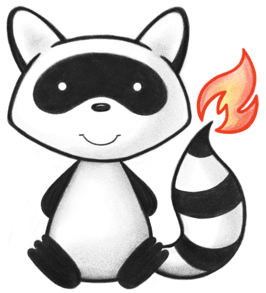
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006import java.util.Date; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039 040// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 041import org.hl7.fhir.r5.model.Annotation; 042import org.hl7.fhir.r5.model.Base; 043import org.hl7.fhir.r5.model.BooleanType; 044import org.hl7.fhir.r5.model.CanonicalType; 045import org.hl7.fhir.r5.model.CodeableConcept; 046import org.hl7.fhir.r5.model.Configuration; 047import org.hl7.fhir.r5.model.DataType; 048import org.hl7.fhir.r5.model.DateTimeType; 049import org.hl7.fhir.r5.model.EnumFactory; 050import org.hl7.fhir.r5.model.Enumeration; 051import org.hl7.fhir.r5.model.Identifier; 052import org.hl7.fhir.r5.model.Period; 053import org.hl7.fhir.r5.model.PrimitiveType; 054import org.hl7.fhir.r5.model.Reference; 055import org.hl7.fhir.r5.model.Timing; 056import org.hl7.fhir.r5.model.UriType; 057/** 058 * A pattern to be followed by resources that represent a specific proposal, plan and/or order for some sort of action or service. 059 */ 060public interface Request extends PatternBase { 061 062 public enum RequestStatus { 063 /** 064 * The request has been created but is not yet complete or ready for action. 065 */ 066 DRAFT, 067 /** 068 * The request is in force and ready to be acted upon. 069 */ 070 ACTIVE, 071 /** 072 * The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future. 073 */ 074 ONHOLD, 075 /** 076 * The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur. 077 */ 078 REVOKED, 079 /** 080 * The activity described by the request has been fully performed. No further activity will occur. 081 */ 082 COMPLETED, 083 /** 084 * This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".). 085 */ 086 ENTEREDINERROR, 087 /** 088 * The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 public static RequestStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("draft".equals(codeString)) 099 return DRAFT; 100 if ("active".equals(codeString)) 101 return ACTIVE; 102 if ("on-hold".equals(codeString)) 103 return ONHOLD; 104 if ("revoked".equals(codeString)) 105 return REVOKED; 106 if ("completed".equals(codeString)) 107 return COMPLETED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case DRAFT: return "draft"; 120 case ACTIVE: return "active"; 121 case ONHOLD: return "on-hold"; 122 case REVOKED: return "revoked"; 123 case COMPLETED: return "completed"; 124 case ENTEREDINERROR: return "entered-in-error"; 125 case UNKNOWN: return "unknown"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getSystem() { 131 switch (this) { 132 case DRAFT: return "http://hl7.org/fhir/request-status"; 133 case ACTIVE: return "http://hl7.org/fhir/request-status"; 134 case ONHOLD: return "http://hl7.org/fhir/request-status"; 135 case REVOKED: return "http://hl7.org/fhir/request-status"; 136 case COMPLETED: return "http://hl7.org/fhir/request-status"; 137 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 138 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case DRAFT: return "The request has been created but is not yet complete or ready for action."; 146 case ACTIVE: return "The request is in force and ready to be acted upon."; 147 case ONHOLD: return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 148 case REVOKED: return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 149 case COMPLETED: return "The activity described by the request has been fully performed. No further activity will occur."; 150 case ENTEREDINERROR: return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 151 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 152 case NULL: return null; 153 default: return "?"; 154 } 155 } 156 public String getDisplay() { 157 switch (this) { 158 case DRAFT: return "Draft"; 159 case ACTIVE: return "Active"; 160 case ONHOLD: return "On Hold"; 161 case REVOKED: return "Revoked"; 162 case COMPLETED: return "Completed"; 163 case ENTEREDINERROR: return "Entered in Error"; 164 case UNKNOWN: return "Unknown"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 } 170 171 public class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 172 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 173 if (codeString == null || "".equals(codeString)) 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("draft".equals(codeString)) 177 return RequestStatus.DRAFT; 178 if ("active".equals(codeString)) 179 return RequestStatus.ACTIVE; 180 if ("on-hold".equals(codeString)) 181 return RequestStatus.ONHOLD; 182 if ("revoked".equals(codeString)) 183 return RequestStatus.REVOKED; 184 if ("completed".equals(codeString)) 185 return RequestStatus.COMPLETED; 186 if ("entered-in-error".equals(codeString)) 187 return RequestStatus.ENTEREDINERROR; 188 if ("unknown".equals(codeString)) 189 return RequestStatus.UNKNOWN; 190 throw new IllegalArgumentException("Unknown RequestStatus code '"+codeString+"'"); 191 } 192 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 193 if (code == null) 194 return null; 195 if (code.isEmpty()) 196 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 200 if ("draft".equals(codeString)) 201 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT, code); 202 if ("active".equals(codeString)) 203 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE, code); 204 if ("on-hold".equals(codeString)) 205 return new Enumeration<RequestStatus>(this, RequestStatus.ONHOLD, code); 206 if ("revoked".equals(codeString)) 207 return new Enumeration<RequestStatus>(this, RequestStatus.REVOKED, code); 208 if ("completed".equals(codeString)) 209 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED, code); 210 if ("entered-in-error".equals(codeString)) 211 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR, code); 212 if ("unknown".equals(codeString)) 213 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN, code); 214 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 215 } 216 public String toCode(RequestStatus code) { 217 if (code == RequestStatus.DRAFT) 218 return "draft"; 219 if (code == RequestStatus.ACTIVE) 220 return "active"; 221 if (code == RequestStatus.ONHOLD) 222 return "on-hold"; 223 if (code == RequestStatus.REVOKED) 224 return "revoked"; 225 if (code == RequestStatus.COMPLETED) 226 return "completed"; 227 if (code == RequestStatus.ENTEREDINERROR) 228 return "entered-in-error"; 229 if (code == RequestStatus.UNKNOWN) 230 return "unknown"; 231 return "?"; 232 } 233 public String toSystem(RequestStatus code) { 234 return code.getSystem(); 235 } 236 } 237 238 public enum RequestIntent { 239 /** 240 * The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act. 241 */ 242 PROPOSAL, 243 /** 244 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 245 */ 246 PLAN, 247 /** 248 * The request represents a legally binding instruction authored by a Patient or RelatedPerson. 249 */ 250 DIRECTIVE, 251 /** 252 * The request represents a request/demand and authorization for action by a Practitioner. 253 */ 254 ORDER, 255 /** 256 * The request represents an original authorization for action. 257 */ 258 ORIGINALORDER, 259 /** 260 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization. 261 */ 262 REFLEXORDER, 263 /** 264 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 265 */ 266 FILLERORDER, 267 /** 268 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 269 */ 270 INSTANCEORDER, 271 /** 272 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used. 273 */ 274 OPTION, 275 /** 276 * added to help the parsers with the generic types 277 */ 278 NULL; 279 public static RequestIntent fromCode(String codeString) throws FHIRException { 280 if (codeString == null || "".equals(codeString)) 281 return null; 282 if ("proposal".equals(codeString)) 283 return PROPOSAL; 284 if ("plan".equals(codeString)) 285 return PLAN; 286 if ("directive".equals(codeString)) 287 return DIRECTIVE; 288 if ("order".equals(codeString)) 289 return ORDER; 290 if ("original-order".equals(codeString)) 291 return ORIGINALORDER; 292 if ("reflex-order".equals(codeString)) 293 return REFLEXORDER; 294 if ("filler-order".equals(codeString)) 295 return FILLERORDER; 296 if ("instance-order".equals(codeString)) 297 return INSTANCEORDER; 298 if ("option".equals(codeString)) 299 return OPTION; 300 if (Configuration.isAcceptInvalidEnums()) 301 return null; 302 else 303 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 304 } 305 public String toCode() { 306 switch (this) { 307 case PROPOSAL: return "proposal"; 308 case PLAN: return "plan"; 309 case DIRECTIVE: return "directive"; 310 case ORDER: return "order"; 311 case ORIGINALORDER: return "original-order"; 312 case REFLEXORDER: return "reflex-order"; 313 case FILLERORDER: return "filler-order"; 314 case INSTANCEORDER: return "instance-order"; 315 case OPTION: return "option"; 316 case NULL: return null; 317 default: return "?"; 318 } 319 } 320 public String getSystem() { 321 switch (this) { 322 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 323 case PLAN: return "http://hl7.org/fhir/request-intent"; 324 case DIRECTIVE: return "http://hl7.org/fhir/request-intent"; 325 case ORDER: return "http://hl7.org/fhir/request-intent"; 326 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 327 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 328 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 329 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 330 case OPTION: return "http://hl7.org/fhir/request-intent"; 331 case NULL: return null; 332 default: return "?"; 333 } 334 } 335 public String getDefinition() { 336 switch (this) { 337 case PROPOSAL: return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 338 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 339 case DIRECTIVE: return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 340 case ORDER: return "The request represents a request/demand and authorization for action by a Practitioner."; 341 case ORIGINALORDER: return "The request represents an original authorization for action."; 342 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 343 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 344 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 345 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 346 case NULL: return null; 347 default: return "?"; 348 } 349 } 350 public String getDisplay() { 351 switch (this) { 352 case PROPOSAL: return "Proposal"; 353 case PLAN: return "Plan"; 354 case DIRECTIVE: return "Directive"; 355 case ORDER: return "Order"; 356 case ORIGINALORDER: return "Original Order"; 357 case REFLEXORDER: return "Reflex Order"; 358 case FILLERORDER: return "Filler Order"; 359 case INSTANCEORDER: return "Instance Order"; 360 case OPTION: return "Option"; 361 case NULL: return null; 362 default: return "?"; 363 } 364 } 365 } 366 367 public class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 368 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 369 if (codeString == null || "".equals(codeString)) 370 if (codeString == null || "".equals(codeString)) 371 return null; 372 if ("proposal".equals(codeString)) 373 return RequestIntent.PROPOSAL; 374 if ("plan".equals(codeString)) 375 return RequestIntent.PLAN; 376 if ("directive".equals(codeString)) 377 return RequestIntent.DIRECTIVE; 378 if ("order".equals(codeString)) 379 return RequestIntent.ORDER; 380 if ("original-order".equals(codeString)) 381 return RequestIntent.ORIGINALORDER; 382 if ("reflex-order".equals(codeString)) 383 return RequestIntent.REFLEXORDER; 384 if ("filler-order".equals(codeString)) 385 return RequestIntent.FILLERORDER; 386 if ("instance-order".equals(codeString)) 387 return RequestIntent.INSTANCEORDER; 388 if ("option".equals(codeString)) 389 return RequestIntent.OPTION; 390 throw new IllegalArgumentException("Unknown RequestIntent code '"+codeString+"'"); 391 } 392 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 393 if (code == null) 394 return null; 395 if (code.isEmpty()) 396 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 397 String codeString = ((PrimitiveType) code).asStringValue(); 398 if (codeString == null || "".equals(codeString)) 399 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 400 if ("proposal".equals(codeString)) 401 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 402 if ("plan".equals(codeString)) 403 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 404 if ("directive".equals(codeString)) 405 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 406 if ("order".equals(codeString)) 407 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 408 if ("original-order".equals(codeString)) 409 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 410 if ("reflex-order".equals(codeString)) 411 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 412 if ("filler-order".equals(codeString)) 413 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 414 if ("instance-order".equals(codeString)) 415 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 416 if ("option".equals(codeString)) 417 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 418 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 419 } 420 public String toCode(RequestIntent code) { 421 if (code == RequestIntent.PROPOSAL) 422 return "proposal"; 423 if (code == RequestIntent.PLAN) 424 return "plan"; 425 if (code == RequestIntent.DIRECTIVE) 426 return "directive"; 427 if (code == RequestIntent.ORDER) 428 return "order"; 429 if (code == RequestIntent.ORIGINALORDER) 430 return "original-order"; 431 if (code == RequestIntent.REFLEXORDER) 432 return "reflex-order"; 433 if (code == RequestIntent.FILLERORDER) 434 return "filler-order"; 435 if (code == RequestIntent.INSTANCEORDER) 436 return "instance-order"; 437 if (code == RequestIntent.OPTION) 438 return "option"; 439 return "?"; 440 } 441 public String toSystem(RequestIntent code) { 442 return code.getSystem(); 443 } 444 } 445 446 public enum RequestPriority { 447 /** 448 * The request has normal priority. 449 */ 450 ROUTINE, 451 /** 452 * The request should be actioned promptly - higher priority than routine. 453 */ 454 URGENT, 455 /** 456 * The request should be actioned as soon as possible - higher priority than urgent. 457 */ 458 ASAP, 459 /** 460 * The request should be actioned immediately - highest possible priority. E.g. an emergency. 461 */ 462 STAT, 463 /** 464 * added to help the parsers with the generic types 465 */ 466 NULL; 467 public static RequestPriority fromCode(String codeString) throws FHIRException { 468 if (codeString == null || "".equals(codeString)) 469 return null; 470 if ("routine".equals(codeString)) 471 return ROUTINE; 472 if ("urgent".equals(codeString)) 473 return URGENT; 474 if ("asap".equals(codeString)) 475 return ASAP; 476 if ("stat".equals(codeString)) 477 return STAT; 478 if (Configuration.isAcceptInvalidEnums()) 479 return null; 480 else 481 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 482 } 483 public String toCode() { 484 switch (this) { 485 case ROUTINE: return "routine"; 486 case URGENT: return "urgent"; 487 case ASAP: return "asap"; 488 case STAT: return "stat"; 489 case NULL: return null; 490 default: return "?"; 491 } 492 } 493 public String getSystem() { 494 switch (this) { 495 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 496 case URGENT: return "http://hl7.org/fhir/request-priority"; 497 case ASAP: return "http://hl7.org/fhir/request-priority"; 498 case STAT: return "http://hl7.org/fhir/request-priority"; 499 case NULL: return null; 500 default: return "?"; 501 } 502 } 503 public String getDefinition() { 504 switch (this) { 505 case ROUTINE: return "The request has normal priority."; 506 case URGENT: return "The request should be actioned promptly - higher priority than routine."; 507 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent."; 508 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 509 case NULL: return null; 510 default: return "?"; 511 } 512 } 513 public String getDisplay() { 514 switch (this) { 515 case ROUTINE: return "Routine"; 516 case URGENT: return "Urgent"; 517 case ASAP: return "ASAP"; 518 case STAT: return "STAT"; 519 case NULL: return null; 520 default: return "?"; 521 } 522 } 523 } 524 525 public class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 526 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 527 if (codeString == null || "".equals(codeString)) 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("routine".equals(codeString)) 531 return RequestPriority.ROUTINE; 532 if ("urgent".equals(codeString)) 533 return RequestPriority.URGENT; 534 if ("asap".equals(codeString)) 535 return RequestPriority.ASAP; 536 if ("stat".equals(codeString)) 537 return RequestPriority.STAT; 538 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 539 } 540 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 541 if (code == null) 542 return null; 543 if (code.isEmpty()) 544 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 545 String codeString = ((PrimitiveType) code).asStringValue(); 546 if (codeString == null || "".equals(codeString)) 547 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 548 if ("routine".equals(codeString)) 549 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 550 if ("urgent".equals(codeString)) 551 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 552 if ("asap".equals(codeString)) 553 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 554 if ("stat".equals(codeString)) 555 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 556 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 557 } 558 public String toCode(RequestPriority code) { 559 if (code == RequestPriority.ROUTINE) 560 return "routine"; 561 if (code == RequestPriority.URGENT) 562 return "urgent"; 563 if (code == RequestPriority.ASAP) 564 return "asap"; 565 if (code == RequestPriority.STAT) 566 return "stat"; 567 return "?"; 568 } 569 public String toSystem(RequestPriority code) { 570 return code.getSystem(); 571 } 572 } 573 574 /** 575 * @return {@link #identifier} (Business identifiers assigned to this {{title}} by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 576 */ 577 public List<Identifier> getIdentifier() throws FHIRException; 578 579 /** 580 * @return Returns a reference to <code>this</code> for easy method chaining 581 */ 582 public Request setIdentifier(List<Identifier> theIdentifier) throws FHIRException; 583 584 /** 585 * @return whether there is more than zero values for identifier 586 */ 587 public boolean hasIdentifier(); 588 /** 589 * @return minimum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 590 */ 591 public int getIdentifierMin(); 592 /** 593 * @return maximum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 594 */ 595 public int getIdentifierMax(); 596 597 public Identifier addIdentifier() throws FHIRException; 598 599 public Request addIdentifier(Identifier t) throws FHIRException; 600 601 /** 602 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 603 */ 604 public Identifier getIdentifierFirstRep() throws FHIRException; 605 606 /** 607 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 608 */ 609 public List<CanonicalType> getInstantiatesCanonical() throws FHIRException; 610 611 /** 612 * @return Returns a reference to <code>this</code> for easy method chaining 613 */ 614 public Request setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) throws FHIRException; 615 616 /** 617 * @return whether there is more than zero values for instantiatesCanonical 618 */ 619 public boolean hasInstantiatesCanonical(); 620 /** 621 * @return minimum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 622 */ 623 public int getInstantiatesCanonicalMin(); 624 /** 625 * @return maximum allowed cardinality for instantiatesCanonical. Note that with patterns, this may be different for the underlying resource 626 */ 627 public int getInstantiatesCanonicalMax(); 628 629 /** 630 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 631 */ 632 public CanonicalType addInstantiatesCanonicalElement() throws FHIRException; 633 634 /** 635 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 636 */ 637 public Request addInstantiatesCanonical(String value) throws FHIRException; 638 639 /** 640 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 641 */ 642 public boolean hasInstantiatesCanonical(String value) throws FHIRException; 643 644 /** 645 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 646 */ 647 public List<UriType> getInstantiatesUri() throws FHIRException; 648 649 /** 650 * @return Returns a reference to <code>this</code> for easy method chaining 651 */ 652 public Request setInstantiatesUri(List<UriType> theInstantiatesUri) throws FHIRException; 653 654 /** 655 * @return whether there is more than zero values for instantiatesUri 656 */ 657 public boolean hasInstantiatesUri(); 658 /** 659 * @return minimum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 660 */ 661 public int getInstantiatesUriMin(); 662 /** 663 * @return maximum allowed cardinality for instantiatesUri. Note that with patterns, this may be different for the underlying resource 664 */ 665 public int getInstantiatesUriMax(); 666 667 /** 668 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 669 */ 670 public UriType addInstantiatesUriElement() throws FHIRException; 671 672 /** 673 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 674 */ 675 public Request addInstantiatesUri(String value) throws FHIRException; 676 677 /** 678 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this {{title}}.) 679 */ 680 public boolean hasInstantiatesUri(String value) throws FHIRException; 681 682 /** 683 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this {{title}}.) 684 */ 685 public List<Reference> getBasedOn() throws FHIRException; 686 687 /** 688 * @return Returns a reference to <code>this</code> for easy method chaining 689 */ 690 public Request setBasedOn(List<Reference> theBasedOn) throws FHIRException; 691 692 /** 693 * @return whether there is more than zero values for basedOn 694 */ 695 public boolean hasBasedOn(); 696 /** 697 * @return minimum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 698 */ 699 public int getBasedOnMin(); 700 /** 701 * @return maximum allowed cardinality for basedOn. Note that with patterns, this may be different for the underlying resource 702 */ 703 public int getBasedOnMax(); 704 705 public Reference addBasedOn() throws FHIRException; 706 707 public Request addBasedOn(Reference t) throws FHIRException; 708 709 /** 710 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 711 */ 712 public Reference getBasedOnFirstRep() throws FHIRException; 713 714 /** 715 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new {{title}}.) 716 */ 717 public List<Reference> getReplaces() throws FHIRException; 718 719 /** 720 * @return Returns a reference to <code>this</code> for easy method chaining 721 */ 722 public Request setReplaces(List<Reference> theReplaces) throws FHIRException; 723 724 /** 725 * @return whether there is more than zero values for replaces 726 */ 727 public boolean hasReplaces(); 728 /** 729 * @return minimum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 730 */ 731 public int getReplacesMin(); 732 /** 733 * @return maximum allowed cardinality for replaces. Note that with patterns, this may be different for the underlying resource 734 */ 735 public int getReplacesMax(); 736 737 public Reference addReplaces() throws FHIRException; 738 739 public Request addReplaces(Reference t) throws FHIRException; 740 741 /** 742 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 743 */ 744 public Reference getReplacesFirstRep() throws FHIRException; 745 746 /** 747 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 748 */ 749 public Identifier getGroupIdentifier() throws FHIRException ; 750 751 /** 752 * @return whether there is more than zero values for groupIdentifier 753 */ 754 public boolean hasGroupIdentifier(); 755 /** 756 * @return minimum allowed cardinality for groupIdentifier. Note that with patterns, this may be different for the underlying resource 757 */ 758 public int getGroupIdentifierMin(); 759 /** 760 * @return maximum allowed cardinality for groupIdentifier. Note that with patterns, this may be different for the underlying resource 761 */ 762 public int getGroupIdentifierMax(); 763 /** 764 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 765 */ 766 public Request setGroupIdentifier(Identifier value) throws FHIRException; 767 768 /** 769 * @return {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 770 */ 771 public Enumeration<RequestStatus> getStatusElement() throws FHIRException; 772 773 /** 774 * @return whether there is more than zero values for status 775 */ 776 public boolean hasStatus(); 777 /** 778 * @return minimum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 779 */ 780 public int getStatusMin() throws FHIRException; 781 /** 782 * @return maximum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 783 */ 784 public int getStatusMax() throws FHIRException; 785 public boolean hasStatusElement(); 786 787 /** 788 * @param value {@link #status} (The current state of the {{title}}.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 789 */ 790 public Request setStatusElement(Enumeration<RequestStatus> value) throws FHIRException; 791 792 /** 793 * @return The current state of the {{title}}. 794 */ 795 public RequestStatus getStatus() throws FHIRException; 796 797 /** 798 * @param value The current state of the {{title}}. 799 */ 800 public Request setStatus(RequestStatus value) throws FHIRException; 801 802 /** 803 * @return {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 804 */ 805 public CodeableConcept getStatusReason() throws FHIRException ; 806 807 /** 808 * @return whether there is more than zero values for statusReason 809 */ 810 public boolean hasStatusReason(); 811 /** 812 * @return minimum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 813 */ 814 public int getStatusReasonMin(); 815 /** 816 * @return maximum allowed cardinality for statusReason. Note that with patterns, this may be different for the underlying resource 817 */ 818 public int getStatusReasonMax(); 819 /** 820 * @param value {@link #statusReason} (Captures the reason for the current state of the {{title}}.) 821 */ 822 public Request setStatusReason(CodeableConcept value) throws FHIRException; 823 824 /** 825 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 826 */ 827 public Enumeration<RequestIntent> getIntentElement() throws FHIRException; 828 829 /** 830 * @return whether there is more than zero values for intent 831 */ 832 public boolean hasIntent(); 833 /** 834 * @return minimum allowed cardinality for intent. Note that with patterns, this may be different for the underlying resource 835 */ 836 public int getIntentMin() throws FHIRException; 837 /** 838 * @return maximum allowed cardinality for intent. Note that with patterns, this may be different for the underlying resource 839 */ 840 public int getIntentMax() throws FHIRException; 841 public boolean hasIntentElement(); 842 843 /** 844 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 845 */ 846 public Request setIntentElement(Enumeration<RequestIntent> value) throws FHIRException; 847 848 /** 849 * @return Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain. 850 */ 851 public RequestIntent getIntent() throws FHIRException; 852 853 /** 854 * @param value Indicates the level of authority/intentionality associated with the {{title}} and where the request fits into the workflow chain. 855 */ 856 public Request setIntent(RequestIntent value) throws FHIRException; 857 858 /** 859 * @return {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 860 */ 861 public Enumeration<RequestPriority> getPriorityElement() throws FHIRException; 862 863 /** 864 * @return whether there is more than zero values for priority 865 */ 866 public boolean hasPriority(); 867 /** 868 * @return minimum allowed cardinality for priority. Note that with patterns, this may be different for the underlying resource 869 */ 870 public int getPriorityMin() throws FHIRException; 871 /** 872 * @return maximum allowed cardinality for priority. Note that with patterns, this may be different for the underlying resource 873 */ 874 public int getPriorityMax() throws FHIRException; 875 public boolean hasPriorityElement(); 876 877 /** 878 * @param value {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 879 */ 880 public Request setPriorityElement(Enumeration<RequestPriority> value) throws FHIRException; 881 882 /** 883 * @return Indicates how quickly the {{title}} should be addressed with respect to other requests. 884 */ 885 public RequestPriority getPriority() throws FHIRException; 886 887 /** 888 * @param value Indicates how quickly the {{title}} should be addressed with respect to other requests. 889 */ 890 public Request setPriority(RequestPriority value) throws FHIRException; 891 892 /** 893 * @return {@link #doNotPerform} (If true indicates that the {{title}} is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 894 */ 895 public BooleanType getDoNotPerformElement() throws FHIRException; 896 897 /** 898 * @return whether there is more than zero values for doNotPerform 899 */ 900 public boolean hasDoNotPerform(); 901 /** 902 * @return minimum allowed cardinality for doNotPerform. Note that with patterns, this may be different for the underlying resource 903 */ 904 public int getDoNotPerformMin() throws FHIRException; 905 /** 906 * @return maximum allowed cardinality for doNotPerform. Note that with patterns, this may be different for the underlying resource 907 */ 908 public int getDoNotPerformMax() throws FHIRException; 909 public boolean hasDoNotPerformElement(); 910 911 /** 912 * @param value {@link #doNotPerform} (If true indicates that the {{title}} is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 913 */ 914 public Request setDoNotPerformElement(BooleanType value) throws FHIRException; 915 916 /** 917 * @return If true indicates that the {{title}} is asking for the specified action to *not* occur. 918 */ 919 public boolean getDoNotPerform() throws FHIRException; 920 921 /** 922 * @param value If true indicates that the {{title}} is asking for the specified action to *not* occur. 923 */ 924 public Request setDoNotPerform(boolean value) throws FHIRException; 925 926 /** 927 * @return {@link #code} (A code that identifies the specific service or action being asked to be done (or not done).) 928 */ 929 public CodeableConcept getCode() throws FHIRException ; 930 931 /** 932 * @return whether there is more than zero values for code 933 */ 934 public boolean hasCode(); 935 /** 936 * @return minimum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 937 */ 938 public int getCodeMin(); 939 /** 940 * @return maximum allowed cardinality for code. Note that with patterns, this may be different for the underlying resource 941 */ 942 public int getCodeMax(); 943 /** 944 * @param value {@link #code} (A code that identifies the specific service or action being asked to be done (or not done).) 945 */ 946 public Request setCode(CodeableConcept value) throws FHIRException; 947 948 /** 949 * @return {@link #subject} (The individual or set of individuals the action is to be performed/not performed on or for.) 950 */ 951 public Reference getSubject() throws FHIRException ; 952 953 /** 954 * @return whether there is more than zero values for subject 955 */ 956 public boolean hasSubject(); 957 /** 958 * @return minimum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 959 */ 960 public int getSubjectMin(); 961 /** 962 * @return maximum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 963 */ 964 public int getSubjectMax(); 965 /** 966 * @param value {@link #subject} (The individual or set of individuals the action is to be performed/not performed on or for.) 967 */ 968 public Request setSubject(Reference value) throws FHIRException; 969 970 /** 971 * @return {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 972 */ 973 public Reference getEncounter() throws FHIRException ; 974 975 /** 976 * @return whether there is more than zero values for encounter 977 */ 978 public boolean hasEncounter(); 979 /** 980 * @return minimum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 981 */ 982 public int getEncounterMin(); 983 /** 984 * @return maximum allowed cardinality for encounter. Note that with patterns, this may be different for the underlying resource 985 */ 986 public int getEncounterMax(); 987 /** 988 * @param value {@link #encounter} (The Encounter during which this {{title}} was created or to which the creation of this record is tightly associated.) 989 */ 990 public Request setEncounter(Reference value) throws FHIRException; 991 992 /** 993 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 994 */ 995 public DataType getOccurrence() throws FHIRException ; 996 997 /** 998 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 999 */ 1000 public DateTimeType getOccurrenceDateTimeType() throws FHIRException; 1001 1002 public boolean hasOccurrenceDateTimeType(); 1003 1004 /** 1005 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1006 */ 1007 public Period getOccurrencePeriod() throws FHIRException; 1008 1009 public boolean hasOccurrencePeriod(); 1010 1011 /** 1012 * @return {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1013 */ 1014 public Timing getOccurrenceTiming() throws FHIRException; 1015 1016 public boolean hasOccurrenceTiming(); 1017 1018 /** 1019 * @return whether there is more than zero values for occurrence 1020 */ 1021 public boolean hasOccurrence(); 1022 /** 1023 * @return minimum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 1024 */ 1025 public int getOccurrenceMin(); 1026 /** 1027 * @return maximum allowed cardinality for occurrence. Note that with patterns, this may be different for the underlying resource 1028 */ 1029 public int getOccurrenceMax(); 1030 /** 1031 * @param value {@link #occurrence} (The date or time(s) at which the activity or service is desired to occur or not occur.) 1032 */ 1033 public Request setOccurrence(DataType value) throws FHIRException; 1034 1035 /** 1036 * @return {@link #authoredOn} (For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1037 */ 1038 public DateTimeType getAuthoredOnElement() throws FHIRException; 1039 1040 /** 1041 * @return whether there is more than zero values for authoredOn 1042 */ 1043 public boolean hasAuthoredOn(); 1044 /** 1045 * @return minimum allowed cardinality for authoredOn. Note that with patterns, this may be different for the underlying resource 1046 */ 1047 public int getAuthoredOnMin() throws FHIRException; 1048 /** 1049 * @return maximum allowed cardinality for authoredOn. Note that with patterns, this may be different for the underlying resource 1050 */ 1051 public int getAuthoredOnMax() throws FHIRException; 1052 public boolean hasAuthoredOnElement(); 1053 1054 /** 1055 * @param value {@link #authoredOn} (For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1056 */ 1057 public Request setAuthoredOnElement(DateTimeType value) throws FHIRException; 1058 1059 /** 1060 * @return For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1061 */ 1062 public Date getAuthoredOn() throws FHIRException; 1063 1064 /** 1065 * @param value For draft {{title}}s, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1066 */ 1067 public Request setAuthoredOn(Date value) throws FHIRException; 1068 1069 /** 1070 * @return {@link #requester} (Who initiated the {{request}} and has responsibility for its activation.) 1071 */ 1072 public Reference getRequester() throws FHIRException ; 1073 1074 /** 1075 * @return whether there is more than zero values for requester 1076 */ 1077 public boolean hasRequester(); 1078 /** 1079 * @return minimum allowed cardinality for requester. Note that with patterns, this may be different for the underlying resource 1080 */ 1081 public int getRequesterMin(); 1082 /** 1083 * @return maximum allowed cardinality for requester. Note that with patterns, this may be different for the underlying resource 1084 */ 1085 public int getRequesterMax(); 1086 /** 1087 * @param value {@link #requester} (Who initiated the {{request}} and has responsibility for its activation.) 1088 */ 1089 public Request setRequester(Reference value) throws FHIRException; 1090 1091 /** 1092 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1093 */ 1094 public DataType getReported() throws FHIRException ; 1095 1096 /** 1097 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1098 */ 1099 public BooleanType getReportedBooleanType() throws FHIRException; 1100 1101 public boolean hasReportedBooleanType(); 1102 1103 /** 1104 * @return {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1105 */ 1106 public Reference getReportedReference() throws FHIRException; 1107 1108 public boolean hasReportedReference(); 1109 1110 /** 1111 * @return whether there is more than zero values for reported 1112 */ 1113 public boolean hasReported(); 1114 /** 1115 * @return minimum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 1116 */ 1117 public int getReportedMin(); 1118 /** 1119 * @return maximum allowed cardinality for reported. Note that with patterns, this may be different for the underlying resource 1120 */ 1121 public int getReportedMax(); 1122 /** 1123 * @param value {@link #reported} (Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.) 1124 */ 1125 public Request setReported(DataType value) throws FHIRException; 1126 1127 /** 1128 * @return {@link #performerType} (The type of individual that is desired to act upon/ not act upon the {{request}}.) 1129 */ 1130 public CodeableConcept getPerformerType() throws FHIRException ; 1131 1132 /** 1133 * @return whether there is more than zero values for performerType 1134 */ 1135 public boolean hasPerformerType(); 1136 /** 1137 * @return minimum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 1138 */ 1139 public int getPerformerTypeMin(); 1140 /** 1141 * @return maximum allowed cardinality for performerType. Note that with patterns, this may be different for the underlying resource 1142 */ 1143 public int getPerformerTypeMax(); 1144 /** 1145 * @param value {@link #performerType} (The type of individual that is desired to act upon/ not act upon the {{request}}.) 1146 */ 1147 public Request setPerformerType(CodeableConcept value) throws FHIRException; 1148 1149 /** 1150 * @return {@link #performer} (Indicates who or what is being asked to perform (or not perform) the {{request}}.) 1151 */ 1152 public Reference getPerformer() throws FHIRException ; 1153 1154 /** 1155 * @return whether there is more than zero values for performer 1156 */ 1157 public boolean hasPerformer(); 1158 /** 1159 * @return minimum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 1160 */ 1161 public int getPerformerMin(); 1162 /** 1163 * @return maximum allowed cardinality for performer. Note that with patterns, this may be different for the underlying resource 1164 */ 1165 public int getPerformerMax(); 1166 /** 1167 * @param value {@link #performer} (Indicates who or what is being asked to perform (or not perform) the {{request}}.) 1168 */ 1169 public Request setPerformer(Reference value) throws FHIRException; 1170 1171 /** 1172 * @return {@link #reasonCode} (Describes why the request is being made in coded or textual form.) 1173 */ 1174 public List<CodeableConcept> getReasonCode() throws FHIRException; 1175 1176 /** 1177 * @return Returns a reference to <code>this</code> for easy method chaining 1178 */ 1179 public Request setReasonCode(List<CodeableConcept> theReasonCode) throws FHIRException; 1180 1181 /** 1182 * @return whether there is more than zero values for reasonCode 1183 */ 1184 public boolean hasReasonCode(); 1185 /** 1186 * @return minimum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 1187 */ 1188 public int getReasonCodeMin(); 1189 /** 1190 * @return maximum allowed cardinality for reasonCode. Note that with patterns, this may be different for the underlying resource 1191 */ 1192 public int getReasonCodeMax(); 1193 1194 public CodeableConcept addReasonCode() throws FHIRException; 1195 1196 public Request addReasonCode(CodeableConcept t) throws FHIRException; 1197 1198 /** 1199 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1200 */ 1201 public CodeableConcept getReasonCodeFirstRep() throws FHIRException; 1202 1203 /** 1204 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this request.) 1205 */ 1206 public List<Reference> getReasonReference() throws FHIRException; 1207 1208 /** 1209 * @return Returns a reference to <code>this</code> for easy method chaining 1210 */ 1211 public Request setReasonReference(List<Reference> theReasonReference) throws FHIRException; 1212 1213 /** 1214 * @return whether there is more than zero values for reasonReference 1215 */ 1216 public boolean hasReasonReference(); 1217 /** 1218 * @return minimum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 1219 */ 1220 public int getReasonReferenceMin(); 1221 /** 1222 * @return maximum allowed cardinality for reasonReference. Note that with patterns, this may be different for the underlying resource 1223 */ 1224 public int getReasonReferenceMax(); 1225 1226 public Reference addReasonReference() throws FHIRException; 1227 1228 public Request addReasonReference(Reference t) throws FHIRException; 1229 1230 /** 1231 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1232 */ 1233 public Reference getReasonReferenceFirstRep() throws FHIRException; 1234 1235 /** 1236 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant in delivering the requested service.) 1237 */ 1238 public List<Reference> getInsurance() throws FHIRException; 1239 1240 /** 1241 * @return Returns a reference to <code>this</code> for easy method chaining 1242 */ 1243 public Request setInsurance(List<Reference> theInsurance) throws FHIRException; 1244 1245 /** 1246 * @return whether there is more than zero values for insurance 1247 */ 1248 public boolean hasInsurance(); 1249 /** 1250 * @return minimum allowed cardinality for insurance. Note that with patterns, this may be different for the underlying resource 1251 */ 1252 public int getInsuranceMin(); 1253 /** 1254 * @return maximum allowed cardinality for insurance. Note that with patterns, this may be different for the underlying resource 1255 */ 1256 public int getInsuranceMax(); 1257 1258 public Reference addInsurance() throws FHIRException; 1259 1260 public Request addInsurance(Reference t) throws FHIRException; 1261 1262 /** 1263 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 1264 */ 1265 public Reference getInsuranceFirstRep() throws FHIRException; 1266 1267 /** 1268 * @return {@link #supportingInfo} (Information that may be needed by/relevant to the performer in their execution of this {{title}}.) 1269 */ 1270 public List<Reference> getSupportingInfo() throws FHIRException; 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public Request setSupportingInfo(List<Reference> theSupportingInfo) throws FHIRException; 1276 1277 /** 1278 * @return whether there is more than zero values for supportingInfo 1279 */ 1280 public boolean hasSupportingInfo(); 1281 /** 1282 * @return minimum allowed cardinality for supportingInfo. Note that with patterns, this may be different for the underlying resource 1283 */ 1284 public int getSupportingInfoMin(); 1285 /** 1286 * @return maximum allowed cardinality for supportingInfo. Note that with patterns, this may be different for the underlying resource 1287 */ 1288 public int getSupportingInfoMax(); 1289 1290 public Reference addSupportingInfo() throws FHIRException; 1291 1292 public Request addSupportingInfo(Reference t) throws FHIRException; 1293 1294 /** 1295 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 1296 */ 1297 public Reference getSupportingInfoFirstRep() throws FHIRException; 1298 1299 /** 1300 * @return {@link #note} (Comments made about the {{title}} by the requester, performer, subject or other participants.) 1301 */ 1302 public List<Annotation> getNote() throws FHIRException; 1303 1304 /** 1305 * @return Returns a reference to <code>this</code> for easy method chaining 1306 */ 1307 public Request setNote(List<Annotation> theNote) throws FHIRException; 1308 1309 /** 1310 * @return whether there is more than zero values for note 1311 */ 1312 public boolean hasNote(); 1313 /** 1314 * @return minimum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 1315 */ 1316 public int getNoteMin(); 1317 /** 1318 * @return maximum allowed cardinality for note. Note that with patterns, this may be different for the underlying resource 1319 */ 1320 public int getNoteMax(); 1321 1322 public Annotation addNote() throws FHIRException; 1323 1324 public Request addNote(Annotation t) throws FHIRException; 1325 1326 /** 1327 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1328 */ 1329 public Annotation getNoteFirstRep() throws FHIRException; 1330 1331 /** 1332 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 1333 */ 1334 public List<Reference> getRelevantHistory() throws FHIRException; 1335 1336 /** 1337 * @return Returns a reference to <code>this</code> for easy method chaining 1338 */ 1339 public Request setRelevantHistory(List<Reference> theRelevantHistory) throws FHIRException; 1340 1341 /** 1342 * @return whether there is more than zero values for relevantHistory 1343 */ 1344 public boolean hasRelevantHistory(); 1345 /** 1346 * @return minimum allowed cardinality for relevantHistory. Note that with patterns, this may be different for the underlying resource 1347 */ 1348 public int getRelevantHistoryMin(); 1349 /** 1350 * @return maximum allowed cardinality for relevantHistory. Note that with patterns, this may be different for the underlying resource 1351 */ 1352 public int getRelevantHistoryMax(); 1353 1354 public Reference addRelevantHistory() throws FHIRException; 1355 1356 public Request addRelevantHistory(Reference t) throws FHIRException; 1357 1358 /** 1359 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 1360 */ 1361 public Reference getRelevantHistoryFirstRep() throws FHIRException; 1362 1363 public String fhirType(); 1364 1365 1366}