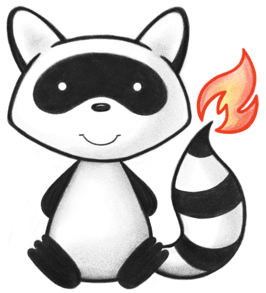
001package org.hl7.fhir.r5.terminologies; 002 003import java.util.HashSet; 004import java.util.List; 005import java.util.Set; 006 007import org.hl7.fhir.r5.model.CanonicalResource; 008import org.hl7.fhir.r5.model.Identifier; 009import org.hl7.fhir.r5.model.ValueSet; 010import org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent; 011import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 012import org.hl7.fhir.utilities.Utilities; 013 014 015public class TerminologyUtilities { 016 017 public static Set<String> listOids(CanonicalResource cr) { 018 Set<String> oids = new HashSet<>(); 019 020 if (cr.hasUrl() && cr.getUrl().startsWith("urn:oid:")) { 021 oids.add(cr.getUrl().substring(8)); 022 } 023 024 for (Identifier id : cr.getIdentifier()) { 025 String v = id.getValue(); 026 if (v != null && v.startsWith("urn:oid:")) { 027 oids.add(v.substring(8)); 028 } 029 } 030 return oids; 031 } 032 033 public static List<String> listSystems(ValueSet vs) { 034 Set<String> res = new HashSet<>(); 035 for (ConceptSetComponent inc : vs.getCompose().getInclude()) { 036 if (inc.hasSystem()) { 037 if (inc.hasVersion()) { 038 res.add(inc.getSystem()+"|"+inc.getVersion()); 039 } else { 040 res.add(inc.getSystem()); 041 } 042 } 043 } 044 for (ConceptSetComponent inc : vs.getCompose().getExclude()) { 045 if (inc.hasSystem()) { 046 if (inc.hasVersion()) { 047 res.add(inc.getSystem()+"|"+inc.getVersion()); 048 } else { 049 res.add(inc.getSystem()); 050 } 051 } 052 } 053 return Utilities.sorted(res); 054 } 055}