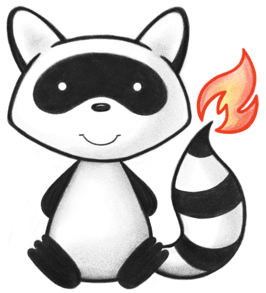
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * This structure is defined to allow the FHIR Validator to validate a CDSHooks Services response body. TODO: This content will be moved to the CDS Hooks specification in the future 050 */ 051@DatatypeDef(name="CDSHooksServices") 052public class CDSHooksServices extends CDSHooksElement implements ICompositeType { 053 054 @Block() 055 public static class CDSHooksServicesServicesComponent extends CDSHooksElement { 056 /** 057 * The hook this Services should be invoked on 058 */ 059 @Child(name = "hook", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 060 @Description(shortDefinition="The hook this Services should be invoked on", formalDefinition="The hook this Services should be invoked on" ) 061 protected StringType hook; 062 063 /** 064 * The human-friendly name of this Services (Recommended) 065 */ 066 @Child(name = "title", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 067 @Description(shortDefinition="The human-friendly name of this Services (Recommended)", formalDefinition="The human-friendly name of this Services (Recommended)" ) 068 protected StringType title; 069 070 /** 071 * The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}``` 072 */ 073 @Child(name = "id", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 074 @Description(shortDefinition="The URL to this service which is available at: {baseUrl}/cds-services/{id}", formalDefinition="The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}```" ) 075 protected CodeType id; 076 077 /** 078 * The description of this Services 079 */ 080 @Child(name = "description", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 081 @Description(shortDefinition="The description of this Services", formalDefinition="The description of this Services" ) 082 protected StringType description; 083 084 /** 085 * Human-friendly description of any preconditions for the use of this CDS Services 086 */ 087 @Child(name = "usageRequirements", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 088 @Description(shortDefinition="Human-friendly description of any preconditions for the use of this CDS Services", formalDefinition="Human-friendly description of any preconditions for the use of this CDS Services" ) 089 protected StringType usageRequirements; 090 091 /** 092 * An object containing key/value pairs of FHIR queries that this Services is requesting the CDS Client to perform and provide on each Services call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query 093 */ 094 @Child(name = "prefetch", type = {Base.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 095 @Description(shortDefinition="Key/value pairs of FHIR queries the CDS Client provides on each call", formalDefinition="An object containing key/value pairs of FHIR queries that this Services is requesting the CDS Client to perform and provide on each Services call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query" ) 096 protected List<CDSHooksServicesServicesPrefetchComponent> prefetchList; 097 098 private static final long serialVersionUID = 1303422447L; 099 100 /** 101 * Constructor 102 */ 103 public CDSHooksServicesServicesComponent() { 104 super(); 105 } 106 107 /** 108 * Constructor 109 */ 110 public CDSHooksServicesServicesComponent(String hook, String id, String description) { 111 super(); 112 this.setHook(hook); 113 this.setId(id); 114 this.setDescription(description); 115 } 116 117 /** 118 * @return {@link #hook} (The hook this Services should be invoked on). This is the underlying object with id, value and extensions. The accessor "getHook" gives direct access to the value 119 */ 120 public StringType getHookElement() { 121 if (this.hook == null) 122 if (Configuration.errorOnAutoCreate()) 123 throw new Error("Attempt to auto-create CDSHooksServicesServicesComponent.hook"); 124 else if (Configuration.doAutoCreate()) 125 this.hook = new StringType(); // bb 126 return this.hook; 127 } 128 129 public boolean hasHookElement() { 130 return this.hook != null && !this.hook.isEmpty(); 131 } 132 133 public boolean hasHook() { 134 return this.hook != null && !this.hook.isEmpty(); 135 } 136 137 /** 138 * @param value {@link #hook} (The hook this Services should be invoked on). This is the underlying object with id, value and extensions. The accessor "getHook" gives direct access to the value 139 */ 140 public CDSHooksServicesServicesComponent setHookElement(StringType value) { 141 this.hook = value; 142 return this; 143 } 144 145 /** 146 * @return The hook this Services should be invoked on 147 */ 148 public String getHook() { 149 return this.hook == null ? null : this.hook.getValue(); 150 } 151 152 /** 153 * @param value The hook this Services should be invoked on 154 */ 155 public CDSHooksServicesServicesComponent setHook(String value) { 156 if (this.hook == null) 157 this.hook = new StringType(); 158 this.hook.setValue(value); 159 return this; 160 } 161 162 /** 163 * @return {@link #title} (The human-friendly name of this Services (Recommended)). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 164 */ 165 public StringType getTitleElement() { 166 if (this.title == null) 167 if (Configuration.errorOnAutoCreate()) 168 throw new Error("Attempt to auto-create CDSHooksServicesServicesComponent.title"); 169 else if (Configuration.doAutoCreate()) 170 this.title = new StringType(); // bb 171 return this.title; 172 } 173 174 public boolean hasTitleElement() { 175 return this.title != null && !this.title.isEmpty(); 176 } 177 178 public boolean hasTitle() { 179 return this.title != null && !this.title.isEmpty(); 180 } 181 182 /** 183 * @param value {@link #title} (The human-friendly name of this Services (Recommended)). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 184 */ 185 public CDSHooksServicesServicesComponent setTitleElement(StringType value) { 186 this.title = value; 187 return this; 188 } 189 190 /** 191 * @return The human-friendly name of this Services (Recommended) 192 */ 193 public String getTitle() { 194 return this.title == null ? null : this.title.getValue(); 195 } 196 197 /** 198 * @param value The human-friendly name of this Services (Recommended) 199 */ 200 public CDSHooksServicesServicesComponent setTitle(String value) { 201 if (Utilities.noString(value)) 202 this.title = null; 203 else { 204 if (this.title == null) 205 this.title = new StringType(); 206 this.title.setValue(value); 207 } 208 return this; 209 } 210 211 /** 212 * @return {@link #id} (The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}```). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 213 */ 214 public CodeType getIdElement() { 215 if (this.id == null) 216 if (Configuration.errorOnAutoCreate()) 217 throw new Error("Attempt to auto-create CDSHooksServicesServicesComponent.id"); 218 else if (Configuration.doAutoCreate()) 219 this.id = new CodeType(); // bb 220 return this.id; 221 } 222 223 public boolean hasIdElement() { 224 return this.id != null && !this.id.isEmpty(); 225 } 226 227 public boolean hasId() { 228 return this.id != null && !this.id.isEmpty(); 229 } 230 231 /** 232 * @param value {@link #id} (The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}```). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 233 */ 234 public CDSHooksServicesServicesComponent setIdElement(CodeType value) { 235 this.id = value; 236 return this; 237 } 238 239 /** 240 * @return The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}``` 241 */ 242 public String getId() { 243 return this.id == null ? null : this.id.getValue(); 244 } 245 246 /** 247 * @param value The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}``` 248 */ 249 public CDSHooksServicesServicesComponent setId(String value) { 250 if (this.id == null) 251 this.id = new CodeType(); 252 this.id.setValue(value); 253 return this; 254 } 255 256 /** 257 * @return {@link #description} (The description of this Services). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 258 */ 259 public StringType getDescriptionElement() { 260 if (this.description == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create CDSHooksServicesServicesComponent.description"); 263 else if (Configuration.doAutoCreate()) 264 this.description = new StringType(); // bb 265 return this.description; 266 } 267 268 public boolean hasDescriptionElement() { 269 return this.description != null && !this.description.isEmpty(); 270 } 271 272 public boolean hasDescription() { 273 return this.description != null && !this.description.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #description} (The description of this Services). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 278 */ 279 public CDSHooksServicesServicesComponent setDescriptionElement(StringType value) { 280 this.description = value; 281 return this; 282 } 283 284 /** 285 * @return The description of this Services 286 */ 287 public String getDescription() { 288 return this.description == null ? null : this.description.getValue(); 289 } 290 291 /** 292 * @param value The description of this Services 293 */ 294 public CDSHooksServicesServicesComponent setDescription(String value) { 295 if (this.description == null) 296 this.description = new StringType(); 297 this.description.setValue(value); 298 return this; 299 } 300 301 /** 302 * @return {@link #usageRequirements} (Human-friendly description of any preconditions for the use of this CDS Services). This is the underlying object with id, value and extensions. The accessor "getUsageRequirements" gives direct access to the value 303 */ 304 public StringType getUsageRequirementsElement() { 305 if (this.usageRequirements == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create CDSHooksServicesServicesComponent.usageRequirements"); 308 else if (Configuration.doAutoCreate()) 309 this.usageRequirements = new StringType(); // bb 310 return this.usageRequirements; 311 } 312 313 public boolean hasUsageRequirementsElement() { 314 return this.usageRequirements != null && !this.usageRequirements.isEmpty(); 315 } 316 317 public boolean hasUsageRequirements() { 318 return this.usageRequirements != null && !this.usageRequirements.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #usageRequirements} (Human-friendly description of any preconditions for the use of this CDS Services). This is the underlying object with id, value and extensions. The accessor "getUsageRequirements" gives direct access to the value 323 */ 324 public CDSHooksServicesServicesComponent setUsageRequirementsElement(StringType value) { 325 this.usageRequirements = value; 326 return this; 327 } 328 329 /** 330 * @return Human-friendly description of any preconditions for the use of this CDS Services 331 */ 332 public String getUsageRequirements() { 333 return this.usageRequirements == null ? null : this.usageRequirements.getValue(); 334 } 335 336 /** 337 * @param value Human-friendly description of any preconditions for the use of this CDS Services 338 */ 339 public CDSHooksServicesServicesComponent setUsageRequirements(String value) { 340 if (Utilities.noString(value)) 341 this.usageRequirements = null; 342 else { 343 if (this.usageRequirements == null) 344 this.usageRequirements = new StringType(); 345 this.usageRequirements.setValue(value); 346 } 347 return this; 348 } 349 350 /** 351 * @return {@link #prefetch} (An object containing key/value pairs of FHIR queries that this Services is requesting the CDS Client to perform and provide on each Services call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query) 352 */ 353 public List<CDSHooksServicesServicesPrefetchComponent> getPrefetchList() { 354 if (this.prefetchList == null) 355 this.prefetchList = new ArrayList<CDSHooksServicesServicesPrefetchComponent>(); 356 return this.prefetchList; 357 } 358 359 /** 360 * @return Returns a reference to <code>this</code> for easy method chaining 361 */ 362 public CDSHooksServicesServicesComponent setPrefetchList(List<CDSHooksServicesServicesPrefetchComponent> thePrefetch) { 363 this.prefetchList = thePrefetch; 364 return this; 365 } 366 367 public boolean hasPrefetch() { 368 if (this.prefetchList == null) 369 return false; 370 for (CDSHooksServicesServicesPrefetchComponent item : this.prefetchList) 371 if (!item.isEmpty()) 372 return true; 373 return false; 374 } 375 376 public CDSHooksServicesServicesComponent addPrefetch(CDSHooksServicesServicesPrefetchComponent t) { //3b 377 if (t == null) 378 return this; 379 if (this.prefetchList == null) 380 this.prefetchList = new ArrayList<CDSHooksServicesServicesPrefetchComponent>(); 381 this.prefetchList.add(t); 382 return this; 383 } 384 385 protected void listChildren(List<Property> children) { 386 super.listChildren(children); 387 children.add(new Property("hook", "string", "The hook this Services should be invoked on", 0, 1, hook)); 388 children.add(new Property("title", "string", "The human-friendly name of this Services (Recommended)", 0, 1, title)); 389 children.add(new Property("id", "code", "The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}```", 0, 1, id)); 390 children.add(new Property("description", "string", "The description of this Services", 0, 1, description)); 391 children.add(new Property("usageRequirements", "string", "Human-friendly description of any preconditions for the use of this CDS Services", 0, 1, usageRequirements)); 392 children.add(new Property("prefetch", "Base", "An object containing key/value pairs of FHIR queries that this Services is requesting the CDS Client to perform and provide on each Services call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query", 0, java.lang.Integer.MAX_VALUE, prefetchList)); 393 } 394 395 @Override 396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 397 switch (_hash) { 398 case 3208483: /*hook*/ return new Property("hook", "string", "The hook this Services should be invoked on", 0, 1, hook); 399 case 110371416: /*title*/ return new Property("title", "string", "The human-friendly name of this Services (Recommended)", 0, 1, title); 400 case 3355: /*id*/ return new Property("id", "code", "The {id} portion of the URL to this service which is available at ```{baseUrl}/cds-services/{id}```", 0, 1, id); 401 case -1724546052: /*description*/ return new Property("description", "string", "The description of this Services", 0, 1, description); 402 case -512224047: /*usageRequirements*/ return new Property("usageRequirements", "string", "Human-friendly description of any preconditions for the use of this CDS Services", 0, 1, usageRequirements); 403 case -1288666633: /*prefetch*/ return new Property("prefetch", "Base", "An object containing key/value pairs of FHIR queries that this Services is requesting the CDS Client to perform and provide on each Services call. The key is a string that describes the type of data being requested and the value is a string representing the FHIR query", 0, java.lang.Integer.MAX_VALUE, prefetchList); 404 default: return super.getNamedProperty(_hash, _name, _checkValid); 405 } 406 407 } 408 409 @Override 410 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 411 switch (hash) { 412 case 3208483: /*hook*/ return this.hook == null ? new Base[0] : new Base[] {this.hook}; // StringType 413 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 414 case 3355: /*id*/ return this.id == null ? new Base[0] : new Base[] {this.id}; // CodeType 415 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 416 case -512224047: /*usageRequirements*/ return this.usageRequirements == null ? new Base[0] : new Base[] {this.usageRequirements}; // StringType 417 case -1288666633: /*prefetch*/ return this.prefetchList == null ? new Base[0] : this.prefetchList.toArray(new Base[this.prefetchList.size()]); // CDSHooksServicesServicesPrefetchComponent 418 default: return super.getProperty(hash, name, checkValid); 419 } 420 421 } 422 423 @Override 424 public Base setProperty(int hash, String name, Base value) throws FHIRException { 425 switch (hash) { 426 case 3208483: // hook 427 this.hook = TypeConvertor.castToString(value); // StringType 428 return value; 429 case 110371416: // title 430 this.title = TypeConvertor.castToString(value); // StringType 431 return value; 432 case 3355: // id 433 this.id = TypeConvertor.castToCode(value); // CodeType 434 return value; 435 case -1724546052: // description 436 this.description = TypeConvertor.castToString(value); // StringType 437 return value; 438 case -512224047: // usageRequirements 439 this.usageRequirements = TypeConvertor.castToString(value); // StringType 440 return value; 441 case -1288666633: // prefetch 442 this.getPrefetchList().add((CDSHooksServicesServicesPrefetchComponent) value); // CDSHooksServicesServicesPrefetchComponent 443 return value; 444 default: return super.setProperty(hash, name, value); 445 } 446 447 } 448 449 @Override 450 public Base setProperty(String name, Base value) throws FHIRException { 451 if (name.equals("hook")) { 452 this.hook = TypeConvertor.castToString(value); // StringType 453 } else if (name.equals("title")) { 454 this.title = TypeConvertor.castToString(value); // StringType 455 } else if (name.equals("id")) { 456 this.id = TypeConvertor.castToCode(value); // CodeType 457 } else if (name.equals("description")) { 458 this.description = TypeConvertor.castToString(value); // StringType 459 } else if (name.equals("usageRequirements")) { 460 this.usageRequirements = TypeConvertor.castToString(value); // StringType 461 } else if (name.equals("prefetch")) { 462 this.getPrefetchList().add((CDSHooksServicesServicesPrefetchComponent) value); // CDSHooksServicesServicesPrefetchComponent 463 } else 464 return super.setProperty(name, value); 465 return value; 466 } 467 468 @Override 469 public Base makeProperty(int hash, String name) throws FHIRException { 470 switch (hash) { 471 case 3208483: return getHookElement(); 472 case 110371416: return getTitleElement(); 473 case 3355: return getIdElement(); 474 case -1724546052: return getDescriptionElement(); 475 case -512224047: return getUsageRequirementsElement(); 476 case -1288666633: /*div*/ 477 throw new Error("Unable to make an instance of the abstract property 'prefetch'"); 478 default: return super.makeProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case 3208483: /*hook*/ return new String[] {"string"}; 487 case 110371416: /*title*/ return new String[] {"string"}; 488 case 3355: /*id*/ return new String[] {"code"}; 489 case -1724546052: /*description*/ return new String[] {"string"}; 490 case -512224047: /*usageRequirements*/ return new String[] {"string"}; 491 case -1288666633: /*prefetch*/ return new String[] {"Base"}; 492 default: return super.getTypesForProperty(hash, name); 493 } 494 495 } 496 497 @Override 498 public Base addChild(String name) throws FHIRException { 499 if (name.equals("hook")) { 500 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.hook"); 501 } 502 else if (name.equals("title")) { 503 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.title"); 504 } 505 else if (name.equals("id")) { 506 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.id"); 507 } 508 else if (name.equals("description")) { 509 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.description"); 510 } 511 else if (name.equals("usageRequirements")) { 512 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.usageRequirements"); 513 } 514 else if (name.equals("prefetch")) { 515 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksServices.services.prefetch"); 516 } 517 else 518 return super.addChild(name); 519 } 520 521 public CDSHooksServicesServicesComponent copy() { 522 CDSHooksServicesServicesComponent dst = new CDSHooksServicesServicesComponent(); 523 copyValues(dst); 524 return dst; 525 } 526 527 public void copyValues(CDSHooksServicesServicesComponent dst) { 528 super.copyValues(dst); 529 dst.hook = hook == null ? null : hook.copy(); 530 dst.title = title == null ? null : title.copy(); 531 dst.id = id == null ? null : id.copy(); 532 dst.description = description == null ? null : description.copy(); 533 dst.usageRequirements = usageRequirements == null ? null : usageRequirements.copy(); 534 if (prefetchList != null) { 535 dst.prefetchList = new ArrayList<CDSHooksServicesServicesPrefetchComponent>(); 536 for (CDSHooksServicesServicesPrefetchComponent i : prefetchList) 537 dst.prefetchList.add(i.copy()); 538 }; 539 } 540 541 @Override 542 public boolean equalsDeep(Base other_) { 543 if (!super.equalsDeep(other_)) 544 return false; 545 if (!(other_ instanceof CDSHooksServicesServicesComponent)) 546 return false; 547 CDSHooksServicesServicesComponent o = (CDSHooksServicesServicesComponent) other_; 548 return compareDeep(hook, o.hook, true) && compareDeep(title, o.title, true) && compareDeep(id, o.id, true) 549 && compareDeep(description, o.description, true) && compareDeep(usageRequirements, o.usageRequirements, true) 550 && compareDeep(prefetchList, o.prefetchList, true); 551 } 552 553 @Override 554 public boolean equalsShallow(Base other_) { 555 if (!super.equalsShallow(other_)) 556 return false; 557 if (!(other_ instanceof CDSHooksServicesServicesComponent)) 558 return false; 559 CDSHooksServicesServicesComponent o = (CDSHooksServicesServicesComponent) other_; 560 return compareValues(hook, o.hook, true) && compareValues(title, o.title, true) && compareValues(id, o.id, true) 561 && compareValues(description, o.description, true) && compareValues(usageRequirements, o.usageRequirements, true) 562 ; 563 } 564 565 public boolean isEmpty() { 566 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(hook, title, id, description 567 , usageRequirements, prefetchList); 568 } 569 570 public String fhirType() { 571 return "CDSHooksServices.services"; 572 573 } 574 575 } 576 577 @Block() 578 public static class CDSHooksServicesServicesPrefetchComponent extends LogicalBase { 579 /** 580 * Key of FHIR query - name for client to use when sending to Services 581 */ 582 @Child(name = "key", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="Key of FHIR query - name for client to use when sending to Services", formalDefinition="Key of FHIR query - name for client to use when sending to Services" ) 584 protected CodeType key; 585 586 /** 587 * Value of FHIR query - FHIR Query for client to perform 588 */ 589 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="Value of FHIR query - FHIR Query for client to perform", formalDefinition="Value of FHIR query - FHIR Query for client to perform" ) 591 protected StringType value; 592 593 private static final long serialVersionUID = -1496585526L; 594 595 /** 596 * Constructor 597 */ 598 public CDSHooksServicesServicesPrefetchComponent() { 599 super(); 600 } 601 602 /** 603 * Constructor 604 */ 605 public CDSHooksServicesServicesPrefetchComponent(String key, String value) { 606 super(); 607 this.setKey(key); 608 this.setValue(value); 609 } 610 611 /** 612 * @return {@link #key} (Key of FHIR query - name for client to use when sending to Services). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 613 */ 614 public CodeType getKeyElement() { 615 if (this.key == null) 616 if (Configuration.errorOnAutoCreate()) 617 throw new Error("Attempt to auto-create CDSHooksServicesServicesPrefetchComponent.key"); 618 else if (Configuration.doAutoCreate()) 619 this.key = new CodeType(); // bb 620 return this.key; 621 } 622 623 public boolean hasKeyElement() { 624 return this.key != null && !this.key.isEmpty(); 625 } 626 627 public boolean hasKey() { 628 return this.key != null && !this.key.isEmpty(); 629 } 630 631 /** 632 * @param value {@link #key} (Key of FHIR query - name for client to use when sending to Services). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 633 */ 634 public CDSHooksServicesServicesPrefetchComponent setKeyElement(CodeType value) { 635 this.key = value; 636 return this; 637 } 638 639 /** 640 * @return Key of FHIR query - name for client to use when sending to Services 641 */ 642 public String getKey() { 643 return this.key == null ? null : this.key.getValue(); 644 } 645 646 /** 647 * @param value Key of FHIR query - name for client to use when sending to Services 648 */ 649 public CDSHooksServicesServicesPrefetchComponent setKey(String value) { 650 if (this.key == null) 651 this.key = new CodeType(); 652 this.key.setValue(value); 653 return this; 654 } 655 656 /** 657 * @return {@link #value} (Value of FHIR query - FHIR Query for client to perform). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 658 */ 659 public StringType getValueElement() { 660 if (this.value == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create CDSHooksServicesServicesPrefetchComponent.value"); 663 else if (Configuration.doAutoCreate()) 664 this.value = new StringType(); // bb 665 return this.value; 666 } 667 668 public boolean hasValueElement() { 669 return this.value != null && !this.value.isEmpty(); 670 } 671 672 public boolean hasValue() { 673 return this.value != null && !this.value.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #value} (Value of FHIR query - FHIR Query for client to perform). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 678 */ 679 public CDSHooksServicesServicesPrefetchComponent setValueElement(StringType value) { 680 this.value = value; 681 return this; 682 } 683 684 /** 685 * @return Value of FHIR query - FHIR Query for client to perform 686 */ 687 public String getValue() { 688 return this.value == null ? null : this.value.getValue(); 689 } 690 691 /** 692 * @param value Value of FHIR query - FHIR Query for client to perform 693 */ 694 public CDSHooksServicesServicesPrefetchComponent setValue(String value) { 695 if (this.value == null) 696 this.value = new StringType(); 697 this.value.setValue(value); 698 return this; 699 } 700 701 protected void listChildren(List<Property> children) { 702 super.listChildren(children); 703 children.add(new Property("key", "code", "Key of FHIR query - name for client to use when sending to Services", 0, 1, key)); 704 children.add(new Property("value", "string", "Value of FHIR query - FHIR Query for client to perform", 0, 1, value)); 705 } 706 707 @Override 708 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 709 switch (_hash) { 710 case 106079: /*key*/ return new Property("key", "code", "Key of FHIR query - name for client to use when sending to Services", 0, 1, key); 711 case 111972721: /*value*/ return new Property("value", "string", "Value of FHIR query - FHIR Query for client to perform", 0, 1, value); 712 default: return super.getNamedProperty(_hash, _name, _checkValid); 713 } 714 715 } 716 717 @Override 718 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 719 switch (hash) { 720 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // CodeType 721 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 722 default: return super.getProperty(hash, name, checkValid); 723 } 724 725 } 726 727 @Override 728 public Base setProperty(int hash, String name, Base value) throws FHIRException { 729 switch (hash) { 730 case 106079: // key 731 this.key = TypeConvertor.castToCode(value); // CodeType 732 return value; 733 case 111972721: // value 734 this.value = TypeConvertor.castToString(value); // StringType 735 return value; 736 default: return super.setProperty(hash, name, value); 737 } 738 739 } 740 741 @Override 742 public Base setProperty(String name, Base value) throws FHIRException { 743 if (name.equals("key")) { 744 this.key = TypeConvertor.castToCode(value); // CodeType 745 } else if (name.equals("value")) { 746 this.value = TypeConvertor.castToString(value); // StringType 747 } else 748 return super.setProperty(name, value); 749 return value; 750 } 751 752 @Override 753 public Base makeProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case 106079: return getKeyElement(); 756 case 111972721: return getValueElement(); 757 default: return super.makeProperty(hash, name); 758 } 759 760 } 761 762 @Override 763 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 764 switch (hash) { 765 case 106079: /*key*/ return new String[] {"code"}; 766 case 111972721: /*value*/ return new String[] {"string"}; 767 default: return super.getTypesForProperty(hash, name); 768 } 769 770 } 771 772 @Override 773 public Base addChild(String name) throws FHIRException { 774 if (name.equals("key")) { 775 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.prefetch.key"); 776 } 777 else if (name.equals("value")) { 778 throw new FHIRException("Cannot call addChild on a singleton property CDSHooksServices.services.prefetch.value"); 779 } 780 else 781 return super.addChild(name); 782 } 783 784 public CDSHooksServicesServicesPrefetchComponent copy() { 785 CDSHooksServicesServicesPrefetchComponent dst = new CDSHooksServicesServicesPrefetchComponent(); 786 copyValues(dst); 787 return dst; 788 } 789 790 public void copyValues(CDSHooksServicesServicesPrefetchComponent dst) { 791 super.copyValues(dst); 792 dst.key = key == null ? null : key.copy(); 793 dst.value = value == null ? null : value.copy(); 794 } 795 796 @Override 797 public boolean equalsDeep(Base other_) { 798 if (!super.equalsDeep(other_)) 799 return false; 800 if (!(other_ instanceof CDSHooksServicesServicesPrefetchComponent)) 801 return false; 802 CDSHooksServicesServicesPrefetchComponent o = (CDSHooksServicesServicesPrefetchComponent) other_; 803 return compareDeep(key, o.key, true) && compareDeep(value, o.value, true); 804 } 805 806 @Override 807 public boolean equalsShallow(Base other_) { 808 if (!super.equalsShallow(other_)) 809 return false; 810 if (!(other_ instanceof CDSHooksServicesServicesPrefetchComponent)) 811 return false; 812 CDSHooksServicesServicesPrefetchComponent o = (CDSHooksServicesServicesPrefetchComponent) other_; 813 return compareValues(key, o.key, true) && compareValues(value, o.value, true); 814 } 815 816 public boolean isEmpty() { 817 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, value); 818 } 819 820 public String fhirType() { 821 return "CDSHooksServices.services.prefetch"; 822 823 } 824 825 } 826 827 /** 828 * A list of CDS services 829 */ 830 @Child(name = "services", type = {CDSHooksElement.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 831 @Description(shortDefinition="A list of CDS services", formalDefinition="A list of CDS services" ) 832 protected List<CDSHooksServicesServicesComponent> servicesList; 833 834 private static final long serialVersionUID = 721056483L; 835 836 /** 837 * Constructor 838 */ 839 public CDSHooksServices() { 840 super(); 841 } 842 843 /** 844 * @return {@link #services} (A list of CDS services) 845 */ 846 public List<CDSHooksServicesServicesComponent> getServicesList() { 847 if (this.servicesList == null) 848 this.servicesList = new ArrayList<CDSHooksServicesServicesComponent>(); 849 return this.servicesList; 850 } 851 852 /** 853 * @return Returns a reference to <code>this</code> for easy method chaining 854 */ 855 public CDSHooksServices setServicesList(List<CDSHooksServicesServicesComponent> theServices) { 856 this.servicesList = theServices; 857 return this; 858 } 859 860 public boolean hasServices() { 861 if (this.servicesList == null) 862 return false; 863 for (CDSHooksServicesServicesComponent item : this.servicesList) 864 if (!item.isEmpty()) 865 return true; 866 return false; 867 } 868 869 public CDSHooksServicesServicesComponent addServices() { //3a 870 CDSHooksServicesServicesComponent t = new CDSHooksServicesServicesComponent(); 871 if (this.servicesList == null) 872 this.servicesList = new ArrayList<CDSHooksServicesServicesComponent>(); 873 this.servicesList.add(t); 874 return t; 875 } 876 877 public CDSHooksServices addServices(CDSHooksServicesServicesComponent t) { //3b 878 if (t == null) 879 return this; 880 if (this.servicesList == null) 881 this.servicesList = new ArrayList<CDSHooksServicesServicesComponent>(); 882 this.servicesList.add(t); 883 return this; 884 } 885 886 /** 887 * @return The first repetition of repeating field {@link #services}, creating it if it does not already exist {3} 888 */ 889 public CDSHooksServicesServicesComponent getServicesFirstRep() { 890 if (getServicesList().isEmpty()) { 891 addServices(); 892 } 893 return getServicesList().get(0); 894 } 895 896 protected void listChildren(List<Property> children) { 897 super.listChildren(children); 898 children.add(new Property("services", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "A list of CDS services", 0, java.lang.Integer.MAX_VALUE, servicesList)); 899 } 900 901 @Override 902 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 903 switch (_hash) { 904 case 1379209310: /*services*/ return new Property("services", "http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement", "A list of CDS services", 0, java.lang.Integer.MAX_VALUE, servicesList); 905 default: return super.getNamedProperty(_hash, _name, _checkValid); 906 } 907 908 } 909 910 @Override 911 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 912 switch (hash) { 913 case 1379209310: /*services*/ return this.servicesList == null ? new Base[0] : this.servicesList.toArray(new Base[this.servicesList.size()]); // CDSHooksServicesServicesComponent 914 default: return super.getProperty(hash, name, checkValid); 915 } 916 917 } 918 919 @Override 920 public Base setProperty(int hash, String name, Base value) throws FHIRException { 921 switch (hash) { 922 case 1379209310: // services 923 this.getServicesList().add((CDSHooksServicesServicesComponent) value); // CDSHooksServicesServicesComponent 924 return value; 925 default: return super.setProperty(hash, name, value); 926 } 927 928 } 929 930 @Override 931 public Base setProperty(String name, Base value) throws FHIRException { 932 if (name.equals("services")) { 933 this.getServicesList().add((CDSHooksServicesServicesComponent) value); // CDSHooksServicesServicesComponent 934 } else 935 return super.setProperty(name, value); 936 return value; 937 } 938 939 @Override 940 public Base makeProperty(int hash, String name) throws FHIRException { 941 switch (hash) { 942 case 1379209310: return addServices(); 943 default: return super.makeProperty(hash, name); 944 } 945 946 } 947 948 @Override 949 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 950 switch (hash) { 951 case 1379209310: /*services*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/CDSHooksElement"}; 952 default: return super.getTypesForProperty(hash, name); 953 } 954 955 } 956 957 @Override 958 public Base addChild(String name) throws FHIRException { 959 if (name.equals("services")) { 960 throw new FHIRException("Cannot call addChild on an abstract type CDSHooksServices.services"); 961 } 962 else 963 return super.addChild(name); 964 } 965 966 public String fhirType() { 967 return "CDSHooksServices"; 968 969 } 970 971 public CDSHooksServices copy() { 972 CDSHooksServices dst = new CDSHooksServices(); 973 copyValues(dst); 974 return dst; 975 } 976 977 public void copyValues(CDSHooksServices dst) { 978 super.copyValues(dst); 979 if (servicesList != null) { 980 dst.servicesList = new ArrayList<CDSHooksServicesServicesComponent>(); 981 for (CDSHooksServicesServicesComponent i : servicesList) 982 dst.servicesList.add(i.copy()); 983 }; 984 } 985 986 protected CDSHooksServices typedCopy() { 987 return copy(); 988 } 989 990 @Override 991 public boolean equalsDeep(Base other_) { 992 if (!super.equalsDeep(other_)) 993 return false; 994 if (!(other_ instanceof CDSHooksServices)) 995 return false; 996 CDSHooksServices o = (CDSHooksServices) other_; 997 return compareDeep(servicesList, o.servicesList, true); 998 } 999 1000 @Override 1001 public boolean equalsShallow(Base other_) { 1002 if (!super.equalsShallow(other_)) 1003 return false; 1004 if (!(other_ instanceof CDSHooksServices)) 1005 return false; 1006 CDSHooksServices o = (CDSHooksServices) other_; 1007 return true; 1008 } 1009 1010 public boolean isEmpty() { 1011 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(servicesList); 1012 } 1013 1014 1015} 1016