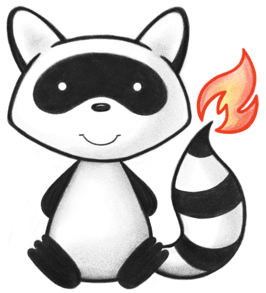
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks order-select context. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHookOrderSelectContext") 052public class CDSHookOrderSelectContext extends CDSHookContext implements ICompositeType { 053 054 /** 055 * For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 056 */ 057 @Child(name = "userId", type = {UrlType.class}, order=0, min=1, max=1, modifier=false, summary=false) 058 @Description(shortDefinition="The id of the current user. Must be in the format [ResourceType]/[id].", formalDefinition="For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123." ) 059 protected UrlType userId; 060 061 /** 062 * The FHIR Patient.id of the current patient in context 063 */ 064 @Child(name = "patientId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 065 @Description(shortDefinition="The FHIR Patient.id of the current patient in context", formalDefinition="The FHIR Patient.id of the current patient in context" ) 066 protected IdType patientId; 067 068 /** 069 * The FHIR Encounter.id of the current encounter in context 070 */ 071 @Child(name = "encounterId", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=false) 072 @Description(shortDefinition="The FHIR Encounter.id of the current encounter in context", formalDefinition="The FHIR Encounter.id of the current encounter in context" ) 073 protected IdType encounterId; 074 075 /** 076 * The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103 077 */ 078 @Child(name = "selections", type = {UriType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 079 @Description(shortDefinition="The FHIR ids of the newly selected order(s)", formalDefinition="The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103" ) 080 protected List<UriType> selectionsList; 081 082 /** 083 * FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status 084 */ 085 @Child(name = "draftOrders", type = {Bundle.class}, order=4, min=1, max=1, modifier=false, summary=false) 086 @Description(shortDefinition="FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", formalDefinition="FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status" ) 087 protected Bundle draftOrders; 088 089 private static final long serialVersionUID = -1513767262L; 090 091 /** 092 * Constructor 093 */ 094 public CDSHookOrderSelectContext() { 095 super(); 096 } 097 098 /** 099 * Constructor 100 */ 101 public CDSHookOrderSelectContext(String userId, String patientId, Bundle draftOrders) { 102 super(); 103 this.setUserId(userId); 104 this.setPatientId(patientId); 105 this.setDraftOrders(draftOrders); 106 } 107 108 /** 109 * @return {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 110 */ 111 public UrlType getUserIdElement() { 112 if (this.userId == null) 113 if (Configuration.errorOnAutoCreate()) 114 throw new Error("Attempt to auto-create CDSHookOrderSelectContext.userId"); 115 else if (Configuration.doAutoCreate()) 116 this.userId = new UrlType(); // bb 117 return this.userId; 118 } 119 120 public boolean hasUserIdElement() { 121 return this.userId != null && !this.userId.isEmpty(); 122 } 123 124 public boolean hasUserId() { 125 return this.userId != null && !this.userId.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 130 */ 131 public CDSHookOrderSelectContext setUserIdElement(UrlType value) { 132 this.userId = value; 133 return this; 134 } 135 136 /** 137 * @return For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 138 */ 139 public String getUserId() { 140 return this.userId == null ? null : this.userId.getValue(); 141 } 142 143 /** 144 * @param value For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 145 */ 146 public CDSHookOrderSelectContext setUserId(String value) { 147 if (this.userId == null) 148 this.userId = new UrlType(); 149 this.userId.setValue(value); 150 return this; 151 } 152 153 /** 154 * @return {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 155 */ 156 public IdType getPatientIdElement() { 157 if (this.patientId == null) 158 if (Configuration.errorOnAutoCreate()) 159 throw new Error("Attempt to auto-create CDSHookOrderSelectContext.patientId"); 160 else if (Configuration.doAutoCreate()) 161 this.patientId = new IdType(); // bb 162 return this.patientId; 163 } 164 165 public boolean hasPatientIdElement() { 166 return this.patientId != null && !this.patientId.isEmpty(); 167 } 168 169 public boolean hasPatientId() { 170 return this.patientId != null && !this.patientId.isEmpty(); 171 } 172 173 /** 174 * @param value {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 175 */ 176 public CDSHookOrderSelectContext setPatientIdElement(IdType value) { 177 this.patientId = value; 178 return this; 179 } 180 181 /** 182 * @return The FHIR Patient.id of the current patient in context 183 */ 184 public String getPatientId() { 185 return this.patientId == null ? null : this.patientId.getValue(); 186 } 187 188 /** 189 * @param value The FHIR Patient.id of the current patient in context 190 */ 191 public CDSHookOrderSelectContext setPatientId(String value) { 192 if (this.patientId == null) 193 this.patientId = new IdType(); 194 this.patientId.setValue(value); 195 return this; 196 } 197 198 /** 199 * @return {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 200 */ 201 public IdType getEncounterIdElement() { 202 if (this.encounterId == null) 203 if (Configuration.errorOnAutoCreate()) 204 throw new Error("Attempt to auto-create CDSHookOrderSelectContext.encounterId"); 205 else if (Configuration.doAutoCreate()) 206 this.encounterId = new IdType(); // bb 207 return this.encounterId; 208 } 209 210 public boolean hasEncounterIdElement() { 211 return this.encounterId != null && !this.encounterId.isEmpty(); 212 } 213 214 public boolean hasEncounterId() { 215 return this.encounterId != null && !this.encounterId.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 220 */ 221 public CDSHookOrderSelectContext setEncounterIdElement(IdType value) { 222 this.encounterId = value; 223 return this; 224 } 225 226 /** 227 * @return The FHIR Encounter.id of the current encounter in context 228 */ 229 public String getEncounterId() { 230 return this.encounterId == null ? null : this.encounterId.getValue(); 231 } 232 233 /** 234 * @param value The FHIR Encounter.id of the current encounter in context 235 */ 236 public CDSHookOrderSelectContext setEncounterId(String value) { 237 if (Utilities.noString(value)) 238 this.encounterId = null; 239 else { 240 if (this.encounterId == null) 241 this.encounterId = new IdType(); 242 this.encounterId.setValue(value); 243 } 244 return this; 245 } 246 247 /** 248 * @return {@link #selections} (The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103) 249 */ 250 public List<UriType> getSelectionsList() { 251 if (this.selectionsList == null) 252 this.selectionsList = new ArrayList<UriType>(); 253 return this.selectionsList; 254 } 255 256 /** 257 * @return Returns a reference to <code>this</code> for easy method chaining 258 */ 259 public CDSHookOrderSelectContext setSelectionsList(List<UriType> theSelections) { 260 this.selectionsList = theSelections; 261 return this; 262 } 263 264 public boolean hasSelections() { 265 if (this.selectionsList == null) 266 return false; 267 for (UriType item : this.selectionsList) 268 if (!item.isEmpty()) 269 return true; 270 return false; 271 } 272 273 /** 274 * @return {@link #selections} (The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103) 275 */ 276 public UriType addSelectionsElement() {//2 277 UriType t = new UriType(); 278 if (this.selectionsList == null) 279 this.selectionsList = new ArrayList<UriType>(); 280 this.selectionsList.add(t); 281 return t; 282 } 283 284 /** 285 * @param value {@link #selections} (The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103) 286 */ 287 public CDSHookOrderSelectContext addSelections(String value) { //1 288 UriType t = new UriType(); 289 t.setValue(value); 290 if (this.selectionsList == null) 291 this.selectionsList = new ArrayList<UriType>(); 292 this.selectionsList.add(t); 293 return this; 294 } 295 296 /** 297 * @param value {@link #selections} (The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103) 298 */ 299 public boolean hasSelections(String value) { 300 if (this.selectionsList == null) 301 return false; 302 for (UriType v : this.selectionsList) 303 if (v.getValue().equals(value)) // uri 304 return true; 305 return false; 306 } 307 308 /** 309 * @return {@link #draftOrders} (FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status) 310 */ 311 public Bundle getDraftOrders() { 312 if (this.draftOrders == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create CDSHookOrderSelectContext.draftOrders"); 315 else if (Configuration.doAutoCreate()) 316 this.draftOrders = new Bundle(); // cc 317 return this.draftOrders; 318 } 319 320 public boolean hasDraftOrders() { 321 return this.draftOrders != null && !this.draftOrders.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #draftOrders} (FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status) 326 */ 327 public CDSHookOrderSelectContext setDraftOrders(Bundle value) { 328 this.draftOrders = value; 329 return this; 330 } 331 332 protected void listChildren(List<Property> children) { 333 super.listChildren(children); 334 children.add(new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId)); 335 children.add(new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId)); 336 children.add(new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId)); 337 children.add(new Property("selections", "uri", "The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103", 0, java.lang.Integer.MAX_VALUE, selectionsList)); 338 children.add(new Property("draftOrders", "Bundle", "FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", 0, 1, draftOrders)); 339 } 340 341 @Override 342 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 343 switch (_hash) { 344 case -836030906: /*userId*/ return new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId); 345 case -343587072: /*patientId*/ return new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId); 346 case 107147694: /*encounterId*/ return new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId); 347 case -1655324569: /*selections*/ return new Property("selections", "uri", "The selections field references FHIR resources in the draftOrders Bundle. For example, MedicationRequest/103", 0, java.lang.Integer.MAX_VALUE, selectionsList); 348 case 155667430: /*draftOrders*/ return new Property("draftOrders", "Bundle", "FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", 0, 1, draftOrders); 349 default: return super.getNamedProperty(_hash, _name, _checkValid); 350 } 351 352 } 353 354 @Override 355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 356 switch (hash) { 357 case -836030906: /*userId*/ return this.userId == null ? new Base[0] : new Base[] {this.userId}; // UrlType 358 case -343587072: /*patientId*/ return this.patientId == null ? new Base[0] : new Base[] {this.patientId}; // IdType 359 case 107147694: /*encounterId*/ return this.encounterId == null ? new Base[0] : new Base[] {this.encounterId}; // IdType 360 case -1655324569: /*selections*/ return this.selectionsList == null ? new Base[0] : this.selectionsList.toArray(new Base[this.selectionsList.size()]); // UriType 361 case 155667430: /*draftOrders*/ return this.draftOrders == null ? new Base[0] : new Base[] {this.draftOrders}; // Bundle 362 default: return super.getProperty(hash, name, checkValid); 363 } 364 365 } 366 367 @Override 368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 369 switch (hash) { 370 case -836030906: // userId 371 this.userId = TypeConvertor.castToUrl(value); // UrlType 372 return value; 373 case -343587072: // patientId 374 this.patientId = TypeConvertor.castToId(value); // IdType 375 return value; 376 case 107147694: // encounterId 377 this.encounterId = TypeConvertor.castToId(value); // IdType 378 return value; 379 case -1655324569: // selections 380 this.getSelectionsList().add(TypeConvertor.castToUri(value)); // UriType 381 return value; 382 case 155667430: // draftOrders 383 this.draftOrders = (Bundle) value; // Bundle 384 return value; 385 default: return super.setProperty(hash, name, value); 386 } 387 388 } 389 390 @Override 391 public Base setProperty(String name, Base value) throws FHIRException { 392 if (name.equals("userId")) { 393 this.userId = TypeConvertor.castToUrl(value); // UrlType 394 } else if (name.equals("patientId")) { 395 this.patientId = TypeConvertor.castToId(value); // IdType 396 } else if (name.equals("encounterId")) { 397 this.encounterId = TypeConvertor.castToId(value); // IdType 398 } else if (name.equals("selections")) { 399 this.getSelectionsList().add(TypeConvertor.castToUri(value)); // UriType 400 } else if (name.equals("draftOrders")) { 401 this.draftOrders = (Bundle) value; // Bundle 402 } else 403 return super.setProperty(name, value); 404 return value; 405 } 406 407 @Override 408 public Base makeProperty(int hash, String name) throws FHIRException { 409 switch (hash) { 410 case -836030906: return getUserIdElement(); 411 case -343587072: return getPatientIdElement(); 412 case 107147694: return getEncounterIdElement(); 413 case -1655324569: return addSelectionsElement(); 414 case 155667430: return getDraftOrders(); 415 default: return super.makeProperty(hash, name); 416 } 417 418 } 419 420 @Override 421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 422 switch (hash) { 423 case -836030906: /*userId*/ return new String[] {"url"}; 424 case -343587072: /*patientId*/ return new String[] {"id"}; 425 case 107147694: /*encounterId*/ return new String[] {"id"}; 426 case -1655324569: /*selections*/ return new String[] {"uri"}; 427 case 155667430: /*draftOrders*/ return new String[] {"Bundle"}; 428 default: return super.getTypesForProperty(hash, name); 429 } 430 431 } 432 433 @Override 434 public Base addChild(String name) throws FHIRException { 435 if (name.equals("userId")) { 436 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSelectContext.userId"); 437 } 438 else if (name.equals("patientId")) { 439 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSelectContext.patientId"); 440 } 441 else if (name.equals("encounterId")) { 442 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSelectContext.encounterId"); 443 } 444 else if (name.equals("selections")) { 445 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSelectContext.selections"); 446 } 447 else if (name.equals("draftOrders")) { 448 this.draftOrders = new Bundle(); 449 return this.draftOrders; 450 } 451 else 452 return super.addChild(name); 453 } 454 455 public String fhirType() { 456 return "CDSHookOrderSelectContext"; 457 458 } 459 460 public CDSHookOrderSelectContext copy() { 461 CDSHookOrderSelectContext dst = new CDSHookOrderSelectContext(); 462 copyValues(dst); 463 return dst; 464 } 465 466 public void copyValues(CDSHookOrderSelectContext dst) { 467 super.copyValues(dst); 468 dst.userId = userId == null ? null : userId.copy(); 469 dst.patientId = patientId == null ? null : patientId.copy(); 470 dst.encounterId = encounterId == null ? null : encounterId.copy(); 471 if (selectionsList != null) { 472 dst.selectionsList = new ArrayList<UriType>(); 473 for (UriType i : selectionsList) 474 dst.selectionsList.add(i.copy()); 475 }; 476 dst.draftOrders = draftOrders == null ? null : draftOrders.copy(); 477 } 478 479 protected CDSHookOrderSelectContext typedCopy() { 480 return copy(); 481 } 482 483 @Override 484 public boolean equalsDeep(Base other_) { 485 if (!super.equalsDeep(other_)) 486 return false; 487 if (!(other_ instanceof CDSHookOrderSelectContext)) 488 return false; 489 CDSHookOrderSelectContext o = (CDSHookOrderSelectContext) other_; 490 return compareDeep(userId, o.userId, true) && compareDeep(patientId, o.patientId, true) && compareDeep(encounterId, o.encounterId, true) 491 && compareDeep(selectionsList, o.selectionsList, true) && compareDeep(draftOrders, o.draftOrders, true) 492 ; 493 } 494 495 @Override 496 public boolean equalsShallow(Base other_) { 497 if (!super.equalsShallow(other_)) 498 return false; 499 if (!(other_ instanceof CDSHookOrderSelectContext)) 500 return false; 501 CDSHookOrderSelectContext o = (CDSHookOrderSelectContext) other_; 502 return compareValues(userId, o.userId, true) && compareValues(patientId, o.patientId, true) && compareValues(encounterId, o.encounterId, true) 503 && compareValues(selectionsList, o.selectionsList, true); 504 } 505 506 public boolean isEmpty() { 507 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(userId, patientId, encounterId 508 , selectionsList, draftOrders); 509 } 510 511 512} 513