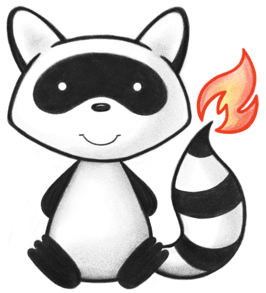
001package org.hl7.fhir.r5.openapi; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.io.InputStream; 036import java.io.OutputStream; 037import java.io.OutputStreamWriter; 038 039import org.hl7.fhir.utilities.FileUtilities; 040import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 041 042import com.google.gson.Gson; 043import com.google.gson.GsonBuilder; 044import com.google.gson.JsonObject; 045import com.google.gson.JsonParser; 046import com.google.gson.JsonSyntaxException; 047 048@MarkedToMoveToAdjunctPackage 049public class Writer extends BaseWriter { 050 051 private OutputStream stream; 052 053 public Writer(OutputStream stream) { 054 super( new JsonObject()); 055 this.stream = stream; 056 object.addProperty("openapi", "3.0.2"); 057 } 058 059 public Writer(OutputStream stream, InputStream template) throws JsonSyntaxException, IOException { 060 super(parse(template)); 061 this.stream = stream; 062 object.addProperty("openapi", "3.0.2"); 063 } 064 065 private static JsonObject parse(InputStream template) throws JsonSyntaxException, IOException { 066 JsonParser parser = new com.google.gson.JsonParser(); 067 return parser.parse(FileUtilities.streamToString(template)).getAsJsonObject(); 068 } 069 070 public void commit() throws IOException { 071 Gson gson = new GsonBuilder().setPrettyPrinting().create(); 072 String json = gson.toJson(object); 073 OutputStreamWriter sw = new OutputStreamWriter(stream, "UTF-8"); 074 sw.write('\ufeff'); // Unicode BOM, translates to UTF-8 with the configured outputstreamwriter 075 sw.write(json); 076 sw.flush(); 077 sw.close(); 078 } 079 080 public InfoWriter info() { 081 return new InfoWriter(ensureObject("info")); 082 } 083 084 public PathItemWriter path(String path) { 085 return new PathItemWriter(ensureMapObject("paths", path)); 086 } 087 088 public Writer pathRef(String path, String url) { 089 ensureMapObject("paths", path).addProperty("$ref", url); 090 return this; 091 } 092 093 094 public ServerWriter server(String url) { 095 return new ServerWriter(ensureArrayObject("servers", "url", url)); 096 } 097 098 099 public TagWriter tag(String name) { 100 return new TagWriter(ensureArrayObject("tags", "name", name)); 101 } 102 103 public ExternalDocsWriter externalDocs() { 104 return new ExternalDocsWriter(ensureObject("externalDocs")); 105 } 106 107 108 public ComponentsWriter components() { 109 return new ComponentsWriter(ensureObject("components")); 110 } 111 112}