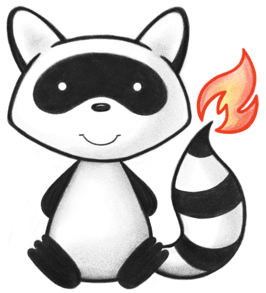
001package org.hl7.fhir.r5.openapi; 002 003import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032 */ 033 034 035 036import com.google.gson.JsonObject; 037 038@MarkedToMoveToAdjunctPackage 039public class ParameterWriter extends BaseWriter { 040 041 public enum ParameterLocation { 042 query, header, path, cookie; 043 } 044 public enum ParameterStyle { 045 matrix, label, form, simple, spaceDelimited, pipeDelimited, deepObject; 046 } 047 048 public ParameterWriter(JsonObject object) { 049 super(object); 050 } 051 052 public ParameterWriter in(ParameterLocation value) { 053 object.addProperty("in", value.toString()); 054 return this; 055 } 056 057 public ParameterWriter name(String value) { 058 object.addProperty("name", value); 059 return this; 060 } 061 062 public ParameterWriter allowEmptyValue() { 063 object.addProperty("allowEmptyValue", true); 064 return this; 065 } 066 067 public ParameterWriter description(String value) { 068 if (value != null) 069 object.addProperty("description", value); 070 return this; 071 } 072 073 public ParameterWriter required(boolean value) { 074 object.addProperty("required", value); 075 return this; 076 } 077 078 public ParameterWriter deprecated(boolean value) { 079 object.addProperty("deprecated", value); 080 return this; 081 } 082 083 public ParameterWriter allowEmptyValue(boolean value) { 084 object.addProperty("allowEmptyValue", value); 085 return this; 086 } 087 088 089 public ParameterWriter style(ParameterStyle value) { 090 object.addProperty("style", value.toString()); 091 return this; 092 } 093 094 095 public ParameterWriter explode(boolean value) { 096 object.addProperty("explode", value); 097 return this; 098 } 099 100 public ParameterWriter allowReserved(boolean value) { 101 object.addProperty("allowReserved", value); 102 return this; 103 } 104 105 106 public ParameterWriter schema(JsonObject jsonSchema) { 107 object.add("schema", jsonSchema); 108 return this; 109 } 110 111 public SchemaWriter schema() { 112 JsonObject so = new JsonObject(); 113 object.add("schema", so); 114 return new SchemaWriter(so); 115 } 116 117 public ParameterWriter schemaRef(String name, String uri) { 118 JsonObject schema = new JsonObject(); 119 schema.addProperty("$ref", uri); 120 object.add("schema", schema); 121 return this; 122 } 123 124 125 public ParameterWriter example(JsonObject example) { 126 object.add("example", example); 127 return this; 128 } 129 130}