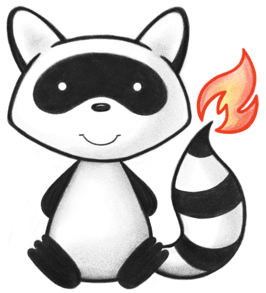
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.xhtml.NodeType; 039import org.hl7.fhir.utilities.xhtml.XhtmlComposer; 040import org.hl7.fhir.utilities.xhtml.XhtmlNode; 041 042 043public class XhtmlType extends PrimitiveType<String> { 044 045 private Narrative place; 046 047 public XhtmlType(Narrative place) { 048 super(); 049 this.place = place; 050 } 051 052 public XhtmlType() { 053 // "<div xmlns=\""+FormatUtilities.XHTML_NS+"\"></div>" 054 } 055 056 @Override 057 public String fhirType() { 058 return "xhtml"; 059 } 060 061 @Override 062 protected void listChildren(List<Property> result) { 063 } 064 065 @Override 066 public String getIdBase() { 067 return null; 068 } 069 070 @Override 071 public void setIdBase(String value) { 072 } 073 074 @Override 075 public PrimitiveType<String> copy() { 076 return null; 077 } 078 079 public String getValue() { 080 return primitiveValue(); 081 } 082 083 public XhtmlNode getXhtml() { 084 return place == null ? new XhtmlNode(NodeType.Element, "div") : place.getDiv(); 085 } 086 087 @Override 088 public Base setProperty(int hash, String name, Base value) throws FHIRException { 089 if ("value".equals(name)) { 090 if (value instanceof StringType) { 091 // div is already generated with getValue, we cannot just overwrite it 092 place.getDiv().setValueAsString(((StringType) value).asStringValue()); 093 } else { 094 place.setDiv(TypeConvertor.castToXhtml(value)); 095 } 096 return value; 097 } else 098 return super.setProperty(hash, name, value); 099 } 100 101 @Override 102 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 103 if ("value".equals(name)) 104 return new Base[] {this}; 105 return super.getProperty(hash, name, checkValid); 106 } 107 108 @Override 109 public String primitiveValue() { 110 try { 111 return new XhtmlComposer(false).compose(getXhtml()); 112 } catch (IOException e) { 113 } 114 return null; 115 } 116 117 @Override 118 public boolean isPrimitive() { 119 return true; 120 } 121 122 @Override 123 public boolean hasPrimitiveValue() { 124 return true; 125 } 126 127 @Override 128 protected String encode(String theValue) { 129 return theValue; 130 } 131 132 @Override 133 protected String parse(String theValue) { 134 return theValue; 135 } 136 137 public Narrative getPlace() { 138 return place; 139 } 140 141 public void setPlace(Narrative place) { 142 this.place = place; 143 } 144 145 @Override 146 public Base setXhtml(XhtmlNode node) { 147 return place.setDiv(node); 148 } 149 150 151}