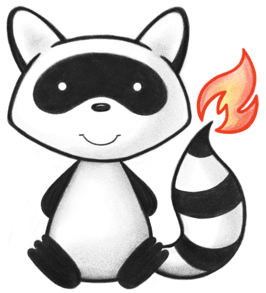
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.NamingSystem; 010import org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent; 011import org.hl7.fhir.r5.renderers.utils.RenderingContext; 012import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 013import org.hl7.fhir.r5.terminologies.CodeSystemUtilities; 014import org.hl7.fhir.r5.utils.EOperationOutcome; 015import org.hl7.fhir.r5.utils.ToolingExtensions; 016import org.hl7.fhir.utilities.xhtml.XhtmlNode; 017 018public class NamingSystemRenderer extends ResourceRenderer { 019 020 021 public NamingSystemRenderer(RenderingContext context) { 022 super(context); 023 } 024 025 @Override 026 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper r) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 027 if (r.isDirect()) { 028 renderResourceTechDetails(r, x); 029 genSummaryTable(status, x, (NamingSystem) r.getBase()); 030 render(status, x, (NamingSystem) r.getBase()); 031 } else { 032 // the intention is to change this in the future 033 x.para().tx("NamingSystemRenderer only renders native resources directly"); 034 } 035 } 036 037 @Override 038 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 039 return canonicalTitle(r); 040 } 041 042 043 044 public void render(RenderingStatus status, XhtmlNode x, NamingSystem ns) throws FHIRFormatError, DefinitionException, IOException { 045 x.h3().tx(context.formatPhrase(RenderingContext.GENERAL_SUMM)); 046 XhtmlNode tbl = x.table("grid"); 047 row(tbl, (context.formatPhrase(RenderingContext.GENERAL_DEFINING_URL)), ns.getUrl()); 048 if (ns.hasVersion()) { 049 row(tbl, (context.formatPhrase(RenderingContext.GENERAL_VER)), ns.getVersion()); 050 } 051 if (ns.hasName()) { 052 row(tbl, (context.formatPhrase(RenderingContext.GENERAL_NAME)), gt(ns.getNameElement())); 053 } 054 if (ns.hasTitle()) { 055 row(tbl, (context.formatPhrase(RenderingContext.GENERAL_TITLE)), gt(ns.getTitleElement())); 056 } 057 row(tbl, (context.formatPhrase(RenderingContext.GENERAL_STATUS)), ns.getStatus().toCode()); 058 if (ns.hasDescription()) { 059 addMarkdown(row(tbl, (context.formatPhrase(RenderingContext.GENERAL_DEFINITION))), ns.getDescription()); 060 } 061 if (ns.hasPublisher()) { 062 row(tbl, (context.formatPhrase(RenderingContext.CANON_REND_PUBLISHER)), gt(ns.getPublisherElement())); 063 } 064 if (ns.hasExtension(ToolingExtensions.EXT_WORKGROUP)) { 065 renderCommitteeLink(row(tbl, "Committee"), ns); 066 } 067 if (CodeSystemUtilities.hasOID(ns)) { 068 row(tbl, context.formatPhrase(RenderingContext.GENERAL_OID)).tx(context.formatPhrase(RenderingContext.CODE_SYS_FOR_OID, CodeSystemUtilities.getOID(ns))); 069 } 070 if (ns.hasCopyright()) { 071 addMarkdown(row(tbl, (context.formatPhrase(RenderingContext.GENERAL_COPYRIGHT))), ns.getCopyright()); 072 } 073 boolean hasPreferred = false; 074 boolean hasPeriod = false; 075 boolean hasComment = false; 076 for (NamingSystemUniqueIdComponent id : ns.getUniqueId()) { 077 hasPreferred = hasPreferred || id.hasPreferred(); 078 hasPeriod = hasPeriod || id.hasPeriod(); 079 hasComment = hasComment || id.hasComment(); 080 } 081 x.h3().tx(context.formatPhrase(RenderingContext.NAME_SYS_IDEN)); 082 tbl = x.table("grid"); 083 XhtmlNode tr = tbl.tr(); 084 tr.td().b().tx((context.formatPhrase(RenderingContext.GENERAL_TYPE))); 085 tr.td().b().tx((context.formatPhrase(RenderingContext.GENERAL_VALUE))); 086 if (hasPreferred) { 087 tr.td().b().tx((context.formatPhrase(RenderingContext.GENERAL_PREFERRED))); 088 } 089 if (hasPeriod) { 090 tr.td().b().tx((context.formatPhrase(RenderingContext.NAME_SYS_PER))); 091 } 092 if (hasComment) { 093 tr.td().b().tx((context.formatPhrase(RenderingContext.GENERAL_COMMENT))); 094 } 095 for (NamingSystemUniqueIdComponent id : ns.getUniqueId()) { 096 tr = tbl.tr(); 097 tr.td().tx(id.getType().getDisplay()); 098 tr.td().tx(id.getValue()); 099 if (hasPreferred) { 100 tr.td().tx(id.getPreferredElement().primitiveValue()); 101 } 102 if (hasPeriod) { 103 tr.td().tx(displayDataType(id.getPeriod())); 104 } 105 if (hasComment) { 106 tr.td().tx(id.getComment()); 107 } 108 } 109 } 110 111 private XhtmlNode row(XhtmlNode tbl, String name) { 112 XhtmlNode tr = tbl.tr(); 113 XhtmlNode td = tr.td(); 114 td.tx((name)); 115 return tr.td(); 116 } 117 private XhtmlNode row(XhtmlNode tbl, String name, String value) { 118 XhtmlNode td = row(tbl, name); 119 td.tx(value); 120 return td; 121 } 122 123 public void describe(XhtmlNode x, NamingSystem ns) { 124 x.tx(display(ns)); 125 } 126 127 public String display(NamingSystem ns) { 128 return ns.present(); 129 } 130 131}