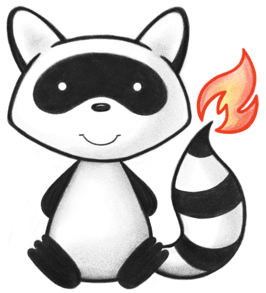
001package org.hl7.fhir.r5.testfactory.dataprovider; 002 003import java.io.FileInputStream; 004import java.io.FileNotFoundException; 005import java.io.IOException; 006import java.util.ArrayList; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.utilities.CSVReader; 011import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 012 013@MarkedToMoveToAdjunctPackage 014public class CSVDataProvider extends TableDataProvider { 015 016 private CSVReader csv; 017 private List<String> cols = new ArrayList<String>(); 018 private int counter; 019 private String filename; 020 021 protected CSVDataProvider(String filename) throws FHIRException, FileNotFoundException, IOException { 022 super(); 023 024 this.filename = filename; 025 reset(); 026 } 027 028 @Override 029 public List<String> columns() { 030 return cols; 031 } 032 033 @Override 034 public boolean nextRow() throws FHIRException { 035 try { 036 counter++; 037 return csv.line(); 038 } catch (IOException e) { 039 throw new FHIRException("Error moving to next row: " + e.getMessage(), e); 040 } 041 } 042 043 @Override 044 public List<String> cells() { 045 List<String> cells = new ArrayList<String>(); 046 cells.add(""+counter); 047 for (String s : csv.getCells()) { 048 cells.add(s.trim()); 049 } 050 return cells; 051 } 052 053 @Override 054 public String cell(String name) { 055 if ("counter".equals(name)) { 056 return ""+counter; 057 } else { 058 return csv.cell(name).trim(); 059 } 060 } 061 062 @Override 063 public void reset() throws FHIRException { 064 try { 065 this.csv = new CSVReader(new FileInputStream(filename)); 066 067 cols.add("counter"); 068 for (String s : csv.readHeaders()) { 069 cols.add(s.trim()); 070 } 071 } catch (Exception e) { 072 throw new FHIRException("Error moving to next row: " + e.getMessage(), e); 073 } 074 } 075 076}