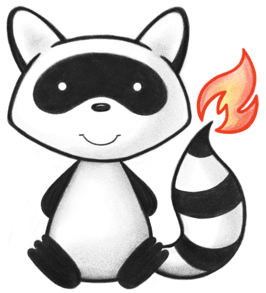
001package org.hl7.fhir.r5.terminologies.utilities; 002 003import org.hl7.fhir.r5.model.Parameters; 004import org.hl7.fhir.r5.model.Parameters.ParametersParameterComponent; 005import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 006import org.hl7.fhir.utilities.Utilities; 007 008 009//URL: http://snomed.info/sct/[module]/version/[e.g. 20150131]' 010//International: 900000000000207008 011//US: 731000124108 012//Australia: 32506021000036107 013//Belgium: 11000172109 014//Canada: 20611000087101 015//Spain: 449081005 016//Denmark: 554471000005108 017//Netherlands: 11000146104 018//Sweden: 45991000052106 019//Switzerland: 2011000195101 020//UK: 83821000000107 021//IPS: 827022005 022 023@MarkedToMoveToAdjunctPackage 024public class SnomedUtilities { 025 026 public static String getVersionFromParameters(Parameters p, String version) { 027 for (ParametersParameterComponent pp : p.getParameter()) { 028 switch (pp.getName()) { 029 case "system-version" : 030 if (version == null) { 031 return pp.getValue().primitiveValue(); 032 } 033 case "force-system-version": 034 return pp.getValue().primitiveValue(); 035 } 036 } 037 return version; 038 } 039 040 public static String getEditionFromVersion(String version) { 041 if (version == null) { 042 return null; 043 } 044 if (version.startsWith("http://snomed.info/sct/")) { 045 version = version.substring(23); 046 } 047 if (version.contains("/")) { 048 version = version.substring(0, version.indexOf("/")); 049 } 050 if (Utilities.existsInList(version, "900000000000207008", "449081005", "11000221109", "32506021000036107", "11000234105", "11000172109", 051 "20621000087109", "20611000087101", "554471000005108", "11000181102", "11000229106", 052 "11000274103", "1121000189102", "11000220105", "11000146104", "21000210109", "51000202101", 053 "11000267109", "900000001000122104", "45991000052106", "2011000195101", "83821000000107", 054 "999000021000000109", "5631000179106", "731000124108", "599100012410")) { 055 return version; 056 } else { 057 return null; 058 } 059 } 060 061 public static String getSctLink(String version, String code, Parameters p) { 062 if (!Utilities.noString(code) && !code.contains(":")) { 063 version = SnomedUtilities.getVersionFromParameters(p, version); 064 String edId = SnomedUtilities.getEditionFromVersion(version); 065 if (edId != null) { 066 // if there's a version that's an edition, then: 067 // http://snomed.info/sct/11000172109/id//371305003 068 return "http://snomed.info/sct/"+edId+"/id/"+code; 069 } else { 070 // no, version: 071 return "http://snomed.info/id/"+code; 072 } 073 } else { 074 return "https://browser.ihtsdotools.org/"; 075 } 076 } 077} 078 079