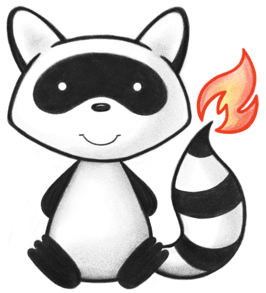
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.ImplementationGuide; 010import org.hl7.fhir.r5.renderers.utils.RenderingContext; 011import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 012import org.hl7.fhir.r5.utils.EOperationOutcome; 013import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 014import org.hl7.fhir.utilities.xhtml.XhtmlNode; 015 016@MarkedToMoveToAdjunctPackage 017public class ImplementationGuideRenderer extends ResourceRenderer { 018 019 public ImplementationGuideRenderer(RenderingContext context) { 020 super(context); 021 } 022 023 @Override 024 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper r) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 025 if (r.isDirect()) { 026 renderResourceTechDetails(r, x); 027 genSummaryTable(status, x, (ImplementationGuide) r.getBase()); 028 render(status, x, (ImplementationGuide) r.getBase()); 029 } else { 030 // the intention is to change this in the future 031 x.para().tx("ImplementationGuideRenderer only renders native resources directly"); 032 } 033 } 034 035 @Override 036 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 037 return canonicalTitle(r); 038 } 039 040 041 public void render(RenderingStatus status, XhtmlNode x, ImplementationGuide ig) throws FHIRFormatError, DefinitionException, IOException { 042 043 x.h2().addText(ig.getName()); 044 x.para().tx(context.formatPhrase(RenderingContext.IMP_GUIDE_URL)+" "); 045 x.pre().tx(ig.getUrl()); 046 addMarkdown(x, ig.getDescription()); 047 } 048 049 public void describe(XhtmlNode x, ImplementationGuide ig) { 050 x.tx(display(ig)); 051 } 052 053 public String display(ImplementationGuide ig) { 054 return ig.present(); 055 } 056 057 058}