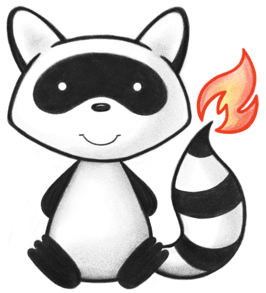
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006import java.util.Date; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039 040// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 041import org.hl7.fhir.r5.model.Annotation; 042import org.hl7.fhir.r5.model.Base; 043import org.hl7.fhir.r5.model.BooleanType; 044import org.hl7.fhir.r5.model.CanonicalType; 045import org.hl7.fhir.r5.model.CodeableConcept; 046import org.hl7.fhir.r5.model.Configuration; 047import org.hl7.fhir.r5.model.DataType; 048import org.hl7.fhir.r5.model.DateTimeType; 049import org.hl7.fhir.r5.model.EnumFactory; 050import org.hl7.fhir.r5.model.Enumeration; 051import org.hl7.fhir.r5.model.Identifier; 052import org.hl7.fhir.r5.model.Period; 053import org.hl7.fhir.r5.model.PrimitiveType; 054import org.hl7.fhir.r5.model.Reference; 055import org.hl7.fhir.r5.model.Timing; 056import org.hl7.fhir.r5.model.UriType; 057/** 058 * A pattern to be followed by resources that represent the performance of some activity, possibly in accordance with a request or service definition. 059 */ 060public interface Event extends PatternBase { 061 062 public enum EventStatus { 063 /** 064 * The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes. 065 */ 066 PREPARATION, 067 /** 068 * The event is currently occurring. 069 */ 070 INPROGRESS, 071 /** 072 * The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific. 073 */ 074 NOTDONE, 075 /** 076 * The event has been temporarily stopped but is expected to resume in the future. 077 */ 078 ONHOLD, 079 /** 080 * The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred. 081 */ 082 STOPPED, 083 /** 084 * The event has now concluded. 085 */ 086 COMPLETED, 087 /** 088 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".). 089 */ 090 ENTEREDINERROR, 091 /** 092 * The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 093 */ 094 UNKNOWN, 095 /** 096 * added to help the parsers with the generic types 097 */ 098 NULL; 099 public static EventStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("preparation".equals(codeString)) 103 return PREPARATION; 104 if ("in-progress".equals(codeString)) 105 return INPROGRESS; 106 if ("not-done".equals(codeString)) 107 return NOTDONE; 108 if ("on-hold".equals(codeString)) 109 return ONHOLD; 110 if ("stopped".equals(codeString)) 111 return STOPPED; 112 if ("completed".equals(codeString)) 113 return COMPLETED; 114 if ("entered-in-error".equals(codeString)) 115 return ENTEREDINERROR; 116 if ("unknown".equals(codeString)) 117 return UNKNOWN; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown EventStatus code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case PREPARATION: return "preparation"; 126 case INPROGRESS: return "in-progress"; 127 case NOTDONE: return "not-done"; 128 case ONHOLD: return "on-hold"; 129 case STOPPED: return "stopped"; 130 case COMPLETED: return "completed"; 131 case ENTEREDINERROR: return "entered-in-error"; 132 case UNKNOWN: return "unknown"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getSystem() { 138 switch (this) { 139 case PREPARATION: return "http://hl7.org/fhir/event-status"; 140 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 141 case NOTDONE: return "http://hl7.org/fhir/event-status"; 142 case ONHOLD: return "http://hl7.org/fhir/event-status"; 143 case STOPPED: return "http://hl7.org/fhir/event-status"; 144 case COMPLETED: return "http://hl7.org/fhir/event-status"; 145 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 146 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 147 case NULL: return null; 148 default: return "?"; 149 } 150 } 151 public String getDefinition() { 152 switch (this) { 153 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 154 case INPROGRESS: return "The event is currently occurring."; 155 case NOTDONE: return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 156 case ONHOLD: return "The event has been temporarily stopped but is expected to resume in the future."; 157 case STOPPED: return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 158 case COMPLETED: return "The event has now concluded."; 159 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 160 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 161 case NULL: return null; 162 default: return "?"; 163 } 164 } 165 public String getDisplay() { 166 switch (this) { 167 case PREPARATION: return "Preparation"; 168 case INPROGRESS: return "In Progress"; 169 case NOTDONE: return "Not Done"; 170 case ONHOLD: return "On Hold"; 171 case STOPPED: return "Stopped"; 172 case COMPLETED: return "Completed"; 173 case ENTEREDINERROR: return "Entered in Error"; 174 case UNKNOWN: return "Unknown"; 175 case NULL: return null; 176 default: return "?"; 177 } 178 } 179 } 180 181 public class EventStatusEnumFactory implements EnumFactory<EventStatus> { 182 public EventStatus fromCode(String codeString) throws IllegalArgumentException { 183 if (codeString == null || "".equals(codeString)) 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("preparation".equals(codeString)) 187 return EventStatus.PREPARATION; 188 if ("in-progress".equals(codeString)) 189 return EventStatus.INPROGRESS; 190 if ("not-done".equals(codeString)) 191 return EventStatus.NOTDONE; 192 if ("on-hold".equals(codeString)) 193 return EventStatus.ONHOLD; 194 if ("stopped".equals(codeString)) 195 return EventStatus.STOPPED; 196 if ("completed".equals(codeString)) 197 return EventStatus.COMPLETED; 198 if ("entered-in-error".equals(codeString)) 199 return EventStatus.ENTEREDINERROR; 200 if ("unknown".equals(codeString)) 201 return EventStatus.UNKNOWN; 202 throw new IllegalArgumentException("Unknown EventStatus code '"+codeString+"'"); 203 } 204 public Enumeration<EventStatus> fromType(PrimitiveType<?> code) throws FHIRException { 205 if (code == null) 206 return null; 207 if (code.isEmpty()) 208 return new Enumeration<EventStatus>(this, EventStatus.NULL, code); 209 String codeString = ((PrimitiveType) code).asStringValue(); 210 if (codeString == null || "".equals(codeString)) 211 return new Enumeration<EventStatus>(this, EventStatus.NULL, code); 212 if ("preparation".equals(codeString)) 213 return new Enumeration<EventStatus>(this, EventStatus.PREPARATION, code); 214 if ("in-progress".equals(codeString)) 215 return new Enumeration<EventStatus>(this, EventStatus.INPROGRESS, code); 216 if ("not-done".equals(codeString)) 217 return new Enumeration<EventStatus>(this, EventStatus.NOTDONE, code); 218 if ("on-hold".equals(codeString)) 219 return new Enumeration<EventStatus>(this, EventStatus.ONHOLD, code); 220 if ("stopped".equals(codeString)) 221 return new Enumeration<EventStatus>(this, EventStatus.STOPPED, code); 222 if ("completed".equals(codeString)) 223 return new Enumeration<EventStatus>(this, EventStatus.COMPLETED, code); 224 if ("entered-in-error".equals(codeString)) 225 return new Enumeration<EventStatus>(this, EventStatus.ENTEREDINERROR, code); 226 if ("unknown".equals(codeString)) 227 return new Enumeration<EventStatus>(this, EventStatus.UNKNOWN, code); 228 throw new FHIRException("Unknown EventStatus code '"+codeString+"'"); 229 } 230 public String toCode(EventStatus code) { 231 if (code == EventStatus.NULL) 232 return null; 233 if (code == EventStatus.PREPARATION) 234 return "preparation"; 235 if (code == EventStatus.INPROGRESS) 236 return "in-progress"; 237 if (code == EventStatus.NOTDONE) 238 return "not-done"; 239 if (code == EventStatus.ONHOLD) 240 return "on-hold"; 241 if (code == EventStatus.STOPPED) 242 return "stopped"; 243 if (code == EventStatus.COMPLETED) 244 return "completed"; 245 if (code == EventStatus.ENTEREDINERROR) 246 return "entered-in-error"; 247 if (code == EventStatus.UNKNOWN) 248 return "unknown"; 249 return "?"; 250 } 251 public String toSystem(EventStatus code) { 252 return code.getSystem(); 253 } 254 } 255 256 public interface EventPerformerComponent extends PatternBase { 257 /** 258 * @return {@link #function} (Distinguishes the type of involvement of the performer in the {{title}}. [Consider adding examples].) 259 */ 260 public CodeableConcept getFunction() throws FHIRException ; 261 262 /** 263 * @return whether there is more than zero values for function 264 */ 265 public boolean hasFunction(); 266 /** 267 * @return minimum allowed cardinality for function. Note that with patterns, this may be different for the underlying resource 268 */ 269 public int getFunctionMin(); 270 /** 271 * @return maximum allowed cardinality for function. Note that with patterns, this may be different for the underlying resource 272 */ 273 public int getFunctionMax(); 274 /** 275 * @param value {@link #function} (Distinguishes the type of involvement of the performer in the {{title}}. [Consider adding examples].) 276 */ 277 public EventPerformerComponent setFunction(CodeableConcept value) throws FHIRException; 278 279 /** 280 * @return {@link #actor} (Indicates who or what performed the {{title}}.) 281 */ 282 public Reference getActor() throws FHIRException ; 283 284 /** 285 * @return whether there is more than zero values for actor 286 */ 287 public boolean hasActor(); 288 /** 289 * @return minimum allowed cardinality for actor. Note that with patterns, this may be different for the underlying resource 290 */ 291 public int getActorMin(); 292 /** 293 * @return maximum allowed cardinality for actor. Note that with patterns, this may be different for the underlying resource 294 */ 295 public int getActorMax(); 296 /** 297 * @param value {@link #actor} (Indicates who or what performed the {{title}}.) 298 */ 299 public EventPerformerComponent setActor(Reference value) throws FHIRException; 300 301 } 302 303 /** 304 * @return {@link #identifier} (Business identifiers assigned to this {{title}} by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 305 */ 306 public List<Identifier> getIdentifier() throws FHIRException; 307 308 /** 309 * @return Returns a reference to <code>this</code> for easy method chaining 310 */ 311 public Event setIdentifier(List<Identifier> theIdentifier) throws FHIRException; 312 313 /** 314 * @return whether there is more than zero values for identifier 315 */ 316 public boolean hasIdentifier(); 317 /** 318 * @return minimum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 319 */ 320 public int getIdentifierMin(); 321 /** 322 * @return maximum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 323 */ 324 public int getIdentifierMax(); 325 326 public Identifier addIdentifier() throws FHIRException; 327 328 public Event addIdentifier(Identifier t) throws FHIRException; 329 330 /** 331 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 332 */ 333 public Identifier getIdentifierFirstRep() throws FHIRException; 334 335 /** 336 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 337 */ 338 public List<CanonicalType> getInstantiatesCanonical() throws FHIRException; 339 340 /** 341 * @return Returns a reference to <code>this</code> for easy method chaining 342 */ 343 public Event setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) throws FHIRException; 344 345 /** 346 * @return whether there is more than zero values for instantiatesCanonical 347 */ 348 public boolean hasInstantiatesCanonical(); 349 /** 350 * @return minimum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 351 */ 352 public int getInstantiatesCanonicalMin(); 353 /** 354 * @return maximum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 355 */ 356 public int getInstantiatesCanonicalMax(); 357 358 /** 359 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 360 */ 361 public CanonicalType addInstantiatesCanonicalElement() throws FHIRException; 362 363 /** 364 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 365 */ 366 public Event addInstantiatesCanonical(String value) throws FHIRException; 367 368 /** 369 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 370 */ 371 public boolean hasInstantiatesCanonical(String value) throws FHIRException; 372 373 /** 374 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 375 */ 376 public List<UriType> getInstantiatesUri() throws FHIRException; 377 378 /** 379 * @return Returns a reference to <code>this</code> for easy method chaining 380 */ 381 public Event setInstantiatesUri(List<UriType> theInstantiatesUri) throws FHIRException; 382 383 /** 384 * @return whether there is more than zero values for instantiatesUri 385 */ 386 public boolean hasInstantiatesUri(); 387 /** 388 * @return minimum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 389 */ 390 public int getInstantiatesUriMin(); 391 /** 392 * @return maximum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 393 */ 394 public int getInstantiatesUriMax(); 395 396 /** 397 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 398 */ 399 public UriType addInstantiatesUriElement() throws FHIRException; 400 401 /** 402 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 403 */ 404 public Event addInstantiatesUri(String value) throws FHIRException; 405 406 /** 407 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 408 */ 409 public boolean hasInstantiatesUri(String value) throws FHIRException; 410 411 /** 412 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this {{title}}.) 413 */ 414 public List<Reference> getBasedOn() throws FHIRException; 415 416 /** 417 * @return Returns a reference to <code>this</code> for easy method chaining 418 */ 419 public Event setBasedOn(List<Reference> theBasedOn) throws FHIRException; 420 421 /** 422 * @return whether there is more than zero values for basedOn 423 */ 424 public boolean hasBasedOn(); 425 /** 426 * @return minimum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 427 */ 428 public int getBasedOnMin(); 429 /** 430 * @return maximum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 431 */ 432 public int getBasedOnMax(); 433 434 public Reference addBasedOn() throws FHIRException; 435 436 public Event addBasedOn(Reference t) throws FHIRException; 437 438 /** 439 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 440 */ 441 public Reference getBasedOnFirstRep() throws FHIRException; 442 443 /** 444 * @return {@link #partOf} (A larger event of which this particular {{title}} is a component or step.) 445 */ 446 public List<Reference> getPartOf() throws FHIRException; 447 448 /** 449 * @return Returns a reference to <code>this</code> for easy method chaining 450 */ 451 public Event setPartOf(List<Reference> thePartOf) throws FHIRException; 452 453 /** 454 * @return whether there is more than zero values for partOf 455 */ 456 public boolean hasPartOf(); 457 /** 458 * @return minimum allowed cardinality for partOf. Note that with patterns, this may be different for the underlying resource 459 */ 460 public int getPartOfMin(); 461 /** 462 * @return maximum allowed cardinality for partOf. Note that with patterns, this may be different for the underlying resource 463 */ 464 public int getPartOfMax(); 465 466 public Reference addPartOf() throws FHIRException; 467 468 public Event addPartOf(Reference t) throws FHIRException; 469 470 /** 471 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 472 */ 473 public Reference getPartOfFirstRep() throws FHIRException; 474 475 /** 476 * @return {@link #researchStudy} (Indicates that this {{title}} is relevant to the specified research study(ies).) 477 */ 478 public List<Reference> getResearchStudy() throws FHIRException; 479 480 /** 481 * @return Returns a reference to <code>this</code> for easy method chaining 482 */ 483 public Event setResearchStudy(List<Reference> theResearchStudy) throws FHIRException; 484 485 /** 486 * @return whether there is more than zero values for researchStudy 487 */ 488 public boolean hasResearchStudy(); 489 /** 490 * @return minimum allowed cardinality for researchStudy. Note that with patterns, this may be different for the underlying resource 491 */ 492 public int getResearchStudyMin(); 493 /** 494 * @return maximum allowed cardinality for researchStudy. Note that with patterns, this may be different for the underlying resource 495 */ 496 public int getResearchStudyMax(); 497 498 public Reference addResearchStudy() throws FHIRException; 499 500 public Event addResearchStudy(Reference t) throws FHIRException; 501 502 /** 503 * @return The first repetition of repeating field {@link #researchStudy}, creating it if it does not already exist 504 */ 505 public Reference getResearchStudyFirstRep() throws FHIRException; 506 507 /** 508 * @return {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 509 */ 510 public Enumeration<EventStatus> getStatusElement() throws FHIRException; 511 512 /** 513 * @return whether there is more than zero values for status 514 */ 515 public boolean hasStatus(); 516 /** 517 * @return minimum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 518 */ 519 public int getStatusMin() throws FHIRException; 520 /** 521 * @return maximum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 522 */ 523 public int getStatusMax() throws FHIRException; 524 public boolean hasStatusElement(); 525 526 /** 527 * @param value {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 528 */ 529 public Event setStatusElement(Enumeration<EventStatus> value) throws FHIRException; 530 531 /** 532 * @return The current state of the {{title}}. 533 */ 534 public EventStatus getStatus() throws FHIRException; 535 536 /** 537 * @param value The current state of the {{title}}. 538 */ 539 public Event setStatus(EventStatus value) throws FHIRException; 540 541 /** 542 * @return {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 543 */ 544 public CodeableConcept getStatusReason() throws FHIRException ; 545 546 /** 547 * @return whether there is more than zero values for statusReason 548 */ 549 public boolean hasStatusReason(); 550 /** 551 * @return minimum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 552 */ 553 public int getStatusReasonMin(); 554 /** 555 * @return maximum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 556 */ 557 public int getStatusReasonMax(); 558 /** 559 * @param value {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 560 */ 561 public Event setStatusReason(CodeableConcept value) throws FHIRException; 562 563 /** 564 * @return {@link #code} (A code that identifies the specific service or action that was or is being performed.) 565 */ 566 public CodeableConcept getCode() throws FHIRException ; 567 568 /** 569 * @return whether there is more than zero values for code 570 */ 571 public boolean hasCode(); 572 /** 573 * @return minimum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 574 */ 575 public int getCodeMin(); 576 /** 577 * @return maximum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 578 */ 579 public int getCodeMax(); 580 /** 581 * @param value {@link #code} (A code that identifies the specific service or action that was or is being performed.) 582 */ 583 public Event setCode(CodeableConcept value) throws FHIRException; 584 585 /** 586 * @return {@link #subject} (The individual or set of individuals the action is being or was performed on.) 587 */ 588 public Reference getSubject() throws FHIRException ; 589 590 /** 591 * @return whether there is more than zero values for subject 592 */ 593 public boolean hasSubject(); 594 /** 595 * @return minimum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 596 */ 597 public int getSubjectMin(); 598 /** 599 * @return maximum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 600 */ 601 public int getSubjectMax(); 602 /** 603 * @param value {@link #subject} (The individual or set of individuals the action is being or was performed on.) 604 */ 605 public Event setSubject(Reference value) throws FHIRException; 606 607 /** 608 * @return {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 609 */ 610 public Reference getEncounter() throws FHIRException ; 611 612 /** 613 * @return whether there is more than zero values for encounter 614 */ 615 public boolean hasEncounter(); 616 /** 617 * @return minimum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 618 */ 619 public int getEncounterMin(); 620 /** 621 * @return maximum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 622 */ 623 public int getEncounterMax(); 624 /** 625 * @param value {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 626 */ 627 public Event setEncounter(Reference value) throws FHIRException; 628 629 /** 630 * @return {@link #occurrence} (The date, period or timing when the {{title}} did occur or is occurring.) 631 */ 632 public DataType getOccurrence() throws FHIRException ; 633 634 /** 635 * @return {@link #occurrence} (The date, period or timing when the {{title}} did occur or is occurring.) 636 */ 637 public DateTimeType getOccurrenceDateTimeType() throws FHIRException; 638 639 public boolean hasOccurrenceDateTimeType(); 640 641 /** 642 * @return {@link #occurrence} (The date, period or timing when the {{title}} did occur or is occurring.) 643 */ 644 public Period getOccurrencePeriod() throws FHIRException; 645 646 public boolean hasOccurrencePeriod(); 647 648 /** 649 * @return {@link #occurrence} (The date, period or timing when the {{title}} did occur or is occurring.) 650 */ 651 public Timing getOccurrenceTiming() throws FHIRException; 652 653 public boolean hasOccurrenceTiming(); 654 655 /** 656 * @return whether there is more than zero values for occurrence 657 */ 658 public boolean hasOccurrence(); 659 /** 660 * @return minimum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 661 */ 662 public int getOccurrenceMin(); 663 /** 664 * @return maximum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 665 */ 666 public int getOccurrenceMax(); 667 /** 668 * @param value {@link #occurrence} (The date, period or timing when the {{title}} did occur or is occurring.) 669 */ 670 public Event setOccurrence(DataType value) throws FHIRException; 671 672 /** 673 * @return {@link #recorded} (The date the occurrence of the {{title}} was first captured in the record - potentially significantly after the occurrence of the event.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 674 */ 675 public DateTimeType getRecordedElement() throws FHIRException; 676 677 /** 678 * @return whether there is more than zero values for recorded 679 */ 680 public boolean hasRecorded(); 681 /** 682 * @return minimum allowed cardinality for recorded. Note that with patterns, this may be different for the underlying resource 683 */ 684 public int getRecordedMin() throws FHIRException; 685 /** 686 * @return maximum allowed cardinality for recorded. Note that with patterns, this may be different for the underlying resource 687 */ 688 public int getRecordedMax() throws FHIRException; 689 public boolean hasRecordedElement(); 690 691 /** 692 * @param value {@link #recorded} (The date the occurrence of the {{title}} was first captured in the record - potentially significantly after the occurrence of the event.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 693 */ 694 public Event setRecordedElement(DateTimeType value) throws FHIRException; 695 696 /** 697 * @return The date the occurrence of the {{title}} was first captured in the record - potentially significantly after the occurrence of the event. 698 */ 699 public Date getRecorded() throws FHIRException; 700 701 /** 702 * @param value The date the occurrence of the {{title}} was first captured in the record - potentially significantly after the occurrence of the event. 703 */ 704 public Event setRecorded(Date value) throws FHIRException; 705 706 /** 707 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 708 */ 709 public DataType getReported() throws FHIRException ; 710 711 /** 712 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 713 */ 714 public BooleanType getReportedBooleanType() throws FHIRException; 715 716 public boolean hasReportedBooleanType(); 717 718 /** 719 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 720 */ 721 public Reference getReportedReference() throws FHIRException; 722 723 public boolean hasReportedReference(); 724 725 /** 726 * @return whether there is more than zero values for reported 727 */ 728 public boolean hasReported(); 729 /** 730 * @return minimum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 731 */ 732 public int getReportedMin(); 733 /** 734 * @return maximum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 735 */ 736 public int getReportedMax(); 737 /** 738 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 739 */ 740 public Event setReported(DataType value) throws FHIRException; 741 742 /** 743 * @return {@link #performer} (Indicates who or what performed the {{title}} and how they were involved.) 744 */ 745 public List<EventPerformerComponent> getPerformer() throws FHIRException; 746 747 /** 748 * @return Returns a reference to <code>this</code> for easy method chaining 749 */ 750 public Event setPerformer(List<EventPerformerComponent> thePerformer) throws FHIRException; 751 752 /** 753 * @return whether there is more than zero values for performer 754 */ 755 public boolean hasPerformer(); 756 /** 757 * @return minimum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 758 */ 759 public int getPerformerMin(); 760 /** 761 * @return maximum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 762 */ 763 public int getPerformerMax(); 764 765 public EventPerformerComponent addPerformer() throws FHIRException; 766 767 public Event addPerformer(EventPerformerComponent t) throws FHIRException; 768 769 /** 770 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 771 */ 772 public EventPerformerComponent getPerformerFirstRep() throws FHIRException; 773 774 /** 775 * @return {@link #location} (The principal physical location where the {{title}} was performed.) 776 */ 777 public Reference getLocation() throws FHIRException ; 778 779 /** 780 * @return whether there is more than zero values for location 781 */ 782 public boolean hasLocation(); 783 /** 784 * @return minimum allowed cardinality for location. Note that with patterns, this may be different for the underlying resource 785 */ 786 public int getLocationMin(); 787 /** 788 * @return maximum allowed cardinality for location. Note that with patterns, this may be different for the underlying resource 789 */ 790 public int getLocationMax(); 791 /** 792 * @param value {@link #location} (The principal physical location where the {{title}} was performed.) 793 */ 794 public Event setLocation(Reference value) throws FHIRException; 795 796 /** 797 * @return {@link #reasonCode} (Describes why the {{title}} occurred in coded or textual form.) 798 */ 799 public List<CodeableConcept> getReasonCode() throws FHIRException; 800 801 /** 802 * @return Returns a reference to <code>this</code> for easy method chaining 803 */ 804 public Event setReasonCode(List<CodeableConcept> theReasonCode) throws FHIRException; 805 806 /** 807 * @return whether there is more than zero values for reasonCode 808 */ 809 public boolean hasReasonCode(); 810 /** 811 * @return minimum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 812 */ 813 public int getReasonCodeMin(); 814 /** 815 * @return maximum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 816 */ 817 public int getReasonCodeMax(); 818 819 public CodeableConcept addReasonCode() throws FHIRException; 820 821 public Event addReasonCode(CodeableConcept t) throws FHIRException; 822 823 /** 824 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 825 */ 826 public CodeableConcept getReasonCodeFirstRep() throws FHIRException; 827 828 /** 829 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this {{title}}.) 830 */ 831 public List<Reference> getReasonReference() throws FHIRException; 832 833 /** 834 * @return Returns a reference to <code>this</code> for easy method chaining 835 */ 836 public Event setReasonReference(List<Reference> theReasonReference) throws FHIRException; 837 838 /** 839 * @return whether there is more than zero values for reasonReference 840 */ 841 public boolean hasReasonReference(); 842 /** 843 * @return minimum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 844 */ 845 public int getReasonReferenceMin(); 846 /** 847 * @return maximum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 848 */ 849 public int getReasonReferenceMax(); 850 851 public Reference addReasonReference() throws FHIRException; 852 853 public Event addReasonReference(Reference t) throws FHIRException; 854 855 /** 856 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 857 */ 858 public Reference getReasonReferenceFirstRep() throws FHIRException; 859 860 /** 861 * @return {@link #note} (Comments made about the {{title}} by the performer, subject or other participants.) 862 */ 863 public List<Annotation> getNote() throws FHIRException; 864 865 /** 866 * @return Returns a reference to <code>this</code> for easy method chaining 867 */ 868 public Event setNote(List<Annotation> theNote) throws FHIRException; 869 870 /** 871 * @return whether there is more than zero values for note 872 */ 873 public boolean hasNote(); 874 /** 875 * @return minimum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 876 */ 877 public int getNoteMin(); 878 /** 879 * @return maximum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 880 */ 881 public int getNoteMax(); 882 883 public Annotation addNote() throws FHIRException; 884 885 public Event addNote(Annotation t) throws FHIRException; 886 887 /** 888 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 889 */ 890 public Annotation getNoteFirstRep() throws FHIRException; 891 892 public String fhirType(); 893 894 895}