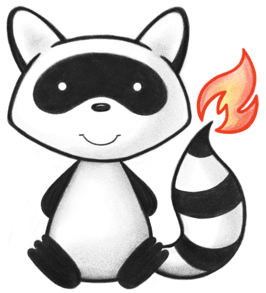
001package org.hl7.fhir.r5.testfactory; 002 003import java.util.ArrayList; 004import java.util.HashMap; 005import java.util.List; 006import java.util.Map; 007 008import org.apache.commons.lang3.NotImplementedException; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.exceptions.PathEngineException; 011import org.hl7.fhir.r5.context.SimpleWorkerContext; 012import org.hl7.fhir.r5.fhirpath.ExpressionNode.CollectionStatus; 013import org.hl7.fhir.r5.fhirpath.FHIRPathEngine; 014import org.hl7.fhir.r5.fhirpath.FHIRPathEngine.IEvaluationContext; 015import org.hl7.fhir.r5.fhirpath.FHIRPathUtilityClasses.FunctionDetails; 016import org.hl7.fhir.r5.fhirpath.TypeDetails; 017import org.hl7.fhir.r5.liquid.GlobalObject; 018import org.hl7.fhir.r5.model.Base; 019import org.hl7.fhir.r5.model.DateTimeType; 020import org.hl7.fhir.r5.model.StringType; 021import org.hl7.fhir.r5.model.ValueSet; 022import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 023 024@MarkedToMoveToAdjunctPackage 025public class TestDataHostServices implements IEvaluationContext { 026 027 private SimpleWorkerContext context; 028 private DateTimeType dt; 029 private StringType pathToSpec; 030 private Map<String, FunctionDefinition> functions = new HashMap<>(); 031 032 public TestDataHostServices(SimpleWorkerContext context, DateTimeType dt, StringType pathToSpec) { 033 super(); 034 this.context = context; 035 this.dt = dt; 036 this.pathToSpec = pathToSpec; 037 } 038 039 public TestDataHostServices registerFunction(FunctionDefinition function) { 040 functions.put(function.name(), function); 041 return this; 042 } 043 044 @Override 045 public List<Base> resolveConstant(FHIRPathEngine engine, Object appContext, String name, boolean beforeContext, boolean explicitConstant) throws PathEngineException { 046 if ("Globals".equals(name)) { 047 List<Base> list = new ArrayList<Base>(); 048 list.add(new GlobalObject(dt, pathToSpec)); 049 return list; 050 } else { 051 return new ArrayList<>(); 052 } 053 } 054 055 @Override 056 public TypeDetails resolveConstantType(FHIRPathEngine engine, Object appContext, String name, boolean explicitConstant) throws PathEngineException { 057 if ("Globals".equals(name)) { 058 return new TypeDetails(CollectionStatus.SINGLETON, "GlobalObject"); 059 } else { 060 return null; // whatever it is, we don't know about it. 061 } 062 } 063 064 @Override 065 public boolean paramIsType(String name, int index) { 066 return false; 067 } 068 069 @Override 070 public boolean log(String argument, List<Base> focus) { 071 return false; 072 } 073 074 @Override 075 public FunctionDetails resolveFunction(FHIRPathEngine engine, String functionName) { 076 FunctionDefinition fd = functions.get(functionName); 077 return fd == null ? null : fd.details(); 078 } 079 080 @Override 081 public TypeDetails checkFunction(FHIRPathEngine engine, Object appContext, String functionName, TypeDetails focus, List<TypeDetails> parameters) throws PathEngineException { 082 FunctionDefinition fd = functions.get(functionName); 083 return fd == null ? null : fd.check(engine, appContext, focus, parameters); 084 } 085 086 @Override 087 public List<Base> executeFunction(FHIRPathEngine engine, Object appContext, List<Base> focus, String functionName, List<List<Base>> parameters) { 088 FunctionDefinition fd = functions.get(functionName); 089 return fd == null ? null : fd.execute(engine, appContext, focus, parameters); 090 } 091 092 @Override 093 public Base resolveReference(FHIRPathEngine engine, Object appContext, String url, Base refContext) { 094 return null; 095 } 096 097 @Override 098 public boolean conformsToProfile(FHIRPathEngine engine, Object appContext, Base item, String url) throws FHIRException { 099 throw new NotImplementedException("Not done yet (TestDataHostServices.conformsToProfile)"); 100 } 101 102 @Override 103 public ValueSet resolveValueSet(FHIRPathEngine engine, Object appContext, String url) { 104 throw new NotImplementedException("Not done yet (TestDataHostServices.resolveValueSet)"); // cause I don't know when we 'd need to do this 105 } 106 107}