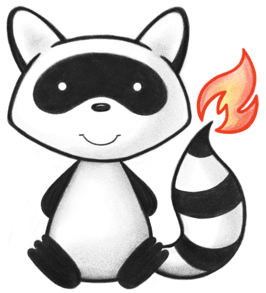
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.ListResource; 010import org.hl7.fhir.r5.renderers.utils.RenderingContext; 011import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 012import org.hl7.fhir.r5.utils.EOperationOutcome; 013import org.hl7.fhir.utilities.xhtml.XhtmlNode; 014 015public class ListRenderer extends ResourceRenderer { 016 017 public ListRenderer(RenderingContext context) { 018 super(context); 019 } 020 021 @Override 022 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 023 ResourceWrapper c = r.child("code"); 024 String cd = c == null ? context.formatPhrase(RenderingContext.LIST_UNSPECIFIED_CODE) : displayCodeableConcept(c); 025 ResourceWrapper s = r.child("subject"); 026 String sd = s == null ? context.formatPhrase(RenderingContext.LIST_UNSPECIFIED_SUBJECT) : displayReference(s); 027 return context.formatPhrase(RenderingContext.LIST_SUMMARY, cd, sd); 028 } 029 030 @Override 031 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper list) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 032 renderResourceTechDetails(list, x); 033 if (list.has("title")) { 034 x.h2().tx(list.primitiveValue("title")); 035 } 036 XhtmlNode t = x.table("clstu"); 037 XhtmlNode tr = t.tr(); 038 if (list.has("date")) { 039 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_DATE, displayDateTime(list.child("date")))+" "); 040 } 041 if (list.has("mode")) { 042 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_MODE, getTranslatedCode(list.child("mode")))+" "); 043 } 044 if (list.has("status")) { 045 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_STAT, getTranslatedCode(list.child("status")))+" "); 046 } 047 if (list.has("code")) { 048 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_CODE, displayDataType(list.child("code")))+" "); 049 } 050 tr = t.tr(); 051 XhtmlNode td = tr.td(); 052 if (list.has("subject")) { 053 td.tx(context.formatPhrase(RenderingContext.LIST_REND_SUB)+" "); 054 renderReference(status, td, list.child("subject")); 055 } 056 if (list.has("encounter")) { 057 td.tx(context.formatPhrase(RenderingContext.LIST_REND_ENC)+" "); 058 renderReference(status, td, list.child("encounter")); 059 } 060 if (list.has("source")) { 061 td.tx(context.formatPhrase(RenderingContext.GENERAL_SRC)+" "); 062 renderReference(status, td, list.child("encounter")); 063 } 064 if (list.has("orderedBy")) { 065 td.tx(context.formatPhrase(RenderingContext.LIST_REND_ORD, displayDataType(list.child("orderedBy")))+" "); 066 } 067 for (ResourceWrapper a : list.children("note")) { 068 renderAnnotation(status, x, a); 069 } 070 boolean flag = false; 071 boolean deleted = false; 072 boolean date = false; 073 for (ResourceWrapper e : list.children("entry")) { 074 flag = flag || e.has("flag"); 075 deleted = deleted || e.has("deleted"); 076 date = date || e.has("date"); 077 } 078 t = x.table("grid"); 079 tr = t.tr().style("backgound-color: #eeeeee"); 080 tr.td().b().tx(context.formatPhrase(RenderingContext.LIST_REND_ITEM)); 081 if (date) { 082 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_DAT)); 083 } 084 if (flag) { 085 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_FLAG)); 086 } 087 if (deleted) { 088 tr.td().tx(context.formatPhrase(RenderingContext.LIST_REND_DEL)); 089 } 090 for (ResourceWrapper e : list.children("entry")) { 091 tr = t.tr(); 092 renderReference(status, tr.td(), e.child("item")); 093 if (date) { 094 tr.td().tx(e.has("date") ? displayDateTime(e.child("date")) : ""); 095 } 096 if (flag) { 097 tr.td().tx(e.has("flag") ? displayDataType(e.child("flag")) : ""); 098 } 099 if (deleted) { 100 tr.td().tx(e.has("deleted") ? e.primitiveValue("deleted") : ""); 101 } 102 } 103 } 104 105 public void describe(XhtmlNode x, ListResource list) { 106 x.tx(display(list)); 107 } 108 109 public String display(ListResource list) { 110 return list.getTitle(); 111 } 112 113 114}