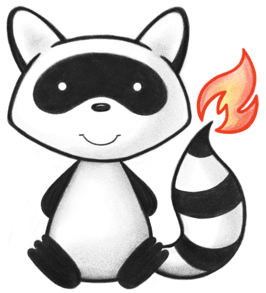
001package org.hl7.fhir.r5.terminologies.validation; 002 003import org.hl7.fhir.exceptions.FHIRException; 004import org.hl7.fhir.r5.model.Parameters.ParametersParameterComponent; 005 006public class VersionInfo { 007 /** 008 * 009 */ 010 private final ValueSetValidator valueSetCheckerSimple; 011 012 /** 013 * @param valueSetCheckerSimple 014 */ 015 VersionInfo(ValueSetValidator valueSetCheckerSimple) { 016 this.valueSetCheckerSimple = valueSetCheckerSimple; 017 } 018 019 private String expansionVersion; 020 private String composeVersion; 021 022 public String getExpansionVersion() { 023 return expansionVersion; 024 } 025 026 public void setExpansionVersion(String expansionVersion) { 027 this.expansionVersion = expansionVersion; 028 } 029 030 public String getComposeVersion() { 031 return composeVersion; 032 } 033 034 public void setComposeVersion(String composeVersion) { 035 this.composeVersion = composeVersion; 036 } 037 038 public String getVersion(String system, String version) { 039 String fixedVersion = getVersionParameter("force-system-version", system); 040 if (fixedVersion != null) { 041 return fixedVersion; 042 } 043 String checkVersion = getVersionParameter("check-system-version", system); 044 if (version != null) { 045 if (checkVersion != null && !version.equals(checkVersion)) { 046 throw new FHIRException("Attempt to use version "+version+" of "+system+", when the expansion parameters limit the use to "+checkVersion); 047 } 048 return version; 049 } 050 if (expansionVersion != null) { 051 if (checkVersion != null && !expansionVersion.equals(checkVersion)) { 052 throw new FHIRException("Attempt to use version "+expansionVersion+" of "+system+", when the expansion parameters limit the use to "+checkVersion); 053 } 054 return expansionVersion; 055 } 056 if (composeVersion != null) { 057 if (checkVersion != null && !composeVersion.equals(checkVersion)) { 058 throw new FHIRException("Attempt to use version "+composeVersion+" of "+system+", when the expansion parameters limit the use to "+checkVersion); 059 } 060 return composeVersion; 061 } 062 return getVersionParameter("system-version", system); 063 } 064 065 private String getVersionParameter(String name, String system) { 066 if (this.valueSetCheckerSimple.expansionProfile != null) { 067 for (ParametersParameterComponent pc : this.valueSetCheckerSimple.expansionProfile.getParameter()) { 068 if (name.equals(pc.getName()) && pc.hasValue()) { 069 String v = pc.getValue().primitiveValue(); 070 if (v != null && v.startsWith(system+"|")) { 071 return v.substring(system.length()+1); 072 } 073 } 074 } 075 } 076 return null; 077 } 078 079}