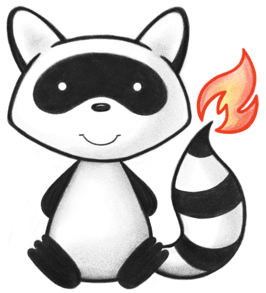
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Response body. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHooksResponse") 052public class CDSHooksResponse extends CDSHooksElement implements ICompositeType { 053 054 public enum CDSActionTypeCodesVS { 055 /** 056 * Create this resource 057 */ 058 CREATE, 059 /** 060 * Update this resource 061 */ 062 UPDATE, 063 /** 064 * Delete this resource 065 */ 066 DELETE, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 public static CDSActionTypeCodesVS fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("create".equals(codeString)) 075 return CREATE; 076 if ("update".equals(codeString)) 077 return UPDATE; 078 if ("delete".equals(codeString)) 079 return DELETE; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown CDSActionTypeCodesVS code '"+codeString+"'"); 084 } 085 public String toCode() { 086 switch (this) { 087 case CREATE: return "create"; 088 case UPDATE: return "update"; 089 case DELETE: return "delete"; 090 case NULL: return null; 091 default: return "?"; 092 } 093 } 094 public String getSystem() { 095 switch (this) { 096 case CREATE: return "http://hl7.org/fhir/tools/CodeSystem/CDSActionType"; 097 case UPDATE: return "http://hl7.org/fhir/tools/CodeSystem/CDSActionType"; 098 case DELETE: return "http://hl7.org/fhir/tools/CodeSystem/CDSActionType"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDefinition() { 104 switch (this) { 105 case CREATE: return "Create this resource"; 106 case UPDATE: return "Update this resource"; 107 case DELETE: return "Delete this resource"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDisplay() { 113 switch (this) { 114 case CREATE: return "create"; 115 case UPDATE: return "update"; 116 case DELETE: return "delete"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 } 122 123 public static class CDSActionTypeCodesVSEnumFactory implements EnumFactory<CDSActionTypeCodesVS> { 124 public CDSActionTypeCodesVS fromCode(String codeString) throws IllegalArgumentException { 125 if (codeString == null || "".equals(codeString)) 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("create".equals(codeString)) 129 return CDSActionTypeCodesVS.CREATE; 130 if ("update".equals(codeString)) 131 return CDSActionTypeCodesVS.UPDATE; 132 if ("delete".equals(codeString)) 133 return CDSActionTypeCodesVS.DELETE; 134 throw new IllegalArgumentException("Unknown CDSActionTypeCodesVS code '"+codeString+"'"); 135 } 136 public Enumeration<CDSActionTypeCodesVS> fromType(PrimitiveType<?> code) throws FHIRException { 137 if (code == null) 138 return null; 139 if (code.isEmpty()) 140 return new Enumeration<CDSActionTypeCodesVS>(this, CDSActionTypeCodesVS.NULL, code); 141 String codeString = ((PrimitiveType) code).asStringValue(); 142 if (codeString == null || "".equals(codeString)) 143 return new Enumeration<CDSActionTypeCodesVS>(this, CDSActionTypeCodesVS.NULL, code); 144 if ("create".equals(codeString)) 145 return new Enumeration<CDSActionTypeCodesVS>(this, CDSActionTypeCodesVS.CREATE, code); 146 if ("update".equals(codeString)) 147 return new Enumeration<CDSActionTypeCodesVS>(this, CDSActionTypeCodesVS.UPDATE, code); 148 if ("delete".equals(codeString)) 149 return new Enumeration<CDSActionTypeCodesVS>(this, CDSActionTypeCodesVS.DELETE, code); 150 throw new FHIRException("Unknown CDSActionTypeCodesVS code '"+codeString+"'"); 151 } 152 public String toCode(CDSActionTypeCodesVS code) { 153 if (code == CDSActionTypeCodesVS.CREATE) 154 return "create"; 155 if (code == CDSActionTypeCodesVS.UPDATE) 156 return "update"; 157 if (code == CDSActionTypeCodesVS.DELETE) 158 return "delete"; 159 return "?"; 160 } 161 public String toSystem(CDSActionTypeCodesVS code) { 162 return code.getSystem(); 163 } 164 } 165 166 public enum CDSIndicatorCodesVS { 167 /** 168 * An information message 169 */ 170 INFO, 171 /** 172 * A warning message 173 */ 174 WARNING, 175 /** 176 * An error message 177 */ 178 ERROR, 179 /** 180 * added to help the parsers with the generic types 181 */ 182 NULL; 183 public static CDSIndicatorCodesVS fromCode(String codeString) throws FHIRException { 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("info".equals(codeString)) 187 return INFO; 188 if ("warning".equals(codeString)) 189 return WARNING; 190 if ("error".equals(codeString)) 191 return ERROR; 192 if (Configuration.isAcceptInvalidEnums()) 193 return null; 194 else 195 throw new FHIRException("Unknown CDSIndicatorCodesVS code '"+codeString+"'"); 196 } 197 public String toCode() { 198 switch (this) { 199 case INFO: return "info"; 200 case WARNING: return "warning"; 201 case ERROR: return "error"; 202 case NULL: return null; 203 default: return "?"; 204 } 205 } 206 public String getSystem() { 207 switch (this) { 208 case INFO: return "http://hl7.org/fhir/tools/CodeSystem/CDSIndicator"; 209 case WARNING: return "http://hl7.org/fhir/tools/CodeSystem/CDSIndicator"; 210 case ERROR: return "http://hl7.org/fhir/tools/CodeSystem/CDSIndicator"; 211 case NULL: return null; 212 default: return "?"; 213 } 214 } 215 public String getDefinition() { 216 switch (this) { 217 case INFO: return "An information message"; 218 case WARNING: return "A warning message"; 219 case ERROR: return "An error message"; 220 case NULL: return null; 221 default: return "?"; 222 } 223 } 224 public String getDisplay() { 225 switch (this) { 226 case INFO: return "info"; 227 case WARNING: return "warning"; 228 case ERROR: return "error"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 } 234 235 public static class CDSIndicatorCodesVSEnumFactory implements EnumFactory<CDSIndicatorCodesVS> { 236 public CDSIndicatorCodesVS fromCode(String codeString) throws IllegalArgumentException { 237 if (codeString == null || "".equals(codeString)) 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("info".equals(codeString)) 241 return CDSIndicatorCodesVS.INFO; 242 if ("warning".equals(codeString)) 243 return CDSIndicatorCodesVS.WARNING; 244 if ("error".equals(codeString)) 245 return CDSIndicatorCodesVS.ERROR; 246 throw new IllegalArgumentException("Unknown CDSIndicatorCodesVS code '"+codeString+"'"); 247 } 248 public Enumeration<CDSIndicatorCodesVS> fromType(PrimitiveType<?> code) throws FHIRException { 249 if (code == null) 250 return null; 251 if (code.isEmpty()) 252 return new Enumeration<CDSIndicatorCodesVS>(this, CDSIndicatorCodesVS.NULL, code); 253 String codeString = ((PrimitiveType) code).asStringValue(); 254 if (codeString == null || "".equals(codeString)) 255 return new Enumeration<CDSIndicatorCodesVS>(this, CDSIndicatorCodesVS.NULL, code); 256 if ("info".equals(codeString)) 257 return new Enumeration<CDSIndicatorCodesVS>(this, CDSIndicatorCodesVS.INFO, code); 258 if ("warning".equals(codeString)) 259 return new Enumeration<CDSIndicatorCodesVS>(this, CDSIndicatorCodesVS.WARNING, code); 260 if ("error".equals(codeString)) 261 return new Enumeration<CDSIndicatorCodesVS>(this, CDSIndicatorCodesVS.ERROR, code); 262 throw new FHIRException("Unknown CDSIndicatorCodesVS code '"+codeString+"'"); 263 } 264 public String toCode(CDSIndicatorCodesVS code) { 265 if (code == CDSIndicatorCodesVS.INFO) 266 return "info"; 267 if (code == CDSIndicatorCodesVS.WARNING) 268 return "warning"; 269 if (code == CDSIndicatorCodesVS.ERROR) 270 return "error"; 271 return "?"; 272 } 273 public String toSystem(CDSIndicatorCodesVS code) { 274 return code.getSystem(); 275 } 276 } 277 278 public enum CDSLinkTypeCodesVS { 279 /** 280 * Indicates that the URL is absolute and should be treated as-is. 281 */ 282 ABSOLUTE, 283 /** 284 * indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters 285 */ 286 SMART, 287 /** 288 * added to help the parsers with the generic types 289 */ 290 NULL; 291 public static CDSLinkTypeCodesVS fromCode(String codeString) throws FHIRException { 292 if (codeString == null || "".equals(codeString)) 293 return null; 294 if ("absolute".equals(codeString)) 295 return ABSOLUTE; 296 if ("smart".equals(codeString)) 297 return SMART; 298 if (Configuration.isAcceptInvalidEnums()) 299 return null; 300 else 301 throw new FHIRException("Unknown CDSLinkTypeCodesVS code '"+codeString+"'"); 302 } 303 public String toCode() { 304 switch (this) { 305 case ABSOLUTE: return "absolute"; 306 case SMART: return "smart"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 public String getSystem() { 312 switch (this) { 313 case ABSOLUTE: return "http://hl7.org/fhir/tools/CodeSystem/CDSLinkType"; 314 case SMART: return "http://hl7.org/fhir/tools/CodeSystem/CDSLinkType"; 315 case NULL: return null; 316 default: return "?"; 317 } 318 } 319 public String getDefinition() { 320 switch (this) { 321 case ABSOLUTE: return "Indicates that the URL is absolute and should be treated as-is."; 322 case SMART: return "indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters"; 323 case NULL: return null; 324 default: return "?"; 325 } 326 } 327 public String getDisplay() { 328 switch (this) { 329 case ABSOLUTE: return "absolute"; 330 case SMART: return "smart"; 331 case NULL: return null; 332 default: return "?"; 333 } 334 } 335 } 336 337 public static class CDSLinkTypeCodesVSEnumFactory implements EnumFactory<CDSLinkTypeCodesVS> { 338 public CDSLinkTypeCodesVS fromCode(String codeString) throws IllegalArgumentException { 339 if (codeString == null || "".equals(codeString)) 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("absolute".equals(codeString)) 343 return CDSLinkTypeCodesVS.ABSOLUTE; 344 if ("smart".equals(codeString)) 345 return CDSLinkTypeCodesVS.SMART; 346 throw new IllegalArgumentException("Unknown CDSLinkTypeCodesVS code '"+codeString+"'"); 347 } 348 public Enumeration<CDSLinkTypeCodesVS> fromType(PrimitiveType<?> code) throws FHIRException { 349 if (code == null) 350 return null; 351 if (code.isEmpty()) 352 return new Enumeration<CDSLinkTypeCodesVS>(this, CDSLinkTypeCodesVS.NULL, code); 353 String codeString = ((PrimitiveType) code).asStringValue(); 354 if (codeString == null || "".equals(codeString)) 355 return new Enumeration<CDSLinkTypeCodesVS>(this, CDSLinkTypeCodesVS.NULL, code); 356 if ("absolute".equals(codeString)) 357 return new Enumeration<CDSLinkTypeCodesVS>(this, CDSLinkTypeCodesVS.ABSOLUTE, code); 358 if ("smart".equals(codeString)) 359 return new Enumeration<CDSLinkTypeCodesVS>(this, CDSLinkTypeCodesVS.SMART, code); 360 throw new FHIRException("Unknown CDSLinkTypeCodesVS code '"+codeString+"'"); 361 } 362 public String toCode(CDSLinkTypeCodesVS code) { 363 if (code == CDSLinkTypeCodesVS.ABSOLUTE) 364 return "absolute"; 365 if (code == CDSLinkTypeCodesVS.SMART) 366 return "smart"; 367 return "?"; 368 } 369 public String toSystem(CDSLinkTypeCodesVS code) { 370 return code.getSystem(); 371 } 372 } 373 374 public enum CDSSelectionBehaviorCodesVS { 375 /** 376 * indicates that the user may choose none or at most one of the suggestions 377 */ 378 ATMOSTONE, 379 /** 380 * indicates that the end user may choose any number of suggestions including none of them and all of them 381 */ 382 ANY, 383 /** 384 * added to help the parsers with the generic types 385 */ 386 NULL; 387 public static CDSSelectionBehaviorCodesVS fromCode(String codeString) throws FHIRException { 388 if (codeString == null || "".equals(codeString)) 389 return null; 390 if ("at-most-one".equals(codeString)) 391 return ATMOSTONE; 392 if ("any".equals(codeString)) 393 return ANY; 394 if (Configuration.isAcceptInvalidEnums()) 395 return null; 396 else 397 throw new FHIRException("Unknown CDSSelectionBehaviorCodesVS code '"+codeString+"'"); 398 } 399 public String toCode() { 400 switch (this) { 401 case ATMOSTONE: return "at-most-one"; 402 case ANY: return "any"; 403 case NULL: return null; 404 default: return "?"; 405 } 406 } 407 public String getSystem() { 408 switch (this) { 409 case ATMOSTONE: return "http://hl7.org/fhir/tools/CodeSystem/CDSSelectionBehavior"; 410 case ANY: return "http://hl7.org/fhir/tools/CodeSystem/CDSSelectionBehavior"; 411 case NULL: return null; 412 default: return "?"; 413 } 414 } 415 public String getDefinition() { 416 switch (this) { 417 case ATMOSTONE: return "indicates that the user may choose none or at most one of the suggestions"; 418 case ANY: return "indicates that the end user may choose any number of suggestions including none of them and all of them"; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 public String getDisplay() { 424 switch (this) { 425 case ATMOSTONE: return "at-most-one"; 426 case ANY: return "any"; 427 case NULL: return null; 428 default: return "?"; 429 } 430 } 431 } 432 433 public static class CDSSelectionBehaviorCodesVSEnumFactory implements EnumFactory<CDSSelectionBehaviorCodesVS> { 434 public CDSSelectionBehaviorCodesVS fromCode(String codeString) throws IllegalArgumentException { 435 if (codeString == null || "".equals(codeString)) 436 if (codeString == null || "".equals(codeString)) 437 return null; 438 if ("at-most-one".equals(codeString)) 439 return CDSSelectionBehaviorCodesVS.ATMOSTONE; 440 if ("any".equals(codeString)) 441 return CDSSelectionBehaviorCodesVS.ANY; 442 throw new IllegalArgumentException("Unknown CDSSelectionBehaviorCodesVS code '"+codeString+"'"); 443 } 444 public Enumeration<CDSSelectionBehaviorCodesVS> fromType(PrimitiveType<?> code) throws FHIRException { 445 if (code == null) 446 return null; 447 if (code.isEmpty()) 448 return new Enumeration<CDSSelectionBehaviorCodesVS>(this, CDSSelectionBehaviorCodesVS.NULL, code); 449 String codeString = ((PrimitiveType) code).asStringValue(); 450 if (codeString == null || "".equals(codeString)) 451 return new Enumeration<CDSSelectionBehaviorCodesVS>(this, CDSSelectionBehaviorCodesVS.NULL, code); 452 if ("at-most-one".equals(codeString)) 453 return new Enumeration<CDSSelectionBehaviorCodesVS>(this, CDSSelectionBehaviorCodesVS.ATMOSTONE, code); 454 if ("any".equals(codeString)) 455 return new Enumeration<CDSSelectionBehaviorCodesVS>(this, CDSSelectionBehaviorCodesVS.ANY, code); 456 throw new FHIRException("Unknown CDSSelectionBehaviorCodesVS code '"+codeString+"'"); 457 } 458 public String toCode(CDSSelectionBehaviorCodesVS code) { 459 if (code == CDSSelectionBehaviorCodesVS.ATMOSTONE) 460 return "at-most-one"; 461 if (code == CDSSelectionBehaviorCodesVS.ANY) 462 return "any"; 463 return "?"; 464 } 465 public String toSystem(CDSSelectionBehaviorCodesVS code) { 466 return code.getSystem(); 467 } 468 } 469 470 @Block() 471 public static class CDSHooksResponseCardsComponent extends CDSHooksElement { 472 /** 473 * Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint. 474 */ 475 @Child(name = "uuid", type = {UuidType.class}, order=1, min=0, max=1, modifier=false, summary=false) 476 @Description(shortDefinition="Unique identifier of the card (for logging/feedback)", formalDefinition="Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint." ) 477 protected UuidType uuid; 478 479 /** 480 * One-sentence, <140-character summary message for display to the user inside of this card. 481 */ 482 @Child(name = "summary", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 483 @Description(shortDefinition="Summary message for display to the user (<140 char)", formalDefinition="One-sentence, <140-character summary message for display to the user inside of this card." ) 484 protected StringType summary; 485 486 /** 487 * Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...'). 488 */ 489 @Child(name = "detail", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 490 @Description(shortDefinition="Optional detailed information to display (GitHub Flavored Markdown)", formalDefinition="Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...')." ) 491 protected MarkdownType detail; 492 493 /** 494 * Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical. 495 */ 496 @Child(name = "indicator", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 497 @Description(shortDefinition="info, warning, critical - Urgency/importance of what this card conveys", formalDefinition="Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical." ) 498 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/tools/ValueSet/CDSIndicator") 499 protected Enumeration<CDSIndicatorCodesVS> indicator; 500 501 /** 502 * The source should be the primary source of guidance for the decision support the card represents. 503 */ 504 @Child(name = "source", type = {CDSHooksElement.class}, order=5, min=1, max=1, modifier=false, summary=false) 505 @Description(shortDefinition="The primary source of guidance for the content the card represents.", formalDefinition="The source should be the primary source of guidance for the decision support the card represents." ) 506 protected CDSHooksResponseCardsSourceComponent source; 507 508 /** 509 * Allows a service to suggest a set of changes in the context of the current activity (e.g. changing the dose of a medication currently being prescribed, for the order-sign activity). 510 */ 511 @Child(name = "suggestions", type = {CDSHooksElement.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 512 @Description(shortDefinition="Suggest a set of changes in the context of the current activity", formalDefinition="Allows a service to suggest a set of changes in the context of the current activity (e.g. changing the dose of a medication currently being prescribed, for the order-sign activity)." ) 513 protected List<CDSHooksResponseCardsSuggestionsComponent> suggestionsList; 514 515 /** 516 * Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them 517 */ 518 @Child(name = "selectionBehavior", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 519 @Description(shortDefinition="at-most-one, any - intended selection behavior of the suggestions in the card", formalDefinition="Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them" ) 520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/tools/ValueSet/CDSSelectionBehavior") 521 protected Enumeration<CDSSelectionBehaviorCodesVS> selectionBehavior; 522 523 /** 524 * Override reasons can be selected by the end user when overriding a card without taking the suggested recommendations. The CDS service MAY return a list of override reasons to the CDS client. 525 */ 526 @Child(name = "overrideReasons", type = {Coding.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 527 @Description(shortDefinition="Can be selected by the end user when overriding a card without taking the suggested recommendations.", formalDefinition="Override reasons can be selected by the end user when overriding a card without taking the suggested recommendations. The CDS service MAY return a list of override reasons to the CDS client." ) 528 protected List<Coding> overrideReasonsList; 529 530 /** 531 * Allows a service to suggest a link to an app that the user might want to run for additional information or to help guide a decision. 532 */ 533 @Child(name = "links", type = {CDSHooksElement.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 534 @Description(shortDefinition="Link to an app that the user might want to run for additional information or to help guide a decision", formalDefinition="Allows a service to suggest a link to an app that the user might want to run for additional information or to help guide a decision." ) 535 protected List<CDSHooksResponseCardsLinksComponent> linksList; 536 537 private static final long serialVersionUID = -1553644263L; 538 539 /** 540 * Constructor 541 */ 542 public CDSHooksResponseCardsComponent() { 543 super(); 544 } 545 546 /** 547 * Constructor 548 */ 549 public CDSHooksResponseCardsComponent(String summary, CDSHooksResponseCardsSourceComponent source) { 550 super(); 551 this.setSummary(summary); 552 this.setSource(source); 553 } 554 555 /** 556 * @return {@link #uuid} (Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint.). This is the underlying object with id, value and extensions. The accessor "getUuid" gives direct access to the value 557 */ 558 public UuidType getUuidElement() { 559 if (this.uuid == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create CDSHooksResponseCardsComponent.uuid"); 562 else if (Configuration.doAutoCreate()) 563 this.uuid = new UuidType(); // bb 564 return this.uuid; 565 } 566 567 public boolean hasUuidElement() { 568 return this.uuid != null && !this.uuid.isEmpty(); 569 } 570 571 public boolean hasUuid() { 572 return this.uuid != null && !this.uuid.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #uuid} (Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint.). This is the underlying object with id, value and extensions. The accessor "getUuid" gives direct access to the value 577 */ 578 public CDSHooksResponseCardsComponent setUuidElement(UuidType value) { 579 this.uuid = value; 580 return this; 581 } 582 583 /** 584 * @return Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint. 585 */ 586 public String getUuid() { 587 return this.uuid == null ? null : this.uuid.getValue(); 588 } 589 590 /** 591 * @param value Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint. 592 */ 593 public CDSHooksResponseCardsComponent setUuid(String value) { 594 if (Utilities.noString(value)) 595 this.uuid = null; 596 else { 597 if (this.uuid == null) 598 this.uuid = new UuidType(); 599 this.uuid.setValue(value); 600 } 601 return this; 602 } 603 604 /** 605 * @return {@link #summary} (One-sentence, <140-character summary message for display to the user inside of this card.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 606 */ 607 public StringType getSummaryElement() { 608 if (this.summary == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create CDSHooksResponseCardsComponent.summary"); 611 else if (Configuration.doAutoCreate()) 612 this.summary = new StringType(); // bb 613 return this.summary; 614 } 615 616 public boolean hasSummaryElement() { 617 return this.summary != null && !this.summary.isEmpty(); 618 } 619 620 public boolean hasSummary() { 621 return this.summary != null && !this.summary.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #summary} (One-sentence, <140-character summary message for display to the user inside of this card.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 626 */ 627 public CDSHooksResponseCardsComponent setSummaryElement(StringType value) { 628 this.summary = value; 629 return this; 630 } 631 632 /** 633 * @return One-sentence, <140-character summary message for display to the user inside of this card. 634 */ 635 public String getSummary() { 636 return this.summary == null ? null : this.summary.getValue(); 637 } 638 639 /** 640 * @param value One-sentence, <140-character summary message for display to the user inside of this card. 641 */ 642 public CDSHooksResponseCardsComponent setSummary(String value) { 643 if (this.summary == null) 644 this.summary = new StringType(); 645 this.summary.setValue(value); 646 return this; 647 } 648 649 /** 650 * @return {@link #detail} (Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...').). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 651 */ 652 public MarkdownType getDetailElement() { 653 if (this.detail == null) 654 if (Configuration.errorOnAutoCreate()) 655 throw new Error("Attempt to auto-create CDSHooksResponseCardsComponent.detail"); 656 else if (Configuration.doAutoCreate()) 657 this.detail = new MarkdownType(); // bb 658 return this.detail; 659 } 660 661 public boolean hasDetailElement() { 662 return this.detail != null && !this.detail.isEmpty(); 663 } 664 665 public boolean hasDetail() { 666 return this.detail != null && !this.detail.isEmpty(); 667 } 668 669 /** 670 * @param value {@link #detail} (Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...').). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 671 */ 672 public CDSHooksResponseCardsComponent setDetailElement(MarkdownType value) { 673 this.detail = value; 674 return this; 675 } 676 677 /** 678 * @return Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...'). 679 */ 680 public String getDetail() { 681 return this.detail == null ? null : this.detail.getValue(); 682 } 683 684 /** 685 * @param value Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...'). 686 */ 687 public CDSHooksResponseCardsComponent setDetail(String value) { 688 if (Utilities.noString(value)) 689 this.detail = null; 690 else { 691 if (this.detail == null) 692 this.detail = new MarkdownType(); 693 this.detail.setValue(value); 694 } 695 return this; 696 } 697 698 /** 699 * @return {@link #indicator} (Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical.). This is the underlying object with id, value and extensions. The accessor "getIndicator" gives direct access to the value 700 */ 701 public Enumeration<CDSIndicatorCodesVS> getIndicatorElement() { 702 if (this.indicator == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create CDSHooksResponseCardsComponent.indicator"); 705 else if (Configuration.doAutoCreate()) 706 this.indicator = new Enumeration<CDSIndicatorCodesVS>(new CDSIndicatorCodesVSEnumFactory()); // bb 707 return this.indicator; 708 } 709 710 public boolean hasIndicatorElement() { 711 return this.indicator != null && !this.indicator.isEmpty(); 712 } 713 714 public boolean hasIndicator() { 715 return this.indicator != null && !this.indicator.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #indicator} (Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical.). This is the underlying object with id, value and extensions. The accessor "getIndicator" gives direct access to the value 720 */ 721 public CDSHooksResponseCardsComponent setIndicatorElement(Enumeration<CDSIndicatorCodesVS> value) { 722 this.indicator = value; 723 return this; 724 } 725 726 /** 727 * @return Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical. 728 */ 729 public CDSIndicatorCodesVS getIndicator() { 730 return this.indicator == null ? null : this.indicator.getValue(); 731 } 732 733 /** 734 * @param value Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical. 735 */ 736 public CDSHooksResponseCardsComponent setIndicator(CDSIndicatorCodesVS value) { 737 if (value == null) 738 this.indicator = null; 739 else { 740 if (this.indicator == null) 741 this.indicator = new Enumeration<CDSIndicatorCodesVS>(new CDSIndicatorCodesVSEnumFactory()); 742 this.indicator.setValue(value); 743 } 744 return this; 745 } 746 747 /** 748 * @return {@link #source} (The source should be the primary source of guidance for the decision support the card represents.) 749 */ 750 public CDSHooksResponseCardsSourceComponent getSource() { 751 return this.source; 752 } 753 754 public boolean hasSource() { 755 return this.source != null && !this.source.isEmpty(); 756 } 757 758 /** 759 * @param value {@link #source} (The source should be the primary source of guidance for the decision support the card represents.) 760 */ 761 public CDSHooksResponseCardsComponent setSource(CDSHooksResponseCardsSourceComponent value) { 762 this.source = value; 763 return this; 764 } 765 766 /** 767 * @return {@link #suggestions} (Allows a service to suggest a set of changes in the context of the current activity (e.g. changing the dose of a medication currently being prescribed, for the order-sign activity).) 768 */ 769 public List<CDSHooksResponseCardsSuggestionsComponent> getSuggestionsList() { 770 if (this.suggestionsList == null) 771 this.suggestionsList = new ArrayList<CDSHooksResponseCardsSuggestionsComponent>(); 772 return this.suggestionsList; 773 } 774 775 /** 776 * @return Returns a reference to <code>this</code> for easy method chaining 777 */ 778 public CDSHooksResponseCardsComponent setSuggestionsList(List<CDSHooksResponseCardsSuggestionsComponent> theSuggestions) { 779 this.suggestionsList = theSuggestions; 780 return this; 781 } 782 783 public boolean hasSuggestions() { 784 if (this.suggestionsList == null) 785 return false; 786 for (CDSHooksResponseCardsSuggestionsComponent item : this.suggestionsList) 787 if (!item.isEmpty()) 788 return true; 789 return false; 790 } 791 792 public CDSHooksResponseCardsSuggestionsComponent addSuggestions() { //3a 793 CDSHooksResponseCardsSuggestionsComponent t = new CDSHooksResponseCardsSuggestionsComponent(); 794 if (this.suggestionsList == null) 795 this.suggestionsList = new ArrayList<CDSHooksResponseCardsSuggestionsComponent>(); 796 this.suggestionsList.add(t); 797 return t; 798 } 799 800 public CDSHooksResponseCardsComponent addSuggestions(CDSHooksResponseCardsSuggestionsComponent t) { //3b 801 if (t == null) 802 return this; 803 if (this.suggestionsList == null) 804 this.suggestionsList = new ArrayList<CDSHooksResponseCardsSuggestionsComponent>(); 805 this.suggestionsList.add(t); 806 return this; 807 } 808 809 /** 810 * @return The first repetition of repeating field {@link #suggestions}, creating it if it does not already exist {3} 811 */ 812 public CDSHooksResponseCardsSuggestionsComponent getSuggestionsFirstRep() { 813 if (getSuggestionsList().isEmpty()) { 814 addSuggestions(); 815 } 816 return getSuggestionsList().get(0); 817 } 818 819 /** 820 * @return {@link #selectionBehavior} (Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 821 */ 822 public Enumeration<CDSSelectionBehaviorCodesVS> getSelectionBehaviorElement() { 823 if (this.selectionBehavior == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create CDSHooksResponseCardsComponent.selectionBehavior"); 826 else if (Configuration.doAutoCreate()) 827 this.selectionBehavior = new Enumeration<CDSSelectionBehaviorCodesVS>(new CDSSelectionBehaviorCodesVSEnumFactory()); // bb 828 return this.selectionBehavior; 829 } 830 831 public boolean hasSelectionBehaviorElement() { 832 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 833 } 834 835 public boolean hasSelectionBehavior() { 836 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #selectionBehavior} (Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 841 */ 842 public CDSHooksResponseCardsComponent setSelectionBehaviorElement(Enumeration<CDSSelectionBehaviorCodesVS> value) { 843 this.selectionBehavior = value; 844 return this; 845 } 846 847 /** 848 * @return Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them 849 */ 850 public CDSSelectionBehaviorCodesVS getSelectionBehavior() { 851 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 852 } 853 854 /** 855 * @param value Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them 856 */ 857 public CDSHooksResponseCardsComponent setSelectionBehavior(CDSSelectionBehaviorCodesVS value) { 858 if (value == null) 859 this.selectionBehavior = null; 860 else { 861 if (this.selectionBehavior == null) 862 this.selectionBehavior = new Enumeration<CDSSelectionBehaviorCodesVS>(new CDSSelectionBehaviorCodesVSEnumFactory()); 863 this.selectionBehavior.setValue(value); 864 } 865 return this; 866 } 867 868 /** 869 * @return {@link #overrideReasons} (Override reasons can be selected by the end user when overriding a card without taking the suggested recommendations. The CDS service MAY return a list of override reasons to the CDS client.) 870 */ 871 public List<Coding> getOverrideReasonsList() { 872 if (this.overrideReasonsList == null) 873 this.overrideReasonsList = new ArrayList<Coding>(); 874 return this.overrideReasonsList; 875 } 876 877 /** 878 * @return Returns a reference to <code>this</code> for easy method chaining 879 */ 880 public CDSHooksResponseCardsComponent setOverrideReasonsList(List<Coding> theOverrideReasons) { 881 this.overrideReasonsList = theOverrideReasons; 882 return this; 883 } 884 885 public boolean hasOverrideReasons() { 886 if (this.overrideReasonsList == null) 887 return false; 888 for (Coding item : this.overrideReasonsList) 889 if (!item.isEmpty()) 890 return true; 891 return false; 892 } 893 894 public Coding addOverrideReasons() { //3a 895 Coding t = new Coding(); 896 if (this.overrideReasonsList == null) 897 this.overrideReasonsList = new ArrayList<Coding>(); 898 this.overrideReasonsList.add(t); 899 return t; 900 } 901 902 public CDSHooksResponseCardsComponent addOverrideReasons(Coding t) { //3b 903 if (t == null) 904 return this; 905 if (this.overrideReasonsList == null) 906 this.overrideReasonsList = new ArrayList<Coding>(); 907 this.overrideReasonsList.add(t); 908 return this; 909 } 910 911 /** 912 * @return The first repetition of repeating field {@link #overrideReasons}, creating it if it does not already exist {3} 913 */ 914 public Coding getOverrideReasonsFirstRep() { 915 if (getOverrideReasonsList().isEmpty()) { 916 addOverrideReasons(); 917 } 918 return getOverrideReasonsList().get(0); 919 } 920 921 /** 922 * @return {@link #links} (Allows a service to suggest a link to an app that the user might want to run for additional information or to help guide a decision.) 923 */ 924 public List<CDSHooksResponseCardsLinksComponent> getLinksList() { 925 if (this.linksList == null) 926 this.linksList = new ArrayList<CDSHooksResponseCardsLinksComponent>(); 927 return this.linksList; 928 } 929 930 /** 931 * @return Returns a reference to <code>this</code> for easy method chaining 932 */ 933 public CDSHooksResponseCardsComponent setLinksList(List<CDSHooksResponseCardsLinksComponent> theLinks) { 934 this.linksList = theLinks; 935 return this; 936 } 937 938 public boolean hasLinks() { 939 if (this.linksList == null) 940 return false; 941 for (CDSHooksResponseCardsLinksComponent item : this.linksList) 942 if (!item.isEmpty()) 943 return true; 944 return false; 945 } 946 947 public CDSHooksResponseCardsLinksComponent addLinks() { //3a 948 CDSHooksResponseCardsLinksComponent t = new CDSHooksResponseCardsLinksComponent(); 949 if (this.linksList == null) 950 this.linksList = new ArrayList<CDSHooksResponseCardsLinksComponent>(); 951 this.linksList.add(t); 952 return t; 953 } 954 955 public CDSHooksResponseCardsComponent addLinks(CDSHooksResponseCardsLinksComponent t) { //3b 956 if (t == null) 957 return this; 958 if (this.linksList == null) 959 this.linksList = new ArrayList<CDSHooksResponseCardsLinksComponent>(); 960 this.linksList.add(t); 961 return this; 962 } 963 964 /** 965 * @return The first repetition of repeating field {@link #links}, creating it if it does not already exist {3} 966 */ 967 public CDSHooksResponseCardsLinksComponent getLinksFirstRep() { 968 if (getLinksList().isEmpty()) { 969 addLinks(); 970 } 971 return getLinksList().get(0); 972 } 973 974 protected void listChildren(List<Property> children) { 975 super.listChildren(children); 976 children.add(new Property("uuid", "uuid", "Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint.", 0, 1, uuid)); 977 children.add(new Property("summary", "string", "One-sentence, <140-character summary message for display to the user inside of this card.", 0, 1, summary)); 978 children.add(new Property("detail", "markdown", "Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...').", 0, 1, detail)); 979 children.add(new Property("indicator", "code", "Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical.", 0, 1, indicator)); 980 children.add(new Property("source", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "The source should be the primary source of guidance for the decision support the card represents.", 0, 1, source)); 981 children.add(new Property("suggestions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Allows a service to suggest a set of changes in the context of the current activity (e.g. changing the dose of a medication currently being prescribed, for the order-sign activity).", 0, java.lang.Integer.MAX_VALUE, suggestionsList)); 982 children.add(new Property("selectionBehavior", "code", "Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them", 0, 1, selectionBehavior)); 983 children.add(new Property("overrideReasons", "Coding", "Override reasons can be selected by the end user when overriding a card without taking the suggested recommendations. The CDS service MAY return a list of override reasons to the CDS client.", 0, java.lang.Integer.MAX_VALUE, overrideReasonsList)); 984 children.add(new Property("links", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Allows a service to suggest a link to an app that the user might want to run for additional information or to help guide a decision.", 0, java.lang.Integer.MAX_VALUE, linksList)); 985 } 986 987 @Override 988 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 989 switch (_hash) { 990 case 3601339: /*uuid*/ return new Property("uuid", "uuid", "Unique identifier of the card. MAY be used for auditing and logging cards and SHALL be included in any subsequent calls to the CDS service's feedback endpoint.", 0, 1, uuid); 991 case -1857640538: /*summary*/ return new Property("summary", "string", "One-sentence, <140-character summary message for display to the user inside of this card.", 0, 1, summary); 992 case -1335224239: /*detail*/ return new Property("detail", "markdown", "Optional detailed information to display; if provided MUST be represented in (GitHub Flavored) Markdown. (For non-urgent cards, the CDS Client MAY hide these details until the user clicks a link like 'view more details...').", 0, 1, detail); 993 case -711999985: /*indicator*/ return new Property("indicator", "code", "Urgency/importance of what this card conveys. Allowed values, in order of increasing urgency, are: info, warning, critical.", 0, 1, indicator); 994 case -896505829: /*source*/ return new Property("source", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "The source should be the primary source of guidance for the decision support the card represents.", 0, 1, source); 995 case -1525319953: /*suggestions*/ return new Property("suggestions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Allows a service to suggest a set of changes in the context of the current activity (e.g. changing the dose of a medication currently being prescribed, for the order-sign activity).", 0, java.lang.Integer.MAX_VALUE, suggestionsList); 996 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Describes the intended selection behavior of the suggestions in the card. Allowed values are: at-most-one, indicating that the user may choose none or at most one of the suggestions; any, indicating that the end user may choose any number of suggestions including none of them and all of them", 0, 1, selectionBehavior); 997 case -1554410173: /*overrideReasons*/ return new Property("overrideReasons", "Coding", "Override reasons can be selected by the end user when overriding a card without taking the suggested recommendations. The CDS service MAY return a list of override reasons to the CDS client.", 0, java.lang.Integer.MAX_VALUE, overrideReasonsList); 998 case 102977465: /*links*/ return new Property("links", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Allows a service to suggest a link to an app that the user might want to run for additional information or to help guide a decision.", 0, java.lang.Integer.MAX_VALUE, linksList); 999 default: return super.getNamedProperty(_hash, _name, _checkValid); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1006 switch (hash) { 1007 case 3601339: /*uuid*/ return this.uuid == null ? new Base[0] : new Base[] {this.uuid}; // UuidType 1008 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // StringType 1009 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // MarkdownType 1010 case -711999985: /*indicator*/ return this.indicator == null ? new Base[0] : new Base[] {this.indicator}; // Enumeration<CDSIndicatorCodesVS> 1011 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // CDSHooksResponseCardsSourceComponent 1012 case -1525319953: /*suggestions*/ return this.suggestionsList == null ? new Base[0] : this.suggestionsList.toArray(new Base[this.suggestionsList.size()]); // CDSHooksResponseCardsSuggestionsComponent 1013 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<CDSSelectionBehaviorCodesVS> 1014 case -1554410173: /*overrideReasons*/ return this.overrideReasonsList == null ? new Base[0] : this.overrideReasonsList.toArray(new Base[this.overrideReasonsList.size()]); // Coding 1015 case 102977465: /*links*/ return this.linksList == null ? new Base[0] : this.linksList.toArray(new Base[this.linksList.size()]); // CDSHooksResponseCardsLinksComponent 1016 default: return super.getProperty(hash, name, checkValid); 1017 } 1018 1019 } 1020 1021 @Override 1022 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1023 switch (hash) { 1024 case 3601339: // uuid 1025 this.uuid = TypeConvertor.castToUuid(value); // UuidType 1026 return value; 1027 case -1857640538: // summary 1028 this.summary = TypeConvertor.castToString(value); // StringType 1029 return value; 1030 case -1335224239: // detail 1031 this.detail = TypeConvertor.castToMarkdown(value); // MarkdownType 1032 return value; 1033 case -711999985: // indicator 1034 value = new CDSIndicatorCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 1035 this.indicator = (Enumeration) value; // Enumeration<CDSIndicatorCodesVS> 1036 return value; 1037 case -896505829: // source 1038 this.source = (CDSHooksResponseCardsSourceComponent) value; // CDSHooksResponseCardsSourceComponent 1039 return value; 1040 case -1525319953: // suggestions 1041 this.getSuggestionsList().add((CDSHooksResponseCardsSuggestionsComponent) value); // CDSHooksResponseCardsSuggestionsComponent 1042 return value; 1043 case 168639486: // selectionBehavior 1044 value = new CDSSelectionBehaviorCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 1045 this.selectionBehavior = (Enumeration) value; // Enumeration<CDSSelectionBehaviorCodesVS> 1046 return value; 1047 case -1554410173: // overrideReasons 1048 this.getOverrideReasonsList().add(TypeConvertor.castToCoding(value)); // Coding 1049 return value; 1050 case 102977465: // links 1051 this.getLinksList().add((CDSHooksResponseCardsLinksComponent) value); // CDSHooksResponseCardsLinksComponent 1052 return value; 1053 default: return super.setProperty(hash, name, value); 1054 } 1055 1056 } 1057 1058 @Override 1059 public Base setProperty(String name, Base value) throws FHIRException { 1060 if (name.equals("uuid")) { 1061 this.uuid = TypeConvertor.castToUuid(value); // UuidType 1062 } else if (name.equals("summary")) { 1063 this.summary = TypeConvertor.castToString(value); // StringType 1064 } else if (name.equals("detail")) { 1065 this.detail = TypeConvertor.castToMarkdown(value); // MarkdownType 1066 } else if (name.equals("indicator")) { 1067 value = new CDSIndicatorCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 1068 this.indicator = (Enumeration) value; // Enumeration<CDSIndicatorCodesVS> 1069 } else if (name.equals("source")) { 1070 this.source = (CDSHooksResponseCardsSourceComponent) value; // CDSHooksResponseCardsSourceComponent 1071 } else if (name.equals("suggestions")) { 1072 this.getSuggestionsList().add((CDSHooksResponseCardsSuggestionsComponent) value); // CDSHooksResponseCardsSuggestionsComponent 1073 } else if (name.equals("selectionBehavior")) { 1074 value = new CDSSelectionBehaviorCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 1075 this.selectionBehavior = (Enumeration) value; // Enumeration<CDSSelectionBehaviorCodesVS> 1076 } else if (name.equals("overrideReasons")) { 1077 this.getOverrideReasonsList().add(TypeConvertor.castToCoding(value)); // Coding 1078 } else if (name.equals("links")) { 1079 this.getLinksList().add((CDSHooksResponseCardsLinksComponent) value); // CDSHooksResponseCardsLinksComponent 1080 } else 1081 return super.setProperty(name, value); 1082 return value; 1083 } 1084 1085 @Override 1086 public Base makeProperty(int hash, String name) throws FHIRException { 1087 switch (hash) { 1088 case 3601339: return getUuidElement(); 1089 case -1857640538: return getSummaryElement(); 1090 case -1335224239: return getDetailElement(); 1091 case -711999985: return getIndicatorElement(); 1092 case -896505829: return getSource(); 1093 case -1525319953: return addSuggestions(); 1094 case 168639486: return getSelectionBehaviorElement(); 1095 case -1554410173: return addOverrideReasons(); 1096 case 102977465: return addLinks(); 1097 default: return super.makeProperty(hash, name); 1098 } 1099 1100 } 1101 1102 @Override 1103 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1104 switch (hash) { 1105 case 3601339: /*uuid*/ return new String[] {"uuid"}; 1106 case -1857640538: /*summary*/ return new String[] {"string"}; 1107 case -1335224239: /*detail*/ return new String[] {"markdown"}; 1108 case -711999985: /*indicator*/ return new String[] {"code"}; 1109 case -896505829: /*source*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 1110 case -1525319953: /*suggestions*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 1111 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 1112 case -1554410173: /*overrideReasons*/ return new String[] {"Coding"}; 1113 case 102977465: /*links*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 1114 default: return super.getTypesForProperty(hash, name); 1115 } 1116 1117 } 1118 1119 @Override 1120 public Base addChild(String name) throws FHIRException { 1121 if (name.equals("uuid")) { 1122 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.uuid"); 1123 } 1124 else if (name.equals("summary")) { 1125 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.summary"); 1126 } 1127 else if (name.equals("detail")) { 1128 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.detail"); 1129 } 1130 else if (name.equals("indicator")) { 1131 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.indicator"); 1132 } 1133 else if (name.equals("source")) { 1134 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards.source"); 1135 } 1136 else if (name.equals("suggestions")) { 1137 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards.suggestions"); 1138 } 1139 else if (name.equals("selectionBehavior")) { 1140 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.selectionBehavior"); 1141 } 1142 else if (name.equals("overrideReasons")) { 1143 return addOverrideReasons(); 1144 } 1145 else if (name.equals("links")) { 1146 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards.links"); 1147 } 1148 else 1149 return super.addChild(name); 1150 } 1151 1152 public CDSHooksResponseCardsComponent copy() { 1153 CDSHooksResponseCardsComponent dst = new CDSHooksResponseCardsComponent(); 1154 copyValues(dst); 1155 return dst; 1156 } 1157 1158 public void copyValues(CDSHooksResponseCardsComponent dst) { 1159 super.copyValues(dst); 1160 dst.uuid = uuid == null ? null : uuid.copy(); 1161 dst.summary = summary == null ? null : summary.copy(); 1162 dst.detail = detail == null ? null : detail.copy(); 1163 dst.indicator = indicator == null ? null : indicator.copy(); 1164 dst.source = source == null ? null : source.copy(); 1165 if (suggestionsList != null) { 1166 dst.suggestionsList = new ArrayList<CDSHooksResponseCardsSuggestionsComponent>(); 1167 for (CDSHooksResponseCardsSuggestionsComponent i : suggestionsList) 1168 dst.suggestionsList.add(i.copy()); 1169 }; 1170 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 1171 if (overrideReasonsList != null) { 1172 dst.overrideReasonsList = new ArrayList<Coding>(); 1173 for (Coding i : overrideReasonsList) 1174 dst.overrideReasonsList.add(i.copy()); 1175 }; 1176 if (linksList != null) { 1177 dst.linksList = new ArrayList<CDSHooksResponseCardsLinksComponent>(); 1178 for (CDSHooksResponseCardsLinksComponent i : linksList) 1179 dst.linksList.add(i.copy()); 1180 }; 1181 } 1182 1183 @Override 1184 public boolean equalsDeep(Base other_) { 1185 if (!super.equalsDeep(other_)) 1186 return false; 1187 if (!(other_ instanceof CDSHooksResponseCardsComponent)) 1188 return false; 1189 CDSHooksResponseCardsComponent o = (CDSHooksResponseCardsComponent) other_; 1190 return compareDeep(uuid, o.uuid, true) && compareDeep(summary, o.summary, true) && compareDeep(detail, o.detail, true) 1191 && compareDeep(indicator, o.indicator, true) && compareDeep(source, o.source, true) && compareDeep(suggestionsList, o.suggestionsList, true) 1192 && compareDeep(selectionBehavior, o.selectionBehavior, true) && compareDeep(overrideReasonsList, o.overrideReasonsList, true) 1193 && compareDeep(linksList, o.linksList, true); 1194 } 1195 1196 @Override 1197 public boolean equalsShallow(Base other_) { 1198 if (!super.equalsShallow(other_)) 1199 return false; 1200 if (!(other_ instanceof CDSHooksResponseCardsComponent)) 1201 return false; 1202 CDSHooksResponseCardsComponent o = (CDSHooksResponseCardsComponent) other_; 1203 return compareValues(uuid, o.uuid, true) && compareValues(summary, o.summary, true) && compareValues(detail, o.detail, true) 1204 && compareValues(indicator, o.indicator, true) && compareValues(selectionBehavior, o.selectionBehavior, true) 1205 ; 1206 } 1207 1208 public boolean isEmpty() { 1209 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uuid, summary, detail, indicator 1210 , source, suggestionsList, selectionBehavior, overrideReasonsList, linksList); 1211 } 1212 1213 public String fhirType() { 1214 return "CDSHooksResponse.cards"; 1215 1216 } 1217 1218 } 1219 1220 @Block() 1221 public static class CDSHooksResponseCardsSourceComponent extends CDSHooksElement { 1222 /** 1223 * A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink. 1224 */ 1225 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1226 @Description(shortDefinition="Short, human-readable label to display for the source.", formalDefinition="A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink." ) 1227 protected StringType label; 1228 1229 /** 1230 * An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card. 1231 */ 1232 @Child(name = "url", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1233 @Description(shortDefinition="Link for when user clicks for more information about the source", formalDefinition="An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card." ) 1234 protected UrlType url; 1235 1236 /** 1237 * An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience. 1238 */ 1239 @Child(name = "icon", type = {UrlType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1240 @Description(shortDefinition="absolute URL to an icon for the source (<100x100 PNG))", formalDefinition="An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience." ) 1241 protected UrlType icon; 1242 1243 /** 1244 * A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics 1245 */ 1246 @Child(name = "topic", type = {Coding.class}, order=4, min=0, max=1, modifier=false, summary=false) 1247 @Description(shortDefinition="Describes the content of the card - can be useful for filtering, searching or ordered display", formalDefinition="A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics" ) 1248 protected Coding topic; 1249 1250 private static final long serialVersionUID = -271286704L; 1251 1252 /** 1253 * Constructor 1254 */ 1255 public CDSHooksResponseCardsSourceComponent() { 1256 super(); 1257 } 1258 1259 /** 1260 * Constructor 1261 */ 1262 public CDSHooksResponseCardsSourceComponent(String label) { 1263 super(); 1264 this.setLabel(label); 1265 } 1266 1267 /** 1268 * @return {@link #label} (A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1269 */ 1270 public StringType getLabelElement() { 1271 if (this.label == null) 1272 if (Configuration.errorOnAutoCreate()) 1273 throw new Error("Attempt to auto-create CDSHooksResponseCardsSourceComponent.label"); 1274 else if (Configuration.doAutoCreate()) 1275 this.label = new StringType(); // bb 1276 return this.label; 1277 } 1278 1279 public boolean hasLabelElement() { 1280 return this.label != null && !this.label.isEmpty(); 1281 } 1282 1283 public boolean hasLabel() { 1284 return this.label != null && !this.label.isEmpty(); 1285 } 1286 1287 /** 1288 * @param value {@link #label} (A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1289 */ 1290 public CDSHooksResponseCardsSourceComponent setLabelElement(StringType value) { 1291 this.label = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink. 1297 */ 1298 public String getLabel() { 1299 return this.label == null ? null : this.label.getValue(); 1300 } 1301 1302 /** 1303 * @param value A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink. 1304 */ 1305 public CDSHooksResponseCardsSourceComponent setLabel(String value) { 1306 if (this.label == null) 1307 this.label = new StringType(); 1308 this.label.setValue(value); 1309 return this; 1310 } 1311 1312 /** 1313 * @return {@link #url} (An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1314 */ 1315 public UrlType getUrlElement() { 1316 if (this.url == null) 1317 if (Configuration.errorOnAutoCreate()) 1318 throw new Error("Attempt to auto-create CDSHooksResponseCardsSourceComponent.url"); 1319 else if (Configuration.doAutoCreate()) 1320 this.url = new UrlType(); // bb 1321 return this.url; 1322 } 1323 1324 public boolean hasUrlElement() { 1325 return this.url != null && !this.url.isEmpty(); 1326 } 1327 1328 public boolean hasUrl() { 1329 return this.url != null && !this.url.isEmpty(); 1330 } 1331 1332 /** 1333 * @param value {@link #url} (An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1334 */ 1335 public CDSHooksResponseCardsSourceComponent setUrlElement(UrlType value) { 1336 this.url = value; 1337 return this; 1338 } 1339 1340 /** 1341 * @return An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card. 1342 */ 1343 public String getUrl() { 1344 return this.url == null ? null : this.url.getValue(); 1345 } 1346 1347 /** 1348 * @param value An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card. 1349 */ 1350 public CDSHooksResponseCardsSourceComponent setUrl(String value) { 1351 if (Utilities.noString(value)) 1352 this.url = null; 1353 else { 1354 if (this.url == null) 1355 this.url = new UrlType(); 1356 this.url.setValue(value); 1357 } 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #icon} (An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience.). This is the underlying object with id, value and extensions. The accessor "getIcon" gives direct access to the value 1363 */ 1364 public UrlType getIconElement() { 1365 if (this.icon == null) 1366 if (Configuration.errorOnAutoCreate()) 1367 throw new Error("Attempt to auto-create CDSHooksResponseCardsSourceComponent.icon"); 1368 else if (Configuration.doAutoCreate()) 1369 this.icon = new UrlType(); // bb 1370 return this.icon; 1371 } 1372 1373 public boolean hasIconElement() { 1374 return this.icon != null && !this.icon.isEmpty(); 1375 } 1376 1377 public boolean hasIcon() { 1378 return this.icon != null && !this.icon.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #icon} (An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience.). This is the underlying object with id, value and extensions. The accessor "getIcon" gives direct access to the value 1383 */ 1384 public CDSHooksResponseCardsSourceComponent setIconElement(UrlType value) { 1385 this.icon = value; 1386 return this; 1387 } 1388 1389 /** 1390 * @return An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience. 1391 */ 1392 public String getIcon() { 1393 return this.icon == null ? null : this.icon.getValue(); 1394 } 1395 1396 /** 1397 * @param value An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience. 1398 */ 1399 public CDSHooksResponseCardsSourceComponent setIcon(String value) { 1400 if (Utilities.noString(value)) 1401 this.icon = null; 1402 else { 1403 if (this.icon == null) 1404 this.icon = new UrlType(); 1405 this.icon.setValue(value); 1406 } 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #topic} (A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics) 1412 */ 1413 public Coding getTopic() { 1414 if (this.topic == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create CDSHooksResponseCardsSourceComponent.topic"); 1417 else if (Configuration.doAutoCreate()) 1418 this.topic = new Coding(); // cc 1419 return this.topic; 1420 } 1421 1422 public boolean hasTopic() { 1423 return this.topic != null && !this.topic.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #topic} (A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics) 1428 */ 1429 public CDSHooksResponseCardsSourceComponent setTopic(Coding value) { 1430 this.topic = value; 1431 return this; 1432 } 1433 1434 protected void listChildren(List<Property> children) { 1435 super.listChildren(children); 1436 children.add(new Property("label", "string", "A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink.", 0, 1, label)); 1437 children.add(new Property("url", "url", "An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card.", 0, 1, url)); 1438 children.add(new Property("icon", "url", "An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience.", 0, 1, icon)); 1439 children.add(new Property("topic", "Coding", "A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics", 0, 1, topic)); 1440 } 1441 1442 @Override 1443 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1444 switch (_hash) { 1445 case 102727412: /*label*/ return new Property("label", "string", "A short, human-readable label to display for the source of the information displayed on this card. If a url is also specified, this MAY be the text for the hyperlink.", 0, 1, label); 1446 case 116079: /*url*/ return new Property("url", "url", "An optional absolute URL to load (via GET, in a browser context) when a user clicks on this link to learn more about the organization or data set that provided the information on this card.", 0, 1, url); 1447 case 3226745: /*icon*/ return new Property("icon", "url", "An absolute URL to an icon for the source of this card. The icon returned by this URL SHOULD be a 100x100 pixel PNG image without any transparent regions. The CDS Client may ignore or scale the image during display as appropriate for user experience.", 0, 1, icon); 1448 case 110546223: /*topic*/ return new Property("topic", "Coding", "A topic describes the content of the card by providing a high-level categorization that can be useful for filtering, searching or ordered display of related cards in the CDS client's UI. This specification does not prescribe a standard set of topics", 0, 1, topic); 1449 default: return super.getNamedProperty(_hash, _name, _checkValid); 1450 } 1451 1452 } 1453 1454 @Override 1455 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1456 switch (hash) { 1457 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 1458 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 1459 case 3226745: /*icon*/ return this.icon == null ? new Base[0] : new Base[] {this.icon}; // UrlType 1460 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // Coding 1461 default: return super.getProperty(hash, name, checkValid); 1462 } 1463 1464 } 1465 1466 @Override 1467 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1468 switch (hash) { 1469 case 102727412: // label 1470 this.label = TypeConvertor.castToString(value); // StringType 1471 return value; 1472 case 116079: // url 1473 this.url = TypeConvertor.castToUrl(value); // UrlType 1474 return value; 1475 case 3226745: // icon 1476 this.icon = TypeConvertor.castToUrl(value); // UrlType 1477 return value; 1478 case 110546223: // topic 1479 this.topic = TypeConvertor.castToCoding(value); // Coding 1480 return value; 1481 default: return super.setProperty(hash, name, value); 1482 } 1483 1484 } 1485 1486 @Override 1487 public Base setProperty(String name, Base value) throws FHIRException { 1488 if (name.equals("label")) { 1489 this.label = TypeConvertor.castToString(value); // StringType 1490 } else if (name.equals("url")) { 1491 this.url = TypeConvertor.castToUrl(value); // UrlType 1492 } else if (name.equals("icon")) { 1493 this.icon = TypeConvertor.castToUrl(value); // UrlType 1494 } else if (name.equals("topic")) { 1495 this.topic = TypeConvertor.castToCoding(value); // Coding 1496 } else 1497 return super.setProperty(name, value); 1498 return value; 1499 } 1500 1501 @Override 1502 public Base makeProperty(int hash, String name) throws FHIRException { 1503 switch (hash) { 1504 case 102727412: return getLabelElement(); 1505 case 116079: return getUrlElement(); 1506 case 3226745: return getIconElement(); 1507 case 110546223: return getTopic(); 1508 default: return super.makeProperty(hash, name); 1509 } 1510 1511 } 1512 1513 @Override 1514 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1515 switch (hash) { 1516 case 102727412: /*label*/ return new String[] {"string"}; 1517 case 116079: /*url*/ return new String[] {"url"}; 1518 case 3226745: /*icon*/ return new String[] {"url"}; 1519 case 110546223: /*topic*/ return new String[] {"Coding"}; 1520 default: return super.getTypesForProperty(hash, name); 1521 } 1522 1523 } 1524 1525 @Override 1526 public Base addChild(String name) throws FHIRException { 1527 if (name.equals("label")) { 1528 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.source.label"); 1529 } 1530 else if (name.equals("url")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.source.url"); 1532 } 1533 else if (name.equals("icon")) { 1534 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.source.icon"); 1535 } 1536 else if (name.equals("topic")) { 1537 this.topic = new Coding(); 1538 return this.topic; 1539 } 1540 else 1541 return super.addChild(name); 1542 } 1543 1544 public CDSHooksResponseCardsSourceComponent copy() { 1545 CDSHooksResponseCardsSourceComponent dst = new CDSHooksResponseCardsSourceComponent(); 1546 copyValues(dst); 1547 return dst; 1548 } 1549 1550 public void copyValues(CDSHooksResponseCardsSourceComponent dst) { 1551 super.copyValues(dst); 1552 dst.label = label == null ? null : label.copy(); 1553 dst.url = url == null ? null : url.copy(); 1554 dst.icon = icon == null ? null : icon.copy(); 1555 dst.topic = topic == null ? null : topic.copy(); 1556 } 1557 1558 @Override 1559 public boolean equalsDeep(Base other_) { 1560 if (!super.equalsDeep(other_)) 1561 return false; 1562 if (!(other_ instanceof CDSHooksResponseCardsSourceComponent)) 1563 return false; 1564 CDSHooksResponseCardsSourceComponent o = (CDSHooksResponseCardsSourceComponent) other_; 1565 return compareDeep(label, o.label, true) && compareDeep(url, o.url, true) && compareDeep(icon, o.icon, true) 1566 && compareDeep(topic, o.topic, true); 1567 } 1568 1569 @Override 1570 public boolean equalsShallow(Base other_) { 1571 if (!super.equalsShallow(other_)) 1572 return false; 1573 if (!(other_ instanceof CDSHooksResponseCardsSourceComponent)) 1574 return false; 1575 CDSHooksResponseCardsSourceComponent o = (CDSHooksResponseCardsSourceComponent) other_; 1576 return compareValues(label, o.label, true) && compareValues(url, o.url, true) && compareValues(icon, o.icon, true) 1577 ; 1578 } 1579 1580 public boolean isEmpty() { 1581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, url, icon, topic 1582 ); 1583 } 1584 1585 public String fhirType() { 1586 return "CDSHooksResponse.cards.source"; 1587 1588 } 1589 1590 } 1591 1592 @Block() 1593 public static class CDSHooksResponseCardsSuggestionsComponent extends CDSHooksElement { 1594 /** 1595 * Human-readable label to display for this suggestion 1596 */ 1597 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1598 @Description(shortDefinition="Human-readable label to display for this suggestion", formalDefinition="Human-readable label to display for this suggestion" ) 1599 protected StringType label; 1600 1601 /** 1602 * Unique identifier, used for auditing and logging suggestions 1603 */ 1604 @Child(name = "uuid", type = {UuidType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1605 @Description(shortDefinition="Unique identifier, used for auditing and logging suggestions", formalDefinition="Unique identifier, used for auditing and logging suggestions" ) 1606 protected UuidType uuid; 1607 1608 /** 1609 * When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card 1610 */ 1611 @Child(name = "isRecommended", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1612 @Description(shortDefinition="", formalDefinition="When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card" ) 1613 protected BooleanType isRecommended; 1614 1615 /** 1616 * Defines a suggested action. Within a suggestion, all actions are logically AND'd together, such that a user selecting a suggestion selects all of the actions within it 1617 */ 1618 @Child(name = "actions", type = {CDSHooksElement.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1619 @Description(shortDefinition="Defines a suggested action (all apply)", formalDefinition="Defines a suggested action. Within a suggestion, all actions are logically AND'd together, such that a user selecting a suggestion selects all of the actions within it" ) 1620 protected List<CDSHooksResponseCardsSuggestionsActionsComponent> actionsList; 1621 1622 private static final long serialVersionUID = 89077716L; 1623 1624 /** 1625 * Constructor 1626 */ 1627 public CDSHooksResponseCardsSuggestionsComponent() { 1628 super(); 1629 } 1630 1631 /** 1632 * Constructor 1633 */ 1634 public CDSHooksResponseCardsSuggestionsComponent(String label) { 1635 super(); 1636 this.setLabel(label); 1637 } 1638 1639 /** 1640 * @return {@link #label} (Human-readable label to display for this suggestion). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1641 */ 1642 public StringType getLabelElement() { 1643 if (this.label == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsComponent.label"); 1646 else if (Configuration.doAutoCreate()) 1647 this.label = new StringType(); // bb 1648 return this.label; 1649 } 1650 1651 public boolean hasLabelElement() { 1652 return this.label != null && !this.label.isEmpty(); 1653 } 1654 1655 public boolean hasLabel() { 1656 return this.label != null && !this.label.isEmpty(); 1657 } 1658 1659 /** 1660 * @param value {@link #label} (Human-readable label to display for this suggestion). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1661 */ 1662 public CDSHooksResponseCardsSuggestionsComponent setLabelElement(StringType value) { 1663 this.label = value; 1664 return this; 1665 } 1666 1667 /** 1668 * @return Human-readable label to display for this suggestion 1669 */ 1670 public String getLabel() { 1671 return this.label == null ? null : this.label.getValue(); 1672 } 1673 1674 /** 1675 * @param value Human-readable label to display for this suggestion 1676 */ 1677 public CDSHooksResponseCardsSuggestionsComponent setLabel(String value) { 1678 if (this.label == null) 1679 this.label = new StringType(); 1680 this.label.setValue(value); 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #uuid} (Unique identifier, used for auditing and logging suggestions). This is the underlying object with id, value and extensions. The accessor "getUuid" gives direct access to the value 1686 */ 1687 public UuidType getUuidElement() { 1688 if (this.uuid == null) 1689 if (Configuration.errorOnAutoCreate()) 1690 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsComponent.uuid"); 1691 else if (Configuration.doAutoCreate()) 1692 this.uuid = new UuidType(); // bb 1693 return this.uuid; 1694 } 1695 1696 public boolean hasUuidElement() { 1697 return this.uuid != null && !this.uuid.isEmpty(); 1698 } 1699 1700 public boolean hasUuid() { 1701 return this.uuid != null && !this.uuid.isEmpty(); 1702 } 1703 1704 /** 1705 * @param value {@link #uuid} (Unique identifier, used for auditing and logging suggestions). This is the underlying object with id, value and extensions. The accessor "getUuid" gives direct access to the value 1706 */ 1707 public CDSHooksResponseCardsSuggestionsComponent setUuidElement(UuidType value) { 1708 this.uuid = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return Unique identifier, used for auditing and logging suggestions 1714 */ 1715 public String getUuid() { 1716 return this.uuid == null ? null : this.uuid.getValue(); 1717 } 1718 1719 /** 1720 * @param value Unique identifier, used for auditing and logging suggestions 1721 */ 1722 public CDSHooksResponseCardsSuggestionsComponent setUuid(String value) { 1723 if (Utilities.noString(value)) 1724 this.uuid = null; 1725 else { 1726 if (this.uuid == null) 1727 this.uuid = new UuidType(); 1728 this.uuid.setValue(value); 1729 } 1730 return this; 1731 } 1732 1733 /** 1734 * @return {@link #isRecommended} (When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card). This is the underlying object with id, value and extensions. The accessor "getIsRecommended" gives direct access to the value 1735 */ 1736 public BooleanType getIsRecommendedElement() { 1737 if (this.isRecommended == null) 1738 if (Configuration.errorOnAutoCreate()) 1739 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsComponent.isRecommended"); 1740 else if (Configuration.doAutoCreate()) 1741 this.isRecommended = new BooleanType(); // bb 1742 return this.isRecommended; 1743 } 1744 1745 public boolean hasIsRecommendedElement() { 1746 return this.isRecommended != null && !this.isRecommended.isEmpty(); 1747 } 1748 1749 public boolean hasIsRecommended() { 1750 return this.isRecommended != null && !this.isRecommended.isEmpty(); 1751 } 1752 1753 /** 1754 * @param value {@link #isRecommended} (When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card). This is the underlying object with id, value and extensions. The accessor "getIsRecommended" gives direct access to the value 1755 */ 1756 public CDSHooksResponseCardsSuggestionsComponent setIsRecommendedElement(BooleanType value) { 1757 this.isRecommended = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card 1763 */ 1764 public boolean getIsRecommended() { 1765 return this.isRecommended == null || this.isRecommended.isEmpty() ? false : this.isRecommended.getValue(); 1766 } 1767 1768 /** 1769 * @param value When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card 1770 */ 1771 public CDSHooksResponseCardsSuggestionsComponent setIsRecommended(boolean value) { 1772 if (this.isRecommended == null) 1773 this.isRecommended = new BooleanType(); 1774 this.isRecommended.setValue(value); 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #actions} (Defines a suggested action. Within a suggestion, all actions are logically AND'd together, such that a user selecting a suggestion selects all of the actions within it) 1780 */ 1781 public List<CDSHooksResponseCardsSuggestionsActionsComponent> getActionsList() { 1782 if (this.actionsList == null) 1783 this.actionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 1784 return this.actionsList; 1785 } 1786 1787 /** 1788 * @return Returns a reference to <code>this</code> for easy method chaining 1789 */ 1790 public CDSHooksResponseCardsSuggestionsComponent setActionsList(List<CDSHooksResponseCardsSuggestionsActionsComponent> theActions) { 1791 this.actionsList = theActions; 1792 return this; 1793 } 1794 1795 public boolean hasActions() { 1796 if (this.actionsList == null) 1797 return false; 1798 for (CDSHooksResponseCardsSuggestionsActionsComponent item : this.actionsList) 1799 if (!item.isEmpty()) 1800 return true; 1801 return false; 1802 } 1803 1804 public CDSHooksResponseCardsSuggestionsActionsComponent addActions() { //3a 1805 CDSHooksResponseCardsSuggestionsActionsComponent t = new CDSHooksResponseCardsSuggestionsActionsComponent(); 1806 if (this.actionsList == null) 1807 this.actionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 1808 this.actionsList.add(t); 1809 return t; 1810 } 1811 1812 public CDSHooksResponseCardsSuggestionsComponent addActions(CDSHooksResponseCardsSuggestionsActionsComponent t) { //3b 1813 if (t == null) 1814 return this; 1815 if (this.actionsList == null) 1816 this.actionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 1817 this.actionsList.add(t); 1818 return this; 1819 } 1820 1821 /** 1822 * @return The first repetition of repeating field {@link #actions}, creating it if it does not already exist {3} 1823 */ 1824 public CDSHooksResponseCardsSuggestionsActionsComponent getActionsFirstRep() { 1825 if (getActionsList().isEmpty()) { 1826 addActions(); 1827 } 1828 return getActionsList().get(0); 1829 } 1830 1831 protected void listChildren(List<Property> children) { 1832 super.listChildren(children); 1833 children.add(new Property("label", "string", "Human-readable label to display for this suggestion", 0, 1, label)); 1834 children.add(new Property("uuid", "uuid", "Unique identifier, used for auditing and logging suggestions", 0, 1, uuid)); 1835 children.add(new Property("isRecommended", "boolean", "When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card", 0, 1, isRecommended)); 1836 children.add(new Property("actions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Defines a suggested action. Within a suggestion, all actions are logically AND'd together, such that a user selecting a suggestion selects all of the actions within it", 0, java.lang.Integer.MAX_VALUE, actionsList)); 1837 } 1838 1839 @Override 1840 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1841 switch (_hash) { 1842 case 102727412: /*label*/ return new Property("label", "string", "Human-readable label to display for this suggestion", 0, 1, label); 1843 case 3601339: /*uuid*/ return new Property("uuid", "uuid", "Unique identifier, used for auditing and logging suggestions", 0, 1, uuid); 1844 case 27884241: /*isRecommended*/ return new Property("isRecommended", "boolean", "When there are multiple suggestions, allows a service to indicate that a specific suggestion is recommended from all the available suggestions on the card", 0, 1, isRecommended); 1845 case -1161803523: /*actions*/ return new Property("actions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "Defines a suggested action. Within a suggestion, all actions are logically AND'd together, such that a user selecting a suggestion selects all of the actions within it", 0, java.lang.Integer.MAX_VALUE, actionsList); 1846 default: return super.getNamedProperty(_hash, _name, _checkValid); 1847 } 1848 1849 } 1850 1851 @Override 1852 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1853 switch (hash) { 1854 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 1855 case 3601339: /*uuid*/ return this.uuid == null ? new Base[0] : new Base[] {this.uuid}; // UuidType 1856 case 27884241: /*isRecommended*/ return this.isRecommended == null ? new Base[0] : new Base[] {this.isRecommended}; // BooleanType 1857 case -1161803523: /*actions*/ return this.actionsList == null ? new Base[0] : this.actionsList.toArray(new Base[this.actionsList.size()]); // CDSHooksResponseCardsSuggestionsActionsComponent 1858 default: return super.getProperty(hash, name, checkValid); 1859 } 1860 1861 } 1862 1863 @Override 1864 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1865 switch (hash) { 1866 case 102727412: // label 1867 this.label = TypeConvertor.castToString(value); // StringType 1868 return value; 1869 case 3601339: // uuid 1870 this.uuid = TypeConvertor.castToUuid(value); // UuidType 1871 return value; 1872 case 27884241: // isRecommended 1873 this.isRecommended = TypeConvertor.castToBoolean(value); // BooleanType 1874 return value; 1875 case -1161803523: // actions 1876 this.getActionsList().add((CDSHooksResponseCardsSuggestionsActionsComponent) value); // CDSHooksResponseCardsSuggestionsActionsComponent 1877 return value; 1878 default: return super.setProperty(hash, name, value); 1879 } 1880 1881 } 1882 1883 @Override 1884 public Base setProperty(String name, Base value) throws FHIRException { 1885 if (name.equals("label")) { 1886 this.label = TypeConvertor.castToString(value); // StringType 1887 } else if (name.equals("uuid")) { 1888 this.uuid = TypeConvertor.castToUuid(value); // UuidType 1889 } else if (name.equals("isRecommended")) { 1890 this.isRecommended = TypeConvertor.castToBoolean(value); // BooleanType 1891 } else if (name.equals("actions")) { 1892 this.getActionsList().add((CDSHooksResponseCardsSuggestionsActionsComponent) value); // CDSHooksResponseCardsSuggestionsActionsComponent 1893 } else 1894 return super.setProperty(name, value); 1895 return value; 1896 } 1897 1898 @Override 1899 public Base makeProperty(int hash, String name) throws FHIRException { 1900 switch (hash) { 1901 case 102727412: return getLabelElement(); 1902 case 3601339: return getUuidElement(); 1903 case 27884241: return getIsRecommendedElement(); 1904 case -1161803523: return addActions(); 1905 default: return super.makeProperty(hash, name); 1906 } 1907 1908 } 1909 1910 @Override 1911 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1912 switch (hash) { 1913 case 102727412: /*label*/ return new String[] {"string"}; 1914 case 3601339: /*uuid*/ return new String[] {"uuid"}; 1915 case 27884241: /*isRecommended*/ return new String[] {"boolean"}; 1916 case -1161803523: /*actions*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 1917 default: return super.getTypesForProperty(hash, name); 1918 } 1919 1920 } 1921 1922 @Override 1923 public Base addChild(String name) throws FHIRException { 1924 if (name.equals("label")) { 1925 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.label"); 1926 } 1927 else if (name.equals("uuid")) { 1928 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.uuid"); 1929 } 1930 else if (name.equals("isRecommended")) { 1931 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.isRecommended"); 1932 } 1933 else if (name.equals("actions")) { 1934 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards.suggestions.actions"); 1935 } 1936 else 1937 return super.addChild(name); 1938 } 1939 1940 public CDSHooksResponseCardsSuggestionsComponent copy() { 1941 CDSHooksResponseCardsSuggestionsComponent dst = new CDSHooksResponseCardsSuggestionsComponent(); 1942 copyValues(dst); 1943 return dst; 1944 } 1945 1946 public void copyValues(CDSHooksResponseCardsSuggestionsComponent dst) { 1947 super.copyValues(dst); 1948 dst.label = label == null ? null : label.copy(); 1949 dst.uuid = uuid == null ? null : uuid.copy(); 1950 dst.isRecommended = isRecommended == null ? null : isRecommended.copy(); 1951 if (actionsList != null) { 1952 dst.actionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 1953 for (CDSHooksResponseCardsSuggestionsActionsComponent i : actionsList) 1954 dst.actionsList.add(i.copy()); 1955 }; 1956 } 1957 1958 @Override 1959 public boolean equalsDeep(Base other_) { 1960 if (!super.equalsDeep(other_)) 1961 return false; 1962 if (!(other_ instanceof CDSHooksResponseCardsSuggestionsComponent)) 1963 return false; 1964 CDSHooksResponseCardsSuggestionsComponent o = (CDSHooksResponseCardsSuggestionsComponent) other_; 1965 return compareDeep(label, o.label, true) && compareDeep(uuid, o.uuid, true) && compareDeep(isRecommended, o.isRecommended, true) 1966 && compareDeep(actionsList, o.actionsList, true); 1967 } 1968 1969 @Override 1970 public boolean equalsShallow(Base other_) { 1971 if (!super.equalsShallow(other_)) 1972 return false; 1973 if (!(other_ instanceof CDSHooksResponseCardsSuggestionsComponent)) 1974 return false; 1975 CDSHooksResponseCardsSuggestionsComponent o = (CDSHooksResponseCardsSuggestionsComponent) other_; 1976 return compareValues(label, o.label, true) && compareValues(uuid, o.uuid, true) && compareValues(isRecommended, o.isRecommended, true) 1977 ; 1978 } 1979 1980 public boolean isEmpty() { 1981 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, uuid, isRecommended 1982 , actionsList); 1983 } 1984 1985 public String fhirType() { 1986 return "CDSHooksResponse.cards.suggestions"; 1987 1988 } 1989 1990 } 1991 1992 @Block() 1993 public static class CDSHooksResponseCardsSuggestionsActionsComponent extends CDSHooksElement { 1994 /** 1995 * The type of action being performed. Allowed values are: create, update, delete. 1996 */ 1997 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1998 @Description(shortDefinition="create, update, delete - type of action performed", formalDefinition="The type of action being performed. Allowed values are: create, update, delete." ) 1999 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/tools/ValueSet/CDSActionType") 2000 protected Enumeration<CDSActionTypeCodesVS> type; 2001 2002 /** 2003 * Human-readable description of the suggested action that MAY be presented to the end-user. 2004 */ 2005 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2006 @Description(shortDefinition="Human-readable description of the suggested action (MAY be presented to the end-user)", formalDefinition="Human-readable description of the suggested action that MAY be presented to the end-user." ) 2007 protected StringType description; 2008 2009 /** 2010 * When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields. 2011 */ 2012 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=false) 2013 @Description(shortDefinition="FHIR resource to create/update", formalDefinition="When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields." ) 2014 protected Resource resource; 2015 2016 /** 2017 * A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete. 2018 */ 2019 @Child(name = "resourceId", type = {UrlType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2020 @Description(shortDefinition="A relative reference to the relevant resource.", formalDefinition="A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete." ) 2021 protected UrlType resourceId; 2022 2023 private static final long serialVersionUID = -1667751535L; 2024 2025 /** 2026 * Constructor 2027 */ 2028 public CDSHooksResponseCardsSuggestionsActionsComponent() { 2029 super(); 2030 } 2031 2032 /** 2033 * Constructor 2034 */ 2035 public CDSHooksResponseCardsSuggestionsActionsComponent(CDSActionTypeCodesVS type) { 2036 super(); 2037 this.setType(type); 2038 } 2039 2040 /** 2041 * @return {@link #type} (The type of action being performed. Allowed values are: create, update, delete.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2042 */ 2043 public Enumeration<CDSActionTypeCodesVS> getTypeElement() { 2044 if (this.type == null) 2045 if (Configuration.errorOnAutoCreate()) 2046 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsActionsComponent.type"); 2047 else if (Configuration.doAutoCreate()) 2048 this.type = new Enumeration<CDSActionTypeCodesVS>(new CDSActionTypeCodesVSEnumFactory()); // bb 2049 return this.type; 2050 } 2051 2052 public boolean hasTypeElement() { 2053 return this.type != null && !this.type.isEmpty(); 2054 } 2055 2056 public boolean hasType() { 2057 return this.type != null && !this.type.isEmpty(); 2058 } 2059 2060 /** 2061 * @param value {@link #type} (The type of action being performed. Allowed values are: create, update, delete.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2062 */ 2063 public CDSHooksResponseCardsSuggestionsActionsComponent setTypeElement(Enumeration<CDSActionTypeCodesVS> value) { 2064 this.type = value; 2065 return this; 2066 } 2067 2068 /** 2069 * @return The type of action being performed. Allowed values are: create, update, delete. 2070 */ 2071 public CDSActionTypeCodesVS getType() { 2072 return this.type == null ? null : this.type.getValue(); 2073 } 2074 2075 /** 2076 * @param value The type of action being performed. Allowed values are: create, update, delete. 2077 */ 2078 public CDSHooksResponseCardsSuggestionsActionsComponent setType(CDSActionTypeCodesVS value) { 2079 if (this.type == null) 2080 this.type = new Enumeration<CDSActionTypeCodesVS>(new CDSActionTypeCodesVSEnumFactory()); 2081 this.type.setValue(value); 2082 return this; 2083 } 2084 2085 /** 2086 * @return {@link #description} (Human-readable description of the suggested action that MAY be presented to the end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2087 */ 2088 public StringType getDescriptionElement() { 2089 if (this.description == null) 2090 if (Configuration.errorOnAutoCreate()) 2091 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsActionsComponent.description"); 2092 else if (Configuration.doAutoCreate()) 2093 this.description = new StringType(); // bb 2094 return this.description; 2095 } 2096 2097 public boolean hasDescriptionElement() { 2098 return this.description != null && !this.description.isEmpty(); 2099 } 2100 2101 public boolean hasDescription() { 2102 return this.description != null && !this.description.isEmpty(); 2103 } 2104 2105 /** 2106 * @param value {@link #description} (Human-readable description of the suggested action that MAY be presented to the end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2107 */ 2108 public CDSHooksResponseCardsSuggestionsActionsComponent setDescriptionElement(StringType value) { 2109 this.description = value; 2110 return this; 2111 } 2112 2113 /** 2114 * @return Human-readable description of the suggested action that MAY be presented to the end-user. 2115 */ 2116 public String getDescription() { 2117 return this.description == null ? null : this.description.getValue(); 2118 } 2119 2120 /** 2121 * @param value Human-readable description of the suggested action that MAY be presented to the end-user. 2122 */ 2123 public CDSHooksResponseCardsSuggestionsActionsComponent setDescription(String value) { 2124 if (Utilities.noString(value)) 2125 this.description = null; 2126 else { 2127 if (this.description == null) 2128 this.description = new StringType(); 2129 this.description.setValue(value); 2130 } 2131 return this; 2132 } 2133 2134 /** 2135 * @return {@link #resource} (When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields.) 2136 */ 2137 public Resource getResource() { 2138 return this.resource; 2139 } 2140 2141 public boolean hasResource() { 2142 return this.resource != null && !this.resource.isEmpty(); 2143 } 2144 2145 /** 2146 * @param value {@link #resource} (When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields.) 2147 */ 2148 public CDSHooksResponseCardsSuggestionsActionsComponent setResource(Resource value) { 2149 this.resource = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return {@link #resourceId} (A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete.). This is the underlying object with id, value and extensions. The accessor "getResourceId" gives direct access to the value 2155 */ 2156 public UrlType getResourceIdElement() { 2157 if (this.resourceId == null) 2158 if (Configuration.errorOnAutoCreate()) 2159 throw new Error("Attempt to auto-create CDSHooksResponseCardsSuggestionsActionsComponent.resourceId"); 2160 else if (Configuration.doAutoCreate()) 2161 this.resourceId = new UrlType(); // bb 2162 return this.resourceId; 2163 } 2164 2165 public boolean hasResourceIdElement() { 2166 return this.resourceId != null && !this.resourceId.isEmpty(); 2167 } 2168 2169 public boolean hasResourceId() { 2170 return this.resourceId != null && !this.resourceId.isEmpty(); 2171 } 2172 2173 /** 2174 * @param value {@link #resourceId} (A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete.). This is the underlying object with id, value and extensions. The accessor "getResourceId" gives direct access to the value 2175 */ 2176 public CDSHooksResponseCardsSuggestionsActionsComponent setResourceIdElement(UrlType value) { 2177 this.resourceId = value; 2178 return this; 2179 } 2180 2181 /** 2182 * @return A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete. 2183 */ 2184 public String getResourceId() { 2185 return this.resourceId == null ? null : this.resourceId.getValue(); 2186 } 2187 2188 /** 2189 * @param value A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete. 2190 */ 2191 public CDSHooksResponseCardsSuggestionsActionsComponent setResourceId(String value) { 2192 if (Utilities.noString(value)) 2193 this.resourceId = null; 2194 else { 2195 if (this.resourceId == null) 2196 this.resourceId = new UrlType(); 2197 this.resourceId.setValue(value); 2198 } 2199 return this; 2200 } 2201 2202 protected void listChildren(List<Property> children) { 2203 super.listChildren(children); 2204 children.add(new Property("type", "code", "The type of action being performed. Allowed values are: create, update, delete.", 0, 1, type)); 2205 children.add(new Property("description", "string", "Human-readable description of the suggested action that MAY be presented to the end-user.", 0, 1, description)); 2206 children.add(new Property("resource", "Resource", "When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields.", 0, 1, resource)); 2207 children.add(new Property("resourceId", "url", "A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete.", 0, 1, resourceId)); 2208 } 2209 2210 @Override 2211 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2212 switch (_hash) { 2213 case 3575610: /*type*/ return new Property("type", "code", "The type of action being performed. Allowed values are: create, update, delete.", 0, 1, type); 2214 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable description of the suggested action that MAY be presented to the end-user.", 0, 1, description); 2215 case -341064690: /*resource*/ return new Property("resource", "Resource", "When the type attribute is create, the resource attribute SHALL contain a new FHIR resource to be created. For update, this holds the updated resource in its entirety and not just the changed fields.", 0, 1, resource); 2216 case -1345650231: /*resourceId*/ return new Property("resourceId", "url", "A relative reference to the relevant resource. SHOULD be provided when the type attribute is delete.", 0, 1, resourceId); 2217 default: return super.getNamedProperty(_hash, _name, _checkValid); 2218 } 2219 2220 } 2221 2222 @Override 2223 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2224 switch (hash) { 2225 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<CDSActionTypeCodesVS> 2226 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2227 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 2228 case -1345650231: /*resourceId*/ return this.resourceId == null ? new Base[0] : new Base[] {this.resourceId}; // UrlType 2229 default: return super.getProperty(hash, name, checkValid); 2230 } 2231 2232 } 2233 2234 @Override 2235 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2236 switch (hash) { 2237 case 3575610: // type 2238 value = new CDSActionTypeCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 2239 this.type = (Enumeration) value; // Enumeration<CDSActionTypeCodesVS> 2240 return value; 2241 case -1724546052: // description 2242 this.description = TypeConvertor.castToString(value); // StringType 2243 return value; 2244 case -341064690: // resource 2245 this.resource = (Resource) value; // Resource 2246 return value; 2247 case -1345650231: // resourceId 2248 this.resourceId = TypeConvertor.castToUrl(value); // UrlType 2249 return value; 2250 default: return super.setProperty(hash, name, value); 2251 } 2252 2253 } 2254 2255 @Override 2256 public Base setProperty(String name, Base value) throws FHIRException { 2257 if (name.equals("type")) { 2258 value = new CDSActionTypeCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 2259 this.type = (Enumeration) value; // Enumeration<CDSActionTypeCodesVS> 2260 } else if (name.equals("description")) { 2261 this.description = TypeConvertor.castToString(value); // StringType 2262 } else if (name.equals("resource")) { 2263 this.resource = (Resource) value; // Resource 2264 } else if (name.equals("resourceId")) { 2265 this.resourceId = TypeConvertor.castToUrl(value); // UrlType 2266 } else 2267 return super.setProperty(name, value); 2268 return value; 2269 } 2270 2271 @Override 2272 public Base makeProperty(int hash, String name) throws FHIRException { 2273 switch (hash) { 2274 case 3575610: return getTypeElement(); 2275 case -1724546052: return getDescriptionElement(); 2276 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 2277 case -1345650231: return getResourceIdElement(); 2278 default: return super.makeProperty(hash, name); 2279 } 2280 2281 } 2282 2283 @Override 2284 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2285 switch (hash) { 2286 case 3575610: /*type*/ return new String[] {"code"}; 2287 case -1724546052: /*description*/ return new String[] {"string"}; 2288 case -341064690: /*resource*/ return new String[] {"Resource"}; 2289 case -1345650231: /*resourceId*/ return new String[] {"url"}; 2290 default: return super.getTypesForProperty(hash, name); 2291 } 2292 2293 } 2294 2295 @Override 2296 public Base addChild(String name) throws FHIRException { 2297 if (name.equals("type")) { 2298 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.actions.type"); 2299 } 2300 else if (name.equals("description")) { 2301 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.actions.description"); 2302 } 2303 else if (name.equals("resource")) { 2304 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards.suggestions.actions.resource"); 2305 } 2306 else if (name.equals("resourceId")) { 2307 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.suggestions.actions.resourceId"); 2308 } 2309 else 2310 return super.addChild(name); 2311 } 2312 2313 public CDSHooksResponseCardsSuggestionsActionsComponent copy() { 2314 CDSHooksResponseCardsSuggestionsActionsComponent dst = new CDSHooksResponseCardsSuggestionsActionsComponent(); 2315 copyValues(dst); 2316 return dst; 2317 } 2318 2319 public void copyValues(CDSHooksResponseCardsSuggestionsActionsComponent dst) { 2320 super.copyValues(dst); 2321 dst.type = type == null ? null : type.copy(); 2322 dst.description = description == null ? null : description.copy(); 2323 dst.resource = resource == null ? null : resource.copy(); 2324 dst.resourceId = resourceId == null ? null : resourceId.copy(); 2325 } 2326 2327 @Override 2328 public boolean equalsDeep(Base other_) { 2329 if (!super.equalsDeep(other_)) 2330 return false; 2331 if (!(other_ instanceof CDSHooksResponseCardsSuggestionsActionsComponent)) 2332 return false; 2333 CDSHooksResponseCardsSuggestionsActionsComponent o = (CDSHooksResponseCardsSuggestionsActionsComponent) other_; 2334 return compareDeep(type, o.type, true) && compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 2335 && compareDeep(resourceId, o.resourceId, true); 2336 } 2337 2338 @Override 2339 public boolean equalsShallow(Base other_) { 2340 if (!super.equalsShallow(other_)) 2341 return false; 2342 if (!(other_ instanceof CDSHooksResponseCardsSuggestionsActionsComponent)) 2343 return false; 2344 CDSHooksResponseCardsSuggestionsActionsComponent o = (CDSHooksResponseCardsSuggestionsActionsComponent) other_; 2345 return compareValues(type, o.type, true) && compareValues(description, o.description, true) && compareValues(resourceId, o.resourceId, true) 2346 ; 2347 } 2348 2349 public boolean isEmpty() { 2350 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, description, resource 2351 , resourceId); 2352 } 2353 2354 public String fhirType() { 2355 return "CDSHooksResponse.cards.suggestions.actions"; 2356 2357 } 2358 2359 } 2360 2361 @Block() 2362 public static class CDSHooksResponseCardsLinksComponent extends CDSHooksElement { 2363 /** 2364 * Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link). 2365 */ 2366 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2367 @Description(shortDefinition="Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link).", formalDefinition="Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link)." ) 2368 protected StringType label; 2369 2370 /** 2371 * URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash. 2372 */ 2373 @Child(name = "url", type = {UrlType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2374 @Description(shortDefinition="URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash.", formalDefinition="URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash." ) 2375 protected UrlType url; 2376 2377 /** 2378 * The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters 2379 */ 2380 @Child(name = "type", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2381 @Description(shortDefinition="absolute, smart - how to use the link", formalDefinition="The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters" ) 2382 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/tools/ValueSet/CDSLinkType") 2383 protected Enumeration<CDSLinkTypeCodesVS> type; 2384 2385 /** 2386 * An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it. 2387 */ 2388 @Child(name = "appContext", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2389 @Description(shortDefinition="Allows the CDS Service to share information from the CDS card with a subsequently launched SMART app", formalDefinition="An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it." ) 2390 protected StringType appContext; 2391 2392 private static final long serialVersionUID = 1992417446L; 2393 2394 /** 2395 * Constructor 2396 */ 2397 public CDSHooksResponseCardsLinksComponent() { 2398 super(); 2399 } 2400 2401 /** 2402 * Constructor 2403 */ 2404 public CDSHooksResponseCardsLinksComponent(String label, String url) { 2405 super(); 2406 this.setLabel(label); 2407 this.setUrl(url); 2408 } 2409 2410 /** 2411 * @return {@link #label} (Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link).). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2412 */ 2413 public StringType getLabelElement() { 2414 if (this.label == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create CDSHooksResponseCardsLinksComponent.label"); 2417 else if (Configuration.doAutoCreate()) 2418 this.label = new StringType(); // bb 2419 return this.label; 2420 } 2421 2422 public boolean hasLabelElement() { 2423 return this.label != null && !this.label.isEmpty(); 2424 } 2425 2426 public boolean hasLabel() { 2427 return this.label != null && !this.label.isEmpty(); 2428 } 2429 2430 /** 2431 * @param value {@link #label} (Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link).). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2432 */ 2433 public CDSHooksResponseCardsLinksComponent setLabelElement(StringType value) { 2434 this.label = value; 2435 return this; 2436 } 2437 2438 /** 2439 * @return Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link). 2440 */ 2441 public String getLabel() { 2442 return this.label == null ? null : this.label.getValue(); 2443 } 2444 2445 /** 2446 * @param value Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link). 2447 */ 2448 public CDSHooksResponseCardsLinksComponent setLabel(String value) { 2449 if (this.label == null) 2450 this.label = new StringType(); 2451 this.label.setValue(value); 2452 return this; 2453 } 2454 2455 /** 2456 * @return {@link #url} (URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2457 */ 2458 public UrlType getUrlElement() { 2459 if (this.url == null) 2460 if (Configuration.errorOnAutoCreate()) 2461 throw new Error("Attempt to auto-create CDSHooksResponseCardsLinksComponent.url"); 2462 else if (Configuration.doAutoCreate()) 2463 this.url = new UrlType(); // bb 2464 return this.url; 2465 } 2466 2467 public boolean hasUrlElement() { 2468 return this.url != null && !this.url.isEmpty(); 2469 } 2470 2471 public boolean hasUrl() { 2472 return this.url != null && !this.url.isEmpty(); 2473 } 2474 2475 /** 2476 * @param value {@link #url} (URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2477 */ 2478 public CDSHooksResponseCardsLinksComponent setUrlElement(UrlType value) { 2479 this.url = value; 2480 return this; 2481 } 2482 2483 /** 2484 * @return URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash. 2485 */ 2486 public String getUrl() { 2487 return this.url == null ? null : this.url.getValue(); 2488 } 2489 2490 /** 2491 * @param value URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash. 2492 */ 2493 public CDSHooksResponseCardsLinksComponent setUrl(String value) { 2494 if (this.url == null) 2495 this.url = new UrlType(); 2496 this.url.setValue(value); 2497 return this; 2498 } 2499 2500 /** 2501 * @return {@link #type} (The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2502 */ 2503 public Enumeration<CDSLinkTypeCodesVS> getTypeElement() { 2504 if (this.type == null) 2505 if (Configuration.errorOnAutoCreate()) 2506 throw new Error("Attempt to auto-create CDSHooksResponseCardsLinksComponent.type"); 2507 else if (Configuration.doAutoCreate()) 2508 this.type = new Enumeration<CDSLinkTypeCodesVS>(new CDSLinkTypeCodesVSEnumFactory()); // bb 2509 return this.type; 2510 } 2511 2512 public boolean hasTypeElement() { 2513 return this.type != null && !this.type.isEmpty(); 2514 } 2515 2516 public boolean hasType() { 2517 return this.type != null && !this.type.isEmpty(); 2518 } 2519 2520 /** 2521 * @param value {@link #type} (The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2522 */ 2523 public CDSHooksResponseCardsLinksComponent setTypeElement(Enumeration<CDSLinkTypeCodesVS> value) { 2524 this.type = value; 2525 return this; 2526 } 2527 2528 /** 2529 * @return The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters 2530 */ 2531 public CDSLinkTypeCodesVS getType() { 2532 return this.type == null ? null : this.type.getValue(); 2533 } 2534 2535 /** 2536 * @param value The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters 2537 */ 2538 public CDSHooksResponseCardsLinksComponent setType(CDSLinkTypeCodesVS value) { 2539 if (value == null) 2540 this.type = null; 2541 else { 2542 if (this.type == null) 2543 this.type = new Enumeration<CDSLinkTypeCodesVS>(new CDSLinkTypeCodesVSEnumFactory()); 2544 this.type.setValue(value); 2545 } 2546 return this; 2547 } 2548 2549 /** 2550 * @return {@link #appContext} (An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it.). This is the underlying object with id, value and extensions. The accessor "getAppContext" gives direct access to the value 2551 */ 2552 public StringType getAppContextElement() { 2553 if (this.appContext == null) 2554 if (Configuration.errorOnAutoCreate()) 2555 throw new Error("Attempt to auto-create CDSHooksResponseCardsLinksComponent.appContext"); 2556 else if (Configuration.doAutoCreate()) 2557 this.appContext = new StringType(); // bb 2558 return this.appContext; 2559 } 2560 2561 public boolean hasAppContextElement() { 2562 return this.appContext != null && !this.appContext.isEmpty(); 2563 } 2564 2565 public boolean hasAppContext() { 2566 return this.appContext != null && !this.appContext.isEmpty(); 2567 } 2568 2569 /** 2570 * @param value {@link #appContext} (An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it.). This is the underlying object with id, value and extensions. The accessor "getAppContext" gives direct access to the value 2571 */ 2572 public CDSHooksResponseCardsLinksComponent setAppContextElement(StringType value) { 2573 this.appContext = value; 2574 return this; 2575 } 2576 2577 /** 2578 * @return An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it. 2579 */ 2580 public String getAppContext() { 2581 return this.appContext == null ? null : this.appContext.getValue(); 2582 } 2583 2584 /** 2585 * @param value An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it. 2586 */ 2587 public CDSHooksResponseCardsLinksComponent setAppContext(String value) { 2588 if (Utilities.noString(value)) 2589 this.appContext = null; 2590 else { 2591 if (this.appContext == null) 2592 this.appContext = new StringType(); 2593 this.appContext.setValue(value); 2594 } 2595 return this; 2596 } 2597 2598 protected void listChildren(List<Property> children) { 2599 super.listChildren(children); 2600 children.add(new Property("label", "string", "Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link).", 0, 1, label)); 2601 children.add(new Property("url", "url", "URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash.", 0, 1, url)); 2602 children.add(new Property("type", "code", "The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters", 0, 1, type)); 2603 children.add(new Property("appContext", "string", "An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it.", 0, 1, appContext)); 2604 } 2605 2606 @Override 2607 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2608 switch (_hash) { 2609 case 102727412: /*label*/ return new Property("label", "string", "Human-readable label to display for this link (e.g. the CDS Client might render this as the underlined text of a clickable link).", 0, 1, label); 2610 case 116079: /*url*/ return new Property("url", "url", "URL to load (via GET, in a browser context) when a user clicks on this link. Note that this MAY be a 'deep link' with context embedded in path segments, query parameters, or a hash.", 0, 1, url); 2611 case 3575610: /*type*/ return new Property("type", "code", "The type of the given URL. There are two possible values for this field. A type of absolute indicates that the URL is absolute and should be treated as-is. A type of smart indicates that the URL is a SMART app launch URL and the CDS Client should ensure the SMART app launch URL is populated with the appropriate SMART launch parameters", 0, 1, type); 2612 case 2084035662: /*appContext*/ return new Property("appContext", "string", "An optional field that allows the CDS Service to share information from the CDS card with a subsequently launched SMART app. The appContext field should only be valued if the link type is smart and is not valid for absolute links. The appContext field and value will be sent to the SMART app as part of the OAuth 2.0 access token response, alongside the other SMART launch parameters when the SMART app is launched. Note that appContext could be escaped JSON, base64 encoded XML, or even a simple string, so long as the SMART app can recognize it.", 0, 1, appContext); 2613 default: return super.getNamedProperty(_hash, _name, _checkValid); 2614 } 2615 2616 } 2617 2618 @Override 2619 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2620 switch (hash) { 2621 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 2622 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 2623 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<CDSLinkTypeCodesVS> 2624 case 2084035662: /*appContext*/ return this.appContext == null ? new Base[0] : new Base[] {this.appContext}; // StringType 2625 default: return super.getProperty(hash, name, checkValid); 2626 } 2627 2628 } 2629 2630 @Override 2631 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2632 switch (hash) { 2633 case 102727412: // label 2634 this.label = TypeConvertor.castToString(value); // StringType 2635 return value; 2636 case 116079: // url 2637 this.url = TypeConvertor.castToUrl(value); // UrlType 2638 return value; 2639 case 3575610: // type 2640 value = new CDSLinkTypeCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 2641 this.type = (Enumeration) value; // Enumeration<CDSLinkTypeCodesVS> 2642 return value; 2643 case 2084035662: // appContext 2644 this.appContext = TypeConvertor.castToString(value); // StringType 2645 return value; 2646 default: return super.setProperty(hash, name, value); 2647 } 2648 2649 } 2650 2651 @Override 2652 public Base setProperty(String name, Base value) throws FHIRException { 2653 if (name.equals("label")) { 2654 this.label = TypeConvertor.castToString(value); // StringType 2655 } else if (name.equals("url")) { 2656 this.url = TypeConvertor.castToUrl(value); // UrlType 2657 } else if (name.equals("type")) { 2658 value = new CDSLinkTypeCodesVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 2659 this.type = (Enumeration) value; // Enumeration<CDSLinkTypeCodesVS> 2660 } else if (name.equals("appContext")) { 2661 this.appContext = TypeConvertor.castToString(value); // StringType 2662 } else 2663 return super.setProperty(name, value); 2664 return value; 2665 } 2666 2667 @Override 2668 public Base makeProperty(int hash, String name) throws FHIRException { 2669 switch (hash) { 2670 case 102727412: return getLabelElement(); 2671 case 116079: return getUrlElement(); 2672 case 3575610: return getTypeElement(); 2673 case 2084035662: return getAppContextElement(); 2674 default: return super.makeProperty(hash, name); 2675 } 2676 2677 } 2678 2679 @Override 2680 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2681 switch (hash) { 2682 case 102727412: /*label*/ return new String[] {"string"}; 2683 case 116079: /*url*/ return new String[] {"url"}; 2684 case 3575610: /*type*/ return new String[] {"code"}; 2685 case 2084035662: /*appContext*/ return new String[] {"string"}; 2686 default: return super.getTypesForProperty(hash, name); 2687 } 2688 2689 } 2690 2691 @Override 2692 public Base addChild(String name) throws FHIRException { 2693 if (name.equals("label")) { 2694 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.links.label"); 2695 } 2696 else if (name.equals("url")) { 2697 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.links.url"); 2698 } 2699 else if (name.equals("type")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.links.type"); 2701 } 2702 else if (name.equals("appContext")) { 2703 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksResponse.cards.links.appContext"); 2704 } 2705 else 2706 return super.addChild(name); 2707 } 2708 2709 public CDSHooksResponseCardsLinksComponent copy() { 2710 CDSHooksResponseCardsLinksComponent dst = new CDSHooksResponseCardsLinksComponent(); 2711 copyValues(dst); 2712 return dst; 2713 } 2714 2715 public void copyValues(CDSHooksResponseCardsLinksComponent dst) { 2716 super.copyValues(dst); 2717 dst.label = label == null ? null : label.copy(); 2718 dst.url = url == null ? null : url.copy(); 2719 dst.type = type == null ? null : type.copy(); 2720 dst.appContext = appContext == null ? null : appContext.copy(); 2721 } 2722 2723 @Override 2724 public boolean equalsDeep(Base other_) { 2725 if (!super.equalsDeep(other_)) 2726 return false; 2727 if (!(other_ instanceof CDSHooksResponseCardsLinksComponent)) 2728 return false; 2729 CDSHooksResponseCardsLinksComponent o = (CDSHooksResponseCardsLinksComponent) other_; 2730 return compareDeep(label, o.label, true) && compareDeep(url, o.url, true) && compareDeep(type, o.type, true) 2731 && compareDeep(appContext, o.appContext, true); 2732 } 2733 2734 @Override 2735 public boolean equalsShallow(Base other_) { 2736 if (!super.equalsShallow(other_)) 2737 return false; 2738 if (!(other_ instanceof CDSHooksResponseCardsLinksComponent)) 2739 return false; 2740 CDSHooksResponseCardsLinksComponent o = (CDSHooksResponseCardsLinksComponent) other_; 2741 return compareValues(label, o.label, true) && compareValues(url, o.url, true) && compareValues(type, o.type, true) 2742 && compareValues(appContext, o.appContext, true); 2743 } 2744 2745 public boolean isEmpty() { 2746 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, url, type, appContext 2747 ); 2748 } 2749 2750 public String fhirType() { 2751 return "CDSHooksResponse.cards.links"; 2752 2753 } 2754 2755 } 2756 2757 /** 2758 * An array of Cards. Cards can provide a combination of information (for reading), suggested actions (to be applied if a user selects them), and links (to launch an app if the user selects them). 2759 */ 2760 @Child(name = "cards", type = {CDSHooksElement.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2761 @Description(shortDefinition="An array of Cards that provide information, suggested actions, and links", formalDefinition="An array of Cards. Cards can provide a combination of information (for reading), suggested actions (to be applied if a user selects them), and links (to launch an app if the user selects them)." ) 2762 protected List<CDSHooksResponseCardsComponent> cardsList; 2763 2764 /** 2765 * An array of Actions that the CDS Service proposes to auto-apply 2766 */ 2767 @Child(name = "systemActions", type = {CDSHooksResponseCardsSuggestionsActionsComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2768 @Description(shortDefinition="An array of Actions that the CDS Service proposes to auto-apply", formalDefinition="An array of Actions that the CDS Service proposes to auto-apply" ) 2769 protected List<CDSHooksResponseCardsSuggestionsActionsComponent> systemActionsList; 2770 2771 private static final long serialVersionUID = -131860217L; 2772 2773 /** 2774 * Constructor 2775 */ 2776 public CDSHooksResponse() { 2777 super(); 2778 } 2779 2780 /** 2781 * @return {@link #cards} (An array of Cards. Cards can provide a combination of information (for reading), suggested actions (to be applied if a user selects them), and links (to launch an app if the user selects them).) 2782 */ 2783 public List<CDSHooksResponseCardsComponent> getCardsList() { 2784 if (this.cardsList == null) 2785 this.cardsList = new ArrayList<CDSHooksResponseCardsComponent>(); 2786 return this.cardsList; 2787 } 2788 2789 /** 2790 * @return Returns a reference to <code>this</code> for easy method chaining 2791 */ 2792 public CDSHooksResponse setCardsList(List<CDSHooksResponseCardsComponent> theCards) { 2793 this.cardsList = theCards; 2794 return this; 2795 } 2796 2797 public boolean hasCards() { 2798 if (this.cardsList == null) 2799 return false; 2800 for (CDSHooksResponseCardsComponent item : this.cardsList) 2801 if (!item.isEmpty()) 2802 return true; 2803 return false; 2804 } 2805 2806 public CDSHooksResponseCardsComponent addCards() { //3a 2807 CDSHooksResponseCardsComponent t = new CDSHooksResponseCardsComponent(); 2808 if (this.cardsList == null) 2809 this.cardsList = new ArrayList<CDSHooksResponseCardsComponent>(); 2810 this.cardsList.add(t); 2811 return t; 2812 } 2813 2814 public CDSHooksResponse addCards(CDSHooksResponseCardsComponent t) { //3b 2815 if (t == null) 2816 return this; 2817 if (this.cardsList == null) 2818 this.cardsList = new ArrayList<CDSHooksResponseCardsComponent>(); 2819 this.cardsList.add(t); 2820 return this; 2821 } 2822 2823 /** 2824 * @return The first repetition of repeating field {@link #cards}, creating it if it does not already exist {3} 2825 */ 2826 public CDSHooksResponseCardsComponent getCardsFirstRep() { 2827 if (getCardsList().isEmpty()) { 2828 addCards(); 2829 } 2830 return getCardsList().get(0); 2831 } 2832 2833 /** 2834 * @return {@link #systemActions} (An array of Actions that the CDS Service proposes to auto-apply) 2835 */ 2836 public List<CDSHooksResponseCardsSuggestionsActionsComponent> getSystemActionsList() { 2837 if (this.systemActionsList == null) 2838 this.systemActionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 2839 return this.systemActionsList; 2840 } 2841 2842 /** 2843 * @return Returns a reference to <code>this</code> for easy method chaining 2844 */ 2845 public CDSHooksResponse setSystemActionsList(List<CDSHooksResponseCardsSuggestionsActionsComponent> theSystemActions) { 2846 this.systemActionsList = theSystemActions; 2847 return this; 2848 } 2849 2850 public boolean hasSystemActions() { 2851 if (this.systemActionsList == null) 2852 return false; 2853 for (CDSHooksResponseCardsSuggestionsActionsComponent item : this.systemActionsList) 2854 if (!item.isEmpty()) 2855 return true; 2856 return false; 2857 } 2858 2859 public CDSHooksResponseCardsSuggestionsActionsComponent addSystemActions() { //3a 2860 CDSHooksResponseCardsSuggestionsActionsComponent t = new CDSHooksResponseCardsSuggestionsActionsComponent(); 2861 if (this.systemActionsList == null) 2862 this.systemActionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 2863 this.systemActionsList.add(t); 2864 return t; 2865 } 2866 2867 public CDSHooksResponse addSystemActions(CDSHooksResponseCardsSuggestionsActionsComponent t) { //3b 2868 if (t == null) 2869 return this; 2870 if (this.systemActionsList == null) 2871 this.systemActionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 2872 this.systemActionsList.add(t); 2873 return this; 2874 } 2875 2876 /** 2877 * @return The first repetition of repeating field {@link #systemActions}, creating it if it does not already exist {3} 2878 */ 2879 public CDSHooksResponseCardsSuggestionsActionsComponent getSystemActionsFirstRep() { 2880 if (getSystemActionsList().isEmpty()) { 2881 addSystemActions(); 2882 } 2883 return getSystemActionsList().get(0); 2884 } 2885 2886 protected void listChildren(List<Property> children) { 2887 super.listChildren(children); 2888 children.add(new Property("cards", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "An array of Cards. Cards can provide a combination of information (for reading), suggested actions (to be applied if a user selects them), and links (to launch an app if the user selects them).", 0, java.lang.Integer.MAX_VALUE, cardsList)); 2889 children.add(new Property("systemActions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksResponse@CDSHooksResponse.cards.suggestions.actions", "An array of Actions that the CDS Service proposes to auto-apply", 0, java.lang.Integer.MAX_VALUE, systemActionsList)); 2890 } 2891 2892 @Override 2893 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2894 switch (_hash) { 2895 case 94431075: /*cards*/ return new Property("cards", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "An array of Cards. Cards can provide a combination of information (for reading), suggested actions (to be applied if a user selects them), and links (to launch an app if the user selects them).", 0, java.lang.Integer.MAX_VALUE, cardsList); 2896 case 867134414: /*systemActions*/ return new Property("systemActions", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksResponse@CDSHooksResponse.cards.suggestions.actions", "An array of Actions that the CDS Service proposes to auto-apply", 0, java.lang.Integer.MAX_VALUE, systemActionsList); 2897 default: return super.getNamedProperty(_hash, _name, _checkValid); 2898 } 2899 2900 } 2901 2902 @Override 2903 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2904 switch (hash) { 2905 case 94431075: /*cards*/ return this.cardsList == null ? new Base[0] : this.cardsList.toArray(new Base[this.cardsList.size()]); // CDSHooksResponseCardsComponent 2906 case 867134414: /*systemActions*/ return this.systemActionsList == null ? new Base[0] : this.systemActionsList.toArray(new Base[this.systemActionsList.size()]); // CDSHooksResponseCardsSuggestionsActionsComponent 2907 default: return super.getProperty(hash, name, checkValid); 2908 } 2909 2910 } 2911 2912 @Override 2913 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2914 switch (hash) { 2915 case 94431075: // cards 2916 this.getCardsList().add((CDSHooksResponseCardsComponent) value); // CDSHooksResponseCardsComponent 2917 return value; 2918 case 867134414: // systemActions 2919 this.getSystemActionsList().add((CDSHooksResponseCardsSuggestionsActionsComponent) value); // CDSHooksResponseCardsSuggestionsActionsComponent 2920 return value; 2921 default: return super.setProperty(hash, name, value); 2922 } 2923 2924 } 2925 2926 @Override 2927 public Base setProperty(String name, Base value) throws FHIRException { 2928 if (name.equals("cards")) { 2929 this.getCardsList().add((CDSHooksResponseCardsComponent) value); // CDSHooksResponseCardsComponent 2930 } else if (name.equals("systemActions")) { 2931 this.getSystemActionsList().add((CDSHooksResponseCardsSuggestionsActionsComponent) value); // CDSHooksResponseCardsSuggestionsActionsComponent 2932 } else 2933 return super.setProperty(name, value); 2934 return value; 2935 } 2936 2937 @Override 2938 public Base makeProperty(int hash, String name) throws FHIRException { 2939 switch (hash) { 2940 case 94431075: return addCards(); 2941 case 867134414: return addSystemActions(); 2942 default: return super.makeProperty(hash, name); 2943 } 2944 2945 } 2946 2947 @Override 2948 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2949 switch (hash) { 2950 case 94431075: /*cards*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 2951 case 867134414: /*systemActions*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksResponse@CDSHooksResponse.cards.suggestions.actions"}; 2952 default: return super.getTypesForProperty(hash, name); 2953 } 2954 2955 } 2956 2957 @Override 2958 public Base addChild(String name) throws FHIRException { 2959 if (name.equals("cards")) { 2960 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksResponse.cards"); 2961 } 2962 else if (name.equals("systemActions")) { 2963 return addSystemActions(); 2964 } 2965 else 2966 return super.addChild(name); 2967 } 2968 2969 public String fhirType() { 2970 return "CDSHooksResponse"; 2971 2972 } 2973 2974 public CDSHooksResponse copy() { 2975 CDSHooksResponse dst = new CDSHooksResponse(); 2976 copyValues(dst); 2977 return dst; 2978 } 2979 2980 public void copyValues(CDSHooksResponse dst) { 2981 super.copyValues(dst); 2982 if (cardsList != null) { 2983 dst.cardsList = new ArrayList<CDSHooksResponseCardsComponent>(); 2984 for (CDSHooksResponseCardsComponent i : cardsList) 2985 dst.cardsList.add(i.copy()); 2986 }; 2987 if (systemActionsList != null) { 2988 dst.systemActionsList = new ArrayList<CDSHooksResponseCardsSuggestionsActionsComponent>(); 2989 for (CDSHooksResponseCardsSuggestionsActionsComponent i : systemActionsList) 2990 dst.systemActionsList.add(i.copy()); 2991 }; 2992 } 2993 2994 protected CDSHooksResponse typedCopy() { 2995 return copy(); 2996 } 2997 2998 @Override 2999 public boolean equalsDeep(Base other_) { 3000 if (!super.equalsDeep(other_)) 3001 return false; 3002 if (!(other_ instanceof CDSHooksResponse)) 3003 return false; 3004 CDSHooksResponse o = (CDSHooksResponse) other_; 3005 return compareDeep(cardsList, o.cardsList, true) && compareDeep(systemActionsList, o.systemActionsList, true) 3006 ; 3007 } 3008 3009 @Override 3010 public boolean equalsShallow(Base other_) { 3011 if (!super.equalsShallow(other_)) 3012 return false; 3013 if (!(other_ instanceof CDSHooksResponse)) 3014 return false; 3015 CDSHooksResponse o = (CDSHooksResponse) other_; 3016 return true; 3017 } 3018 3019 public boolean isEmpty() { 3020 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cardsList, systemActionsList 3021 ); 3022 } 3023 3024 3025} 3026