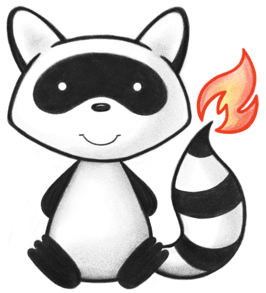
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.ActorDefinition; 010import org.hl7.fhir.r5.model.CapabilityStatement; 011import org.hl7.fhir.r5.model.Library; 012import org.hl7.fhir.r5.model.UrlType; 013import org.hl7.fhir.r5.renderers.utils.RenderingContext; 014import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 015import org.hl7.fhir.r5.utils.EOperationOutcome; 016import org.hl7.fhir.utilities.xhtml.XhtmlNode; 017 018public class ActorDefinitionRenderer extends ResourceRenderer { 019 020 021 public ActorDefinitionRenderer(RenderingContext context) { 022 super(context); 023 } 024 025 @Override 026 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper r) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 027 renderResourceTechDetails(r, x); 028 genSummaryTable(status, x, r); 029 render(status, x, r); 030 } 031 032 @Override 033 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 034 return canonicalTitle(r); 035 } 036 037 public void render(RenderingStatus status, XhtmlNode x, ResourceWrapper acd) throws FHIRFormatError, DefinitionException, IOException { 038 XhtmlNode tbl = x.table("grid"); 039 XhtmlNode tr = tbl.tr(); 040 tr.td().b().tx(context.formatPhrase(RenderingContext.ACTOR_DEF_ACT, context.getTranslated(acd.child("name"))) + " "); 041 tr.td().tx(context.getTranslated(acd.child("title"))); 042 tr.td().tx(context.formatPhrase(RenderingContext.ACTOR_DEF_TYP, acd.primitiveValue("type")) + " "); 043 XhtmlNode td = tbl.tr().td().colspan("3"); 044 addMarkdown(td, context.getTranslated(acd.child("documentation"))); 045 if (acd.has("reference")) { 046 tbl.tr().td().tx(context.formatPhrase(RenderingContext.GENERAL_REFS)); 047 td = tr.td().colspan("2"); 048 boolean first = true; 049 for (ResourceWrapper t : acd.children("reference")) { 050 if (first) first = false; else x.br(); 051 renderUri(status, td, t); 052 } 053 } 054 if (acd.has("capabilities")) { 055 tbl.tr().td().tx(context.formatPhrase(RenderingContext.ACTOR_DEF_CAP)); 056 td = tr.td().colspan("2"); 057 renderCanonical(status, td, acd.child("capabilities")); 058 } 059 if (acd.has("derivedFrom")) { 060 tbl.tr().td().tx(context.formatPhrase(RenderingContext.ACTOR_DEF_DER)); 061 td = tr.td().colspan("2"); 062 boolean first = true; 063 for (ResourceWrapper t : acd.children("reference")) { 064 if (first) first = false; else x.br(); 065 renderUri(status, td, t); 066 } 067 } 068 } 069 070 public void describe(XhtmlNode x, Library lib) { 071 x.tx(display(lib)); 072 } 073 074 public String display(Library lib) { 075 return lib.present(); 076 } 077 078}