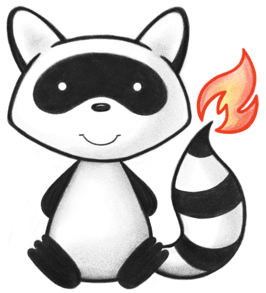
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Request Body. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHooksRequest") 052public class CDSHooksRequest extends CDSHooksElement implements ICompositeType { 053 054 @Block() 055 public static class CDSHooksRequestFhirAuthorizationComponent extends CDSHooksElement { 056 /** 057 * This is the OAuth 2.0 access token that provides access to the FHIR server 058 */ 059 @Child(name = "accessToken", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 060 @Description(shortDefinition="This is the OAuth 2.0 access token that provides access to the FHIR server", formalDefinition="This is the OAuth 2.0 access token that provides access to the FHIR server" ) 061 protected StringType accessToken; 062 063 /** 064 * Fixed value: Bearer 065 */ 066 @Child(name = "tokenType", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 067 @Description(shortDefinition="Fixed value: Bearer", formalDefinition="Fixed value: Bearer" ) 068 protected CodeType tokenType; 069 070 /** 071 * The lifetime in seconds of the access token. 072 */ 073 @Child(name = "expiresIn", type = {IntegerType.class}, order=3, min=1, max=1, modifier=false, summary=false) 074 @Description(shortDefinition="The lifetime in seconds of the access token.", formalDefinition="The lifetime in seconds of the access token." ) 075 protected IntegerType expiresIn; 076 077 /** 078 * The scopes the access token grants the CDS Service 079 */ 080 @Child(name = "scope", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 081 @Description(shortDefinition="The scopes the access token grants the CDS Service", formalDefinition="The scopes the access token grants the CDS Service" ) 082 protected StringType scope; 083 084 /** 085 * The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 086 */ 087 @Child(name = "subject", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=false) 088 @Description(shortDefinition="The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", formalDefinition="The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server" ) 089 protected StringType subject; 090 091 /** 092 * The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 093 */ 094 @Child(name = "patient", type = {IdType.class}, order=6, min=0, max=1, modifier=false, summary=false) 095 @Description(shortDefinition="The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", formalDefinition="The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server" ) 096 protected IdType patient; 097 098 private static final long serialVersionUID = 265340325L; 099 100 /** 101 * Constructor 102 */ 103 public CDSHooksRequestFhirAuthorizationComponent() { 104 super(); 105 } 106 107 /** 108 * Constructor 109 */ 110 public CDSHooksRequestFhirAuthorizationComponent(String accessToken, String tokenType, int expiresIn, String scope, String subject) { 111 super(); 112 this.setAccessToken(accessToken); 113 this.setTokenType(tokenType); 114 this.setExpiresIn(expiresIn); 115 this.setScope(scope); 116 this.setSubject(subject); 117 } 118 119 /** 120 * @return {@link #accessToken} (This is the OAuth 2.0 access token that provides access to the FHIR server). This is the underlying object with id, value and extensions. The accessor "getAccessToken" gives direct access to the value 121 */ 122 public StringType getAccessTokenElement() { 123 if (this.accessToken == null) 124 if (Configuration.errorOnAutoCreate()) 125 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.accessToken"); 126 else if (Configuration.doAutoCreate()) 127 this.accessToken = new StringType(); // bb 128 return this.accessToken; 129 } 130 131 public boolean hasAccessTokenElement() { 132 return this.accessToken != null && !this.accessToken.isEmpty(); 133 } 134 135 public boolean hasAccessToken() { 136 return this.accessToken != null && !this.accessToken.isEmpty(); 137 } 138 139 /** 140 * @param value {@link #accessToken} (This is the OAuth 2.0 access token that provides access to the FHIR server). This is the underlying object with id, value and extensions. The accessor "getAccessToken" gives direct access to the value 141 */ 142 public CDSHooksRequestFhirAuthorizationComponent setAccessTokenElement(StringType value) { 143 this.accessToken = value; 144 return this; 145 } 146 147 /** 148 * @return This is the OAuth 2.0 access token that provides access to the FHIR server 149 */ 150 public String getAccessToken() { 151 return this.accessToken == null ? null : this.accessToken.getValue(); 152 } 153 154 /** 155 * @param value This is the OAuth 2.0 access token that provides access to the FHIR server 156 */ 157 public CDSHooksRequestFhirAuthorizationComponent setAccessToken(String value) { 158 if (this.accessToken == null) 159 this.accessToken = new StringType(); 160 this.accessToken.setValue(value); 161 return this; 162 } 163 164 /** 165 * @return {@link #tokenType} (Fixed value: Bearer). This is the underlying object with id, value and extensions. The accessor "getTokenType" gives direct access to the value 166 */ 167 public CodeType getTokenTypeElement() { 168 if (this.tokenType == null) 169 if (Configuration.errorOnAutoCreate()) 170 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.tokenType"); 171 else if (Configuration.doAutoCreate()) 172 this.tokenType = new CodeType(); // bb 173 return this.tokenType; 174 } 175 176 public boolean hasTokenTypeElement() { 177 return this.tokenType != null && !this.tokenType.isEmpty(); 178 } 179 180 public boolean hasTokenType() { 181 return this.tokenType != null && !this.tokenType.isEmpty(); 182 } 183 184 /** 185 * @param value {@link #tokenType} (Fixed value: Bearer). This is the underlying object with id, value and extensions. The accessor "getTokenType" gives direct access to the value 186 */ 187 public CDSHooksRequestFhirAuthorizationComponent setTokenTypeElement(CodeType value) { 188 this.tokenType = value; 189 return this; 190 } 191 192 /** 193 * @return Fixed value: Bearer 194 */ 195 public String getTokenType() { 196 return this.tokenType == null ? null : this.tokenType.getValue(); 197 } 198 199 /** 200 * @param value Fixed value: Bearer 201 */ 202 public CDSHooksRequestFhirAuthorizationComponent setTokenType(String value) { 203 if (this.tokenType == null) 204 this.tokenType = new CodeType(); 205 this.tokenType.setValue(value); 206 return this; 207 } 208 209 /** 210 * @return {@link #expiresIn} (The lifetime in seconds of the access token.). This is the underlying object with id, value and extensions. The accessor "getExpiresIn" gives direct access to the value 211 */ 212 public IntegerType getExpiresInElement() { 213 if (this.expiresIn == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.expiresIn"); 216 else if (Configuration.doAutoCreate()) 217 this.expiresIn = new IntegerType(); // bb 218 return this.expiresIn; 219 } 220 221 public boolean hasExpiresInElement() { 222 return this.expiresIn != null && !this.expiresIn.isEmpty(); 223 } 224 225 public boolean hasExpiresIn() { 226 return this.expiresIn != null && !this.expiresIn.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #expiresIn} (The lifetime in seconds of the access token.). This is the underlying object with id, value and extensions. The accessor "getExpiresIn" gives direct access to the value 231 */ 232 public CDSHooksRequestFhirAuthorizationComponent setExpiresInElement(IntegerType value) { 233 this.expiresIn = value; 234 return this; 235 } 236 237 /** 238 * @return The lifetime in seconds of the access token. 239 */ 240 public int getExpiresIn() { 241 return this.expiresIn == null || this.expiresIn.isEmpty() ? 0 : this.expiresIn.getValue(); 242 } 243 244 /** 245 * @param value The lifetime in seconds of the access token. 246 */ 247 public CDSHooksRequestFhirAuthorizationComponent setExpiresIn(int value) { 248 if (this.expiresIn == null) 249 this.expiresIn = new IntegerType(); 250 this.expiresIn.setValue(value); 251 return this; 252 } 253 254 /** 255 * @return {@link #scope} (The scopes the access token grants the CDS Service). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 256 */ 257 public StringType getScopeElement() { 258 if (this.scope == null) 259 if (Configuration.errorOnAutoCreate()) 260 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.scope"); 261 else if (Configuration.doAutoCreate()) 262 this.scope = new StringType(); // bb 263 return this.scope; 264 } 265 266 public boolean hasScopeElement() { 267 return this.scope != null && !this.scope.isEmpty(); 268 } 269 270 public boolean hasScope() { 271 return this.scope != null && !this.scope.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #scope} (The scopes the access token grants the CDS Service). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 276 */ 277 public CDSHooksRequestFhirAuthorizationComponent setScopeElement(StringType value) { 278 this.scope = value; 279 return this; 280 } 281 282 /** 283 * @return The scopes the access token grants the CDS Service 284 */ 285 public String getScope() { 286 return this.scope == null ? null : this.scope.getValue(); 287 } 288 289 /** 290 * @param value The scopes the access token grants the CDS Service 291 */ 292 public CDSHooksRequestFhirAuthorizationComponent setScope(String value) { 293 if (this.scope == null) 294 this.scope = new StringType(); 295 this.scope.setValue(value); 296 return this; 297 } 298 299 /** 300 * @return {@link #subject} (The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server). This is the underlying object with id, value and extensions. The accessor "getSubject" gives direct access to the value 301 */ 302 public StringType getSubjectElement() { 303 if (this.subject == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.subject"); 306 else if (Configuration.doAutoCreate()) 307 this.subject = new StringType(); // bb 308 return this.subject; 309 } 310 311 public boolean hasSubjectElement() { 312 return this.subject != null && !this.subject.isEmpty(); 313 } 314 315 public boolean hasSubject() { 316 return this.subject != null && !this.subject.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #subject} (The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server). This is the underlying object with id, value and extensions. The accessor "getSubject" gives direct access to the value 321 */ 322 public CDSHooksRequestFhirAuthorizationComponent setSubjectElement(StringType value) { 323 this.subject = value; 324 return this; 325 } 326 327 /** 328 * @return The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 329 */ 330 public String getSubject() { 331 return this.subject == null ? null : this.subject.getValue(); 332 } 333 334 /** 335 * @param value The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 336 */ 337 public CDSHooksRequestFhirAuthorizationComponent setSubject(String value) { 338 if (this.subject == null) 339 this.subject = new StringType(); 340 this.subject.setValue(value); 341 return this; 342 } 343 344 /** 345 * @return {@link #patient} (The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server). This is the underlying object with id, value and extensions. The accessor "getPatient" gives direct access to the value 346 */ 347 public IdType getPatientElement() { 348 if (this.patient == null) 349 if (Configuration.errorOnAutoCreate()) 350 throw new Error("Attempt to auto-create CDSHooksRequestFhirAuthorizationComponent.patient"); 351 else if (Configuration.doAutoCreate()) 352 this.patient = new IdType(); // bb 353 return this.patient; 354 } 355 356 public boolean hasPatientElement() { 357 return this.patient != null && !this.patient.isEmpty(); 358 } 359 360 public boolean hasPatient() { 361 return this.patient != null && !this.patient.isEmpty(); 362 } 363 364 /** 365 * @param value {@link #patient} (The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server). This is the underlying object with id, value and extensions. The accessor "getPatient" gives direct access to the value 366 */ 367 public CDSHooksRequestFhirAuthorizationComponent setPatientElement(IdType value) { 368 this.patient = value; 369 return this; 370 } 371 372 /** 373 * @return The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 374 */ 375 public String getPatient() { 376 return this.patient == null ? null : this.patient.getValue(); 377 } 378 379 /** 380 * @param value The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server 381 */ 382 public CDSHooksRequestFhirAuthorizationComponent setPatient(String value) { 383 if (Utilities.noString(value)) 384 this.patient = null; 385 else { 386 if (this.patient == null) 387 this.patient = new IdType(); 388 this.patient.setValue(value); 389 } 390 return this; 391 } 392 393 protected void listChildren(List<Property> children) { 394 super.listChildren(children); 395 children.add(new Property("accessToken", "string", "This is the OAuth 2.0 access token that provides access to the FHIR server", 0, 1, accessToken)); 396 children.add(new Property("tokenType", "code", "Fixed value: Bearer", 0, 1, tokenType)); 397 children.add(new Property("expiresIn", "integer", "The lifetime in seconds of the access token.", 0, 1, expiresIn)); 398 children.add(new Property("scope", "string", "The scopes the access token grants the CDS Service", 0, 1, scope)); 399 children.add(new Property("subject", "string", "The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", 0, 1, subject)); 400 children.add(new Property("patient", "id", "The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", 0, 1, patient)); 401 } 402 403 @Override 404 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 405 switch (_hash) { 406 case -1042689291: /*accessToken*/ return new Property("accessToken", "string", "This is the OAuth 2.0 access token that provides access to the FHIR server", 0, 1, accessToken); 407 case 141498579: /*tokenType*/ return new Property("tokenType", "code", "Fixed value: Bearer", 0, 1, tokenType); 408 case 250196857: /*expiresIn*/ return new Property("expiresIn", "integer", "The lifetime in seconds of the access token.", 0, 1, expiresIn); 409 case 109264468: /*scope*/ return new Property("scope", "string", "The scopes the access token grants the CDS Service", 0, 1, scope); 410 case -1867885268: /*subject*/ return new Property("subject", "string", "The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", 0, 1, subject); 411 case -791418107: /*patient*/ return new Property("patient", "id", "The OAuth 2.0 client identifier of the CDS Service, as registered with the CDS Client's authorization server", 0, 1, patient); 412 default: return super.getNamedProperty(_hash, _name, _checkValid); 413 } 414 415 } 416 417 @Override 418 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 419 switch (hash) { 420 case -1042689291: /*accessToken*/ return this.accessToken == null ? new Base[0] : new Base[] {this.accessToken}; // StringType 421 case 141498579: /*tokenType*/ return this.tokenType == null ? new Base[0] : new Base[] {this.tokenType}; // CodeType 422 case 250196857: /*expiresIn*/ return this.expiresIn == null ? new Base[0] : new Base[] {this.expiresIn}; // IntegerType 423 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // StringType 424 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // StringType 425 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // IdType 426 default: return super.getProperty(hash, name, checkValid); 427 } 428 429 } 430 431 @Override 432 public Base setProperty(int hash, String name, Base value) throws FHIRException { 433 switch (hash) { 434 case -1042689291: // accessToken 435 this.accessToken = TypeConvertor.castToString(value); // StringType 436 return value; 437 case 141498579: // tokenType 438 this.tokenType = TypeConvertor.castToCode(value); // CodeType 439 return value; 440 case 250196857: // expiresIn 441 this.expiresIn = TypeConvertor.castToInteger(value); // IntegerType 442 return value; 443 case 109264468: // scope 444 this.scope = TypeConvertor.castToString(value); // StringType 445 return value; 446 case -1867885268: // subject 447 this.subject = TypeConvertor.castToString(value); // StringType 448 return value; 449 case -791418107: // patient 450 this.patient = TypeConvertor.castToId(value); // IdType 451 return value; 452 default: return super.setProperty(hash, name, value); 453 } 454 455 } 456 457 @Override 458 public Base setProperty(String name, Base value) throws FHIRException { 459 if (name.equals("accessToken")) { 460 this.accessToken = TypeConvertor.castToString(value); // StringType 461 } else if (name.equals("tokenType")) { 462 this.tokenType = TypeConvertor.castToCode(value); // CodeType 463 } else if (name.equals("expiresIn")) { 464 this.expiresIn = TypeConvertor.castToInteger(value); // IntegerType 465 } else if (name.equals("scope")) { 466 this.scope = TypeConvertor.castToString(value); // StringType 467 } else if (name.equals("subject")) { 468 this.subject = TypeConvertor.castToString(value); // StringType 469 } else if (name.equals("patient")) { 470 this.patient = TypeConvertor.castToId(value); // IdType 471 } else 472 return super.setProperty(name, value); 473 return value; 474 } 475 476 @Override 477 public Base makeProperty(int hash, String name) throws FHIRException { 478 switch (hash) { 479 case -1042689291: return getAccessTokenElement(); 480 case 141498579: return getTokenTypeElement(); 481 case 250196857: return getExpiresInElement(); 482 case 109264468: return getScopeElement(); 483 case -1867885268: return getSubjectElement(); 484 case -791418107: return getPatientElement(); 485 default: return super.makeProperty(hash, name); 486 } 487 488 } 489 490 @Override 491 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 492 switch (hash) { 493 case -1042689291: /*accessToken*/ return new String[] {"string"}; 494 case 141498579: /*tokenType*/ return new String[] {"code"}; 495 case 250196857: /*expiresIn*/ return new String[] {"integer"}; 496 case 109264468: /*scope*/ return new String[] {"string"}; 497 case -1867885268: /*subject*/ return new String[] {"string"}; 498 case -791418107: /*patient*/ return new String[] {"id"}; 499 default: return super.getTypesForProperty(hash, name); 500 } 501 502 } 503 504 @Override 505 public Base addChild(String name) throws FHIRException { 506 if (name.equals("accessToken")) { 507 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.accessToken"); 508 } 509 else if (name.equals("tokenType")) { 510 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.tokenType"); 511 } 512 else if (name.equals("expiresIn")) { 513 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.expiresIn"); 514 } 515 else if (name.equals("scope")) { 516 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.scope"); 517 } 518 else if (name.equals("subject")) { 519 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.subject"); 520 } 521 else if (name.equals("patient")) { 522 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirAuthorization.patient"); 523 } 524 else 525 return super.addChild(name); 526 } 527 528 public CDSHooksRequestFhirAuthorizationComponent copy() { 529 CDSHooksRequestFhirAuthorizationComponent dst = new CDSHooksRequestFhirAuthorizationComponent(); 530 copyValues(dst); 531 return dst; 532 } 533 534 public void copyValues(CDSHooksRequestFhirAuthorizationComponent dst) { 535 super.copyValues(dst); 536 dst.accessToken = accessToken == null ? null : accessToken.copy(); 537 dst.tokenType = tokenType == null ? null : tokenType.copy(); 538 dst.expiresIn = expiresIn == null ? null : expiresIn.copy(); 539 dst.scope = scope == null ? null : scope.copy(); 540 dst.subject = subject == null ? null : subject.copy(); 541 dst.patient = patient == null ? null : patient.copy(); 542 } 543 544 @Override 545 public boolean equalsDeep(Base other_) { 546 if (!super.equalsDeep(other_)) 547 return false; 548 if (!(other_ instanceof CDSHooksRequestFhirAuthorizationComponent)) 549 return false; 550 CDSHooksRequestFhirAuthorizationComponent o = (CDSHooksRequestFhirAuthorizationComponent) other_; 551 return compareDeep(accessToken, o.accessToken, true) && compareDeep(tokenType, o.tokenType, true) 552 && compareDeep(expiresIn, o.expiresIn, true) && compareDeep(scope, o.scope, true) && compareDeep(subject, o.subject, true) 553 && compareDeep(patient, o.patient, true); 554 } 555 556 @Override 557 public boolean equalsShallow(Base other_) { 558 if (!super.equalsShallow(other_)) 559 return false; 560 if (!(other_ instanceof CDSHooksRequestFhirAuthorizationComponent)) 561 return false; 562 CDSHooksRequestFhirAuthorizationComponent o = (CDSHooksRequestFhirAuthorizationComponent) other_; 563 return compareValues(accessToken, o.accessToken, true) && compareValues(tokenType, o.tokenType, true) 564 && compareValues(expiresIn, o.expiresIn, true) && compareValues(scope, o.scope, true) && compareValues(subject, o.subject, true) 565 && compareValues(patient, o.patient, true); 566 } 567 568 public boolean isEmpty() { 569 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(accessToken, tokenType, expiresIn 570 , scope, subject, patient); 571 } 572 573 public String fhirType() { 574 return "CDSHooksRequest.fhirAuthorization"; 575 576 } 577 578 } 579 580 @Block() 581 public static class CDSHooksRequestPrefetchComponent extends LogicalBase { 582 /** 583 * Key of FHIR query - name for client to use when sending to Request 584 */ 585 @Child(name = "key", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 586 @Description(shortDefinition="Key of FHIR query - name for client to use when sending to Request", formalDefinition="Key of FHIR query - name for client to use when sending to Request" ) 587 protected CodeType key; 588 589 /** 590 * Value of FHIR query - FHIR Query for client to perform 591 */ 592 @Child(name = "value", type = {Resource.class}, order=2, min=1, max=1, modifier=false, summary=false) 593 @Description(shortDefinition="Value of FHIR query - FHIR Query for client to perform", formalDefinition="Value of FHIR query - FHIR Query for client to perform" ) 594 protected Resource value; 595 596 private static final long serialVersionUID = -18668409L; 597 598 /** 599 * Constructor 600 */ 601 public CDSHooksRequestPrefetchComponent() { 602 super(); 603 } 604 605 /** 606 * Constructor 607 */ 608 public CDSHooksRequestPrefetchComponent(String key, Resource value) { 609 super(); 610 this.setKey(key); 611 this.setValue(value); 612 } 613 614 /** 615 * @return {@link #key} (Key of FHIR query - name for client to use when sending to Request). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 616 */ 617 public CodeType getKeyElement() { 618 if (this.key == null) 619 if (Configuration.errorOnAutoCreate()) 620 throw new Error("Attempt to auto-create CDSHooksRequestPrefetchComponent.key"); 621 else if (Configuration.doAutoCreate()) 622 this.key = new CodeType(); // bb 623 return this.key; 624 } 625 626 public boolean hasKeyElement() { 627 return this.key != null && !this.key.isEmpty(); 628 } 629 630 public boolean hasKey() { 631 return this.key != null && !this.key.isEmpty(); 632 } 633 634 /** 635 * @param value {@link #key} (Key of FHIR query - name for client to use when sending to Request). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 636 */ 637 public CDSHooksRequestPrefetchComponent setKeyElement(CodeType value) { 638 this.key = value; 639 return this; 640 } 641 642 /** 643 * @return Key of FHIR query - name for client to use when sending to Request 644 */ 645 public String getKey() { 646 return this.key == null ? null : this.key.getValue(); 647 } 648 649 /** 650 * @param value Key of FHIR query - name for client to use when sending to Request 651 */ 652 public CDSHooksRequestPrefetchComponent setKey(String value) { 653 if (this.key == null) 654 this.key = new CodeType(); 655 this.key.setValue(value); 656 return this; 657 } 658 659 /** 660 * @return {@link #value} (Value of FHIR query - FHIR Query for client to perform) 661 */ 662 public Resource getValue() { 663 return this.value; 664 } 665 666 public boolean hasValue() { 667 return this.value != null && !this.value.isEmpty(); 668 } 669 670 /** 671 * @param value {@link #value} (Value of FHIR query - FHIR Query for client to perform) 672 */ 673 public CDSHooksRequestPrefetchComponent setValue(Resource value) { 674 this.value = value; 675 return this; 676 } 677 678 protected void listChildren(List<Property> children) { 679 super.listChildren(children); 680 children.add(new Property("key", "code", "Key of FHIR query - name for client to use when sending to Request", 0, 1, key)); 681 children.add(new Property("value", "Resource", "Value of FHIR query - FHIR Query for client to perform", 0, 1, value)); 682 } 683 684 @Override 685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 686 switch (_hash) { 687 case 106079: /*key*/ return new Property("key", "code", "Key of FHIR query - name for client to use when sending to Request", 0, 1, key); 688 case 111972721: /*value*/ return new Property("value", "Resource", "Value of FHIR query - FHIR Query for client to perform", 0, 1, value); 689 default: return super.getNamedProperty(_hash, _name, _checkValid); 690 } 691 692 } 693 694 @Override 695 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 696 switch (hash) { 697 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // CodeType 698 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Resource 699 default: return super.getProperty(hash, name, checkValid); 700 } 701 702 } 703 704 @Override 705 public Base setProperty(int hash, String name, Base value) throws FHIRException { 706 switch (hash) { 707 case 106079: // key 708 this.key = TypeConvertor.castToCode(value); // CodeType 709 return value; 710 case 111972721: // value 711 this.value = (Resource) value; // Resource 712 return value; 713 default: return super.setProperty(hash, name, value); 714 } 715 716 } 717 718 @Override 719 public Base setProperty(String name, Base value) throws FHIRException { 720 if (name.equals("key")) { 721 this.key = TypeConvertor.castToCode(value); // CodeType 722 } else if (name.equals("value")) { 723 this.value = (Resource) value; // Resource 724 } else 725 return super.setProperty(name, value); 726 return value; 727 } 728 729 @Override 730 public Base makeProperty(int hash, String name) throws FHIRException { 731 switch (hash) { 732 case 106079: return getKeyElement(); 733 case 111972721: throw new FHIRException("Cannot make property value as it is not a complex type"); // Resource 734 default: return super.makeProperty(hash, name); 735 } 736 737 } 738 739 @Override 740 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 741 switch (hash) { 742 case 106079: /*key*/ return new String[] {"code"}; 743 case 111972721: /*value*/ return new String[] {"Resource"}; 744 default: return super.getTypesForProperty(hash, name); 745 } 746 747 } 748 749 @Override 750 public Base addChild(String name) throws FHIRException { 751 if (name.equals("key")) { 752 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.prefetch.key"); 753 } 754 else if (name.equals("value")) { 755 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksRequest.prefetch.value"); 756 } 757 else 758 return super.addChild(name); 759 } 760 761 public CDSHooksRequestPrefetchComponent copy() { 762 CDSHooksRequestPrefetchComponent dst = new CDSHooksRequestPrefetchComponent(); 763 copyValues(dst); 764 return dst; 765 } 766 767 public void copyValues(CDSHooksRequestPrefetchComponent dst) { 768 super.copyValues(dst); 769 dst.key = key == null ? null : key.copy(); 770 dst.value = value == null ? null : value.copy(); 771 } 772 773 @Override 774 public boolean equalsDeep(Base other_) { 775 if (!super.equalsDeep(other_)) 776 return false; 777 if (!(other_ instanceof CDSHooksRequestPrefetchComponent)) 778 return false; 779 CDSHooksRequestPrefetchComponent o = (CDSHooksRequestPrefetchComponent) other_; 780 return compareDeep(key, o.key, true) && compareDeep(value, o.value, true); 781 } 782 783 @Override 784 public boolean equalsShallow(Base other_) { 785 if (!super.equalsShallow(other_)) 786 return false; 787 if (!(other_ instanceof CDSHooksRequestPrefetchComponent)) 788 return false; 789 CDSHooksRequestPrefetchComponent o = (CDSHooksRequestPrefetchComponent) other_; 790 return compareValues(key, o.key, true); 791 } 792 793 public boolean isEmpty() { 794 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, value); 795 } 796 797 public String fhirType() { 798 return "CDSHooksRequest.prefetch"; 799 800 } 801 802 } 803 804 /** 805 * The hook that triggered this CDS Service call 806 */ 807 @Child(name = "hook", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=false) 808 @Description(shortDefinition="The hook that triggered this CDS Service call", formalDefinition="The hook that triggered this CDS Service call" ) 809 protected CodeType hook; 810 811 /** 812 * While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation 813 */ 814 @Child(name = "hookInstance", type = {UuidType.class}, order=1, min=1, max=1, modifier=false, summary=false) 815 @Description(shortDefinition="A universally unique identifier (UUID) for this particular hook call", formalDefinition="While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation" ) 816 protected UuidType hookInstance; 817 818 /** 819 * The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged 820 */ 821 @Child(name = "fhirServer", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=false) 822 @Description(shortDefinition="The base URL of the CDS Client's FHIR server", formalDefinition="The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged" ) 823 protected UrlType fhirServer; 824 825 /** 826 * A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token 827 */ 828 @Child(name = "fhirAuthorization", type = {CDSHooksElement.class}, order=3, min=0, max=1, modifier=false, summary=false) 829 @Description(shortDefinition="A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token", formalDefinition="A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token" ) 830 protected CDSHooksRequestFhirAuthorizationComponent fhirAuthorization; 831 832 /** 833 * Hook-specific contextual data that the CDS service will need 834 */ 835 @Child(name = "context", type = {CDSHookContext.class}, order=4, min=1, max=1, modifier=false, summary=false) 836 @Description(shortDefinition="Hook-specific contextual data that the CDS service will need", formalDefinition="Hook-specific contextual data that the CDS service will need" ) 837 protected CDSHookContext context; 838 839 /** 840 * An object containing key/value pairs of FHIR queries that this Request is requesting the CDS Client to perform and provide on each Request call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query 841 */ 842 @Child(name = "prefetch", type = {Base.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 843 @Description(shortDefinition="Key/value pairs of FHIR queries the CDS Client provides on each call", formalDefinition="An object containing key/value pairs of FHIR queries that this Request is requesting the CDS Client to perform and provide on each Request call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query" ) 844 protected List<CDSHooksRequestPrefetchComponent> prefetchList; 845 846 private static final long serialVersionUID = 1427298453L; 847 848 /** 849 * Constructor 850 */ 851 public CDSHooksRequest() { 852 super(); 853 } 854 855 /** 856 * Constructor 857 */ 858 public CDSHooksRequest(String hook, String hookInstance, CDSHookContext context) { 859 super(); 860 this.setHook(hook); 861 this.setHookInstance(hookInstance); 862 this.setContext(context); 863 } 864 865 /** 866 * @return {@link #hook} (The hook that triggered this CDS Service call). This is the underlying object with id, value and extensions. The accessor "getHook" gives direct access to the value 867 */ 868 public CodeType getHookElement() { 869 if (this.hook == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create CDSHooksRequest.hook"); 872 else if (Configuration.doAutoCreate()) 873 this.hook = new CodeType(); // bb 874 return this.hook; 875 } 876 877 public boolean hasHookElement() { 878 return this.hook != null && !this.hook.isEmpty(); 879 } 880 881 public boolean hasHook() { 882 return this.hook != null && !this.hook.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #hook} (The hook that triggered this CDS Service call). This is the underlying object with id, value and extensions. The accessor "getHook" gives direct access to the value 887 */ 888 public CDSHooksRequest setHookElement(CodeType value) { 889 this.hook = value; 890 return this; 891 } 892 893 /** 894 * @return The hook that triggered this CDS Service call 895 */ 896 public String getHook() { 897 return this.hook == null ? null : this.hook.getValue(); 898 } 899 900 /** 901 * @param value The hook that triggered this CDS Service call 902 */ 903 public CDSHooksRequest setHook(String value) { 904 if (this.hook == null) 905 this.hook = new CodeType(); 906 this.hook.setValue(value); 907 return this; 908 } 909 910 /** 911 * @return {@link #hookInstance} (While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation). This is the underlying object with id, value and extensions. The accessor "getHookInstance" gives direct access to the value 912 */ 913 public UuidType getHookInstanceElement() { 914 if (this.hookInstance == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create CDSHooksRequest.hookInstance"); 917 else if (Configuration.doAutoCreate()) 918 this.hookInstance = new UuidType(); // bb 919 return this.hookInstance; 920 } 921 922 public boolean hasHookInstanceElement() { 923 return this.hookInstance != null && !this.hookInstance.isEmpty(); 924 } 925 926 public boolean hasHookInstance() { 927 return this.hookInstance != null && !this.hookInstance.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #hookInstance} (While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation). This is the underlying object with id, value and extensions. The accessor "getHookInstance" gives direct access to the value 932 */ 933 public CDSHooksRequest setHookInstanceElement(UuidType value) { 934 this.hookInstance = value; 935 return this; 936 } 937 938 /** 939 * @return While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation 940 */ 941 public String getHookInstance() { 942 return this.hookInstance == null ? null : this.hookInstance.getValue(); 943 } 944 945 /** 946 * @param value While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation 947 */ 948 public CDSHooksRequest setHookInstance(String value) { 949 if (this.hookInstance == null) 950 this.hookInstance = new UuidType(); 951 this.hookInstance.setValue(value); 952 return this; 953 } 954 955 /** 956 * @return {@link #fhirServer} (The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged). This is the underlying object with id, value and extensions. The accessor "getFhirServer" gives direct access to the value 957 */ 958 public UrlType getFhirServerElement() { 959 if (this.fhirServer == null) 960 if (Configuration.errorOnAutoCreate()) 961 throw new Error("Attempt to auto-create CDSHooksRequest.fhirServer"); 962 else if (Configuration.doAutoCreate()) 963 this.fhirServer = new UrlType(); // bb 964 return this.fhirServer; 965 } 966 967 public boolean hasFhirServerElement() { 968 return this.fhirServer != null && !this.fhirServer.isEmpty(); 969 } 970 971 public boolean hasFhirServer() { 972 return this.fhirServer != null && !this.fhirServer.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #fhirServer} (The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged). This is the underlying object with id, value and extensions. The accessor "getFhirServer" gives direct access to the value 977 */ 978 public CDSHooksRequest setFhirServerElement(UrlType value) { 979 this.fhirServer = value; 980 return this; 981 } 982 983 /** 984 * @return The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged 985 */ 986 public String getFhirServer() { 987 return this.fhirServer == null ? null : this.fhirServer.getValue(); 988 } 989 990 /** 991 * @param value The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged 992 */ 993 public CDSHooksRequest setFhirServer(String value) { 994 if (Utilities.noString(value)) 995 this.fhirServer = null; 996 else { 997 if (this.fhirServer == null) 998 this.fhirServer = new UrlType(); 999 this.fhirServer.setValue(value); 1000 } 1001 return this; 1002 } 1003 1004 /** 1005 * @return {@link #fhirAuthorization} (A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token) 1006 */ 1007 public CDSHooksRequestFhirAuthorizationComponent getFhirAuthorization() { 1008 return this.fhirAuthorization; 1009 } 1010 1011 public boolean hasFhirAuthorization() { 1012 return this.fhirAuthorization != null && !this.fhirAuthorization.isEmpty(); 1013 } 1014 1015 /** 1016 * @param value {@link #fhirAuthorization} (A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token) 1017 */ 1018 public CDSHooksRequest setFhirAuthorization(CDSHooksRequestFhirAuthorizationComponent value) { 1019 this.fhirAuthorization = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #context} (Hook-specific contextual data that the CDS service will need) 1025 */ 1026 public CDSHookContext getContext() { 1027 return this.context; 1028 } 1029 1030 public boolean hasContext() { 1031 return this.context != null && !this.context.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #context} (Hook-specific contextual data that the CDS service will need) 1036 */ 1037 public CDSHooksRequest setContext(CDSHookContext value) { 1038 this.context = value; 1039 return this; 1040 } 1041 1042 /** 1043 * @return {@link #prefetch} (An object containing key/value pairs of FHIR queries that this Request is requesting the CDS Client to perform and provide on each Request call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query) 1044 */ 1045 public List<CDSHooksRequestPrefetchComponent> getPrefetchList() { 1046 if (this.prefetchList == null) 1047 this.prefetchList = new ArrayList<CDSHooksRequestPrefetchComponent>(); 1048 return this.prefetchList; 1049 } 1050 1051 /** 1052 * @return Returns a reference to <code>this</code> for easy method chaining 1053 */ 1054 public CDSHooksRequest setPrefetchList(List<CDSHooksRequestPrefetchComponent> thePrefetch) { 1055 this.prefetchList = thePrefetch; 1056 return this; 1057 } 1058 1059 public boolean hasPrefetch() { 1060 if (this.prefetchList == null) 1061 return false; 1062 for (CDSHooksRequestPrefetchComponent item : this.prefetchList) 1063 if (!item.isEmpty()) 1064 return true; 1065 return false; 1066 } 1067 1068 public CDSHooksRequest addPrefetch(CDSHooksRequestPrefetchComponent t) { //3b 1069 if (t == null) 1070 return this; 1071 if (this.prefetchList == null) 1072 this.prefetchList = new ArrayList<CDSHooksRequestPrefetchComponent>(); 1073 this.prefetchList.add(t); 1074 return this; 1075 } 1076 1077 protected void listChildren(List<Property> children) { 1078 super.listChildren(children); 1079 children.add(new Property("hook", "code", "The hook that triggered this CDS Service call", 0, 1, hook)); 1080 children.add(new Property("hookInstance", "uuid", "While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation", 0, 1, hookInstance)); 1081 children.add(new Property("fhirServer", "url", "The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged", 0, 1, fhirServer)); 1082 children.add(new Property("fhirAuthorization", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token", 0, 1, fhirAuthorization)); 1083 children.add(new Property("context", "http://hl7.org/fhir/tools/StructureDefinition/CDSHookContext", "Hook-specific contextual data that the CDS service will need", 0, 1, context)); 1084 children.add(new Property("prefetch", "Base", "An object containing key/value pairs of FHIR queries that this Request is requesting the CDS Client to perform and provide on each Request call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query", 0, java.lang.Integer.MAX_VALUE, prefetchList)); 1085 } 1086 1087 @Override 1088 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1089 switch (_hash) { 1090 case 3208483: /*hook*/ return new Property("hook", "code", "The hook that triggered this CDS Service call", 0, 1, hook); 1091 case -1195894056: /*hookInstance*/ return new Property("hookInstance", "uuid", "While working in the CDS Client, a user can perform multiple actions in series or in parallel. For example, a clinician might prescribe two drugs in a row; each prescription action would be assigned a unique hookInstance. This allows a CDS Service to uniquely identify each hook invocation", 0, 1, hookInstance); 1092 case 1314459790: /*fhirServer*/ return new Property("fhirServer", "url", "The base URL of the CDS Client's FHIR server. If fhirAuthorization is provided, this field is REQUIRED. The scheme MUST be https when production data is exchanged", 0, 1, fhirServer); 1093 case 331089102: /*fhirAuthorization*/ return new Property("fhirAuthorization", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "A structure holding an OAuth 2.0 bearer access token granting the CDS Service access to FHIR resources, along with supplemental information relating to the token", 0, 1, fhirAuthorization); 1094 case 951530927: /*context*/ return new Property("context", "http://hl7.org/fhir/tools/StructureDefinition/CDSHookContext", "Hook-specific contextual data that the CDS service will need", 0, 1, context); 1095 case -1288666633: /*prefetch*/ return new Property("prefetch", "Base", "An object containing key/value pairs of FHIR queries that this Request is requesting the CDS Client to perform and provide on each Request call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query", 0, java.lang.Integer.MAX_VALUE, prefetchList); 1096 default: return super.getNamedProperty(_hash, _name, _checkValid); 1097 } 1098 1099 } 1100 1101 @Override 1102 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1103 switch (hash) { 1104 case 3208483: /*hook*/ return this.hook == null ? new Base[0] : new Base[] {this.hook}; // CodeType 1105 case -1195894056: /*hookInstance*/ return this.hookInstance == null ? new Base[0] : new Base[] {this.hookInstance}; // UuidType 1106 case 1314459790: /*fhirServer*/ return this.fhirServer == null ? new Base[0] : new Base[] {this.fhirServer}; // UrlType 1107 case 331089102: /*fhirAuthorization*/ return this.fhirAuthorization == null ? new Base[0] : new Base[] {this.fhirAuthorization}; // CDSHooksRequestFhirAuthorizationComponent 1108 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // CDSHookContext 1109 case -1288666633: /*prefetch*/ return this.prefetchList == null ? new Base[0] : this.prefetchList.toArray(new Base[this.prefetchList.size()]); // CDSHooksRequestPrefetchComponent 1110 default: return super.getProperty(hash, name, checkValid); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1117 switch (hash) { 1118 case 3208483: // hook 1119 this.hook = TypeConvertor.castToCode(value); // CodeType 1120 return value; 1121 case -1195894056: // hookInstance 1122 this.hookInstance = TypeConvertor.castToUuid(value); // UuidType 1123 return value; 1124 case 1314459790: // fhirServer 1125 this.fhirServer = TypeConvertor.castToUrl(value); // UrlType 1126 return value; 1127 case 331089102: // fhirAuthorization 1128 this.fhirAuthorization = (CDSHooksRequestFhirAuthorizationComponent) value; // CDSHooksRequestFhirAuthorizationComponent 1129 return value; 1130 case 951530927: // context 1131 this.context = (CDSHookContext) value; // CDSHookContext 1132 return value; 1133 case -1288666633: // prefetch 1134 this.getPrefetchList().add((CDSHooksRequestPrefetchComponent) value); // CDSHooksRequestPrefetchComponent 1135 return value; 1136 default: return super.setProperty(hash, name, value); 1137 } 1138 1139 } 1140 1141 @Override 1142 public Base setProperty(String name, Base value) throws FHIRException { 1143 if (name.equals("hook")) { 1144 this.hook = TypeConvertor.castToCode(value); // CodeType 1145 } else if (name.equals("hookInstance")) { 1146 this.hookInstance = TypeConvertor.castToUuid(value); // UuidType 1147 } else if (name.equals("fhirServer")) { 1148 this.fhirServer = TypeConvertor.castToUrl(value); // UrlType 1149 } else if (name.equals("fhirAuthorization")) { 1150 this.fhirAuthorization = (CDSHooksRequestFhirAuthorizationComponent) value; // CDSHooksRequestFhirAuthorizationComponent 1151 } else if (name.equals("context")) { 1152 this.context = (CDSHookContext) value; // CDSHookContext 1153 } else if (name.equals("prefetch")) { 1154 this.getPrefetchList().add((CDSHooksRequestPrefetchComponent) value); // CDSHooksRequestPrefetchComponent 1155 } else 1156 return super.setProperty(name, value); 1157 return value; 1158 } 1159 1160 @Override 1161 public Base makeProperty(int hash, String name) throws FHIRException { 1162 switch (hash) { 1163 case 3208483: return getHookElement(); 1164 case -1195894056: return getHookInstanceElement(); 1165 case 1314459790: return getFhirServerElement(); 1166 case 331089102: return getFhirAuthorization(); 1167 case 951530927: /*div*/ 1168 throw new Error("Unable to make an instance of the abstract property 'context'"); 1169 case -1288666633: /*div*/ 1170 throw new Error("Unable to make an instance of the abstract property 'prefetch'"); 1171 default: return super.makeProperty(hash, name); 1172 } 1173 1174 } 1175 1176 @Override 1177 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1178 switch (hash) { 1179 case 3208483: /*hook*/ return new String[] {"code"}; 1180 case -1195894056: /*hookInstance*/ return new String[] {"uuid"}; 1181 case 1314459790: /*fhirServer*/ return new String[] {"url"}; 1182 case 331089102: /*fhirAuthorization*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 1183 case 951530927: /*context*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHookContext"}; 1184 case -1288666633: /*prefetch*/ return new String[] {"Base"}; 1185 default: return super.getTypesForProperty(hash, name); 1186 } 1187 1188 } 1189 1190 @Override 1191 public Base addChild(String name) throws FHIRException { 1192 if (name.equals("hook")) { 1193 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.hook"); 1194 } 1195 else if (name.equals("hookInstance")) { 1196 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.hookInstance"); 1197 } 1198 else if (name.equals("fhirServer")) { 1199 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksRequest.fhirServer"); 1200 } 1201 else if (name.equals("fhirAuthorization")) { 1202 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksRequest.fhirAuthorization"); 1203 } 1204 else if (name.equals("context")) { 1205 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksRequest.context"); 1206 } 1207 else if (name.equals("prefetch")) { 1208 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksRequest.prefetch"); 1209 } 1210 else 1211 return super.addChild(name); 1212 } 1213 1214 public String fhirType() { 1215 return "CDSHooksRequest"; 1216 1217 } 1218 1219 public CDSHooksRequest copy() { 1220 CDSHooksRequest dst = new CDSHooksRequest(); 1221 copyValues(dst); 1222 return dst; 1223 } 1224 1225 public void copyValues(CDSHooksRequest dst) { 1226 super.copyValues(dst); 1227 dst.hook = hook == null ? null : hook.copy(); 1228 dst.hookInstance = hookInstance == null ? null : hookInstance.copy(); 1229 dst.fhirServer = fhirServer == null ? null : fhirServer.copy(); 1230 dst.fhirAuthorization = fhirAuthorization == null ? null : fhirAuthorization.copy(); 1231 dst.context = context == null ? null : context.copy(); 1232 if (prefetchList != null) { 1233 dst.prefetchList = new ArrayList<CDSHooksRequestPrefetchComponent>(); 1234 for (CDSHooksRequestPrefetchComponent i : prefetchList) 1235 dst.prefetchList.add(i.copy()); 1236 }; 1237 } 1238 1239 protected CDSHooksRequest typedCopy() { 1240 return copy(); 1241 } 1242 1243 @Override 1244 public boolean equalsDeep(Base other_) { 1245 if (!super.equalsDeep(other_)) 1246 return false; 1247 if (!(other_ instanceof CDSHooksRequest)) 1248 return false; 1249 CDSHooksRequest o = (CDSHooksRequest) other_; 1250 return compareDeep(hook, o.hook, true) && compareDeep(hookInstance, o.hookInstance, true) && compareDeep(fhirServer, o.fhirServer, true) 1251 && compareDeep(fhirAuthorization, o.fhirAuthorization, true) && compareDeep(context, o.context, true) 1252 && compareDeep(prefetchList, o.prefetchList, true); 1253 } 1254 1255 @Override 1256 public boolean equalsShallow(Base other_) { 1257 if (!super.equalsShallow(other_)) 1258 return false; 1259 if (!(other_ instanceof CDSHooksRequest)) 1260 return false; 1261 CDSHooksRequest o = (CDSHooksRequest) other_; 1262 return compareValues(hook, o.hook, true) && compareValues(hookInstance, o.hookInstance, true) && compareValues(fhirServer, o.fhirServer, true) 1263 ; 1264 } 1265 1266 public boolean isEmpty() { 1267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(hook, hookInstance, fhirServer 1268 , fhirAuthorization, context, prefetchList); 1269 } 1270 1271 1272} 1273