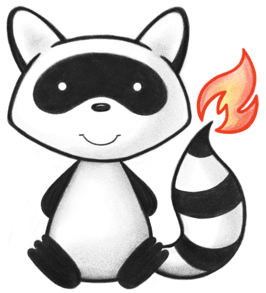
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006import java.util.Date; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039 040// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 041import org.hl7.fhir.r5.model.Annotation; 042import org.hl7.fhir.r5.model.Base; 043import org.hl7.fhir.r5.model.BooleanType; 044import org.hl7.fhir.r5.model.CanonicalType; 045import org.hl7.fhir.r5.model.CodeableConcept; 046import org.hl7.fhir.r5.model.Configuration; 047import org.hl7.fhir.r5.model.DataType; 048import org.hl7.fhir.r5.model.DateTimeType; 049import org.hl7.fhir.r5.model.EnumFactory; 050import org.hl7.fhir.r5.model.Enumeration; 051import org.hl7.fhir.r5.model.Identifier; 052import org.hl7.fhir.r5.model.Period; 053import org.hl7.fhir.r5.model.PrimitiveType; 054import org.hl7.fhir.r5.model.Reference; 055import org.hl7.fhir.r5.model.Timing; 056import org.hl7.fhir.r5.model.UriType; 057/** 058 * A pattern to be followed by resources that represent a specific proposal, plan and/or order for some sort of action or service. 059 */ 060public interface Request extends PatternBase { 061 062 public enum RequestStatus { 063 /** 064 * The request has been created but is not yet complete or ready for action. 065 */ 066 DRAFT, 067 /** 068 * The request is in force and ready to be acted upon. 069 */ 070 ACTIVE, 071 /** 072 * The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future. 073 */ 074 ONHOLD, 075 /** 076 * The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur. 077 */ 078 REVOKED, 079 /** 080 * The activity described by the request has been fully performed. No further activity will occur. 081 */ 082 COMPLETED, 083 /** 084 * This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".). 085 */ 086 ENTEREDINERROR, 087 /** 088 * The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 public static RequestStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("draft".equals(codeString)) 099 return DRAFT; 100 if ("active".equals(codeString)) 101 return ACTIVE; 102 if ("on-hold".equals(codeString)) 103 return ONHOLD; 104 if ("revoked".equals(codeString)) 105 return REVOKED; 106 if ("completed".equals(codeString)) 107 return COMPLETED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case DRAFT: return "draft"; 120 case ACTIVE: return "active"; 121 case ONHOLD: return "on-hold"; 122 case REVOKED: return "revoked"; 123 case COMPLETED: return "completed"; 124 case ENTEREDINERROR: return "entered-in-error"; 125 case UNKNOWN: return "unknown"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getSystem() { 131 switch (this) { 132 case DRAFT: return "http://hl7.org/fhir/request-status"; 133 case ACTIVE: return "http://hl7.org/fhir/request-status"; 134 case ONHOLD: return "http://hl7.org/fhir/request-status"; 135 case REVOKED: return "http://hl7.org/fhir/request-status"; 136 case COMPLETED: return "http://hl7.org/fhir/request-status"; 137 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 138 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case DRAFT: return "The request has been created but is not yet complete or ready for action."; 146 case ACTIVE: return "The request is in force and ready to be acted upon."; 147 case ONHOLD: return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 148 case REVOKED: return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 149 case COMPLETED: return "The activity described by the request has been fully performed. No further activity will occur."; 150 case ENTEREDINERROR: return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 151 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 152 case NULL: return null; 153 default: return "?"; 154 } 155 } 156 public String getDisplay() { 157 switch (this) { 158 case DRAFT: return "Draft"; 159 case ACTIVE: return "Active"; 160 case ONHOLD: return "On Hold"; 161 case REVOKED: return "Revoked"; 162 case COMPLETED: return "Completed"; 163 case ENTEREDINERROR: return "Entered in Error"; 164 case UNKNOWN: return "Unknown"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 } 170 171 public class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 172 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 173 if (codeString == null || "".equals(codeString)) 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("draft".equals(codeString)) 177 return RequestStatus.DRAFT; 178 if ("active".equals(codeString)) 179 return RequestStatus.ACTIVE; 180 if ("on-hold".equals(codeString)) 181 return RequestStatus.ONHOLD; 182 if ("revoked".equals(codeString)) 183 return RequestStatus.REVOKED; 184 if ("completed".equals(codeString)) 185 return RequestStatus.COMPLETED; 186 if ("entered-in-error".equals(codeString)) 187 return RequestStatus.ENTEREDINERROR; 188 if ("unknown".equals(codeString)) 189 return RequestStatus.UNKNOWN; 190 throw new IllegalArgumentException("Unknown RequestStatus code '"+codeString+"'"); 191 } 192 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 193 if (code == null) 194 return null; 195 if (code.isEmpty()) 196 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 200 if ("draft".equals(codeString)) 201 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT, code); 202 if ("active".equals(codeString)) 203 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE, code); 204 if ("on-hold".equals(codeString)) 205 return new Enumeration<RequestStatus>(this, RequestStatus.ONHOLD, code); 206 if ("revoked".equals(codeString)) 207 return new Enumeration<RequestStatus>(this, RequestStatus.REVOKED, code); 208 if ("completed".equals(codeString)) 209 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED, code); 210 if ("entered-in-error".equals(codeString)) 211 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR, code); 212 if ("unknown".equals(codeString)) 213 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN, code); 214 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 215 } 216 public String toCode(RequestStatus code) { 217 if (code == RequestStatus.NULL) 218 return null; 219 if (code == RequestStatus.DRAFT) 220 return "draft"; 221 if (code == RequestStatus.ACTIVE) 222 return "active"; 223 if (code == RequestStatus.ONHOLD) 224 return "on-hold"; 225 if (code == RequestStatus.REVOKED) 226 return "revoked"; 227 if (code == RequestStatus.COMPLETED) 228 return "completed"; 229 if (code == RequestStatus.ENTEREDINERROR) 230 return "entered-in-error"; 231 if (code == RequestStatus.UNKNOWN) 232 return "unknown"; 233 return "?"; 234 } 235 public String toSystem(RequestStatus code) { 236 return code.getSystem(); 237 } 238 } 239 240 public enum RequestIntent { 241 /** 242 * The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act. 243 */ 244 PROPOSAL, 245 /** 246 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 247 */ 248 PLAN, 249 /** 250 * The request represents a legally binding instruction authored by a Patient or RelatedPerson. 251 */ 252 DIRECTIVE, 253 /** 254 * The request represents a request/demand and authorization for action by a Practitioner. 255 */ 256 ORDER, 257 /** 258 * The request represents an original authorization for action. 259 */ 260 ORIGINALORDER, 261 /** 262 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization. 263 */ 264 REFLEXORDER, 265 /** 266 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 267 */ 268 FILLERORDER, 269 /** 270 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 271 */ 272 INSTANCEORDER, 273 /** 274 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used. 275 */ 276 OPTION, 277 /** 278 * added to help the parsers with the generic types 279 */ 280 NULL; 281 public static RequestIntent fromCode(String codeString) throws FHIRException { 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("proposal".equals(codeString)) 285 return PROPOSAL; 286 if ("plan".equals(codeString)) 287 return PLAN; 288 if ("directive".equals(codeString)) 289 return DIRECTIVE; 290 if ("order".equals(codeString)) 291 return ORDER; 292 if ("original-order".equals(codeString)) 293 return ORIGINALORDER; 294 if ("reflex-order".equals(codeString)) 295 return REFLEXORDER; 296 if ("filler-order".equals(codeString)) 297 return FILLERORDER; 298 if ("instance-order".equals(codeString)) 299 return INSTANCEORDER; 300 if ("option".equals(codeString)) 301 return OPTION; 302 if (Configuration.isAcceptInvalidEnums()) 303 return null; 304 else 305 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 306 } 307 public String toCode() { 308 switch (this) { 309 case PROPOSAL: return "proposal"; 310 case PLAN: return "plan"; 311 case DIRECTIVE: return "directive"; 312 case ORDER: return "order"; 313 case ORIGINALORDER: return "original-order"; 314 case REFLEXORDER: return "reflex-order"; 315 case FILLERORDER: return "filler-order"; 316 case INSTANCEORDER: return "instance-order"; 317 case OPTION: return "option"; 318 case NULL: return null; 319 default: return "?"; 320 } 321 } 322 public String getSystem() { 323 switch (this) { 324 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 325 case PLAN: return "http://hl7.org/fhir/request-intent"; 326 case DIRECTIVE: return "http://hl7.org/fhir/request-intent"; 327 case ORDER: return "http://hl7.org/fhir/request-intent"; 328 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 329 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 330 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 331 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 332 case OPTION: return "http://hl7.org/fhir/request-intent"; 333 case NULL: return null; 334 default: return "?"; 335 } 336 } 337 public String getDefinition() { 338 switch (this) { 339 case PROPOSAL: return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 340 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 341 case DIRECTIVE: return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 342 case ORDER: return "The request represents a request/demand and authorization for action by a Practitioner."; 343 case ORIGINALORDER: return "The request represents an original authorization for action."; 344 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 345 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 346 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 347 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 348 case NULL: return null; 349 default: return "?"; 350 } 351 } 352 public String getDisplay() { 353 switch (this) { 354 case PROPOSAL: return "Proposal"; 355 case PLAN: return "Plan"; 356 case DIRECTIVE: return "Directive"; 357 case ORDER: return "Order"; 358 case ORIGINALORDER: return "Original Order"; 359 case REFLEXORDER: return "Reflex Order"; 360 case FILLERORDER: return "Filler Order"; 361 case INSTANCEORDER: return "Instance Order"; 362 case OPTION: return "Option"; 363 case NULL: return null; 364 default: return "?"; 365 } 366 } 367 } 368 369 public class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 370 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 371 if (codeString == null || "".equals(codeString)) 372 if (codeString == null || "".equals(codeString)) 373 return null; 374 if ("proposal".equals(codeString)) 375 return RequestIntent.PROPOSAL; 376 if ("plan".equals(codeString)) 377 return RequestIntent.PLAN; 378 if ("directive".equals(codeString)) 379 return RequestIntent.DIRECTIVE; 380 if ("order".equals(codeString)) 381 return RequestIntent.ORDER; 382 if ("original-order".equals(codeString)) 383 return RequestIntent.ORIGINALORDER; 384 if ("reflex-order".equals(codeString)) 385 return RequestIntent.REFLEXORDER; 386 if ("filler-order".equals(codeString)) 387 return RequestIntent.FILLERORDER; 388 if ("instance-order".equals(codeString)) 389 return RequestIntent.INSTANCEORDER; 390 if ("option".equals(codeString)) 391 return RequestIntent.OPTION; 392 throw new IllegalArgumentException("Unknown RequestIntent code '"+codeString+"'"); 393 } 394 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 395 if (code == null) 396 return null; 397 if (code.isEmpty()) 398 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 399 String codeString = ((PrimitiveType) code).asStringValue(); 400 if (codeString == null || "".equals(codeString)) 401 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 402 if ("proposal".equals(codeString)) 403 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 404 if ("plan".equals(codeString)) 405 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 406 if ("directive".equals(codeString)) 407 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 408 if ("order".equals(codeString)) 409 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 410 if ("original-order".equals(codeString)) 411 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 412 if ("reflex-order".equals(codeString)) 413 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 414 if ("filler-order".equals(codeString)) 415 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 416 if ("instance-order".equals(codeString)) 417 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 418 if ("option".equals(codeString)) 419 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 420 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 421 } 422 public String toCode(RequestIntent code) { 423 if (code == RequestIntent.NULL) 424 return null; 425 if (code == RequestIntent.PROPOSAL) 426 return "proposal"; 427 if (code == RequestIntent.PLAN) 428 return "plan"; 429 if (code == RequestIntent.DIRECTIVE) 430 return "directive"; 431 if (code == RequestIntent.ORDER) 432 return "order"; 433 if (code == RequestIntent.ORIGINALORDER) 434 return "original-order"; 435 if (code == RequestIntent.REFLEXORDER) 436 return "reflex-order"; 437 if (code == RequestIntent.FILLERORDER) 438 return "filler-order"; 439 if (code == RequestIntent.INSTANCEORDER) 440 return "instance-order"; 441 if (code == RequestIntent.OPTION) 442 return "option"; 443 return "?"; 444 } 445 public String toSystem(RequestIntent code) { 446 return code.getSystem(); 447 } 448 } 449 450 public enum RequestPriority { 451 /** 452 * The request has normal priority. 453 */ 454 ROUTINE, 455 /** 456 * The request should be actioned promptly - higher priority than routine. 457 */ 458 URGENT, 459 /** 460 * The request should be actioned as soon as possible - higher priority than urgent. 461 */ 462 ASAP, 463 /** 464 * The request should be actioned immediately - highest possible priority. E.g. an emergency. 465 */ 466 STAT, 467 /** 468 * added to help the parsers with the generic types 469 */ 470 NULL; 471 public static RequestPriority fromCode(String codeString) throws FHIRException { 472 if (codeString == null || "".equals(codeString)) 473 return null; 474 if ("routine".equals(codeString)) 475 return ROUTINE; 476 if ("urgent".equals(codeString)) 477 return URGENT; 478 if ("asap".equals(codeString)) 479 return ASAP; 480 if ("stat".equals(codeString)) 481 return STAT; 482 if (Configuration.isAcceptInvalidEnums()) 483 return null; 484 else 485 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 486 } 487 public String toCode() { 488 switch (this) { 489 case ROUTINE: return "routine"; 490 case URGENT: return "urgent"; 491 case ASAP: return "asap"; 492 case STAT: return "stat"; 493 case NULL: return null; 494 default: return "?"; 495 } 496 } 497 public String getSystem() { 498 switch (this) { 499 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 500 case URGENT: return "http://hl7.org/fhir/request-priority"; 501 case ASAP: return "http://hl7.org/fhir/request-priority"; 502 case STAT: return "http://hl7.org/fhir/request-priority"; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 public String getDefinition() { 508 switch (this) { 509 case ROUTINE: return "The request has normal priority."; 510 case URGENT: return "The request should be actioned promptly - higher priority than routine."; 511 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent."; 512 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 513 case NULL: return null; 514 default: return "?"; 515 } 516 } 517 public String getDisplay() { 518 switch (this) { 519 case ROUTINE: return "Routine"; 520 case URGENT: return "Urgent"; 521 case ASAP: return "ASAP"; 522 case STAT: return "STAT"; 523 case NULL: return null; 524 default: return "?"; 525 } 526 } 527 } 528 529 public class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 530 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 531 if (codeString == null || "".equals(codeString)) 532 if (codeString == null || "".equals(codeString)) 533 return null; 534 if ("routine".equals(codeString)) 535 return RequestPriority.ROUTINE; 536 if ("urgent".equals(codeString)) 537 return RequestPriority.URGENT; 538 if ("asap".equals(codeString)) 539 return RequestPriority.ASAP; 540 if ("stat".equals(codeString)) 541 return RequestPriority.STAT; 542 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 543 } 544 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 545 if (code == null) 546 return null; 547 if (code.isEmpty()) 548 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 549 String codeString = ((PrimitiveType) code).asStringValue(); 550 if (codeString == null || "".equals(codeString)) 551 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 552 if ("routine".equals(codeString)) 553 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 554 if ("urgent".equals(codeString)) 555 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 556 if ("asap".equals(codeString)) 557 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 558 if ("stat".equals(codeString)) 559 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 560 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 561 } 562 public String toCode(RequestPriority code) { 563 if (code == RequestPriority.NULL) 564 return null; 565 if (code == RequestPriority.ROUTINE) 566 return "routine"; 567 if (code == RequestPriority.URGENT) 568 return "urgent"; 569 if (code == RequestPriority.ASAP) 570 return "asap"; 571 if (code == RequestPriority.STAT) 572 return "stat"; 573 return "?"; 574 } 575 public String toSystem(RequestPriority code) { 576 return code.getSystem(); 577 } 578 } 579 580 /** 581 * @return {@link #identifier} (Business identifiers assigned to this {{title}} by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 582 */ 583 public List<Identifier> getIdentifier() throws FHIRException; 584 585 /** 586 * @return Returns a reference to <code>this</code> for easy method chaining 587 */ 588 public Request setIdentifier(List<Identifier> theIdentifier) throws FHIRException; 589 590 /** 591 * @return whether there is more than zero values for identifier 592 */ 593 public boolean hasIdentifier(); 594 /** 595 * @return minimum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 596 */ 597 public int getIdentifierMin(); 598 /** 599 * @return maximum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 600 */ 601 public int getIdentifierMax(); 602 603 public Identifier addIdentifier() throws FHIRException; 604 605 public Request addIdentifier(Identifier t) throws FHIRException; 606 607 /** 608 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 609 */ 610 public Identifier getIdentifierFirstRep() throws FHIRException; 611 612 /** 613 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 614 */ 615 public List<CanonicalType> getInstantiatesCanonical() throws FHIRException; 616 617 /** 618 * @return Returns a reference to <code>this</code> for easy method chaining 619 */ 620 public Request setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) throws FHIRException; 621 622 /** 623 * @return whether there is more than zero values for instantiatesCanonical 624 */ 625 public boolean hasInstantiatesCanonical(); 626 /** 627 * @return minimum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 628 */ 629 public int getInstantiatesCanonicalMin(); 630 /** 631 * @return maximum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 632 */ 633 public int getInstantiatesCanonicalMax(); 634 635 /** 636 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 637 */ 638 public CanonicalType addInstantiatesCanonicalElement() throws FHIRException; 639 640 /** 641 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 642 */ 643 public Request addInstantiatesCanonical(String value) throws FHIRException; 644 645 /** 646 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 647 */ 648 public boolean hasInstantiatesCanonical(String value) throws FHIRException; 649 650 /** 651 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 652 */ 653 public List<UriType> getInstantiatesUri() throws FHIRException; 654 655 /** 656 * @return Returns a reference to <code>this</code> for easy method chaining 657 */ 658 public Request setInstantiatesUri(List<UriType> theInstantiatesUri) throws FHIRException; 659 660 /** 661 * @return whether there is more than zero values for instantiatesUri 662 */ 663 public boolean hasInstantiatesUri(); 664 /** 665 * @return minimum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 666 */ 667 public int getInstantiatesUriMin(); 668 /** 669 * @return maximum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 670 */ 671 public int getInstantiatesUriMax(); 672 673 /** 674 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 675 */ 676 public UriType addInstantiatesUriElement() throws FHIRException; 677 678 /** 679 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 680 */ 681 public Request addInstantiatesUri(String value) throws FHIRException; 682 683 /** 684 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 685 */ 686 public boolean hasInstantiatesUri(String value) throws FHIRException; 687 688 /** 689 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this {{title}}.) 690 */ 691 public List<Reference> getBasedOn() throws FHIRException; 692 693 /** 694 * @return Returns a reference to <code>this</code> for easy method chaining 695 */ 696 public Request setBasedOn(List<Reference> theBasedOn) throws FHIRException; 697 698 /** 699 * @return whether there is more than zero values for basedOn 700 */ 701 public boolean hasBasedOn(); 702 /** 703 * @return minimum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 704 */ 705 public int getBasedOnMin(); 706 /** 707 * @return maximum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 708 */ 709 public int getBasedOnMax(); 710 711 public Reference addBasedOn() throws FHIRException; 712 713 public Request addBasedOn(Reference t) throws FHIRException; 714 715 /** 716 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 717 */ 718 public Reference getBasedOnFirstRep() throws FHIRException; 719 720 /** 721 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new {{title}}.) 722 */ 723 public List<Reference> getReplaces() throws FHIRException; 724 725 /** 726 * @return Returns a reference to <code>this</code> for easy method chaining 727 */ 728 public Request setReplaces(List<Reference> theReplaces) throws FHIRException; 729 730 /** 731 * @return whether there is more than zero values for replaces 732 */ 733 public boolean hasReplaces(); 734 /** 735 * @return minimum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 736 */ 737 public int getReplacesMin(); 738 /** 739 * @return maximum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 740 */ 741 public int getReplacesMax(); 742 743 public Reference addReplaces() throws FHIRException; 744 745 public Request addReplaces(Reference t) throws FHIRException; 746 747 /** 748 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 749 */ 750 public Reference getReplacesFirstRep() throws FHIRException; 751 752 /** 753 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 754 */ 755 public Identifier getGroupIdentifier() throws FHIRException ; 756 757 /** 758 * @return whether there is more than zero values for groupIdentifier 759 */ 760 public boolean hasGroupIdentifier(); 761 /** 762 * @return minimum allowed cardinality for groupIdentifier. Note that with patterns, this may be different for the underlying resource 763 */ 764 public int getGroupIdentifierMin(); 765 /** 766 * @return maximum allowed cardinality for groupIdentifier. Note that with patterns, this may be different for the underlying resource 767 */ 768 public int getGroupIdentifierMax(); 769 /** 770 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 771 */ 772 public Request setGroupIdentifier(Identifier value) throws FHIRException; 773 774 /** 775 * @return {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 776 */ 777 public Enumeration<RequestStatus> getStatusElement() throws FHIRException; 778 779 /** 780 * @return whether there is more than zero values for status 781 */ 782 public boolean hasStatus(); 783 /** 784 * @return minimum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 785 */ 786 public int getStatusMin() throws FHIRException; 787 /** 788 * @return maximum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 789 */ 790 public int getStatusMax() throws FHIRException; 791 public boolean hasStatusElement(); 792 793 /** 794 * @param value {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 795 */ 796 public Request setStatusElement(Enumeration<RequestStatus> value) throws FHIRException; 797 798 /** 799 * @return The current state of the {{title}}. 800 */ 801 public RequestStatus getStatus() throws FHIRException; 802 803 /** 804 * @param value The current state of the {{title}}. 805 */ 806 public Request setStatus(RequestStatus value) throws FHIRException; 807 808 /** 809 * @return {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 810 */ 811 public CodeableConcept getStatusReason() throws FHIRException ; 812 813 /** 814 * @return whether there is more than zero values for statusReason 815 */ 816 public boolean hasStatusReason(); 817 /** 818 * @return minimum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 819 */ 820 public int getStatusReasonMin(); 821 /** 822 * @return maximum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 823 */ 824 public int getStatusReasonMax(); 825 /** 826 * @param value {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 827 */ 828 public Request setStatusReason(CodeableConcept value) throws FHIRException; 829 830 /** 831 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 832 */ 833 public Enumeration<RequestIntent> getIntentElement() throws FHIRException; 834 835 /** 836 * @return whether there is more than zero values for intent 837 */ 838 public boolean hasIntent(); 839 /** 840 * @return minimum allowed cardinality for intent. Note that with patterns, this may be different for the underlying resource 841 */ 842 public int getIntentMin() throws FHIRException; 843 /** 844 * @return maximum allowed cardinality for intent. Note that with patterns, this may be different for the underlying resource 845 */ 846 public int getIntentMax() throws FHIRException; 847 public boolean hasIntentElement(); 848 849 /** 850 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 851 */ 852 public Request setIntentElement(Enumeration<RequestIntent> value) throws FHIRException; 853 854 /** 855 * @return Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain. 856 */ 857 public RequestIntent getIntent() throws FHIRException; 858 859 /** 860 * @param value Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain. 861 */ 862 public Request setIntent(RequestIntent value) throws FHIRException; 863 864 /** 865 * @return {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 866 */ 867 public Enumeration<RequestPriority> getPriorityElement() throws FHIRException; 868 869 /** 870 * @return whether there is more than zero values for priority 871 */ 872 public boolean hasPriority(); 873 /** 874 * @return minimum allowed cardinality for priority. Note that with patterns, this may be different for the underlying resource 875 */ 876 public int getPriorityMin() throws FHIRException; 877 /** 878 * @return maximum allowed cardinality for priority. Note that with patterns, this may be different for the underlying resource 879 */ 880 public int getPriorityMax() throws FHIRException; 881 public boolean hasPriorityElement(); 882 883 /** 884 * @param value {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 885 */ 886 public Request setPriorityElement(Enumeration<RequestPriority> value) throws FHIRException; 887 888 /** 889 * @return Indicates how quickly the {{title}} should be addressed with respect to other requests. 890 */ 891 public RequestPriority getPriority() throws FHIRException; 892 893 /** 894 * @param value Indicates how quickly the {{title}} should be addressed with respect to other requests. 895 */ 896 public Request setPriority(RequestPriority value) throws FHIRException; 897 898 /** 899 * @return {@link #doNotPerform} (If true indicates that the {{title}} is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 900 */ 901 public BooleanType getDoNotPerformElement() throws FHIRException; 902 903 /** 904 * @return whether there is more than zero values for doNotPerform 905 */ 906 public boolean hasDoNotPerform(); 907 /** 908 * @return minimum allowed cardinality for doNotPerform. Note that with patterns, this may be different for the underlying resource 909 */ 910 public int getDoNotPerformMin() throws FHIRException; 911 /** 912 * @return maximum allowed cardinality for doNotPerform. Note that with patterns, this may be different for the underlying resource 913 */ 914 public int getDoNotPerformMax() throws FHIRException; 915 public boolean hasDoNotPerformElement(); 916 917 /** 918 * @param value {@link #doNotPerform} (If true indicates that the {{title}} is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 919 */ 920 public Request setDoNotPerformElement(BooleanType value) throws FHIRException; 921 922 /** 923 * @return If true indicates that the {{title}} is asking for the specified action to *not* occur. 924 */ 925 public boolean getDoNotPerform() throws FHIRException; 926 927 /** 928 * @param value If true indicates that the {{title}} is asking for the specified action to *not* occur. 929 */ 930 public Request setDoNotPerform(boolean value) throws FHIRException; 931 932 /** 933 * @return {@link #code} (A code that identifies the specific service or action being asked to be done (or not done).) 934 */ 935 public CodeableConcept getCode() throws FHIRException ; 936 937 /** 938 * @return whether there is more than zero values for code 939 */ 940 public boolean hasCode(); 941 /** 942 * @return minimum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 943 */ 944 public int getCodeMin(); 945 /** 946 * @return maximum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 947 */ 948 public int getCodeMax(); 949 /** 950 * @param value {@link #code} (A code that identifies the specific service or action being asked to be done (or not done).) 951 */ 952 public Request setCode(CodeableConcept value) throws FHIRException; 953 954 /** 955 * @return {@link #subject} (The individual or set of individuals the action is to be performed/not performed on or for.) 956 */ 957 public Reference getSubject() throws FHIRException ; 958 959 /** 960 * @return whether there is more than zero values for subject 961 */ 962 public boolean hasSubject(); 963 /** 964 * @return minimum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 965 */ 966 public int getSubjectMin(); 967 /** 968 * @return maximum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 969 */ 970 public int getSubjectMax(); 971 /** 972 * @param value {@link #subject} (The individual or set of individuals the action is to be performed/not performed on or for.) 973 */ 974 public Request setSubject(Reference value) throws FHIRException; 975 976 /** 977 * @return {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 978 */ 979 public Reference getEncounter() throws FHIRException ; 980 981 /** 982 * @return whether there is more than zero values for encounter 983 */ 984 public boolean hasEncounter(); 985 /** 986 * @return minimum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 987 */ 988 public int getEncounterMin(); 989 /** 990 * @return maximum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 991 */ 992 public int getEncounterMax(); 993 /** 994 * @param value {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 995 */ 996 public Request setEncounter(Reference value) throws FHIRException; 997 998 /** 999 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1000 */ 1001 public DataType getOccurrence() throws FHIRException ; 1002 1003 /** 1004 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1005 */ 1006 public DateTimeType getOccurrenceDateTimeType() throws FHIRException; 1007 1008 public boolean hasOccurrenceDateTimeType(); 1009 1010 /** 1011 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1012 */ 1013 public Period getOccurrencePeriod() throws FHIRException; 1014 1015 public boolean hasOccurrencePeriod(); 1016 1017 /** 1018 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1019 */ 1020 public Timing getOccurrenceTiming() throws FHIRException; 1021 1022 public boolean hasOccurrenceTiming(); 1023 1024 /** 1025 * @return whether there is more than zero values for occurrence 1026 */ 1027 public boolean hasOccurrence(); 1028 /** 1029 * @return minimum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 1030 */ 1031 public int getOccurrenceMin(); 1032 /** 1033 * @return maximum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 1034 */ 1035 public int getOccurrenceMax(); 1036 /** 1037 * @param value {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1038 */ 1039 public Request setOccurrence(DataType value) throws FHIRException; 1040 1041 /** 1042 * @return {@link #authoredOn} (For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1043 */ 1044 public DateTimeType getAuthoredOnElement() throws FHIRException; 1045 1046 /** 1047 * @return whether there is more than zero values for authoredOn 1048 */ 1049 public boolean hasAuthoredOn(); 1050 /** 1051 * @return minimum allowed cardinality for authoredOn. Note that with patterns, this may be different for the underlying resource 1052 */ 1053 public int getAuthoredOnMin() throws FHIRException; 1054 /** 1055 * @return maximum allowed cardinality for authoredOn. Note that with patterns, this may be different for the underlying resource 1056 */ 1057 public int getAuthoredOnMax() throws FHIRException; 1058 public boolean hasAuthoredOnElement(); 1059 1060 /** 1061 * @param value {@link #authoredOn} (For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1062 */ 1063 public Request setAuthoredOnElement(DateTimeType value) throws FHIRException; 1064 1065 /** 1066 * @return For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1067 */ 1068 public Date getAuthoredOn() throws FHIRException; 1069 1070 /** 1071 * @param value For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1072 */ 1073 public Request setAuthoredOn(Date value) throws FHIRException; 1074 1075 /** 1076 * @return {@link #requester} (Who initiated the {{request}} and has responsibility for its activation.) 1077 */ 1078 public Reference getRequester() throws FHIRException ; 1079 1080 /** 1081 * @return whether there is more than zero values for requester 1082 */ 1083 public boolean hasRequester(); 1084 /** 1085 * @return minimum allowed cardinality for requester. Note that with patterns, this may be different for the underlying resource 1086 */ 1087 public int getRequesterMin(); 1088 /** 1089 * @return maximum allowed cardinality for requester. Note that with patterns, this may be different for the underlying resource 1090 */ 1091 public int getRequesterMax(); 1092 /** 1093 * @param value {@link #requester} (Who initiated the {{request}} and has responsibility for its activation.) 1094 */ 1095 public Request setRequester(Reference value) throws FHIRException; 1096 1097 /** 1098 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1099 */ 1100 public DataType getReported() throws FHIRException ; 1101 1102 /** 1103 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1104 */ 1105 public BooleanType getReportedBooleanType() throws FHIRException; 1106 1107 public boolean hasReportedBooleanType(); 1108 1109 /** 1110 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1111 */ 1112 public Reference getReportedReference() throws FHIRException; 1113 1114 public boolean hasReportedReference(); 1115 1116 /** 1117 * @return whether there is more than zero values for reported 1118 */ 1119 public boolean hasReported(); 1120 /** 1121 * @return minimum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 1122 */ 1123 public int getReportedMin(); 1124 /** 1125 * @return maximum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 1126 */ 1127 public int getReportedMax(); 1128 /** 1129 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1130 */ 1131 public Request setReported(DataType value) throws FHIRException; 1132 1133 /** 1134 * @return {@link #performerType} (The type of individual that is desired to act upon/ not act upon the {{request}}.) 1135 */ 1136 public CodeableConcept getPerformerType() throws FHIRException ; 1137 1138 /** 1139 * @return whether there is more than zero values for performerType 1140 */ 1141 public boolean hasPerformerType(); 1142 /** 1143 * @return minimum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 1144 */ 1145 public int getPerformerTypeMin(); 1146 /** 1147 * @return maximum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 1148 */ 1149 public int getPerformerTypeMax(); 1150 /** 1151 * @param value {@link #performerType} (The type of individual that is desired to act upon/ not act upon the {{request}}.) 1152 */ 1153 public Request setPerformerType(CodeableConcept value) throws FHIRException; 1154 1155 /** 1156 * @return {@link #performer} (Indicates who or what is being asked to perform (or not perform) the {{request}}.) 1157 */ 1158 public Reference getPerformer() throws FHIRException ; 1159 1160 /** 1161 * @return whether there is more than zero values for performer 1162 */ 1163 public boolean hasPerformer(); 1164 /** 1165 * @return minimum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 1166 */ 1167 public int getPerformerMin(); 1168 /** 1169 * @return maximum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 1170 */ 1171 public int getPerformerMax(); 1172 /** 1173 * @param value {@link #performer} (Indicates who or what is being asked to perform (or not perform) the {{request}}.) 1174 */ 1175 public Request setPerformer(Reference value) throws FHIRException; 1176 1177 /** 1178 * @return {@link #reasonCode} (Describes why the request is being made in coded or textual form.) 1179 */ 1180 public List<CodeableConcept> getReasonCode() throws FHIRException; 1181 1182 /** 1183 * @return Returns a reference to <code>this</code> for easy method chaining 1184 */ 1185 public Request setReasonCode(List<CodeableConcept> theReasonCode) throws FHIRException; 1186 1187 /** 1188 * @return whether there is more than zero values for reasonCode 1189 */ 1190 public boolean hasReasonCode(); 1191 /** 1192 * @return minimum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 1193 */ 1194 public int getReasonCodeMin(); 1195 /** 1196 * @return maximum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 1197 */ 1198 public int getReasonCodeMax(); 1199 1200 public CodeableConcept addReasonCode() throws FHIRException; 1201 1202 public Request addReasonCode(CodeableConcept t) throws FHIRException; 1203 1204 /** 1205 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1206 */ 1207 public CodeableConcept getReasonCodeFirstRep() throws FHIRException; 1208 1209 /** 1210 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this request.) 1211 */ 1212 public List<Reference> getReasonReference() throws FHIRException; 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public Request setReasonReference(List<Reference> theReasonReference) throws FHIRException; 1218 1219 /** 1220 * @return whether there is more than zero values for reasonReference 1221 */ 1222 public boolean hasReasonReference(); 1223 /** 1224 * @return minimum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 1225 */ 1226 public int getReasonReferenceMin(); 1227 /** 1228 * @return maximum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 1229 */ 1230 public int getReasonReferenceMax(); 1231 1232 public Reference addReasonReference() throws FHIRException; 1233 1234 public Request addReasonReference(Reference t) throws FHIRException; 1235 1236 /** 1237 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1238 */ 1239 public Reference getReasonReferenceFirstRep() throws FHIRException; 1240 1241 /** 1242 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant in delivering the requested service.) 1243 */ 1244 public List<Reference> getInsurance() throws FHIRException; 1245 1246 /** 1247 * @return Returns a reference to <code>this</code> for easy method chaining 1248 */ 1249 public Request setInsurance(List<Reference> theInsurance) throws FHIRException; 1250 1251 /** 1252 * @return whether there is more than zero values for insurance 1253 */ 1254 public boolean hasInsurance(); 1255 /** 1256 * @return minimum allowed cardinality for insurance. Note that with patterns, this may be different for the underlying resource 1257 */ 1258 public int getInsuranceMin(); 1259 /** 1260 * @return maximum allowed cardinality for insurance. Note that with patterns, this may be different for the underlying resource 1261 */ 1262 public int getInsuranceMax(); 1263 1264 public Reference addInsurance() throws FHIRException; 1265 1266 public Request addInsurance(Reference t) throws FHIRException; 1267 1268 /** 1269 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 1270 */ 1271 public Reference getInsuranceFirstRep() throws FHIRException; 1272 1273 /** 1274 * @return {@link #supportingInfo} (Information that may be needed by/relevant to the performer in their execution of this {{title}}.) 1275 */ 1276 public List<Reference> getSupportingInfo() throws FHIRException; 1277 1278 /** 1279 * @return Returns a reference to <code>this</code> for easy method chaining 1280 */ 1281 public Request setSupportingInfo(List<Reference> theSupportingInfo) throws FHIRException; 1282 1283 /** 1284 * @return whether there is more than zero values for supportingInfo 1285 */ 1286 public boolean hasSupportingInfo(); 1287 /** 1288 * @return minimum allowed cardinality for supportingInfo. Note that with patterns, this may be different for the underlying resource 1289 */ 1290 public int getSupportingInfoMin(); 1291 /** 1292 * @return maximum allowed cardinality for supportingInfo. Note that with patterns, this may be different for the underlying resource 1293 */ 1294 public int getSupportingInfoMax(); 1295 1296 public Reference addSupportingInfo() throws FHIRException; 1297 1298 public Request addSupportingInfo(Reference t) throws FHIRException; 1299 1300 /** 1301 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 1302 */ 1303 public Reference getSupportingInfoFirstRep() throws FHIRException; 1304 1305 /** 1306 * @return {@link #note} (Comments made about the {{title}} by the requester, performer, subject or other participants.) 1307 */ 1308 public List<Annotation> getNote() throws FHIRException; 1309 1310 /** 1311 * @return Returns a reference to <code>this</code> for easy method chaining 1312 */ 1313 public Request setNote(List<Annotation> theNote) throws FHIRException; 1314 1315 /** 1316 * @return whether there is more than zero values for note 1317 */ 1318 public boolean hasNote(); 1319 /** 1320 * @return minimum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 1321 */ 1322 public int getNoteMin(); 1323 /** 1324 * @return maximum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 1325 */ 1326 public int getNoteMax(); 1327 1328 public Annotation addNote() throws FHIRException; 1329 1330 public Request addNote(Annotation t) throws FHIRException; 1331 1332 /** 1333 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1334 */ 1335 public Annotation getNoteFirstRep() throws FHIRException; 1336 1337 /** 1338 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 1339 */ 1340 public List<Reference> getRelevantHistory() throws FHIRException; 1341 1342 /** 1343 * @return Returns a reference to <code>this</code> for easy method chaining 1344 */ 1345 public Request setRelevantHistory(List<Reference> theRelevantHistory) throws FHIRException; 1346 1347 /** 1348 * @return whether there is more than zero values for relevantHistory 1349 */ 1350 public boolean hasRelevantHistory(); 1351 /** 1352 * @return minimum allowed cardinality for relevantHistory. Note that with patterns, this may be different for the underlying resource 1353 */ 1354 public int getRelevantHistoryMin(); 1355 /** 1356 * @return maximum allowed cardinality for relevantHistory. Note that with patterns, this may be different for the underlying resource 1357 */ 1358 public int getRelevantHistoryMax(); 1359 1360 public Reference addRelevantHistory() throws FHIRException; 1361 1362 public Request addRelevantHistory(Reference t) throws FHIRException; 1363 1364 /** 1365 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 1366 */ 1367 public Reference getRelevantHistoryFirstRep() throws FHIRException; 1368 1369 public String fhirType(); 1370 1371 1372}