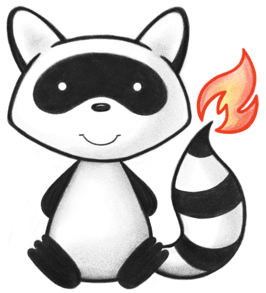
001package org.hl7.fhir.r5.tools; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.tools.Enumerations.*; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.*; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * A standard format for test cases used throughout the FHIR ecosystem 050 */ 051@DatatypeDef(name="TestCases") 052public class TestCases extends Resource implements ICompositeType { 053 054 @Block() 055 public static class TestCasesModeComponent extends LogicalBase { 056 /** 057 * The code by which the mode is identified when passed to runner 058 */ 059 @Child(name = "code", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 060 @Description(shortDefinition="The code that identifies the mode", formalDefinition="The code by which the mode is identified when passed to runner" ) 061 protected StringType code; 062 063 /** 064 * Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode 065 */ 066 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 067 @Description(shortDefinition="Description of what this mode exists / why it was defined", formalDefinition="Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode" ) 068 protected StringType description; 069 070 private static final long serialVersionUID = 1295492279L; 071 072 /** 073 * Constructor 074 */ 075 public TestCasesModeComponent() { 076 super(); 077 } 078 079 /** 080 * Constructor 081 */ 082 public TestCasesModeComponent(String code) { 083 super(); 084 this.setCode(code); 085 } 086 087 /** 088 * @return {@link #code} (The code by which the mode is identified when passed to runner). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 089 */ 090 public StringType getCodeElement() { 091 if (this.code == null) 092 if (Configuration.errorOnAutoCreate()) 093 throw new Error("Attempt to auto-create TestCasesModeComponent.code"); 094 else if (Configuration.doAutoCreate()) 095 this.code = new StringType(); // bb 096 return this.code; 097 } 098 099 public boolean hasCodeElement() { 100 return this.code != null && !this.code.isEmpty(); 101 } 102 103 public boolean hasCode() { 104 return this.code != null && !this.code.isEmpty(); 105 } 106 107 /** 108 * @param value {@link #code} (The code by which the mode is identified when passed to runner). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 109 */ 110 public TestCasesModeComponent setCodeElement(StringType value) { 111 this.code = value; 112 return this; 113 } 114 115 /** 116 * @return The code by which the mode is identified when passed to runner 117 */ 118 public String getCode() { 119 return this.code == null ? null : this.code.getValue(); 120 } 121 122 /** 123 * @param value The code by which the mode is identified when passed to runner 124 */ 125 public TestCasesModeComponent setCode(String value) { 126 if (this.code == null) 127 this.code = new StringType(); 128 this.code.setValue(value); 129 return this; 130 } 131 132 /** 133 * @return {@link #description} (Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 134 */ 135 public StringType getDescriptionElement() { 136 if (this.description == null) 137 if (Configuration.errorOnAutoCreate()) 138 throw new Error("Attempt to auto-create TestCasesModeComponent.description"); 139 else if (Configuration.doAutoCreate()) 140 this.description = new StringType(); // bb 141 return this.description; 142 } 143 144 public boolean hasDescriptionElement() { 145 return this.description != null && !this.description.isEmpty(); 146 } 147 148 public boolean hasDescription() { 149 return this.description != null && !this.description.isEmpty(); 150 } 151 152 /** 153 * @param value {@link #description} (Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 154 */ 155 public TestCasesModeComponent setDescriptionElement(StringType value) { 156 this.description = value; 157 return this; 158 } 159 160 /** 161 * @return Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode 162 */ 163 public String getDescription() { 164 return this.description == null ? null : this.description.getValue(); 165 } 166 167 /** 168 * @param value Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode 169 */ 170 public TestCasesModeComponent setDescription(String value) { 171 if (Utilities.noString(value)) 172 this.description = null; 173 else { 174 if (this.description == null) 175 this.description = new StringType(); 176 this.description.setValue(value); 177 } 178 return this; 179 } 180 181 protected void listChildren(List<Property> children) { 182 super.listChildren(children); 183 children.add(new Property("code", "string", "The code by which the mode is identified when passed to runner", 0, 1, code)); 184 children.add(new Property("description", "string", "Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode", 0, 1, description)); 185 } 186 187 @Override 188 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 189 switch (_hash) { 190 case 3059181: /*code*/ return new Property("code", "string", "The code by which the mode is identified when passed to runner", 0, 1, code); 191 case -1724546052: /*description*/ return new Property("description", "string", "Description of what this mode does / why it was defined. This should explain to a tester when they should use the mode", 0, 1, description); 192 default: return super.getNamedProperty(_hash, _name, _checkValid); 193 } 194 195 } 196 197 @Override 198 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 199 switch (hash) { 200 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // StringType 201 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 202 default: return super.getProperty(hash, name, checkValid); 203 } 204 205 } 206 207 @Override 208 public Base setProperty(int hash, String name, Base value) throws FHIRException { 209 switch (hash) { 210 case 3059181: // code 211 this.code = TypeConvertor.castToString(value); // StringType 212 return value; 213 case -1724546052: // description 214 this.description = TypeConvertor.castToString(value); // StringType 215 return value; 216 default: return super.setProperty(hash, name, value); 217 } 218 219 } 220 221 @Override 222 public Base setProperty(String name, Base value) throws FHIRException { 223 if (name.equals("code")) { 224 this.code = TypeConvertor.castToString(value); // StringType 225 } else if (name.equals("description")) { 226 this.description = TypeConvertor.castToString(value); // StringType 227 } else 228 return super.setProperty(name, value); 229 return value; 230 } 231 232 @Override 233 public Base makeProperty(int hash, String name) throws FHIRException { 234 switch (hash) { 235 case 3059181: return getCodeElement(); 236 case -1724546052: return getDescriptionElement(); 237 default: return super.makeProperty(hash, name); 238 } 239 240 } 241 242 @Override 243 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 244 switch (hash) { 245 case 3059181: /*code*/ return new String[] {"string"}; 246 case -1724546052: /*description*/ return new String[] {"string"}; 247 default: return super.getTypesForProperty(hash, name); 248 } 249 250 } 251 252 @Override 253 public Base addChild(String name) throws FHIRException { 254 if (name.equals("code")) { 255 throw new FHIRException("Cannot call addChild on a singleton property TestCases.mode.code"); 256 } 257 else if (name.equals("description")) { 258 throw new FHIRException("Cannot call addChild on a singleton property TestCases.mode.description"); 259 } 260 else 261 return super.addChild(name); 262 } 263 264 public TestCasesModeComponent copy() { 265 TestCasesModeComponent dst = new TestCasesModeComponent(); 266 copyValues(dst); 267 return dst; 268 } 269 270 public void copyValues(TestCasesModeComponent dst) { 271 super.copyValues(dst); 272 dst.code = code == null ? null : code.copy(); 273 dst.description = description == null ? null : description.copy(); 274 } 275 276 @Override 277 public boolean equalsDeep(Base other_) { 278 if (!super.equalsDeep(other_)) 279 return false; 280 if (!(other_ instanceof TestCasesModeComponent)) 281 return false; 282 TestCasesModeComponent o = (TestCasesModeComponent) other_; 283 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true); 284 } 285 286 @Override 287 public boolean equalsShallow(Base other_) { 288 if (!super.equalsShallow(other_)) 289 return false; 290 if (!(other_ instanceof TestCasesModeComponent)) 291 return false; 292 TestCasesModeComponent o = (TestCasesModeComponent) other_; 293 return compareValues(code, o.code, true) && compareValues(description, o.description, true); 294 } 295 296 public boolean isEmpty() { 297 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description); 298 } 299 300 public String fhirType() { 301 return "TestCases.mode"; 302 303 } 304 305 } 306 307 @Block() 308 public static class TestCasesSuiteComponent extends LogicalBase { 309 /** 310 * The name by which this suite is known by in the test system. The name must be unique in the amongst the suites 311 */ 312 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 313 @Description(shortDefinition="The name of this suite - unique in the TestCases resource", formalDefinition="The name by which this suite is known by in the test system. The name must be unique in the amongst the suites" ) 314 protected StringType name; 315 316 /** 317 * Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run 318 */ 319 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 320 @Description(shortDefinition="Description of what this suite does / why it was defined", formalDefinition="Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run" ) 321 protected StringType description; 322 323 /** 324 * If this mode is not passed to the runner, then this suite will not be run 325 */ 326 @Child(name = "mode", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 327 @Description(shortDefinition="mode required to run this suite", formalDefinition="If this mode is not passed to the runner, then this suite will not be run" ) 328 protected CodeType mode; 329 330 /** 331 * The resources used in the tests in this suite. How exactly they are used depends on the definition of the runner 332 */ 333 @Child(name = "resource", type = {Base.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 334 @Description(shortDefinition="Resources used in the tests in this suite", formalDefinition="The resources used in the tests in this suite. How exactly they are used depends on the definition of the runner" ) 335 protected List<TestCasesSuiteResourceComponent> resourceList; 336 337 /** 338 * A parameter passed to the runner when executing tests. Which parameters are valid, and how exactly the parameter is used are used depends on the definition of the runner 339 */ 340 @Child(name = "parameter", type = {Base.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 341 @Description(shortDefinition="Parameter passed to the runner", formalDefinition="A parameter passed to the runner when executing tests. Which parameters are valid, and how exactly the parameter is used are used depends on the definition of the runner" ) 342 protected List<TestCasesSuiteParameterComponent> parameterList; 343 344 /** 345 * An actual test in the test suite 346 */ 347 @Child(name = "test", type = {Base.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 348 @Description(shortDefinition="A test in the test suite", formalDefinition="An actual test in the test suite" ) 349 protected List<TestCasesSuiteTestComponent> testList; 350 351 private static final long serialVersionUID = 622063903L; 352 353 /** 354 * Constructor 355 */ 356 public TestCasesSuiteComponent() { 357 super(); 358 } 359 360 /** 361 * Constructor 362 */ 363 public TestCasesSuiteComponent(String name) { 364 super(); 365 this.setName(name); 366 } 367 368 /** 369 * @return {@link #name} (The name by which this suite is known by in the test system. The name must be unique in the amongst the suites). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 370 */ 371 public StringType getNameElement() { 372 if (this.name == null) 373 if (Configuration.errorOnAutoCreate()) 374 throw new Error("Attempt to auto-create TestCasesSuiteComponent.name"); 375 else if (Configuration.doAutoCreate()) 376 this.name = new StringType(); // bb 377 return this.name; 378 } 379 380 public boolean hasNameElement() { 381 return this.name != null && !this.name.isEmpty(); 382 } 383 384 public boolean hasName() { 385 return this.name != null && !this.name.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #name} (The name by which this suite is known by in the test system. The name must be unique in the amongst the suites). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 390 */ 391 public TestCasesSuiteComponent setNameElement(StringType value) { 392 this.name = value; 393 return this; 394 } 395 396 /** 397 * @return The name by which this suite is known by in the test system. The name must be unique in the amongst the suites 398 */ 399 public String getName() { 400 return this.name == null ? null : this.name.getValue(); 401 } 402 403 /** 404 * @param value The name by which this suite is known by in the test system. The name must be unique in the amongst the suites 405 */ 406 public TestCasesSuiteComponent setName(String value) { 407 if (this.name == null) 408 this.name = new StringType(); 409 this.name.setValue(value); 410 return this; 411 } 412 413 /** 414 * @return {@link #description} (Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 415 */ 416 public StringType getDescriptionElement() { 417 if (this.description == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create TestCasesSuiteComponent.description"); 420 else if (Configuration.doAutoCreate()) 421 this.description = new StringType(); // bb 422 return this.description; 423 } 424 425 public boolean hasDescriptionElement() { 426 return this.description != null && !this.description.isEmpty(); 427 } 428 429 public boolean hasDescription() { 430 return this.description != null && !this.description.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #description} (Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 435 */ 436 public TestCasesSuiteComponent setDescriptionElement(StringType value) { 437 this.description = value; 438 return this; 439 } 440 441 /** 442 * @return Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run 443 */ 444 public String getDescription() { 445 return this.description == null ? null : this.description.getValue(); 446 } 447 448 /** 449 * @param value Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run 450 */ 451 public TestCasesSuiteComponent setDescription(String value) { 452 if (Utilities.noString(value)) 453 this.description = null; 454 else { 455 if (this.description == null) 456 this.description = new StringType(); 457 this.description.setValue(value); 458 } 459 return this; 460 } 461 462 /** 463 * @return {@link #mode} (If this mode is not passed to the runner, then this suite will not be run). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 464 */ 465 public CodeType getModeElement() { 466 if (this.mode == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create TestCasesSuiteComponent.mode"); 469 else if (Configuration.doAutoCreate()) 470 this.mode = new CodeType(); // bb 471 return this.mode; 472 } 473 474 public boolean hasModeElement() { 475 return this.mode != null && !this.mode.isEmpty(); 476 } 477 478 public boolean hasMode() { 479 return this.mode != null && !this.mode.isEmpty(); 480 } 481 482 /** 483 * @param value {@link #mode} (If this mode is not passed to the runner, then this suite will not be run). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 484 */ 485 public TestCasesSuiteComponent setModeElement(CodeType value) { 486 this.mode = value; 487 return this; 488 } 489 490 /** 491 * @return If this mode is not passed to the runner, then this suite will not be run 492 */ 493 public String getMode() { 494 return this.mode == null ? null : this.mode.getValue(); 495 } 496 497 /** 498 * @param value If this mode is not passed to the runner, then this suite will not be run 499 */ 500 public TestCasesSuiteComponent setMode(String value) { 501 if (Utilities.noString(value)) 502 this.mode = null; 503 else { 504 if (this.mode == null) 505 this.mode = new CodeType(); 506 this.mode.setValue(value); 507 } 508 return this; 509 } 510 511 /** 512 * @return {@link #resource} (The resources used in the tests in this suite. How exactly they are used depends on the definition of the runner) 513 */ 514 public List<TestCasesSuiteResourceComponent> getResourceList() { 515 if (this.resourceList == null) 516 this.resourceList = new ArrayList<TestCasesSuiteResourceComponent>(); 517 return this.resourceList; 518 } 519 520 /** 521 * @return Returns a reference to <code>this</code> for easy method chaining 522 */ 523 public TestCasesSuiteComponent setResourceList(List<TestCasesSuiteResourceComponent> theResource) { 524 this.resourceList = theResource; 525 return this; 526 } 527 528 public boolean hasResource() { 529 if (this.resourceList == null) 530 return false; 531 for (TestCasesSuiteResourceComponent item : this.resourceList) 532 if (!item.isEmpty()) 533 return true; 534 return false; 535 } 536 537 public TestCasesSuiteComponent addResource(TestCasesSuiteResourceComponent t) { //3b 538 if (t == null) 539 return this; 540 if (this.resourceList == null) 541 this.resourceList = new ArrayList<TestCasesSuiteResourceComponent>(); 542 this.resourceList.add(t); 543 return this; 544 } 545 546 /** 547 * @return {@link #parameter} (A parameter passed to the runner when executing tests. Which parameters are valid, and how exactly the parameter is used are used depends on the definition of the runner) 548 */ 549 public List<TestCasesSuiteParameterComponent> getParameterList() { 550 if (this.parameterList == null) 551 this.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 552 return this.parameterList; 553 } 554 555 /** 556 * @return Returns a reference to <code>this</code> for easy method chaining 557 */ 558 public TestCasesSuiteComponent setParameterList(List<TestCasesSuiteParameterComponent> theParameter) { 559 this.parameterList = theParameter; 560 return this; 561 } 562 563 public boolean hasParameter() { 564 if (this.parameterList == null) 565 return false; 566 for (TestCasesSuiteParameterComponent item : this.parameterList) 567 if (!item.isEmpty()) 568 return true; 569 return false; 570 } 571 572 public TestCasesSuiteComponent addParameter(TestCasesSuiteParameterComponent t) { //3b 573 if (t == null) 574 return this; 575 if (this.parameterList == null) 576 this.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 577 this.parameterList.add(t); 578 return this; 579 } 580 581 /** 582 * @return {@link #test} (An actual test in the test suite) 583 */ 584 public List<TestCasesSuiteTestComponent> getTestList() { 585 if (this.testList == null) 586 this.testList = new ArrayList<TestCasesSuiteTestComponent>(); 587 return this.testList; 588 } 589 590 /** 591 * @return Returns a reference to <code>this</code> for easy method chaining 592 */ 593 public TestCasesSuiteComponent setTestList(List<TestCasesSuiteTestComponent> theTest) { 594 this.testList = theTest; 595 return this; 596 } 597 598 public boolean hasTest() { 599 if (this.testList == null) 600 return false; 601 for (TestCasesSuiteTestComponent item : this.testList) 602 if (!item.isEmpty()) 603 return true; 604 return false; 605 } 606 607 public TestCasesSuiteComponent addTest(TestCasesSuiteTestComponent t) { //3b 608 if (t == null) 609 return this; 610 if (this.testList == null) 611 this.testList = new ArrayList<TestCasesSuiteTestComponent>(); 612 this.testList.add(t); 613 return this; 614 } 615 616 protected void listChildren(List<Property> children) { 617 super.listChildren(children); 618 children.add(new Property("name", "string", "The name by which this suite is known by in the test system. The name must be unique in the amongst the suites", 0, 1, name)); 619 children.add(new Property("description", "string", "Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run", 0, 1, description)); 620 children.add(new Property("mode", "code", "If this mode is not passed to the runner, then this suite will not be run", 0, 1, mode)); 621 children.add(new Property("resource", "Base", "The resources used in the tests in this suite. How exactly they are used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, resourceList)); 622 children.add(new Property("parameter", "Base", "A parameter passed to the runner when executing tests. Which parameters are valid, and how exactly the parameter is used are used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, parameterList)); 623 children.add(new Property("test", "Base", "An actual test in the test suite", 0, java.lang.Integer.MAX_VALUE, testList)); 624 } 625 626 @Override 627 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 628 switch (_hash) { 629 case 3373707: /*name*/ return new Property("name", "string", "The name by which this suite is known by in the test system. The name must be unique in the amongst the suites", 0, 1, name); 630 case -1724546052: /*description*/ return new Property("description", "string", "Description of what this suite does / why it was defined. This should explain to a tester what they should know when deciding which tests to run", 0, 1, description); 631 case 3357091: /*mode*/ return new Property("mode", "code", "If this mode is not passed to the runner, then this suite will not be run", 0, 1, mode); 632 case -341064690: /*resource*/ return new Property("resource", "Base", "The resources used in the tests in this suite. How exactly they are used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, resourceList); 633 case 1954460585: /*parameter*/ return new Property("parameter", "Base", "A parameter passed to the runner when executing tests. Which parameters are valid, and how exactly the parameter is used are used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, parameterList); 634 case 3556498: /*test*/ return new Property("test", "Base", "An actual test in the test suite", 0, java.lang.Integer.MAX_VALUE, testList); 635 default: return super.getNamedProperty(_hash, _name, _checkValid); 636 } 637 638 } 639 640 @Override 641 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 642 switch (hash) { 643 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 644 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 645 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeType 646 case -341064690: /*resource*/ return this.resourceList == null ? new Base[0] : this.resourceList.toArray(new Base[this.resourceList.size()]); // TestCasesSuiteResourceComponent 647 case 1954460585: /*parameter*/ return this.parameterList == null ? new Base[0] : this.parameterList.toArray(new Base[this.parameterList.size()]); // TestCasesSuiteParameterComponent 648 case 3556498: /*test*/ return this.testList == null ? new Base[0] : this.testList.toArray(new Base[this.testList.size()]); // TestCasesSuiteTestComponent 649 default: return super.getProperty(hash, name, checkValid); 650 } 651 652 } 653 654 @Override 655 public Base setProperty(int hash, String name, Base value) throws FHIRException { 656 switch (hash) { 657 case 3373707: // name 658 this.name = TypeConvertor.castToString(value); // StringType 659 return value; 660 case -1724546052: // description 661 this.description = TypeConvertor.castToString(value); // StringType 662 return value; 663 case 3357091: // mode 664 this.mode = TypeConvertor.castToCode(value); // CodeType 665 return value; 666 case -341064690: // resource 667 this.getResourceList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 668 return value; 669 case 1954460585: // parameter 670 this.getParameterList().add((TestCasesSuiteParameterComponent) value); // TestCasesSuiteParameterComponent 671 return value; 672 case 3556498: // test 673 this.getTestList().add((TestCasesSuiteTestComponent) value); // TestCasesSuiteTestComponent 674 return value; 675 default: return super.setProperty(hash, name, value); 676 } 677 678 } 679 680 @Override 681 public Base setProperty(String name, Base value) throws FHIRException { 682 if (name.equals("name")) { 683 this.name = TypeConvertor.castToString(value); // StringType 684 } else if (name.equals("description")) { 685 this.description = TypeConvertor.castToString(value); // StringType 686 } else if (name.equals("mode")) { 687 this.mode = TypeConvertor.castToCode(value); // CodeType 688 } else if (name.equals("resource")) { 689 this.getResourceList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 690 } else if (name.equals("parameter")) { 691 this.getParameterList().add((TestCasesSuiteParameterComponent) value); // TestCasesSuiteParameterComponent 692 } else if (name.equals("test")) { 693 this.getTestList().add((TestCasesSuiteTestComponent) value); // TestCasesSuiteTestComponent 694 } else 695 return super.setProperty(name, value); 696 return value; 697 } 698 699 @Override 700 public Base makeProperty(int hash, String name) throws FHIRException { 701 switch (hash) { 702 case 3373707: return getNameElement(); 703 case -1724546052: return getDescriptionElement(); 704 case 3357091: return getModeElement(); 705 case -341064690: /*div*/ 706 throw new Error("Unable to make an instance of the abstract property 'resource'"); 707 case 1954460585: /*div*/ 708 throw new Error("Unable to make an instance of the abstract property 'parameter'"); 709 case 3556498: /*div*/ 710 throw new Error("Unable to make an instance of the abstract property 'test'"); 711 default: return super.makeProperty(hash, name); 712 } 713 714 } 715 716 @Override 717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case 3373707: /*name*/ return new String[] {"string"}; 720 case -1724546052: /*description*/ return new String[] {"string"}; 721 case 3357091: /*mode*/ return new String[] {"code"}; 722 case -341064690: /*resource*/ return new String[] {"Base"}; 723 case 1954460585: /*parameter*/ return new String[] {"Base"}; 724 case 3556498: /*test*/ return new String[] {"Base"}; 725 default: return super.getTypesForProperty(hash, name); 726 } 727 728 } 729 730 @Override 731 public Base addChild(String name) throws FHIRException { 732 if (name.equals("name")) { 733 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.name"); 734 } 735 else if (name.equals("description")) { 736 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.description"); 737 } 738 else if (name.equals("mode")) { 739 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.mode"); 740 } 741 else if (name.equals("resource")) { 742 throw new FHIRException("Cannot call addChild on an abstract type TestCases.suite.resource"); 743 } 744 else if (name.equals("parameter")) { 745 throw new FHIRException("Cannot call addChild on an abstract type TestCases.suite.parameter"); 746 } 747 else if (name.equals("test")) { 748 throw new FHIRException("Cannot call addChild on an abstract type TestCases.suite.test"); 749 } 750 else 751 return super.addChild(name); 752 } 753 754 public TestCasesSuiteComponent copy() { 755 TestCasesSuiteComponent dst = new TestCasesSuiteComponent(); 756 copyValues(dst); 757 return dst; 758 } 759 760 public void copyValues(TestCasesSuiteComponent dst) { 761 super.copyValues(dst); 762 dst.name = name == null ? null : name.copy(); 763 dst.description = description == null ? null : description.copy(); 764 dst.mode = mode == null ? null : mode.copy(); 765 if (resourceList != null) { 766 dst.resourceList = new ArrayList<TestCasesSuiteResourceComponent>(); 767 for (TestCasesSuiteResourceComponent i : resourceList) 768 dst.resourceList.add(i.copy()); 769 }; 770 if (parameterList != null) { 771 dst.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 772 for (TestCasesSuiteParameterComponent i : parameterList) 773 dst.parameterList.add(i.copy()); 774 }; 775 if (testList != null) { 776 dst.testList = new ArrayList<TestCasesSuiteTestComponent>(); 777 for (TestCasesSuiteTestComponent i : testList) 778 dst.testList.add(i.copy()); 779 }; 780 } 781 782 @Override 783 public boolean equalsDeep(Base other_) { 784 if (!super.equalsDeep(other_)) 785 return false; 786 if (!(other_ instanceof TestCasesSuiteComponent)) 787 return false; 788 TestCasesSuiteComponent o = (TestCasesSuiteComponent) other_; 789 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(mode, o.mode, true) 790 && compareDeep(resourceList, o.resourceList, true) && compareDeep(parameterList, o.parameterList, true) 791 && compareDeep(testList, o.testList, true); 792 } 793 794 @Override 795 public boolean equalsShallow(Base other_) { 796 if (!super.equalsShallow(other_)) 797 return false; 798 if (!(other_ instanceof TestCasesSuiteComponent)) 799 return false; 800 TestCasesSuiteComponent o = (TestCasesSuiteComponent) other_; 801 return compareValues(name, o.name, true) && compareValues(description, o.description, true) && compareValues(mode, o.mode, true) 802 ; 803 } 804 805 public boolean isEmpty() { 806 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, mode 807 , resourceList, parameterList, testList); 808 } 809 810 public String fhirType() { 811 return "TestCases.suite"; 812 813 } 814 815 } 816 817 @Block() 818 public static class TestCasesSuiteResourceComponent extends LogicalBase { 819 /** 820 * A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are 821 */ 822 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 823 @Description(shortDefinition="A name for this resource (per runner definition)", formalDefinition="A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are" ) 824 protected StringType name; 825 826 /** 827 * A file containing a resource used in the tests 828 */ 829 @Child(name = "file", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 830 @Description(shortDefinition="A file containing a resource used in the tests", formalDefinition="A file containing a resource used in the tests" ) 831 protected StringType file; 832 833 /** 834 * An inline resource used in the tests. How exactly it is used depends on the definition of the runner. 835 */ 836 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=false) 837 @Description(shortDefinition="An inline resource used in the tests", formalDefinition="An inline resource used in the tests. How exactly it is used depends on the definition of the runner." ) 838 protected Resource resource; 839 840 /** 841 * If this mode is not passed to the runner, then this resource will not be used 842 */ 843 @Child(name = "mode", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 844 @Description(shortDefinition="A mode that must be true for this resource to be used", formalDefinition="If this mode is not passed to the runner, then this resource will not be used" ) 845 protected CodeType mode; 846 847 private static final long serialVersionUID = 1036724891L; 848 849 /** 850 * Constructor 851 */ 852 public TestCasesSuiteResourceComponent() { 853 super(); 854 } 855 856 /** 857 * @return {@link #name} (A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 858 */ 859 public StringType getNameElement() { 860 if (this.name == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create TestCasesSuiteResourceComponent.name"); 863 else if (Configuration.doAutoCreate()) 864 this.name = new StringType(); // bb 865 return this.name; 866 } 867 868 public boolean hasNameElement() { 869 return this.name != null && !this.name.isEmpty(); 870 } 871 872 public boolean hasName() { 873 return this.name != null && !this.name.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #name} (A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 878 */ 879 public TestCasesSuiteResourceComponent setNameElement(StringType value) { 880 this.name = value; 881 return this; 882 } 883 884 /** 885 * @return A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are 886 */ 887 public String getName() { 888 return this.name == null ? null : this.name.getValue(); 889 } 890 891 /** 892 * @param value A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are 893 */ 894 public TestCasesSuiteResourceComponent setName(String value) { 895 if (Utilities.noString(value)) 896 this.name = null; 897 else { 898 if (this.name == null) 899 this.name = new StringType(); 900 this.name.setValue(value); 901 } 902 return this; 903 } 904 905 /** 906 * @return {@link #file} (A file containing a resource used in the tests). This is the underlying object with id, value and extensions. The accessor "getFile" gives direct access to the value 907 */ 908 public StringType getFileElement() { 909 if (this.file == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create TestCasesSuiteResourceComponent.file"); 912 else if (Configuration.doAutoCreate()) 913 this.file = new StringType(); // bb 914 return this.file; 915 } 916 917 public boolean hasFileElement() { 918 return this.file != null && !this.file.isEmpty(); 919 } 920 921 public boolean hasFile() { 922 return this.file != null && !this.file.isEmpty(); 923 } 924 925 /** 926 * @param value {@link #file} (A file containing a resource used in the tests). This is the underlying object with id, value and extensions. The accessor "getFile" gives direct access to the value 927 */ 928 public TestCasesSuiteResourceComponent setFileElement(StringType value) { 929 this.file = value; 930 return this; 931 } 932 933 /** 934 * @return A file containing a resource used in the tests 935 */ 936 public String getFile() { 937 return this.file == null ? null : this.file.getValue(); 938 } 939 940 /** 941 * @param value A file containing a resource used in the tests 942 */ 943 public TestCasesSuiteResourceComponent setFile(String value) { 944 if (Utilities.noString(value)) 945 this.file = null; 946 else { 947 if (this.file == null) 948 this.file = new StringType(); 949 this.file.setValue(value); 950 } 951 return this; 952 } 953 954 /** 955 * @return {@link #resource} (An inline resource used in the tests. How exactly it is used depends on the definition of the runner.) 956 */ 957 public Resource getResource() { 958 return this.resource; 959 } 960 961 public boolean hasResource() { 962 return this.resource != null && !this.resource.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #resource} (An inline resource used in the tests. How exactly it is used depends on the definition of the runner.) 967 */ 968 public TestCasesSuiteResourceComponent setResource(Resource value) { 969 this.resource = value; 970 return this; 971 } 972 973 /** 974 * @return {@link #mode} (If this mode is not passed to the runner, then this resource will not be used). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 975 */ 976 public CodeType getModeElement() { 977 if (this.mode == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create TestCasesSuiteResourceComponent.mode"); 980 else if (Configuration.doAutoCreate()) 981 this.mode = new CodeType(); // bb 982 return this.mode; 983 } 984 985 public boolean hasModeElement() { 986 return this.mode != null && !this.mode.isEmpty(); 987 } 988 989 public boolean hasMode() { 990 return this.mode != null && !this.mode.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #mode} (If this mode is not passed to the runner, then this resource will not be used). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 995 */ 996 public TestCasesSuiteResourceComponent setModeElement(CodeType value) { 997 this.mode = value; 998 return this; 999 } 1000 1001 /** 1002 * @return If this mode is not passed to the runner, then this resource will not be used 1003 */ 1004 public String getMode() { 1005 return this.mode == null ? null : this.mode.getValue(); 1006 } 1007 1008 /** 1009 * @param value If this mode is not passed to the runner, then this resource will not be used 1010 */ 1011 public TestCasesSuiteResourceComponent setMode(String value) { 1012 if (Utilities.noString(value)) 1013 this.mode = null; 1014 else { 1015 if (this.mode == null) 1016 this.mode = new CodeType(); 1017 this.mode.setValue(value); 1018 } 1019 return this; 1020 } 1021 1022 protected void listChildren(List<Property> children) { 1023 super.listChildren(children); 1024 children.add(new Property("name", "string", "A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are", 0, 1, name)); 1025 children.add(new Property("file", "string", "A file containing a resource used in the tests", 0, 1, file)); 1026 children.add(new Property("resource", "Resource", "An inline resource used in the tests. How exactly it is used depends on the definition of the runner.", 0, 1, resource)); 1027 children.add(new Property("mode", "code", "If this mode is not passed to the runner, then this resource will not be used", 0, 1, mode)); 1028 } 1029 1030 @Override 1031 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1032 switch (_hash) { 1033 case 3373707: /*name*/ return new Property("name", "string", "A name that identifies this resource. The runner definition defines whether there must be a name, and what names there are", 0, 1, name); 1034 case 3143036: /*file*/ return new Property("file", "string", "A file containing a resource used in the tests", 0, 1, file); 1035 case -341064690: /*resource*/ return new Property("resource", "Resource", "An inline resource used in the tests. How exactly it is used depends on the definition of the runner.", 0, 1, resource); 1036 case 3357091: /*mode*/ return new Property("mode", "code", "If this mode is not passed to the runner, then this resource will not be used", 0, 1, mode); 1037 default: return super.getNamedProperty(_hash, _name, _checkValid); 1038 } 1039 1040 } 1041 1042 @Override 1043 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1044 switch (hash) { 1045 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1046 case 3143036: /*file*/ return this.file == null ? new Base[0] : new Base[] {this.file}; // StringType 1047 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 1048 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeType 1049 default: return super.getProperty(hash, name, checkValid); 1050 } 1051 1052 } 1053 1054 @Override 1055 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1056 switch (hash) { 1057 case 3373707: // name 1058 this.name = TypeConvertor.castToString(value); // StringType 1059 return value; 1060 case 3143036: // file 1061 this.file = TypeConvertor.castToString(value); // StringType 1062 return value; 1063 case -341064690: // resource 1064 this.resource = (Resource) value; // Resource 1065 return value; 1066 case 3357091: // mode 1067 this.mode = TypeConvertor.castToCode(value); // CodeType 1068 return value; 1069 default: return super.setProperty(hash, name, value); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base setProperty(String name, Base value) throws FHIRException { 1076 if (name.equals("name")) { 1077 this.name = TypeConvertor.castToString(value); // StringType 1078 } else if (name.equals("file")) { 1079 this.file = TypeConvertor.castToString(value); // StringType 1080 } else if (name.equals("resource")) { 1081 this.resource = (Resource) value; // Resource 1082 } else if (name.equals("mode")) { 1083 this.mode = TypeConvertor.castToCode(value); // CodeType 1084 } else 1085 return super.setProperty(name, value); 1086 return value; 1087 } 1088 1089 @Override 1090 public Base makeProperty(int hash, String name) throws FHIRException { 1091 switch (hash) { 1092 case 3373707: return getNameElement(); 1093 case 3143036: return getFileElement(); 1094 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 1095 case 3357091: return getModeElement(); 1096 default: return super.makeProperty(hash, name); 1097 } 1098 1099 } 1100 1101 @Override 1102 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1103 switch (hash) { 1104 case 3373707: /*name*/ return new String[] {"string"}; 1105 case 3143036: /*file*/ return new String[] {"string"}; 1106 case -341064690: /*resource*/ return new String[] {"Resource"}; 1107 case 3357091: /*mode*/ return new String[] {"code"}; 1108 default: return super.getTypesForProperty(hash, name); 1109 } 1110 1111 } 1112 1113 @Override 1114 public Base addChild(String name) throws FHIRException { 1115 if (name.equals("name")) { 1116 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.resource.name"); 1117 } 1118 else if (name.equals("file")) { 1119 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.resource.file"); 1120 } 1121 else if (name.equals("resource")) { 1122 throw new FHIRException("Cannot call addChild on an abstract type TestCases.suite.resource.resource"); 1123 } 1124 else if (name.equals("mode")) { 1125 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.resource.mode"); 1126 } 1127 else 1128 return super.addChild(name); 1129 } 1130 1131 public TestCasesSuiteResourceComponent copy() { 1132 TestCasesSuiteResourceComponent dst = new TestCasesSuiteResourceComponent(); 1133 copyValues(dst); 1134 return dst; 1135 } 1136 1137 public void copyValues(TestCasesSuiteResourceComponent dst) { 1138 super.copyValues(dst); 1139 dst.name = name == null ? null : name.copy(); 1140 dst.file = file == null ? null : file.copy(); 1141 dst.resource = resource == null ? null : resource.copy(); 1142 dst.mode = mode == null ? null : mode.copy(); 1143 } 1144 1145 @Override 1146 public boolean equalsDeep(Base other_) { 1147 if (!super.equalsDeep(other_)) 1148 return false; 1149 if (!(other_ instanceof TestCasesSuiteResourceComponent)) 1150 return false; 1151 TestCasesSuiteResourceComponent o = (TestCasesSuiteResourceComponent) other_; 1152 return compareDeep(name, o.name, true) && compareDeep(file, o.file, true) && compareDeep(resource, o.resource, true) 1153 && compareDeep(mode, o.mode, true); 1154 } 1155 1156 @Override 1157 public boolean equalsShallow(Base other_) { 1158 if (!super.equalsShallow(other_)) 1159 return false; 1160 if (!(other_ instanceof TestCasesSuiteResourceComponent)) 1161 return false; 1162 TestCasesSuiteResourceComponent o = (TestCasesSuiteResourceComponent) other_; 1163 return compareValues(name, o.name, true) && compareValues(file, o.file, true) && compareValues(mode, o.mode, true) 1164 ; 1165 } 1166 1167 public boolean isEmpty() { 1168 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, file, resource, mode 1169 ); 1170 } 1171 1172 public String fhirType() { 1173 return "TestCases.suite.resource"; 1174 1175 } 1176 1177 } 1178 1179 @Block() 1180 public static class TestCasesSuiteParameterComponent extends LogicalBase { 1181 /** 1182 * Name of parameter 1183 */ 1184 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1185 @Description(shortDefinition="Name of parameter", formalDefinition="Name of parameter" ) 1186 protected StringType name; 1187 1188 /** 1189 * The value of the parameter 1190 */ 1191 @Child(name = "value", type = {StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, DateTimeType.class, UriType.class, Coding.class, Quantity.class}, order=2, min=1, max=1, modifier=false, summary=false) 1192 @Description(shortDefinition="Value of this parameter", formalDefinition="The value of the parameter" ) 1193 protected DataType value; 1194 1195 /** 1196 * If this mode is not passed to the runner, then this parameter will not be used 1197 */ 1198 @Child(name = "mode", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1199 @Description(shortDefinition="A mode that must be true for this parameter to be used", formalDefinition="If this mode is not passed to the runner, then this parameter will not be used" ) 1200 protected CodeType mode; 1201 1202 private static final long serialVersionUID = -410765611L; 1203 1204 /** 1205 * Constructor 1206 */ 1207 public TestCasesSuiteParameterComponent() { 1208 super(); 1209 } 1210 1211 /** 1212 * Constructor 1213 */ 1214 public TestCasesSuiteParameterComponent(String name, DataType value) { 1215 super(); 1216 this.setName(name); 1217 this.setValue(value); 1218 } 1219 1220 /** 1221 * @return {@link #name} (Name of parameter). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1222 */ 1223 public StringType getNameElement() { 1224 if (this.name == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create TestCasesSuiteParameterComponent.name"); 1227 else if (Configuration.doAutoCreate()) 1228 this.name = new StringType(); // bb 1229 return this.name; 1230 } 1231 1232 public boolean hasNameElement() { 1233 return this.name != null && !this.name.isEmpty(); 1234 } 1235 1236 public boolean hasName() { 1237 return this.name != null && !this.name.isEmpty(); 1238 } 1239 1240 /** 1241 * @param value {@link #name} (Name of parameter). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1242 */ 1243 public TestCasesSuiteParameterComponent setNameElement(StringType value) { 1244 this.name = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return Name of parameter 1250 */ 1251 public String getName() { 1252 return this.name == null ? null : this.name.getValue(); 1253 } 1254 1255 /** 1256 * @param value Name of parameter 1257 */ 1258 public TestCasesSuiteParameterComponent setName(String value) { 1259 if (this.name == null) 1260 this.name = new StringType(); 1261 this.name.setValue(value); 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #value} (The value of the parameter) 1267 */ 1268 public DataType getValue() { 1269 return this.value; 1270 } 1271 1272 /** 1273 * @return {@link #value} (The value of the parameter) 1274 */ 1275 public StringType getValueStringType() throws FHIRException { 1276 if (this.value == null) 1277 this.value = new StringType(); 1278 if (!(this.value instanceof StringType)) 1279 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1280 return (StringType) this.value; 1281 } 1282 1283 public boolean hasValueStringType() { 1284 return this.value instanceof StringType; 1285 } 1286 1287 /** 1288 * @return {@link #value} (The value of the parameter) 1289 */ 1290 public BooleanType getValueBooleanType() throws FHIRException { 1291 if (this.value == null) 1292 this.value = new BooleanType(); 1293 if (!(this.value instanceof BooleanType)) 1294 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1295 return (BooleanType) this.value; 1296 } 1297 1298 public boolean hasValueBooleanType() { 1299 return this.value instanceof BooleanType; 1300 } 1301 1302 /** 1303 * @return {@link #value} (The value of the parameter) 1304 */ 1305 public IntegerType getValueIntegerType() throws FHIRException { 1306 if (this.value == null) 1307 this.value = new IntegerType(); 1308 if (!(this.value instanceof IntegerType)) 1309 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1310 return (IntegerType) this.value; 1311 } 1312 1313 public boolean hasValueIntegerType() { 1314 return this.value instanceof IntegerType; 1315 } 1316 1317 /** 1318 * @return {@link #value} (The value of the parameter) 1319 */ 1320 public DecimalType getValueDecimalType() throws FHIRException { 1321 if (this.value == null) 1322 this.value = new DecimalType(); 1323 if (!(this.value instanceof DecimalType)) 1324 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1325 return (DecimalType) this.value; 1326 } 1327 1328 public boolean hasValueDecimalType() { 1329 return this.value instanceof DecimalType; 1330 } 1331 1332 /** 1333 * @return {@link #value} (The value of the parameter) 1334 */ 1335 public DateTimeType getValueDateTimeType() throws FHIRException { 1336 if (this.value == null) 1337 this.value = new DateTimeType(); 1338 if (!(this.value instanceof DateTimeType)) 1339 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1340 return (DateTimeType) this.value; 1341 } 1342 1343 public boolean hasValueDateTimeType() { 1344 return this.value instanceof DateTimeType; 1345 } 1346 1347 /** 1348 * @return {@link #value} (The value of the parameter) 1349 */ 1350 public UriType getValueUriType() throws FHIRException { 1351 if (this.value == null) 1352 this.value = new UriType(); 1353 if (!(this.value instanceof UriType)) 1354 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 1355 return (UriType) this.value; 1356 } 1357 1358 public boolean hasValueUriType() { 1359 return this.value instanceof UriType; 1360 } 1361 1362 /** 1363 * @return {@link #value} (The value of the parameter) 1364 */ 1365 public Coding getValueCoding() throws FHIRException { 1366 if (this.value == null) 1367 this.value = new Coding(); 1368 if (!(this.value instanceof Coding)) 1369 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 1370 return (Coding) this.value; 1371 } 1372 1373 public boolean hasValueCoding() { 1374 return this.value instanceof Coding; 1375 } 1376 1377 /** 1378 * @return {@link #value} (The value of the parameter) 1379 */ 1380 public Quantity getValueQuantity() throws FHIRException { 1381 if (this.value == null) 1382 this.value = new Quantity(); 1383 if (!(this.value instanceof Quantity)) 1384 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1385 return (Quantity) this.value; 1386 } 1387 1388 public boolean hasValueQuantity() { 1389 return this.value instanceof Quantity; 1390 } 1391 1392 public boolean hasValue() { 1393 return this.value != null && !this.value.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #value} (The value of the parameter) 1398 */ 1399 public TestCasesSuiteParameterComponent setValue(DataType value) { 1400 if (value != null && !(value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType || value instanceof DateTimeType || value instanceof UriType || value instanceof Coding || value instanceof Quantity)) 1401 throw new FHIRException("Not the right type for TestCases.suite.parameter.value[x]: "+value.fhirType()); 1402 this.value = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #mode} (If this mode is not passed to the runner, then this parameter will not be used). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1408 */ 1409 public CodeType getModeElement() { 1410 if (this.mode == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create TestCasesSuiteParameterComponent.mode"); 1413 else if (Configuration.doAutoCreate()) 1414 this.mode = new CodeType(); // bb 1415 return this.mode; 1416 } 1417 1418 public boolean hasModeElement() { 1419 return this.mode != null && !this.mode.isEmpty(); 1420 } 1421 1422 public boolean hasMode() { 1423 return this.mode != null && !this.mode.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #mode} (If this mode is not passed to the runner, then this parameter will not be used). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1428 */ 1429 public TestCasesSuiteParameterComponent setModeElement(CodeType value) { 1430 this.mode = value; 1431 return this; 1432 } 1433 1434 /** 1435 * @return If this mode is not passed to the runner, then this parameter will not be used 1436 */ 1437 public String getMode() { 1438 return this.mode == null ? null : this.mode.getValue(); 1439 } 1440 1441 /** 1442 * @param value If this mode is not passed to the runner, then this parameter will not be used 1443 */ 1444 public TestCasesSuiteParameterComponent setMode(String value) { 1445 if (Utilities.noString(value)) 1446 this.mode = null; 1447 else { 1448 if (this.mode == null) 1449 this.mode = new CodeType(); 1450 this.mode.setValue(value); 1451 } 1452 return this; 1453 } 1454 1455 protected void listChildren(List<Property> children) { 1456 super.listChildren(children); 1457 children.add(new Property("name", "string", "Name of parameter", 0, 1, name)); 1458 children.add(new Property("value[x]", "string|boolean|integer|decimal|dateTime|uri|Coding|Quantity", "The value of the parameter", 0, 1, value)); 1459 children.add(new Property("mode", "code", "If this mode is not passed to the runner, then this parameter will not be used", 0, 1, mode)); 1460 } 1461 1462 @Override 1463 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1464 switch (_hash) { 1465 case 3373707: /*name*/ return new Property("name", "string", "Name of parameter", 0, 1, name); 1466 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|boolean|integer|decimal|dateTime|uri|Coding|Quantity", "The value of the parameter", 0, 1, value); 1467 case 111972721: /*value*/ return new Property("value[x]", "string|boolean|integer|decimal|dateTime|uri|Coding|Quantity", "The value of the parameter", 0, 1, value); 1468 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the parameter", 0, 1, value); 1469 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the parameter", 0, 1, value); 1470 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the parameter", 0, 1, value); 1471 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the parameter", 0, 1, value); 1472 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the parameter", 0, 1, value); 1473 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the parameter", 0, 1, value); 1474 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of the parameter", 0, 1, value); 1475 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the parameter", 0, 1, value); 1476 case 3357091: /*mode*/ return new Property("mode", "code", "If this mode is not passed to the runner, then this parameter will not be used", 0, 1, mode); 1477 default: return super.getNamedProperty(_hash, _name, _checkValid); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1484 switch (hash) { 1485 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1486 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1487 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeType 1488 default: return super.getProperty(hash, name, checkValid); 1489 } 1490 1491 } 1492 1493 @Override 1494 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1495 switch (hash) { 1496 case 3373707: // name 1497 this.name = TypeConvertor.castToString(value); // StringType 1498 return value; 1499 case 111972721: // value 1500 this.value = TypeConvertor.castToType(value); // DataType 1501 return value; 1502 case 3357091: // mode 1503 this.mode = TypeConvertor.castToCode(value); // CodeType 1504 return value; 1505 default: return super.setProperty(hash, name, value); 1506 } 1507 1508 } 1509 1510 @Override 1511 public Base setProperty(String name, Base value) throws FHIRException { 1512 if (name.equals("name")) { 1513 this.name = TypeConvertor.castToString(value); // StringType 1514 } else if (name.equals("value[x]")) { 1515 this.value = TypeConvertor.castToType(value); // DataType 1516 } else if (name.equals("mode")) { 1517 this.mode = TypeConvertor.castToCode(value); // CodeType 1518 } else 1519 return super.setProperty(name, value); 1520 return value; 1521 } 1522 1523 @Override 1524 public Base makeProperty(int hash, String name) throws FHIRException { 1525 switch (hash) { 1526 case 3373707: return getNameElement(); 1527 case -1410166417: return getValue(); 1528 case 111972721: return getValue(); 1529 case 3357091: return getModeElement(); 1530 default: return super.makeProperty(hash, name); 1531 } 1532 1533 } 1534 1535 @Override 1536 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1537 switch (hash) { 1538 case 3373707: /*name*/ return new String[] {"string"}; 1539 case 111972721: /*value*/ return new String[] {"string", "boolean", "integer", "decimal", "dateTime", "uri", "Coding", "Quantity"}; 1540 case 3357091: /*mode*/ return new String[] {"code"}; 1541 default: return super.getTypesForProperty(hash, name); 1542 } 1543 1544 } 1545 1546 @Override 1547 public Base addChild(String name) throws FHIRException { 1548 if (name.equals("name")) { 1549 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.parameter.name"); 1550 } 1551 else if (name.equals("valueString")) { 1552 this.value = new StringType(); 1553 return this.value; 1554 } 1555 else if (name.equals("valueBoolean")) { 1556 this.value = new BooleanType(); 1557 return this.value; 1558 } 1559 else if (name.equals("valueInteger")) { 1560 this.value = new IntegerType(); 1561 return this.value; 1562 } 1563 else if (name.equals("valueDecimal")) { 1564 this.value = new DecimalType(); 1565 return this.value; 1566 } 1567 else if (name.equals("valueDateTime")) { 1568 this.value = new DateTimeType(); 1569 return this.value; 1570 } 1571 else if (name.equals("valueUri")) { 1572 this.value = new UriType(); 1573 return this.value; 1574 } 1575 else if (name.equals("valueCoding")) { 1576 this.value = new Coding(); 1577 return this.value; 1578 } 1579 else if (name.equals("valueQuantity")) { 1580 this.value = new Quantity(); 1581 return this.value; 1582 } 1583 else if (name.equals("mode")) { 1584 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.parameter.mode"); 1585 } 1586 else 1587 return super.addChild(name); 1588 } 1589 1590 public TestCasesSuiteParameterComponent copy() { 1591 TestCasesSuiteParameterComponent dst = new TestCasesSuiteParameterComponent(); 1592 copyValues(dst); 1593 return dst; 1594 } 1595 1596 public void copyValues(TestCasesSuiteParameterComponent dst) { 1597 super.copyValues(dst); 1598 dst.name = name == null ? null : name.copy(); 1599 dst.value = value == null ? null : value.copy(); 1600 dst.mode = mode == null ? null : mode.copy(); 1601 } 1602 1603 @Override 1604 public boolean equalsDeep(Base other_) { 1605 if (!super.equalsDeep(other_)) 1606 return false; 1607 if (!(other_ instanceof TestCasesSuiteParameterComponent)) 1608 return false; 1609 TestCasesSuiteParameterComponent o = (TestCasesSuiteParameterComponent) other_; 1610 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true) && compareDeep(mode, o.mode, true) 1611 ; 1612 } 1613 1614 @Override 1615 public boolean equalsShallow(Base other_) { 1616 if (!super.equalsShallow(other_)) 1617 return false; 1618 if (!(other_ instanceof TestCasesSuiteParameterComponent)) 1619 return false; 1620 TestCasesSuiteParameterComponent o = (TestCasesSuiteParameterComponent) other_; 1621 return compareValues(name, o.name, true) && compareValues(mode, o.mode, true); 1622 } 1623 1624 public boolean isEmpty() { 1625 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value, mode); 1626 } 1627 1628 public String fhirType() { 1629 return "TestCases.suite.parameter"; 1630 1631 } 1632 1633 } 1634 1635 @Block() 1636 public static class TestCasesSuiteTestComponent extends LogicalBase { 1637 /** 1638 * The name by which this test is known by in the test system. The name must be unique in the suite 1639 */ 1640 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1641 @Description(shortDefinition="The name of this test - unique in the suite", formalDefinition="The name by which this test is known by in the test system. The name must be unique in the suite" ) 1642 protected StringType name; 1643 1644 /** 1645 * Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results 1646 */ 1647 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1648 @Description(shortDefinition="Description of what this test does / why it was defined", formalDefinition="Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results" ) 1649 protected StringType description; 1650 1651 /** 1652 * A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner 1653 */ 1654 @Child(name = "operation", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1655 @Description(shortDefinition="Operation that is executed during this test (per definition of runner)", formalDefinition="A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner" ) 1656 protected CodeType operation; 1657 1658 /** 1659 * If this mode is not passed to the runner, then this test will not be run 1660 */ 1661 @Child(name = "mode", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1662 @Description(shortDefinition="mode required to run this test", formalDefinition="If this mode is not passed to the runner, then this test will not be run" ) 1663 protected StringType mode; 1664 1665 /** 1666 * 1667 */ 1668 @Child(name = "parameter", type = {TestCasesSuiteParameterComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1669 @Description(shortDefinition="", formalDefinition="" ) 1670 protected List<TestCasesSuiteParameterComponent> parameterList; 1671 1672 /** 1673 * The resources used when executing this test. How exactly they are used depends on the definition of the runner. 1674 */ 1675 @Child(name = "input", type = {TestCasesSuiteResourceComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1676 @Description(shortDefinition="Resources used when executing this test (per runner definition)", formalDefinition="The resources used when executing this test. How exactly they are used depends on the definition of the runner." ) 1677 protected List<TestCasesSuiteResourceComponent> inputList; 1678 1679 /** 1680 * Resources expected as output from this test. Often, but not always, these resources are Matchetype resources. How exactly it is used depends on the definition of the runner 1681 */ 1682 @Child(name = "output", type = {TestCasesSuiteResourceComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1683 @Description(shortDefinition="Resources expected as output from this test (per runner definition, often Matchetypes)", formalDefinition="Resources expected as output from this test. Often, but not always, these resources are Matchetype resources. How exactly it is used depends on the definition of the runner" ) 1684 protected List<TestCasesSuiteResourceComponent> outputList; 1685 1686 private static final long serialVersionUID = 330781544L; 1687 1688 /** 1689 * Constructor 1690 */ 1691 public TestCasesSuiteTestComponent() { 1692 super(); 1693 } 1694 1695 /** 1696 * Constructor 1697 */ 1698 public TestCasesSuiteTestComponent(String name) { 1699 super(); 1700 this.setName(name); 1701 } 1702 1703 /** 1704 * @return {@link #name} (The name by which this test is known by in the test system. The name must be unique in the suite). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1705 */ 1706 public StringType getNameElement() { 1707 if (this.name == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create TestCasesSuiteTestComponent.name"); 1710 else if (Configuration.doAutoCreate()) 1711 this.name = new StringType(); // bb 1712 return this.name; 1713 } 1714 1715 public boolean hasNameElement() { 1716 return this.name != null && !this.name.isEmpty(); 1717 } 1718 1719 public boolean hasName() { 1720 return this.name != null && !this.name.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #name} (The name by which this test is known by in the test system. The name must be unique in the suite). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1725 */ 1726 public TestCasesSuiteTestComponent setNameElement(StringType value) { 1727 this.name = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return The name by which this test is known by in the test system. The name must be unique in the suite 1733 */ 1734 public String getName() { 1735 return this.name == null ? null : this.name.getValue(); 1736 } 1737 1738 /** 1739 * @param value The name by which this test is known by in the test system. The name must be unique in the suite 1740 */ 1741 public TestCasesSuiteTestComponent setName(String value) { 1742 if (this.name == null) 1743 this.name = new StringType(); 1744 this.name.setValue(value); 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #description} (Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1750 */ 1751 public StringType getDescriptionElement() { 1752 if (this.description == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create TestCasesSuiteTestComponent.description"); 1755 else if (Configuration.doAutoCreate()) 1756 this.description = new StringType(); // bb 1757 return this.description; 1758 } 1759 1760 public boolean hasDescriptionElement() { 1761 return this.description != null && !this.description.isEmpty(); 1762 } 1763 1764 public boolean hasDescription() { 1765 return this.description != null && !this.description.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #description} (Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1770 */ 1771 public TestCasesSuiteTestComponent setDescriptionElement(StringType value) { 1772 this.description = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results 1778 */ 1779 public String getDescription() { 1780 return this.description == null ? null : this.description.getValue(); 1781 } 1782 1783 /** 1784 * @param value Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results 1785 */ 1786 public TestCasesSuiteTestComponent setDescription(String value) { 1787 if (Utilities.noString(value)) 1788 this.description = null; 1789 else { 1790 if (this.description == null) 1791 this.description = new StringType(); 1792 this.description.setValue(value); 1793 } 1794 return this; 1795 } 1796 1797 /** 1798 * @return {@link #operation} (A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner). This is the underlying object with id, value and extensions. The accessor "getOperation" gives direct access to the value 1799 */ 1800 public CodeType getOperationElement() { 1801 if (this.operation == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create TestCasesSuiteTestComponent.operation"); 1804 else if (Configuration.doAutoCreate()) 1805 this.operation = new CodeType(); // bb 1806 return this.operation; 1807 } 1808 1809 public boolean hasOperationElement() { 1810 return this.operation != null && !this.operation.isEmpty(); 1811 } 1812 1813 public boolean hasOperation() { 1814 return this.operation != null && !this.operation.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #operation} (A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner). This is the underlying object with id, value and extensions. The accessor "getOperation" gives direct access to the value 1819 */ 1820 public TestCasesSuiteTestComponent setOperationElement(CodeType value) { 1821 this.operation = value; 1822 return this; 1823 } 1824 1825 /** 1826 * @return A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner 1827 */ 1828 public String getOperation() { 1829 return this.operation == null ? null : this.operation.getValue(); 1830 } 1831 1832 /** 1833 * @param value A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner 1834 */ 1835 public TestCasesSuiteTestComponent setOperation(String value) { 1836 if (Utilities.noString(value)) 1837 this.operation = null; 1838 else { 1839 if (this.operation == null) 1840 this.operation = new CodeType(); 1841 this.operation.setValue(value); 1842 } 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #mode} (If this mode is not passed to the runner, then this test will not be run). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1848 */ 1849 public StringType getModeElement() { 1850 if (this.mode == null) 1851 if (Configuration.errorOnAutoCreate()) 1852 throw new Error("Attempt to auto-create TestCasesSuiteTestComponent.mode"); 1853 else if (Configuration.doAutoCreate()) 1854 this.mode = new StringType(); // bb 1855 return this.mode; 1856 } 1857 1858 public boolean hasModeElement() { 1859 return this.mode != null && !this.mode.isEmpty(); 1860 } 1861 1862 public boolean hasMode() { 1863 return this.mode != null && !this.mode.isEmpty(); 1864 } 1865 1866 /** 1867 * @param value {@link #mode} (If this mode is not passed to the runner, then this test will not be run). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1868 */ 1869 public TestCasesSuiteTestComponent setModeElement(StringType value) { 1870 this.mode = value; 1871 return this; 1872 } 1873 1874 /** 1875 * @return If this mode is not passed to the runner, then this test will not be run 1876 */ 1877 public String getMode() { 1878 return this.mode == null ? null : this.mode.getValue(); 1879 } 1880 1881 /** 1882 * @param value If this mode is not passed to the runner, then this test will not be run 1883 */ 1884 public TestCasesSuiteTestComponent setMode(String value) { 1885 if (Utilities.noString(value)) 1886 this.mode = null; 1887 else { 1888 if (this.mode == null) 1889 this.mode = new StringType(); 1890 this.mode.setValue(value); 1891 } 1892 return this; 1893 } 1894 1895 /** 1896 * @return {@link #parameter} () 1897 */ 1898 public List<TestCasesSuiteParameterComponent> getParameterList() { 1899 if (this.parameterList == null) 1900 this.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 1901 return this.parameterList; 1902 } 1903 1904 /** 1905 * @return Returns a reference to <code>this</code> for easy method chaining 1906 */ 1907 public TestCasesSuiteTestComponent setParameterList(List<TestCasesSuiteParameterComponent> theParameter) { 1908 this.parameterList = theParameter; 1909 return this; 1910 } 1911 1912 public boolean hasParameter() { 1913 if (this.parameterList == null) 1914 return false; 1915 for (TestCasesSuiteParameterComponent item : this.parameterList) 1916 if (!item.isEmpty()) 1917 return true; 1918 return false; 1919 } 1920 1921 public TestCasesSuiteParameterComponent addParameter() { //3a 1922 TestCasesSuiteParameterComponent t = new TestCasesSuiteParameterComponent(); 1923 if (this.parameterList == null) 1924 this.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 1925 this.parameterList.add(t); 1926 return t; 1927 } 1928 1929 public TestCasesSuiteTestComponent addParameter(TestCasesSuiteParameterComponent t) { //3b 1930 if (t == null) 1931 return this; 1932 if (this.parameterList == null) 1933 this.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 1934 this.parameterList.add(t); 1935 return this; 1936 } 1937 1938 /** 1939 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1940 */ 1941 public TestCasesSuiteParameterComponent getParameterFirstRep() { 1942 if (getParameterList().isEmpty()) { 1943 addParameter(); 1944 } 1945 return getParameterList().get(0); 1946 } 1947 1948 /** 1949 * @return {@link #input} (The resources used when executing this test. How exactly they are used depends on the definition of the runner.) 1950 */ 1951 public List<TestCasesSuiteResourceComponent> getInputList() { 1952 if (this.inputList == null) 1953 this.inputList = new ArrayList<TestCasesSuiteResourceComponent>(); 1954 return this.inputList; 1955 } 1956 1957 /** 1958 * @return Returns a reference to <code>this</code> for easy method chaining 1959 */ 1960 public TestCasesSuiteTestComponent setInputList(List<TestCasesSuiteResourceComponent> theInput) { 1961 this.inputList = theInput; 1962 return this; 1963 } 1964 1965 public boolean hasInput() { 1966 if (this.inputList == null) 1967 return false; 1968 for (TestCasesSuiteResourceComponent item : this.inputList) 1969 if (!item.isEmpty()) 1970 return true; 1971 return false; 1972 } 1973 1974 public TestCasesSuiteResourceComponent addInput() { //3a 1975 TestCasesSuiteResourceComponent t = new TestCasesSuiteResourceComponent(); 1976 if (this.inputList == null) 1977 this.inputList = new ArrayList<TestCasesSuiteResourceComponent>(); 1978 this.inputList.add(t); 1979 return t; 1980 } 1981 1982 public TestCasesSuiteTestComponent addInput(TestCasesSuiteResourceComponent t) { //3b 1983 if (t == null) 1984 return this; 1985 if (this.inputList == null) 1986 this.inputList = new ArrayList<TestCasesSuiteResourceComponent>(); 1987 this.inputList.add(t); 1988 return this; 1989 } 1990 1991 /** 1992 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 1993 */ 1994 public TestCasesSuiteResourceComponent getInputFirstRep() { 1995 if (getInputList().isEmpty()) { 1996 addInput(); 1997 } 1998 return getInputList().get(0); 1999 } 2000 2001 /** 2002 * @return {@link #output} (Resources expected as output from this test. Often, but not always, these resources are Matchetype resources. How exactly it is used depends on the definition of the runner) 2003 */ 2004 public List<TestCasesSuiteResourceComponent> getOutputList() { 2005 if (this.outputList == null) 2006 this.outputList = new ArrayList<TestCasesSuiteResourceComponent>(); 2007 return this.outputList; 2008 } 2009 2010 /** 2011 * @return Returns a reference to <code>this</code> for easy method chaining 2012 */ 2013 public TestCasesSuiteTestComponent setOutputList(List<TestCasesSuiteResourceComponent> theOutput) { 2014 this.outputList = theOutput; 2015 return this; 2016 } 2017 2018 public boolean hasOutput() { 2019 if (this.outputList == null) 2020 return false; 2021 for (TestCasesSuiteResourceComponent item : this.outputList) 2022 if (!item.isEmpty()) 2023 return true; 2024 return false; 2025 } 2026 2027 public TestCasesSuiteResourceComponent addOutput() { //3a 2028 TestCasesSuiteResourceComponent t = new TestCasesSuiteResourceComponent(); 2029 if (this.outputList == null) 2030 this.outputList = new ArrayList<TestCasesSuiteResourceComponent>(); 2031 this.outputList.add(t); 2032 return t; 2033 } 2034 2035 public TestCasesSuiteTestComponent addOutput(TestCasesSuiteResourceComponent t) { //3b 2036 if (t == null) 2037 return this; 2038 if (this.outputList == null) 2039 this.outputList = new ArrayList<TestCasesSuiteResourceComponent>(); 2040 this.outputList.add(t); 2041 return this; 2042 } 2043 2044 /** 2045 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 2046 */ 2047 public TestCasesSuiteResourceComponent getOutputFirstRep() { 2048 if (getOutputList().isEmpty()) { 2049 addOutput(); 2050 } 2051 return getOutputList().get(0); 2052 } 2053 2054 protected void listChildren(List<Property> children) { 2055 super.listChildren(children); 2056 children.add(new Property("name", "string", "The name by which this test is known by in the test system. The name must be unique in the suite", 0, 1, name)); 2057 children.add(new Property("description", "string", "Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results", 0, 1, description)); 2058 children.add(new Property("operation", "code", "A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner", 0, 1, operation)); 2059 children.add(new Property("mode", "string", "If this mode is not passed to the runner, then this test will not be run", 0, 1, mode)); 2060 children.add(new Property("parameter", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.parameter", "", 0, java.lang.Integer.MAX_VALUE, parameterList)); 2061 children.add(new Property("input", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource", "The resources used when executing this test. How exactly they are used depends on the definition of the runner.", 0, java.lang.Integer.MAX_VALUE, inputList)); 2062 children.add(new Property("output", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource", "Resources expected as output from this test. Often, but not always, these resources are Matchetype resources. How exactly it is used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, outputList)); 2063 } 2064 2065 @Override 2066 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2067 switch (_hash) { 2068 case 3373707: /*name*/ return new Property("name", "string", "The name by which this test is known by in the test system. The name must be unique in the suite", 0, 1, name); 2069 case -1724546052: /*description*/ return new Property("description", "string", "Description of what this test does / why it was defined. This should explain to a tester what they should know when looking at failing test results", 0, 1, description); 2070 case 1662702951: /*operation*/ return new Property("operation", "code", "A code that identifies the operation executed for this test. One of the codes defined in the definition of the runner", 0, 1, operation); 2071 case 3357091: /*mode*/ return new Property("mode", "string", "If this mode is not passed to the runner, then this test will not be run", 0, 1, mode); 2072 case 1954460585: /*parameter*/ return new Property("parameter", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.parameter", "", 0, java.lang.Integer.MAX_VALUE, parameterList); 2073 case 100358090: /*input*/ return new Property("input", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource", "The resources used when executing this test. How exactly they are used depends on the definition of the runner.", 0, java.lang.Integer.MAX_VALUE, inputList); 2074 case -1005512447: /*output*/ return new Property("output", "http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource", "Resources expected as output from this test. Often, but not always, these resources are Matchetype resources. How exactly it is used depends on the definition of the runner", 0, java.lang.Integer.MAX_VALUE, outputList); 2075 default: return super.getNamedProperty(_hash, _name, _checkValid); 2076 } 2077 2078 } 2079 2080 @Override 2081 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2082 switch (hash) { 2083 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2084 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2085 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // CodeType 2086 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // StringType 2087 case 1954460585: /*parameter*/ return this.parameterList == null ? new Base[0] : this.parameterList.toArray(new Base[this.parameterList.size()]); // TestCasesSuiteParameterComponent 2088 case 100358090: /*input*/ return this.inputList == null ? new Base[0] : this.inputList.toArray(new Base[this.inputList.size()]); // TestCasesSuiteResourceComponent 2089 case -1005512447: /*output*/ return this.outputList == null ? new Base[0] : this.outputList.toArray(new Base[this.outputList.size()]); // TestCasesSuiteResourceComponent 2090 default: return super.getProperty(hash, name, checkValid); 2091 } 2092 2093 } 2094 2095 @Override 2096 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2097 switch (hash) { 2098 case 3373707: // name 2099 this.name = TypeConvertor.castToString(value); // StringType 2100 return value; 2101 case -1724546052: // description 2102 this.description = TypeConvertor.castToString(value); // StringType 2103 return value; 2104 case 1662702951: // operation 2105 this.operation = TypeConvertor.castToCode(value); // CodeType 2106 return value; 2107 case 3357091: // mode 2108 this.mode = TypeConvertor.castToString(value); // StringType 2109 return value; 2110 case 1954460585: // parameter 2111 this.getParameterList().add((TestCasesSuiteParameterComponent) value); // TestCasesSuiteParameterComponent 2112 return value; 2113 case 100358090: // input 2114 this.getInputList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 2115 return value; 2116 case -1005512447: // output 2117 this.getOutputList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 2118 return value; 2119 default: return super.setProperty(hash, name, value); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base setProperty(String name, Base value) throws FHIRException { 2126 if (name.equals("name")) { 2127 this.name = TypeConvertor.castToString(value); // StringType 2128 } else if (name.equals("description")) { 2129 this.description = TypeConvertor.castToString(value); // StringType 2130 } else if (name.equals("operation")) { 2131 this.operation = TypeConvertor.castToCode(value); // CodeType 2132 } else if (name.equals("mode")) { 2133 this.mode = TypeConvertor.castToString(value); // StringType 2134 } else if (name.equals("parameter")) { 2135 this.getParameterList().add((TestCasesSuiteParameterComponent) value); // TestCasesSuiteParameterComponent 2136 } else if (name.equals("input")) { 2137 this.getInputList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 2138 } else if (name.equals("output")) { 2139 this.getOutputList().add((TestCasesSuiteResourceComponent) value); // TestCasesSuiteResourceComponent 2140 } else 2141 return super.setProperty(name, value); 2142 return value; 2143 } 2144 2145 @Override 2146 public Base makeProperty(int hash, String name) throws FHIRException { 2147 switch (hash) { 2148 case 3373707: return getNameElement(); 2149 case -1724546052: return getDescriptionElement(); 2150 case 1662702951: return getOperationElement(); 2151 case 3357091: return getModeElement(); 2152 case 1954460585: return addParameter(); 2153 case 100358090: return addInput(); 2154 case -1005512447: return addOutput(); 2155 default: return super.makeProperty(hash, name); 2156 } 2157 2158 } 2159 2160 @Override 2161 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2162 switch (hash) { 2163 case 3373707: /*name*/ return new String[] {"string"}; 2164 case -1724546052: /*description*/ return new String[] {"string"}; 2165 case 1662702951: /*operation*/ return new String[] {"code"}; 2166 case 3357091: /*mode*/ return new String[] {"string"}; 2167 case 1954460585: /*parameter*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.parameter"}; 2168 case 100358090: /*input*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource"}; 2169 case -1005512447: /*output*/ return new String[] {"http://hl7.org/fhir/tools/StructureDefinition/TestCases@TestCases.suite.resource"}; 2170 default: return super.getTypesForProperty(hash, name); 2171 } 2172 2173 } 2174 2175 @Override 2176 public Base addChild(String name) throws FHIRException { 2177 if (name.equals("name")) { 2178 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.test.name"); 2179 } 2180 else if (name.equals("description")) { 2181 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.test.description"); 2182 } 2183 else if (name.equals("operation")) { 2184 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.test.operation"); 2185 } 2186 else if (name.equals("mode")) { 2187 throw new FHIRException("Cannot call addChild on a singleton property TestCases.suite.test.mode"); 2188 } 2189 else if (name.equals("parameter")) { 2190 return addParameter(); 2191 } 2192 else if (name.equals("input")) { 2193 return addInput(); 2194 } 2195 else if (name.equals("output")) { 2196 return addOutput(); 2197 } 2198 else 2199 return super.addChild(name); 2200 } 2201 2202 public TestCasesSuiteTestComponent copy() { 2203 TestCasesSuiteTestComponent dst = new TestCasesSuiteTestComponent(); 2204 copyValues(dst); 2205 return dst; 2206 } 2207 2208 public void copyValues(TestCasesSuiteTestComponent dst) { 2209 super.copyValues(dst); 2210 dst.name = name == null ? null : name.copy(); 2211 dst.description = description == null ? null : description.copy(); 2212 dst.operation = operation == null ? null : operation.copy(); 2213 dst.mode = mode == null ? null : mode.copy(); 2214 if (parameterList != null) { 2215 dst.parameterList = new ArrayList<TestCasesSuiteParameterComponent>(); 2216 for (TestCasesSuiteParameterComponent i : parameterList) 2217 dst.parameterList.add(i.copy()); 2218 }; 2219 if (inputList != null) { 2220 dst.inputList = new ArrayList<TestCasesSuiteResourceComponent>(); 2221 for (TestCasesSuiteResourceComponent i : inputList) 2222 dst.inputList.add(i.copy()); 2223 }; 2224 if (outputList != null) { 2225 dst.outputList = new ArrayList<TestCasesSuiteResourceComponent>(); 2226 for (TestCasesSuiteResourceComponent i : outputList) 2227 dst.outputList.add(i.copy()); 2228 }; 2229 } 2230 2231 @Override 2232 public boolean equalsDeep(Base other_) { 2233 if (!super.equalsDeep(other_)) 2234 return false; 2235 if (!(other_ instanceof TestCasesSuiteTestComponent)) 2236 return false; 2237 TestCasesSuiteTestComponent o = (TestCasesSuiteTestComponent) other_; 2238 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(operation, o.operation, true) 2239 && compareDeep(mode, o.mode, true) && compareDeep(parameterList, o.parameterList, true) && compareDeep(inputList, o.inputList, true) 2240 && compareDeep(outputList, o.outputList, true); 2241 } 2242 2243 @Override 2244 public boolean equalsShallow(Base other_) { 2245 if (!super.equalsShallow(other_)) 2246 return false; 2247 if (!(other_ instanceof TestCasesSuiteTestComponent)) 2248 return false; 2249 TestCasesSuiteTestComponent o = (TestCasesSuiteTestComponent) other_; 2250 return compareValues(name, o.name, true) && compareValues(description, o.description, true) && compareValues(operation, o.operation, true) 2251 && compareValues(mode, o.mode, true); 2252 } 2253 2254 public boolean isEmpty() { 2255 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, operation 2256 , mode, parameterList, inputList, outputList); 2257 } 2258 2259 public String fhirType() { 2260 return "TestCases.suite.test"; 2261 2262 } 2263 2264// Additional Code from TestCasesSuiteTestComponent.java: 2265 2266 public String getParameterStr(String name) { 2267 for (TestCasesSuiteParameterComponent p : getParameterList()) { 2268 if (name.equals(p.getName())) { 2269 return p.getValue().primitiveValue(); 2270 } 2271 } 2272 2273 return null; 2274 } 2275 2276 public DataType getParameter(String name) { 2277 for (TestCasesSuiteParameterComponent p : getParameterList()) { 2278 if (name.equals(p.getName())) { 2279 return p.getValue(); 2280 } 2281 } 2282 2283 return null; 2284 } 2285 2286 public TestCasesSuiteResourceComponent getInput(String name) { 2287 for (TestCasesSuiteResourceComponent input : getInputList()) { 2288 if (name.equals(input.getName())) { 2289 return input; 2290 } 2291 } 2292 return null; 2293 } 2294// end addition 2295 } 2296 2297 /** 2298 * An absolute URI that is used to identify test tests. 2299 */ 2300 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2301 @Description(shortDefinition="Canonical identifier for these tests, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify test tests." ) 2302 protected UriType url; 2303 2304 /** 2305 * The identifier that is used to identify this version of these tests. Version must use SemVer 2306 */ 2307 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2308 @Description(shortDefinition="Business version of the test set (semver)", formalDefinition="The identifier that is used to identify this version of these tests. Version must use SemVer" ) 2309 protected StringType version; 2310 2311 /** 2312 * A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2313 */ 2314 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2315 @Description(shortDefinition="Name for these tests", formalDefinition="A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2316 protected StringType name; 2317 2318 /** 2319 * General description of these teats. 2320 */ 2321 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2322 @Description(shortDefinition="General Description of these tests", formalDefinition="General description of these teats." ) 2323 protected MarkdownType description; 2324 2325 /** 2326 * URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool. 2327 */ 2328 @Child(name = "runner", type = {UrlType.class}, order=4, min=1, max=1, modifier=false, summary=false) 2329 @Description(shortDefinition="URL Documentation for a runner that executes these tests", formalDefinition="URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool." ) 2330 protected UrlType runner; 2331 2332 /** 2333 * A mode that can be passed to a runner running these these tests, that affects test content and influences how the tests are executed or evaulated (or even if they run) 2334 */ 2335 @Child(name = "mode", type = {Base.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2336 @Description(shortDefinition="A mode that can be passed to runner - affects test content", formalDefinition="A mode that can be passed to a runner running these these tests, that affects test content and influences how the tests are executed or evaulated (or even if they run)" ) 2337 protected List<TestCasesModeComponent> modeList; 2338 2339 /** 2340 * A suite of tests that all share a common set up, and can be executed as a group 2341 */ 2342 @Child(name = "suite", type = {Base.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2343 @Description(shortDefinition="A suite of tests that share a common set up", formalDefinition="A suite of tests that all share a common set up, and can be executed as a group" ) 2344 protected List<TestCasesSuiteComponent> suiteList; 2345 2346 private static final long serialVersionUID = -1998407452L; 2347 2348 /** 2349 * Constructor 2350 */ 2351 public TestCases() { 2352 super(); 2353 } 2354 2355 /** 2356 * Constructor 2357 */ 2358 public TestCases(String runner) { 2359 super(); 2360 this.setRunner(runner); 2361 } 2362 2363 /** 2364 * @return {@link #url} (An absolute URI that is used to identify test tests.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2365 */ 2366 public UriType getUrlElement() { 2367 if (this.url == null) 2368 if (Configuration.errorOnAutoCreate()) 2369 throw new Error("Attempt to auto-create TestCases.url"); 2370 else if (Configuration.doAutoCreate()) 2371 this.url = new UriType(); // bb 2372 return this.url; 2373 } 2374 2375 public boolean hasUrlElement() { 2376 return this.url != null && !this.url.isEmpty(); 2377 } 2378 2379 public boolean hasUrl() { 2380 return this.url != null && !this.url.isEmpty(); 2381 } 2382 2383 /** 2384 * @param value {@link #url} (An absolute URI that is used to identify test tests.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2385 */ 2386 public TestCases setUrlElement(UriType value) { 2387 this.url = value; 2388 return this; 2389 } 2390 2391 /** 2392 * @return An absolute URI that is used to identify test tests. 2393 */ 2394 public String getUrl() { 2395 return this.url == null ? null : this.url.getValue(); 2396 } 2397 2398 /** 2399 * @param value An absolute URI that is used to identify test tests. 2400 */ 2401 public TestCases setUrl(String value) { 2402 if (Utilities.noString(value)) 2403 this.url = null; 2404 else { 2405 if (this.url == null) 2406 this.url = new UriType(); 2407 this.url.setValue(value); 2408 } 2409 return this; 2410 } 2411 2412 /** 2413 * @return {@link #version} (The identifier that is used to identify this version of these tests. Version must use SemVer). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2414 */ 2415 public StringType getVersionElement() { 2416 if (this.version == null) 2417 if (Configuration.errorOnAutoCreate()) 2418 throw new Error("Attempt to auto-create TestCases.version"); 2419 else if (Configuration.doAutoCreate()) 2420 this.version = new StringType(); // bb 2421 return this.version; 2422 } 2423 2424 public boolean hasVersionElement() { 2425 return this.version != null && !this.version.isEmpty(); 2426 } 2427 2428 public boolean hasVersion() { 2429 return this.version != null && !this.version.isEmpty(); 2430 } 2431 2432 /** 2433 * @param value {@link #version} (The identifier that is used to identify this version of these tests. Version must use SemVer). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2434 */ 2435 public TestCases setVersionElement(StringType value) { 2436 this.version = value; 2437 return this; 2438 } 2439 2440 /** 2441 * @return The identifier that is used to identify this version of these tests. Version must use SemVer 2442 */ 2443 public String getVersion() { 2444 return this.version == null ? null : this.version.getValue(); 2445 } 2446 2447 /** 2448 * @param value The identifier that is used to identify this version of these tests. Version must use SemVer 2449 */ 2450 public TestCases setVersion(String value) { 2451 if (Utilities.noString(value)) 2452 this.version = null; 2453 else { 2454 if (this.version == null) 2455 this.version = new StringType(); 2456 this.version.setValue(value); 2457 } 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #name} (A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2463 */ 2464 public StringType getNameElement() { 2465 if (this.name == null) 2466 if (Configuration.errorOnAutoCreate()) 2467 throw new Error("Attempt to auto-create TestCases.name"); 2468 else if (Configuration.doAutoCreate()) 2469 this.name = new StringType(); // bb 2470 return this.name; 2471 } 2472 2473 public boolean hasNameElement() { 2474 return this.name != null && !this.name.isEmpty(); 2475 } 2476 2477 public boolean hasName() { 2478 return this.name != null && !this.name.isEmpty(); 2479 } 2480 2481 /** 2482 * @param value {@link #name} (A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2483 */ 2484 public TestCases setNameElement(StringType value) { 2485 this.name = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2491 */ 2492 public String getName() { 2493 return this.name == null ? null : this.name.getValue(); 2494 } 2495 2496 /** 2497 * @param value A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2498 */ 2499 public TestCases setName(String value) { 2500 if (Utilities.noString(value)) 2501 this.name = null; 2502 else { 2503 if (this.name == null) 2504 this.name = new StringType(); 2505 this.name.setValue(value); 2506 } 2507 return this; 2508 } 2509 2510 /** 2511 * @return {@link #description} (General description of these teats.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2512 */ 2513 public MarkdownType getDescriptionElement() { 2514 if (this.description == null) 2515 if (Configuration.errorOnAutoCreate()) 2516 throw new Error("Attempt to auto-create TestCases.description"); 2517 else if (Configuration.doAutoCreate()) 2518 this.description = new MarkdownType(); // bb 2519 return this.description; 2520 } 2521 2522 public boolean hasDescriptionElement() { 2523 return this.description != null && !this.description.isEmpty(); 2524 } 2525 2526 public boolean hasDescription() { 2527 return this.description != null && !this.description.isEmpty(); 2528 } 2529 2530 /** 2531 * @param value {@link #description} (General description of these teats.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2532 */ 2533 public TestCases setDescriptionElement(MarkdownType value) { 2534 this.description = value; 2535 return this; 2536 } 2537 2538 /** 2539 * @return General description of these teats. 2540 */ 2541 public String getDescription() { 2542 return this.description == null ? null : this.description.getValue(); 2543 } 2544 2545 /** 2546 * @param value General description of these teats. 2547 */ 2548 public TestCases setDescription(String value) { 2549 if (Utilities.noString(value)) 2550 this.description = null; 2551 else { 2552 if (this.description == null) 2553 this.description = new MarkdownType(); 2554 this.description.setValue(value); 2555 } 2556 return this; 2557 } 2558 2559 /** 2560 * @return {@link #runner} (URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool.). This is the underlying object with id, value and extensions. The accessor "getRunner" gives direct access to the value 2561 */ 2562 public UrlType getRunnerElement() { 2563 if (this.runner == null) 2564 if (Configuration.errorOnAutoCreate()) 2565 throw new Error("Attempt to auto-create TestCases.runner"); 2566 else if (Configuration.doAutoCreate()) 2567 this.runner = new UrlType(); // bb 2568 return this.runner; 2569 } 2570 2571 public boolean hasRunnerElement() { 2572 return this.runner != null && !this.runner.isEmpty(); 2573 } 2574 2575 public boolean hasRunner() { 2576 return this.runner != null && !this.runner.isEmpty(); 2577 } 2578 2579 /** 2580 * @param value {@link #runner} (URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool.). This is the underlying object with id, value and extensions. The accessor "getRunner" gives direct access to the value 2581 */ 2582 public TestCases setRunnerElement(UrlType value) { 2583 this.runner = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool. 2589 */ 2590 public String getRunner() { 2591 return this.runner == null ? null : this.runner.getValue(); 2592 } 2593 2594 /** 2595 * @param value URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool. 2596 */ 2597 public TestCases setRunner(String value) { 2598 if (this.runner == null) 2599 this.runner = new UrlType(); 2600 this.runner.setValue(value); 2601 return this; 2602 } 2603 2604 /** 2605 * @return {@link #mode} (A mode that can be passed to a runner running these these tests, that affects test content and influences how the tests are executed or evaulated (or even if they run)) 2606 */ 2607 public List<TestCasesModeComponent> getModeList() { 2608 if (this.modeList == null) 2609 this.modeList = new ArrayList<TestCasesModeComponent>(); 2610 return this.modeList; 2611 } 2612 2613 /** 2614 * @return Returns a reference to <code>this</code> for easy method chaining 2615 */ 2616 public TestCases setModeList(List<TestCasesModeComponent> theMode) { 2617 this.modeList = theMode; 2618 return this; 2619 } 2620 2621 public boolean hasMode() { 2622 if (this.modeList == null) 2623 return false; 2624 for (TestCasesModeComponent item : this.modeList) 2625 if (!item.isEmpty()) 2626 return true; 2627 return false; 2628 } 2629 2630 public TestCases addMode(TestCasesModeComponent t) { //3b 2631 if (t == null) 2632 return this; 2633 if (this.modeList == null) 2634 this.modeList = new ArrayList<TestCasesModeComponent>(); 2635 this.modeList.add(t); 2636 return this; 2637 } 2638 2639 /** 2640 * @return {@link #suite} (A suite of tests that all share a common set up, and can be executed as a group) 2641 */ 2642 public List<TestCasesSuiteComponent> getSuiteList() { 2643 if (this.suiteList == null) 2644 this.suiteList = new ArrayList<TestCasesSuiteComponent>(); 2645 return this.suiteList; 2646 } 2647 2648 /** 2649 * @return Returns a reference to <code>this</code> for easy method chaining 2650 */ 2651 public TestCases setSuiteList(List<TestCasesSuiteComponent> theSuite) { 2652 this.suiteList = theSuite; 2653 return this; 2654 } 2655 2656 public boolean hasSuite() { 2657 if (this.suiteList == null) 2658 return false; 2659 for (TestCasesSuiteComponent item : this.suiteList) 2660 if (!item.isEmpty()) 2661 return true; 2662 return false; 2663 } 2664 2665 public TestCases addSuite(TestCasesSuiteComponent t) { //3b 2666 if (t == null) 2667 return this; 2668 if (this.suiteList == null) 2669 this.suiteList = new ArrayList<TestCasesSuiteComponent>(); 2670 this.suiteList.add(t); 2671 return this; 2672 } 2673 2674 protected void listChildren(List<Property> children) { 2675 super.listChildren(children); 2676 children.add(new Property("url", "uri", "An absolute URI that is used to identify test tests.", 0, 1, url)); 2677 children.add(new Property("version", "string", "The identifier that is used to identify this version of these tests. Version must use SemVer", 0, 1, version)); 2678 children.add(new Property("name", "string", "A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2679 children.add(new Property("description", "markdown", "General description of these teats.", 0, 1, description)); 2680 children.add(new Property("runner", "url", "URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool.", 0, 1, runner)); 2681 children.add(new Property("mode", "Base", "A mode that can be passed to a runner running these these tests, that affects test content and influences how the tests are executed or evaulated (or even if they run)", 0, java.lang.Integer.MAX_VALUE, modeList)); 2682 children.add(new Property("suite", "Base", "A suite of tests that all share a common set up, and can be executed as a group", 0, java.lang.Integer.MAX_VALUE, suiteList)); 2683 } 2684 2685 @Override 2686 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2687 switch (_hash) { 2688 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify test tests.", 0, 1, url); 2689 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of these tests. Version must use SemVer", 0, 1, version); 2690 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the tests. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2691 case -1724546052: /*description*/ return new Property("description", "markdown", "General description of these teats.", 0, 1, description); 2692 case -919806160: /*runner*/ return new Property("runner", "url", "URL of documentation that explains how a runner would read these tests, and use them to actually test out a tool.", 0, 1, runner); 2693 case 3357091: /*mode*/ return new Property("mode", "Base", "A mode that can be passed to a runner running these these tests, that affects test content and influences how the tests are executed or evaulated (or even if they run)", 0, java.lang.Integer.MAX_VALUE, modeList); 2694 case 109795064: /*suite*/ return new Property("suite", "Base", "A suite of tests that all share a common set up, and can be executed as a group", 0, java.lang.Integer.MAX_VALUE, suiteList); 2695 default: return super.getNamedProperty(_hash, _name, _checkValid); 2696 } 2697 2698 } 2699 2700 @Override 2701 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2702 switch (hash) { 2703 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2704 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2705 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2706 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2707 case -919806160: /*runner*/ return this.runner == null ? new Base[0] : new Base[] {this.runner}; // UrlType 2708 case 3357091: /*mode*/ return this.modeList == null ? new Base[0] : this.modeList.toArray(new Base[this.modeList.size()]); // TestCasesModeComponent 2709 case 109795064: /*suite*/ return this.suiteList == null ? new Base[0] : this.suiteList.toArray(new Base[this.suiteList.size()]); // TestCasesSuiteComponent 2710 default: return super.getProperty(hash, name, checkValid); 2711 } 2712 2713 } 2714 2715 @Override 2716 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2717 switch (hash) { 2718 case 116079: // url 2719 this.url = TypeConvertor.castToUri(value); // UriType 2720 return value; 2721 case 351608024: // version 2722 this.version = TypeConvertor.castToString(value); // StringType 2723 return value; 2724 case 3373707: // name 2725 this.name = TypeConvertor.castToString(value); // StringType 2726 return value; 2727 case -1724546052: // description 2728 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2729 return value; 2730 case -919806160: // runner 2731 this.runner = TypeConvertor.castToUrl(value); // UrlType 2732 return value; 2733 case 3357091: // mode 2734 this.getModeList().add((TestCasesModeComponent) value); // TestCasesModeComponent 2735 return value; 2736 case 109795064: // suite 2737 this.getSuiteList().add((TestCasesSuiteComponent) value); // TestCasesSuiteComponent 2738 return value; 2739 default: return super.setProperty(hash, name, value); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base setProperty(String name, Base value) throws FHIRException { 2746 if (name.equals("url")) { 2747 this.url = TypeConvertor.castToUri(value); // UriType 2748 } else if (name.equals("version")) { 2749 this.version = TypeConvertor.castToString(value); // StringType 2750 } else if (name.equals("name")) { 2751 this.name = TypeConvertor.castToString(value); // StringType 2752 } else if (name.equals("description")) { 2753 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2754 } else if (name.equals("runner")) { 2755 this.runner = TypeConvertor.castToUrl(value); // UrlType 2756 } else if (name.equals("mode")) { 2757 this.getModeList().add((TestCasesModeComponent) value); // TestCasesModeComponent 2758 } else if (name.equals("suite")) { 2759 this.getSuiteList().add((TestCasesSuiteComponent) value); // TestCasesSuiteComponent 2760 } else 2761 return super.setProperty(name, value); 2762 return value; 2763 } 2764 2765 @Override 2766 public Base makeProperty(int hash, String name) throws FHIRException { 2767 switch (hash) { 2768 case 116079: return getUrlElement(); 2769 case 351608024: return getVersionElement(); 2770 case 3373707: return getNameElement(); 2771 case -1724546052: return getDescriptionElement(); 2772 case -919806160: return getRunnerElement(); 2773 case 3357091: /*div*/ 2774 throw new Error("Unable to make an instance of the abstract property 'mode'"); 2775 case 109795064: /*div*/ 2776 throw new Error("Unable to make an instance of the abstract property 'suite'"); 2777 default: return super.makeProperty(hash, name); 2778 } 2779 2780 } 2781 2782 @Override 2783 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2784 switch (hash) { 2785 case 116079: /*url*/ return new String[] {"uri"}; 2786 case 351608024: /*version*/ return new String[] {"string"}; 2787 case 3373707: /*name*/ return new String[] {"string"}; 2788 case -1724546052: /*description*/ return new String[] {"markdown"}; 2789 case -919806160: /*runner*/ return new String[] {"url"}; 2790 case 3357091: /*mode*/ return new String[] {"Base"}; 2791 case 109795064: /*suite*/ return new String[] {"Base"}; 2792 default: return super.getTypesForProperty(hash, name); 2793 } 2794 2795 } 2796 2797 @Override 2798 public Base addChild(String name) throws FHIRException { 2799 if (name.equals("url")) { 2800 throw new FHIRException("Cannot call addChild on a singleton property TestCases.url"); 2801 } 2802 else if (name.equals("version")) { 2803 throw new FHIRException("Cannot call addChild on a singleton property TestCases.version"); 2804 } 2805 else if (name.equals("name")) { 2806 throw new FHIRException("Cannot call addChild on a singleton property TestCases.name"); 2807 } 2808 else if (name.equals("description")) { 2809 throw new FHIRException("Cannot call addChild on a singleton property TestCases.description"); 2810 } 2811 else if (name.equals("runner")) { 2812 throw new FHIRException("Cannot call addChild on a singleton property TestCases.runner"); 2813 } 2814 else if (name.equals("mode")) { 2815 throw new FHIRException("Cannot call addChild on an abstract type TestCases.mode"); 2816 } 2817 else if (name.equals("suite")) { 2818 throw new FHIRException("Cannot call addChild on an abstract type TestCases.suite"); 2819 } 2820 else 2821 return super.addChild(name); 2822 } 2823 2824 public String fhirType() { 2825 return "TestCases"; 2826 2827 } 2828 2829 public TestCases copy() { 2830 TestCases dst = new TestCases(); 2831 copyValues(dst); 2832 return dst; 2833 } 2834 2835 public void copyValues(TestCases dst) { 2836 super.copyValues(dst); 2837 dst.url = url == null ? null : url.copy(); 2838 dst.version = version == null ? null : version.copy(); 2839 dst.name = name == null ? null : name.copy(); 2840 dst.description = description == null ? null : description.copy(); 2841 dst.runner = runner == null ? null : runner.copy(); 2842 if (modeList != null) { 2843 dst.modeList = new ArrayList<TestCasesModeComponent>(); 2844 for (TestCasesModeComponent i : modeList) 2845 dst.modeList.add(i.copy()); 2846 }; 2847 if (suiteList != null) { 2848 dst.suiteList = new ArrayList<TestCasesSuiteComponent>(); 2849 for (TestCasesSuiteComponent i : suiteList) 2850 dst.suiteList.add(i.copy()); 2851 }; 2852 } 2853 2854 protected TestCases typedCopy() { 2855 return copy(); 2856 } 2857 2858 @Override 2859 public boolean equalsDeep(Base other_) { 2860 if (!super.equalsDeep(other_)) 2861 return false; 2862 if (!(other_ instanceof TestCases)) 2863 return false; 2864 TestCases o = (TestCases) other_; 2865 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 2866 && compareDeep(description, o.description, true) && compareDeep(runner, o.runner, true) && compareDeep(modeList, o.modeList, true) 2867 && compareDeep(suiteList, o.suiteList, true); 2868 } 2869 2870 @Override 2871 public boolean equalsShallow(Base other_) { 2872 if (!super.equalsShallow(other_)) 2873 return false; 2874 if (!(other_ instanceof TestCases)) 2875 return false; 2876 TestCases o = (TestCases) other_; 2877 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2878 && compareValues(description, o.description, true) && compareValues(runner, o.runner, true); 2879 } 2880 2881 public boolean isEmpty() { 2882 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, version, name, description 2883 , runner, modeList, suiteList); 2884 } 2885 2886 @Override 2887 public ResourceType getResourceType() { 2888 return ResourceType.Custom; 2889 } 2890 2891 public String getCustomResourceName() { 2892 return "TestCases"; 2893 } 2894 2895 2896} 2897