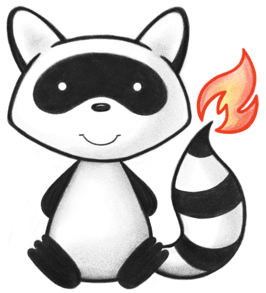
001package org.hl7.fhir.r5.terminologies.utilities; 002 003import org.hl7.fhir.r5.model.Coding; 004import org.hl7.fhir.r5.model.ValueSet; 005import org.hl7.fhir.r5.terminologies.utilities.TerminologyCache.CacheToken; 006 007public class CodingValidationRequest { 008 private Coding coding; 009 private ValidationResult result; 010 private CacheToken cacheToken; 011 private String vs; 012 private ValueSet vsObj; 013 014 public CodingValidationRequest(Coding coding) { 015 super(); 016 this.coding = coding; 017 } 018 019 public CodingValidationRequest(Coding coding, String vs) { 020 super(); 021 this.coding = coding; 022 this.vs = vs; 023 } 024 025 public CodingValidationRequest(Coding coding, ValueSet vsObj) { 026 super(); 027 this.coding = coding; 028 this.vsObj = vsObj; 029 } 030 031 public String getVs() { 032 return vs; 033 } 034 035 public ValueSet getVsObj() { 036 return vsObj; 037 } 038 039 public ValidationResult getResult() { 040 return result; 041 } 042 043 public void setResult(ValidationResult result) { 044 this.result = result; 045 } 046 047 public Coding getCoding() { 048 return coding; 049 } 050 051 public boolean hasResult() { 052 return result != null; 053 } 054 055 /** 056 * internal logic; external users of batch validation should ignore this property 057 * 058 * @return 059 */ 060 public CacheToken getCacheToken() { 061 return cacheToken; 062 } 063 064 /** 065 * internal logic; external users of batch validation should ignore this property 066 * 067 * @param cacheToken 068 */ 069 public void setCacheToken(CacheToken cacheToken) { 070 this.cacheToken = cacheToken; 071 } 072 073 074}