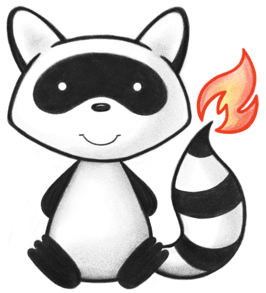
001package org.hl7.fhir.r5.test.utils; 002 003 import java.io.IOException; 004import java.io.InputStream; 005import java.util.ArrayList; 006import java.util.List; 007 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.r5.context.IContextResourceLoader; 010import org.hl7.fhir.r5.formats.JsonParser; 011import org.hl7.fhir.r5.formats.XmlParser; 012import org.hl7.fhir.r5.model.Bundle; 013import org.hl7.fhir.r5.model.CodeSystem; 014import org.hl7.fhir.r5.model.Resource; 015import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 016import org.hl7.fhir.r5.terminologies.client.TerminologyClientR5.TerminologyClientR5Factory; 017import org.hl7.fhir.utilities.npm.NpmPackage; 018import org.hl7.fhir.utilities.npm.NpmPackage.PackageResourceInformation; 019 020public class TestPackageLoader implements IContextResourceLoader { 021 022 private List<String> types; 023 024 public TestPackageLoader(List<String> types) { 025 this.types = types; 026 } 027 028 @Override 029 public Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException { 030 return null; 031 } 032 033 @Override 034 public Resource loadResource(InputStream stream, boolean isJson) throws FHIRException, IOException { 035 return isJson ? new JsonParser().parse(stream) : new XmlParser().parse(stream); 036 } 037 038 @Override 039 public List<String> getTypes() { 040 return types; 041 } 042 043 @Override 044 public String getResourcePath(Resource resource) { 045 return resource.fhirType().toLowerCase()+"-"+resource.getId()+".html"; 046 } 047 048 @Override 049 public IContextResourceLoader getNewLoader(NpmPackage npm) { 050 return this; 051 } 052 053 @Override 054 public List<CodeSystem> getCodeSystems() { 055 return new ArrayList<>(); 056 } 057 058 @Override 059 public void setPatchUrls(boolean value) { 060 061 } 062 063 @Override 064 public String patchUrl(String url, String resourceType) { 065 return url; 066 } 067 068 @Override 069 public IContextResourceLoader setLoadProfiles(boolean value) { 070 return this; 071 } 072 073 @Override 074 public boolean wantLoad(NpmPackage pi, PackageResourceInformation pri) { 075 return true; 076 } 077 078 @Override 079 public ITerminologyClientFactory txFactory() { 080 return new TerminologyClientR5Factory(); 081 } 082 083}