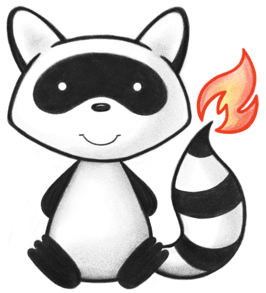
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.tools.Enumerations.*; 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.r5.model.*; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Services Element with Extensions. TODO: This content will be moved to the CDS Hooks specification in the future 049 */ 050@DatatypeDef(name="CDSHooksElement") 051public abstract class CDSHooksElement extends LogicalBase implements ICompositeType { 052 053 /** 054 * A JSON object that has properties where the names are defined by the extension definitions 055 */ 056 @Child(name = "extension", type = {CDSHooksExtensions.class}, order=0, min=0, max=1, modifier=false, summary=false) 057 @Description(shortDefinition="Object that has Named Extension Properties", formalDefinition="A JSON object that has properties where the names are defined by the extension definitions" ) 058 protected CDSHooksExtensions extension; 059 060 private static final long serialVersionUID = 362241525L; 061 062 /** 063 * Constructor 064 */ 065 public CDSHooksElement() { 066 super(); 067 } 068 069 /** 070 * @return {@link #extension} (A JSON object that has properties where the names are defined by the extension definitions) 071 */ 072 public CDSHooksExtensions getExtension() { 073 if (this.extension == null) 074 if (Configuration.errorOnAutoCreate()) 075 throw new Error("Attempt to auto-create CDSHooksElement.extension"); 076 else if (Configuration.doAutoCreate()) 077 this.extension = new CDSHooksExtensions(); // cc 078 return this.extension; 079 } 080 081 public boolean hasExtension() { 082 return this.extension != null && !this.extension.isEmpty(); 083 } 084 085 /** 086 * @param value {@link #extension} (A JSON object that has properties where the names are defined by the extension definitions) 087 */ 088 public CDSHooksElement setExtension(CDSHooksExtensions value) { 089 this.extension = value; 090 return this; 091 } 092 093 protected void listChildren(List<Property> children) { 094 super.listChildren(children); 095 children.add(new Property("extension", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksExtensions", "A JSON object that has properties where the names are defined by the extension definitions", 0, 1, extension)); 096 } 097 098 @Override 099 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 100 switch (_hash) { 101 case -612557761: /*extension*/ return new Property("extension", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksExtensions", "A JSON object that has properties where the names are defined by the extension definitions", 0, 1, extension); 102 default: return super.getNamedProperty(_hash, _name, _checkValid); 103 } 104 105 } 106 107 @Override 108 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 109 switch (hash) { 110 case -612557761: /*extension*/ return this.extension == null ? new Base[0] : new Base[] {this.extension}; // CDSHooksExtensions 111 default: return super.getProperty(hash, name, checkValid); 112 } 113 114 } 115 116 @Override 117 public Base setProperty(int hash, String name, Base value) throws FHIRException { 118 switch (hash) { 119 case -612557761: // extension 120 this.extension = (CDSHooksExtensions) value; // CDSHooksExtensions 121 return value; 122 default: return super.setProperty(hash, name, value); 123 } 124 125 } 126 127 @Override 128 public Base setProperty(String name, Base value) throws FHIRException { 129 if (name.equals("extension")) { 130 this.extension = (CDSHooksExtensions) value; // CDSHooksExtensions 131 } else 132 return super.setProperty(name, value); 133 return value; 134 } 135 136 @Override 137 public Base makeProperty(int hash, String name) throws FHIRException { 138 switch (hash) { 139 case -612557761: return getExtension(); 140 default: return super.makeProperty(hash, name); 141 } 142 143 } 144 145 @Override 146 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 147 switch (hash) { 148 case -612557761: /*extension*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksExtensions"}; 149 default: return super.getTypesForProperty(hash, name); 150 } 151 152 } 153 154 @Override 155 public Base addChild(String name) throws FHIRException { 156 if (name.equals("extension")) { 157 this.extension = new CDSHooksExtensions(); 158 return this.extension; 159 } 160 else 161 return super.addChild(name); 162 } 163 164 public String fhirType() { 165 return "CDSHooksElement"; 166 167 } 168 169 public abstract CDSHooksElement copy(); 170 171 public void copyValues(CDSHooksElement dst) { 172 super.copyValues(dst); 173 dst.extension = extension == null ? null : extension.copy(); 174 } 175 176 @Override 177 public boolean equalsDeep(Base other_) { 178 if (!super.equalsDeep(other_)) 179 return false; 180 if (!(other_ instanceof CDSHooksElement)) 181 return false; 182 CDSHooksElement o = (CDSHooksElement) other_; 183 return compareDeep(extension, o.extension, true); 184 } 185 186 @Override 187 public boolean equalsShallow(Base other_) { 188 if (!super.equalsShallow(other_)) 189 return false; 190 if (!(other_ instanceof CDSHooksElement)) 191 return false; 192 CDSHooksElement o = (CDSHooksElement) other_; 193 return true; 194 } 195 196 public boolean isEmpty() { 197 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(extension); 198 } 199 200 201} 202