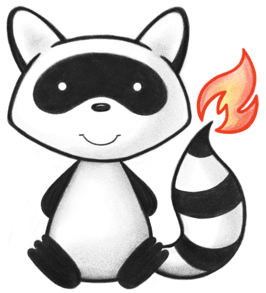
001package org.hl7.fhir.r5.terminologies.validation; 002 003import java.util.List; 004 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.model.OperationOutcome.OperationOutcomeIssueComponent; 007import org.hl7.fhir.r5.terminologies.utilities.TerminologyServiceErrorClass; 008import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 009 010@MarkedToMoveToAdjunctPackage 011public class VSCheckerException extends FHIRException { 012 013 private List<OperationOutcomeIssueComponent> issues; 014 private boolean warning; 015 private TerminologyServiceErrorClass type; 016 017 public VSCheckerException(String message, List<OperationOutcomeIssueComponent> issues) { 018 super(message); 019 this.issues = issues; 020 } 021 022 public VSCheckerException(String message, List<OperationOutcomeIssueComponent> issues, boolean warning) { 023 super(message); 024 this.issues = issues; 025 this.warning = warning; 026 } 027 028 public VSCheckerException(String message, List<OperationOutcomeIssueComponent> issues, TerminologyServiceErrorClass type) { 029 super(message); 030 this.issues = issues; 031 this.type = type; 032 } 033 034 public List<OperationOutcomeIssueComponent> getIssues() { 035 return issues; 036 } 037 038 private static final long serialVersionUID = -5889505119633054187L; 039 040 public boolean isWarning() { 041 return warning; 042 } 043 044 public TerminologyServiceErrorClass getType() { 045 return type; 046 } 047 048 public void setType(TerminologyServiceErrorClass type) { 049 this.type = type; 050 } 051 052}