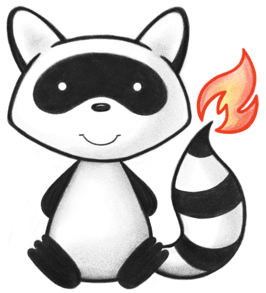
001package org.hl7.fhir.r5.utils; 002 003import org.hl7.fhir.exceptions.FHIRException; 004import org.hl7.fhir.r5.model.Configuration; 005import org.hl7.fhir.r5.model.EnumFactory; 006import org.hl7.fhir.r5.model.Enumeration; 007import org.hl7.fhir.r5.model.PrimitiveType; 008 009public enum GuideParameterCode { 010 /** 011 * If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext. 012 */ 013 APPLY, 014 /** 015 * The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type. 016 */ 017 PATHRESOURCE, 018 /** 019 * The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder. 020 */ 021 PATHPAGES, 022 /** 023 * The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control. 024 */ 025 PATHTXCACHE, 026 /** 027 * The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT. 028 */ 029 EXPANSIONPARAMETER, 030 /** 031 * The value of this string 0..1 parameter is either "warning" or "error" (default = "error"). If the value is "warning" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links. 032 */ 033 RULEBROKENLINKS, 034 /** 035 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML. 036 */ 037 GENERATEXML, 038 /** 039 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON. 040 */ 041 GENERATEJSON, 042 /** 043 * The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle. 044 */ 045 GENERATETURTLE, 046 /** 047 * The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating). 048 */ 049 HTMLTEMPLATE, 050 /** 051 * added to help the parsers with the generic types 052 */ 053 NULL; 054 public static GuideParameterCode fromCode(String codeString) throws FHIRException { 055 if (codeString == null || "".equals(codeString)) 056 return null; 057 if ("apply".equals(codeString)) 058 return APPLY; 059 if ("path-resource".equals(codeString)) 060 return PATHRESOURCE; 061 if ("path-pages".equals(codeString)) 062 return PATHPAGES; 063 if ("path-tx-cache".equals(codeString)) 064 return PATHTXCACHE; 065 if ("expansion-parameter".equals(codeString)) 066 return EXPANSIONPARAMETER; 067 if ("rule-broken-links".equals(codeString)) 068 return RULEBROKENLINKS; 069 if ("generate-xml".equals(codeString)) 070 return GENERATEXML; 071 if ("generate-json".equals(codeString)) 072 return GENERATEJSON; 073 if ("generate-turtle".equals(codeString)) 074 return GENERATETURTLE; 075 if ("html-template".equals(codeString)) 076 return HTMLTEMPLATE; 077 if (Configuration.isAcceptInvalidEnums()) 078 return null; 079 else 080 throw new FHIRException("Unknown GuideParameterCode code '"+codeString+"'"); 081 } 082 public String toCode() { 083 switch (this) { 084 case APPLY: return "apply"; 085 case PATHRESOURCE: return "path-resource"; 086 case PATHPAGES: return "path-pages"; 087 case PATHTXCACHE: return "path-tx-cache"; 088 case EXPANSIONPARAMETER: return "expansion-parameter"; 089 case RULEBROKENLINKS: return "rule-broken-links"; 090 case GENERATEXML: return "generate-xml"; 091 case GENERATEJSON: return "generate-json"; 092 case GENERATETURTLE: return "generate-turtle"; 093 case HTMLTEMPLATE: return "html-template"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case APPLY: return "http://hl7.org/fhir/guide-parameter-code"; 101 case PATHRESOURCE: return "http://hl7.org/fhir/guide-parameter-code"; 102 case PATHPAGES: return "http://hl7.org/fhir/guide-parameter-code"; 103 case PATHTXCACHE: return "http://hl7.org/fhir/guide-parameter-code"; 104 case EXPANSIONPARAMETER: return "http://hl7.org/fhir/guide-parameter-code"; 105 case RULEBROKENLINKS: return "http://hl7.org/fhir/guide-parameter-code"; 106 case GENERATEXML: return "http://hl7.org/fhir/guide-parameter-code"; 107 case GENERATEJSON: return "http://hl7.org/fhir/guide-parameter-code"; 108 case GENERATETURTLE: return "http://hl7.org/fhir/guide-parameter-code"; 109 case HTMLTEMPLATE: return "http://hl7.org/fhir/guide-parameter-code"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case APPLY: return "If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext."; 117 case PATHRESOURCE: return "The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type."; 118 case PATHPAGES: return "The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder."; 119 case PATHTXCACHE: return "The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control."; 120 case EXPANSIONPARAMETER: return "The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT."; 121 case RULEBROKENLINKS: return "The value of this string 0..1 parameter is either \"warning\" or \"error\" (default = \"error\"). If the value is \"warning\" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links."; 122 case GENERATEXML: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML."; 123 case GENERATEJSON: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON."; 124 case GENERATETURTLE: return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle."; 125 case HTMLTEMPLATE: return "The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating)."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case APPLY: return "Apply Metadata Value"; 133 case PATHRESOURCE: return "Resource Path"; 134 case PATHPAGES: return "Pages Path"; 135 case PATHTXCACHE: return "Terminology Cache Path"; 136 case EXPANSIONPARAMETER: return "Expansion Profile"; 137 case RULEBROKENLINKS: return "Broken Links Rule"; 138 case GENERATEXML: return "Generate XML"; 139 case GENERATEJSON: return "Generate JSON"; 140 case GENERATETURTLE: return "Generate Turtle"; 141 case HTMLTEMPLATE: return "HTML Template"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public class GuideParameterCodeEnumFactory implements EnumFactory<GuideParameterCode> { 147 public GuideParameterCode fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("apply".equals(codeString)) 152 return GuideParameterCode.APPLY; 153 if ("path-resource".equals(codeString)) 154 return GuideParameterCode.PATHRESOURCE; 155 if ("path-pages".equals(codeString)) 156 return GuideParameterCode.PATHPAGES; 157 if ("path-tx-cache".equals(codeString)) 158 return GuideParameterCode.PATHTXCACHE; 159 if ("expansion-parameter".equals(codeString)) 160 return GuideParameterCode.EXPANSIONPARAMETER; 161 if ("rule-broken-links".equals(codeString)) 162 return GuideParameterCode.RULEBROKENLINKS; 163 if ("generate-xml".equals(codeString)) 164 return GuideParameterCode.GENERATEXML; 165 if ("generate-json".equals(codeString)) 166 return GuideParameterCode.GENERATEJSON; 167 if ("generate-turtle".equals(codeString)) 168 return GuideParameterCode.GENERATETURTLE; 169 if ("html-template".equals(codeString)) 170 return GuideParameterCode.HTMLTEMPLATE; 171 throw new IllegalArgumentException("Unknown GuideParameterCode code '"+codeString+"'"); 172 } 173 public Enumeration<GuideParameterCode> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 178 String codeString = ((PrimitiveType) code).asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 181 if ("apply".equals(codeString)) 182 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.APPLY, code); 183 if ("path-resource".equals(codeString)) 184 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHRESOURCE, code); 185 if ("path-pages".equals(codeString)) 186 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHPAGES, code); 187 if ("path-tx-cache".equals(codeString)) 188 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHTXCACHE, code); 189 if ("expansion-parameter".equals(codeString)) 190 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.EXPANSIONPARAMETER, code); 191 if ("rule-broken-links".equals(codeString)) 192 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.RULEBROKENLINKS, code); 193 if ("generate-xml".equals(codeString)) 194 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEXML, code); 195 if ("generate-json".equals(codeString)) 196 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEJSON, code); 197 if ("generate-turtle".equals(codeString)) 198 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATETURTLE, code); 199 if ("html-template".equals(codeString)) 200 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.HTMLTEMPLATE, code); 201 throw new FHIRException("Unknown GuideParameterCode code '"+codeString+"'"); 202 } 203 public String toCode(GuideParameterCode code) { 204 if (code == GuideParameterCode.APPLY) 205 return "apply"; 206 if (code == GuideParameterCode.PATHRESOURCE) 207 return "path-resource"; 208 if (code == GuideParameterCode.PATHPAGES) 209 return "path-pages"; 210 if (code == GuideParameterCode.PATHTXCACHE) 211 return "path-tx-cache"; 212 if (code == GuideParameterCode.EXPANSIONPARAMETER) 213 return "expansion-parameter"; 214 if (code == GuideParameterCode.RULEBROKENLINKS) 215 return "rule-broken-links"; 216 if (code == GuideParameterCode.GENERATEXML) 217 return "generate-xml"; 218 if (code == GuideParameterCode.GENERATEJSON) 219 return "generate-json"; 220 if (code == GuideParameterCode.GENERATETURTLE) 221 return "generate-turtle"; 222 if (code == GuideParameterCode.HTMLTEMPLATE) 223 return "html-template"; 224 return "?"; 225 } 226 public String toSystem(GuideParameterCode code) { 227 return code.getSystem(); 228 } 229 } 230}