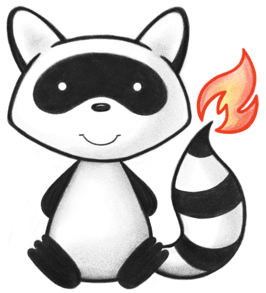
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRFormatError; 008import org.hl7.fhir.r5.model.CompartmentDefinition; 009import org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent; 010import org.hl7.fhir.r5.model.Resource; 011import org.hl7.fhir.r5.model.StringType; 012import org.hl7.fhir.r5.renderers.utils.BaseWrappers.ResourceWrapper; 013import org.hl7.fhir.r5.renderers.utils.RenderingContext; 014import org.hl7.fhir.r5.renderers.utils.Resolver.ResourceContext; 015import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 016import org.hl7.fhir.utilities.xhtml.XhtmlNode; 017import org.hl7.fhir.utilities.xhtml.XhtmlParser; 018 019public class CompartmentDefinitionRenderer extends ResourceRenderer { 020 021 public CompartmentDefinitionRenderer(RenderingContext context) { 022 super(context); 023 } 024 025 public CompartmentDefinitionRenderer(RenderingContext context, ResourceContext rcontext) { 026 super(context, rcontext); 027 } 028 029 public boolean render(XhtmlNode x, Resource dr) throws FHIRFormatError, DefinitionException, IOException { 030 return render(x, (CompartmentDefinition) dr); 031 } 032 033 public boolean render(XhtmlNode x, CompartmentDefinition cpd) throws FHIRFormatError, DefinitionException, IOException { 034 StringBuilder in = new StringBuilder(); 035 StringBuilder out = new StringBuilder(); 036 for (CompartmentDefinitionResourceComponent cc: cpd.getResource()) { 037 CommaSeparatedStringBuilder rules = new CommaSeparatedStringBuilder(); 038 if (!cc.hasParam()) { 039 out.append(" <li><a href=\"").append(cc.getCode().toLowerCase()).append(".html\">").append(cc.getCode()).append("</a></li>\r\n"); 040 } else if (!rules.equals("{def}")) { 041 for (StringType p : cc.getParam()) 042 rules.append(p.asStringValue()); 043 in.append(" <tr><td><a href=\"").append(cc.getCode().toLowerCase()).append(".html\">").append(cc.getCode()).append("</a></td><td>").append(rules.toString()).append("</td></tr>\r\n"); 044 } 045 } 046 XhtmlNode xn; 047 xn = new XhtmlParser().parseFragment("<div><p>\r\nThe following resources may be in this compartment:\r\n</p>\r\n" + 048 "<table class=\"grid\">\r\n"+ 049 " <tr><td><b>Resource</b></td><td><b>Inclusion Criteria</b></td></tr>\r\n"+ 050 in.toString()+ 051 "</table>\r\n"+ 052 "<p>\r\nA resource is in this compartment if the nominated search parameter (or chain) refers to the patient resource that defines the compartment.\r\n</p>\r\n" + 053 "<p>\r\n\r\n</p>\r\n" + 054 "<p>\r\nThe following resources are never in this compartment:\r\n</p>\r\n" + 055 "<ul>\r\n"+ 056 out.toString()+ 057 "</ul></div>\r\n"); 058 x.getChildNodes().addAll(xn.getChildNodes()); 059 return true; 060 } 061 062 public void describe(XhtmlNode x, CompartmentDefinition cd) { 063 x.tx(display(cd)); 064 } 065 066 public String display(CompartmentDefinition cd) { 067 return cd.present(); 068 } 069 070 @Override 071 public String display(Resource r) throws UnsupportedEncodingException, IOException { 072 return ((CompartmentDefinition) r).present(); 073 } 074 075 public String display(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 076 if (r.has("title")) { 077 return r.children("title").get(0).getBase().primitiveValue(); 078 } 079 if (r.has("name")) { 080 return r.children("name").get(0).getBase().primitiveValue(); 081 } 082 return "??"; 083 } 084 085}