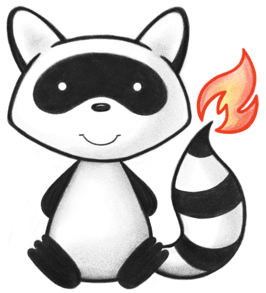
001package org.hl7.fhir.r5.utils; 002 003import org.hl7.fhir.r5.context.IWorkerContext; 004import org.hl7.fhir.r5.model.Base; 005 006import java.util.List; 007 008/** 009 @deprecated This interface only exists to provide backward compatibility for the following two projects: 010 <a href="https://github.com/cqframework/clinical-reasoning">clinical-reasoning</a> 011 <a href="https://github.com/cqframework/clinical_quality_language/">clinical_quality-language</a> 012 013 Due to a circular dependency, they cannot be updated without a release of HAPI, which requires backwards 014 compatibility with core version 6.1.2.2 015 **/ 016@Deprecated 017public class FHIRPathEngine extends org.hl7.fhir.r5.fhirpath.FHIRPathEngine { 018 019 public interface IEvaluationContext extends org.hl7.fhir.r5.fhirpath.FHIRPathEngine.IEvaluationContext{ } 020 public FHIRPathEngine(IWorkerContext worker) { 021 super(worker); 022 } 023 024 public org.hl7.fhir.r5.model.ExpressionNode parse(String string) { 025 return new org.hl7.fhir.r5.model.ExpressionNode(super.parse(string)); 026 } 027 028 public List<Base> evaluate(Base base, org.hl7.fhir.r5.model.ExpressionNode expressionNode) { 029 return super.evaluate(base, expressionNode); 030 } 031}