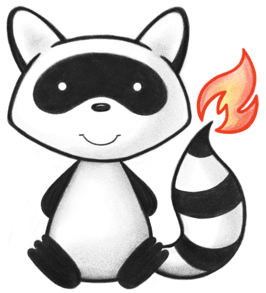
001package org.hl7.fhir.r5.utils; 002 003import org.hl7.fhir.exceptions.FHIRException; 004import org.hl7.fhir.r5.model.Configuration; 005import org.hl7.fhir.r5.model.EnumFactory; 006import org.hl7.fhir.r5.model.Enumeration; 007import org.hl7.fhir.r5.model.PrimitiveType; 008import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 009 010@MarkedToMoveToAdjunctPackage 011public enum GuideParameterCode { 012 /** 013 * If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext. 014 */ 015 APPLY, 016 /** 017 * The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type. 018 */ 019 PATHRESOURCE, 020 /** 021 * The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder. 022 */ 023 PATHPAGES, 024 /** 025 * The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control. 026 */ 027 PATHTXCACHE, 028 /** 029 * The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT. 030 */ 031 EXPANSIONPARAMETER, 032 /** 033 * The value of this string 0..1 parameter is either "warning" or "error" (default = "error"). If the value is "warning" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links. 034 */ 035 RULEBROKENLINKS, 036 /** 037 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML. 038 */ 039 GENERATEXML, 040 /** 041 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON. 042 */ 043 GENERATEJSON, 044 /** 045 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle. 046 */ 047 GENERATETURTLE, 048 /** 049 * The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating). 050 */ 051 HTMLTEMPLATE, 052 /** 053 * added to help the parsers with the generic types 054 */ 055 NULL; 056 public static GuideParameterCode fromCode(String codeString) throws FHIRException { 057 if (codeString == null || "".equals(codeString)) 058 return null; 059 if ("apply".equals(codeString)) 060 return APPLY; 061 if ("path-resource".equals(codeString)) 062 return PATHRESOURCE; 063 if ("path-pages".equals(codeString)) 064 return PATHPAGES; 065 if ("path-tx-cache".equals(codeString)) 066 return PATHTXCACHE; 067 if ("expansion-parameter".equals(codeString)) 068 return EXPANSIONPARAMETER; 069 if ("rule-broken-links".equals(codeString)) 070 return RULEBROKENLINKS; 071 if ("generate-xml".equals(codeString)) 072 return GENERATEXML; 073 if ("generate-json".equals(codeString)) 074 return GENERATEJSON; 075 if ("generate-turtle".equals(codeString)) 076 return GENERATETURTLE; 077 if ("html-template".equals(codeString)) 078 return HTMLTEMPLATE; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown GuideParameterCode code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case APPLY: return "apply"; 087 case PATHRESOURCE: return "path-resource"; 088 case PATHPAGES: return "path-pages"; 089 case PATHTXCACHE: return "path-tx-cache"; 090 case EXPANSIONPARAMETER: return "expansion-parameter"; 091 case RULEBROKENLINKS: return "rule-broken-links"; 092 case GENERATEXML: return "generate-xml"; 093 case GENERATEJSON: return "generate-json"; 094 case GENERATETURTLE: return "generate-turtle"; 095 case HTMLTEMPLATE: return "html-template"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case APPLY: return "http://hl7.org/fhir/guide-parameter-code"; 103 case PATHRESOURCE: return "http://hl7.org/fhir/guide-parameter-code"; 104 case PATHPAGES: return "http://hl7.org/fhir/guide-parameter-code"; 105 case PATHTXCACHE: return "http://hl7.org/fhir/guide-parameter-code"; 106 case EXPANSIONPARAMETER: return "http://hl7.org/fhir/guide-parameter-code"; 107 case RULEBROKENLINKS: return "http://hl7.org/fhir/guide-parameter-code"; 108 case GENERATEXML: return "http://hl7.org/fhir/guide-parameter-code"; 109 case GENERATEJSON: return "http://hl7.org/fhir/guide-parameter-code"; 110 case GENERATETURTLE: return "http://hl7.org/fhir/guide-parameter-code"; 111 case HTMLTEMPLATE: return "http://hl7.org/fhir/guide-parameter-code"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDefinition() { 117 switch (this) { 118 case APPLY: return "If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext."; 119 case PATHRESOURCE: return "The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type."; 120 case PATHPAGES: return "The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder."; 121 case PATHTXCACHE: return "The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control."; 122 case EXPANSIONPARAMETER: return "The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT."; 123 case RULEBROKENLINKS: return "The value of this string 0..1 parameter is either \"warning\" or \"error\" (default = \"error\"). If the value is \"warning\" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links."; 124 case GENERATEXML: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML."; 125 case GENERATEJSON: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON."; 126 case GENERATETURTLE: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle."; 127 case HTMLTEMPLATE: return "The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating)."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case APPLY: return "Apply Metadata Value"; 135 case PATHRESOURCE: return "Resource Path"; 136 case PATHPAGES: return "Pages Path"; 137 case PATHTXCACHE: return "Terminology Cache Path"; 138 case EXPANSIONPARAMETER: return "Expansion Profile"; 139 case RULEBROKENLINKS: return "Broken Links Rule"; 140 case GENERATEXML: return "Generate XML"; 141 case GENERATEJSON: return "Generate JSON"; 142 case GENERATETURTLE: return "Generate Turtle"; 143 case HTMLTEMPLATE: return "HTML Template"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public class GuideParameterCodeEnumFactory implements EnumFactory<GuideParameterCode> { 149 public GuideParameterCode fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("apply".equals(codeString)) 154 return GuideParameterCode.APPLY; 155 if ("path-resource".equals(codeString)) 156 return GuideParameterCode.PATHRESOURCE; 157 if ("path-pages".equals(codeString)) 158 return GuideParameterCode.PATHPAGES; 159 if ("path-tx-cache".equals(codeString)) 160 return GuideParameterCode.PATHTXCACHE; 161 if ("expansion-parameter".equals(codeString)) 162 return GuideParameterCode.EXPANSIONPARAMETER; 163 if ("rule-broken-links".equals(codeString)) 164 return GuideParameterCode.RULEBROKENLINKS; 165 if ("generate-xml".equals(codeString)) 166 return GuideParameterCode.GENERATEXML; 167 if ("generate-json".equals(codeString)) 168 return GuideParameterCode.GENERATEJSON; 169 if ("generate-turtle".equals(codeString)) 170 return GuideParameterCode.GENERATETURTLE; 171 if ("html-template".equals(codeString)) 172 return GuideParameterCode.HTMLTEMPLATE; 173 throw new IllegalArgumentException("Unknown GuideParameterCode code '"+codeString+"'"); 174 } 175 public Enumeration<GuideParameterCode> fromType(PrimitiveType<?> code) throws FHIRException { 176 if (code == null) 177 return null; 178 if (code.isEmpty()) 179 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 180 String codeString = ((PrimitiveType) code).asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 183 if ("apply".equals(codeString)) 184 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.APPLY, code); 185 if ("path-resource".equals(codeString)) 186 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHRESOURCE, code); 187 if ("path-pages".equals(codeString)) 188 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHPAGES, code); 189 if ("path-tx-cache".equals(codeString)) 190 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHTXCACHE, code); 191 if ("expansion-parameter".equals(codeString)) 192 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.EXPANSIONPARAMETER, code); 193 if ("rule-broken-links".equals(codeString)) 194 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.RULEBROKENLINKS, code); 195 if ("generate-xml".equals(codeString)) 196 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEXML, code); 197 if ("generate-json".equals(codeString)) 198 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEJSON, code); 199 if ("generate-turtle".equals(codeString)) 200 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATETURTLE, code); 201 if ("html-template".equals(codeString)) 202 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.HTMLTEMPLATE, code); 203 throw new FHIRException("Unknown GuideParameterCode code '"+codeString+"'"); 204 } 205 public String toCode(GuideParameterCode code) { 206 if (code == GuideParameterCode.NULL) 207 return null; 208 if (code == GuideParameterCode.APPLY) 209 return "apply"; 210 if (code == GuideParameterCode.PATHRESOURCE) 211 return "path-resource"; 212 if (code == GuideParameterCode.PATHPAGES) 213 return "path-pages"; 214 if (code == GuideParameterCode.PATHTXCACHE) 215 return "path-tx-cache"; 216 if (code == GuideParameterCode.EXPANSIONPARAMETER) 217 return "expansion-parameter"; 218 if (code == GuideParameterCode.RULEBROKENLINKS) 219 return "rule-broken-links"; 220 if (code == GuideParameterCode.GENERATEXML) 221 return "generate-xml"; 222 if (code == GuideParameterCode.GENERATEJSON) 223 return "generate-json"; 224 if (code == GuideParameterCode.GENERATETURTLE) 225 return "generate-turtle"; 226 if (code == GuideParameterCode.HTMLTEMPLATE) 227 return "html-template"; 228 return "?"; 229 } 230 public String toSystem(GuideParameterCode code) { 231 return code.getSystem(); 232 } 233 } 234}