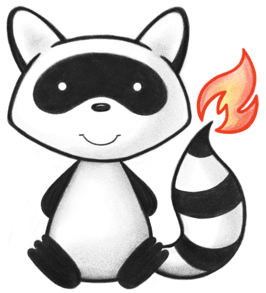
001package org.hl7.fhir.r5.testfactory.dataprovider; 002 003import java.sql.DriverManager; 004import java.util.List; 005import java.util.Locale; 006 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 009import org.hl7.fhir.utilities.Utilities; 010 011@MarkedToMoveToAdjunctPackage 012public abstract class TableDataProvider { 013 014 public abstract List<String> columns(); 015 public abstract boolean nextRow() throws FHIRException; 016 public abstract List<String> cells() throws FHIRException; 017 public abstract String cell(String name) throws FHIRException; 018 public abstract void reset() throws FHIRException; 019 020 021 public static TableDataProvider forFile(String path, Locale locale) throws FHIRException { 022 try { 023 String filename = path; 024 String sheetname = null; 025 String range = null; 026 027 if (path.contains("!")) { 028 range = path.substring(path.indexOf("!")+1); 029 path = path.substring(0, path.indexOf("!")); 030 } 031 if (path.contains(";")) { 032 filename = path.substring(0, path.indexOf(";")); 033 sheetname = path.substring(path.indexOf(";")+1); 034 } 035 String extension = Utilities.getFileExtension(filename); 036 if (Utilities.existsInList(extension, "csv", "txt")) { 037 return new CSVDataProvider(filename); 038 } else if (Utilities.existsInList(extension, "xlsx")) { 039 return new ExcelDataProvider(filename, sheetname, range, locale); 040 } else if (Utilities.existsInList(extension, "db")) { 041 return new SQLDataProvider(DriverManager.getConnection("jdbc:sqlite:"+filename), sheetname); 042 } else { 043 throw new FHIRException("Unknown File Type "+path); 044 } 045 } catch (Exception e) { 046 throw new FHIRException(e); 047 } 048 } 049 050}