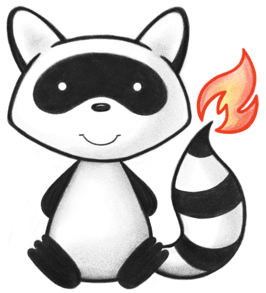
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Patient-View context. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHookPatientViewContext") 052public class CDSHookPatientViewContext extends CDSHookContext implements ICompositeType { 053 054 /** 055 * For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 056 */ 057 @Child(name = "userId", type = {UrlType.class}, order=0, min=1, max=1, modifier=false, summary=false) 058 @Description(shortDefinition="The id of the current user. Must be in the format [ResourceType]/[id].", formalDefinition="For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123." ) 059 protected UrlType userId; 060 061 /** 062 * The FHIR Patient.id of the current patient in context 063 */ 064 @Child(name = "patientId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 065 @Description(shortDefinition="The FHIR Patient.id of the current patient in context", formalDefinition="The FHIR Patient.id of the current patient in context" ) 066 protected IdType patientId; 067 068 /** 069 * The FHIR Encounter.id of the current encounter in context 070 */ 071 @Child(name = "encounterId", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=false) 072 @Description(shortDefinition="The FHIR Encounter.id of the current encounter in context", formalDefinition="The FHIR Encounter.id of the current encounter in context" ) 073 protected IdType encounterId; 074 075 private static final long serialVersionUID = -1001646309L; 076 077 /** 078 * Constructor 079 */ 080 public CDSHookPatientViewContext() { 081 super(); 082 } 083 084 /** 085 * Constructor 086 */ 087 public CDSHookPatientViewContext(String userId, String patientId) { 088 super(); 089 this.setUserId(userId); 090 this.setPatientId(patientId); 091 } 092 093 /** 094 * @return {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 095 */ 096 public UrlType getUserIdElement() { 097 if (this.userId == null) 098 if (Configuration.errorOnAutoCreate()) 099 throw new Error("Attempt to auto-create CDSHookPatientViewContext.userId"); 100 else if (Configuration.doAutoCreate()) 101 this.userId = new UrlType(); // bb 102 return this.userId; 103 } 104 105 public boolean hasUserIdElement() { 106 return this.userId != null && !this.userId.isEmpty(); 107 } 108 109 public boolean hasUserId() { 110 return this.userId != null && !this.userId.isEmpty(); 111 } 112 113 /** 114 * @param value {@link #userId} (For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.). This is the underlying object with id, value and extensions. The accessor "getUserId" gives direct access to the value 115 */ 116 public CDSHookPatientViewContext setUserIdElement(UrlType value) { 117 this.userId = value; 118 return this; 119 } 120 121 /** 122 * @return For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 123 */ 124 public String getUserId() { 125 return this.userId == null ? null : this.userId.getValue(); 126 } 127 128 /** 129 * @param value For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123. 130 */ 131 public CDSHookPatientViewContext setUserId(String value) { 132 if (this.userId == null) 133 this.userId = new UrlType(); 134 this.userId.setValue(value); 135 return this; 136 } 137 138 /** 139 * @return {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 140 */ 141 public IdType getPatientIdElement() { 142 if (this.patientId == null) 143 if (Configuration.errorOnAutoCreate()) 144 throw new Error("Attempt to auto-create CDSHookPatientViewContext.patientId"); 145 else if (Configuration.doAutoCreate()) 146 this.patientId = new IdType(); // bb 147 return this.patientId; 148 } 149 150 public boolean hasPatientIdElement() { 151 return this.patientId != null && !this.patientId.isEmpty(); 152 } 153 154 public boolean hasPatientId() { 155 return this.patientId != null && !this.patientId.isEmpty(); 156 } 157 158 /** 159 * @param value {@link #patientId} (The FHIR Patient.id of the current patient in context). This is the underlying object with id, value and extensions. The accessor "getPatientId" gives direct access to the value 160 */ 161 public CDSHookPatientViewContext setPatientIdElement(IdType value) { 162 this.patientId = value; 163 return this; 164 } 165 166 /** 167 * @return The FHIR Patient.id of the current patient in context 168 */ 169 public String getPatientId() { 170 return this.patientId == null ? null : this.patientId.getValue(); 171 } 172 173 /** 174 * @param value The FHIR Patient.id of the current patient in context 175 */ 176 public CDSHookPatientViewContext setPatientId(String value) { 177 if (this.patientId == null) 178 this.patientId = new IdType(); 179 this.patientId.setValue(value); 180 return this; 181 } 182 183 /** 184 * @return {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 185 */ 186 public IdType getEncounterIdElement() { 187 if (this.encounterId == null) 188 if (Configuration.errorOnAutoCreate()) 189 throw new Error("Attempt to auto-create CDSHookPatientViewContext.encounterId"); 190 else if (Configuration.doAutoCreate()) 191 this.encounterId = new IdType(); // bb 192 return this.encounterId; 193 } 194 195 public boolean hasEncounterIdElement() { 196 return this.encounterId != null && !this.encounterId.isEmpty(); 197 } 198 199 public boolean hasEncounterId() { 200 return this.encounterId != null && !this.encounterId.isEmpty(); 201 } 202 203 /** 204 * @param value {@link #encounterId} (The FHIR Encounter.id of the current encounter in context). This is the underlying object with id, value and extensions. The accessor "getEncounterId" gives direct access to the value 205 */ 206 public CDSHookPatientViewContext setEncounterIdElement(IdType value) { 207 this.encounterId = value; 208 return this; 209 } 210 211 /** 212 * @return The FHIR Encounter.id of the current encounter in context 213 */ 214 public String getEncounterId() { 215 return this.encounterId == null ? null : this.encounterId.getValue(); 216 } 217 218 /** 219 * @param value The FHIR Encounter.id of the current encounter in context 220 */ 221 public CDSHookPatientViewContext setEncounterId(String value) { 222 if (Utilities.noString(value)) 223 this.encounterId = null; 224 else { 225 if (this.encounterId == null) 226 this.encounterId = new IdType(); 227 this.encounterId.setValue(value); 228 } 229 return this; 230 } 231 232 protected void listChildren(List<Property> children) { 233 super.listChildren(children); 234 children.add(new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId)); 235 children.add(new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId)); 236 children.add(new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId)); 237 } 238 239 @Override 240 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 241 switch (_hash) { 242 case -836030906: /*userId*/ return new Property("userId", "url", "For this hook, the user is expected to be of type Practitioner, PractitionerRole, Patient, or RelatedPerson. Patient or RelatedPerson are appropriate when a patient or their proxy are viewing the record. For example, Practitioner/abc or Patient/123.", 0, 1, userId); 243 case -343587072: /*patientId*/ return new Property("patientId", "id", "The FHIR Patient.id of the current patient in context", 0, 1, patientId); 244 case 107147694: /*encounterId*/ return new Property("encounterId", "id", "The FHIR Encounter.id of the current encounter in context", 0, 1, encounterId); 245 default: return super.getNamedProperty(_hash, _name, _checkValid); 246 } 247 248 } 249 250 @Override 251 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 252 switch (hash) { 253 case -836030906: /*userId*/ return this.userId == null ? new Base[0] : new Base[] {this.userId}; // UrlType 254 case -343587072: /*patientId*/ return this.patientId == null ? new Base[0] : new Base[] {this.patientId}; // IdType 255 case 107147694: /*encounterId*/ return this.encounterId == null ? new Base[0] : new Base[] {this.encounterId}; // IdType 256 default: return super.getProperty(hash, name, checkValid); 257 } 258 259 } 260 261 @Override 262 public Base setProperty(int hash, String name, Base value) throws FHIRException { 263 switch (hash) { 264 case -836030906: // userId 265 this.userId = TypeConvertor.castToUrl(value); // UrlType 266 return value; 267 case -343587072: // patientId 268 this.patientId = TypeConvertor.castToId(value); // IdType 269 return value; 270 case 107147694: // encounterId 271 this.encounterId = TypeConvertor.castToId(value); // IdType 272 return value; 273 default: return super.setProperty(hash, name, value); 274 } 275 276 } 277 278 @Override 279 public Base setProperty(String name, Base value) throws FHIRException { 280 if (name.equals("userId")) { 281 this.userId = TypeConvertor.castToUrl(value); // UrlType 282 } else if (name.equals("patientId")) { 283 this.patientId = TypeConvertor.castToId(value); // IdType 284 } else if (name.equals("encounterId")) { 285 this.encounterId = TypeConvertor.castToId(value); // IdType 286 } else 287 return super.setProperty(name, value); 288 return value; 289 } 290 291 @Override 292 public Base makeProperty(int hash, String name) throws FHIRException { 293 switch (hash) { 294 case -836030906: return getUserIdElement(); 295 case -343587072: return getPatientIdElement(); 296 case 107147694: return getEncounterIdElement(); 297 default: return super.makeProperty(hash, name); 298 } 299 300 } 301 302 @Override 303 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 304 switch (hash) { 305 case -836030906: /*userId*/ return new String[] {"url"}; 306 case -343587072: /*patientId*/ return new String[] {"id"}; 307 case 107147694: /*encounterId*/ return new String[] {"id"}; 308 default: return super.getTypesForProperty(hash, name); 309 } 310 311 } 312 313 @Override 314 public Base addChild(String name) throws FHIRException { 315 if (name.equals("userId")) { 316 throw new FHIRException("Cannot call addChild on a singleton property CDSHookPatientViewContext.userId"); 317 } 318 else if (name.equals("patientId")) { 319 throw new FHIRException("Cannot call addChild on a singleton property CDSHookPatientViewContext.patientId"); 320 } 321 else if (name.equals("encounterId")) { 322 throw new FHIRException("Cannot call addChild on a singleton property CDSHookPatientViewContext.encounterId"); 323 } 324 else 325 return super.addChild(name); 326 } 327 328 public String fhirType() { 329 return "CDSHookPatientViewContext"; 330 331 } 332 333 public CDSHookPatientViewContext copy() { 334 CDSHookPatientViewContext dst = new CDSHookPatientViewContext(); 335 copyValues(dst); 336 return dst; 337 } 338 339 public void copyValues(CDSHookPatientViewContext dst) { 340 super.copyValues(dst); 341 dst.userId = userId == null ? null : userId.copy(); 342 dst.patientId = patientId == null ? null : patientId.copy(); 343 dst.encounterId = encounterId == null ? null : encounterId.copy(); 344 } 345 346 protected CDSHookPatientViewContext typedCopy() { 347 return copy(); 348 } 349 350 @Override 351 public boolean equalsDeep(Base other_) { 352 if (!super.equalsDeep(other_)) 353 return false; 354 if (!(other_ instanceof CDSHookPatientViewContext)) 355 return false; 356 CDSHookPatientViewContext o = (CDSHookPatientViewContext) other_; 357 return compareDeep(userId, o.userId, true) && compareDeep(patientId, o.patientId, true) && compareDeep(encounterId, o.encounterId, true) 358 ; 359 } 360 361 @Override 362 public boolean equalsShallow(Base other_) { 363 if (!super.equalsShallow(other_)) 364 return false; 365 if (!(other_ instanceof CDSHookPatientViewContext)) 366 return false; 367 CDSHookPatientViewContext o = (CDSHookPatientViewContext) other_; 368 return compareValues(userId, o.userId, true) && compareValues(patientId, o.patientId, true) && compareValues(encounterId, o.encounterId, true) 369 ; 370 } 371 372 public boolean isEmpty() { 373 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(userId, patientId, encounterId 374 ); 375 } 376 377 378} 379