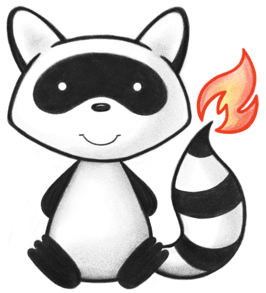
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.ActivityDefinition; 041import org.hl7.fhir.r5.model.BooleanType; 042import org.hl7.fhir.r5.model.CanonicalType; 043import org.hl7.fhir.r5.model.CodeableConcept; 044import org.hl7.fhir.r5.model.ContactDetail; 045import org.hl7.fhir.r5.model.DataType; 046import org.hl7.fhir.r5.model.DateTimeType; 047import org.hl7.fhir.r5.model.DateType; 048import org.hl7.fhir.r5.model.Enumeration; 049import org.hl7.fhir.r5.model.Enumerations.PublicationStatus; 050import org.hl7.fhir.r5.model.Identifier; 051import org.hl7.fhir.r5.model.MarkdownType; 052import org.hl7.fhir.r5.model.Period; 053import org.hl7.fhir.r5.model.Reference; 054import org.hl7.fhir.r5.model.StringType; 055import org.hl7.fhir.r5.model.UriType; 056import org.hl7.fhir.r5.model.UsageContext; 057 058public class ActivityDefinitionDefinitionImpl extends PatternBaseImpl implements Definition { 059 060 private ActivityDefinition wrapped; 061 062 public ActivityDefinitionDefinitionImpl(ActivityDefinition wrapped) { 063 super(wrapped); 064 this.wrapped = wrapped; 065 } 066 067 public int getUrlMin() { 068 return 0; 069 } 070 071 public int getUrlMax() { 072 return 1; 073 } 074 075 public UriType getUrlElement() throws FHIRException { 076 return wrapped.getUrlElement(); 077 } 078 079 080 public boolean hasUrlElement() { 081 return wrapped.hasUrlElement(); 082 } 083 084 085 public boolean hasUrl() { 086 return wrapped.hasUrl(); 087 } 088 089 090 public Definition setUrlElement(UriType value) throws FHIRException { 091 wrapped.setUrlElement(value); 092 return this; 093 094 } 095 096 097 public String getUrl() throws FHIRException { 098 return wrapped.getUrl(); 099 } 100 101 public Definition setUrl(String value) throws FHIRException { 102 wrapped.setUrl(value); 103 return this; 104 105} 106 107 public int getIdentifierMin() { 108 return 0; 109 } 110 111 public int getIdentifierMax() { 112 return 2147483647; 113 } 114 115 public Identifier getIdentifier() throws FHIRException { 116 return wrapped.getIdentifierFirstRep(); 117 } 118 119 public boolean hasIdentifier() { 120 return wrapped.hasIdentifier(); 121 } 122 123 public Definition setIdentifier(Identifier value) throws FHIRException { 124 wrapped.getIdentifier().clear(); 125 if (value != null) 126 wrapped.getIdentifier().add(value); 127 return this; 128 129 } 130 131 public int getVersionMin() { 132 return 0; 133 } 134 135 public int getVersionMax() { 136 return 1; 137 } 138 139 public StringType getVersionElement() throws FHIRException { 140 return wrapped.getVersionElement(); 141 } 142 143 144 public boolean hasVersionElement() { 145 return wrapped.hasVersionElement(); 146 } 147 148 149 public boolean hasVersion() { 150 return wrapped.hasVersion(); 151 } 152 153 154 public Definition setVersionElement(StringType value) throws FHIRException { 155 wrapped.setVersionElement(value); 156 return this; 157 158 } 159 160 161 public String getVersion() throws FHIRException { 162 return wrapped.getVersion(); 163 } 164 165 public Definition setVersion(String value) throws FHIRException { 166 wrapped.setVersion(value); 167 return this; 168 169} 170 171 public int getTitleMin() { 172 return 0; 173 } 174 175 public int getTitleMax() { 176 return 1; 177 } 178 179 public StringType getTitleElement() throws FHIRException { 180 return wrapped.getTitleElement(); 181 } 182 183 184 public boolean hasTitleElement() { 185 return wrapped.hasTitleElement(); 186 } 187 188 189 public boolean hasTitle() { 190 return wrapped.hasTitle(); 191 } 192 193 194 public Definition setTitleElement(StringType value) throws FHIRException { 195 wrapped.setTitleElement(value); 196 return this; 197 198 } 199 200 201 public String getTitle() throws FHIRException { 202 return wrapped.getTitle(); 203 } 204 205 public Definition setTitle(String value) throws FHIRException { 206 wrapped.setTitle(value); 207 return this; 208 209} 210 211 public int getDerivedFromCanonicalMin() { 212 return 0; 213 } 214 215 public int getDerivedFromCanonicalMax() { 216 return 0; 217 } 218 219 public List<CanonicalType> getDerivedFromCanonical() throws FHIRException { 220 throw new FHIRException("The pattern property 'derivedFromCanonical' is not supported in 'ActivityDefinition'"); 221 } 222 223 public Definition setDerivedFromCanonical(List<CanonicalType> theDerivedFromCanonical) throws FHIRException { 224 225 throw new FHIRException("The pattern property 'derivedFromCanonical' is not supported in 'ActivityDefinition'"); 226 } 227 228 public boolean hasDerivedFromCanonical() { 229 return false; 230 } 231 232 233 public CanonicalType addDerivedFromCanonicalElement() throws FHIRException { 234 throw new FHIRException("The pattern property 'derivedFromCanonical' is not supported in 'ActivityDefinition'"); 235 } 236 237 238 public Definition addDerivedFromCanonical(String value) throws FHIRException { 239 throw new FHIRException("The pattern property 'derivedFromCanonical' is not supported in 'ActivityDefinition'"); 240 } 241 242 243 public boolean hasDerivedFromCanonical(String value) { 244 return false; 245 } 246 247 248 public int getDerivedFromUriMin() { 249 return 0; 250 } 251 252 public int getDerivedFromUriMax() { 253 return 0; 254 } 255 256 public List<UriType> getDerivedFromUri() throws FHIRException { 257 throw new FHIRException("The pattern property 'derivedFromUri' is not supported in 'ActivityDefinition'"); 258 } 259 260 public Definition setDerivedFromUri(List<UriType> theDerivedFromUri) throws FHIRException { 261 262 throw new FHIRException("The pattern property 'derivedFromUri' is not supported in 'ActivityDefinition'"); 263 } 264 265 public boolean hasDerivedFromUri() { 266 return false; 267 } 268 269 270 public UriType addDerivedFromUriElement() throws FHIRException { 271 throw new FHIRException("The pattern property 'derivedFromUri' is not supported in 'ActivityDefinition'"); 272 } 273 274 275 public Definition addDerivedFromUri(String value) throws FHIRException { 276 throw new FHIRException("The pattern property 'derivedFromUri' is not supported in 'ActivityDefinition'"); 277 } 278 279 280 public boolean hasDerivedFromUri(String value) { 281 return false; 282 } 283 284 285 public int getPartOfMin() { 286 return 0; 287 } 288 289 public int getPartOfMax() { 290 return 0; 291 } 292 293 public List<CanonicalType> getPartOf() throws FHIRException { 294 throw new FHIRException("The pattern property 'partOf' is not supported in 'ActivityDefinition'"); 295 } 296 297 public Definition setPartOf(List<CanonicalType> thePartOf) throws FHIRException { 298 299 throw new FHIRException("The pattern property 'partOf' is not supported in 'ActivityDefinition'"); 300 } 301 302 public boolean hasPartOf() { 303 return false; 304 } 305 306 307 public CanonicalType addPartOfElement() throws FHIRException { 308 throw new FHIRException("The pattern property 'partOf' is not supported in 'ActivityDefinition'"); 309 } 310 311 312 public Definition addPartOf(String value) throws FHIRException { 313 throw new FHIRException("The pattern property 'partOf' is not supported in 'ActivityDefinition'"); 314 } 315 316 317 public boolean hasPartOf(String value) { 318 return false; 319 } 320 321 322 public int getReplacesMin() { 323 return 0; 324 } 325 326 public int getReplacesMax() { 327 return 0; 328 } 329 330 public List<CanonicalType> getReplaces() throws FHIRException { 331 throw new FHIRException("The pattern property 'replaces' is not supported in 'ActivityDefinition'"); 332 } 333 334 public Definition setReplaces(List<CanonicalType> theReplaces) throws FHIRException { 335 336 throw new FHIRException("The pattern property 'replaces' is not supported in 'ActivityDefinition'"); 337 } 338 339 public boolean hasReplaces() { 340 return false; 341 } 342 343 344 public CanonicalType addReplacesElement() throws FHIRException { 345 throw new FHIRException("The pattern property 'replaces' is not supported in 'ActivityDefinition'"); 346 } 347 348 349 public Definition addReplaces(String value) throws FHIRException { 350 throw new FHIRException("The pattern property 'replaces' is not supported in 'ActivityDefinition'"); 351 } 352 353 354 public boolean hasReplaces(String value) { 355 return false; 356 } 357 358 359 public int getStatusMin() { 360 return 0; 361 } 362 363 public int getStatusMax() { 364 return 0; 365 } 366 367 public Enumeration<PublicationStatus> getStatusElement() throws FHIRException { 368 throw new FHIRException("The pattern property 'status' is not supported in 'ActivityDefinition'"); 369 } 370 371 372 public boolean hasStatusElement() { 373 return false; 374 } 375 376 377 public boolean hasStatus() { 378 return false; 379 } 380 381 382 public Definition setStatusElement(Enumeration<PublicationStatus> value) throws FHIRException { 383 throw new FHIRException("The pattern property 'status' is not supported in 'ActivityDefinition'"); 384 } 385 386 387 public PublicationStatus getStatus() throws FHIRException { 388 throw new FHIRException("The pattern property 'status' is not supported in 'ActivityDefinition'"); 389 } 390 391 public Definition setStatus(PublicationStatus value) throws FHIRException { 392 throw new FHIRException("The pattern property 'status' is not supported in 'ActivityDefinition'"); 393} 394 395 public int getExperimentalMin() { 396 return 0; 397 } 398 399 public int getExperimentalMax() { 400 return 1; 401 } 402 403 public BooleanType getExperimentalElement() throws FHIRException { 404 return wrapped.getExperimentalElement(); 405 } 406 407 408 public boolean hasExperimentalElement() { 409 return wrapped.hasExperimentalElement(); 410 } 411 412 413 public boolean hasExperimental() { 414 return wrapped.hasExperimental(); 415 } 416 417 418 public Definition setExperimentalElement(BooleanType value) throws FHIRException { 419 wrapped.setExperimentalElement(value); 420 return this; 421 422 } 423 424 425 public boolean getExperimental() throws FHIRException { 426 return wrapped.getExperimental(); 427 } 428 429 public Definition setExperimental(boolean value) throws FHIRException { 430 wrapped.setExperimental(value); 431 return this; 432 433} 434 435 public int getSubjectMin() { 436 return 0; 437 } 438 439 public int getSubjectMax() { 440 return 0; 441 } 442 443 public DataType getSubject() throws FHIRException { 444 throw new FHIRException("The pattern property 'subject[x]' is not supported in 'ActivityDefinition'"); 445 } 446 447 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 448 throw new FHIRException("The pattern property 'subject[x]' is not supported in 'ActivityDefinition'"); 449 } 450 451 public boolean hasSubjectCodeableConcept() { 452 return false; 453 } 454 455 public Reference getSubjectReference() throws FHIRException { 456 throw new FHIRException("The pattern property 'subject[x]' is not supported in 'ActivityDefinition'"); 457 } 458 459 public boolean hasSubjectReference() { 460 return false; 461 } 462 463 public boolean hasSubject() { 464 return false; 465 } 466 467 public Definition setSubject(DataType value) throws FHIRException { 468 throw new FHIRException("The pattern property 'subject[x]' is not supported in 'ActivityDefinition'"); 469 } 470 471 public int getDateMin() { 472 return 0; 473 } 474 475 public int getDateMax() { 476 return 1; 477 } 478 479 public DateTimeType getDateElement() throws FHIRException { 480 return wrapped.getDateElement(); 481 } 482 483 484 public boolean hasDateElement() { 485 return wrapped.hasDateElement(); 486 } 487 488 489 public boolean hasDate() { 490 return wrapped.hasDate(); 491 } 492 493 494 public Definition setDateElement(DateTimeType value) throws FHIRException { 495 wrapped.setDateElement(value); 496 return this; 497 498 } 499 500 501 public Date getDate() throws FHIRException { 502 return wrapped.getDate(); 503 } 504 505 public Definition setDate(Date value) throws FHIRException { 506 wrapped.setDate(value); 507 return this; 508 509} 510 511 public int getPublisherMin() { 512 return 0; 513 } 514 515 public int getPublisherMax() { 516 return 0; 517 } 518 519 public Reference getPublisher() throws FHIRException { 520 throw new FHIRException("The pattern property 'publisher' is not supported in 'ActivityDefinition'"); 521 } 522 523 public boolean hasPublisher() { 524 return false; 525 } 526 527 public Definition setPublisher(Reference value) throws FHIRException { 528 throw new FHIRException("The pattern property 'publisher' is not supported in 'ActivityDefinition'"); 529 } 530 531 public int getContactMin() { 532 return 0; 533 } 534 535 public int getContactMax() { 536 return 2147483647; 537 } 538 539 public List<ContactDetail> getContact() throws FHIRException { 540 return wrapped.getContact(); 541 } 542 543 public Definition setContact(List<ContactDetail> theContact) throws FHIRException { 544 545 wrapped.setContact(theContact); 546 return this; 547 548 } 549 550 public boolean hasContact() { 551 return wrapped.hasContact(); 552 } 553 554 555 public ContactDetail addContact() throws FHIRException { 556 throw new FHIRException("The pattern property 'contact' is not supported in 'ActivityDefinition'"); 557 } 558 559 560 public Definition addContact(ContactDetail t) throws FHIRException { 561 throw new FHIRException("The pattern property 'contact' is not supported in 'ActivityDefinition'"); 562 } 563 564 565 public ContactDetail getContactFirstRep() throws FHIRException { 566 567 return wrapped.getContactFirstRep(); 568 } 569 570 public int getDescriptionMin() { 571 return 0; 572 } 573 574 public int getDescriptionMax() { 575 return 1; 576 } 577 578 public MarkdownType getDescriptionElement() throws FHIRException { 579 return wrapped.getDescriptionElement(); 580 } 581 582 583 public boolean hasDescriptionElement() { 584 return wrapped.hasDescriptionElement(); 585 } 586 587 588 public boolean hasDescription() { 589 return wrapped.hasDescription(); 590 } 591 592 593 public Definition setDescriptionElement(MarkdownType value) throws FHIRException { 594 wrapped.setDescriptionElement(value); 595 return this; 596 597 } 598 599 600 public String getDescription() throws FHIRException { 601 return wrapped.getDescription(); 602 } 603 604 public Definition setDescription(String value) throws FHIRException { 605 wrapped.setDescription(value); 606 return this; 607 608} 609 610 public int getUseContextMin() { 611 return 0; 612 } 613 614 public int getUseContextMax() { 615 return 2147483647; 616 } 617 618 public List<UsageContext> getUseContext() throws FHIRException { 619 return wrapped.getUseContext(); 620 } 621 622 public Definition setUseContext(List<UsageContext> theUseContext) throws FHIRException { 623 624 wrapped.setUseContext(theUseContext); 625 return this; 626 627 } 628 629 public boolean hasUseContext() { 630 return wrapped.hasUseContext(); 631 } 632 633 634 public UsageContext addUseContext() throws FHIRException { 635 throw new FHIRException("The pattern property 'useContext' is not supported in 'ActivityDefinition'"); 636 } 637 638 639 public Definition addUseContext(UsageContext t) throws FHIRException { 640 throw new FHIRException("The pattern property 'useContext' is not supported in 'ActivityDefinition'"); 641 } 642 643 644 public UsageContext getUseContextFirstRep() throws FHIRException { 645 646 return wrapped.getUseContextFirstRep(); 647 } 648 649 public int getJurisdictionMin() { 650 return 0; 651 } 652 653 public int getJurisdictionMax() { 654 return 2147483647; 655 } 656 657 public List<CodeableConcept> getJurisdiction() throws FHIRException { 658 return wrapped.getJurisdiction(); 659 } 660 661 public Definition setJurisdiction(List<CodeableConcept> theJurisdiction) throws FHIRException { 662 663 wrapped.setJurisdiction(theJurisdiction); 664 return this; 665 666 } 667 668 public boolean hasJurisdiction() { 669 return wrapped.hasJurisdiction(); 670 } 671 672 673 public CodeableConcept addJurisdiction() throws FHIRException { 674 throw new FHIRException("The pattern property 'jurisdiction' is not supported in 'ActivityDefinition'"); 675 } 676 677 678 public Definition addJurisdiction(CodeableConcept t) throws FHIRException { 679 throw new FHIRException("The pattern property 'jurisdiction' is not supported in 'ActivityDefinition'"); 680 } 681 682 683 public CodeableConcept getJurisdictionFirstRep() throws FHIRException { 684 685 return wrapped.getJurisdictionFirstRep(); 686 } 687 688 public int getPurposeMin() { 689 return 0; 690 } 691 692 public int getPurposeMax() { 693 return 1; 694 } 695 696 public MarkdownType getPurposeElement() throws FHIRException { 697 return wrapped.getPurposeElement(); 698 } 699 700 701 public boolean hasPurposeElement() { 702 return wrapped.hasPurposeElement(); 703 } 704 705 706 public boolean hasPurpose() { 707 return wrapped.hasPurpose(); 708 } 709 710 711 public Definition setPurposeElement(MarkdownType value) throws FHIRException { 712 wrapped.setPurposeElement(value); 713 return this; 714 715 } 716 717 718 public String getPurpose() throws FHIRException { 719 return wrapped.getPurpose(); 720 } 721 722 public Definition setPurpose(String value) throws FHIRException { 723 wrapped.setPurpose(value); 724 return this; 725 726} 727 728 public int getCopyrightMin() { 729 return 0; 730 } 731 732 public int getCopyrightMax() { 733 return 1; 734 } 735 736 public MarkdownType getCopyrightElement() throws FHIRException { 737 return wrapped.getCopyrightElement(); 738 } 739 740 741 public boolean hasCopyrightElement() { 742 return wrapped.hasCopyrightElement(); 743 } 744 745 746 public boolean hasCopyright() { 747 return wrapped.hasCopyright(); 748 } 749 750 751 public Definition setCopyrightElement(MarkdownType value) throws FHIRException { 752 wrapped.setCopyrightElement(value); 753 return this; 754 755 } 756 757 758 public String getCopyright() throws FHIRException { 759 return wrapped.getCopyright(); 760 } 761 762 public Definition setCopyright(String value) throws FHIRException { 763 wrapped.setCopyright(value); 764 return this; 765 766} 767 768 public int getApprovalDateMin() { 769 return 0; 770 } 771 772 public int getApprovalDateMax() { 773 return 1; 774 } 775 776 public DateType getApprovalDateElement() throws FHIRException { 777 return wrapped.getApprovalDateElement(); 778 } 779 780 781 public boolean hasApprovalDateElement() { 782 return wrapped.hasApprovalDateElement(); 783 } 784 785 786 public boolean hasApprovalDate() { 787 return wrapped.hasApprovalDate(); 788 } 789 790 791 public Definition setApprovalDateElement(DateType value) throws FHIRException { 792 wrapped.setApprovalDateElement(value); 793 return this; 794 795 } 796 797 798 public Date getApprovalDate() throws FHIRException { 799 return wrapped.getApprovalDate(); 800 } 801 802 public Definition setApprovalDate(Date value) throws FHIRException { 803 wrapped.setApprovalDate(value); 804 return this; 805 806} 807 808 public int getLastReviewDateMin() { 809 return 0; 810 } 811 812 public int getLastReviewDateMax() { 813 return 1; 814 } 815 816 public DateType getLastReviewDateElement() throws FHIRException { 817 return wrapped.getLastReviewDateElement(); 818 } 819 820 821 public boolean hasLastReviewDateElement() { 822 return wrapped.hasLastReviewDateElement(); 823 } 824 825 826 public boolean hasLastReviewDate() { 827 return wrapped.hasLastReviewDate(); 828 } 829 830 831 public Definition setLastReviewDateElement(DateType value) throws FHIRException { 832 wrapped.setLastReviewDateElement(value); 833 return this; 834 835 } 836 837 838 public Date getLastReviewDate() throws FHIRException { 839 return wrapped.getLastReviewDate(); 840 } 841 842 public Definition setLastReviewDate(Date value) throws FHIRException { 843 wrapped.setLastReviewDate(value); 844 return this; 845 846} 847 848 public int getEffectivePeriodMin() { 849 return 0; 850 } 851 852 public int getEffectivePeriodMax() { 853 return 1; 854 } 855 856 public Period getEffectivePeriod() throws FHIRException { 857 return wrapped.getEffectivePeriod(); 858 } 859 860 public boolean hasEffectivePeriod() { 861 return wrapped.hasEffectivePeriod(); 862 } 863 864 public Definition setEffectivePeriod(Period value) throws FHIRException { 865 wrapped.setEffectivePeriod(value); 866 return this; 867 868 } 869 870 public int getPerformerTypeMin() { 871 return 0; 872 } 873 874 public int getPerformerTypeMax() { 875 return 0; 876 } 877 878 public CodeableConcept getPerformerType() throws FHIRException { 879 throw new FHIRException("The pattern property 'performerType' is not supported in 'ActivityDefinition'"); 880 } 881 882 public boolean hasPerformerType() { 883 return false; 884 } 885 886 public Definition setPerformerType(CodeableConcept value) throws FHIRException { 887 throw new FHIRException("The pattern property 'performerType' is not supported in 'ActivityDefinition'"); 888 } 889 890 public String fhirType() { 891 return "ActivityDefinition"; 892} 893 894 895}