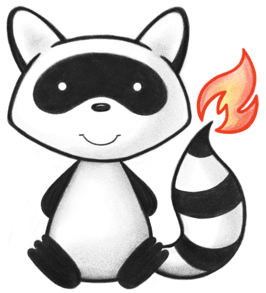
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.CanonicalType; 010import org.hl7.fhir.r5.model.Enumeration; 011import org.hl7.fhir.r5.model.Enumerations.FHIRTypes; 012import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAll; 013import org.hl7.fhir.r5.model.Extension; 014import org.hl7.fhir.r5.model.OperationDefinition; 015import org.hl7.fhir.r5.model.OperationDefinition.OperationDefinitionParameterComponent; 016import org.hl7.fhir.r5.model.OperationDefinition.OperationParameterScope; 017import org.hl7.fhir.r5.model.Resource; 018import org.hl7.fhir.r5.model.StructureDefinition; 019import org.hl7.fhir.r5.renderers.utils.RenderingContext; 020import org.hl7.fhir.r5.renderers.utils.RenderingContext.KnownLinkType; 021import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 022import org.hl7.fhir.r5.utils.EOperationOutcome; 023import org.hl7.fhir.r5.utils.ToolingExtensions; 024import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 025import org.hl7.fhir.utilities.StandardsStatus; 026import org.hl7.fhir.utilities.Utilities; 027import org.hl7.fhir.utilities.xhtml.XhtmlNode; 028 029public class OperationDefinitionRenderer extends TerminologyRenderer { 030 031 032 public OperationDefinitionRenderer(RenderingContext context) { 033 super(context); 034 } 035 036 @Override 037 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper r) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 038 if (r.isDirect()) { 039 renderResourceTechDetails(r, x); 040 genSummaryTable(status, x, (OperationDefinition) r.getBase()); 041 render(status, x, (OperationDefinition) r.getBase()); 042 } else { 043 // the intention is to change this in the future 044 x.para().tx("OperationDefinitionRenderer only renders native resources directly"); 045 } 046 } 047 048 @Override 049 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 050 return canonicalTitle(r); 051 } 052 053 public void render(RenderingStatus status, XhtmlNode x, OperationDefinition opd) throws IOException, FHIRException, EOperationOutcome { 054 if (context.isShowSummaryTable()) { 055 x.h2().addText(opd.getName()); 056 x.para().addText(Utilities.capitalize(opd.getKind().toString())+": "+opd.getName()); 057 x.para().tx(context.formatPhrase(RenderingContext.OP_DEF_OFFIC)+" "); 058 x.pre().tx(opd.getUrl()); 059 addMarkdown(x, opd.getDescription());} 060 061 if (opd.getSystem()) 062 x.para().tx(context.formatPhrase(RenderingContext.OP_DEF_URLS, opd.getCode())); 063 for (Enumeration<VersionIndependentResourceTypesAll> c : opd.getResource()) { 064 if (opd.getType()) 065 x.para().tx(context.formatPhrase(RenderingContext.OP_DEF_URL, c.getCode()+"/$"+opd.getCode())); 066 if (opd.getInstance()) 067 x.para().tx(context.formatPhrase(RenderingContext.OP_DEF_URL, c.getCode()+"/[id]/$"+opd.getCode())); 068 } 069 070 if (opd.hasInputProfile()) { 071 XhtmlNode p = x.para(); 072 p.tx(context.formatPhrase(RenderingContext.OP_DEF_INPAR)); 073 StructureDefinition sd = context.getContext().fetchResource(StructureDefinition.class, opd.getInputProfile(), opd); 074 if (sd == null) { 075 p.pre().tx(opd.getInputProfile()); 076 } else { 077 p.ah(context.prefixLocalHref(sd.getWebPath())).tx(sd.present()); 078 } 079 } 080 if (opd.hasOutputProfile()) { 081 XhtmlNode p = x.para(); 082 p.tx(context.formatPhrase(RenderingContext.OP_DEF_OUTPAR)); 083 StructureDefinition sd = context.getContext().fetchResource(StructureDefinition.class, opd.getOutputProfile(), opd); 084 if (sd == null) { 085 p.pre().tx(opd.getOutputProfile()); 086 } else { 087 p.ah(context.prefixLocalHref(sd.getWebPath())).tx(sd.present()); 088 } 089 } 090 091 x.h3().tx(context.formatPhrase(RenderingContext.GENERAL_PARS)); 092 //x.para().tx(context.formatPhrase(RenderingContext.GENERAL_PARS)); 093 XhtmlNode tbl = x.table( "grid"); 094 XhtmlNode tr = tbl.tr(); 095 tr.td().b().tx(context.formatPhrase(RenderingContext.OP_DEF_USE)); 096 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_NAME)); 097 tr.td().b().tx(context.formatPhrase(RenderingContext.OP_DEF_SCO)); 098 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_CARDINALITY)); 099 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_TYPE)); 100 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_BINDING)); 101 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_DOCUMENTATION)); 102 for (OperationDefinitionParameterComponent p : opd.getParameter()) { 103 genOpParam(tbl, "", p, opd); 104 } 105 addMarkdown(x, opd.getComment()); 106 } 107 108 public void describe(XhtmlNode x, OperationDefinition opd) { 109 x.tx(display(opd)); 110 } 111 112 public String display(OperationDefinition opd) { 113 return opd.present(); 114 } 115 116 @Override 117 public String display(Resource r) throws UnsupportedEncodingException, IOException { 118 return ((OperationDefinition) r).present(); 119 } 120 121 private void genOpParam(XhtmlNode tbl, String path, OperationDefinitionParameterComponent p, Resource opd) throws EOperationOutcome, FHIRException, IOException { 122 XhtmlNode tr; 123 tr = tbl.tr(); 124 tr.td().addText(p.getUse().toString()); 125 XhtmlNode td = tr.td(); 126 td.addText(path+p.getName()); 127 StandardsStatus ss = ToolingExtensions.getStandardsStatus(p); 128 genStandardsStatus(td, ss); 129 td = tr.td(); 130 if (p.hasScope()) { 131 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 132 for (Enumeration<OperationParameterScope> s : p.getScope()) { 133 b.append(s.getCode()); 134 } 135 td.tx(b.toString()); 136 } 137 tr.td().addText(Integer.toString(p.getMin())+".."+p.getMax()); 138 td = tr.td(); 139 String actualType = translateTypeToVersion(p.getTypeElement()); 140 StructureDefinition sd = actualType != null ? context.getWorker().fetchTypeDefinition(actualType) : null; 141 if (sd == null) 142 td.tx(p.hasType() ? actualType : ""); 143 else if (sd.getAbstract() && p.hasExtension(ToolingExtensions.EXT_ALLOWED_TYPE)) { 144 boolean first = true; 145 for (Extension ex : p.getExtensionsByUrl(ToolingExtensions.EXT_ALLOWED_TYPE)) { 146 if (first) first = false; else td.tx(" | "); 147 String s = ex.getValue().primitiveValue(); 148 StructureDefinition sdt = context.getWorker().fetchTypeDefinition(s); 149 if (sdt == null) 150 td.tx(p.hasType() ? actualType : ""); 151 else 152 td.ah(context.prefixLocalHref(sdt.getWebPath())).tx(s); 153 } 154 } else 155 td.ah(context.prefixLocalHref(sd.getWebPath())).tx(actualType); 156 if (p.hasTargetProfile()) { 157 td.tx(" ("); 158 boolean first = true; 159 for (CanonicalType tp : p.getTargetProfile()) { 160 if (first) { first = false;} else {td.tx(", ");}; 161 StructureDefinition sdt = context.getWorker().fetchTypeDefinition(tp.asStringValue()); 162 if (sdt == null || !sdt.hasWebPath()) { 163 td.code().tx(tp.asStringValue()); 164 } else { 165 td.ah(context.prefixLocalHref(sdt.getWebPath()), tp.asStringValue()).tx(sdt.present()); 166 } 167 } 168 td.tx(")"); 169 } 170 if (p.hasSearchType()) { 171 td.br(); 172 td.tx("("); 173 td.ah(context.prefixLocalHref(context.getLink(KnownLinkType.SPEC) == null ? "search.html#"+p.getSearchType().toCode() : Utilities.pathURL(context.getLink(KnownLinkType.SPEC), "search.html#"+p.getSearchType().toCode()))).tx(p.getSearchType().toCode()); 174 td.tx(")"); 175 } 176 td = tr.td(); 177 if (p.hasBinding() && p.getBinding().hasValueSet()) { 178 AddVsRef(p.getBinding().getValueSet(), td, opd); 179 td.tx(" ("+p.getBinding().getStrength().getDisplay()+")"); 180 } 181 addMarkdown(tr.td(), p.getDocumentation()); 182 if (!p.hasType()) { 183 for (OperationDefinitionParameterComponent pp : p.getPart()) { 184 genOpParam(tbl, path+p.getName()+".", pp, opd); 185 } 186 } 187 } 188 189 public static final String EXT_OPDEF_ORIGINAL_TYPE = "http://hl7.org/fhir/4.0/StructureDefinition/extension-OperationDefinition.parameter.type"; 190 191 private String translateTypeToVersion(Enumeration<FHIRTypes> src) { 192 if (src.hasExtension(EXT_OPDEF_ORIGINAL_TYPE)) { 193 return src.getExtensionString(EXT_OPDEF_ORIGINAL_TYPE); 194 } else { 195 return src.asStringValue(); 196 } 197 } 198 199 200}