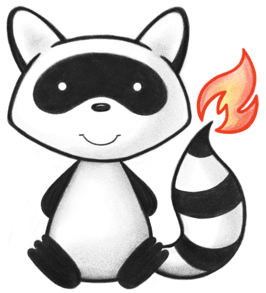
001package org.hl7.fhir.r5.test.utils; 002 003import java.io.File; 004import java.io.IOException; 005import java.io.InputStream; 006import java.util.HashMap; 007import java.util.Map; 008 009import org.fhir.ucum.UcumEssenceService; 010import org.hl7.fhir.r5.context.IWorkerContext; 011import org.hl7.fhir.r5.context.SimpleWorkerContext; 012import org.hl7.fhir.r5.context.TerminologyCache; 013import org.hl7.fhir.r5.model.Parameters; 014import org.hl7.fhir.r5.utils.R5Hacker; 015import org.hl7.fhir.utilities.Utilities; 016import org.hl7.fhir.utilities.VersionUtilities; 017import org.hl7.fhir.utilities.npm.BasePackageCacheManager.InputStreamWithSrc; 018import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager; 019import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager.FilesystemPackageCacheMode; 020import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager.IPackageProvider; 021import org.hl7.fhir.utilities.npm.NpmPackage; 022import org.hl7.fhir.utilities.npm.ToolsVersion; 023import org.hl7.fhir.utilities.tests.BaseTestingUtilities; 024import org.hl7.fhir.utilities.tests.TestConfig; 025 026public class TestingUtilities extends BaseTestingUtilities { 027 028 public static class PackageProvider implements IPackageProvider { 029 030 @Override 031 public boolean handlesPackage(String id, String version) { 032 return id.equals("hl7.fhir.r5.core"); 033 } 034 035 @Override 036 public InputStreamWithSrc provide(String id, String version) throws IOException { 037 return new InputStreamWithSrc(TestingUtilities.loadR5CorePackageSource(), "Test Case Repository", "5.0.0"); 038 } 039 040 } 041 042 static public Map<String, IWorkerContext> fcontexts; 043 044 final static public String DEFAULT_CONTEXT_VERSION = "5.0.0"; 045 046 /** Get an existing instantiation of a WorkerContext if available 047 * 048 * This uses the DEFAULT_CONTEXT_VERSION 049 * */ 050 public static IWorkerContext getSharedWorkerContext() { 051 return getSharedWorkerContext(DEFAULT_CONTEXT_VERSION); 052 } 053 054 /** 055 * Get an existing instantiation of a WorkerContext if available 056 * 057 * @param version FHIR Version to get context for 058 * @return 059 */ 060 public static IWorkerContext getSharedWorkerContext(String version) { 061 if (!Utilities.existsInList(version, "1.0.2", "3.0.1", "4.0.1", "4.3.0", "5.0.0")) { 062 throw new Error("illegal version: "+version); 063 064 } 065 066 String v = VersionUtilities.getMajMin(version); 067 if (fcontexts == null) { 068 fcontexts = new HashMap<>(); 069 } 070 if (!fcontexts.containsKey(v)) { 071 IWorkerContext fcontext = getWorkerContext(version); 072 fcontexts.put(v, fcontext); 073 } 074 return fcontexts.get(v); 075 } 076 077 public static IWorkerContext getWorkerContext(String version) { 078 079 FilesystemPackageCacheManager pcm; 080 try { 081 pcm = new FilesystemPackageCacheManager(org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager.FilesystemPackageCacheMode.USER); 082 IWorkerContext fcontext = null; 083 if (VersionUtilities.isR5Ver(version)) { 084 // for purposes of stability, the R5 core package comes from the test case repository 085 fcontext = getWorkerContext(loadR5CorePackage()); 086 } else { 087 fcontext = getWorkerContext(pcm.loadPackage(VersionUtilities.packageForVersion(version), version)); 088 } 089 fcontext.setUcumService(new UcumEssenceService(TestingUtilities.loadTestResourceStream("ucum", "ucum-essence.xml"))); 090 fcontext.setExpansionProfile(new Parameters()); 091 if (!fcontext.hasPackage("hl7.terminology.r5", null)) { 092 NpmPackage utg = pcm.loadPackage("hl7.terminology.r5"); 093 System.out.println("Loading THO: "+utg.name()+"#"+utg.version()); 094 fcontext.loadFromPackage(utg, new TestPackageLoader(Utilities.strings("CodeSystem", "ValueSet"))); 095 } 096 if (!fcontext.hasPackage("hl7.fhir.uv.extensions", null)) { 097 NpmPackage ext = pcm.loadPackage("hl7.fhir.uv.extensions", "1.0.0"); 098 System.out.println("Loading Extensions: "+ext.name()+"#"+ext.version()); 099 fcontext.loadFromPackage(ext, new TestPackageLoader(Utilities.strings("CodeSystem", "ValueSet", "StructureDefinition"))); 100 } 101 return fcontext; 102 } catch (Exception e) { 103 e.printStackTrace(); 104 throw new Error(e); 105 } 106 } 107 108 public static NpmPackage loadR5CorePackage() throws IOException { 109 return NpmPackage.fromPackage(loadR5CorePackageSource()); 110 } 111 112 private static InputStream loadR5CorePackageSource() throws IOException { 113 return TestingUtilities.loadTestResourceStream("r5", "packages", "hl7.fhir.r5.core.tgz"); 114 } 115 116 public static String getTerminologyCacheDirectory() { 117 return TestConfig.getInstance().getTxCacheDirectory("org.hl7.fhir.r5"); 118 } 119 120 public static SimpleWorkerContext getWorkerContext(NpmPackage npmPackage) throws Exception { 121 SimpleWorkerContext swc = new SimpleWorkerContext.SimpleWorkerContextBuilder().withAllowLoadingDuplicates(true).withUserAgent(TestConstants.USER_AGENT) 122 .withTerminologyCachePath(getTerminologyCacheDirectory()).fromPackage(npmPackage); 123 TerminologyCache.setCacheErrors(true); 124 return swc; 125 } 126 127 public static SimpleWorkerContext getWorkerContext(NpmPackage npmPackage, IWorkerContext.IContextResourceLoader loader) throws Exception { 128 SimpleWorkerContext swc = new SimpleWorkerContext.SimpleWorkerContextBuilder().withAllowLoadingDuplicates(true).withUserAgent(TestConstants.USER_AGENT) 129 .withTerminologyCachePath(getTerminologyCacheDirectory()).fromPackage(npmPackage, loader, true); 130 TerminologyCache.setCacheErrors(true); 131 return swc; 132 } 133 134 static public String fixedpath; 135 static public String contentpath; 136 137 public static String home() { 138 if (fixedpath != null) 139 return fixedpath; 140 String s = System.getenv("FHIR_HOME"); 141 if (!Utilities.noString(s)) 142 return s; 143 s = "C:\\work\\org.hl7.fhir\\build"; 144 // FIXME: change this back 145 s = "/Users/jamesagnew/git/fhir"; 146 if (new File(s).exists()) 147 return s; 148 throw new Error("FHIR Home directory not configured"); 149 } 150 151 152 public static String content() throws IOException { 153 if (contentpath != null) 154 return contentpath; 155 String s = "R:\\fhir\\publish"; 156 if (new File(s).exists()) 157 return s; 158 return Utilities.path(home(), "publish"); 159 } 160 161 // diretory that contains all the US implementation guides 162 public static String us() { 163 if (fixedpath != null) 164 return fixedpath; 165 String s = System.getenv("FHIR_HOME"); 166 if (!Utilities.noString(s)) 167 return s; 168 s = "C:\\work\\org.hl7.fhir.us"; 169 if (new File(s).exists()) 170 return s; 171 throw new Error("FHIR US directory not configured"); 172 } 173 174 public static void injectCorePackageLoader() { 175 FilesystemPackageCacheManager.setPackageProvider(new TestingUtilities.PackageProvider()); 176 } 177 178 179}