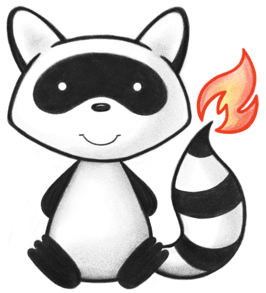
001package org.hl7.fhir.r5.utils; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r5.model.Base; 008import org.hl7.fhir.r5.model.Element; 009import org.hl7.fhir.r5.model.ElementDefinition; 010import org.hl7.fhir.r5.model.Property; 011import org.hl7.fhir.r5.model.Resource; 012import org.hl7.fhir.r5.model.StringType; 013import org.hl7.fhir.r5.model.StructureDefinition; 014import org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent; 015import org.hl7.fhir.utilities.Utilities; 016 017public class FHIRPathUtilityClasses { 018 019 public static class FHIRConstant extends Base { 020 021 private static final long serialVersionUID = -8933773658248269439L; 022 private String value; 023 024 public FHIRConstant(String value) { 025 this.value = value; 026 } 027 028 @Override 029 public String fhirType() { 030 return "%constant"; 031 } 032 033 @Override 034 protected void listChildren(List<Property> result) { 035 } 036 037 @Override 038 public String getIdBase() { 039 return null; 040 } 041 042 @Override 043 public void setIdBase(String value) { 044 } 045 046 public String getValue() { 047 return value; 048 } 049 050 @Override 051 public String primitiveValue() { 052 return value; 053 } 054 055 @Override 056 public Base copy() { 057 throw new Error("Not Implemented"); 058 } 059 } 060 061 public static class ClassTypeInfo extends Base { 062 private static final long serialVersionUID = 4909223114071029317L; 063 private Base instance; 064 065 public ClassTypeInfo(Base instance) { 066 super(); 067 this.instance = instance; 068 } 069 070 @Override 071 public String fhirType() { 072 return "ClassInfo"; 073 } 074 075 @Override 076 protected void listChildren(List<Property> result) { 077 } 078 079 @Override 080 public String getIdBase() { 081 return null; 082 } 083 084 @Override 085 public void setIdBase(String value) { 086 } 087 088 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 089 if (name.equals("name")) { 090 return new Base[]{new StringType(getName())}; 091 } else if (name.equals("namespace")) { 092 return new Base[]{new StringType(getNamespace())}; 093 } else { 094 return super.getProperty(hash, name, checkValid); 095 } 096 } 097 098 private String getNamespace() { 099 if ((instance instanceof Resource)) { 100 return "FHIR"; 101 } else if (!(instance instanceof Element) || ((Element)instance).isDisallowExtensions()) { 102 return "System"; 103 } else { 104 return "FHIR"; 105 } 106 } 107 108 private String getName() { 109 if ((instance instanceof Resource)) { 110 return instance.fhirType(); 111 } else if (!(instance instanceof Element) || ((Element)instance).isDisallowExtensions()) { 112 return Utilities.capitalize(instance.fhirType()); 113 } else { 114 return instance.fhirType(); 115 } 116 } 117 118 @Override 119 public Base copy() { 120 throw new Error("Not Implemented"); 121 } 122 } 123 124 public static class TypedElementDefinition { 125 private ElementDefinition element; 126 private String type; 127 private StructureDefinition src; 128 public TypedElementDefinition(StructureDefinition src, ElementDefinition element, String type) { 129 super(); 130 this.element = element; 131 this.type = type; 132 this.src = src; 133 } 134 public TypedElementDefinition(ElementDefinition element) { 135 super(); 136 this.element = element; 137 } 138 public ElementDefinition getElement() { 139 return element; 140 } 141 public String getType() { 142 return type; 143 } 144 public List<TypeRefComponent> getTypes() { 145 List<TypeRefComponent> res = new ArrayList<ElementDefinition.TypeRefComponent>(); 146 for (TypeRefComponent tr : element.getType()) { 147 if (type == null || type.equals(tr.getCode())) { 148 res.add(tr); 149 } 150 } 151 return res; 152 } 153 public boolean hasType(String tn) { 154 if (type != null) { 155 return tn.equals(type); 156 } else { 157 for (TypeRefComponent t : element.getType()) { 158 if (tn.equals(t.getCode())) { 159 return true; 160 } 161 } 162 return false; 163 } 164 } 165 public StructureDefinition getSrc() { 166 return src; 167 } 168 } 169 170 171 public static class FunctionDetails { 172 private String description; 173 private int minParameters; 174 private int maxParameters; 175 public FunctionDetails(String description, int minParameters, int maxParameters) { 176 super(); 177 this.description = description; 178 this.minParameters = minParameters; 179 this.maxParameters = maxParameters; 180 } 181 public String getDescription() { 182 return description; 183 } 184 public int getMinParameters() { 185 return minParameters; 186 } 187 public int getMaxParameters() { 188 return maxParameters; 189 } 190 191 } 192}