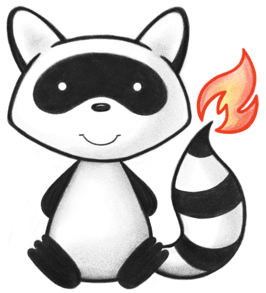
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006import java.util.Date; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039 040// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 041import org.hl7.fhir.r5.model.BooleanType; 042import org.hl7.fhir.r5.model.CanonicalType; 043import org.hl7.fhir.r5.model.CodeableConcept; 044import org.hl7.fhir.r5.model.ContactDetail; 045import org.hl7.fhir.r5.model.DataType; 046import org.hl7.fhir.r5.model.DateTimeType; 047import org.hl7.fhir.r5.model.DateType; 048import org.hl7.fhir.r5.model.Enumeration; 049import org.hl7.fhir.r5.model.Enumerations.PublicationStatus; 050import org.hl7.fhir.r5.model.Identifier; 051import org.hl7.fhir.r5.model.MarkdownType; 052import org.hl7.fhir.r5.model.Period; 053import org.hl7.fhir.r5.model.Reference; 054import org.hl7.fhir.r5.model.StringType; 055import org.hl7.fhir.r5.model.UriType; 056import org.hl7.fhir.r5.model.UsageContext; 057/** 058 * A pattern to be followed by resources that represent a specific proposal, plan and/or order for some sort of action or service. 059 */ 060public interface Definition extends PatternBase { 061 062 /** 063 * @return {@link #url} (An absolute URL that is used to identify this {{title}} when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this {{title}} is (or will be) published. The URL SHOULD include the major version of the {{title}}. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 064 */ 065 public UriType getUrlElement() throws FHIRException; 066 067 /** 068 * @return whether there is more than zero values for url 069 */ 070 public boolean hasUrl(); 071 /** 072 * @return minimum allowed cardinality for url. Note that with patterns, this may be different for the underlying resource 073 */ 074 public int getUrlMin() throws FHIRException; 075 /** 076 * @return maximum allowed cardinality for url. Note that with patterns, this may be different for the underlying resource 077 */ 078 public int getUrlMax() throws FHIRException; 079 public boolean hasUrlElement(); 080 081 /** 082 * @param value {@link #url} (An absolute URL that is used to identify this {{title}} when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this {{title}} is (or will be) published. The URL SHOULD include the major version of the {{title}}. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 083 */ 084 public Definition setUrlElement(UriType value) throws FHIRException; 085 086 /** 087 * @return An absolute URL that is used to identify this {{title}} when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this {{title}} is (or will be) published. The URL SHOULD include the major version of the {{title}}. For more information see [Technical and Business Versions](resource.html#versions). 088 */ 089 public String getUrl() throws FHIRException; 090 091 /** 092 * @param value An absolute URL that is used to identify this {{title}} when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this {{title}} is (or will be) published. The URL SHOULD include the major version of the {{title}}. For more information see [Technical and Business Versions](resource.html#versions). 093 */ 094 public Definition setUrl(String value) throws FHIRException; 095 096 /** 097 * @return {@link #identifier} (Business identifiers assigned to this {{title}} by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 098 */ 099 public Identifier getIdentifier() throws FHIRException ; 100 101 /** 102 * @return whether there is more than zero values for identifier 103 */ 104 public boolean hasIdentifier(); 105 /** 106 * @return minimum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 107 */ 108 public int getIdentifierMin(); 109 /** 110 * @return maximum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 111 */ 112 public int getIdentifierMax(); 113 /** 114 * @param value {@link #identifier} (Business identifiers assigned to this {{title}} by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 115 */ 116 public Definition setIdentifier(Identifier value) throws FHIRException; 117 118 /** 119 * @return {@link #version} (The identifier that is used to identify this version of the {{title}} when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the {{title}} author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 120 */ 121 public StringType getVersionElement() throws FHIRException; 122 123 /** 124 * @return whether there is more than zero values for version 125 */ 126 public boolean hasVersion(); 127 /** 128 * @return minimum allowed cardinality for version. Note that with patterns, this may be different for the underlying resource 129 */ 130 public int getVersionMin() throws FHIRException; 131 /** 132 * @return maximum allowed cardinality for version. Note that with patterns, this may be different for the underlying resource 133 */ 134 public int getVersionMax() throws FHIRException; 135 public boolean hasVersionElement(); 136 137 /** 138 * @param value {@link #version} (The identifier that is used to identify this version of the {{title}} when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the {{title}} author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 139 */ 140 public Definition setVersionElement(StringType value) throws FHIRException; 141 142 /** 143 * @return The identifier that is used to identify this version of the {{title}} when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the {{title}} author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 144 */ 145 public String getVersion() throws FHIRException; 146 147 /** 148 * @param value The identifier that is used to identify this version of the {{title}} when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the {{title}} author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 149 */ 150 public Definition setVersion(String value) throws FHIRException; 151 152 /** 153 * @return {@link #title} (A short, descriptive, user-friendly title for the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 154 */ 155 public StringType getTitleElement() throws FHIRException; 156 157 /** 158 * @return whether there is more than zero values for title 159 */ 160 public boolean hasTitle(); 161 /** 162 * @return minimum allowed cardinality for title. Note that with patterns, this may be different for the underlying resource 163 */ 164 public int getTitleMin() throws FHIRException; 165 /** 166 * @return maximum allowed cardinality for title. Note that with patterns, this may be different for the underlying resource 167 */ 168 public int getTitleMax() throws FHIRException; 169 public boolean hasTitleElement(); 170 171 /** 172 * @param value {@link #title} (A short, descriptive, user-friendly title for the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 173 */ 174 public Definition setTitleElement(StringType value) throws FHIRException; 175 176 /** 177 * @return A short, descriptive, user-friendly title for the {{title}}. 178 */ 179 public String getTitle() throws FHIRException; 180 181 /** 182 * @param value A short, descriptive, user-friendly title for the {{title}}. 183 */ 184 public Definition setTitle(String value) throws FHIRException; 185 186 /** 187 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 188 */ 189 public List<CanonicalType> getDerivedFromCanonical() throws FHIRException; 190 191 /** 192 * @return Returns a reference to <code>this</code> for easy method chaining 193 */ 194 public Definition setDerivedFromCanonical(List<CanonicalType> theDerivedFromCanonical) throws FHIRException; 195 196 /** 197 * @return whether there is more than zero values for derivedFromCanonical 198 */ 199 public boolean hasDerivedFromCanonical(); 200 /** 201 * @return minimum allowed cardinality for derivedFromCanonical. Note that with patterns, this may be different for the underlying resource 202 */ 203 public int getDerivedFromCanonicalMin(); 204 /** 205 * @return maximum allowed cardinality for derivedFromCanonical. Note that with patterns, this may be different for the underlying resource 206 */ 207 public int getDerivedFromCanonicalMax(); 208 209 /** 210 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 211 */ 212 public CanonicalType addDerivedFromCanonicalElement() throws FHIRException; 213 214 /** 215 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 216 */ 217 public Definition addDerivedFromCanonical(String value) throws FHIRException; 218 219 /** 220 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 221 */ 222 public boolean hasDerivedFromCanonical(String value) throws FHIRException; 223 224 /** 225 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 226 */ 227 public List<UriType> getDerivedFromUri() throws FHIRException; 228 229 /** 230 * @return Returns a reference to <code>this</code> for easy method chaining 231 */ 232 public Definition setDerivedFromUri(List<UriType> theDerivedFromUri) throws FHIRException; 233 234 /** 235 * @return whether there is more than zero values for derivedFromUri 236 */ 237 public boolean hasDerivedFromUri(); 238 /** 239 * @return minimum allowed cardinality for derivedFromUri. Note that with patterns, this may be different for the underlying resource 240 */ 241 public int getDerivedFromUriMin(); 242 /** 243 * @return maximum allowed cardinality for derivedFromUri. Note that with patterns, this may be different for the underlying resource 244 */ 245 public int getDerivedFromUriMax(); 246 247 /** 248 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 249 */ 250 public UriType addDerivedFromUriElement() throws FHIRException; 251 252 /** 253 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 254 */ 255 public Definition addDerivedFromUri(String value) throws FHIRException; 256 257 /** 258 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this definition.) 259 */ 260 public boolean hasDerivedFromUri(String value) throws FHIRException; 261 262 /** 263 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 264 */ 265 public List<CanonicalType> getPartOf() throws FHIRException; 266 267 /** 268 * @return Returns a reference to <code>this</code> for easy method chaining 269 */ 270 public Definition setPartOf(List<CanonicalType> thePartOf) throws FHIRException; 271 272 /** 273 * @return whether there is more than zero values for partOf 274 */ 275 public boolean hasPartOf(); 276 /** 277 * @return minimum allowed cardinality for partOf. Note that with patterns, this may be different for the underlying resource 278 */ 279 public int getPartOfMin(); 280 /** 281 * @return maximum allowed cardinality for partOf. Note that with patterns, this may be different for the underlying resource 282 */ 283 public int getPartOfMax(); 284 285 /** 286 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 287 */ 288 public CanonicalType addPartOfElement() throws FHIRException; 289 290 /** 291 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 292 */ 293 public Definition addPartOf(String value) throws FHIRException; 294 295 /** 296 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 297 */ 298 public boolean hasPartOf(String value) throws FHIRException; 299 300 /** 301 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 302 */ 303 public List<CanonicalType> getReplaces() throws FHIRException; 304 305 /** 306 * @return Returns a reference to <code>this</code> for easy method chaining 307 */ 308 public Definition setReplaces(List<CanonicalType> theReplaces) throws FHIRException; 309 310 /** 311 * @return whether there is more than zero values for replaces 312 */ 313 public boolean hasReplaces(); 314 /** 315 * @return minimum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 316 */ 317 public int getReplacesMin(); 318 /** 319 * @return maximum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 320 */ 321 public int getReplacesMax(); 322 323 /** 324 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 325 */ 326 public CanonicalType addReplacesElement() throws FHIRException; 327 328 /** 329 * @param value {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 330 */ 331 public Definition addReplaces(String value) throws FHIRException; 332 333 /** 334 * @param value {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 335 */ 336 public boolean hasReplaces(String value) throws FHIRException; 337 338 /** 339 * @return {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 340 */ 341 public Enumeration<PublicationStatus> getStatusElement() throws FHIRException; 342 343 /** 344 * @return whether there is more than zero values for status 345 */ 346 public boolean hasStatus(); 347 /** 348 * @return minimum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 349 */ 350 public int getStatusMin() throws FHIRException; 351 /** 352 * @return maximum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 353 */ 354 public int getStatusMax() throws FHIRException; 355 public boolean hasStatusElement(); 356 357 /** 358 * @param value {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 359 */ 360 public Definition setStatusElement(Enumeration<PublicationStatus> value) throws FHIRException; 361 362 /** 363 * @return The current state of the {{title}}. 364 */ 365 public PublicationStatus getStatus() throws FHIRException; 366 367 /** 368 * @param value The current state of the {{title}}. 369 */ 370 public Definition setStatus(PublicationStatus value) throws FHIRException; 371 372 /** 373 * @return {@link #experimental} (A flag to indicate that this {{title}} is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 374 */ 375 public BooleanType getExperimentalElement() throws FHIRException; 376 377 /** 378 * @return whether there is more than zero values for experimental 379 */ 380 public boolean hasExperimental(); 381 /** 382 * @return minimum allowed cardinality for experimental. Note that with patterns, this may be different for the underlying resource 383 */ 384 public int getExperimentalMin() throws FHIRException; 385 /** 386 * @return maximum allowed cardinality for experimental. Note that with patterns, this may be different for the underlying resource 387 */ 388 public int getExperimentalMax() throws FHIRException; 389 public boolean hasExperimentalElement(); 390 391 /** 392 * @param value {@link #experimental} (A flag to indicate that this {{title}} is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 393 */ 394 public Definition setExperimentalElement(BooleanType value) throws FHIRException; 395 396 /** 397 * @return A flag to indicate that this {{title}} is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 398 */ 399 public boolean getExperimental() throws FHIRException; 400 401 /** 402 * @param value A flag to indicate that this {{title}} is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 403 */ 404 public Definition setExperimental(boolean value) throws FHIRException; 405 406 /** 407 * @return {@link #subject} (A code or group definition that describes the intended subject of instantiations of this definition.) 408 */ 409 public DataType getSubject() throws FHIRException ; 410 411 /** 412 * @return {@link #subject} (A code or group definition that describes the intended subject of instantiations of this definition.) 413 */ 414 public CodeableConcept getSubjectCodeableConcept() throws FHIRException; 415 416 public boolean hasSubjectCodeableConcept(); 417 418 /** 419 * @return {@link #subject} (A code or group definition that describes the intended subject of instantiations of this definition.) 420 */ 421 public Reference getSubjectReference() throws FHIRException; 422 423 public boolean hasSubjectReference(); 424 425 /** 426 * @return whether there is more than zero values for subject 427 */ 428 public boolean hasSubject(); 429 /** 430 * @return minimum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 431 */ 432 public int getSubjectMin(); 433 /** 434 * @return maximum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 435 */ 436 public int getSubjectMax(); 437 /** 438 * @param value {@link #subject} (A code or group definition that describes the intended subject of instantiations of this definition.) 439 */ 440 public Definition setSubject(DataType value) throws FHIRException; 441 442 /** 443 * @return {@link #date} (For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 444 */ 445 public DateTimeType getDateElement() throws FHIRException; 446 447 /** 448 * @return whether there is more than zero values for date 449 */ 450 public boolean hasDate(); 451 /** 452 * @return minimum allowed cardinality for date. Note that with patterns, this may be different for the underlying resource 453 */ 454 public int getDateMin() throws FHIRException; 455 /** 456 * @return maximum allowed cardinality for date. Note that with patterns, this may be different for the underlying resource 457 */ 458 public int getDateMax() throws FHIRException; 459 public boolean hasDateElement(); 460 461 /** 462 * @param value {@link #date} (For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 463 */ 464 public Definition setDateElement(DateTimeType value) throws FHIRException; 465 466 /** 467 * @return For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal. 468 */ 469 public Date getDate() throws FHIRException; 470 471 /** 472 * @param value For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal. 473 */ 474 public Definition setDate(Date value) throws FHIRException; 475 476 /** 477 * @return {@link #publisher} (Helps establish the "authority/credibility" of the {{title}}. May also allow for contact.) 478 */ 479 public Reference getPublisher() throws FHIRException ; 480 481 /** 482 * @return whether there is more than zero values for publisher 483 */ 484 public boolean hasPublisher(); 485 /** 486 * @return minimum allowed cardinality for publisher. Note that with patterns, this may be different for the underlying resource 487 */ 488 public int getPublisherMin(); 489 /** 490 * @return maximum allowed cardinality for publisher. Note that with patterns, this may be different for the underlying resource 491 */ 492 public int getPublisherMax(); 493 /** 494 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the {{title}}. May also allow for contact.) 495 */ 496 public Definition setPublisher(Reference value) throws FHIRException; 497 498 /** 499 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 500 */ 501 public List<ContactDetail> getContact() throws FHIRException; 502 503 /** 504 * @return Returns a reference to <code>this</code> for easy method chaining 505 */ 506 public Definition setContact(List<ContactDetail> theContact) throws FHIRException; 507 508 /** 509 * @return whether there is more than zero values for contact 510 */ 511 public boolean hasContact(); 512 /** 513 * @return minimum allowed cardinality for contact. Note that with patterns, this may be different for the underlying resource 514 */ 515 public int getContactMin(); 516 /** 517 * @return maximum allowed cardinality for contact. Note that with patterns, this may be different for the underlying resource 518 */ 519 public int getContactMax(); 520 521 public ContactDetail addContact() throws FHIRException; 522 523 public Definition addContact(ContactDetail t) throws FHIRException; 524 525 /** 526 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 527 */ 528 public ContactDetail getContactFirstRep() throws FHIRException; 529 530 /** 531 * @return {@link #description} (A free text natural language description of the {{title}} from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 532 */ 533 public MarkdownType getDescriptionElement() throws FHIRException; 534 535 /** 536 * @return whether there is more than zero values for description 537 */ 538 public boolean hasDescription(); 539 /** 540 * @return minimum allowed cardinality for description. Note that with patterns, this may be different for the underlying resource 541 */ 542 public int getDescriptionMin() throws FHIRException; 543 /** 544 * @return maximum allowed cardinality for description. Note that with patterns, this may be different for the underlying resource 545 */ 546 public int getDescriptionMax() throws FHIRException; 547 public boolean hasDescriptionElement(); 548 549 /** 550 * @param value {@link #description} (A free text natural language description of the {{title}} from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 551 */ 552 public Definition setDescriptionElement(MarkdownType value) throws FHIRException; 553 554 /** 555 * @return A free text natural language description of the {{title}} from the consumer's perspective. 556 */ 557 public String getDescription() throws FHIRException; 558 559 /** 560 * @param value A free text natural language description of the {{title}} from the consumer's perspective. 561 */ 562 public Definition setDescription(String value) throws FHIRException; 563 564 /** 565 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.) 566 */ 567 public List<UsageContext> getUseContext() throws FHIRException; 568 569 /** 570 * @return Returns a reference to <code>this</code> for easy method chaining 571 */ 572 public Definition setUseContext(List<UsageContext> theUseContext) throws FHIRException; 573 574 /** 575 * @return whether there is more than zero values for useContext 576 */ 577 public boolean hasUseContext(); 578 /** 579 * @return minimum allowed cardinality for useContext. Note that with patterns, this may be different for the underlying resource 580 */ 581 public int getUseContextMin(); 582 /** 583 * @return maximum allowed cardinality for useContext. Note that with patterns, this may be different for the underlying resource 584 */ 585 public int getUseContextMax(); 586 587 public UsageContext addUseContext() throws FHIRException; 588 589 public Definition addUseContext(UsageContext t) throws FHIRException; 590 591 /** 592 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 593 */ 594 public UsageContext getUseContextFirstRep() throws FHIRException; 595 596 /** 597 * @return {@link #jurisdiction} (A jurisdiction in which the {{title}} is intended to be used.) 598 */ 599 public List<CodeableConcept> getJurisdiction() throws FHIRException; 600 601 /** 602 * @return Returns a reference to <code>this</code> for easy method chaining 603 */ 604 public Definition setJurisdiction(List<CodeableConcept> theJurisdiction) throws FHIRException; 605 606 /** 607 * @return whether there is more than zero values for jurisdiction 608 */ 609 public boolean hasJurisdiction(); 610 /** 611 * @return minimum allowed cardinality for jurisdiction. Note that with patterns, this may be different for the underlying resource 612 */ 613 public int getJurisdictionMin(); 614 /** 615 * @return maximum allowed cardinality for jurisdiction. Note that with patterns, this may be different for the underlying resource 616 */ 617 public int getJurisdictionMax(); 618 619 public CodeableConcept addJurisdiction() throws FHIRException; 620 621 public Definition addJurisdiction(CodeableConcept t) throws FHIRException; 622 623 /** 624 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 625 */ 626 public CodeableConcept getJurisdictionFirstRep() throws FHIRException; 627 628 /** 629 * @return {@link #purpose} (Explains why this {{title}} is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 630 */ 631 public MarkdownType getPurposeElement() throws FHIRException; 632 633 /** 634 * @return whether there is more than zero values for purpose 635 */ 636 public boolean hasPurpose(); 637 /** 638 * @return minimum allowed cardinality for purpose. Note that with patterns, this may be different for the underlying resource 639 */ 640 public int getPurposeMin() throws FHIRException; 641 /** 642 * @return maximum allowed cardinality for purpose. Note that with patterns, this may be different for the underlying resource 643 */ 644 public int getPurposeMax() throws FHIRException; 645 public boolean hasPurposeElement(); 646 647 /** 648 * @param value {@link #purpose} (Explains why this {{title}} is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 649 */ 650 public Definition setPurposeElement(MarkdownType value) throws FHIRException; 651 652 /** 653 * @return Explains why this {{title}} is needed and why it has been designed as it has. 654 */ 655 public String getPurpose() throws FHIRException; 656 657 /** 658 * @param value Explains why this {{title}} is needed and why it has been designed as it has. 659 */ 660 public Definition setPurpose(String value) throws FHIRException; 661 662 /** 663 * @return {@link #copyright} (A copyright statement relating to the {{title}} and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 664 */ 665 public MarkdownType getCopyrightElement() throws FHIRException; 666 667 /** 668 * @return whether there is more than zero values for copyright 669 */ 670 public boolean hasCopyright(); 671 /** 672 * @return minimum allowed cardinality for copyright. Note that with patterns, this may be different for the underlying resource 673 */ 674 public int getCopyrightMin() throws FHIRException; 675 /** 676 * @return maximum allowed cardinality for copyright. Note that with patterns, this may be different for the underlying resource 677 */ 678 public int getCopyrightMax() throws FHIRException; 679 public boolean hasCopyrightElement(); 680 681 /** 682 * @param value {@link #copyright} (A copyright statement relating to the {{title}} and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 683 */ 684 public Definition setCopyrightElement(MarkdownType value) throws FHIRException; 685 686 /** 687 * @return A copyright statement relating to the {{title}} and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the {{title}}. 688 */ 689 public String getCopyright() throws FHIRException; 690 691 /** 692 * @param value A copyright statement relating to the {{title}} and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the {{title}}. 693 */ 694 public Definition setCopyright(String value) throws FHIRException; 695 696 /** 697 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 698 */ 699 public DateType getApprovalDateElement() throws FHIRException; 700 701 /** 702 * @return whether there is more than zero values for approvalDate 703 */ 704 public boolean hasApprovalDate(); 705 /** 706 * @return minimum allowed cardinality for approvalDate. Note that with patterns, this may be different for the underlying resource 707 */ 708 public int getApprovalDateMin() throws FHIRException; 709 /** 710 * @return maximum allowed cardinality for approvalDate. Note that with patterns, this may be different for the underlying resource 711 */ 712 public int getApprovalDateMax() throws FHIRException; 713 public boolean hasApprovalDateElement(); 714 715 /** 716 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 717 */ 718 public Definition setApprovalDateElement(DateType value) throws FHIRException; 719 720 /** 721 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 722 */ 723 public Date getApprovalDate() throws FHIRException; 724 725 /** 726 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 727 */ 728 public Definition setApprovalDate(Date value) throws FHIRException; 729 730 /** 731 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 732 */ 733 public DateType getLastReviewDateElement() throws FHIRException; 734 735 /** 736 * @return whether there is more than zero values for lastReviewDate 737 */ 738 public boolean hasLastReviewDate(); 739 /** 740 * @return minimum allowed cardinality for lastReviewDate. Note that with patterns, this may be different for the underlying resource 741 */ 742 public int getLastReviewDateMin() throws FHIRException; 743 /** 744 * @return maximum allowed cardinality for lastReviewDate. Note that with patterns, this may be different for the underlying resource 745 */ 746 public int getLastReviewDateMax() throws FHIRException; 747 public boolean hasLastReviewDateElement(); 748 749 /** 750 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 751 */ 752 public Definition setLastReviewDateElement(DateType value) throws FHIRException; 753 754 /** 755 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 756 */ 757 public Date getLastReviewDate() throws FHIRException; 758 759 /** 760 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 761 */ 762 public Definition setLastReviewDate(Date value) throws FHIRException; 763 764 /** 765 * @return {@link #effectivePeriod} (The period during which the {{title}} content was or is planned to be effective.) 766 */ 767 public Period getEffectivePeriod() throws FHIRException ; 768 769 /** 770 * @return whether there is more than zero values for effectivePeriod 771 */ 772 public boolean hasEffectivePeriod(); 773 /** 774 * @return minimum allowed cardinality for effectivePeriod. Note that with patterns, this may be different for the underlying resource 775 */ 776 public int getEffectivePeriodMin(); 777 /** 778 * @return maximum allowed cardinality for effectivePeriod. Note that with patterns, this may be different for the underlying resource 779 */ 780 public int getEffectivePeriodMax(); 781 /** 782 * @param value {@link #effectivePeriod} (The period during which the {{title}} content was or is planned to be effective.) 783 */ 784 public Definition setEffectivePeriod(Period value) throws FHIRException; 785 786 /** 787 * @return {@link #performerType} (The type of individual that is expected to act upon instances of this definition.) 788 */ 789 public CodeableConcept getPerformerType() throws FHIRException ; 790 791 /** 792 * @return whether there is more than zero values for performerType 793 */ 794 public boolean hasPerformerType(); 795 /** 796 * @return minimum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 797 */ 798 public int getPerformerTypeMin(); 799 /** 800 * @return maximum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 801 */ 802 public int getPerformerTypeMax(); 803 /** 804 * @param value {@link #performerType} (The type of individual that is expected to act upon instances of this definition.) 805 */ 806 public Definition setPerformerType(CodeableConcept value) throws FHIRException; 807 808 public String fhirType(); 809 810 811}