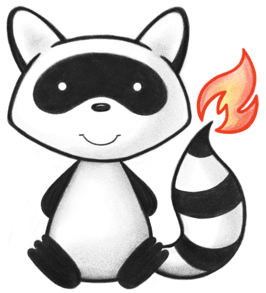
001package org.hl7.fhir.r5.terminologies; 002 003import java.util.ArrayList; 004import java.util.HashMap; 005import java.util.List; 006import java.util.Map; 007 008import org.hl7.fhir.r5.model.Enumerations.FHIRVersion; 009 010public class TerminologyServerDetails { 011 public enum ServerAuthorizationMethod { 012 OPEN, 013 TOKEN, 014 SMART_ON_FHIR 015 } 016 private String name; 017 private ServerAuthorizationMethod auth; 018 private Map<FHIRVersion, String> endpoints = new HashMap<>(); 019 private List<String> codeSystems = new ArrayList<>(); 020 021 public boolean handlesSystem(String uri, String version) { 022 for (String s : codeSystems) { 023 if (s.contains("|")) { 024 String u = s.substring(0, s.lastIndexOf("|")); 025 String v = s.substring(s.lastIndexOf("|")+1); 026 if (v.equals(version) && (s.equals(uri) || uri.matches(s))) { 027 return true; 028 } 029 } else { 030 if (s.equals(uri) || uri.matches(s)) { 031 return true; 032 } 033 } 034 } 035 return false; 036 } 037 038 public String getName() { 039 return name; 040 } 041 042 public ServerAuthorizationMethod getAuth() { 043 return auth; 044 } 045 046 public Map<FHIRVersion, String> getEndpoints() { 047 return endpoints; 048 } 049 050 public List<String> getCodeSystems() { 051 return codeSystems; 052 } 053 054 055}