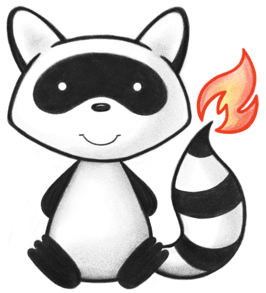
001package org.hl7.fhir.r5.tools; 002 003// generated 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, \ 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this \ 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, \ 015 this list of conditions and the following disclaimer in the documentation \ 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 030 POSSIBILITY OF SUCH DAMAGE. 031 */ 032 033// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 034 035 036 037import java.io.IOException; 038import org.hl7.fhir.exceptions.FHIRFormatError; 039 040import org.hl7.fhir.r5.formats.JsonCreator; 041import org.hl7.fhir.r5.formats.JsonParserBase; 042import org.hl7.fhir.r5.model.*; 043import org.hl7.fhir.utilities.Utilities; 044 045import com.google.gson.JsonArray; 046import com.google.gson.JsonObject; 047 048public class ToolsJsonParser extends org.hl7.fhir.r5.formats.JsonParser { 049 050 public ToolsJsonParser(JsonCreator json) { 051 super(); 052 this.json = json; 053 } 054 055 public ToolsJsonParser(boolean allowUnknownContent, boolean allowComments) { 056 super(); 057 setAllowUnknownContent(allowUnknownContent); 058 setAllowComments(allowComments); 059 } 060 061 public Resource parseResource(JsonObject json) throws IOException, FHIRFormatError { 062 if (!json.has("resourceType")) { 063 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 064 } 065 String t = json.get("resourceType").getAsString(); 066 if (Utilities.noString(t)) { 067 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 068 } else if (t.equals("TestCases")) { 069 return parseTestCases(json); 070 071 } else { 072 throw new FHIRFormatError("Unknown/Unrecognised resource type '"+t+"' (in property 'resourceType')"); 073 } 074 } 075 076 protected void parseCDSHookContextProperties(JsonObject json, CDSHookContext res) throws IOException, FHIRFormatError { 077 parseBaseProperties(json, res); 078 } 079 080 protected void parseCDSHooksElementProperties(JsonObject json, CDSHooksElement res) throws IOException, FHIRFormatError { 081 parseBaseProperties(json, res); 082 if (json.has("extension")) 083 res.setExtension(parseCDSHooksExtensions(getJObject(json, "extension"))); 084 } 085 086 protected CDSHookOrderSelectContext parseCDSHookOrderSelectContext(JsonObject json) throws IOException, FHIRFormatError { 087 CDSHookOrderSelectContext res = new CDSHookOrderSelectContext(); 088 parseCDSHookOrderSelectContextProperties(json, res); 089 return res; 090 } 091 092 protected void parseCDSHookOrderSelectContextProperties(JsonObject json, CDSHookOrderSelectContext res) throws IOException, FHIRFormatError { 093 parseCDSHookContextProperties(json, res); 094 if (json.has("userId")) 095 res.setUserIdElement(parseUrl(json.get("userId").getAsString())); 096 if (json.has("_userId")) 097 parseElementProperties(getJObject(json, "_userId"), res.getUserIdElement()); 098 if (json.has("patientId")) 099 res.setPatientIdElement(parseId(json.get("patientId").getAsString())); 100 if (json.has("_patientId")) 101 parseElementProperties(getJObject(json, "_patientId"), res.getPatientIdElement()); 102 if (json.has("encounterId")) 103 res.setEncounterIdElement(parseId(json.get("encounterId").getAsString())); 104 if (json.has("_encounterId")) 105 parseElementProperties(getJObject(json, "_encounterId"), res.getEncounterIdElement()); 106 if (json.has("selections")) { 107 JsonArray array = getJArray(json, "selections"); 108 for (int i = 0; i < array.size(); i++) { 109 if (array.get(i).isJsonNull()) { 110 res.getSelections().add(new UriType()); 111 } else {; 112 res.getSelections().add(parseUri(array.get(i).getAsString())); 113 } 114 } 115 }; 116 if (json.has("_selections")) { 117 JsonArray array = getJArray(json, "_selections"); 118 for (int i = 0; i < array.size(); i++) { 119 if (i == res.getSelections().size()) 120 res.getSelections().add(parseUri(null)); 121 if (array.get(i) instanceof JsonObject) 122 parseElementProperties(array.get(i).getAsJsonObject(), res.getSelections().get(i)); 123 } 124 }; 125 if (json.has("draftOrders")) 126 res.setDraftOrders(parseBundle(getJObject(json, "draftOrders"))); 127 } 128 129 protected CDSHookOrderSignContext parseCDSHookOrderSignContext(JsonObject json) throws IOException, FHIRFormatError { 130 CDSHookOrderSignContext res = new CDSHookOrderSignContext(); 131 parseCDSHookOrderSignContextProperties(json, res); 132 return res; 133 } 134 135 protected void parseCDSHookOrderSignContextProperties(JsonObject json, CDSHookOrderSignContext res) throws IOException, FHIRFormatError { 136 parseCDSHookContextProperties(json, res); 137 if (json.has("userId")) 138 res.setUserIdElement(parseUrl(json.get("userId").getAsString())); 139 if (json.has("_userId")) 140 parseElementProperties(getJObject(json, "_userId"), res.getUserIdElement()); 141 if (json.has("patientId")) 142 res.setPatientIdElement(parseId(json.get("patientId").getAsString())); 143 if (json.has("_patientId")) 144 parseElementProperties(getJObject(json, "_patientId"), res.getPatientIdElement()); 145 if (json.has("encounterId")) 146 res.setEncounterIdElement(parseId(json.get("encounterId").getAsString())); 147 if (json.has("_encounterId")) 148 parseElementProperties(getJObject(json, "_encounterId"), res.getEncounterIdElement()); 149 if (json.has("draftOrders")) 150 res.setDraftOrders(parseBundle(getJObject(json, "draftOrders"))); 151 } 152 153 protected CDSHookPatientViewContext parseCDSHookPatientViewContext(JsonObject json) throws IOException, FHIRFormatError { 154 CDSHookPatientViewContext res = new CDSHookPatientViewContext(); 155 parseCDSHookPatientViewContextProperties(json, res); 156 return res; 157 } 158 159 protected void parseCDSHookPatientViewContextProperties(JsonObject json, CDSHookPatientViewContext res) throws IOException, FHIRFormatError { 160 parseCDSHookContextProperties(json, res); 161 if (json.has("userId")) 162 res.setUserIdElement(parseUrl(json.get("userId").getAsString())); 163 if (json.has("_userId")) 164 parseElementProperties(getJObject(json, "_userId"), res.getUserIdElement()); 165 if (json.has("patientId")) 166 res.setPatientIdElement(parseId(json.get("patientId").getAsString())); 167 if (json.has("_patientId")) 168 parseElementProperties(getJObject(json, "_patientId"), res.getPatientIdElement()); 169 if (json.has("encounterId")) 170 res.setEncounterIdElement(parseId(json.get("encounterId").getAsString())); 171 if (json.has("_encounterId")) 172 parseElementProperties(getJObject(json, "_encounterId"), res.getEncounterIdElement()); 173 } 174 175 protected CDSHooksExtensions parseCDSHooksExtensions(JsonObject json) throws IOException, FHIRFormatError { 176 CDSHooksExtensions res = new CDSHooksExtensions(); 177 parseCDSHooksExtensionsProperties(json, res); 178 return res; 179 } 180 181 protected void parseCDSHooksExtensionsProperties(JsonObject json, CDSHooksExtensions res) throws IOException, FHIRFormatError { 182 parseElementProperties(json, res); 183 } 184 185 protected CDSHooksRequest parseCDSHooksRequest(JsonObject json) throws IOException, FHIRFormatError { 186 CDSHooksRequest res = new CDSHooksRequest(); 187 parseCDSHooksRequestProperties(json, res); 188 return res; 189 } 190 191 protected void parseCDSHooksRequestProperties(JsonObject json, CDSHooksRequest res) throws IOException, FHIRFormatError { 192 parseCDSHooksElementProperties(json, res); 193 if (json.has("hook")) 194 res.setHookElement(parseCode(json.get("hook").getAsString())); 195 if (json.has("_hook")) 196 parseElementProperties(getJObject(json, "_hook"), res.getHookElement()); 197 if (json.has("hookInstance")) 198 res.setHookInstanceElement(parseUuid(json.get("hookInstance").getAsString())); 199 if (json.has("_hookInstance")) 200 parseElementProperties(getJObject(json, "_hookInstance"), res.getHookInstanceElement()); 201 if (json.has("fhirServer")) 202 res.setFhirServerElement(parseUrl(json.get("fhirServer").getAsString())); 203 if (json.has("_fhirServer")) 204 parseElementProperties(getJObject(json, "_fhirServer"), res.getFhirServerElement()); 205 if (json.has("fhirAuthorization")) 206 res.setFhirAuthorization(parseCDSHooksRequestFhirAuthorizationComponent(getJObject(json, "fhirAuthorization"))); 207 if (json.has("context")) 208 res.setContext(parseCDSHookContext(json, getJObject(json, "context"))); 209 if (json.has("prefetch")) { 210 JsonArray array = getJArray(json, "prefetch"); 211 for (int i = 0; i < array.size(); i++) { 212 res.getPrefetch().add(parseCDSHooksRequestPrefetchComponent(array.get(i).getAsJsonObject())); 213 } 214 }; 215 } 216 217 protected CDSHookContext parseCDSHookContext(JsonObject resource, JsonObject json) throws IOException, FHIRFormatError { 218 if (resource.get("hook").getAsString().equals("patient-view")) { 219 return parseCDSHookPatientViewContext(json); 220 } 221 if (resource.get("hook").getAsString().equals("order-sign")) { 222 return parseCDSHookOrderSignContext(json); 223 } 224 if (resource.get("hook").getAsString().equals("order-select")) { 225 return parseCDSHookOrderSelectContext(json); 226 } 227 throw new FHIRFormatError("Unable to parse CDSHookContext: The content does not meet any of the type specifiers"); 228 } 229 230 protected CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent parseCDSHooksRequestFhirAuthorizationComponent(JsonObject json) throws IOException, FHIRFormatError { 231 CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent res = new CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent(); 232 parseCDSHooksRequestFhirAuthorizationComponentProperties(json, res); 233 return res; 234 } 235 236 protected void parseCDSHooksRequestFhirAuthorizationComponentProperties(JsonObject json, CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent res) throws IOException, FHIRFormatError { 237 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 238 if (json.has("accessToken")) 239 res.setAccessTokenElement(parseString(json.get("accessToken").getAsString())); 240 if (json.has("_accessToken")) 241 parseElementProperties(getJObject(json, "_accessToken"), res.getAccessTokenElement()); 242 if (json.has("tokenType")) 243 res.setTokenTypeElement(parseCode(json.get("tokenType").getAsString())); 244 if (json.has("_tokenType")) 245 parseElementProperties(getJObject(json, "_tokenType"), res.getTokenTypeElement()); 246 if (json.has("expiresIn")) 247 res.setExpiresInElement(parseInteger(json.get("expiresIn").getAsLong())); 248 if (json.has("_expiresIn")) 249 parseElementProperties(getJObject(json, "_expiresIn"), res.getExpiresInElement()); 250 if (json.has("scope")) 251 res.setScopeElement(parseString(json.get("scope").getAsString())); 252 if (json.has("_scope")) 253 parseElementProperties(getJObject(json, "_scope"), res.getScopeElement()); 254 if (json.has("subject")) 255 res.setSubjectElement(parseString(json.get("subject").getAsString())); 256 if (json.has("_subject")) 257 parseElementProperties(getJObject(json, "_subject"), res.getSubjectElement()); 258 if (json.has("patient")) 259 res.setPatientElement(parseId(json.get("patient").getAsString())); 260 if (json.has("_patient")) 261 parseElementProperties(getJObject(json, "_patient"), res.getPatientElement()); 262 } 263 264 protected CDSHooksRequest.CDSHooksRequestPrefetchComponent parseCDSHooksRequestPrefetchComponent(JsonObject json) throws IOException, FHIRFormatError { 265 CDSHooksRequest.CDSHooksRequestPrefetchComponent res = new CDSHooksRequest.CDSHooksRequestPrefetchComponent(); 266 parseCDSHooksRequestPrefetchComponentProperties(json, res); 267 return res; 268 } 269 270 protected void parseCDSHooksRequestPrefetchComponentProperties(JsonObject json, CDSHooksRequest.CDSHooksRequestPrefetchComponent res) throws IOException, FHIRFormatError { 271 parseBaseProperties(json, res); 272 if (json.has("key")) 273 res.setKeyElement(parseCode(json.get("key").getAsString())); 274 if (json.has("_key")) 275 parseElementProperties(getJObject(json, "_key"), res.getKeyElement()); 276 if (json.has("value")) 277 res.setValue(parseResource(getJObject(json, "value"))); 278 } 279 280 protected CDSHooksResponse parseCDSHooksResponse(JsonObject json) throws IOException, FHIRFormatError { 281 CDSHooksResponse res = new CDSHooksResponse(); 282 parseCDSHooksResponseProperties(json, res); 283 return res; 284 } 285 286 protected void parseCDSHooksResponseProperties(JsonObject json, CDSHooksResponse res) throws IOException, FHIRFormatError { 287 parseCDSHooksElementProperties(json, res); 288 if (json.has("cards")) { 289 JsonArray array = getJArray(json, "cards"); 290 for (int i = 0; i < array.size(); i++) { 291 res.getCards().add(parseCDSHooksResponseCardsComponent(array.get(i).getAsJsonObject())); 292 } 293 }; 294 if (json.has("systemActions")) { 295 JsonArray array = getJArray(json, "systemActions"); 296 for (int i = 0; i < array.size(); i++) { 297 res.getSystemActions().add(parseCDSHooksResponseCardsSuggestionsActionsComponent(array.get(i).getAsJsonObject())); 298 } 299 }; 300 } 301 302 protected CDSHooksResponse.CDSHooksResponseCardsComponent parseCDSHooksResponseCardsComponent(JsonObject json) throws IOException, FHIRFormatError { 303 CDSHooksResponse.CDSHooksResponseCardsComponent res = new CDSHooksResponse.CDSHooksResponseCardsComponent(); 304 parseCDSHooksResponseCardsComponentProperties(json, res); 305 return res; 306 } 307 308 protected void parseCDSHooksResponseCardsComponentProperties(JsonObject json, CDSHooksResponse.CDSHooksResponseCardsComponent res) throws IOException, FHIRFormatError { 309 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 310 if (json.has("uuid")) 311 res.setUuidElement(parseUuid(json.get("uuid").getAsString())); 312 if (json.has("_uuid")) 313 parseElementProperties(getJObject(json, "_uuid"), res.getUuidElement()); 314 if (json.has("summary")) 315 res.setSummaryElement(parseString(json.get("summary").getAsString())); 316 if (json.has("_summary")) 317 parseElementProperties(getJObject(json, "_summary"), res.getSummaryElement()); 318 if (json.has("detail")) 319 res.setDetailElement(parseMarkdown(json.get("detail").getAsString())); 320 if (json.has("_detail")) 321 parseElementProperties(getJObject(json, "_detail"), res.getDetailElement()); 322 if (json.has("indicator")) 323 res.setIndicatorElement(parseEnumeration(json.get("indicator").getAsString(), CDSHooksResponse.CDSIndicatorCodesVS.NULL, new CDSHooksResponse.CDSIndicatorCodesVSEnumFactory())); 324 if (json.has("_indicator")) 325 parseElementProperties(getJObject(json, "_indicator"), res.getIndicatorElement()); 326 if (json.has("source")) 327 res.setSource(parseCDSHooksResponseCardsSourceComponent(getJObject(json, "source"))); 328 if (json.has("suggestions")) { 329 JsonArray array = getJArray(json, "suggestions"); 330 for (int i = 0; i < array.size(); i++) { 331 res.getSuggestions().add(parseCDSHooksResponseCardsSuggestionsComponent(array.get(i).getAsJsonObject())); 332 } 333 }; 334 if (json.has("selectionBehavior")) 335 res.setSelectionBehaviorElement(parseEnumeration(json.get("selectionBehavior").getAsString(), CDSHooksResponse.CDSSelectionBehaviorCodesVS.NULL, new CDSHooksResponse.CDSSelectionBehaviorCodesVSEnumFactory())); 336 if (json.has("_selectionBehavior")) 337 parseElementProperties(getJObject(json, "_selectionBehavior"), res.getSelectionBehaviorElement()); 338 if (json.has("overrideReasons")) { 339 JsonArray array = getJArray(json, "overrideReasons"); 340 for (int i = 0; i < array.size(); i++) { 341 res.getOverrideReasons().add(parseCoding(array.get(i).getAsJsonObject())); 342 } 343 }; 344 if (json.has("links")) { 345 JsonArray array = getJArray(json, "links"); 346 for (int i = 0; i < array.size(); i++) { 347 res.getLinks().add(parseCDSHooksResponseCardsLinksComponent(array.get(i).getAsJsonObject())); 348 } 349 }; 350 } 351 352 protected CDSHooksResponse.CDSHooksResponseCardsSourceComponent parseCDSHooksResponseCardsSourceComponent(JsonObject json) throws IOException, FHIRFormatError { 353 CDSHooksResponse.CDSHooksResponseCardsSourceComponent res = new CDSHooksResponse.CDSHooksResponseCardsSourceComponent(); 354 parseCDSHooksResponseCardsSourceComponentProperties(json, res); 355 return res; 356 } 357 358 protected void parseCDSHooksResponseCardsSourceComponentProperties(JsonObject json, CDSHooksResponse.CDSHooksResponseCardsSourceComponent res) throws IOException, FHIRFormatError { 359 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 360 if (json.has("label")) 361 res.setLabelElement(parseString(json.get("label").getAsString())); 362 if (json.has("_label")) 363 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 364 if (json.has("url")) 365 res.setUrlElement(parseUrl(json.get("url").getAsString())); 366 if (json.has("_url")) 367 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 368 if (json.has("icon")) 369 res.setIconElement(parseUrl(json.get("icon").getAsString())); 370 if (json.has("_icon")) 371 parseElementProperties(getJObject(json, "_icon"), res.getIconElement()); 372 if (json.has("topic")) 373 res.setTopic(parseCoding(getJObject(json, "topic"))); 374 } 375 376 protected CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent parseCDSHooksResponseCardsSuggestionsComponent(JsonObject json) throws IOException, FHIRFormatError { 377 CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent res = new CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent(); 378 parseCDSHooksResponseCardsSuggestionsComponentProperties(json, res); 379 return res; 380 } 381 382 protected void parseCDSHooksResponseCardsSuggestionsComponentProperties(JsonObject json, CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent res) throws IOException, FHIRFormatError { 383 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 384 if (json.has("label")) 385 res.setLabelElement(parseString(json.get("label").getAsString())); 386 if (json.has("_label")) 387 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 388 if (json.has("uuid")) 389 res.setUuidElement(parseUuid(json.get("uuid").getAsString())); 390 if (json.has("_uuid")) 391 parseElementProperties(getJObject(json, "_uuid"), res.getUuidElement()); 392 if (json.has("isRecommended")) 393 res.setIsRecommendedElement(parseBoolean(json.get("isRecommended").getAsBoolean())); 394 if (json.has("_isRecommended")) 395 parseElementProperties(getJObject(json, "_isRecommended"), res.getIsRecommendedElement()); 396 if (json.has("actions")) { 397 JsonArray array = getJArray(json, "actions"); 398 for (int i = 0; i < array.size(); i++) { 399 res.getActions().add(parseCDSHooksResponseCardsSuggestionsActionsComponent(array.get(i).getAsJsonObject())); 400 } 401 }; 402 } 403 404 protected CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent parseCDSHooksResponseCardsSuggestionsActionsComponent(JsonObject json) throws IOException, FHIRFormatError { 405 CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent res = new CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent(); 406 parseCDSHooksResponseCardsSuggestionsActionsComponentProperties(json, res); 407 return res; 408 } 409 410 protected void parseCDSHooksResponseCardsSuggestionsActionsComponentProperties(JsonObject json, CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent res) throws IOException, FHIRFormatError { 411 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 412 if (json.has("type")) 413 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), CDSHooksResponse.CDSActionTypeCodesVS.NULL, new CDSHooksResponse.CDSActionTypeCodesVSEnumFactory())); 414 if (json.has("_type")) 415 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 416 if (json.has("description")) 417 res.setDescriptionElement(parseString(json.get("description").getAsString())); 418 if (json.has("_description")) 419 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 420 if (json.has("resource")) 421 res.setResource(parseResource(getJObject(json, "resource"))); 422 if (json.has("resourceId")) 423 res.setResourceIdElement(parseUrl(json.get("resourceId").getAsString())); 424 if (json.has("_resourceId")) 425 parseElementProperties(getJObject(json, "_resourceId"), res.getResourceIdElement()); 426 } 427 428 protected CDSHooksResponse.CDSHooksResponseCardsLinksComponent parseCDSHooksResponseCardsLinksComponent(JsonObject json) throws IOException, FHIRFormatError { 429 CDSHooksResponse.CDSHooksResponseCardsLinksComponent res = new CDSHooksResponse.CDSHooksResponseCardsLinksComponent(); 430 parseCDSHooksResponseCardsLinksComponentProperties(json, res); 431 return res; 432 } 433 434 protected void parseCDSHooksResponseCardsLinksComponentProperties(JsonObject json, CDSHooksResponse.CDSHooksResponseCardsLinksComponent res) throws IOException, FHIRFormatError { 435 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 436 if (json.has("label")) 437 res.setLabelElement(parseString(json.get("label").getAsString())); 438 if (json.has("_label")) 439 parseElementProperties(getJObject(json, "_label"), res.getLabelElement()); 440 if (json.has("url")) 441 res.setUrlElement(parseUrl(json.get("url").getAsString())); 442 if (json.has("_url")) 443 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 444 if (json.has("type")) 445 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), CDSHooksResponse.CDSLinkTypeCodesVS.NULL, new CDSHooksResponse.CDSLinkTypeCodesVSEnumFactory())); 446 if (json.has("_type")) 447 parseElementProperties(getJObject(json, "_type"), res.getTypeElement()); 448 if (json.has("appContext")) 449 res.setAppContextElement(parseString(json.get("appContext").getAsString())); 450 if (json.has("_appContext")) 451 parseElementProperties(getJObject(json, "_appContext"), res.getAppContextElement()); 452 } 453 454 protected CDSHooksServices parseCDSHooksServices(JsonObject json) throws IOException, FHIRFormatError { 455 CDSHooksServices res = new CDSHooksServices(); 456 parseCDSHooksServicesProperties(json, res); 457 return res; 458 } 459 460 protected void parseCDSHooksServicesProperties(JsonObject json, CDSHooksServices res) throws IOException, FHIRFormatError { 461 parseCDSHooksElementProperties(json, res); 462 if (json.has("services")) { 463 JsonArray array = getJArray(json, "services"); 464 for (int i = 0; i < array.size(); i++) { 465 res.getServices().add(parseCDSHooksServicesServicesComponent(array.get(i).getAsJsonObject())); 466 } 467 }; 468 } 469 470 protected CDSHooksServices.CDSHooksServicesServicesComponent parseCDSHooksServicesServicesComponent(JsonObject json) throws IOException, FHIRFormatError { 471 CDSHooksServices.CDSHooksServicesServicesComponent res = new CDSHooksServices.CDSHooksServicesServicesComponent(); 472 parseCDSHooksServicesServicesComponentProperties(json, res); 473 return res; 474 } 475 476 protected void parseCDSHooksServicesServicesComponentProperties(JsonObject json, CDSHooksServices.CDSHooksServicesServicesComponent res) throws IOException, FHIRFormatError { 477 parsehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(json, res); 478 if (json.has("hook")) 479 res.setHookElement(parseString(json.get("hook").getAsString())); 480 if (json.has("_hook")) 481 parseElementProperties(getJObject(json, "_hook"), res.getHookElement()); 482 if (json.has("title")) 483 res.setTitleElement(parseString(json.get("title").getAsString())); 484 if (json.has("_title")) 485 parseElementProperties(getJObject(json, "_title"), res.getTitleElement()); 486 if (json.has("id")) 487 res.setIdElement(parseCode(json.get("id").getAsString())); 488 if (json.has("_id")) 489 parseElementProperties(getJObject(json, "_id"), res.getIdElement()); 490 if (json.has("description")) 491 res.setDescriptionElement(parseString(json.get("description").getAsString())); 492 if (json.has("_description")) 493 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 494 if (json.has("usageRequirements")) 495 res.setUsageRequirementsElement(parseString(json.get("usageRequirements").getAsString())); 496 if (json.has("_usageRequirements")) 497 parseElementProperties(getJObject(json, "_usageRequirements"), res.getUsageRequirementsElement()); 498 if (json.has("prefetch")) { 499 JsonArray array = getJArray(json, "prefetch"); 500 for (int i = 0; i < array.size(); i++) { 501 res.getPrefetch().add(parseCDSHooksServicesServicesPrefetchComponent(array.get(i).getAsJsonObject())); 502 } 503 }; 504 } 505 506 protected CDSHooksServices.CDSHooksServicesServicesPrefetchComponent parseCDSHooksServicesServicesPrefetchComponent(JsonObject json) throws IOException, FHIRFormatError { 507 CDSHooksServices.CDSHooksServicesServicesPrefetchComponent res = new CDSHooksServices.CDSHooksServicesServicesPrefetchComponent(); 508 parseCDSHooksServicesServicesPrefetchComponentProperties(json, res); 509 return res; 510 } 511 512 protected void parseCDSHooksServicesServicesPrefetchComponentProperties(JsonObject json, CDSHooksServices.CDSHooksServicesServicesPrefetchComponent res) throws IOException, FHIRFormatError { 513 parseBaseProperties(json, res); 514 if (json.has("key")) 515 res.setKeyElement(parseCode(json.get("key").getAsString())); 516 if (json.has("_key")) 517 parseElementProperties(getJObject(json, "_key"), res.getKeyElement()); 518 if (json.has("value")) 519 res.setValueElement(parseString(json.get("value").getAsString())); 520 if (json.has("_value")) 521 parseElementProperties(getJObject(json, "_value"), res.getValueElement()); 522 } 523 524 protected TestCases parseTestCases(JsonObject json) throws IOException, FHIRFormatError { 525 TestCases res = new TestCases(); 526 parseTestCasesProperties(json, res); 527 return res; 528 } 529 530 protected void parseTestCasesProperties(JsonObject json, TestCases res) throws IOException, FHIRFormatError { 531 parseResourceProperties(json, res); 532 if (json.has("url")) 533 res.setUrlElement(parseUri(json.get("url").getAsString())); 534 if (json.has("_url")) 535 parseElementProperties(getJObject(json, "_url"), res.getUrlElement()); 536 if (json.has("version")) 537 res.setVersionElement(parseString(json.get("version").getAsString())); 538 if (json.has("_version")) 539 parseElementProperties(getJObject(json, "_version"), res.getVersionElement()); 540 if (json.has("name")) 541 res.setNameElement(parseString(json.get("name").getAsString())); 542 if (json.has("_name")) 543 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 544 if (json.has("description")) 545 res.setDescriptionElement(parseMarkdown(json.get("description").getAsString())); 546 if (json.has("_description")) 547 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 548 if (json.has("runner")) 549 res.setRunnerElement(parseUrl(json.get("runner").getAsString())); 550 if (json.has("_runner")) 551 parseElementProperties(getJObject(json, "_runner"), res.getRunnerElement()); 552 if (json.has("mode")) { 553 JsonArray array = getJArray(json, "mode"); 554 for (int i = 0; i < array.size(); i++) { 555 res.getMode().add(parseTestCasesModeComponent(array.get(i).getAsJsonObject())); 556 } 557 }; 558 if (json.has("suite")) { 559 JsonArray array = getJArray(json, "suite"); 560 for (int i = 0; i < array.size(); i++) { 561 res.getSuite().add(parseTestCasesSuiteComponent(array.get(i).getAsJsonObject())); 562 } 563 }; 564 } 565 566 protected TestCases.TestCasesModeComponent parseTestCasesModeComponent(JsonObject json) throws IOException, FHIRFormatError { 567 TestCases.TestCasesModeComponent res = new TestCases.TestCasesModeComponent(); 568 parseTestCasesModeComponentProperties(json, res); 569 return res; 570 } 571 572 protected void parseTestCasesModeComponentProperties(JsonObject json, TestCases.TestCasesModeComponent res) throws IOException, FHIRFormatError { 573 parseBaseProperties(json, res); 574 if (json.has("code")) 575 res.setCodeElement(parseString(json.get("code").getAsString())); 576 if (json.has("_code")) 577 parseElementProperties(getJObject(json, "_code"), res.getCodeElement()); 578 if (json.has("description")) 579 res.setDescriptionElement(parseString(json.get("description").getAsString())); 580 if (json.has("_description")) 581 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 582 } 583 584 protected TestCases.TestCasesSuiteComponent parseTestCasesSuiteComponent(JsonObject json) throws IOException, FHIRFormatError { 585 TestCases.TestCasesSuiteComponent res = new TestCases.TestCasesSuiteComponent(); 586 parseTestCasesSuiteComponentProperties(json, res); 587 return res; 588 } 589 590 protected void parseTestCasesSuiteComponentProperties(JsonObject json, TestCases.TestCasesSuiteComponent res) throws IOException, FHIRFormatError { 591 parseBaseProperties(json, res); 592 if (json.has("name")) 593 res.setNameElement(parseString(json.get("name").getAsString())); 594 if (json.has("_name")) 595 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 596 if (json.has("description")) 597 res.setDescriptionElement(parseString(json.get("description").getAsString())); 598 if (json.has("_description")) 599 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 600 if (json.has("mode")) 601 res.setModeElement(parseCode(json.get("mode").getAsString())); 602 if (json.has("_mode")) 603 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 604 if (json.has("resource")) { 605 JsonArray array = getJArray(json, "resource"); 606 for (int i = 0; i < array.size(); i++) { 607 res.getResource().add(parseTestCasesSuiteResourceComponent(array.get(i).getAsJsonObject())); 608 } 609 }; 610 if (json.has("parameter")) { 611 JsonArray array = getJArray(json, "parameter"); 612 for (int i = 0; i < array.size(); i++) { 613 res.getParameter().add(parseTestCasesSuiteParameterComponent(array.get(i).getAsJsonObject())); 614 } 615 }; 616 if (json.has("test")) { 617 JsonArray array = getJArray(json, "test"); 618 for (int i = 0; i < array.size(); i++) { 619 res.getTest().add(parseTestCasesSuiteTestComponent(array.get(i).getAsJsonObject())); 620 } 621 }; 622 } 623 624 protected TestCases.TestCasesSuiteResourceComponent parseTestCasesSuiteResourceComponent(JsonObject json) throws IOException, FHIRFormatError { 625 TestCases.TestCasesSuiteResourceComponent res = new TestCases.TestCasesSuiteResourceComponent(); 626 parseTestCasesSuiteResourceComponentProperties(json, res); 627 return res; 628 } 629 630 protected void parseTestCasesSuiteResourceComponentProperties(JsonObject json, TestCases.TestCasesSuiteResourceComponent res) throws IOException, FHIRFormatError { 631 parseBaseProperties(json, res); 632 if (json.has("name")) 633 res.setNameElement(parseString(json.get("name").getAsString())); 634 if (json.has("_name")) 635 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 636 if (json.has("file")) 637 res.setFileElement(parseString(json.get("file").getAsString())); 638 if (json.has("_file")) 639 parseElementProperties(getJObject(json, "_file"), res.getFileElement()); 640 if (json.has("resource")) 641 res.setResource(parseResource(getJObject(json, "resource"))); 642 if (json.has("mode")) 643 res.setModeElement(parseCode(json.get("mode").getAsString())); 644 if (json.has("_mode")) 645 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 646 } 647 648 protected TestCases.TestCasesSuiteParameterComponent parseTestCasesSuiteParameterComponent(JsonObject json) throws IOException, FHIRFormatError { 649 TestCases.TestCasesSuiteParameterComponent res = new TestCases.TestCasesSuiteParameterComponent(); 650 parseTestCasesSuiteParameterComponentProperties(json, res); 651 return res; 652 } 653 654 protected void parseTestCasesSuiteParameterComponentProperties(JsonObject json, TestCases.TestCasesSuiteParameterComponent res) throws IOException, FHIRFormatError { 655 parseBaseProperties(json, res); 656 if (json.has("name")) 657 res.setNameElement(parseString(json.get("name").getAsString())); 658 if (json.has("_name")) 659 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 660 DataType value = parseType("value", json); 661 if (value != null) 662 res.setValue(value); 663 if (json.has("mode")) 664 res.setModeElement(parseCode(json.get("mode").getAsString())); 665 if (json.has("_mode")) 666 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 667 } 668 669 protected TestCases.TestCasesSuiteTestComponent parseTestCasesSuiteTestComponent(JsonObject json) throws IOException, FHIRFormatError { 670 TestCases.TestCasesSuiteTestComponent res = new TestCases.TestCasesSuiteTestComponent(); 671 parseTestCasesSuiteTestComponentProperties(json, res); 672 return res; 673 } 674 675 protected void parseTestCasesSuiteTestComponentProperties(JsonObject json, TestCases.TestCasesSuiteTestComponent res) throws IOException, FHIRFormatError { 676 parseBaseProperties(json, res); 677 if (json.has("name")) 678 res.setNameElement(parseString(json.get("name").getAsString())); 679 if (json.has("_name")) 680 parseElementProperties(getJObject(json, "_name"), res.getNameElement()); 681 if (json.has("description")) 682 res.setDescriptionElement(parseString(json.get("description").getAsString())); 683 if (json.has("_description")) 684 parseElementProperties(getJObject(json, "_description"), res.getDescriptionElement()); 685 if (json.has("operation")) 686 res.setOperationElement(parseCode(json.get("operation").getAsString())); 687 if (json.has("_operation")) 688 parseElementProperties(getJObject(json, "_operation"), res.getOperationElement()); 689 if (json.has("mode")) 690 res.setModeElement(parseString(json.get("mode").getAsString())); 691 if (json.has("_mode")) 692 parseElementProperties(getJObject(json, "_mode"), res.getModeElement()); 693 if (json.has("parameter")) { 694 JsonArray array = getJArray(json, "parameter"); 695 for (int i = 0; i < array.size(); i++) { 696 res.getParameter().add(parseTestCasesSuiteParameterComponent(array.get(i).getAsJsonObject())); 697 } 698 }; 699 if (json.has("input")) { 700 JsonArray array = getJArray(json, "input"); 701 for (int i = 0; i < array.size(); i++) { 702 res.getInput().add(parseTestCasesSuiteResourceComponent(array.get(i).getAsJsonObject())); 703 } 704 }; 705 if (json.has("output")) { 706 JsonArray array = getJArray(json, "output"); 707 for (int i = 0; i < array.size(); i++) { 708 res.getOutput().add(parseTestCasesSuiteResourceComponent(array.get(i).getAsJsonObject())); 709 } 710 }; 711 } 712 713 714 715 716// -- compose --------------------------------------------------------------------------------------------------------------------- 717 718 719 protected void composeCDSHookContextProperties(CDSHookContext element) throws IOException { 720 composeBaseProperties(element); 721 } 722 723 protected void composeCDSHooksElementProperties(CDSHooksElement element) throws IOException { 724 composeBaseProperties(element); 725 if (element.hasExtension()) { 726 composeCDSHooksExtensions("extension", element.getExtension()); 727 } 728 } 729 730 protected void composeCDSHookOrderSelectContext(String name, CDSHookOrderSelectContext element) throws IOException { 731 if (element != null) { 732 open(name); 733 composeCDSHookOrderSelectContextProperties(element); 734 close(); 735 } 736 } 737 738 protected void composeCDSHookOrderSelectContextProperties(CDSHookOrderSelectContext element) throws IOException { 739 composeCDSHookContextProperties(element); 740 if (element.hasUserIdElement()) { 741 composeUrlCore("userId", element.getUserIdElement(), false); 742 composeUrlExtras("userId", element.getUserIdElement(), false); 743 } 744 if (element.hasPatientIdElement()) { 745 composeIdCore("patientId", element.getPatientIdElement(), false); 746 composeIdExtras("patientId", element.getPatientIdElement(), false); 747 } 748 if (element.hasEncounterIdElement()) { 749 composeIdCore("encounterId", element.getEncounterIdElement(), false); 750 composeIdExtras("encounterId", element.getEncounterIdElement(), false); 751 } 752 if (element.hasSelections()) { 753 if (anyHasValue(element.getSelections())) { 754 openArray("selections"); 755 for (UriType e : element.getSelections()) 756 composeUriCore(null, e, e != element.getSelections().get(element.getSelections().size()-1)); 757 closeArray(); 758 } 759 if (anyHasExtras(element.getSelections())) { 760 openArray("_selections"); 761 for (UriType e : element.getSelections()) 762 composeUriExtras(null, e, true); 763 closeArray(); 764 } 765 }; 766 if (element.hasDraftOrders()) { 767 composeBundle("draftOrders", element.getDraftOrders()); 768 } 769 } 770 771 protected void composeCDSHookOrderSignContext(String name, CDSHookOrderSignContext element) throws IOException { 772 if (element != null) { 773 open(name); 774 composeCDSHookOrderSignContextProperties(element); 775 close(); 776 } 777 } 778 779 protected void composeCDSHookOrderSignContextProperties(CDSHookOrderSignContext element) throws IOException { 780 composeCDSHookContextProperties(element); 781 if (element.hasUserIdElement()) { 782 composeUrlCore("userId", element.getUserIdElement(), false); 783 composeUrlExtras("userId", element.getUserIdElement(), false); 784 } 785 if (element.hasPatientIdElement()) { 786 composeIdCore("patientId", element.getPatientIdElement(), false); 787 composeIdExtras("patientId", element.getPatientIdElement(), false); 788 } 789 if (element.hasEncounterIdElement()) { 790 composeIdCore("encounterId", element.getEncounterIdElement(), false); 791 composeIdExtras("encounterId", element.getEncounterIdElement(), false); 792 } 793 if (element.hasDraftOrders()) { 794 composeBundle("draftOrders", element.getDraftOrders()); 795 } 796 } 797 798 protected void composeCDSHookPatientViewContext(String name, CDSHookPatientViewContext element) throws IOException { 799 if (element != null) { 800 open(name); 801 composeCDSHookPatientViewContextProperties(element); 802 close(); 803 } 804 } 805 806 protected void composeCDSHookPatientViewContextProperties(CDSHookPatientViewContext element) throws IOException { 807 composeCDSHookContextProperties(element); 808 if (element.hasUserIdElement()) { 809 composeUrlCore("userId", element.getUserIdElement(), false); 810 composeUrlExtras("userId", element.getUserIdElement(), false); 811 } 812 if (element.hasPatientIdElement()) { 813 composeIdCore("patientId", element.getPatientIdElement(), false); 814 composeIdExtras("patientId", element.getPatientIdElement(), false); 815 } 816 if (element.hasEncounterIdElement()) { 817 composeIdCore("encounterId", element.getEncounterIdElement(), false); 818 composeIdExtras("encounterId", element.getEncounterIdElement(), false); 819 } 820 } 821 822 protected void composeCDSHooksExtensions(String name, CDSHooksExtensions element) throws IOException { 823 if (element != null) { 824 open(name); 825 composeCDSHooksExtensionsProperties(element); 826 close(); 827 } 828 } 829 830 protected void composeCDSHooksExtensionsProperties(CDSHooksExtensions element) throws IOException { 831 composeElementProperties(element); 832 } 833 834 protected void composeCDSHooksRequest(String name, CDSHooksRequest element) throws IOException { 835 if (element != null) { 836 open(name); 837 composeCDSHooksRequestProperties(element); 838 close(); 839 } 840 } 841 842 protected void composeCDSHooksRequestProperties(CDSHooksRequest element) throws IOException { 843 composeCDSHooksElementProperties(element); 844 if (element.hasHookElement()) { 845 composeCodeCore("hook", element.getHookElement(), false); 846 composeCodeExtras("hook", element.getHookElement(), false); 847 } 848 if (element.hasHookInstanceElement()) { 849 composeUuidCore("hookInstance", element.getHookInstanceElement(), false); 850 composeUuidExtras("hookInstance", element.getHookInstanceElement(), false); 851 } 852 if (element.hasFhirServerElement()) { 853 composeUrlCore("fhirServer", element.getFhirServerElement(), false); 854 composeUrlExtras("fhirServer", element.getFhirServerElement(), false); 855 } 856 if (element.hasFhirAuthorization()) { 857 composeCDSHooksRequestFhirAuthorizationComponent("fhirAuthorization", element.getFhirAuthorization()); 858 } 859 if (element.hasContext()) { 860 composeCDSHookContext("context", element.getContext()); 861 } 862 if (element.hasPrefetch()) { 863 openArray("prefetch"); 864 for (CDSHooksRequest.CDSHooksRequestPrefetchComponent e : element.getPrefetch()) 865 composeCDSHooksRequestPrefetchComponent(null, e); 866 closeArray(); 867 }; 868 } 869 870 protected void composeCDSHookContext(String name, CDSHookContext element) throws IOException { 871 if (element instanceof CDSHookPatientViewContext) { 872 composeCDSHookPatientViewContext(name, (CDSHookPatientViewContext) element); 873 } else if (element instanceof CDSHookOrderSignContext) { 874 composeCDSHookOrderSignContext(name, (CDSHookOrderSignContext) element); 875 } else if (element instanceof CDSHookOrderSelectContext) { 876 composeCDSHookOrderSelectContext(name, (CDSHookOrderSelectContext) element); 877 } else { 878 throw new FHIRFormatError("Unable to compose CDSHookContext: Unexpected type "+element.getClass().getName()); 879 } 880 } 881 882 protected void composeCDSHooksRequestFhirAuthorizationComponent(String name, CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent element) throws IOException { 883 if (element != null) { 884 open(name); 885 composeCDSHooksRequestFhirAuthorizationComponentProperties(element); 886 close(); 887 } 888 } 889 890 protected void composeCDSHooksRequestFhirAuthorizationComponentProperties(CDSHooksRequest.CDSHooksRequestFhirAuthorizationComponent element) throws IOException { 891 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 892 if (element.hasAccessTokenElement()) { 893 composeStringCore("accessToken", element.getAccessTokenElement(), false); 894 composeStringExtras("accessToken", element.getAccessTokenElement(), false); 895 } 896 if (element.hasTokenTypeElement()) { 897 composeCodeCore("tokenType", element.getTokenTypeElement(), false); 898 composeCodeExtras("tokenType", element.getTokenTypeElement(), false); 899 } 900 if (element.hasExpiresInElement()) { 901 composeIntegerCore("expiresIn", element.getExpiresInElement(), false); 902 composeIntegerExtras("expiresIn", element.getExpiresInElement(), false); 903 } 904 if (element.hasScopeElement()) { 905 composeStringCore("scope", element.getScopeElement(), false); 906 composeStringExtras("scope", element.getScopeElement(), false); 907 } 908 if (element.hasSubjectElement()) { 909 composeStringCore("subject", element.getSubjectElement(), false); 910 composeStringExtras("subject", element.getSubjectElement(), false); 911 } 912 if (element.hasPatientElement()) { 913 composeIdCore("patient", element.getPatientElement(), false); 914 composeIdExtras("patient", element.getPatientElement(), false); 915 } 916 } 917 918 protected void composeCDSHooksRequestPrefetchComponent(String name, CDSHooksRequest.CDSHooksRequestPrefetchComponent element) throws IOException { 919 if (element != null) { 920 open(name); 921 composeCDSHooksRequestPrefetchComponentProperties(element); 922 close(); 923 } 924 } 925 926 protected void composeCDSHooksRequestPrefetchComponentProperties(CDSHooksRequest.CDSHooksRequestPrefetchComponent element) throws IOException { 927 composeBaseProperties(element); 928 if (element.hasKeyElement()) { 929 composeCodeCore("key", element.getKeyElement(), false); 930 composeCodeExtras("key", element.getKeyElement(), false); 931 } 932 if (element.hasValue()) { 933 open("value"); 934 composeResource(element.getValue()); 935 close(); 936 } 937 } 938 939 protected void composeCDSHooksResponse(String name, CDSHooksResponse element) throws IOException { 940 if (element != null) { 941 open(name); 942 composeCDSHooksResponseProperties(element); 943 close(); 944 } 945 } 946 947 protected void composeCDSHooksResponseProperties(CDSHooksResponse element) throws IOException { 948 composeCDSHooksElementProperties(element); 949 if (element.hasCards()) { 950 openArray("cards"); 951 for (CDSHooksResponse.CDSHooksResponseCardsComponent e : element.getCards()) 952 composeCDSHooksResponseCardsComponent(null, e); 953 closeArray(); 954 }; 955 if (element.hasSystemActions()) { 956 openArray("systemActions"); 957 for (CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent e : element.getSystemActions()) 958 composeCDSHooksResponseCardsSuggestionsActionsComponent(null, e); 959 closeArray(); 960 }; 961 } 962 963 protected void composeCDSHooksResponseCardsComponent(String name, CDSHooksResponse.CDSHooksResponseCardsComponent element) throws IOException { 964 if (element != null) { 965 open(name); 966 composeCDSHooksResponseCardsComponentProperties(element); 967 close(); 968 } 969 } 970 971 protected void composeCDSHooksResponseCardsComponentProperties(CDSHooksResponse.CDSHooksResponseCardsComponent element) throws IOException { 972 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 973 if (element.hasUuidElement()) { 974 composeUuidCore("uuid", element.getUuidElement(), false); 975 composeUuidExtras("uuid", element.getUuidElement(), false); 976 } 977 if (element.hasSummaryElement()) { 978 composeStringCore("summary", element.getSummaryElement(), false); 979 composeStringExtras("summary", element.getSummaryElement(), false); 980 } 981 if (element.hasDetailElement()) { 982 composeMarkdownCore("detail", element.getDetailElement(), false); 983 composeMarkdownExtras("detail", element.getDetailElement(), false); 984 } 985 if (element.hasIndicatorElement()) { 986 composeEnumerationCore("indicator", element.getIndicatorElement(), new CDSHooksResponse.CDSIndicatorCodesVSEnumFactory(), false); 987 composeEnumerationExtras("indicator", element.getIndicatorElement(), new CDSHooksResponse.CDSIndicatorCodesVSEnumFactory(), false); 988 } 989 if (element.hasSource()) { 990 composeCDSHooksResponseCardsSourceComponent("source", element.getSource()); 991 } 992 if (element.hasSuggestions()) { 993 openArray("suggestions"); 994 for (CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent e : element.getSuggestions()) 995 composeCDSHooksResponseCardsSuggestionsComponent(null, e); 996 closeArray(); 997 }; 998 if (element.hasSelectionBehaviorElement()) { 999 composeEnumerationCore("selectionBehavior", element.getSelectionBehaviorElement(), new CDSHooksResponse.CDSSelectionBehaviorCodesVSEnumFactory(), false); 1000 composeEnumerationExtras("selectionBehavior", element.getSelectionBehaviorElement(), new CDSHooksResponse.CDSSelectionBehaviorCodesVSEnumFactory(), false); 1001 } 1002 if (element.hasOverrideReasons()) { 1003 openArray("overrideReasons"); 1004 for (Coding e : element.getOverrideReasons()) 1005 composeCoding(null, e); 1006 closeArray(); 1007 }; 1008 if (element.hasLinks()) { 1009 openArray("links"); 1010 for (CDSHooksResponse.CDSHooksResponseCardsLinksComponent e : element.getLinks()) 1011 composeCDSHooksResponseCardsLinksComponent(null, e); 1012 closeArray(); 1013 }; 1014 } 1015 1016 protected void composeCDSHooksResponseCardsSourceComponent(String name, CDSHooksResponse.CDSHooksResponseCardsSourceComponent element) throws IOException { 1017 if (element != null) { 1018 open(name); 1019 composeCDSHooksResponseCardsSourceComponentProperties(element); 1020 close(); 1021 } 1022 } 1023 1024 protected void composeCDSHooksResponseCardsSourceComponentProperties(CDSHooksResponse.CDSHooksResponseCardsSourceComponent element) throws IOException { 1025 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 1026 if (element.hasLabelElement()) { 1027 composeStringCore("label", element.getLabelElement(), false); 1028 composeStringExtras("label", element.getLabelElement(), false); 1029 } 1030 if (element.hasUrlElement()) { 1031 composeUrlCore("url", element.getUrlElement(), false); 1032 composeUrlExtras("url", element.getUrlElement(), false); 1033 } 1034 if (element.hasIconElement()) { 1035 composeUrlCore("icon", element.getIconElement(), false); 1036 composeUrlExtras("icon", element.getIconElement(), false); 1037 } 1038 if (element.hasTopic()) { 1039 composeCoding("topic", element.getTopic()); 1040 } 1041 } 1042 1043 protected void composeCDSHooksResponseCardsSuggestionsComponent(String name, CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent element) throws IOException { 1044 if (element != null) { 1045 open(name); 1046 composeCDSHooksResponseCardsSuggestionsComponentProperties(element); 1047 close(); 1048 } 1049 } 1050 1051 protected void composeCDSHooksResponseCardsSuggestionsComponentProperties(CDSHooksResponse.CDSHooksResponseCardsSuggestionsComponent element) throws IOException { 1052 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 1053 if (element.hasLabelElement()) { 1054 composeStringCore("label", element.getLabelElement(), false); 1055 composeStringExtras("label", element.getLabelElement(), false); 1056 } 1057 if (element.hasUuidElement()) { 1058 composeUuidCore("uuid", element.getUuidElement(), false); 1059 composeUuidExtras("uuid", element.getUuidElement(), false); 1060 } 1061 if (element.hasIsRecommendedElement()) { 1062 composeBooleanCore("isRecommended", element.getIsRecommendedElement(), false); 1063 composeBooleanExtras("isRecommended", element.getIsRecommendedElement(), false); 1064 } 1065 if (element.hasActions()) { 1066 openArray("actions"); 1067 for (CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent e : element.getActions()) 1068 composeCDSHooksResponseCardsSuggestionsActionsComponent(null, e); 1069 closeArray(); 1070 }; 1071 } 1072 1073 protected void composeCDSHooksResponseCardsSuggestionsActionsComponent(String name, CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent element) throws IOException { 1074 if (element != null) { 1075 open(name); 1076 composeCDSHooksResponseCardsSuggestionsActionsComponentProperties(element); 1077 close(); 1078 } 1079 } 1080 1081 protected void composeCDSHooksResponseCardsSuggestionsActionsComponentProperties(CDSHooksResponse.CDSHooksResponseCardsSuggestionsActionsComponent element) throws IOException { 1082 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 1083 if (element.hasTypeElement()) { 1084 composeEnumerationCore("type", element.getTypeElement(), new CDSHooksResponse.CDSActionTypeCodesVSEnumFactory(), false); 1085 composeEnumerationExtras("type", element.getTypeElement(), new CDSHooksResponse.CDSActionTypeCodesVSEnumFactory(), false); 1086 } 1087 if (element.hasDescriptionElement()) { 1088 composeStringCore("description", element.getDescriptionElement(), false); 1089 composeStringExtras("description", element.getDescriptionElement(), false); 1090 } 1091 if (element.hasResource()) { 1092 open("resource"); 1093 composeResource(element.getResource()); 1094 close(); 1095 } 1096 if (element.hasResourceIdElement()) { 1097 composeUrlCore("resourceId", element.getResourceIdElement(), false); 1098 composeUrlExtras("resourceId", element.getResourceIdElement(), false); 1099 } 1100 } 1101 1102 protected void composeCDSHooksResponseCardsLinksComponent(String name, CDSHooksResponse.CDSHooksResponseCardsLinksComponent element) throws IOException { 1103 if (element != null) { 1104 open(name); 1105 composeCDSHooksResponseCardsLinksComponentProperties(element); 1106 close(); 1107 } 1108 } 1109 1110 protected void composeCDSHooksResponseCardsLinksComponentProperties(CDSHooksResponse.CDSHooksResponseCardsLinksComponent element) throws IOException { 1111 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 1112 if (element.hasLabelElement()) { 1113 composeStringCore("label", element.getLabelElement(), false); 1114 composeStringExtras("label", element.getLabelElement(), false); 1115 } 1116 if (element.hasUrlElement()) { 1117 composeUrlCore("url", element.getUrlElement(), false); 1118 composeUrlExtras("url", element.getUrlElement(), false); 1119 } 1120 if (element.hasTypeElement()) { 1121 composeEnumerationCore("type", element.getTypeElement(), new CDSHooksResponse.CDSLinkTypeCodesVSEnumFactory(), false); 1122 composeEnumerationExtras("type", element.getTypeElement(), new CDSHooksResponse.CDSLinkTypeCodesVSEnumFactory(), false); 1123 } 1124 if (element.hasAppContextElement()) { 1125 composeStringCore("appContext", element.getAppContextElement(), false); 1126 composeStringExtras("appContext", element.getAppContextElement(), false); 1127 } 1128 } 1129 1130 protected void composeCDSHooksServices(String name, CDSHooksServices element) throws IOException { 1131 if (element != null) { 1132 open(name); 1133 composeCDSHooksServicesProperties(element); 1134 close(); 1135 } 1136 } 1137 1138 protected void composeCDSHooksServicesProperties(CDSHooksServices element) throws IOException { 1139 composeCDSHooksElementProperties(element); 1140 if (element.hasServices()) { 1141 openArray("services"); 1142 for (CDSHooksServices.CDSHooksServicesServicesComponent e : element.getServices()) 1143 composeCDSHooksServicesServicesComponent(null, e); 1144 closeArray(); 1145 }; 1146 } 1147 1148 protected void composeCDSHooksServicesServicesComponent(String name, CDSHooksServices.CDSHooksServicesServicesComponent element) throws IOException { 1149 if (element != null) { 1150 open(name); 1151 composeCDSHooksServicesServicesComponentProperties(element); 1152 close(); 1153 } 1154 } 1155 1156 protected void composeCDSHooksServicesServicesComponentProperties(CDSHooksServices.CDSHooksServicesServicesComponent element) throws IOException { 1157 composehttp://hl7.org/fhir/tools/StructureDefinition/CDSHooksElementProperties(element); 1158 if (element.hasHookElement()) { 1159 composeStringCore("hook", element.getHookElement(), false); 1160 composeStringExtras("hook", element.getHookElement(), false); 1161 } 1162 if (element.hasTitleElement()) { 1163 composeStringCore("title", element.getTitleElement(), false); 1164 composeStringExtras("title", element.getTitleElement(), false); 1165 } 1166 if (element.hasIdElement()) { 1167 composeCodeCore("id", element.getIdElement(), false); 1168 composeCodeExtras("id", element.getIdElement(), false); 1169 } 1170 if (element.hasDescriptionElement()) { 1171 composeStringCore("description", element.getDescriptionElement(), false); 1172 composeStringExtras("description", element.getDescriptionElement(), false); 1173 } 1174 if (element.hasUsageRequirementsElement()) { 1175 composeStringCore("usageRequirements", element.getUsageRequirementsElement(), false); 1176 composeStringExtras("usageRequirements", element.getUsageRequirementsElement(), false); 1177 } 1178 if (element.hasPrefetch()) { 1179 openArray("prefetch"); 1180 for (CDSHooksServices.CDSHooksServicesServicesPrefetchComponent e : element.getPrefetch()) 1181 composeCDSHooksServicesServicesPrefetchComponent(null, e); 1182 closeArray(); 1183 }; 1184 } 1185 1186 protected void composeCDSHooksServicesServicesPrefetchComponent(String name, CDSHooksServices.CDSHooksServicesServicesPrefetchComponent element) throws IOException { 1187 if (element != null) { 1188 open(name); 1189 composeCDSHooksServicesServicesPrefetchComponentProperties(element); 1190 close(); 1191 } 1192 } 1193 1194 protected void composeCDSHooksServicesServicesPrefetchComponentProperties(CDSHooksServices.CDSHooksServicesServicesPrefetchComponent element) throws IOException { 1195 composeBaseProperties(element); 1196 if (element.hasKeyElement()) { 1197 composeCodeCore("key", element.getKeyElement(), false); 1198 composeCodeExtras("key", element.getKeyElement(), false); 1199 } 1200 if (element.hasValueElement()) { 1201 composeStringCore("value", element.getValueElement(), false); 1202 composeStringExtras("value", element.getValueElement(), false); 1203 } 1204 } 1205 1206 protected void composeTestCases(String name, TestCases element) throws IOException { 1207 if (element != null) { 1208 prop("resourceType", "TestCases"); 1209 composeTestCasesProperties(element); 1210 } 1211 } 1212 1213 protected void composeTestCasesProperties(TestCases element) throws IOException { 1214 composeResourceProperties(element); 1215 if (element.hasUrlElement()) { 1216 composeUriCore("url", element.getUrlElement(), false); 1217 composeUriExtras("url", element.getUrlElement(), false); 1218 } 1219 if (element.hasVersionElement()) { 1220 composeStringCore("version", element.getVersionElement(), false); 1221 composeStringExtras("version", element.getVersionElement(), false); 1222 } 1223 if (element.hasNameElement()) { 1224 composeStringCore("name", element.getNameElement(), false); 1225 composeStringExtras("name", element.getNameElement(), false); 1226 } 1227 if (element.hasDescriptionElement()) { 1228 composeMarkdownCore("description", element.getDescriptionElement(), false); 1229 composeMarkdownExtras("description", element.getDescriptionElement(), false); 1230 } 1231 if (element.hasRunnerElement()) { 1232 composeUrlCore("runner", element.getRunnerElement(), false); 1233 composeUrlExtras("runner", element.getRunnerElement(), false); 1234 } 1235 if (element.hasMode()) { 1236 openArray("mode"); 1237 for (TestCases.TestCasesModeComponent e : element.getMode()) 1238 composeTestCasesModeComponent(null, e); 1239 closeArray(); 1240 }; 1241 if (element.hasSuite()) { 1242 openArray("suite"); 1243 for (TestCases.TestCasesSuiteComponent e : element.getSuite()) 1244 composeTestCasesSuiteComponent(null, e); 1245 closeArray(); 1246 }; 1247 } 1248 1249 protected void composeTestCasesModeComponent(String name, TestCases.TestCasesModeComponent element) throws IOException { 1250 if (element != null) { 1251 open(name); 1252 composeTestCasesModeComponentProperties(element); 1253 close(); 1254 } 1255 } 1256 1257 protected void composeTestCasesModeComponentProperties(TestCases.TestCasesModeComponent element) throws IOException { 1258 composeBaseProperties(element); 1259 if (element.hasCodeElement()) { 1260 composeStringCore("code", element.getCodeElement(), false); 1261 composeStringExtras("code", element.getCodeElement(), false); 1262 } 1263 if (element.hasDescriptionElement()) { 1264 composeStringCore("description", element.getDescriptionElement(), false); 1265 composeStringExtras("description", element.getDescriptionElement(), false); 1266 } 1267 } 1268 1269 protected void composeTestCasesSuiteComponent(String name, TestCases.TestCasesSuiteComponent element) throws IOException { 1270 if (element != null) { 1271 open(name); 1272 composeTestCasesSuiteComponentProperties(element); 1273 close(); 1274 } 1275 } 1276 1277 protected void composeTestCasesSuiteComponentProperties(TestCases.TestCasesSuiteComponent element) throws IOException { 1278 composeBaseProperties(element); 1279 if (element.hasNameElement()) { 1280 composeStringCore("name", element.getNameElement(), false); 1281 composeStringExtras("name", element.getNameElement(), false); 1282 } 1283 if (element.hasDescriptionElement()) { 1284 composeStringCore("description", element.getDescriptionElement(), false); 1285 composeStringExtras("description", element.getDescriptionElement(), false); 1286 } 1287 if (element.hasModeElement()) { 1288 composeCodeCore("mode", element.getModeElement(), false); 1289 composeCodeExtras("mode", element.getModeElement(), false); 1290 } 1291 if (element.hasResource()) { 1292 openArray("resource"); 1293 for (TestCases.TestCasesSuiteResourceComponent e : element.getResource()) 1294 composeTestCasesSuiteResourceComponent(null, e); 1295 closeArray(); 1296 }; 1297 if (element.hasParameter()) { 1298 openArray("parameter"); 1299 for (TestCases.TestCasesSuiteParameterComponent e : element.getParameter()) 1300 composeTestCasesSuiteParameterComponent(null, e); 1301 closeArray(); 1302 }; 1303 if (element.hasTest()) { 1304 openArray("test"); 1305 for (TestCases.TestCasesSuiteTestComponent e : element.getTest()) 1306 composeTestCasesSuiteTestComponent(null, e); 1307 closeArray(); 1308 }; 1309 } 1310 1311 protected void composeTestCasesSuiteResourceComponent(String name, TestCases.TestCasesSuiteResourceComponent element) throws IOException { 1312 if (element != null) { 1313 open(name); 1314 composeTestCasesSuiteResourceComponentProperties(element); 1315 close(); 1316 } 1317 } 1318 1319 protected void composeTestCasesSuiteResourceComponentProperties(TestCases.TestCasesSuiteResourceComponent element) throws IOException { 1320 composeBaseProperties(element); 1321 if (element.hasNameElement()) { 1322 composeStringCore("name", element.getNameElement(), false); 1323 composeStringExtras("name", element.getNameElement(), false); 1324 } 1325 if (element.hasFileElement()) { 1326 composeStringCore("file", element.getFileElement(), false); 1327 composeStringExtras("file", element.getFileElement(), false); 1328 } 1329 if (element.hasResource()) { 1330 open("resource"); 1331 composeResource(element.getResource()); 1332 close(); 1333 } 1334 if (element.hasModeElement()) { 1335 composeCodeCore("mode", element.getModeElement(), false); 1336 composeCodeExtras("mode", element.getModeElement(), false); 1337 } 1338 } 1339 1340 protected void composeTestCasesSuiteParameterComponent(String name, TestCases.TestCasesSuiteParameterComponent element) throws IOException { 1341 if (element != null) { 1342 open(name); 1343 composeTestCasesSuiteParameterComponentProperties(element); 1344 close(); 1345 } 1346 } 1347 1348 protected void composeTestCasesSuiteParameterComponentProperties(TestCases.TestCasesSuiteParameterComponent element) throws IOException { 1349 composeBaseProperties(element); 1350 if (element.hasNameElement()) { 1351 composeStringCore("name", element.getNameElement(), false); 1352 composeStringExtras("name", element.getNameElement(), false); 1353 } 1354 if (element.hasValue()) { 1355 composeType("value", element.getValue()); 1356 } 1357 if (element.hasModeElement()) { 1358 composeCodeCore("mode", element.getModeElement(), false); 1359 composeCodeExtras("mode", element.getModeElement(), false); 1360 } 1361 } 1362 1363 protected void composeTestCasesSuiteTestComponent(String name, TestCases.TestCasesSuiteTestComponent element) throws IOException { 1364 if (element != null) { 1365 open(name); 1366 composeTestCasesSuiteTestComponentProperties(element); 1367 close(); 1368 } 1369 } 1370 1371 protected void composeTestCasesSuiteTestComponentProperties(TestCases.TestCasesSuiteTestComponent element) throws IOException { 1372 composeBaseProperties(element); 1373 if (element.hasNameElement()) { 1374 composeStringCore("name", element.getNameElement(), false); 1375 composeStringExtras("name", element.getNameElement(), false); 1376 } 1377 if (element.hasDescriptionElement()) { 1378 composeStringCore("description", element.getDescriptionElement(), false); 1379 composeStringExtras("description", element.getDescriptionElement(), false); 1380 } 1381 if (element.hasOperationElement()) { 1382 composeCodeCore("operation", element.getOperationElement(), false); 1383 composeCodeExtras("operation", element.getOperationElement(), false); 1384 } 1385 if (element.hasModeElement()) { 1386 composeStringCore("mode", element.getModeElement(), false); 1387 composeStringExtras("mode", element.getModeElement(), false); 1388 } 1389 if (element.hasParameter()) { 1390 openArray("parameter"); 1391 for (TestCases.TestCasesSuiteParameterComponent e : element.getParameter()) 1392 composeTestCasesSuiteParameterComponent(null, e); 1393 closeArray(); 1394 }; 1395 if (element.hasInput()) { 1396 openArray("input"); 1397 for (TestCases.TestCasesSuiteResourceComponent e : element.getInput()) 1398 composeTestCasesSuiteResourceComponent(null, e); 1399 closeArray(); 1400 }; 1401 if (element.hasOutput()) { 1402 openArray("output"); 1403 for (TestCases.TestCasesSuiteResourceComponent e : element.getOutput()) 1404 composeTestCasesSuiteResourceComponent(null, e); 1405 closeArray(); 1406 }; 1407 } 1408 1409 1410 1411 @Override 1412 protected void composeResource(Resource resource) throws IOException { 1413 if (resource == null) { 1414 throw new Error("Unhandled resource type "+resource.getClass().getName()); 1415 } else if (resource instanceof TestCases) { 1416 composeTestCases("TestCases", (TestCases)resource); 1417 1418 } else 1419 throw new Error("Unhandled resource type "+resource.getClass().getName()); 1420 } 1421 1422}