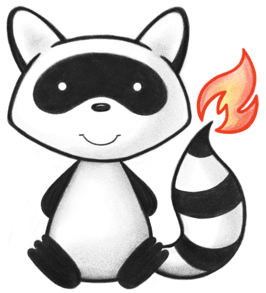
001package org.hl7.fhir.r5.renderers; 002 003import java.io.IOException; 004import java.io.UnsupportedEncodingException; 005 006import org.hl7.fhir.exceptions.DefinitionException; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.r5.model.CanonicalResource; 010import org.hl7.fhir.r5.model.MarkdownType; 011import org.hl7.fhir.r5.renderers.utils.RenderingContext; 012import org.hl7.fhir.r5.renderers.utils.ResourceWrapper; 013import org.hl7.fhir.r5.utils.EOperationOutcome; 014import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 015import org.hl7.fhir.utilities.xhtml.XhtmlNode; 016 017@MarkedToMoveToAdjunctPackage 018public class SubscriptionTopicRenderer extends ResourceRenderer { 019 020 public SubscriptionTopicRenderer(RenderingContext context) { 021 super(context); 022 } 023 024 @Override 025 public String buildSummary(ResourceWrapper r) throws UnsupportedEncodingException, IOException { 026 return canonicalTitle(r); 027 } 028 029 @Override 030 public void buildNarrative(RenderingStatus status, XhtmlNode x, ResourceWrapper st) throws FHIRFormatError, DefinitionException, IOException, FHIRException, EOperationOutcome { 031 renderResourceTechDetails(st, x); 032 genSummaryTable(status, x, (CanonicalResource) st.getResourceNative()); 033 034 XhtmlNode tbl = x.table("grid", false); 035 XhtmlNode ttr = tbl.tr(); 036 ttr.td().b().tx("SubscriptionTopic"); 037 ttr.td().tx(context.getTranslated(st.has("title") ? st.child("title") : st.child("name"))); 038 if (st.has("description")) { 039 ttr = tbl.tr(); 040 ttr.td().b().tx("Description"); 041 addMarkdown(ttr.td(), st.primitiveValue("description")); 042 } 043 if (st.has("copyright")) { 044 generateCopyrightTableRow(tbl, st); 045 } 046 047 048 if (st.has("resourceTrigger")) { 049 TableData td = new TableData(context.formatPhrase(RenderingContext.SUB_TOPIC_RES_TRIG)); 050 for (ResourceWrapper rt : st.children("resourceTrigger")) { 051 TableRowData tr = td.addRow(); 052 if (rt.has("resource")) { 053 tr.value(context.formatPhrase(RenderingContext.GENERAL_RESOURCE), rt.child("resource")); 054 } 055 for (ResourceWrapper t : rt.children("supportedInteraction")) { 056 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_INT), t); 057 } 058 if (rt.has("queryCriteria")) { 059 StringBuilder md = new StringBuilder(); 060 ResourceWrapper qc = rt.child("queryCriteria"); 061 if (qc.has("previous")) { 062 md.append(context.formatPhrase(RenderingContext.SUB_TOPIC_PREV, qc.primitiveValue("previous")+"\r\n")+" "); 063 } 064 if (qc.has("resultForCreate")) { 065 md.append(context.formatPhrase(RenderingContext.SUB_TOPIC_CREATE, qc.primitiveValue("resultForCreate")+"\r\n")+" "); 066 } 067 if (qc.has("current")) { 068 md.append(context.formatPhrase(RenderingContext.SUB_TOPIC_CREATE, qc.primitiveValue("current")+"\r\n")+" "); 069 } 070 if (qc.has("previous")) { 071 md.append(context.formatPhrase(RenderingContext.SUB_TOPIC_DELETE, qc.primitiveValue("resultForDelete")+"\r\n")+" "); 072 } 073 if (qc.has("requireBoth")) { 074 md.append(context.formatPhrase(RenderingContext.SUB_TOPIC_REQ, qc.primitiveValue("requireBoth")+"\r\n")+" "); 075 } 076 tr.value(context.formatPhrase(RenderingContext.GENERAL_CRIT), wrapNC(new MarkdownType(md.toString()))); 077 } 078 if (rt.has("fhirPathCriteria")) { 079 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_FHIR_PATH), rt.child("fhirPathCriteria")); 080 } 081 if (rt.has("description")) { 082 tr.value(context.formatPhrase(RenderingContext.GENERAL_DESC), rt.child("description")); 083 } 084 } 085 renderTable(status, td, x); 086 } 087 088 if (st.has("eventTrigger")) { 089 TableData td = new TableData("Event Triggers"); 090 for (ResourceWrapper rt : st.children("eventTrigger")) { 091 TableRowData tr = td.addRow(); 092 if (rt.has("resource")) { 093 tr.value(context.formatPhrase(RenderingContext.GENERAL_RESOURCE), rt.child("resource")); 094 } 095 if (rt.has("event(")) { 096 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_EVENT), rt.child("event")); 097 } 098 if (rt.has("description")) { 099 tr.value(context.formatPhrase(RenderingContext.GENERAL_DESC), rt.child("description")); 100 } 101 } 102 renderTable(status, td, x); 103 } 104 105 if (st.has("canFilterBy")) { 106 TableData td = new TableData("Can Filter By"); 107 for (ResourceWrapper rt : st.children("canFilterBy")) { 108 TableRowData tr = td.addRow(); 109 if (rt.has("resource")) { 110 tr.value(context.formatPhrase(RenderingContext.GENERAL_RESOURCE), rt.child("resource")); 111 } 112 if (rt.has("filterParameter")) { 113 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_FILT_PAR), rt.child("filterParameter")); 114 } 115 if (rt.has("filterDefinition")) { 116 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_FILT_DEF), rt.child("filterDefinition")); 117 } 118 for (ResourceWrapper t : rt.children("comparator")) { 119 tr.value(context.formatPhrase(RenderingContext.GENERAL_COMPARATORS), t); 120 } 121 for (ResourceWrapper t : rt.children("modifier")) { 122 tr.value(context.formatPhrase(RenderingContext.GENERAL_MODIFIERS), t); 123 } 124 } 125 renderTable(status, td, x); 126 } 127 128 if (st.has("notificationShape")) { 129 TableData td = new TableData("Notification Shapes"); 130 for (ResourceWrapper rt : st.children("notificationShape")) { 131 TableRowData tr = td.addRow(); 132 if (rt.has("resource")) { 133 tr.value(context.formatPhrase(RenderingContext.GENERAL_RESOURCE), rt.child("resource")); 134 } 135 for (ResourceWrapper t : rt.children("include")) { 136 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_INCL), t); 137 } 138 for (ResourceWrapper t : rt.children("revInclude")) { 139 tr.value(context.formatPhrase(RenderingContext.SUB_TOPIC_REV_INCL), t); 140 } 141 } 142 renderTable(status, td, x); 143 } 144 } 145 146}