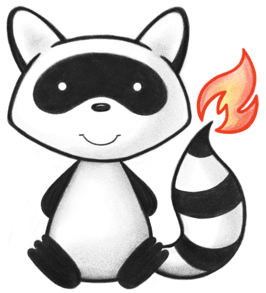
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Order-Sign context. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHookOrderSignContext") 052public class CDSHookOrderSignContext extends CDSHookContext implements ICompositeType { 053 054 /** 055 * For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 056 */ 057 @Child(name = "userId", type = {UrlType.class}, order=0, min=1, max=1, modifier=false, summary=false) 058 @Description(shortDefinition="The id of the current user. Must be in the format [ResourceType]/[id].", formalDefinition="For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123." ) 059 protected UrlType userId; 060 061 /** 062 * The FHIR Patient.id of the current patient in context 063 */ 064 @Child(name = "patientId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 065 @Description(shortDefinition="The FHIR Patient.id of the current patient in context", formalDefinition="The FHIR Patient.id of the current patient in context" ) 066 protected IdType patientId; 067 068 /** 069 * The FHIR Encounter.id of the current encounter in context 070 */ 071 @Child(name = "encounterId", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=false) 072 @Description(shortDefinition="The FHIR Encounter.id of the current encounter in context", formalDefinition="The FHIR Encounter.id of the current encounter in context" ) 073 protected IdType encounterId; 074 075 /** 076 * FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status 077 */ 078 @Child(name = "draftOrders", type = {Bundle.class}, order=3, min=1, max=1, modifier=false, summary=false) 079 @Description(shortDefinition="FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", formalDefinition="FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status" ) 080 protected Bundle draftOrders; 081 082 private static final long serialVersionUID = -590647101L; 083 084 /** 085 * Constructor 086 */ 087 public CDSHookOrderSignContext() { 088 super(); 089 } 090 091 /** 092 * Constructor 093 */ 094 public CDSHookOrderSignContext(String userId, String patientId, Bundle draftOrders) { 095 super(); 096 this.setUserId(userId); 097 this.setPatientId(patientId); 098 this.setDraftOrders(draftOrders); 099 } 100 101 /** 102 * @return {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 103 */ 104 public UrlType getUserIdElement() { 105 if (this.userId == null) 106 if (Configuration.errorOnAutoCreate()) 107 throw new Error("Attempt to auto-create CDSHookOrderSignContext.userId"); 108 else if (Configuration.doAutoCreate()) 109 this.userId = new UrlType(); // bb 110 return this.userId; 111 } 112 113 public boolean hasUserIdElement() { 114 return this.userId != null && !this.userId.isEmpty(); 115 } 116 117 public boolean hasUserId() { 118 return this.userId != null && !this.userId.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 123 */ 124 public CDSHookOrderSignContext setUserIdElement(UrlType value) { 125 this.userId = value; 126 return this; 127 } 128 129 /** 130 * @return For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 131 */ 132 public String getUserId() { 133 return this.userId == null ? null : this.userId.getValue(); 134 } 135 136 /** 137 * @param value For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 138 */ 139 public CDSHookOrderSignContext setUserId(String value) { 140 if (this.userId == null) 141 this.userId = new UrlType(); 142 this.userId.setValue(value); 143 return this; 144 } 145 146 /** 147 * @return {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 148 */ 149 public IdType getPatientIdElement() { 150 if (this.patientId == null) 151 if (Configuration.errorOnAutoCreate()) 152 throw new Error("Attempt to auto-create CDSHookOrderSignContext.patientId"); 153 else if (Configuration.doAutoCreate()) 154 this.patientId = new IdType(); // bb 155 return this.patientId; 156 } 157 158 public boolean hasPatientIdElement() { 159 return this.patientId != null && !this.patientId.isEmpty(); 160 } 161 162 public boolean hasPatientId() { 163 return this.patientId != null && !this.patientId.isEmpty(); 164 } 165 166 /** 167 * @param value {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 168 */ 169 public CDSHookOrderSignContext setPatientIdElement(IdType value) { 170 this.patientId = value; 171 return this; 172 } 173 174 /** 175 * @return The FHIR Patient.id of the current patient in context 176 */ 177 public String getPatientId() { 178 return this.patientId == null ? null : this.patientId.getValue(); 179 } 180 181 /** 182 * @param value The FHIR Patient.id of the current patient in context 183 */ 184 public CDSHookOrderSignContext setPatientId(String value) { 185 if (this.patientId == null) 186 this.patientId = new IdType(); 187 this.patientId.setValue(value); 188 return this; 189 } 190 191 /** 192 * @return {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 193 */ 194 public IdType getEncounterIdElement() { 195 if (this.encounterId == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create CDSHookOrderSignContext.encounterId"); 198 else if (Configuration.doAutoCreate()) 199 this.encounterId = new IdType(); // bb 200 return this.encounterId; 201 } 202 203 public boolean hasEncounterIdElement() { 204 return this.encounterId != null && !this.encounterId.isEmpty(); 205 } 206 207 public boolean hasEncounterId() { 208 return this.encounterId != null && !this.encounterId.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 213 */ 214 public CDSHookOrderSignContext setEncounterIdElement(IdType value) { 215 this.encounterId = value; 216 return this; 217 } 218 219 /** 220 * @return The FHIR Encounter.id of the current encounter in context 221 */ 222 public String getEncounterId() { 223 return this.encounterId == null ? null : this.encounterId.getValue(); 224 } 225 226 /** 227 * @param value The FHIR Encounter.id of the current encounter in context 228 */ 229 public CDSHookOrderSignContext setEncounterId(String value) { 230 if (Utilities.noString(value)) 231 this.encounterId = null; 232 else { 233 if (this.encounterId == null) 234 this.encounterId = new IdType(); 235 this.encounterId.setValue(value); 236 } 237 return this; 238 } 239 240 /** 241 * @return {@link #draftOrders} (FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status) 242 */ 243 public Bundle getDraftOrders() { 244 if (this.draftOrders == null) 245 if (Configuration.errorOnAutoCreate()) 246 throw new Error("Attempt to auto-create CDSHookOrderSignContext.draftOrders"); 247 else if (Configuration.doAutoCreate()) 248 this.draftOrders = new Bundle(); // cc 249 return this.draftOrders; 250 } 251 252 public boolean hasDraftOrders() { 253 return this.draftOrders != null && !this.draftOrders.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #draftOrders} (FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status) 258 */ 259 public CDSHookOrderSignContext setDraftOrders(Bundle value) { 260 this.draftOrders = value; 261 return this; 262 } 263 264 protected void listChildren(List<Property> children) { 265 super.listChildren(children); 266 children.add(new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId)); 267 children.add(new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId)); 268 children.add(new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId)); 269 children.add(new Property("draftOrders", "Bundle", "FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", 0, 1, draftOrders)); 270 } 271 272 @Override 273 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 274 switch (_hash) { 275 case -836030906: /*userId*/ return new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId); 276 case -343587072: /*patientId*/ return new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId); 277 case 107147694: /*encounterId*/ return new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId); 278 case 155667430: /*draftOrders*/ return new Property("draftOrders", "Bundle", "FHIR Bundle of MedicationRequest, NutritionOrder, ServiceRequest, VisionPrescription with draft status", 0, 1, draftOrders); 279 default: return super.getNamedProperty(_hash, _name, _checkValid); 280 } 281 282 } 283 284 @Override 285 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 286 switch (hash) { 287 case -836030906: /*userId*/ return this.userId == null ? new Base[0] : new Base[] {this.userId}; // UrlType 288 case -343587072: /*patientId*/ return this.patientId == null ? new Base[0] : new Base[] {this.patientId}; // IdType 289 case 107147694: /*encounterId*/ return this.encounterId == null ? new Base[0] : new Base[] {this.encounterId}; // IdType 290 case 155667430: /*draftOrders*/ return this.draftOrders == null ? new Base[0] : new Base[] {this.draftOrders}; // Bundle 291 default: return super.getProperty(hash, name, checkValid); 292 } 293 294 } 295 296 @Override 297 public Base setProperty(int hash, String name, Base value) throws FHIRException { 298 switch (hash) { 299 case -836030906: // userId 300 this.userId = TypeConvertor.castToUrl(value); // UrlType 301 return value; 302 case -343587072: // patientId 303 this.patientId = TypeConvertor.castToId(value); // IdType 304 return value; 305 case 107147694: // encounterId 306 this.encounterId = TypeConvertor.castToId(value); // IdType 307 return value; 308 case 155667430: // draftOrders 309 this.draftOrders = (Bundle) value; // Bundle 310 return value; 311 default: return super.setProperty(hash, name, value); 312 } 313 314 } 315 316 @Override 317 public Base setProperty(String name, Base value) throws FHIRException { 318 if (name.equals("userId")) { 319 this.userId = TypeConvertor.castToUrl(value); // UrlType 320 } else if (name.equals("patientId")) { 321 this.patientId = TypeConvertor.castToId(value); // IdType 322 } else if (name.equals("encounterId")) { 323 this.encounterId = TypeConvertor.castToId(value); // IdType 324 } else if (name.equals("draftOrders")) { 325 this.draftOrders = (Bundle) value; // Bundle 326 } else 327 return super.setProperty(name, value); 328 return value; 329 } 330 331 @Override 332 public Base makeProperty(int hash, String name) throws FHIRException { 333 switch (hash) { 334 case -836030906: return getUserIdElement(); 335 case -343587072: return getPatientIdElement(); 336 case 107147694: return getEncounterIdElement(); 337 case 155667430: return getDraftOrders(); 338 default: return super.makeProperty(hash, name); 339 } 340 341 } 342 343 @Override 344 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 345 switch (hash) { 346 case -836030906: /*userId*/ return new String[] {"url"}; 347 case -343587072: /*patientId*/ return new String[] {"id"}; 348 case 107147694: /*encounterId*/ return new String[] {"id"}; 349 case 155667430: /*draftOrders*/ return new String[] {"Bundle"}; 350 default: return super.getTypesForProperty(hash, name); 351 } 352 353 } 354 355 @Override 356 public Base addChild(String name) throws FHIRException { 357 if (name.equals("userId")) { 358 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSignContext.userId"); 359 } 360 else if (name.equals("patientId")) { 361 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSignContext.patientId"); 362 } 363 else if (name.equals("encounterId")) { 364 throw new FHIRException("Cannot call addChild on a singleton property CDSHookOrderSignContext.encounterId"); 365 } 366 else if (name.equals("draftOrders")) { 367 this.draftOrders = new Bundle(); 368 return this.draftOrders; 369 } 370 else 371 return super.addChild(name); 372 } 373 374 public String fhirType() { 375 return "CDSHookOrderSignContext"; 376 377 } 378 379 public CDSHookOrderSignContext copy() { 380 CDSHookOrderSignContext dst = new CDSHookOrderSignContext(); 381 copyValues(dst); 382 return dst; 383 } 384 385 public void copyValues(CDSHookOrderSignContext dst) { 386 super.copyValues(dst); 387 dst.userId = userId == null ? null : userId.copy(); 388 dst.patientId = patientId == null ? null : patientId.copy(); 389 dst.encounterId = encounterId == null ? null : encounterId.copy(); 390 dst.draftOrders = draftOrders == null ? null : draftOrders.copy(); 391 } 392 393 protected CDSHookOrderSignContext typedCopy() { 394 return copy(); 395 } 396 397 @Override 398 public boolean equalsDeep(Base other_) { 399 if (!super.equalsDeep(other_)) 400 return false; 401 if (!(other_ instanceof CDSHookOrderSignContext)) 402 return false; 403 CDSHookOrderSignContext o = (CDSHookOrderSignContext) other_; 404 return compareDeep(userId, o.userId, true) && compareDeep(patientId, o.patientId, true) && compareDeep(encounterId, o.encounterId, true) 405 && compareDeep(draftOrders, o.draftOrders, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other_) { 410 if (!super.equalsShallow(other_)) 411 return false; 412 if (!(other_ instanceof CDSHookOrderSignContext)) 413 return false; 414 CDSHookOrderSignContext o = (CDSHookOrderSignContext) other_; 415 return compareValues(userId, o.userId, true) && compareValues(patientId, o.patientId, true) && compareValues(encounterId, o.encounterId, true) 416 ; 417 } 418 419 public boolean isEmpty() { 420 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(userId, patientId, encounterId 421 , draftOrders); 422 } 423 424 425} 426