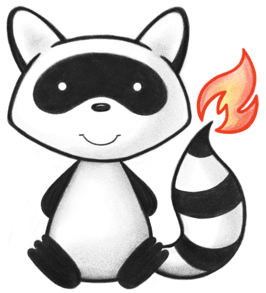
001package org.hl7.fhir.r5.openapi; 002 003import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 004 005import com.google.gson.JsonArray; 006import com.google.gson.JsonObject; 007 008@MarkedToMoveToAdjunctPackage 009public class SchemaWriter extends BaseWriter { 010 011 public enum SchemaType { 012 array, 013 bool, 014 dateTime, 015 number, 016 string; 017 018 public String toCode() { 019 switch (this) { 020 case array: return "array"; 021 case bool: return "boolean"; 022 case dateTime: return "date-time"; 023 case number: return "number"; 024 case string: return "string"; 025 } 026 return "??"; 027 } 028 } 029 030 public SchemaWriter(JsonObject object) { 031 super(object); 032 } 033 034 public SchemaWriter type(SchemaType value) { 035 if (value != null) { 036 if (value == SchemaType.dateTime) { 037 object.addProperty("type", "string"); 038 object.addProperty("pattern", "([0-9]([0-9]([0-9][1-9]|[1-9]0)|[1-9]00)|[1-9]000)(-(0[1-9]|1[0-2])(-(0[1-9]|[1-2][0-9]|3[0-1])(T([01][0-9]|2[0-3]):[0-5][0-9]:([0-5][0-9]|60)(\\.[0-9]+)?(Z|(\\+|-)((0[0-9]|1[0-3]):[0-5][0-9]|14:00)))?)?)?"); 039 040 } else 041 object.addProperty("type", value.toCode()); 042 } 043 return this; 044 } 045 046 public SchemaWriter items() { 047 JsonObject items = new JsonObject(); 048 object.add("items", items); 049 return new SchemaWriter(items); 050 } 051 052 public SchemaWriter enums(String... values) { 053 JsonArray arr = forceArray("enum"); 054 for (String s : values) 055 arr.add(s); 056 return this; 057 } 058 059 public SchemaWriter format(String value) { 060 if (value != null) 061 object.addProperty("format", value); 062 return this; 063 } 064 065}