 case 1028554472: // created 2230 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2231 return value; 2232 case -791418107: // patient 2233 this.patient = TypeConvertor.castToReference(value); // Reference 2234 return value; 2235 case 1524132147: // encounter 2236 this.encounter = TypeConvertor.castToReference(value); // Reference 2237 return value; 2238 case -1496880759: // dateWritten 2239 this.dateWritten = TypeConvertor.castToDateTime(value); // DateTimeType 2240 return value; 2241 case 1430631077: // prescriber 2242 this.prescriber = TypeConvertor.castToReference(value); // Reference 2243 return value; 2244 case -1767318363: // lensSpecification 2245 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); // VisionPrescriptionLensSpecificationComponent 2246 return value; 2247 default: return super.setProperty(hash, name, value); 2248 } 2249 2250 } 2251 2252 @Override 2253 public Base setProperty(String name, Base value) throws FHIRException { 2254 if (name.equals("identifier")) { 2255 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2256 } else if (name.equals("status")) { 2257 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2258 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2259 } else if (name.equals("created")) { 2260 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2261 } else if (name.equals("patient")) { 2262 this.patient = TypeConvertor.castToReference(value); // Reference 2263 } else if (name.equals("encounter")) { 2264 this.encounter = TypeConvertor.castToReference(value); // Reference 2265 } else if (name.equals("dateWritten")) { 2266 this.dateWritten = TypeConvertor.castToDateTime(value); // DateTimeType 2267 } else if (name.equals("prescriber")) { 2268 this.prescriber = TypeConvertor.castToReference(value); // Reference 2269 } else if (name.equals("lensSpecification")) { 2270 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); 2271 } else 2272 return super.setProperty(name, value); 2273 return value; 2274 } 2275 2276 @Override 2277 public void removeChild(String name, Base value) throws FHIRException { 2278 if (name.equals("identifier")) { 2279 this.getIdentifier().remove(value); 2280 } else if (name.equals("status")) { 2281 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2282 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2283 } else if (name.equals("created")) { 2284 this.created = null; 2285 } else if (name.equals("patient")) { 2286 this.patient = null; 2287 } else if (name.equals("encounter")) { 2288 this.encounter = null; 2289 } else if (name.equals("dateWritten")) { 2290 this.dateWritten = null; 2291 } else if (name.equals("prescriber")) { 2292 this.prescriber = null; 2293 } else if (name.equals("lensSpecification")) { 2294 this.getLensSpecification().remove((VisionPrescriptionLensSpecificationComponent) value); 2295 } else 2296 super.removeChild(name, value); 2297 2298 } 2299 2300 @Override 2301 public Base makeProperty(int hash, String name) throws FHIRException { 2302 switch (hash) { 2303 case -1618432855: return addIdentifier(); 2304 case -892481550: return getStatusElement(); 2305 case 1028554472: return getCreatedElement(); 2306 case -791418107: return getPatient(); 2307 case 1524132147: return getEncounter(); 2308 case -1496880759: return getDateWrittenElement(); 2309 case 1430631077: return getPrescriber(); 2310 case -1767318363: return addLensSpecification(); 2311 default: return super.makeProperty(hash, name); 2312 } 2313 2314 } 2315 2316 @Override 2317 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2318 switch (hash) { 2319 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2320 case -892481550: /*status*/ return new String[] {"code"}; 2321 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2322 case -791418107: /*patient*/ return new String[] {"Reference"}; 2323 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2324 case -1496880759: /*dateWritten*/ return new String[] {"dateTime"}; 2325 case 1430631077: /*prescriber*/ return new String[] {"Reference"}; 2326 case -1767318363: /*lensSpecification*/ return new String[] {}; 2327 default: return super.getTypesForProperty(hash, name); 2328 } 2329 2330 } 2331 2332 @Override 2333 public Base addChild(String name) throws FHIRException { 2334 if (name.equals("identifier")) { 2335 return addIdentifier(); 2336 } 2337 else if (name.equals("status")) { 2338 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.status"); 2339 } 2340 else if (name.equals("created")) { 2341 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.created"); 2342 } 2343 else if (name.equals("patient")) { 2344 this.patient = new Reference(); 2345 return this.patient; 2346 } 2347 else if (name.equals("encounter")) { 2348 this.encounter = new Reference(); 2349 return this.encounter; 2350 } 2351 else if (name.equals("dateWritten")) { 2352 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.dateWritten"); 2353 } 2354 else if (name.equals("prescriber")) { 2355 this.prescriber = new Reference(); 2356 return this.prescriber; 2357 } 2358 else if (name.equals("lensSpecification")) { 2359 return addLensSpecification(); 2360 } 2361 else 2362 return super.addChild(name); 2363 } 2364 2365 public String fhirType() { 2366 return "VisionPrescription"; 2367 2368 } 2369 2370 public VisionPrescription copy() { 2371 VisionPrescription dst = new VisionPrescription(); 2372 copyValues(dst); 2373 return dst; 2374 } 2375 2376 public void copyValues(VisionPrescription dst) { 2377 super.copyValues(dst); 2378 if (identifier != null) { 2379 dst.identifier = new ArrayList<Identifier>(); 2380 for (Identifier i : identifier) 2381 dst.identifier.add(i.copy()); 2382 }; 2383 dst.status = status == null ? null : status.copy(); 2384 dst.created = created == null ? null : created.copy(); 2385 dst.patient = patient == null ? null : patient.copy(); 2386 dst.encounter = encounter == null ? null : encounter.copy(); 2387 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 2388 dst.prescriber = prescriber == null ? null : prescriber.copy(); 2389 if (lensSpecification != null) { 2390 dst.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2391 for (VisionPrescriptionLensSpecificationComponent i : lensSpecification) 2392 dst.lensSpecification.add(i.copy()); 2393 }; 2394 } 2395 2396 protected VisionPrescription typedCopy() { 2397 return copy(); 2398 } 2399 2400 @Override 2401 public boolean equalsDeep(Base other_) { 2402 if (!super.equalsDeep(other_)) 2403 return false; 2404 if (!(other_ instanceof VisionPrescription)) 2405 return false; 2406 VisionPrescription o = (VisionPrescription) other_; 2407 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(created, o.created, true) 2408 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) && compareDeep(dateWritten, o.dateWritten, true) 2409 && compareDeep(prescriber, o.prescriber, true) && compareDeep(lensSpecification, o.lensSpecification, true) 2410 ; 2411 } 2412 2413 @Override 2414 public boolean equalsShallow(Base other_) { 2415 if (!super.equalsShallow(other_)) 2416 return false; 2417 if (!(other_ instanceof VisionPrescription)) 2418 return false; 2419 VisionPrescription o = (VisionPrescription) other_; 2420 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(dateWritten, o.dateWritten, true) 2421 ; 2422 } 2423 2424 public boolean isEmpty() { 2425 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created 2426 , patient, encounter, dateWritten, prescriber, lensSpecification); 2427 } 2428 2429 @Override 2430 public ResourceType getResourceType() { 2431 return ResourceType.VisionPrescription; 2432 } 2433 2434 /** 2435 * Search parameter: <b>datewritten</b> 2436 * <p> 2437 * Description: <b>Return prescriptions written on this date</b><br> 2438 * Type: <b>date</b><br> 2439 * Path: <b>VisionPrescription.dateWritten</b><br> 2440 * </p> 2441 */ 2442 @SearchParamDefinition(name="datewritten", path="VisionPrescription.dateWritten", description="Return prescriptions written on this date", type="date" ) 2443 public static final String SP_DATEWRITTEN = "datewritten"; 2444 /** 2445 * <b>Fluent Client</b> search parameter constant for <b>datewritten</b> 2446 * <p> 2447 * Description: <b>Return prescriptions written on this date</b><br> 2448 * Type: <b>date</b><br> 2449 * Path: <b>VisionPrescription.dateWritten</b><br> 2450 * </p> 2451 */ 2452 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATEWRITTEN = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATEWRITTEN); 2453 2454 /** 2455 * Search parameter: <b>prescriber</b> 2456 * <p> 2457 * Description: <b>Who authorized the vision prescription</b><br> 2458 * Type: <b>reference</b><br> 2459 * Path: <b>VisionPrescription.prescriber</b><br> 2460 * </p> 2461 */ 2462 @SearchParamDefinition(name="prescriber", path="VisionPrescription.prescriber", description="Who authorized the vision prescription", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class, PractitionerRole.class } ) 2463 public static final String SP_PRESCRIBER = "prescriber"; 2464 /** 2465 * <b>Fluent Client</b> search parameter constant for <b>prescriber</b> 2466 * <p> 2467 * Description: <b>Who authorized the vision prescription</b><br> 2468 * Type: <b>reference</b><br> 2469 * Path: <b>VisionPrescription.prescriber</b><br> 2470 * </p> 2471 */ 2472 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRESCRIBER); 2473 2474/** 2475 * Constant for fluent queries to be used to add include statements. Specifies 2476 * the path value of "<b>VisionPrescription:prescriber</b>". 2477 */ 2478 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIBER = new ca.uhn.fhir.model.api.Include("VisionPrescription:prescriber").toLocked(); 2479 2480 /** 2481 * Search parameter: <b>status</b> 2482 * <p> 2483 * Description: <b>The status of the vision prescription</b><br> 2484 * Type: <b>token</b><br> 2485 * Path: <b>VisionPrescription.status</b><br> 2486 * </p> 2487 */ 2488 @SearchParamDefinition(name="status", path="VisionPrescription.status", description="The status of the vision prescription", type="token" ) 2489 public static final String SP_STATUS = "status"; 2490 /** 2491 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2492 * <p> 2493 * Description: <b>The status of the vision prescription</b><br> 2494 * Type: <b>token</b><br> 2495 * Path: <b>VisionPrescription.status</b><br> 2496 * </p> 2497 */ 2498 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2499 2500 /** 2501 * Search parameter: <b>encounter</b> 2502 * <p> 2503 * Description: <b>Multiple Resources: 2504 2505* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2506* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2507* [ChargeItem](chargeitem.html): Encounter associated with event 2508* [Claim](claim.html): Encounters associated with a billed line item 2509* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2510* [Communication](communication.html): The Encounter during which this Communication was created 2511* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2512* [Composition](composition.html): Context of the Composition 2513* [Condition](condition.html): The Encounter during which this Condition was created 2514* [DeviceRequest](devicerequest.html): Encounter during which request was created 2515* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2516* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2517* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2518* [Flag](flag.html): Alert relevant during encounter 2519* [ImagingStudy](imagingstudy.html): The context of the study 2520* [List](list.html): Context in which list created 2521* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2522* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2523* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2524* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2525* [Observation](observation.html): Encounter related to the observation 2526* [Procedure](procedure.html): The Encounter during which this Procedure was created 2527* [Provenance](provenance.html): Encounter related to the Provenance 2528* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2529* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2530* [RiskAssessment](riskassessment.html): Where was assessment performed? 2531* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2532* [Task](task.html): Search by encounter 2533* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2534</b><br> 2535 * Type: <b>reference</b><br> 2536 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2537 * </p> 2538 */ 2539 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2540 public static final String SP_ENCOUNTER = "encounter"; 2541 /** 2542 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2543 * <p> 2544 * Description: <b>Multiple Resources: 2545 2546* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2547* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2548* [ChargeItem](chargeitem.html): Encounter associated with event 2549* [Claim](claim.html): Encounters associated with a billed line item 2550* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2551* [Communication](communication.html): The Encounter during which this Communication was created 2552* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2553* [Composition](composition.html): Context of the Composition 2554* [Condition](condition.html): The Encounter during which this Condition was created 2555* [DeviceRequest](devicerequest.html): Encounter during which request was created 2556* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2557* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2558* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2559* [Flag](flag.html): Alert relevant during encounter 2560* [ImagingStudy](imagingstudy.html): The context of the study 2561* [List](list.html): Context in which list created 2562* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2563* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2564* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2565* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2566* [Observation](observation.html): Encounter related to the observation 2567* [Procedure](procedure.html): The Encounter during which this Procedure was created 2568* [Provenance](provenance.html): Encounter related to the Provenance 2569* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2570* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2571* [RiskAssessment](riskassessment.html): Where was assessment performed? 2572* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2573* [Task](task.html): Search by encounter 2574* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2575</b><br> 2576 * Type: <b>reference</b><br> 2577 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2578 * </p> 2579 */ 2580 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2581 2582/** 2583 * Constant for fluent queries to be used to add include statements. Specifies 2584 * the path value of "<b>VisionPrescription:encounter</b>". 2585 */ 2586 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("VisionPrescription:encounter").toLocked(); 2587 2588 /** 2589 * Search parameter: <b>identifier</b> 2590 * <p> 2591 * Description: <b>Multiple Resources: 2592 2593* [Account](account.html): Account number 2594* [AdverseEvent](adverseevent.html): Business identifier for the event 2595* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2596* [Appointment](appointment.html): An Identifier of the Appointment 2597* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2598* [Basic](basic.html): Business identifier 2599* [BodyStructure](bodystructure.html): Bodystructure identifier 2600* [CarePlan](careplan.html): External Ids for this plan 2601* [CareTeam](careteam.html): External Ids for this team 2602* [ChargeItem](chargeitem.html): Business Identifier for item 2603* [Claim](claim.html): The primary identifier of the financial resource 2604* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2605* [ClinicalImpression](clinicalimpression.html): Business identifier 2606* [Communication](communication.html): Unique identifier 2607* [CommunicationRequest](communicationrequest.html): Unique identifier 2608* [Composition](composition.html): Version-independent identifier for the Composition 2609* [Condition](condition.html): A unique identifier of the condition record 2610* [Consent](consent.html): Identifier for this record (external references) 2611* [Contract](contract.html): The identity of the contract 2612* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2613* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2614* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2615* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2616* [DeviceRequest](devicerequest.html): Business identifier for request/order 2617* [DeviceUsage](deviceusage.html): Search by identifier 2618* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2619* [DocumentReference](documentreference.html): Identifier of the attachment binary 2620* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2621* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2622* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2623* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2624* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2625* [Flag](flag.html): Business identifier 2626* [Goal](goal.html): External Ids for this goal 2627* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2628* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2629* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2630* [Immunization](immunization.html): Business identifier 2631* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2632* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2633* [Invoice](invoice.html): Business Identifier for item 2634* [List](list.html): Business identifier 2635* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2636* [Medication](medication.html): Returns medications with this external identifier 2637* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2638* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2639* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2640* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2641* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2642* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2643* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2644* [Observation](observation.html): The unique id for a particular observation 2645* [Person](person.html): A person Identifier 2646* [Procedure](procedure.html): A unique identifier for a procedure 2647* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2648* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2649* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2650* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2651* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2652* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2653* [Specimen](specimen.html): The unique identifier associated with the specimen 2654* [SupplyDelivery](supplydelivery.html): External identifier 2655* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2656* [Task](task.html): Search for a task instance by its business identifier 2657* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2658</b><br> 2659 * Type: <b>token</b><br> 2660 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2661 * </p> 2662 */ 2663 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2664 public static final String SP_IDENTIFIER = "identifier"; 2665 /** 2666 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2667 * <p> 2668 * Description: <b>Multiple Resources: 2669 2670* [Account](account.html): Account number 2671* [AdverseEvent](adverseevent.html): Business identifier for the event 2672* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2673* [Appointment](appointment.html): An Identifier of the Appointment 2674* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2675* [Basic](basic.html): Business identifier 2676* [BodyStructure](bodystructure.html): Bodystructure identifier 2677* [CarePlan](careplan.html): External Ids for this plan 2678* [CareTeam](careteam.html): External Ids for this team 2679* [ChargeItem](chargeitem.html): Business Identifier for item 2680* [Claim](claim.html): The primary identifier of the financial resource 2681* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2682* [ClinicalImpression](clinicalimpression.html): Business identifier 2683* [Communication](communication.html): Unique identifier 2684* [CommunicationRequest](communicationrequest.html): Unique identifier 2685* [Composition](composition.html): Version-independent identifier for the Composition 2686* [Condition](condition.html): A unique identifier of the condition record 2687* [Consent](consent.html): Identifier for this record (external references) 2688* [Contract](contract.html): The identity of the contract 2689* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2690* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2691* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2692* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2693* [DeviceRequest](devicerequest.html): Business identifier for request/order 2694* [DeviceUsage](deviceusage.html): Search by identifier 2695* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2696* [DocumentReference](documentreference.html): Identifier of the attachment binary 2697* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2698* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2699* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2700* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2701* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2702* [Flag](flag.html): Business identifier 2703* [Goal](goal.html): External Ids for this goal 2704* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2705* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2706* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2707* [Immunization](immunization.html): Business identifier 2708* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2709* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2710* [Invoice](invoice.html): Business Identifier for item 2711* [List](list.html): Business identifier 2712* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2713* [Medication](medication.html): Returns medications with this external identifier 2714* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2715* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2716* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2717* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2718* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2719* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2720* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2721* [Observation](observation.html): The unique id for a particular observation 2722* [Person](person.html): A person Identifier 2723* [Procedure](procedure.html): A unique identifier for a procedure 2724* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2725* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2726* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2727* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2728* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2729* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2730* [Specimen](specimen.html): The unique identifier associated with the specimen 2731* [SupplyDelivery](supplydelivery.html): External identifier 2732* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2733* [Task](task.html): Search for a task instance by its business identifier 2734* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2735</b><br> 2736 * Type: <b>token</b><br> 2737 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2738 * </p> 2739 */ 2740 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2741 2742 /** 2743 * Search parameter: <b>patient</b> 2744 * <p> 2745 * Description: <b>Multiple Resources: 2746 2747* [Account](account.html): The entity that caused the expenses 2748* [AdverseEvent](adverseevent.html): Subject impacted by event 2749* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2750* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2751* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2752* [AuditEvent](auditevent.html): Where the activity involved patient data 2753* [Basic](basic.html): Identifies the focus of this resource 2754* [BodyStructure](bodystructure.html): Who this is about 2755* [CarePlan](careplan.html): Who the care plan is for 2756* [CareTeam](careteam.html): Who care team is for 2757* [ChargeItem](chargeitem.html): Individual service was done for/to 2758* [Claim](claim.html): Patient receiving the products or services 2759* [ClaimResponse](claimresponse.html): The subject of care 2760* [ClinicalImpression](clinicalimpression.html): Patient assessed 2761* [Communication](communication.html): Focus of message 2762* [CommunicationRequest](communicationrequest.html): Focus of message 2763* [Composition](composition.html): Who and/or what the composition is about 2764* [Condition](condition.html): Who has the condition? 2765* [Consent](consent.html): Who the consent applies to 2766* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2767* [Coverage](coverage.html): Retrieve coverages for a patient 2768* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2769* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2770* [DetectedIssue](detectedissue.html): Associated patient 2771* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2772* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2773* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2774* [DocumentReference](documentreference.html): Who/what is the subject of the document 2775* [Encounter](encounter.html): The patient present at the encounter 2776* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2777* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2778* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2779* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2780* [Flag](flag.html): The identity of a subject to list flags for 2781* [Goal](goal.html): Who this goal is intended for 2782* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2783* [ImagingSelection](imagingselection.html): Who the study is about 2784* [ImagingStudy](imagingstudy.html): Who the study is about 2785* [Immunization](immunization.html): The patient for the vaccination record 2786* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2787* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2788* [Invoice](invoice.html): Recipient(s) of goods and services 2789* [List](list.html): If all resources have the same subject 2790* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2791* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2792* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2793* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2794* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2795* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2796* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2797* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2798* [Observation](observation.html): The subject that the observation is about (if patient) 2799* [Person](person.html): The Person links to this Patient 2800* [Procedure](procedure.html): Search by subject - a patient 2801* [Provenance](provenance.html): Where the activity involved patient data 2802* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2803* [RelatedPerson](relatedperson.html): The patient this related person is related to 2804* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2805* [ResearchSubject](researchsubject.html): Who or what is part of study 2806* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2807* [ServiceRequest](servicerequest.html): Search by subject - a patient 2808* [Specimen](specimen.html): The patient the specimen comes from 2809* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2810* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2811* [Task](task.html): Search by patient 2812* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2813</b><br> 2814 * Type: <b>reference</b><br> 2815 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2816 * </p> 2817 */ 2818 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2819 public static final String SP_PATIENT = "patient"; 2820 /** 2821 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2822 * <p> 2823 * Description: <b>Multiple Resources: 2824 2825* [Account](account.html): The entity that caused the expenses 2826* [AdverseEvent](adverseevent.html): Subject impacted by event 2827* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2828* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2829* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2830* [AuditEvent](auditevent.html): Where the activity involved patient data 2831* [Basic](basic.html): Identifies the focus of this resource 2832* [BodyStructure](bodystructure.html): Who this is about 2833* [CarePlan](careplan.html): Who the care plan is for 2834* [CareTeam](careteam.html): Who care team is for 2835* [ChargeItem](chargeitem.html): Individual service was done for/to 2836* [Claim](claim.html): Patient receiving the products or services 2837* [ClaimResponse](claimresponse.html): The subject of care 2838* [ClinicalImpression](clinicalimpression.html): Patient assessed 2839* [Communication](communication.html): Focus of message 2840* [CommunicationRequest](communicationrequest.html): Focus of message 2841* [Composition](composition.html): Who and/or what the composition is about 2842* [Condition](condition.html): Who has the condition? 2843* [Consent](consent.html): Who the consent applies to 2844* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2845* [Coverage](coverage.html): Retrieve coverages for a patient 2846* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2847* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2848* [DetectedIssue](detectedissue.html): Associated patient 2849* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2850* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2851* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2852* [DocumentReference](documentreference.html): Who/what is the subject of the document 2853* [Encounter](encounter.html): The patient present at the encounter 2854* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2855* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2856* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2857* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2858* [Flag](flag.html): The identity of a subject to list flags for 2859* [Goal](goal.html): Who this goal is intended for 2860* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2861* [ImagingSelection](imagingselection.html): Who the study is about 2862* [ImagingStudy](imagingstudy.html): Who the study is about 2863* [Immunization](immunization.html): The patient for the vaccination record 2864* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2865* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2866* [Invoice](invoice.html): Recipient(s) of goods and services 2867* [List](list.html): If all resources have the same subject 2868* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2869* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2870* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2871* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2872* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2873* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2874* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2875* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2876* [Observation](observation.html): The subject that the observation is about (if patient) 2877* [Person](person.html): The Person links to this Patient 2878* [Procedure](procedure.html): Search by subject - a patient 2879* [Provenance](provenance.html): Where the activity involved patient data 2880* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2881* [RelatedPerson](relatedperson.html): The patient this related person is related to 2882* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2883* [ResearchSubject](researchsubject.html): Who or what is part of study 2884* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2885* [ServiceRequest](servicerequest.html): Search by subject - a patient 2886* [Specimen](specimen.html): The patient the specimen comes from 2887* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2888* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2889* [Task](task.html): Search by patient 2890* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2891</b><br> 2892 * Type: <b>reference</b><br> 2893 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2894 * </p> 2895 */ 2896 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2897 2898/** 2899 * Constant for fluent queries to be used to add include statements. Specifies 2900 * the path value of "<b>VisionPrescription:patient</b>". 2901 */ 2902 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("VisionPrescription:patient").toLocked(); 2903 2904 2905} 2906