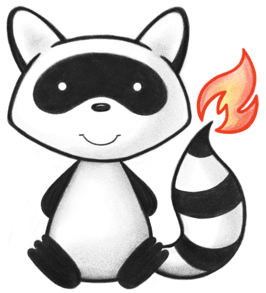
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005import java.util.List; 006 007import org.hl7.fhir.exceptions.DefinitionException; 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.exceptions.FHIRFormatError; 010import org.hl7.fhir.r5.renderers.utils.RenderingContext; 011import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 012import org.hl7.fhir.r5.utils.EOperationOutcome; 013import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 014import org.hl7.fhir.utilities.xhtml.XhtmlNode; 015 016@MarkedToMoveToAdjunctPackage 017public class ProvenanceRenderer extends ResourceRenderer { 018 019 public ProvenanceRenderer(RenderingContext context) { 020 super(context); 021 } 022 023 @Override 024 public String buildSummary(ResourceWrapper prv) throws UnsupportedEncodingException, IOException { 025 return (context.formatPhrase(RenderingContext.PROV_FOR, displayReference(prv.firstChild("target")))+" "); 026 } 027 028 @Override 029 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper prv) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 030 renderResourceTechDetails(prv, x); 031 032 if (prv.has("target")) { 033 List<ResourceWrapper> tl = prv.children("target"); 034 if (tl.size() == 1) { 035 XhtmlNode p = x.para(); 036 p.tx(context.formatPhrase(RenderingContext.PROV_PROV)+" "); 037 renderReference(status, p, tl.get(0)); 038 } else { 039 x.para().tx(context.formatPhrase(RenderingContext.PROV_PROVE)+" "); 040 XhtmlNode ul = x.ul(); 041 for (ResourceWrapper ref : tl) { 042 renderReference(status, ul.li(), ref); 043 } 044 } 045 } 046 // summary table 047 x.para().tx(context.formatPhrase(RenderingContext.GENERAL_SUMM)); 048 XhtmlNode t = x.table("grid", false); 049 XhtmlNode tr; 050 if (prv.has("occurred")) { 051 tr = t.tr(); 052 tr.td().tx(context.formatPhrase(RenderingContext.PROV_OCC)); 053 renderDataType(status, tr.td(), prv.child("occurred")); 054 } 055 if (prv.has("recorded")) { 056 tr = t.tr(); 057 tr.td().tx(context.formatPhrase(RenderingContext.PROV_REC)); 058 renderDataType(status, tr.td(), prv.child("recorded")); 059 } 060 if (prv.has("policy")) { 061 List<ResourceWrapper> tl = prv.children("policy"); 062 tr = t.tr(); 063 tr.td().tx(context.formatPhrase(RenderingContext.PROV_POL)); 064 if (tl.size() == 1) { 065 renderDataType(status, tr.td(), tl.get(0)); 066 } else { 067 XhtmlNode ul = tr.td().ul(); 068 for (ResourceWrapper u : tl) { 069 renderDataType(status, ul.li(), u); 070 } 071 } 072 } 073 if (prv.has("location")) { 074 tr = t.tr(); 075 tr.td().tx(context.formatPhrase(RenderingContext.GENERAL_LOCATION)); 076 renderDataType(status, tr.td(), prv.child("location")); 077 } 078 if (prv.has("activity")) { 079 tr = t.tr(); 080 tr.td().tx(context.formatPhrase(RenderingContext.PROV_ACT)); 081 renderDataType(status, tr.td(), prv.child("activity")); 082 } 083 084 boolean hasType = false; 085 boolean hasRole = false; 086 boolean hasOnBehalfOf = false; 087 for (ResourceWrapper a : prv.children("agent")) { 088 hasType = hasType || a.has("type"); 089 hasRole = hasRole || a.has("role"); 090 hasOnBehalfOf = hasOnBehalfOf || a.has("onBehalfOf"); 091 } 092 x.para().b().tx(context.formatPhrase(RenderingContext.PROV_AGE)); 093 t = x.table("grid", false); 094 tr = t.tr(); 095 if (hasType) { 096 tr.td().b().tx(context.formatPhrase(RenderingContext.GENERAL_TYPE)); 097 } 098 if (hasRole) { 099 tr.td().b().tx(context.formatPhrase(RenderingContext.PROV_ROLE)); 100 } 101 tr.td().b().tx(context.formatPhrase(RenderingContext.PROV_WHO)); 102 if (hasOnBehalfOf) { 103 tr.td().b().tx(context.formatPhrase(RenderingContext.PROV_BEHALF)); 104 } 105 for (ResourceWrapper a : prv.children("agent")) { 106 tr = t.tr(); 107 if (hasType) { 108 if (a.has("type")) { 109 renderDataType(status, tr.td(), a.child("type")); 110 } else { 111 tr.td(); 112 } 113 } 114 if (hasRole) { 115 List<ResourceWrapper> tl = prv.children("role"); 116 if (tl.size() == 0) { 117 tr.td(); 118 } else if (tl.size() == 1) { 119 renderCodeableConcept(status, tr.td(), tl.get(0)); 120 } else { 121 XhtmlNode ul = tr.td().ul(); 122 for (ResourceWrapper cc : tl) { 123 renderCodeableConcept(status, ul.li(), cc); 124 } 125 } 126 } 127 if (a.has("who")) { 128 renderReference(status, tr.td(), a.child("who")); 129 } else { 130 tr.td(); 131 } 132 if (hasOnBehalfOf) { 133 if (a.has("onBehalfOf")) { 134 renderReference(status, tr.td(), a.child("onBehalfOf")); 135 } else { 136 tr.td(); 137 } 138 } 139 } 140 // agent table 141 142 } 143 144 145}